package com.example.myapplication;
import android.animation.AnimatorSet;
import android.animation.ObjectAnimator;
import android.app.AlertDialog;
import android.content.Context;
import android.graphics.Color;
import android.graphics.drawable.ColorDrawable;
import android.util.AttributeSet;
import android.util.Log;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.widget.FrameLayout;
import android.widget.TextView;
import android.widget.Toast;
public class GameView extends FrameLayout {
private static final String TAG = "GameView";
private int widthSize; //viewgroup大小
private int heightSize;
private int marginWidth;
private int viewWidth=200; //实际图片宽高。
private int viewHeight=100;
private int itemsWidth;
private int paddingWidth;
private int[][] array=new int[][]{
new int[]{0,0,1},
new int[]{0,0,2},
new int[]{0,0,3}
};
private float mLastX;
private float mLastY;
private TextView textView1;
private TextView textView2;
private TextView textView3;
private View currentView;
private int currentCloumn;
private int currentCloumn_UP;
public GameView(Context context) {
this(context,null);
}
public GameView(Context context, AttributeSet attrs) {
this(context, attrs,-1);
}
public GameView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
private void initChild() {
textView1=new TextView(this.getContext());
textView2=new TextView(this.getContext());
textView3=new TextView(this.getContext());
textView1.setBackground(new ColorDrawable(Color.RED));
textView2.setBackground(new ColorDrawable(Color.YELLOW));
textView3.setBackground(new ColorDrawable(Color.GREEN));
viewWidth=widthSize/3;
viewHeight=heightSize/3;
textView1.setLayoutParams(new ViewGroup.LayoutParams(viewWidth,viewHeight));
textView2.setLayoutParams(new ViewGroup.LayoutParams(viewWidth,viewHeight));
textView3.setLayoutParams(new ViewGroup.LayoutParams(viewWidth,viewHeight));
textView1.setText("1");
textView2.setText("2");
textView3.setText("3");
textView1.setTextSize(30);
textView2.setTextSize(30);
textView3.setTextSize(30);
addView(textView1);
addView(textView2);
addView(textView3);
int childWidthMeasureSpec = MeasureSpec.makeMeasureSpec(
viewWidth, MeasureSpec.EXACTLY);
// int childHeightMeasureSpec = getChildMeasureSpec(heightMeasureSpec,
// mPaddingTop + mPaddingBottom + lp.topMargin + lp.bottomMargin,
// lp.height);;
int childHeightMeasureSpec = MeasureSpec.makeMeasureSpec(
viewHeight, MeasureSpec.EXACTLY);
textView1.measure(childWidthMeasureSpec,childHeightMeasureSpec);
textView2.measure(childWidthMeasureSpec,childHeightMeasureSpec);
textView3.measure(childWidthMeasureSpec,childHeightMeasureSpec);
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
widthSize = MeasureSpec.getSize(widthMeasureSpec);
heightSize = MeasureSpec.getSize(heightMeasureSpec);
Log.i(TAG,"widthSize="+widthSize+",heightSize="+heightSize);
initChild();
}
@Override
protected void onLayout(boolean changed, int left, int top, int right, int bottom) {
// super.onLayout(changed, left, top, right, bottom);
textView1.layout(0,2*viewHeight,viewWidth,3*viewHeight);
textView2.layout(viewWidth,2*viewHeight,2*viewWidth,3*viewHeight);
textView3.layout(2*viewWidth,2*viewHeight,3*viewWidth,3*viewHeight);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()){
case MotionEvent.ACTION_DOWN:
mLastX=event.getX();
mLastY=event.getY();
if(mLastX<viewWidth){
currentCloumn=0;
}else if(mLastX>viewWidth&mLastX<2*viewWidth){
currentCloumn=1;
}else if(mLastX>viewWidth*2){
currentCloumn=2;
}
break;
case MotionEvent.ACTION_MOVE:
// float x=event.getX();
// float y=event.getY();
//
//
break;
case MotionEvent.ACTION_UP:
float x_up=event.getX();
float y_up=event.getX();
if(x_up<viewWidth){
currentCloumn_UP=0;
}else if(x_up>viewWidth&x_up<2*viewWidth){
currentCloumn_UP=1;
}else if(x_up>viewWidth*2){
currentCloumn_UP=2;
}
arrangeView(x_up,y_up);
isFinish();
break;
}
return true;
}
private void arrangeView(float x, float y) {
if(currentCloumn_UP==currentCloumn){
return;
}
if(getNoneZoroSize(currentCloumn)==0){
return;
}
int lastValue=array[currentCloumn][3-getNoneZoroSize(currentCloumn)];
array[currentCloumn][3-getNoneZoroSize(currentCloumn)]=0;
for (int i=0;i<array.length;i++){
if(currentCloumn_UP==i){
array[currentCloumn_UP][2-getNoneZoroSize(currentCloumn_UP)]=lastValue;
}
}
for (int i=0;i<array.length;i++) {
for (int j=0;j<array[i].length;j++){
if(array[i][j]==1){
moveView(textView1,i*viewWidth,j*viewHeight);
}else if(array[i][j]==2){
moveView(textView2,i*viewWidth,j*viewHeight);
}else if(array[i][j]==3){
moveView(textView3,i*viewWidth,j*viewHeight);
}
}
}
for (int i=0;i<array.length;i++) {
for (int j = 0; j < array[i].length; j++) {
Log.i(TAG,"i:"+i+",j:"+j+",array="+array[i][j]);
}
}
}
void moveView(View view,int x,int y){
ObjectAnimator animX = ObjectAnimator.ofFloat(view, "x", x);
ObjectAnimator animY = ObjectAnimator.ofFloat(view, "y", y);
AnimatorSet animSetXY = new AnimatorSet();
animSetXY.playTogether(animX, animY);
animSetXY.start();
}
private int getNoneZoroSize(int currentCloumn) {
int size=0;
for (int i=0;i<array.length;i++) {
if(currentCloumn!=i)
continue;
for (int j=0;j<3;j++){
if(array[currentCloumn][j]!=0){
size++;
}
}
}
return size;
}
private View getCurrentView(float x, float y) {
if((x<textView1.getRight()&x>textView1.getLeft())&&(y<textView1.getBottom()&y>textView1.getTop())){
return textView1;
}else if((x<textView2.getRight()&x>textView2.getLeft())&&(y<textView2.getBottom()&y>textView2.getTop())){
return textView2;
}else if((x<textView3.getRight()&x>textView3.getLeft())&&(y<textView3.getBottom()&y>textView3.getTop())){
return textView3;
}
return null;
}
class Coordinate{
int x;
int y;
public Coordinate(int x, int y) {
this.x = x;
this.y = y;
}
}
public void isFinish(){
if(array[1][0]==1&array[1][1]==2&array[1][2]==3){
Toast.makeText(this.getContext(),"FINISH",Toast.LENGTH_LONG).show();
new AlertDialog.Builder(this.getContext())
.setMessage("恭喜你,过关了")
.show();
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该资源内项目源码是个人的课程设计、毕业设计,代码都测试ok,都是运行成功后才上传资源,答辩评审平均分达到96分,放心下载使用! ## 项目备注 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。 该资源内项目源码是个人的课程设计,代码都测试ok,都是运行成功后才上传资源,答辩评审平均分达到96分,放心下载使用! ## 项目备注 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。
资源推荐
资源详情
资源评论
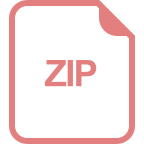
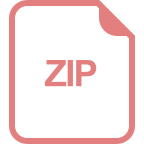
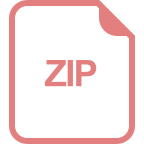
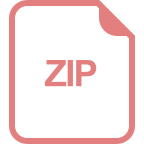
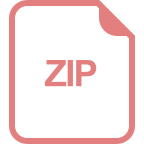
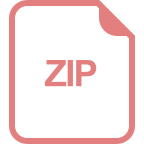
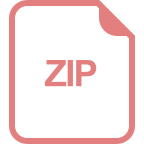
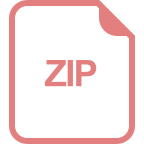
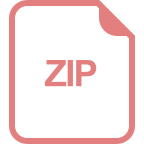
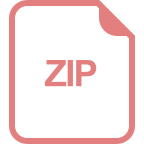
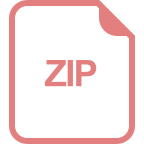
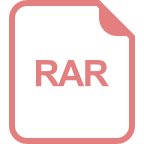
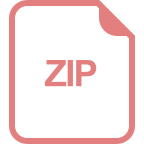
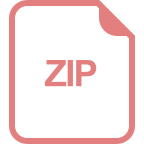
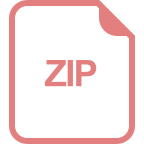
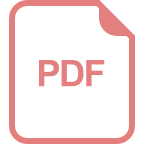
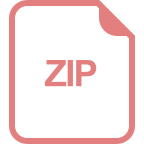
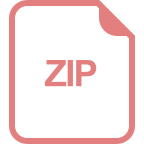
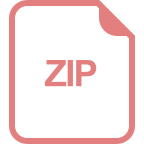
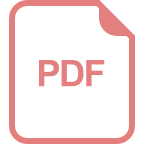
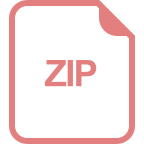
收起资源包目录


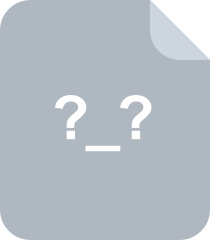


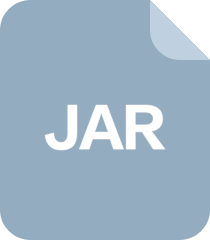
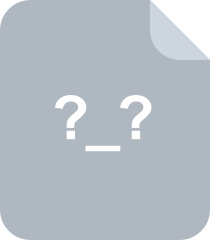







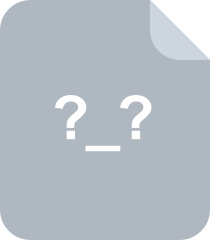





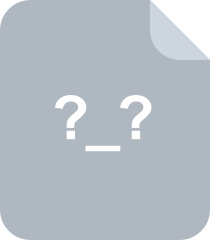





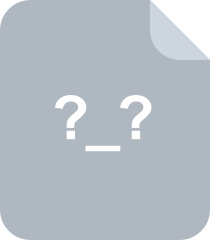
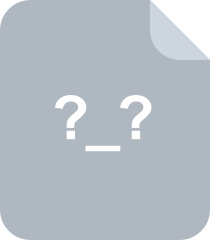


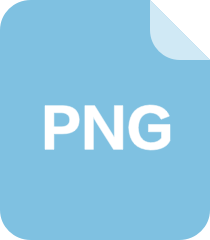
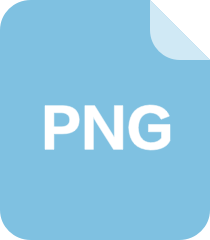

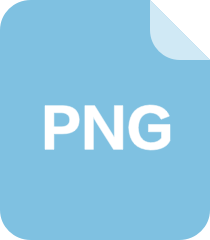
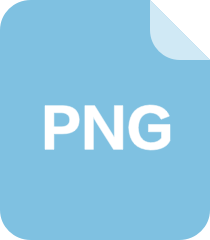

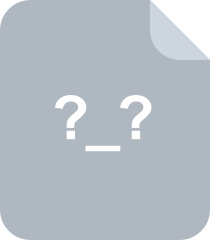

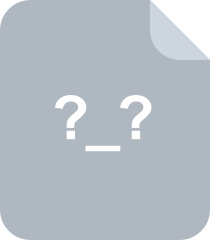
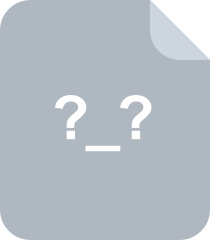

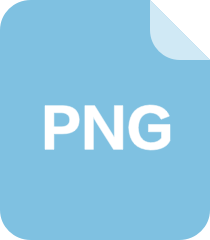
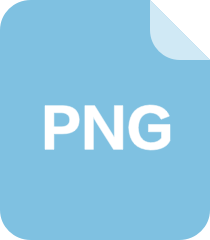

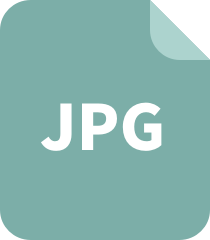
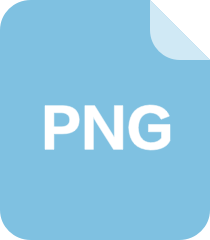
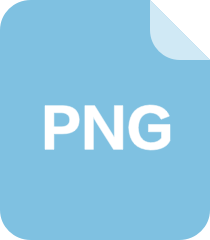

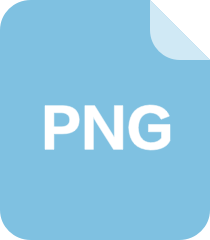
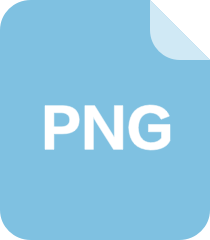

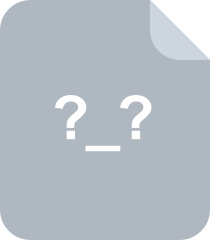
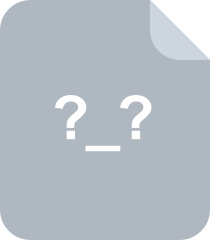
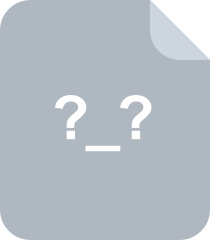

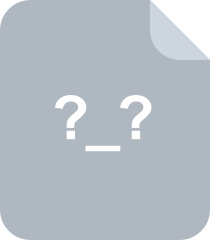

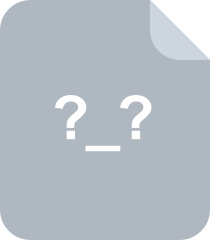
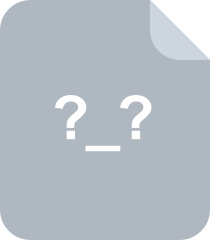
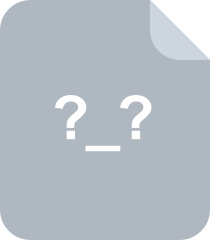
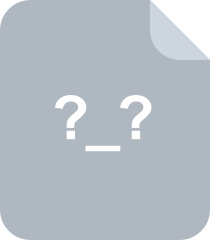
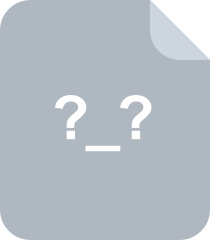
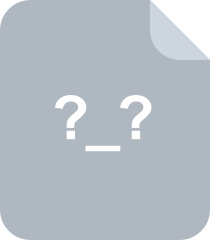
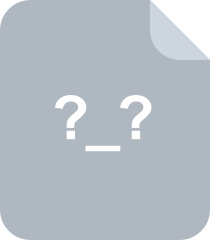
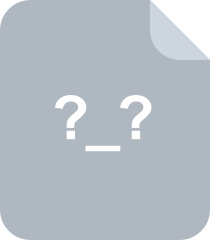
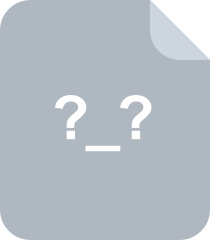
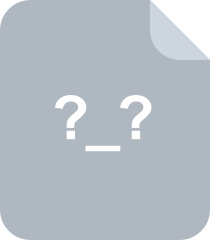
共 35 条
- 1
资源评论
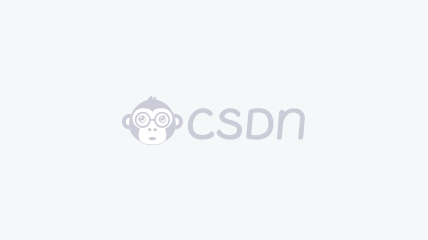

毕业小助手
- 粉丝: 2413
- 资源: 5558
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

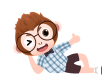
最新资源
- 操作系统介绍(虚拟化CPU、虚拟化内存等).pdf
- SAP SD销售发票自动审批
- 操作系统知识(特征、启动过程).pdf
- gstreamer-1.0-android-universal-1.18.6.tar QGC4.4
- 古诗115-天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙秋思天净沙
- 古诗116-忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母忆母
- 自己动手开发X86操作系统.pdf
- 古诗117-寄天台道士寄天台道士寄天台道士
- 100行代码搞定虚拟语音助手:OpenAI Whisper & StreamLit
- 微信jssdk js文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


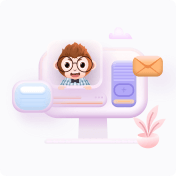
安全验证
文档复制为VIP权益,开通VIP直接复制
