Java 实现FTP自动上传文件是一项常见的任务,尤其在系统集成或者数据备份的场景中。FTP(File Transfer Protocol)是一种用于在网络之间传输文件的标准协议。在Java中,我们可以借助第三方库如Apache Commons Net来轻松实现FTP的功能。 我们需要了解Apache Commons Net库。这个库提供了丰富的FTP相关类和方法,使得在Java程序中执行FTP操作变得简单。例如,`FTPClient`类是主要的FTP客户端接口,可以用来连接到FTP服务器、登录、上传和下载文件以及管理目录。 以下是一个简单的Java FTP文件上传示例: ```java import org.apache.commons.net.ftp.FTP; import org.apache.commons.net.ftp.FTPClient; import java.io.File; import java.io.FileInputStream; import java.io.IOException; public class FtpUploader { public static void main(String[] args) { FTPClient ftpClient = new FTPClient(); try { ftpClient.connect("ftp.example.com"); // 替换为实际的FTP服务器地址 if (ftpClient.login("username", "password")) { // 登录FTP服务器,替换为真实用户名和密码 ftpClient.enterLocalPassiveMode(); // 设置为主动模式 ftpClient.setFileType(FTP.BINARY_FILE_TYPE); // 设置文件传输类型为二进制 File directory = new File("/path/to/directory"); // 需要上传的目录 uploadDirectory(ftpClient, directory, "/remote/path"); // 服务器上的目标路径 ftpClient.logout(); ftpClient.disconnect(); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (ftpClient.isConnected()) { ftpClient.disconnect(); } } catch (IOException ioe) { ioe.printStackTrace(); } } } private static void uploadDirectory(FTPClient ftpClient, File dir, String remotePath) throws IOException { if (dir.isDirectory()) { for (File file : dir.listFiles()) { if (file.isDirectory()) { uploadDirectory(ftpClient, file, remotePath + "/" + file.getName()); } else { FileInputStream fis = new FileInputStream(file); ftpClient.storeFile(remotePath + "/" + file.getName(), fis); fis.close(); } } } } } ``` 在这个例子中,`uploadDirectory`方法递归地上传指定目录及其子目录下的所有文件。`FTPClient`的`storeFile`方法用于将本地文件上传到FTP服务器。注意,实际使用时需要处理异常并进行适当的错误处理。 关于`JTextArea`读取`Log4j`信息,`JTextArea`是Java Swing中的一个组件,用于显示和编辑多行文本。在上述FTP上传过程中,我们可以使用它来实时显示日志信息,以便于监控上传进度和状态。`Log4j`则是一个强大的日志框架,通过配置它可以记录不同级别的日志,如ERROR、WARN、INFO等。在Java代码中,我们可以创建一个Appender,将`Log4j`的日志输出到`JTextArea`: ```java import org.apache.log4j.Logger; import org.apache.log4j.PatternLayout; import org.apache.log4j.ConsoleAppender; import javax.swing.JScrollPane; import javax.swing.JTextArea; public class Log4jToJTextArea { private static final Logger logger = Logger.getLogger(Log4jToJTextArea.class); public static void init() { PatternLayout layout = new PatternLayout("%d{HH:mm:ss} %-5p %c{1}:%L - %m%n"); ConsoleAppender appender = new ConsoleAppender(layout, "JTextAreaAppender"); appender.activateOptions(); logger.addAppender(appender); } public static void logInfo(String message) { logger.info(message); } } // 在Swing GUI中使用 JTextArea textArea = new JTextArea(); textArea.setEditable(false); JScrollPane scrollPane = new JScrollPane(textArea); yourMainFrame.add(scrollPane, BorderLayout.CENTER); Log4jToJTextArea.init(); Log4jToJTextArea.logInfo("FTP上传开始..."); ``` 这样,`Log4j`的日志就会显示在`JTextArea`中,用户可以通过GUI查看上传过程中的详细信息。 至于命令行信息,虽然描述中提到,但Java程序通常不直接处理命令行信息,而是通过控制台输出或者日志记录来呈现。如果需要在命令行界面显示FTP上传的进度,可以考虑在`logInfo`方法中添加输出,并在命令行运行Java程序时查看输出。 由于没有提供具体的压缩包文件内容,我们无法深入讨论其细节。但是,从文件名`a4be9caf9a3d4aeeab6a6ebb6059ba11`来看,这可能是一个哈希值或随机生成的ID,不直接对应于源代码或资源文件。如果这是一个源代码文件,那么它可能包含了上述Java FTP上传功能的实现。为了进一步分析,我们需要实际的文件内容。
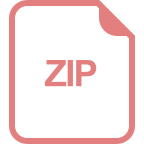
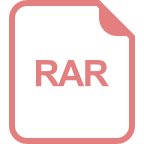
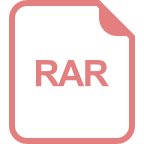
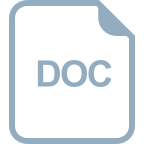
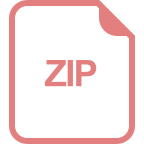
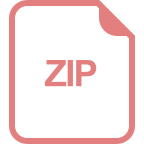
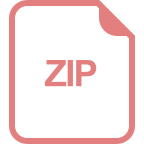
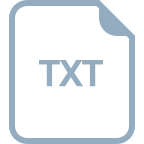
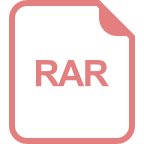
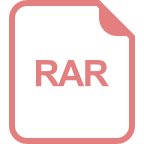
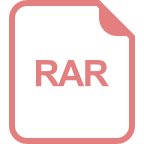
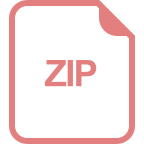
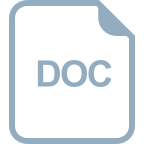
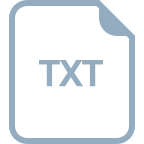
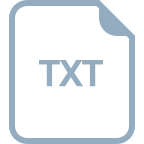
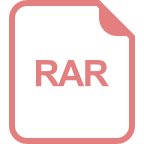
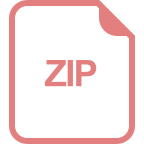


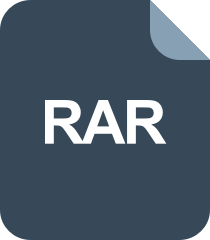
- 1
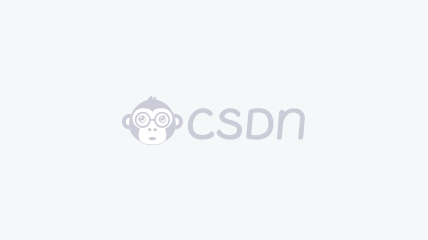

- 粉丝: 8
- 资源: 151
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

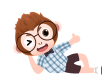
最新资源

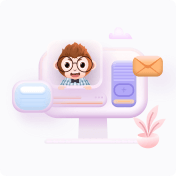
