package com.yuanlrc.movie.controller.admin;
import java.util.Date;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.yuanlrc.movie.bean.CodeMsg;
import com.yuanlrc.movie.bean.PageBean;
import com.yuanlrc.movie.bean.Result;
import com.yuanlrc.movie.config.AppConfig;
import com.yuanlrc.movie.constant.SessionConstant;
import com.yuanlrc.movie.entity.admin.OperaterLog;
import com.yuanlrc.movie.entity.admin.OrderAuth;
import com.yuanlrc.movie.entity.admin.Role;
import com.yuanlrc.movie.entity.admin.User;
import com.yuanlrc.movie.service.admin.DatabaseBakService;
import com.yuanlrc.movie.service.admin.OperaterLogService;
import com.yuanlrc.movie.service.admin.OrderAuthService;
import com.yuanlrc.movie.service.admin.UserService;
import com.yuanlrc.movie.service.common.AccountService;
import com.yuanlrc.movie.service.common.CinemaHallSessionService;
import com.yuanlrc.movie.service.common.CinemaService;
import com.yuanlrc.movie.service.common.MovieService;
import com.yuanlrc.movie.service.common.OrderService;
import com.yuanlrc.movie.service.common.PayLogService;
import com.yuanlrc.movie.util.SessionUtil;
import com.yuanlrc.movie.util.StringUtil;
import com.yuanlrc.movie.util.ValidateEntityUtil;
/**
* 系统控制器
* @author Administrator
*
*/
@RequestMapping("/system")
@Controller
public class SystemController {
@Autowired
private OrderAuthService orderAuthService;
@Autowired
private OperaterLogService operaterLogService;
@Autowired
private UserService userService;
@Autowired
private DatabaseBakService databaseBakService;
@Autowired
private CinemaService cinemaService;
@Autowired
private MovieService movieService;
@Autowired
private AccountService accountService;
@Autowired
private OrderService orderService;
@Autowired
private CinemaHallSessionService cinemaHallSessionService;
@Autowired
private PayLogService payLogService;
@Value("${show.tips.text}")
private String showTipsText;
@Value("${show.tips.url.text}")
private String showTipsUrlText;
@Value("${show.tips.btn.text}")
private String showTipsBtnText;
@Value("${show.tips.url}")
private String showTipsUtl;
private Logger log = LoggerFactory.getLogger(SystemController.class);
/**
* 登录页面
* @param name
* @param model
* @return
*/
@RequestMapping(value="/login",method=RequestMethod.GET)
public String login(Model model){
return "admin/system/login";
}
/**
* 用户登录提交表单处理方法
* @param request
* @param user
* @param cpacha
* @return
*/
@RequestMapping(value="/login",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> login(HttpServletRequest request,User user,String cpacha){
if(user == null){
return Result.error(CodeMsg.DATA_ERROR);
}
//用统一验证实体方法验证是否合法
CodeMsg validate = ValidateEntityUtil.validate(user);
if(validate.getCode() != CodeMsg.SUCCESS.getCode()){
return Result.error(validate);
}
//表示实体信息合法,开始验证验证码是否为空
if(StringUtils.isEmpty(cpacha)){
return Result.error(CodeMsg.CPACHA_EMPTY);
}
//说明验证码不为空,从session里获取验证码
Object attribute = request.getSession().getAttribute("admin_login");
if(attribute == null){
return Result.error(CodeMsg.SESSION_EXPIRED);
}
//表示session未失效,进一步判断用户填写的验证码是否正确
if(!cpacha.equalsIgnoreCase(attribute.toString())){
return Result.error(CodeMsg.CPACHA_ERROR);
}
//表示验证码正确,开始查询数据库,检验密码是否正确
User findByUsername = userService.findByUsername(user.getUsername());
//判断是否为空
if(findByUsername == null){
return Result.error(CodeMsg.ADMIN_USERNAME_NO_EXIST);
}
//表示用户存在,进一步对比密码是否正确
if(!findByUsername.getPassword().equals(user.getPassword())){
return Result.error(CodeMsg.ADMIN_PASSWORD_ERROR);
}
//表示密码正确,接下来判断用户状态是否可用
if(findByUsername.getStatus() == User.ADMIN_USER_STATUS_UNABLE){
return Result.error(CodeMsg.ADMIN_USER_UNABLE);
}
//检查用户所属角色状态是否可用
if(findByUsername.getRole() == null || findByUsername.getRole().getStatus() == Role.ADMIN_ROLE_STATUS_UNABLE){
return Result.error(CodeMsg.ADMIN_USER_ROLE_UNABLE);
}
//检查用户所属角色的权限是否存在
if(findByUsername.getRole().getAuthorities() == null || findByUsername.getRole().getAuthorities().size() == 0){
return Result.error(CodeMsg.ADMIN_USER_ROLE_AUTHORITES_EMPTY);
}
//检查一切符合,可以登录,将用户信息存放至session
request.getSession().setAttribute(SessionConstant.SESSION_USER_LOGIN_KEY, findByUsername);
//销毁session中的验证码
request.getSession().setAttribute("admin_login", null);
//将登陆记录写入日志库
operaterLogService.add("用户【"+user.getUsername()+"】于【" + StringUtil.getFormatterDate(new Date(), "yyyy-MM-dd HH:mm:ss") + "】登录系统!");
log.info("用户成功登录,user = " + findByUsername);
return Result.success(true);
}
/**
* 登录成功后的系统主页
* @param model
* @return
*/
@RequestMapping(value="/index")
public String index(Model model){
model.addAttribute("operatorLogs", operaterLogService.findLastestLog(10));
model.addAttribute("userTotal", accountService.count());
model.addAttribute("movieTotal", movieService.count());
model.addAttribute("cinemaTotal", cinemaService.count());
model.addAttribute("orderTotal", orderService.count());
model.addAttribute("cinemaHallSessionTotal", cinemaHallSessionService.count());
model.addAttribute("payLogTotal", payLogService.count());
model.addAttribute("paySuccessTotal", payLogService.countPaySuccess());
model.addAttribute("moneyTotal", movieService.sumTotalMoney());
model.addAttribute("allPayLogList", payLogService.statsAll(5));
model.addAttribute("paidPayLogList", payLogService.statsPaid(5));
model.addAttribute("topMovieList", movieService.findTopMoneyList());
model.addAttribute("showTipsText", showTipsText);
model.addAttribute("showTipsUrlText", showTipsUrlText);
model.addAttribute("showTipsUtl", showTipsUtl);
model.addAttribute("showTipsBtnText", showTipsBtnText);
return "admin/system/index";
}
/**
* 注销登录
* @return
*/
@RequestMapping(value="/logout")
public String logout(){
User loginedUser = SessionUtil.getLoginedUser();
if(loginedUser != null){
SessionUtil.set(SessionConstant.SESSION_USER_LOGIN_KEY, null);
}
return "redirect:login";
}
/**
* 无权限提示页面
* @return
*/
@RequestMapping(value="/no_right")
public String noRight(){
return "admin/system/no_right";
}
/**
* 修改用户个人信息
* @return
*/
@RequestMapping(value="/update_userinfo",method=RequestMethod.GET)
public String updateUserInfo(){
return "admin/system/update_userinfo";
}
/**
* 修改个人信息保存
* @param user
* @return
*/
@RequestMapping(value="/update_userinfo",method=RequestMethod.POST)
public String updateUserInfo(User user){
User loginedUser = SessionUtil.getLoginedUser();
loginedUser.setEmail(user.getEmail());
loginedUser.setMobile(user.getMobile());
loginedUser.setHeadPic(user.getHeadPic());
//首先保存到数据库
userService.save(loginedUser);
//更新session里的值
SessionUtil.set(SessionConstant.SESSIO
没有合适的资源?快使用搜索试试~ 我知道了~
毕业设计&课设-SpringBoot电影管理系统.zip
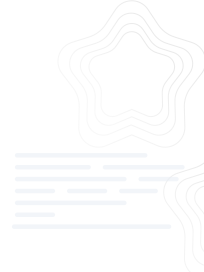
共687个文件
jpg:185个
js:173个
java:114个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 161 浏览量
2024-06-09
09:17:21
上传
评论
收藏 32.18MB ZIP 举报
温馨提示
该资源内项目源码是个人的课程设计,代码都测试ok,都是运行成功后才上传资源,答辩评审平均分达到96分,放心下载使用! ## 项目备注 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。 该资源内项目源码是个人的课程设计,代码都测试ok,都是运行成功后才上传资源,答辩评审平均分达到96分,放心下载使用! ## 项目备注 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。
资源推荐
资源详情
资源评论
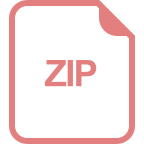
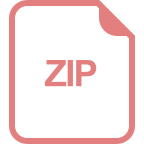
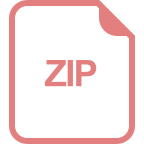
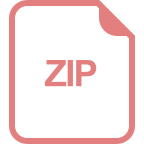
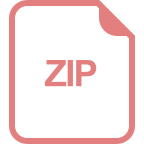
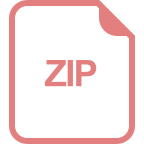
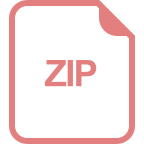
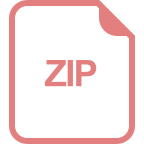
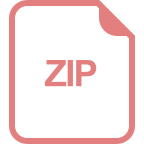
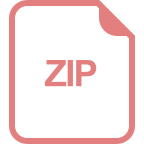
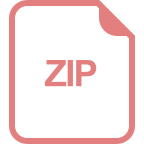
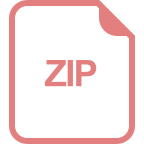
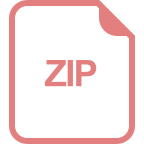
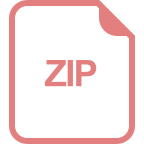
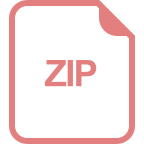
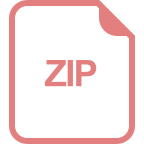
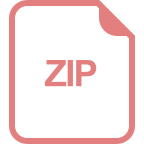
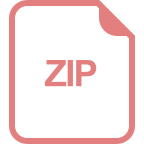
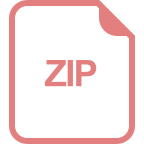
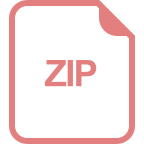
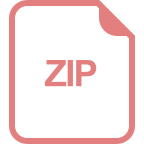
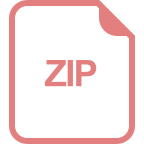
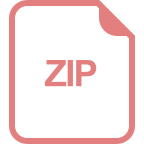
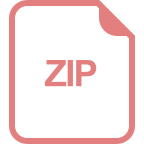
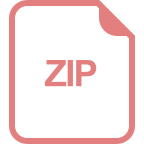
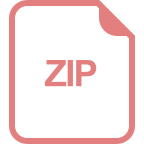
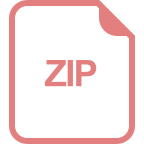
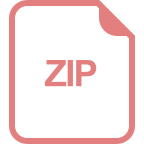
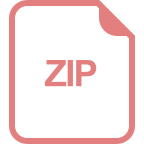
收起资源包目录

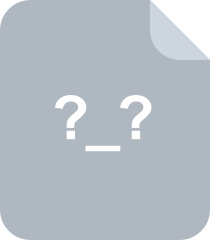
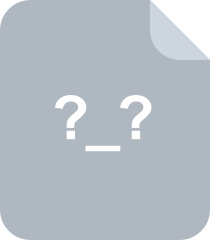
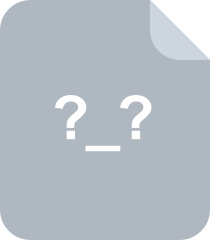
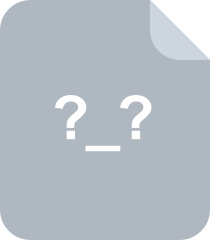
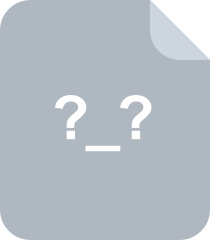
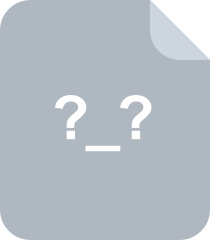
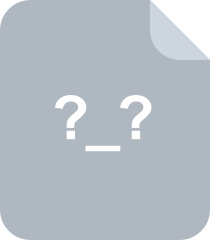
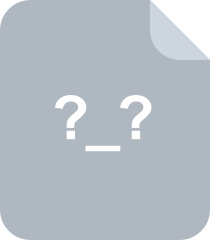
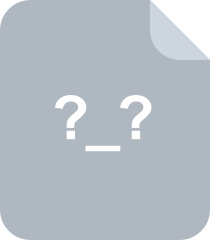
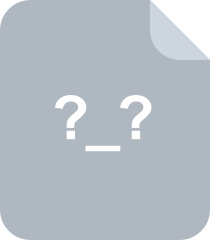
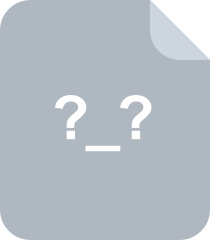
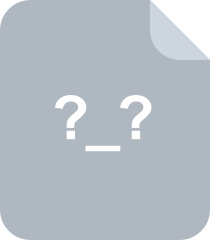
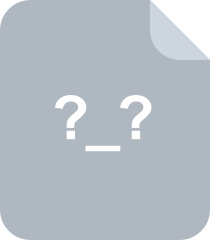
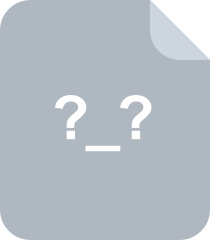
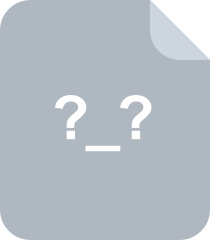
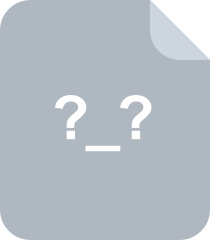
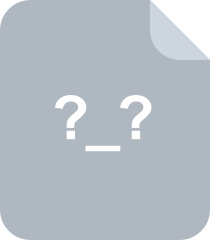
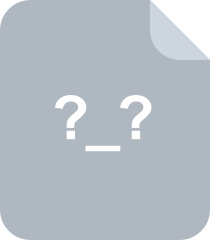
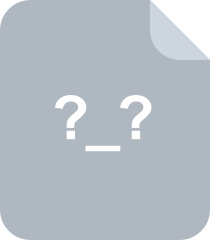
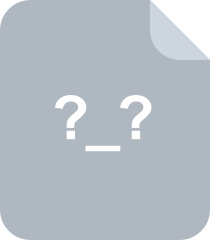
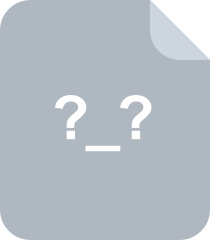
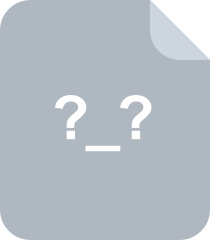
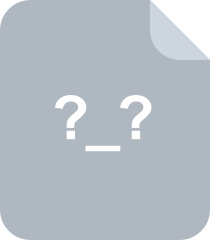
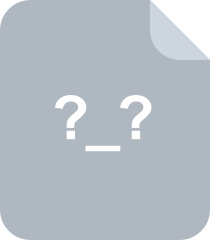
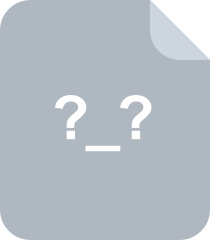
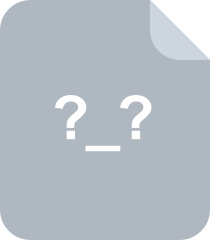
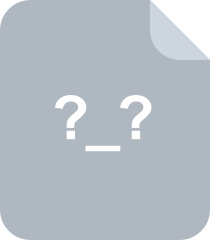
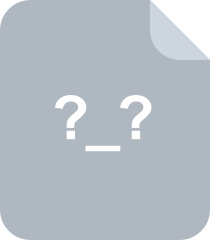
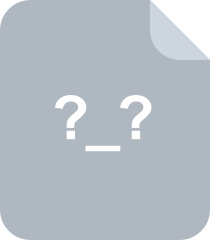
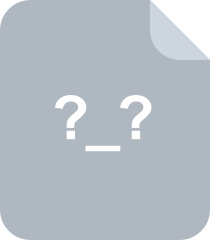
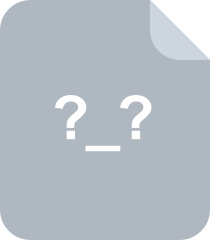
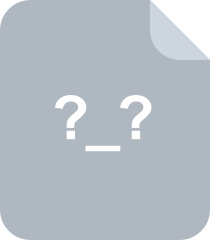
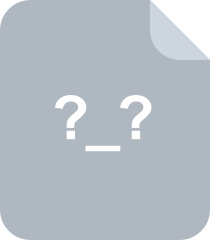
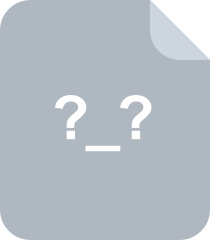
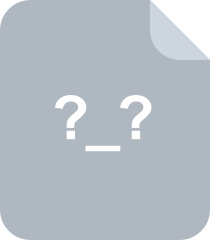
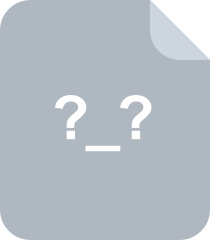
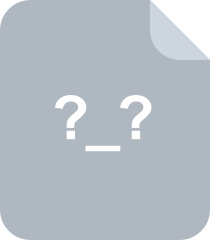
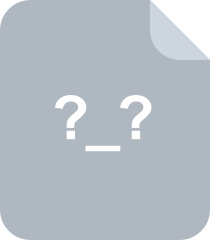
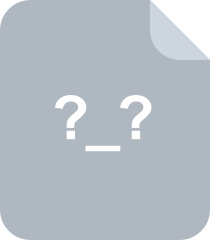
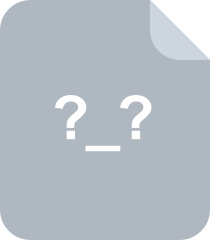
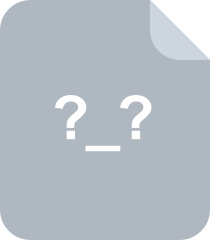
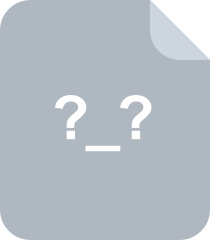
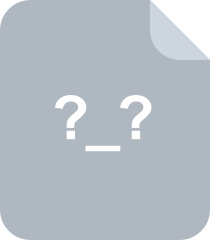
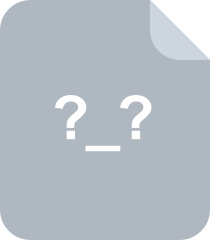
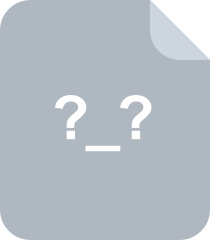
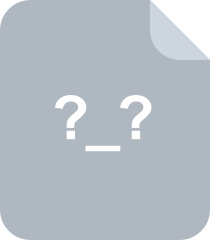
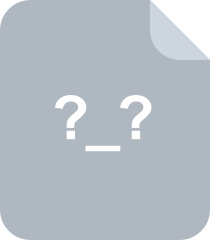
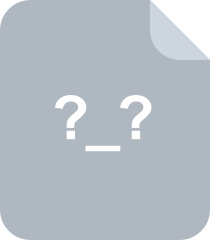
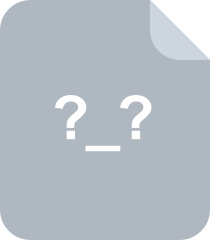
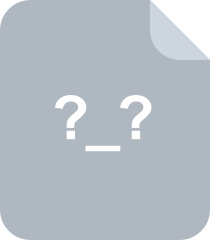
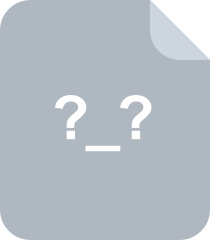
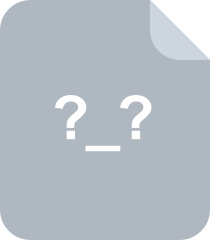
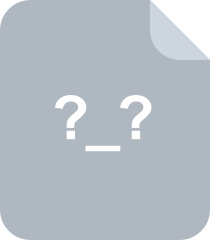
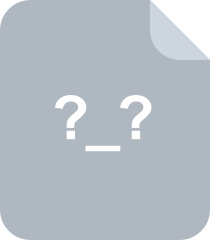
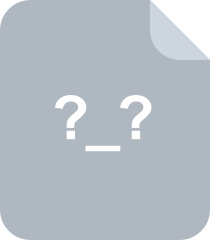
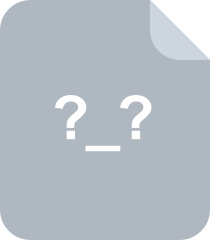
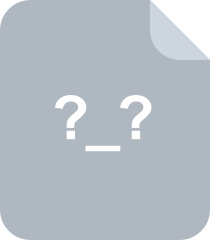
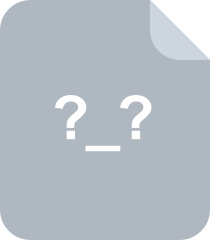
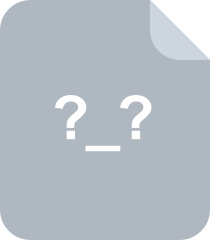
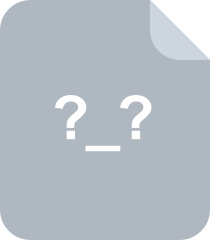
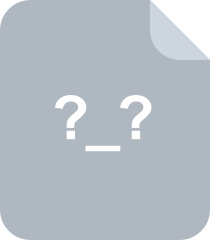
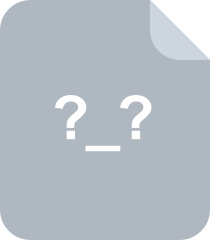
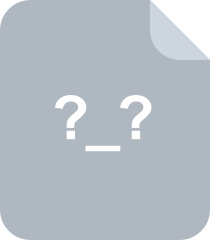
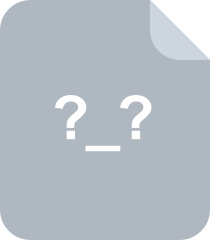
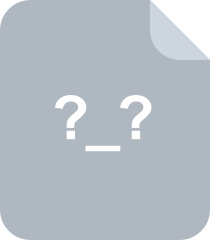
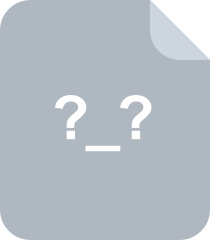
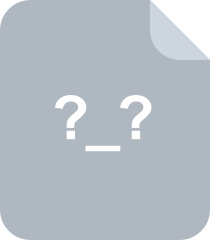
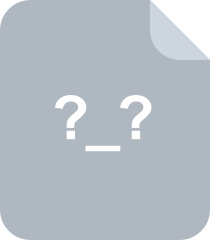
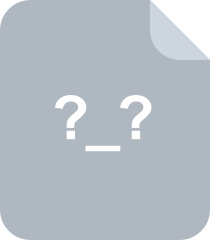
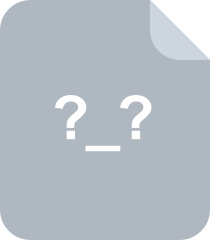
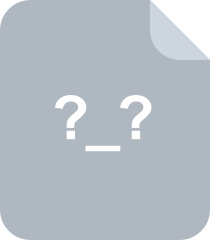
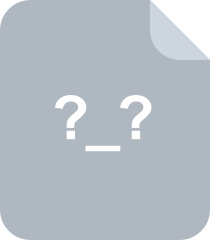
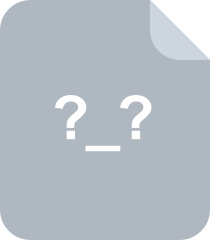
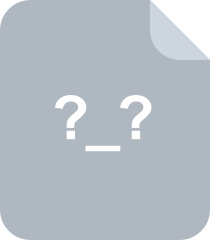
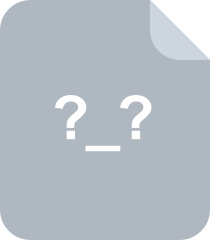
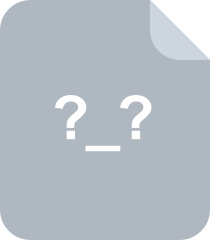
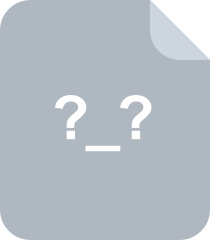
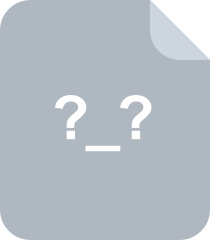
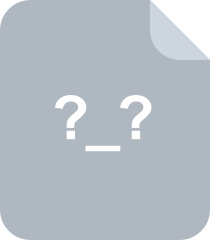
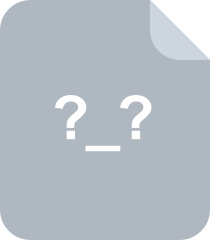
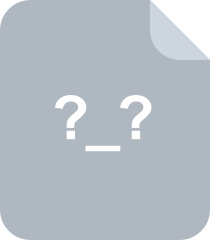
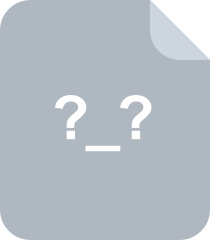
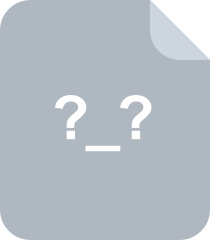
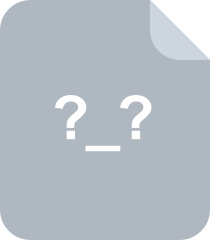
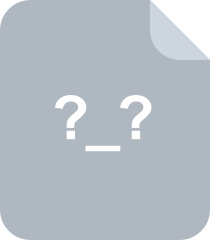
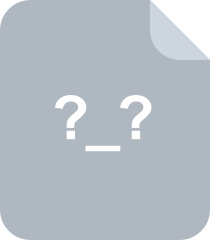
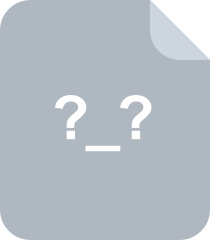
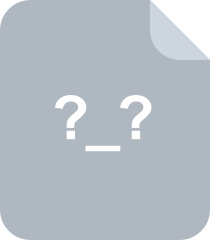
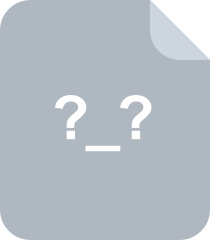
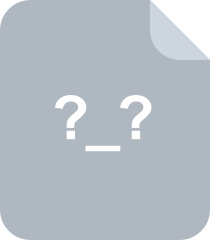
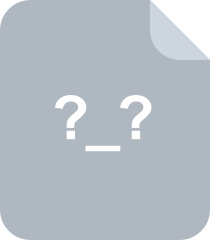
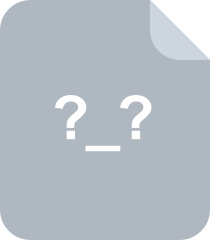
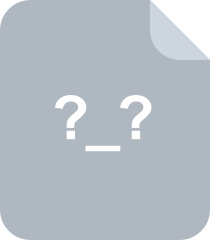
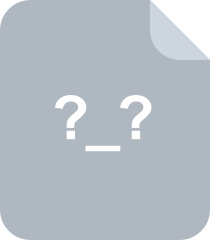
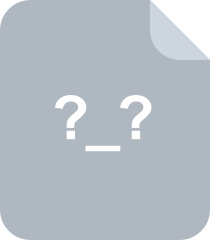
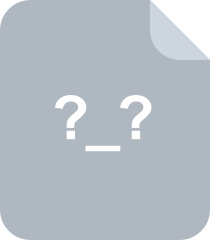
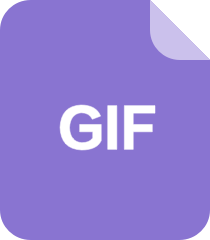
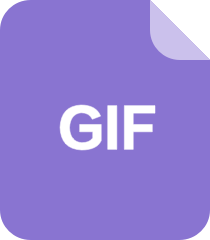
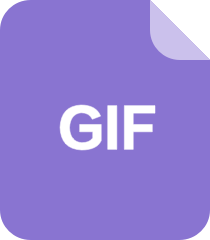
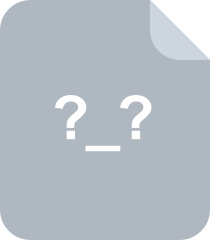
共 687 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
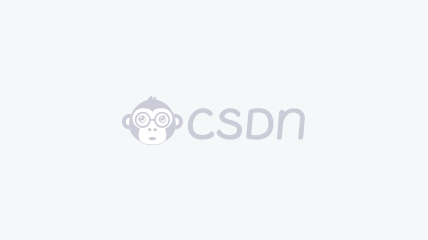

毕业小助手
- 粉丝: 2765
- 资源: 5583
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

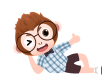
最新资源
- 精选项目-外卖搭伴拼团php后端.zip
- 精选项目-音乐播放器带后端.zip
- 精选项目-游轮中心带后端.zip
- 精选项目-云商城(带php后端).zip
- 单目相机+投影仪标定算法,C++语言,可同时进行相机标定与投影仪标定,标定结果以yml文件格式进行输出 非matlab工具箱 重投影误差均在0.1个像素内
- 线上辅导班系统(代码+数据库+LW)
- 永磁同步电机转速滑模控制Matlab simulink仿真模型,参数已设置好,可直接运行 属于PMSM转速电流双闭环矢量控制系统 电流内环采用PI控制器,转速外环采用滑模控制 波形完美,包含原理
- 锐捷办公云桌面-资料包
- 计算机视觉深度修复领域的创新模型DepthLab及其应用
- 基于Opencv和Python的车道线检测系统(带UI界面) 在自动驾驶中,让汽车保持在车道线内是非常重要的,所以这次我们来说说车道线的检测 我们主要用到的是openCV, numpy, matpl
- openssh-9.8p1 RPM安装包
- openssl 1.1.1s RPM安装包
- 基于长短期记忆网络算法LSTM的时间序列预测 单输入单输出预测 代码含详细注释,不负责 数据存入Excel,替方便,指标计算有决定系数R2,平均绝对误差MAE,平均相对误差MBE
- stm32 远程升级 OTA升级 使用WIFI连接升级 芯片 stm32f103系列 升级方式:wifi模块?自建服务器 升级文件为BIN文件,需要使用配套的exe文件将原来的bin文件内的数据,每隔
- 融合A*改进RRT算法的路径规划代码仿真 全局路径规划 - RRT算法原理 RRT算法,即快速随机树算法(Rapid Random Tree),是LaValle在1998年首次提出的一种高效的路径规划
- foc 基于stm32 弦波无刷电机控制资料 源码 带video教程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


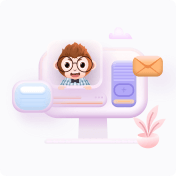
安全验证
文档复制为VIP权益,开通VIP直接复制
