<?php
/**
* Zend Framework
*
* LICENSE
*
* This source file is subject to the new BSD license that is bundled
* with this package in the file LICENSE.txt.
* It is also available through the world-wide-web at this URL:
* http://framework.zend.com/license/new-bsd
* If you did not receive a copy of the license and are unable to
* obtain it through the world-wide-web, please send an email
* to license@zend.com so we can send you a copy immediately.
*
* @category Zend
* @package Zend_Date
* @copyright Copyright (c) 2005-2008 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
* @version $Id: Date.php 13676 2009-01-17 19:36:30Z alexander $
*/
/**
* Include needed Date classes
*/
require_once 'Zend/Date/DateObject.php';
require_once 'Zend/Locale.php';
require_once 'Zend/Locale/Format.php';
require_once 'Zend/Locale/Math.php';
/**
* @category Zend
* @package Zend_Date
* @copyright Copyright (c) 2005-2008 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
*/
class Zend_Date extends Zend_Date_DateObject
{
private $_locale = null;
// Fractional second variables
private $_fractional = 0;
private $_precision = 3;
private static $_options = array(
'format_type' => 'iso', // format for date strings 'iso' or 'php'
'fix_dst' => true, // fix dst on summer/winter time change
'extend_month' => false, // false - addMonth like SQL, true like excel
'cache' => null, // cache to set
'timesync' => null // timesync server to set
);
// Class wide Date Constants
// day formats
const DAY = 'DAY'; // d - 2 digit day of month, 01-31
const DAY_SHORT = 'DAY_SHORT'; // j - 1,2 digit day of month, 1-31
const DAY_SUFFIX = 'DAY_SUFFIX'; // S - english suffix day of month, st-th
const DAY_OF_YEAR = 'DAY_OF_YEAR'; // z - Number of day of year
const WEEKDAY = 'WEEKDAY'; // l - full day name - locale aware, Monday - Sunday
const WEEKDAY_SHORT = 'WEEKDAY_SHORT'; // --- 3 letter day of week - locale aware, Mon-Sun
const WEEKDAY_NARROW = 'WEEKDAY_NARROW'; // --- 1 letter day name - locale aware, M-S
const WEEKDAY_NAME = 'WEEKDAY_NAME'; // D - abbreviated day name, 1-3 letters - locale aware, Mon-Sun
const WEEKDAY_8601 = 'WEEKDAY_8601'; // N - digit weekday ISO 8601, 1-7 1 = monday, 7=sunday
const WEEKDAY_DIGIT = 'WEEKDAY_DIGIT'; // w - weekday, 0-6 0=sunday, 6=saturday
// week formats
const WEEK = 'WEEK'; // W - number of week ISO8601, 1-53
// month formats
const MONTH = 'MONTH'; // m - 2 digit month, 01-12
const MONTH_SHORT = 'MONTH_SHORT'; // n - 1 digit month, no leading zeros, 1-12
const MONTH_DAYS = 'MONTH_DAYS'; // t - Number of days this month
const MONTH_NAME = 'MONTH_NAME'; // F - full month name - locale aware, January-December
const MONTH_NAME_SHORT = 'MONTH_NAME_SHORT'; // M - 3 letter monthname - locale aware, Jan-Dec
const MONTH_NAME_NARROW = 'MONTH_NAME_NARROW'; // --- 1 letter month name - locale aware, J-D
// year formats
const YEAR = 'YEAR'; // Y - 4 digit year
const YEAR_SHORT = 'YEAR_SHORT'; // y - 2 digit year, leading zeros 00-99
const YEAR_8601 = 'YEAR_8601'; // o - number of year ISO8601
const YEAR_SHORT_8601= 'YEAR_SHORT_8601';// --- 2 digit number of year ISO8601
const LEAPYEAR = 'LEAPYEAR'; // L - is leapyear ?, 0-1
// time formats
const MERIDIEM = 'MERIDIEM'; // A,a - AM/PM - locale aware, AM/PM
const SWATCH = 'SWATCH'; // B - Swatch Internet Time
const HOUR = 'HOUR'; // H - 2 digit hour, leading zeros, 00-23
const HOUR_SHORT = 'HOUR_SHORT'; // G - 1 digit hour, no leading zero, 0-23
const HOUR_AM = 'HOUR_AM'; // h - 2 digit hour, leading zeros, 01-12 am/pm
const HOUR_SHORT_AM = 'HOUR_SHORT_AM'; // g - 1 digit hour, no leading zero, 1-12 am/pm
const MINUTE = 'MINUTE'; // i - 2 digit minute, leading zeros, 00-59
const MINUTE_SHORT = 'MINUTE_SHORT'; // --- 1 digit minute, no leading zero, 0-59
const SECOND = 'SECOND'; // s - 2 digit second, leading zeros, 00-59
const SECOND_SHORT = 'SECOND_SHORT'; // --- 1 digit second, no leading zero, 0-59
const MILLISECOND = 'MILLISECOND'; // --- milliseconds
// timezone formats
const TIMEZONE_NAME = 'TIMEZONE_NAME'; // e - timezone string
const DAYLIGHT = 'DAYLIGHT'; // I - is Daylight saving time ?, 0-1
const GMT_DIFF = 'GMT_DIFF'; // O - GMT difference, -1200 +1200
const GMT_DIFF_SEP = 'GMT_DIFF_SEP'; // P - seperated GMT diff, -12:00 +12:00
const TIMEZONE = 'TIMEZONE'; // T - timezone, EST, GMT, MDT
const TIMEZONE_SECS = 'TIMEZONE_SECS'; // Z - timezone offset in seconds, -43200 +43200
// date strings
const ISO_8601 = 'ISO_8601'; // c - ISO 8601 date string
const RFC_2822 = 'RFC_2822'; // r - RFC 2822 date string
const TIMESTAMP = 'TIMESTAMP'; // U - unix timestamp
// additional formats
const ERA = 'ERA'; // --- short name of era, locale aware,
const ERA_NAME = 'ERA_NAME'; // --- full name of era, locale aware,
const DATES = 'DATES'; // --- standard date, locale aware
const DATE_FULL = 'DATE_FULL'; // --- full date, locale aware
const DATE_LONG = 'DATE_LONG'; // --- long date, locale aware
const DATE_MEDIUM = 'DATE_MEDIUM'; // --- medium date, locale aware
const DATE_SHORT = 'DATE_SHORT'; // --- short date, locale aware
const TIMES = 'TIMES'; // --- standard time, locale aware
const TIME_FULL = 'TIME_FULL'; // --- full time, locale aware
const TIME_LONG = 'TIME_LONG'; // --- long time, locale aware
const TIME_MEDIUM = 'TIME_MEDIUM'; // --- medium time, locale aware
const TIME_SHORT = 'TIME_SHORT'; // --- short time, locale aware
const ATOM = 'ATOM'; // --- DATE_ATOM
const COOKIE = 'COOKIE'; // --- DATE_COOKIE
const RFC_822 = 'RFC_822'; // --- DATE_RFC822
const RFC_850 = 'RFC_850'; // --- DATE_RFC850
const RFC_1036 = 'RFC_1036'; // --- DATE_RFC1036
const RFC_1123 = 'RFC_1123'; // --- DATE_RFC1123
const RFC_3339 = 'RFC_3339'; // --- DATE_RFC3339
const RSS = 'RSS'; // --- DATE_RSS
const W3C = 'W3C'; // --- DATE_W3C
/**
* Generates the standard date object, could be a unix timestamp, localized date,
* string, integer, array and so on. Also parts of dates or time are supported
* Always set the default timezone: http://php.net/date_default_timezone_set
* For example, in your bootstrap: date_default_timezone_set('America/Los_Angeles');
* For detailed instructions please look in the docu.
*
* @param string|integer|Zend_Date|array $date OPTIONAL Date value or value of date part to set
* ,depending on $part. If null the actual time is set
* @param string $part OPTIONAL Defines the input format of $date
* @param string|Zend_Locale $locale OPTIONAL Locale for parsing input
* @return Zend_Date
* @throws Zend_Date_Exception
*/
public function __construct($date = null, $part = null, $locale = null)
{
if (($date !== n
没有合适的资源?快使用搜索试试~ 我知道了~
ZendFramework-1.7.6-minimal.tar.gz
需积分: 0 4 下载量 193 浏览量
2009-03-30
15:09:48
上传
评论
收藏 2.46MB GZ 举报
温馨提示
Zend类是整个Zend Framework的基类,之所以有这个类是为了使Zend Framework遵循DRY原则(Don't Repeat Yourself)。这个类只包含静态方法,这些类方法具有Zend Framework中的很多组件都需要的功能。 (Zend类是个功能性的类,它只包含静态方法,也就是说,不需要实例化就可以直接调用Zend的各种功能方法/函数。通俗地说,Zend类相当于我们熟悉的functions.inc.php,而且是corefunctions.inc.php,提供了最核心最常用的函数。 该包为最简版。
资源推荐
资源详情
资源评论
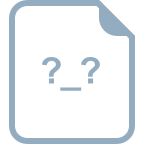
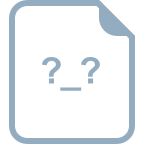
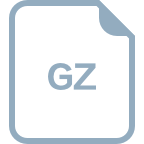
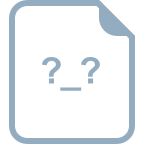
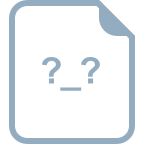
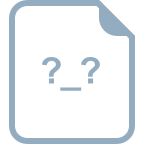
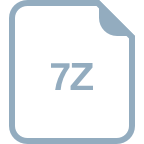
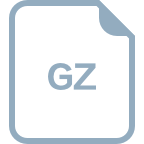
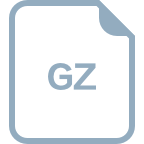
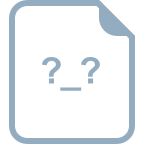
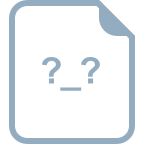
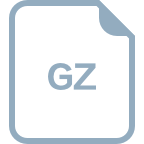
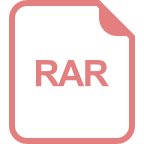
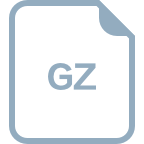
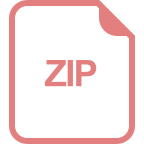
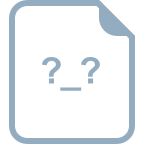
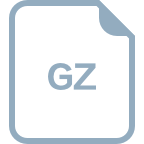
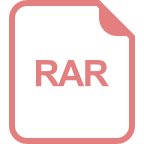
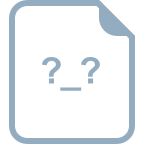
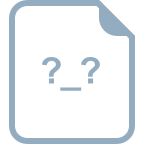
收起资源包目录

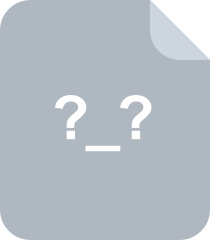
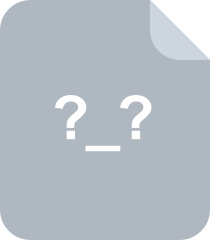
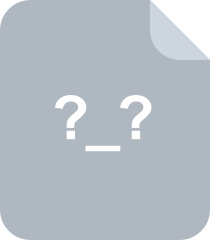
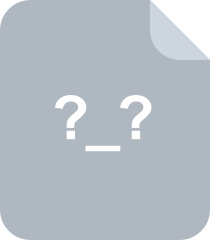
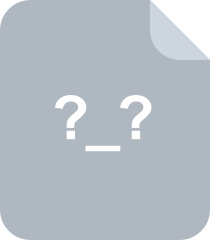
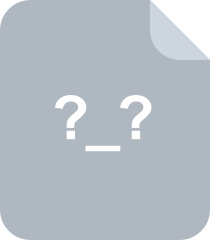
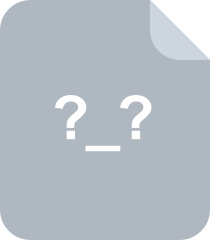
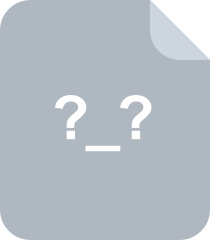
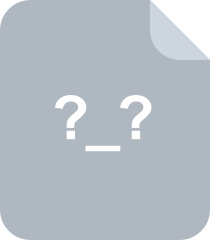
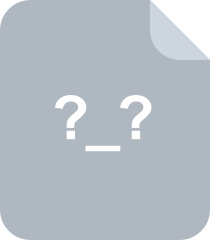
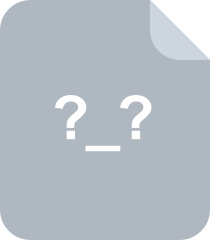
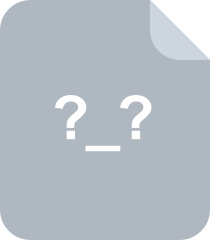
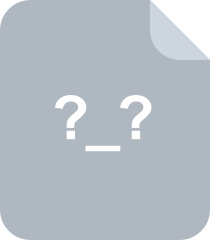
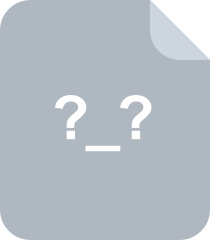
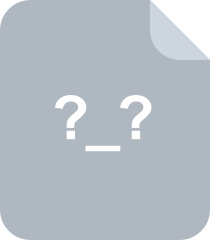
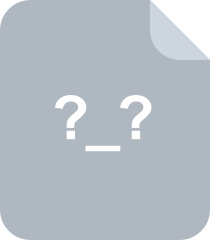
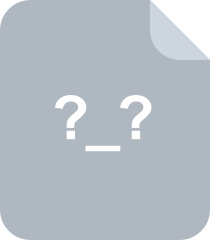
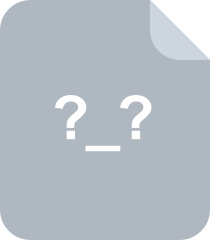
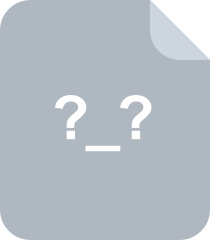
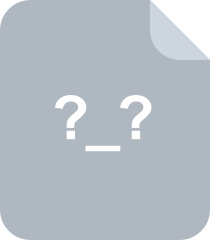
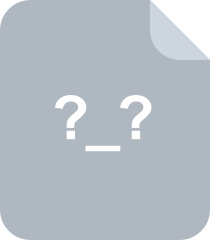
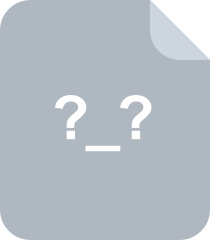
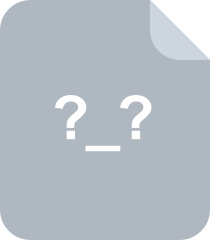
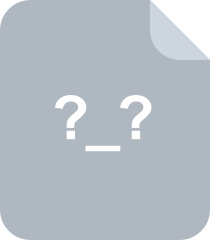
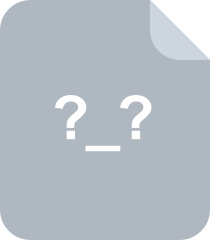
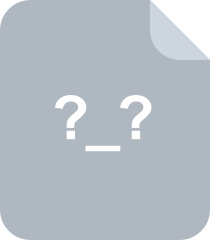
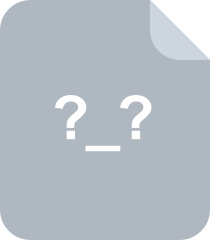
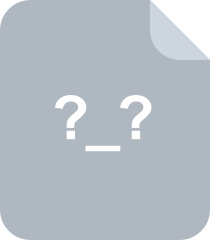
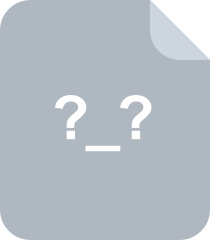
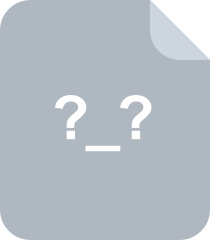
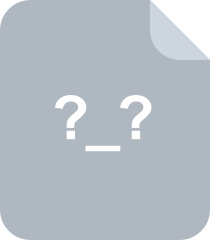
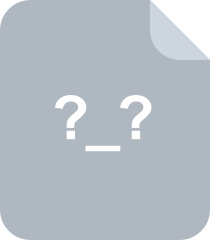
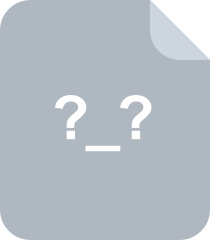
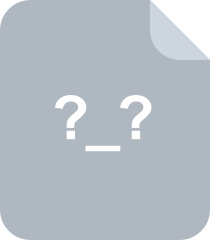
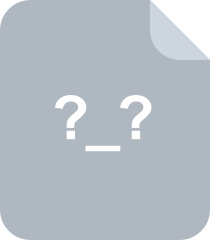
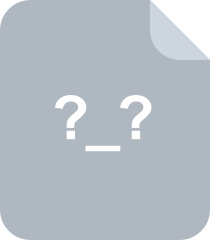
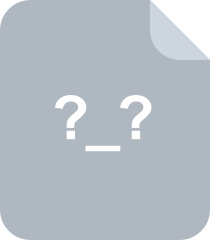
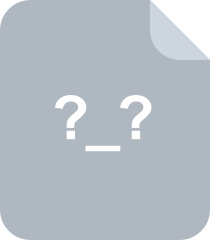
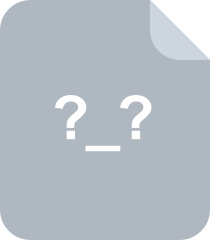
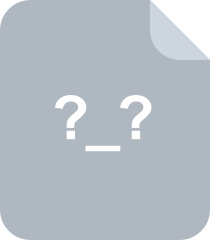
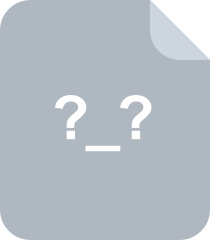
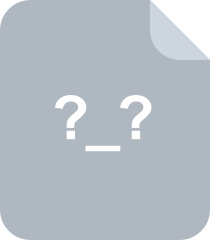
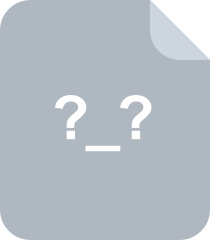
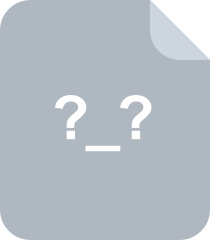
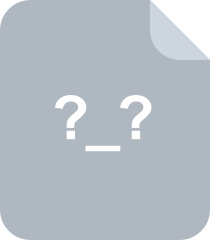
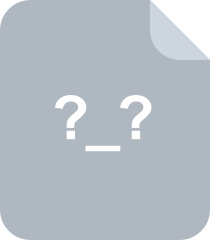
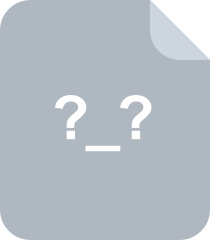
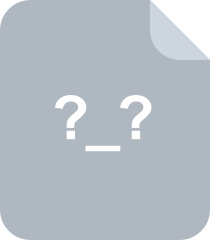
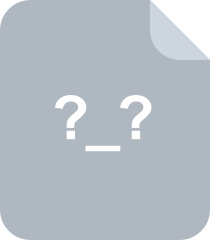
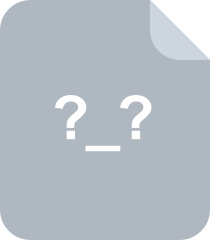
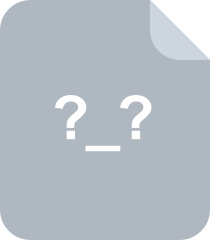
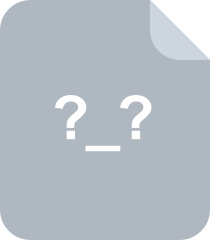
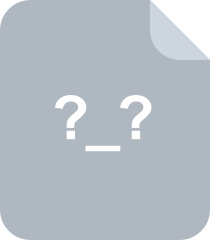
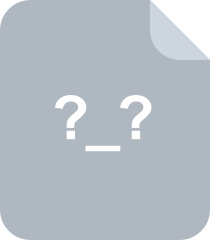
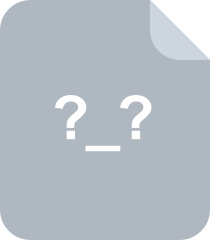
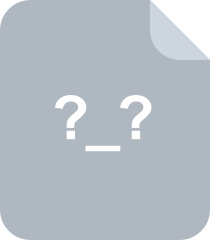
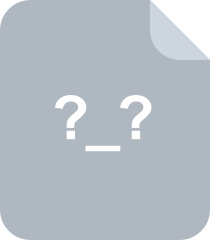
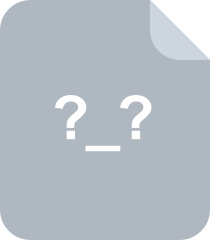
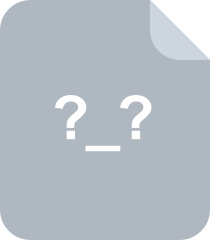
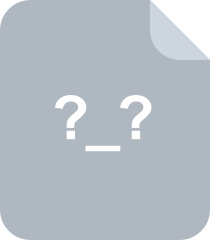
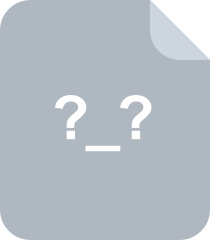
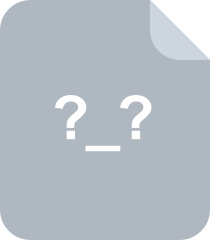
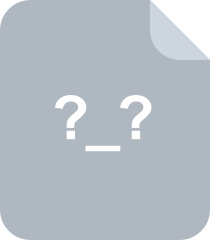
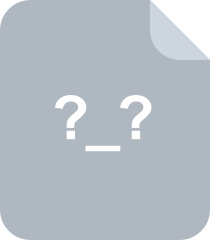
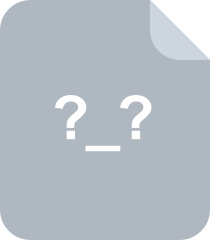
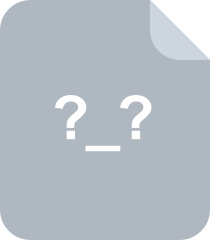
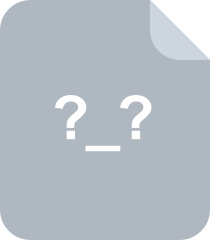
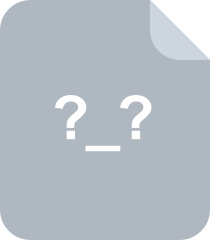
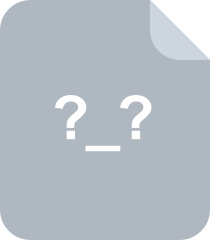
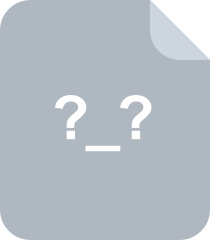
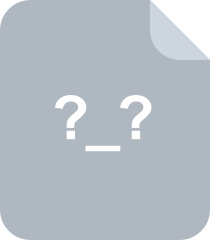
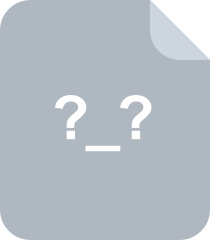
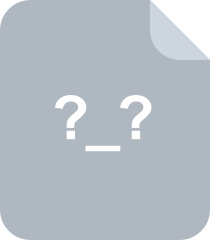
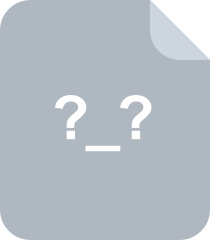
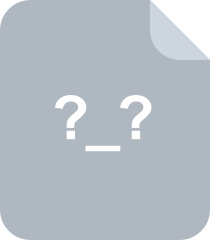
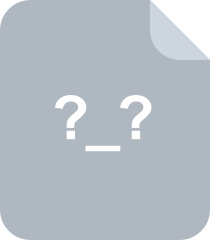
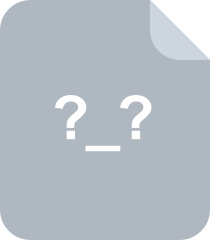
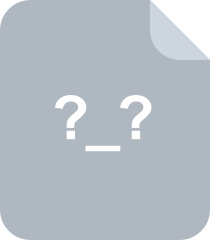
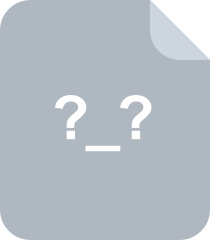
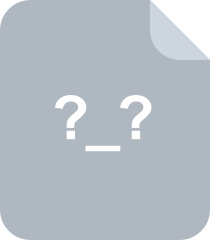
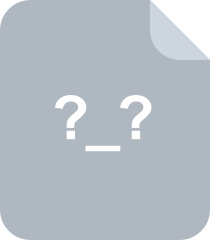
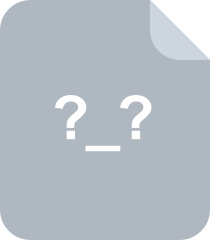
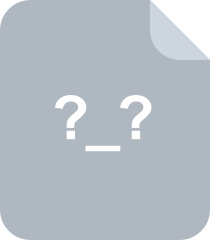
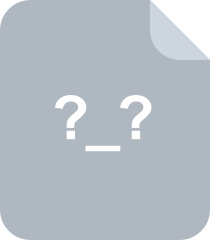
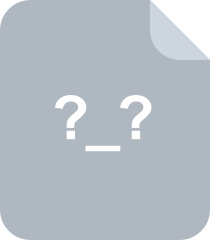
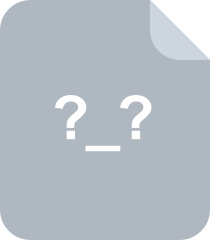
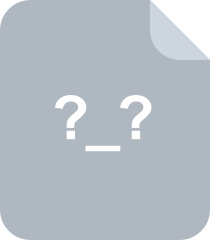
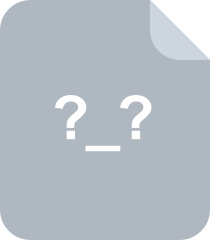
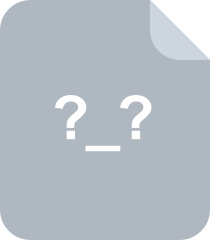
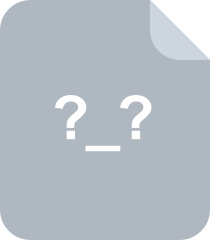
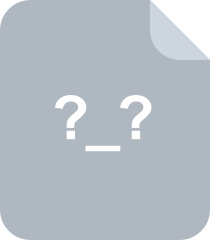
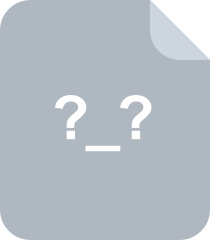
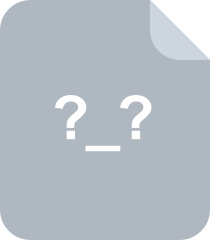
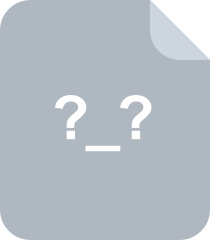
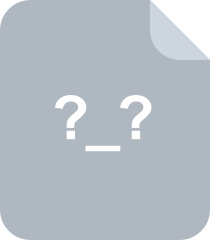
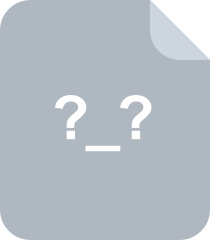
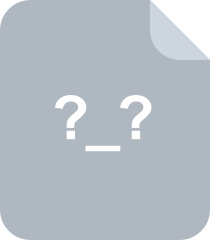
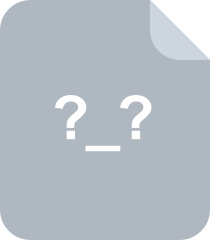
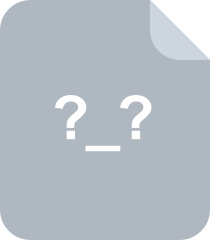
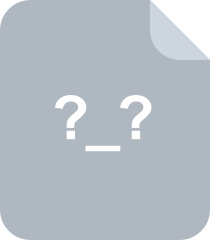
共 1855 条
- 1
- 2
- 3
- 4
- 5
- 6
- 19
资源评论
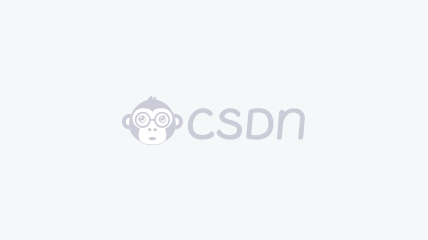

隐形的粉红色独角兽
- 粉丝: 16
- 资源: 121
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

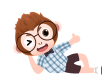
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


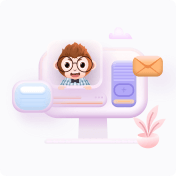
安全验证
文档复制为VIP权益,开通VIP直接复制
