package a;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.Calendar;
import java.util.Hashtable;
public class Rili extends JFrame implements ActionListener, MouseListener {
int year, month, day;
Label label;//定义一个label标签
NotePad notepad;//定义一个类的对象
File file;//文件和目录路径名的抽象表示形式
JTextField showDay[];//定义一个单行文本数组
JLabel title[]; // 星期字段组标签
JButton button1, button2, button3, button4;
JTextField showYear = null;//定义一个文本框
JTextField showMonth = null;
// 定义字符串类型(星期)数组
String 星期[] = {"星期日", "星期一", "星期二", "星期三", "星期四", "星期五", "星期六"};
Calendar calendar; // 日历
int week;// 星期几
JPanel leftPanel, rightPanel;//定义两容器
//构造方法
public Rili(int year, int month, int day) {
super("日历");
this.setSize(600, 300);
// 左容器
//实例化对象
leftPanel = new JPanel();
JPanel leftCenter = new JPanel();
JPanel leftNorth = new JPanel();
// 使用网格布局方式
GridLayout myLayout = new GridLayout(7, 7);
leftCenter.setLayout(myLayout);// 把网格布局放入左容器中
button1 = new JButton("上年");
button2 = new JButton("下年");
button3 = new JButton("上月");
button4 = new JButton("下月");
showYear = new JTextField(4);
// 添加事件
showYear.addActionListener(this);
showYear.setText("" + year);// 向文本内添入数据
// showYear.setEditable(true);// 可修改文本年
showYear.setForeground(Color.black);
showMonth = new JTextField(2);
// 添加事件
showMonth.addActionListener(this);
showMonth.setText("" + month);
// showMonth.setEditable(true);// 可修改文本月
showMonth.setForeground(Color.black);
// 每个按钮都要添加监听事件
button1.addActionListener(this);
button2.addActionListener(this);
button3.addActionListener(this);
button4.addActionListener(this);
title = new JLabel[7];
showDay = new JTextField[42];//生成实例
//把星期字段添加到标签
for (int i = 0; i < 7; i++) {
title[i] = new JLabel();
title[i].setText(星期[i]);
title[i].setBorder(BorderFactory.createRaisedBevelBorder());//标签外表类型为凸
//添加到容器
leftCenter.add(title[i]);
}
title[0].setForeground(Color.red);
title[6].setForeground(Color.red);
for (int j = 0; j < 42; j++) {
showDay[j] = new JTextField();
leftCenter.add(showDay[j]);
}
for (int i = 0; i < 42; i++) {
showDay[i].addMouseListener(this);
}
calendar = Calendar.getInstance();
// 创建一个从左到右显示其组件的 Box
Box box = new Box(BoxLayout.X_AXIS);
//添加box容器
box.add(button1);
box.add(showYear);
box.add(button2);
box.add(button3);
box.add(showMonth);
box.add(button4);
leftNorth.add(box);
leftPanel.setLayout(new BorderLayout());
label = new Label("请在年份输入框输入所查年份(负数表示公元前),并回车确定");
leftPanel.add(label, BorderLayout.SOUTH);
leftPanel.add(leftCenter, BorderLayout.CENTER);
leftPanel.add(leftNorth, BorderLayout.NORTH);
leftPanel.validate();
rightPanel = new JPanel();
this.year = year;
this.month = month;
this.day = day;
JSplitPane split = new JSplitPane(JSplitPane.HORIZONTAL_SPLIT,
leftPanel, rightPanel);
split.setDividerLocation(350);// 设置JSplitPane对象splitPane1的分隔线位置
this.add(split);
rili(year, month);
this.setVisible(true);
this.setResizable(false);
// 文件
//从文件中读内容
file = new File("日历记事本.txt");
Hashtable hashtable = new Hashtable();
if (!file.exists()) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(file));
out.writeObject(hashtable);
out.close();
} catch (IOException e) {
}
}
// 右容器
notepad = new NotePad(this);
rightPanel.add(notepad);
notepad.top(year, month, day);
}
// 年
public void setshowYear(int year) {
this.year = year;
showYear.setText("" + year);
}
public int getshowYear() {
return year;
}
//月
public void setshowMonth(int month) {
if (month <= 12 && month >= 1) {
this.month = month;
} else {
this.month = 1;
}
showMonth.setText("" + month);
}
public int getshowMonth() {
return month;
}
// 设置上年、下年、上月、下月四个按钮
public void actionPerformed(ActionEvent e) {
//判断上年、下年
if (e.getSource() == button1) {
year = year - 1;
showYear.setText("" + year);
setshowYear(year);
rili(year, getshowMonth());
notepad.top(year, month, day);
}
if (e.getSource() == button2) {
year = year + 1;
showYear.setText("" + year);
setshowYear(year);
rili(year, getshowMonth());
notepad.top(year, month, day);
}
//判断上月、下月
if (e.getSource() == button3) {
if (month >= 2) {
month = month - 1;
setshowMonth(month);
rili(getshowYear(), month);
notepad.top(year, month, day);
} else if (month == 1) {
month = 12;
setshowMonth(month);
rili(getshowYear(), month);
notepad.top(year, month, day);
}
showMonth.setText("" + month);
} else if (e.getSource() == button4) {
if (month < 12) {
month = month + 1;
setshowMonth(month);
rili(getshowYear(), month);
} else if (month == 12) {
month = 1;
setshowMonth(month);
rili(getshowYear(), month);
}
showMonth.setText("" + month);
}
if (e.getSource() == showYear) {
try {
year = Integer.valueOf(showYear.getText());
} catch (Exception ee) {
} finally {
rili(year, month);
showYear.setText(String.valueOf(year));
}
}
if (e.getSource() == showMonth) {
try {
month = Integer.valueOf(showMonth.getText());
} catch (Exception a) {
} finally {
rili(year, month);
showMonth.setText(String.valueOf(month));
}
}
}
// 编排号码
public void number(int week, int mDay) {
for (int i = week, n = 1; i < week + mDay; i++) {
showDay[i].setText("" + n);
showDay[i].setEditable(false);
if (n == day) {
showDay[i].setForeground(Color.red);
showDay[i].setFont(new Font("TimesRoman", Font.BOLD, 22));
} else {
showDay[i].setForeground(Color.black);
showDay[i].setFont(new Font("TimesRoman", Font.BOLD, 12));
}
if (i % 7 == 0) {
showDay[i].setBackground(Color.white);
}
if (i % 7 == 6) {
showDay[i].setBackground(Color.white);
}
n++;
}
for (int i = 0; i < week; i++) {

勇敢爱
- 粉丝: 34
- 资源: 150
最新资源
- 基于微信平台的ssm高校毕业论文管理系统小程序(源码 + 数据库+LW+PPT)
- (25152814)VMware相关服务一键启动/关闭.bat
- 机器学习(预测模型):英特尔公司历史股票数据的数据集
- (29953412)个人博客微信QQ小程序源码包.7z
- Java毕设项目:基于spring+mybatis+maven+mysql实现的化妆品配方及工艺管理系统【含源码+数据库+开题报告+任务书+毕业论文】
- (5175244)在Microsoft Visual C++ 6.0环境下通过对Active X控件的编程来实现串口的通信的一般方
- pyinstaller -onefile -add-data "C:\\liteon\\HRZhaoMu\\SmartEsop\\whisper\\assets\\mel-filters.npz
- CFA知识点梳理系列:CFA Level II, Reading 7 Economics of Regulation
- (5857632)串口调试助手 串口调试
- (59423620)指纹识别基于matlab GUI指纹识别【含Matlab源码 1353期】.zip
- 2024最强Java面试八股文-最新面试题
- (6755822)基于TCP的VC++聊天室
- (8424006)动态网页设计
- (13391206)基于51单片机的计算器
- (172705856)软件工程导论(第六版)课后习题答案1
- (174525210)机器学习期末复习题选择题库
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


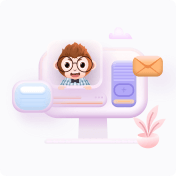
- 1
- 2
- 3
- 4
前往页