/*******************************************************************************
* Copyright (c) 2000, 2005 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.custom;
import java.util.*;
import org.eclipse.swt.*;
import org.eclipse.swt.accessibility.*;
import org.eclipse.swt.dnd.*;
import org.eclipse.swt.events.*;
import org.eclipse.swt.graphics.*;
import org.eclipse.swt.internal.*;
import org.eclipse.swt.printing.*;
import org.eclipse.swt.widgets.*;
/**
* A StyledText is an editable user interface object that displays lines
* of text. The following style attributes can be defined for the text:
* <ul>
* <li>foreground color
* <li>background color
* <li>font style (bold, italic, bold-italic, regular)
* <li>underline
* <li>strikeout
* </ul>
* <p>
* In addition to text style attributes, the background color of a line may
* be specified.
* </p>
* <p>
* There are two ways to use this widget when specifying text style information.
* You may use the API that is defined for StyledText or you may define your own
* LineStyleListener. If you define your own listener, you will be responsible
* for maintaining the text style information for the widget. IMPORTANT: You may
* not define your own listener and use the StyledText API. The following
* StyledText API is not supported if you have defined a LineStyleListener:
* <ul>
* <li>getStyleRangeAtOffset(int)
* <li>getStyleRanges()
* <li>replaceStyleRanges(int,int,StyleRange[])
* <li>setStyleRange(StyleRange)
* <li>setStyleRanges(StyleRange[])
* </ul>
* </p>
* <p>
* There are two ways to use this widget when specifying line background colors.
* You may use the API that is defined for StyledText or you may define your own
* LineBackgroundListener. If you define your own listener, you will be responsible
* for maintaining the line background color information for the widget.
* IMPORTANT: You may not define your own listener and use the StyledText API.
* The following StyledText API is not supported if you have defined a
* LineBackgroundListener:
* <ul>
* <li>getLineBackground(int)
* <li>setLineBackground(int,int,Color)
* </ul>
* </p>
* <p>
* The content implementation for this widget may also be user-defined. To do so,
* you must implement the StyledTextContent interface and use the StyledText API
* setContent(StyledTextContent) to initialize the widget.
* </p>
* <p>
* IMPORTANT: This class is <em>not</em> intended to be subclassed.
* </p>
* <dl>
* <dt><b>Styles:</b><dd>FULL_SELECTION, MULTI, READ_ONLY, SINGLE, WRAP
* <dt><b>Events:</b><dd>ExtendedModify, LineGetBackground, LineGetSegments, LineGetStyle, Modify, Selection, Verify, VerifyKey
* </dl>
*/
public class StyledText extends Canvas {
static final char TAB = '\t';
static final String PlatformLineDelimiter = System.getProperty("line.separator");
static final int BIDI_CARET_WIDTH = 3;
static final int DEFAULT_WIDTH = 64;
static final int DEFAULT_HEIGHT = 64;
static final int V_SCROLL_RATE = 50;
static final int H_SCROLL_RATE = 10;
static final int ExtendedModify = 3000;
static final int LineGetBackground = 3001;
static final int LineGetStyle = 3002;
static final int TextChanging = 3003;
static final int TextSet = 3004;
static final int VerifyKey = 3005;
static final int TextChanged = 3006;
static final int LineGetSegments = 3007;
Color selectionBackground; // selection background color
Color selectionForeground; // selection foreground color
StyledTextContent logicalContent; // native content (default or user specified)
StyledTextContent content; // line wrapping content, same as logicalContent if word wrap is off
DisplayRenderer renderer;
Listener listener;
TextChangeListener textChangeListener; // listener for TextChanging, TextChanged and TextSet events from StyledTextContent
DefaultLineStyler defaultLineStyler;// used for setStyles API when no LineStyleListener is registered
LineCache lineCache;
boolean userLineStyle = false; // true=widget is using a user defined line style listener for line styles. false=widget is using the default line styler to store line styles
boolean userLineBackground = false; // true=widget is using a user defined line background listener for line backgrounds. false=widget is using the default line styler to store line backgrounds
int verticalScrollOffset = 0; // pixel based
int horizontalScrollOffset = 0; // pixel based
int topIndex = 0; // top visible line
int lastPaintTopIndex = -1;
int topOffset = 0; // offset of first character in top line
int clientAreaHeight = 0; // the client area height. Needed to calculate content width for new
// visible lines during Resize callback
int clientAreaWidth = 0; // the client area width. Needed during Resize callback to determine
// if line wrap needs to be recalculated
int lineHeight; // line height=font height
int tabLength = 4; // number of characters in a tab
int leftMargin;
int topMargin;
int rightMargin;
int bottomMargin;
Cursor ibeamCursor;
int columnX; // keep track of the horizontal caret position
// when changing lines/pages. Fixes bug 5935
int caretOffset = 0;
Point selection = new Point(0, 0); // x and y are start and end caret offsets of selection
Point clipboardSelection; // x and y are start and end caret offsets of previous selection
int selectionAnchor; // position of selection anchor. 0 based offset from beginning of text
Point doubleClickSelection; // selection after last mouse double click
boolean editable = true;
boolean wordWrap = false;
boolean doubleClickEnabled = true; // see getDoubleClickEnabled
boolean overwrite = false; // insert/overwrite edit mode
int textLimit = -1; // limits the number of characters the user can type in the widget. Unlimited by default.
Hashtable keyActionMap = new Hashtable();
Color background = null; // workaround for bug 4791
Color foreground = null; //
Clipboard clipboard;
boolean mouseDown = false;
boolean mouseDoubleClick = false; // true=a double click ocurred. Don't do mouse swipe selection.
int autoScrollDirection = SWT.NULL; // the direction of autoscrolling (up, down, right, left)
int autoScrollDistance = 0;
int lastTextChangeStart; // cache data of the
int lastTextChangeNewLineCount; // last text changing
int lastTextChangeNewCharCount; // event for use in the
int lastTextChangeReplaceLineCount; // text changed handler
int lastTextChangeReplaceCharCount;
boolean isMirrored;
boolean bidiColoring = false; // apply the BIDI algorithm on text segments of the same color
Image leftCaretBitmap = null;
Image rightCaretBitmap = null;
int caretDirection = SWT.NULL;
boolean advancing = true;
Caret defaultCaret = null;
boolean updateCaretDirection = true;
final static boolean IS_CARBON, IS_GTK, IS_MOTIF;
final static boolean DOUBLE_BUFFER;
static {
String platform = SWT.getPlatform();
IS_CARBON = "carbon".equals(platform);
IS_GTK = "gtk".equals(platform);
IS_MOTIF = "motif".equals(platform);
DOUBLE_BUFFER = !IS_CARBON;
}
/**
* The Printing class implements printing of a range of text.
* An instance of <class>Printing </class> is returned in the
* StyledText#print(Printer) API. The run() method may be
* invoked from any thread.
*/
static class Printing implements Runnable {
final static int LEFT = 0; // left aligned header/footer segment
final static int CENTER = 1; // centered header/footer segment
final static int RIGHT = 2; // right aligned he
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
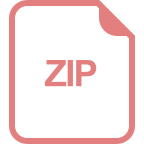
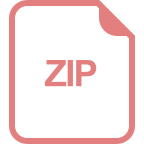
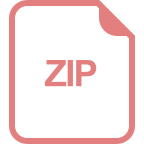
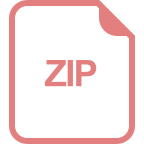
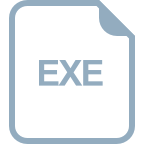
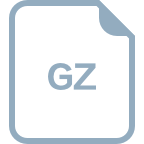
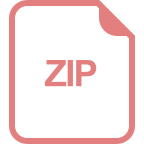
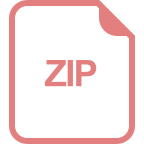
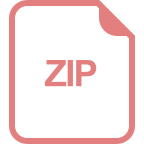
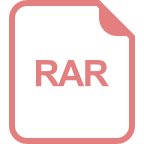
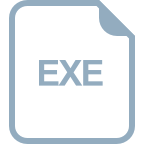
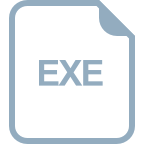
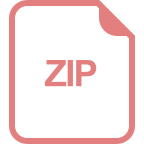
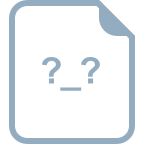
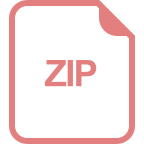
收起资源包目录

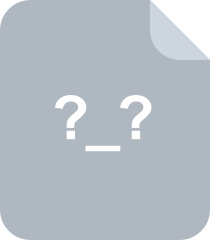
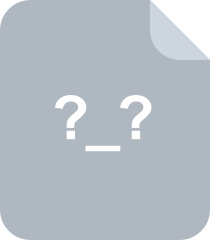
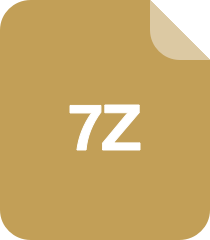
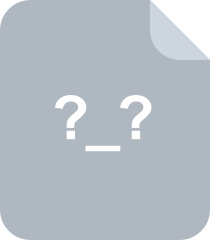
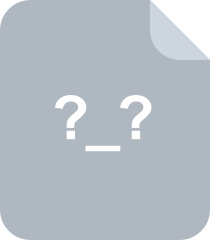
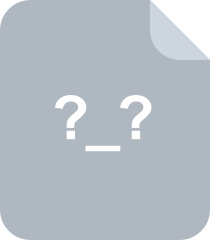
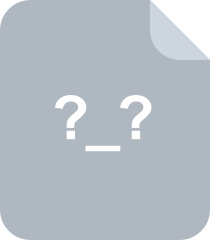
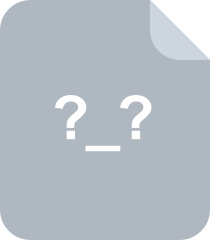
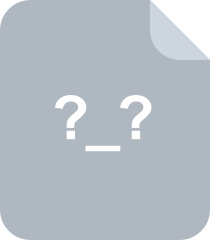
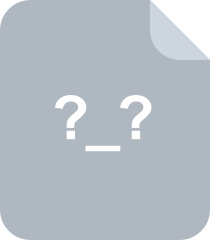
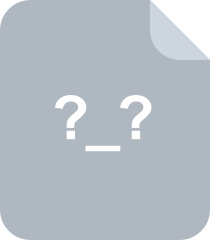
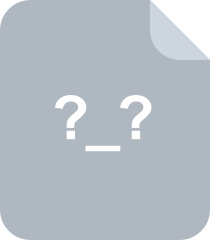
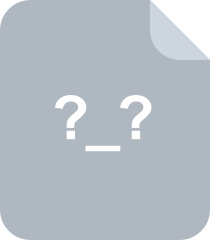
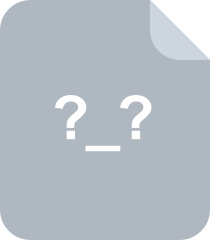
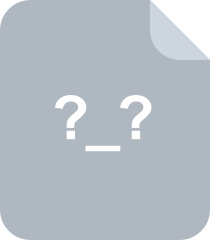
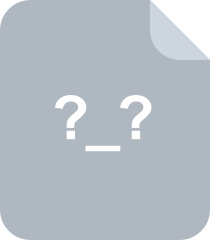
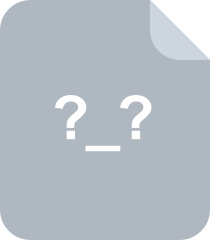
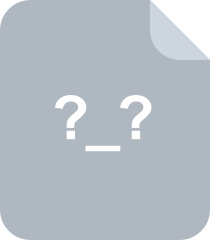
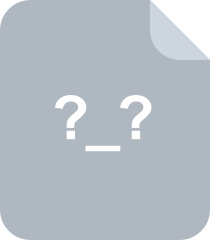
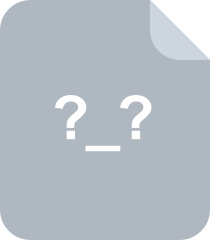
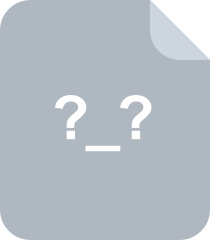
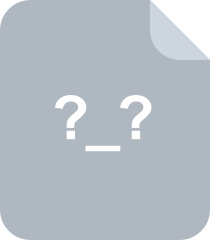
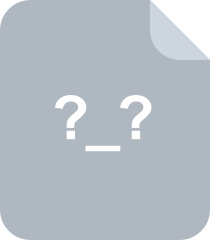
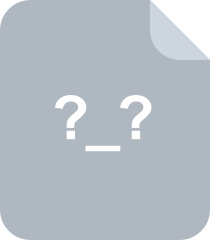
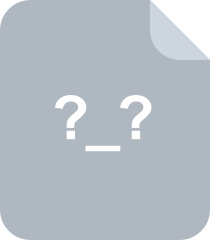
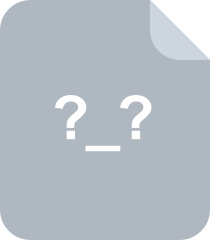
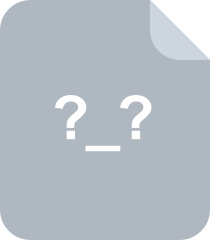
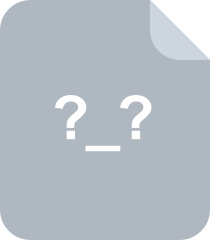
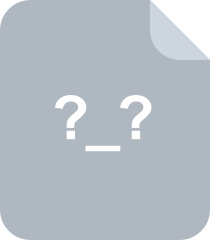
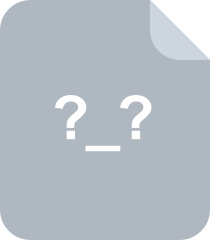
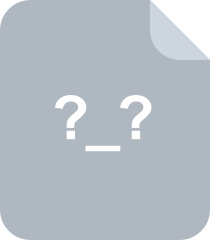
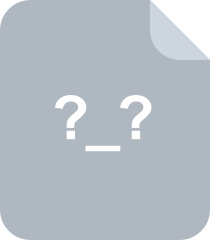
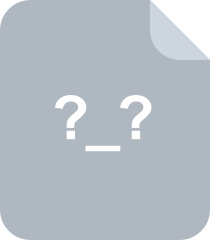
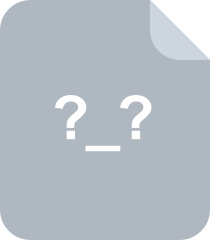
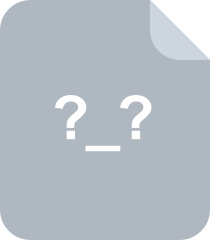
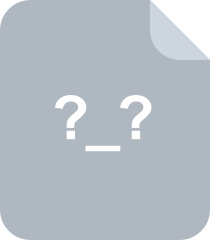
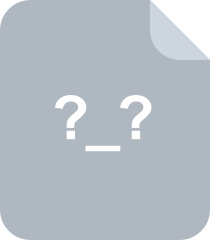
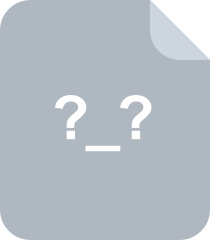
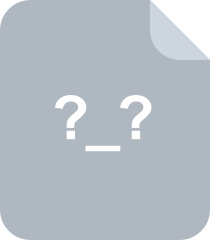
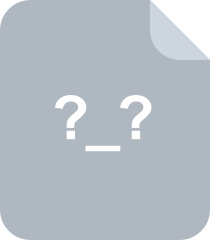
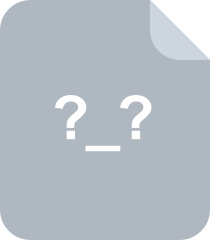
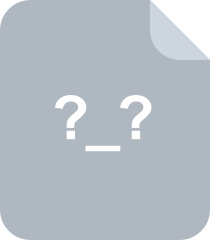
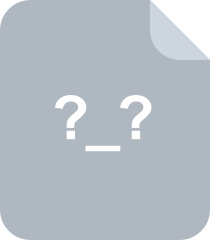
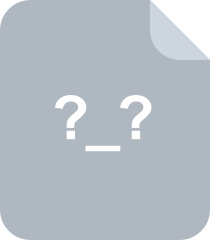
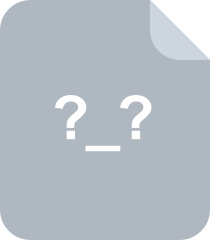
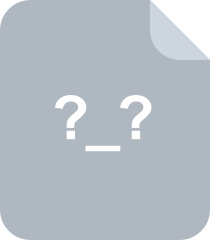
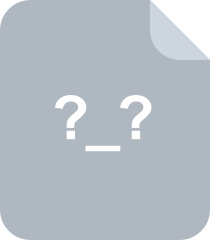
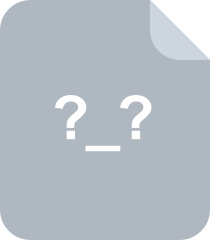
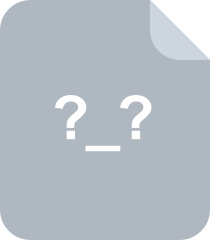
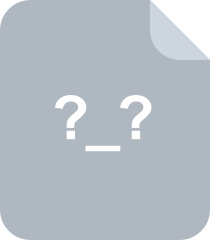
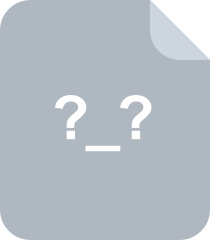
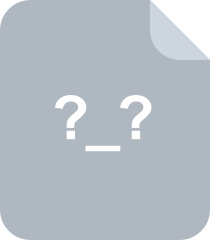
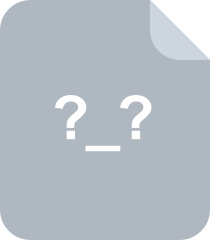
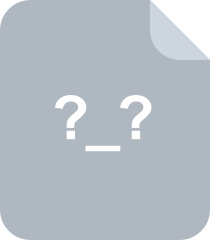
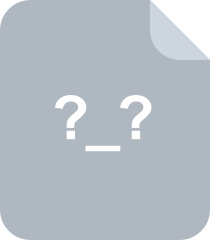
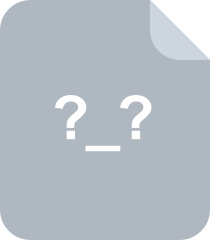
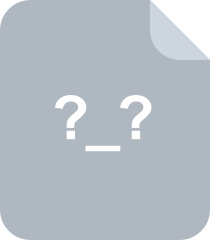
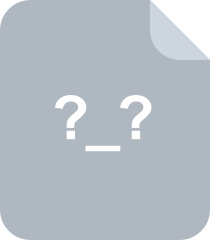
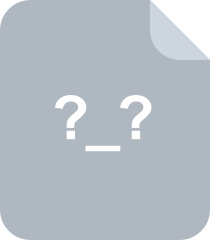
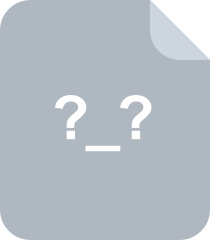
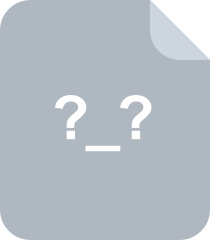
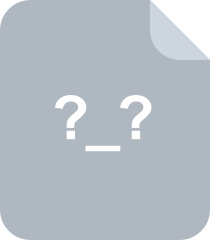
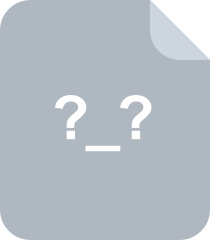
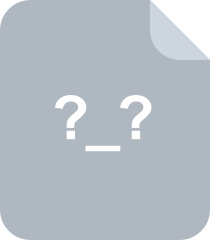
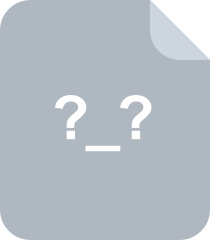
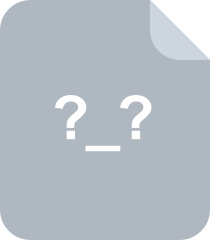
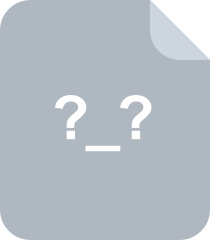
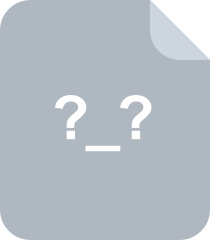
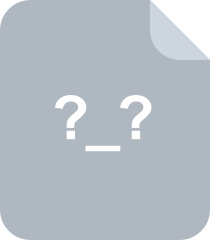
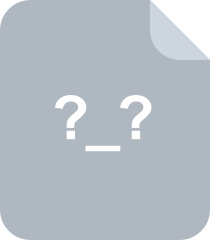
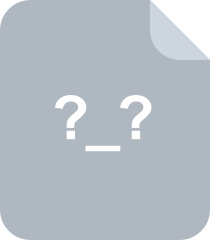
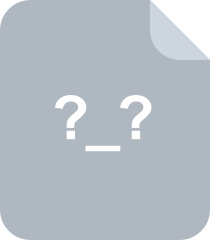
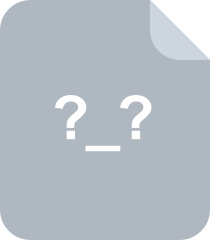
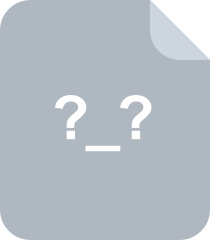
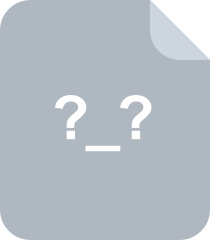
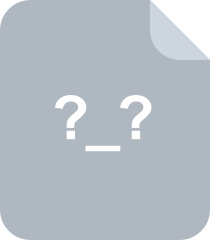
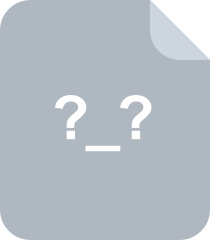
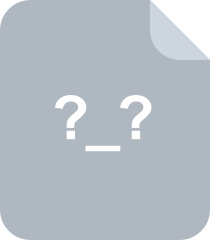
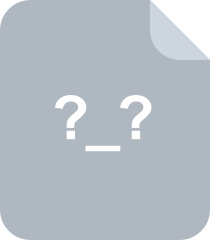
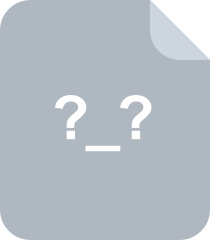
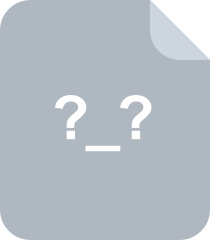
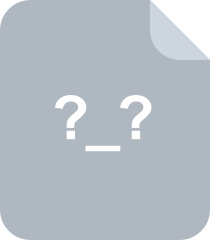
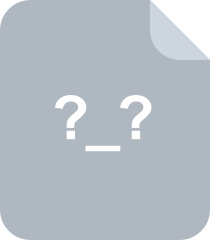
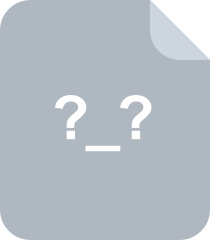
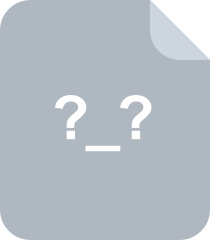
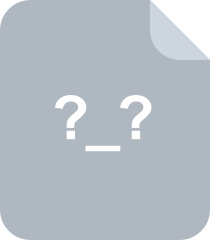
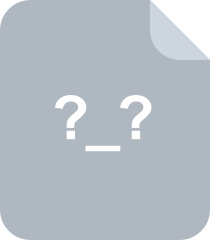
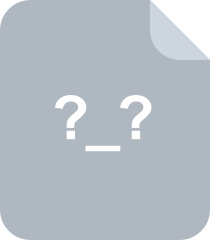
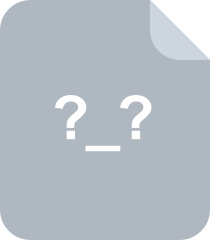
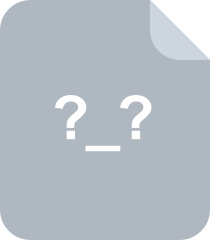
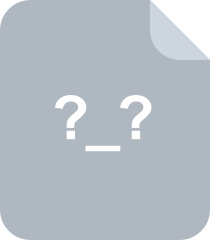
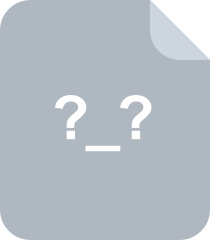
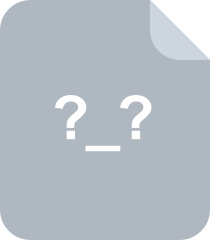
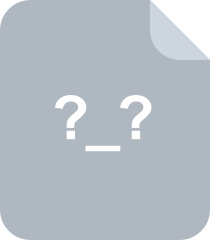
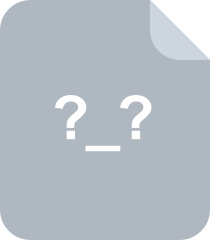
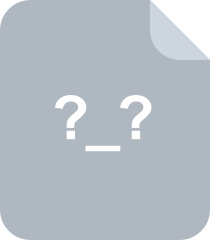
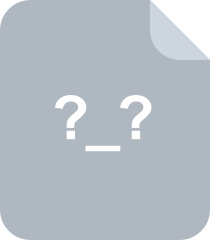
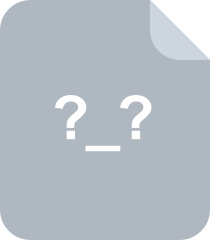
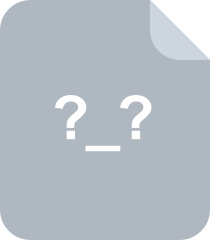
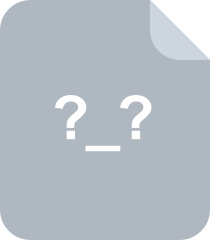
共 1425 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
资源评论
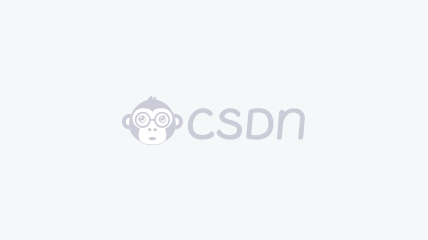

隐形的粉红色独角兽
- 粉丝: 16
- 资源: 121
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

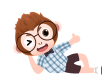
安全验证
文档复制为VIP权益,开通VIP直接复制
