package com.example.demo.controller;
import com.example.demo.auth.jwt.JwtUtil;
import com.example.demo.commom.AjaxResult;
import com.example.demo.commom.Constant;
import com.example.demo.auth.jwt.CustomUserDetailsService;
import com.example.demo.model.User;
import com.example.demo.service.TeamInformationService;
import com.example.demo.service.UserService;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import java.util.HashMap;
import java.util.List;
import java.util.Objects;
@Api(tags = "用户控制器")
@RestController
//@CrossOrigin(origins = "http://localhost:9528")
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@Autowired
private PasswordEncoder passwordEncoder;
@Autowired
private JwtUtil jwtUtil;
@Autowired
private CustomUserDetailsService customUserDetailsService;
@Autowired
private TeamInformationService teamInformationService;
@RequestMapping("/register")
public HashMap<String, Object> register(@RequestParam("username") String username, @RequestParam("password")String password,@RequestParam("status")String status, HttpServletRequest request) {
if(StringUtils.isBlank(username) || StringUtils.isBlank(password) || StringUtils.isBlank(status)){
return AjaxResult.fail(-1,"传输的注册信息不完整");
}
// Validate username length
if (username.length() < 4 || username.length() > 30) {
return AjaxResult.fail(-1, "用户名须介于4到30个字符之间");
}
if(userService.findByUsername(username) != null){
return AjaxResult.fail(-1,"用户名已存在,注册失败");
}
// Validate password strength
if (!password.matches("(?=.*[0-9])(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%^&+=])(?=\\S+$).{8,}")) {
return AjaxResult.fail(-1, "密码至少需要有8个字符且必须包含数字,大写字母,小写字母和特殊字符");
}
String encodedPassword = passwordEncoder.encode(password);
int result = userService.register(username, encodedPassword, status);
if (result == 0) {
return AjaxResult.fail(-1, "注册失败!");
}
// Store the user's information in the session
User user = userService.login(username, encodedPassword);
request.getSession().setAttribute(Constant.SESSION_USERINFO_KEY, user);
return AjaxResult.success("用户注册成功.");
}
@RequestMapping("/login")
public HashMap<String, Object> login(@RequestParam("username") String username, @RequestParam("password")String password, HttpServletRequest request) {
if(StringUtils.isBlank(username) || StringUtils.isBlank(password)){
return AjaxResult.fail(-1,"传输的登录信息不完整");
}
User user = userService.findByUsername(username);
if (user == null || !(passwordEncoder.matches(password, user.getPassword()))) {
return AjaxResult.fail(-1, "登录失败!");
}
// Generate the JWT token using the user details
UserDetails userDetails = customUserDetailsService.loadUserByUsername(username);
String token = jwtUtil.generateToken(userDetails);
// Store the user's information in the session
request.getSession().setAttribute(Constant.SESSION_USERINFO_KEY, user);
// Include the JWT token in the response
HashMap<String, Object> response = AjaxResult.success(user);
response.put("token", token);
return response;
}
@ApiOperation(value = "获取登录用户的状态", notes = "获取当前登录用户的状态")
@RequestMapping("/getLoginUserStatus")
public HashMap<String, Object> getStatus(HttpServletRequest request) {
User user = (User) request.getSession().getAttribute(Constant.SESSION_USERINFO_KEY);
if (user == null) {
return AjaxResult.fail(-1, "用户未登录");
}
User dbUser = userService.findByUsername(user.getUsername());
if (dbUser == null) {
return AjaxResult.fail(-1, "用户非法登录");
}
String status = dbUser.getStatus();
return AjaxResult.success(status);
}
@ApiOperation(value = "获取所有用户", notes = "获取数据库中所有用户信息")
@RequestMapping("/getAllUsers")
public HashMap<String, Object> getAllUsers(HttpServletRequest request) {
User user = (User) request.getSession().getAttribute(Constant.SESSION_USERINFO_KEY);
if (user == null) {
return AjaxResult.fail(-1, "用户未登录");
}
User dbUser = userService.findByUsername(user.getUsername());
if (dbUser == null) {
return AjaxResult.fail(-1, "用户非法登录");
}
List<User> userList = userService.findAllUsers();
return AjaxResult.success(userList);
}
@ApiOperation(value = "获取登录用户信息", notes = "获取当前登录用户的信息")
@RequestMapping("/getLoginUser")
public HashMap<String, Object> getLoginUser(HttpServletRequest request) {
User user = (User) request.getSession().getAttribute(Constant.SESSION_USERINFO_KEY);
if (user == null) {
return AjaxResult.fail(-1, "用户未登录");
}
User dbUser = userService.findByUsername(user.getUsername());
if (dbUser == null) {
return AjaxResult.fail(-1, "用户非法登录");
}
return AjaxResult.success(dbUser);
}
@ApiOperation(value = "修改用户分数", notes = "管理员修改用户的分数")
@RequestMapping("/changeUserScore")
public HashMap<String, Object> changeUserScore(@RequestParam("score") int score, @RequestParam("username") String username,HttpServletRequest request){
if(StringUtils.isBlank(username) || score < 0){
return AjaxResult.fail(-1,"传输的信息不完整或存在非法信息");
}
if(userService.findByUsername(username) == null){
return AjaxResult.fail(-1,"传输的用户名没有用户与其对应");
}
User user = (User) request.getSession().getAttribute(Constant.SESSION_USERINFO_KEY);
if (user == null) {
return AjaxResult.fail(-1, "用户未登录");
}
User dbUser = userService.findByUsername(user.getUsername());
if (dbUser == null) {
return AjaxResult.fail(-1, "用户非法登录");
}
if(!Objects.equals(dbUser.getStatus(), "admin")){
return AjaxResult.fail(-1,"您没有权限修改队员分数.");
}
userService.changeUserScore(username, score);
return AjaxResult.success(200,"修改成功");
}
@ApiOperation(value = "查询队伍中队员", notes = "根据队伍名查询对应队伍的队员")
@RequestMapping("/searchUsersByTeamName")
public HashMap<String, Object> searchUsersByTeamName(@RequestParam("team_name") String team_name){
if(StringUtils.isBlank(team_name)){
return AjaxResult.fail(-1,"队名不能为空");
}
if(teamInformationService.findTeamByName(team_name) == null){
return AjaxResult.fail(-1,"传输的队名没有队伍与其对应");
}
List<User> users = userService.findByTeamname(team_name);
return AjaxResult.success(users);
}
@ApiOperation(value = "修改用户个人信息", notes = "用户修改自己个人信息")
@RequestMapping("/changeUserInfor")
public HashMap<String, Object> updateUserByUserId(@RequestBod
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
vue SpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+vue 的比赛管理系统.zipSpringBoot+Mybatis+
资源推荐
资源详情
资源评论
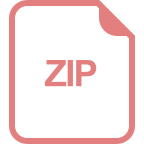
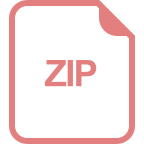
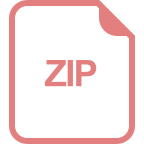
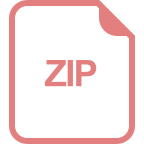
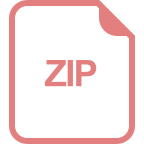
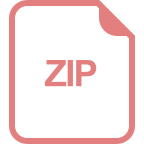
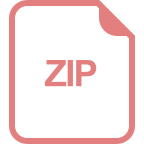
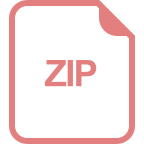
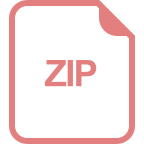
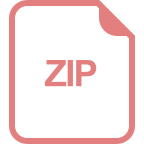
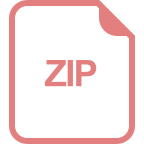
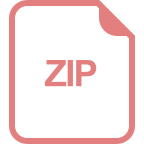
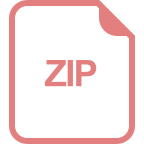
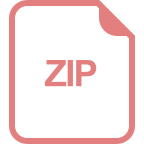
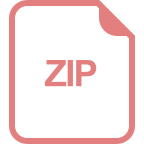
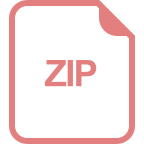
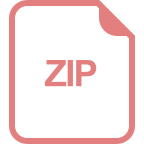
收起资源包目录


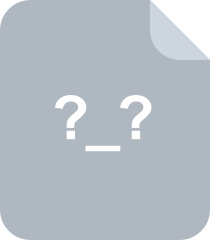
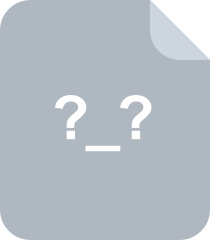






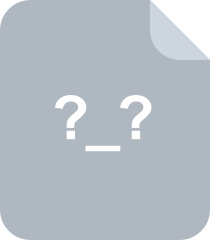



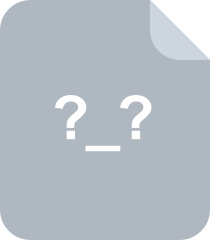
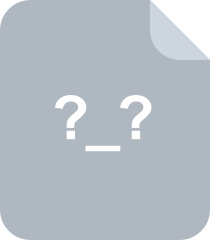
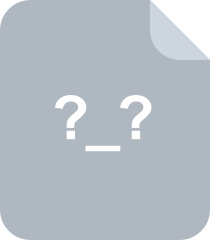
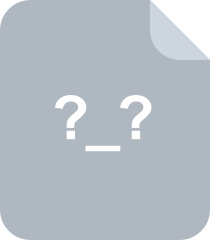
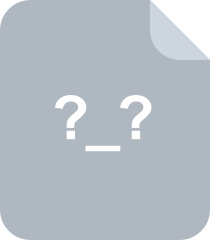

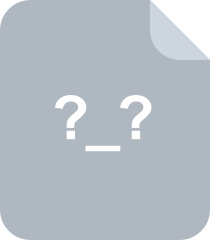
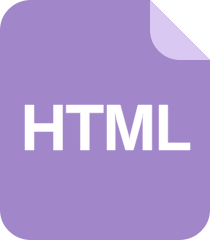


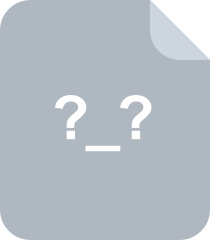
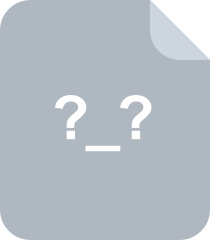
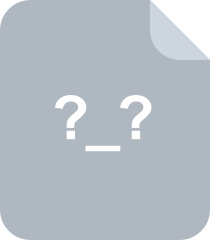
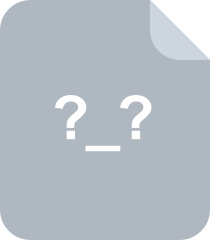
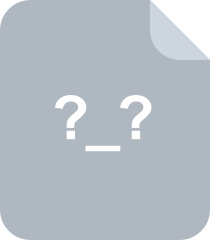
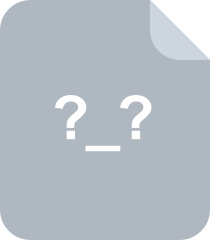
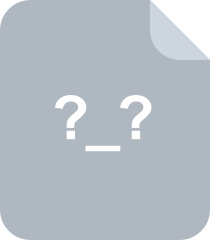
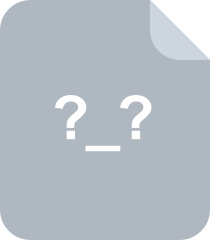

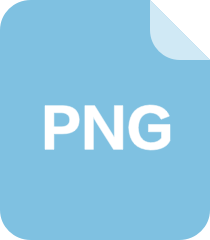
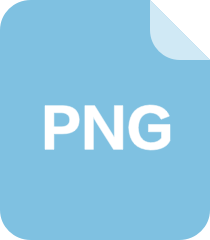

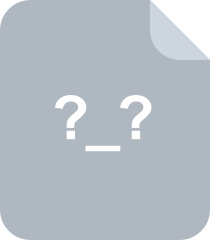
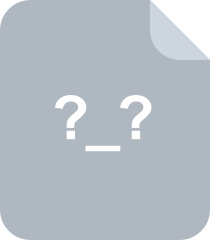
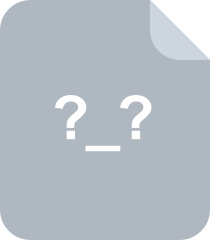
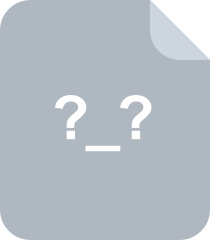
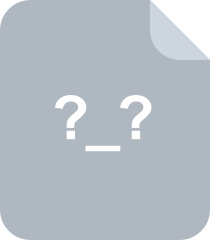
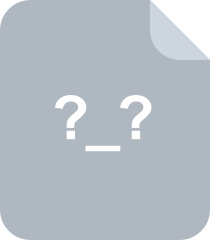
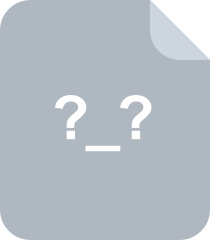
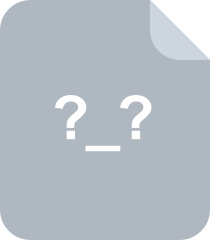

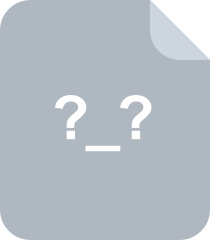
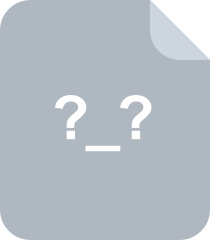
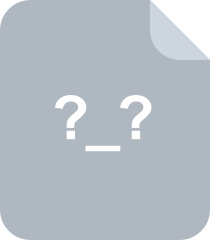




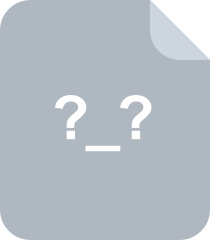

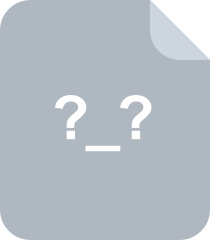
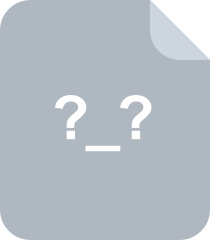
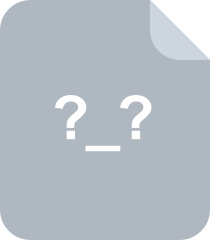
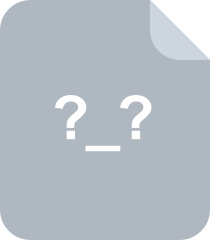

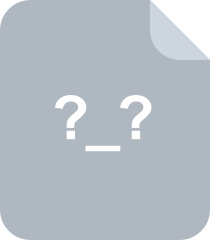
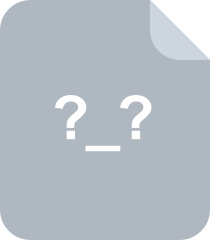
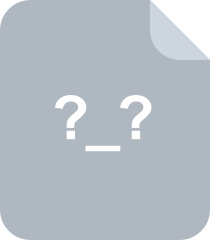
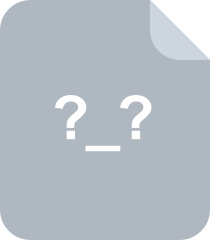
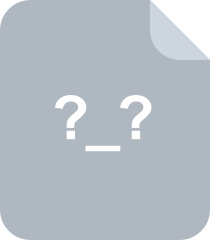

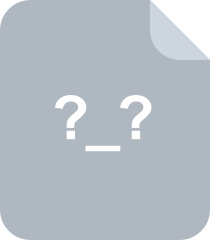
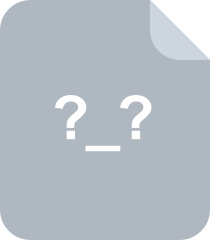
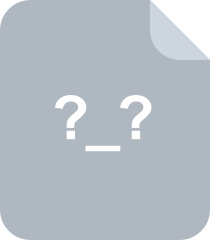
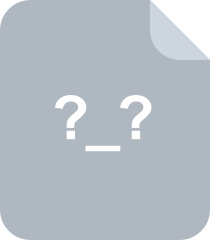

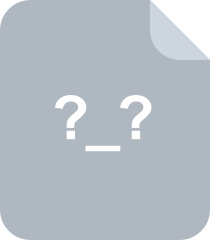
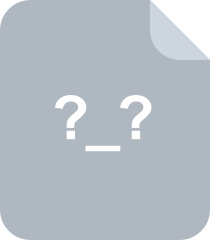
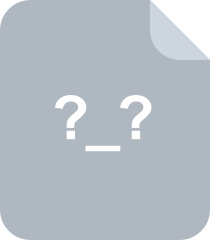
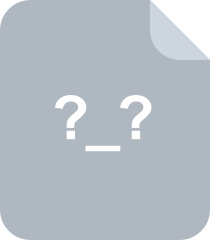
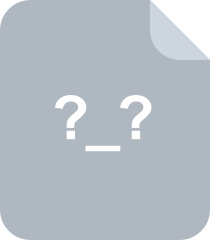


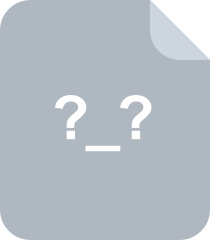
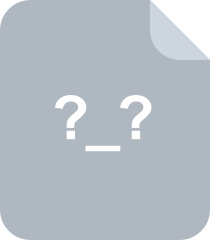
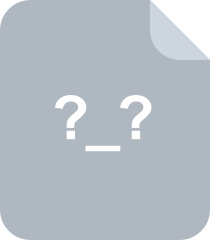

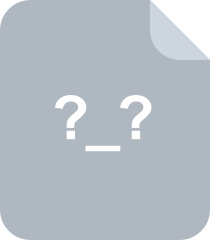
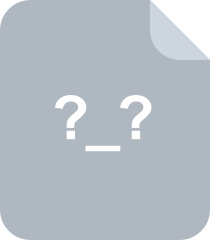
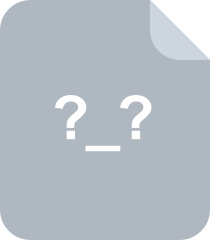
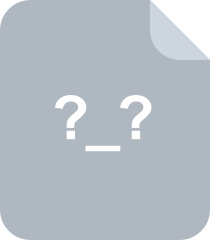
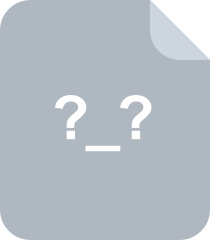

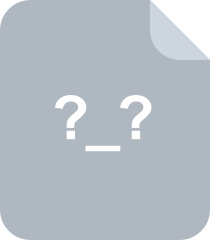
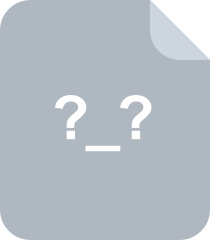
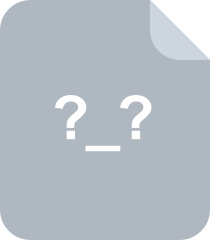
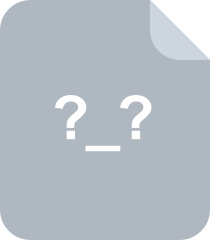
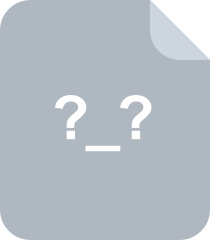
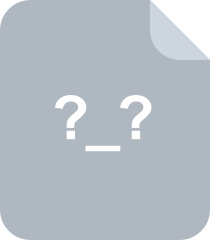


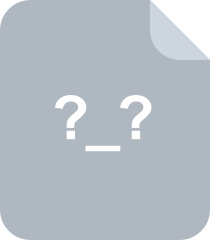
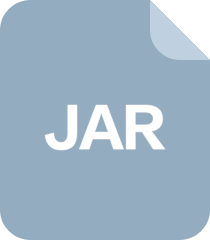
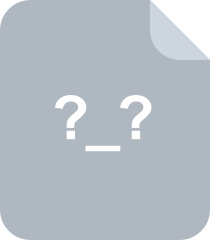
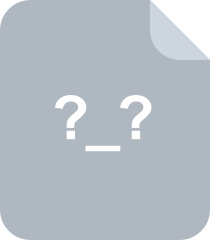
共 68 条
- 1
资源评论
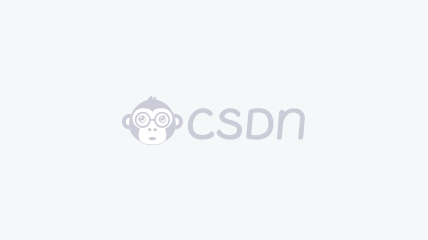

毕业小助手
- 粉丝: 2762
- 资源: 5583
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

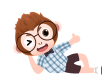
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


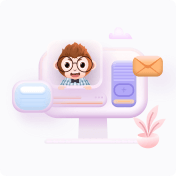
安全验证
文档复制为VIP权益,开通VIP直接复制
