[](http://travis-ci.org/kriskowal/q)
<a href="http://promises-aplus.github.com/promises-spec">
<img src="http://promises-aplus.github.com/promises-spec/assets/logo-small.png"
align="right" alt="Promises/A+ logo" />
</a>
If a function cannot return a value or throw an exception without
blocking, it can return a promise instead. A promise is an object
that represents the return value or the thrown exception that the
function may eventually provide. A promise can also be used as a
proxy for a [remote object][Q-Connection] to overcome latency.
[Q-Connection]: https://github.com/kriskowal/q-connection
On the first pass, promises can mitigate the “[Pyramid of
Doom][POD]”: the situation where code marches to the right faster
than it marches forward.
[POD]: http://calculist.org/blog/2011/12/14/why-coroutines-wont-work-on-the-web/
```javascript
step1(function (value1) {
step2(value1, function(value2) {
step3(value2, function(value3) {
step4(value3, function(value4) {
// Do something with value4
});
});
});
});
```
With a promise library, you can flatten the pyramid.
```javascript
Q.fcall(promisedStep1)
.then(promisedStep2)
.then(promisedStep3)
.then(promisedStep4)
.then(function (value4) {
// Do something with value4
})
.catch(function (error) {
// Handle any error from all above steps
})
.done();
```
With this approach, you also get implicit error propagation, just like `try`,
`catch`, and `finally`. An error in `promisedStep1` will flow all the way to
the `catch` function, where it’s caught and handled. (Here `promisedStepN` is
a version of `stepN` that returns a promise.)
The callback approach is called an “inversion of control”.
A function that accepts a callback instead of a return value
is saying, “Don’t call me, I’ll call you.”. Promises
[un-invert][IOC] the inversion, cleanly separating the input
arguments from control flow arguments. This simplifies the
use and creation of API’s, particularly variadic,
rest and spread arguments.
[IOC]: http://www.slideshare.net/domenicdenicola/callbacks-promises-and-coroutines-oh-my-the-evolution-of-asynchronicity-in-javascript
## Getting Started
The Q module can be loaded as:
- A ``<script>`` tag (creating a ``Q`` global variable): ~2.5 KB minified and
gzipped.
- A Node.js and CommonJS module, available in [npm](https://npmjs.org/) as
the [q](https://npmjs.org/package/q) package
- An AMD module
- A [component](https://github.com/component/component) as ``microjs/q``
- Using [bower](http://bower.io/) as ``q``
- Using [NuGet](http://nuget.org/) as [Q](https://nuget.org/packages/q)
Q can exchange promises with jQuery, Dojo, When.js, WinJS, and more.
## Resources
Our [wiki][] contains a number of useful resources, including:
- A method-by-method [Q API reference][reference].
- A growing [examples gallery][examples], showing how Q can be used to make
everything better. From XHR to database access to accessing the Flickr API,
Q is there for you.
- There are many libraries that produce and consume Q promises for everything
from file system/database access or RPC to templating. For a list of some of
the more popular ones, see [Libraries][].
- If you want materials that introduce the promise concept generally, and the
below tutorial isn't doing it for you, check out our collection of
[presentations, blog posts, and podcasts][resources].
- A guide for those [coming from jQuery's `$.Deferred`][jquery].
We'd also love to have you join the Q-Continuum [mailing list][].
[wiki]: https://github.com/kriskowal/q/wiki
[reference]: https://github.com/kriskowal/q/wiki/API-Reference
[examples]: https://github.com/kriskowal/q/wiki/Examples-Gallery
[Libraries]: https://github.com/kriskowal/q/wiki/Libraries
[resources]: https://github.com/kriskowal/q/wiki/General-Promise-Resources
[jquery]: https://github.com/kriskowal/q/wiki/Coming-from-jQuery
[mailing list]: https://groups.google.com/forum/#!forum/q-continuum
## Tutorial
Promises have a ``then`` method, which you can use to get the eventual
return value (fulfillment) or thrown exception (rejection).
```javascript
promiseMeSomething()
.then(function (value) {
}, function (reason) {
});
```
If ``promiseMeSomething`` returns a promise that gets fulfilled later
with a return value, the first function (the fulfillment handler) will be
called with the value. However, if the ``promiseMeSomething`` function
gets rejected later by a thrown exception, the second function (the
rejection handler) will be called with the exception.
Note that resolution of a promise is always asynchronous: that is, the
fulfillment or rejection handler will always be called in the next turn of the
event loop (i.e. `process.nextTick` in Node). This gives you a nice
guarantee when mentally tracing the flow of your code, namely that
``then`` will always return before either handler is executed.
In this tutorial, we begin with how to consume and work with promises. We'll
talk about how to create them, and thus create functions like
`promiseMeSomething` that return promises, [below](#the-beginning).
### Propagation
The ``then`` method returns a promise, which in this example, I’m
assigning to ``outputPromise``.
```javascript
var outputPromise = getInputPromise()
.then(function (input) {
}, function (reason) {
});
```
The ``outputPromise`` variable becomes a new promise for the return
value of either handler. Since a function can only either return a
value or throw an exception, only one handler will ever be called and it
will be responsible for resolving ``outputPromise``.
- If you return a value in a handler, ``outputPromise`` will get
fulfilled.
- If you throw an exception in a handler, ``outputPromise`` will get
rejected.
- If you return a **promise** in a handler, ``outputPromise`` will
“become” that promise. Being able to become a new promise is useful
for managing delays, combining results, or recovering from errors.
If the ``getInputPromise()`` promise gets rejected and you omit the
rejection handler, the **error** will go to ``outputPromise``:
```javascript
var outputPromise = getInputPromise()
.then(function (value) {
});
```
If the input promise gets fulfilled and you omit the fulfillment handler, the
**value** will go to ``outputPromise``:
```javascript
var outputPromise = getInputPromise()
.then(null, function (error) {
});
```
Q promises provide a ``fail`` shorthand for ``then`` when you are only
interested in handling the error:
```javascript
var outputPromise = getInputPromise()
.fail(function (error) {
});
```
If you are writing JavaScript for modern engines only or using
CoffeeScript, you may use `catch` instead of `fail`.
Promises also have a ``fin`` function that is like a ``finally`` clause.
The final handler gets called, with no arguments, when the promise
returned by ``getInputPromise()`` either returns a value or throws an
error. The value returned or error thrown by ``getInputPromise()``
passes directly to ``outputPromise`` unless the final handler fails, and
may be delayed if the final handler returns a promise.
```javascript
var outputPromise = getInputPromise()
.fin(function () {
// close files, database connections, stop servers, conclude tests
});
```
- If the handler returns a value, the value is ignored
- If the handler throws an error, the error passes to ``outputPromise``
- If the handler returns a promise, ``outputPromise`` gets postponed. The
eventual value or error has the same effect as an immediate return
value or thrown error: a value would be ignored, an error would be
forwarded.
If you are writing JavaScript for modern engines only or using
CoffeeScript, you may use `finally` instead of `fin`.
### Chaining
There are two ways to chain promises. You can chain promises
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
phonegap相机android 此篇文章以cordova 3.3版本编写 据我总结核心步骤: 创建工程 ; 下载插件 ;编译工程; 调用插件; 按照如下步骤就能生产出代码: 打开cmd 控制台 1 使用命令行 建立phonegap工程 2 进入到phonegap工程下的plugins 文件夹当中 ,使用命令行下载官方插件 ;(输入命令: cordova plugin add org.apache.cordova.camera) 4 添加生成 android或者IOS 工程 ;android命令如下cordova platform add android (注:oc的把android换成ios) 5 在命令行输入cordova build 6 最后 将生成的工程导入编辑器(我用的eclipse) 在assets/www目录下在index.html中添加 javascript 调用语句
资源推荐
资源详情
资源评论
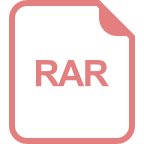
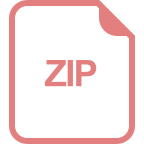
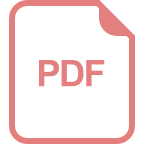
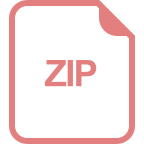
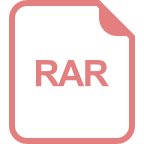
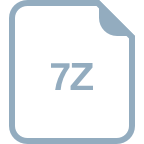
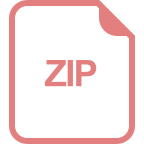
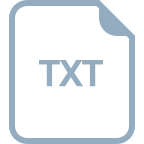
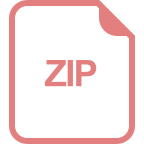
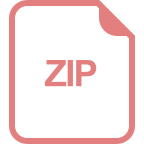
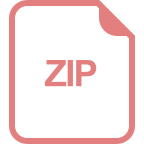
收起资源包目录

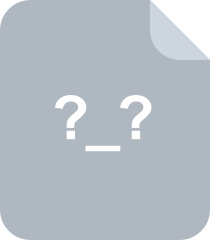
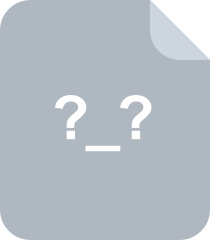
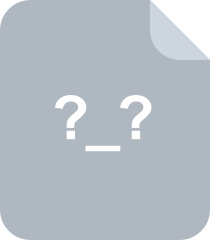
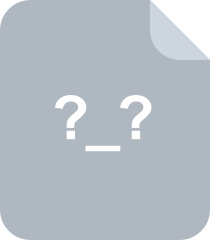
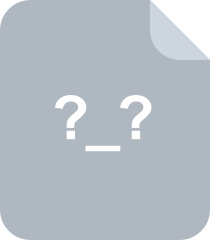
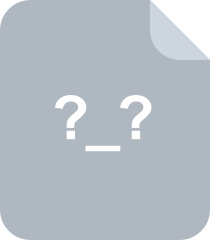
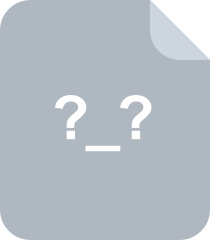
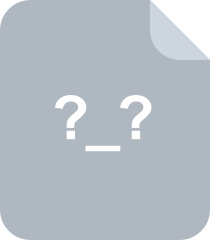
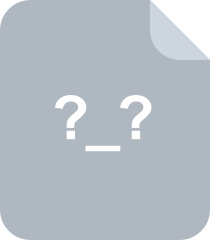
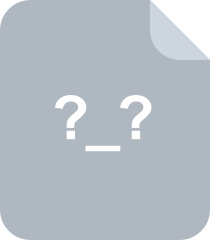
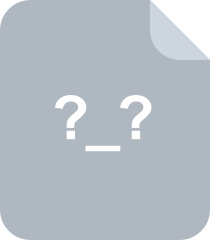
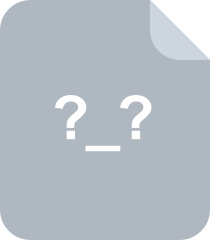
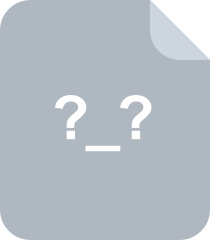
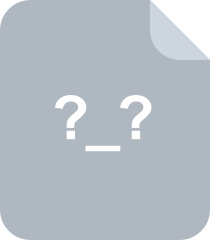
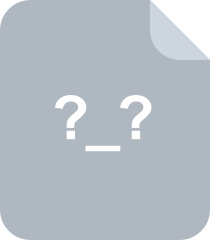
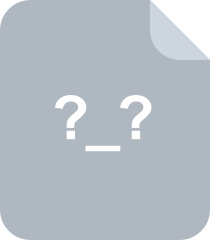
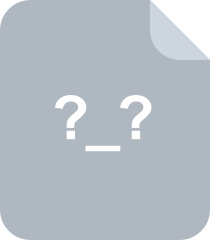
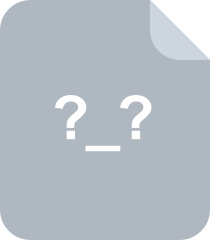
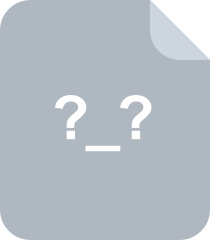
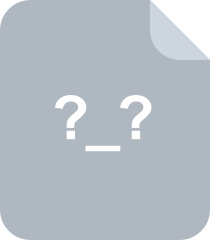
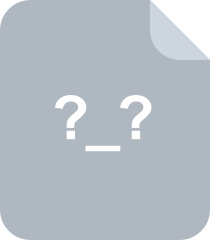
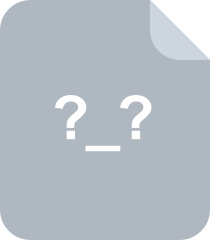
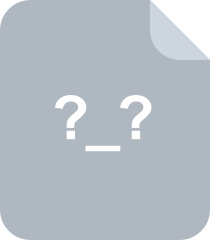
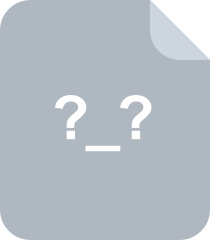
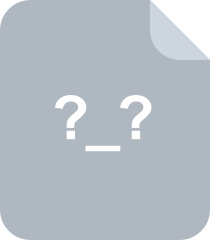
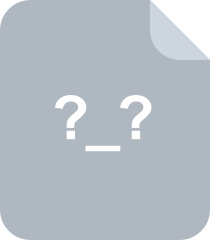
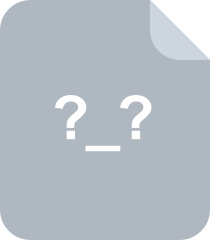
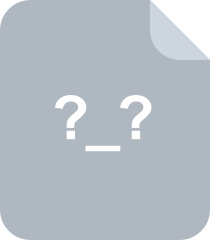
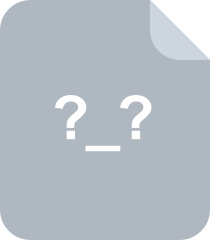
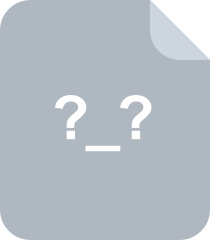
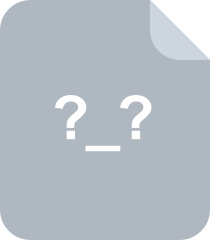
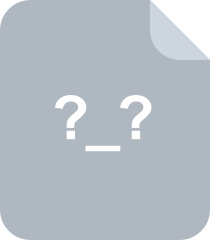
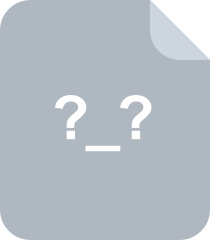
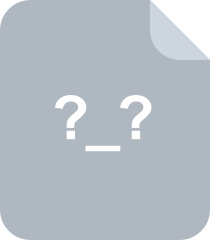
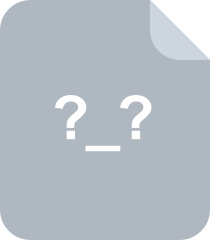
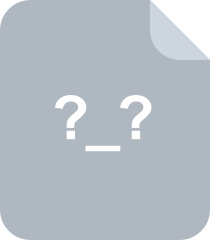
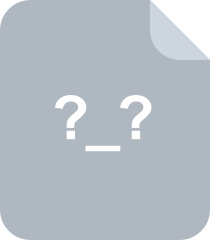
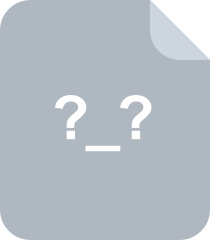
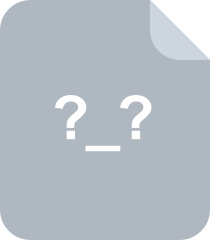
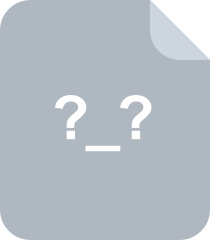
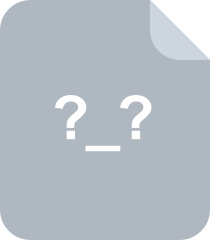
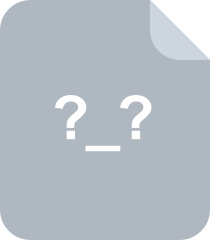
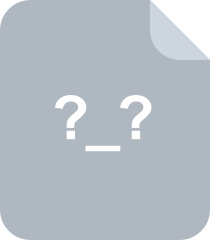
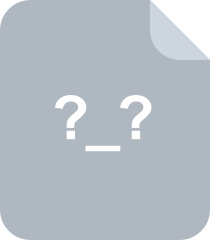
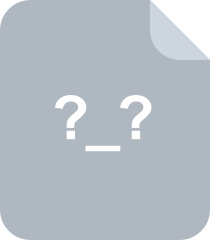
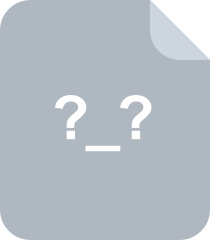
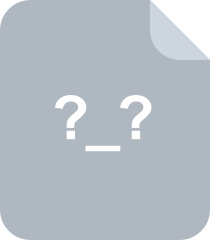
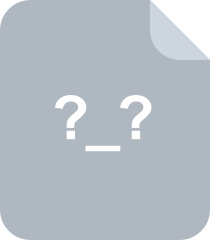
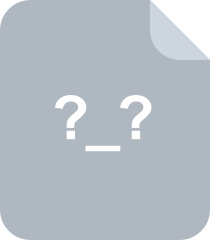
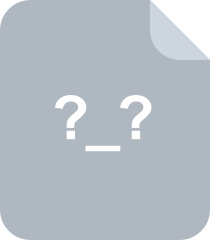
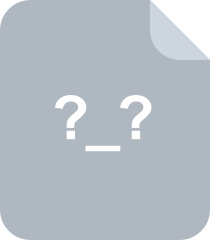
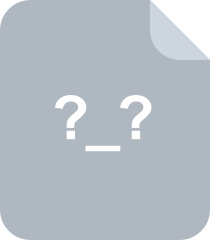
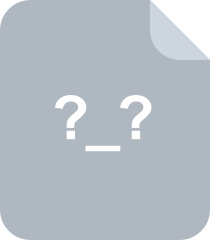
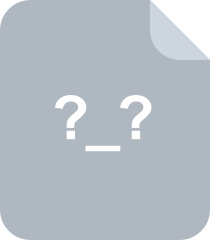
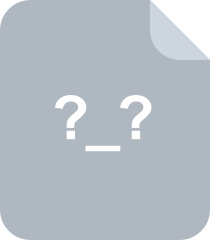
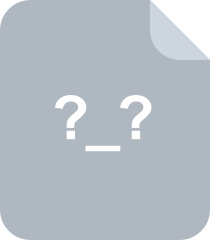
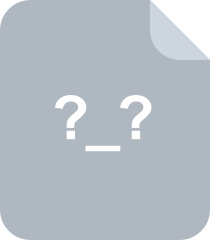
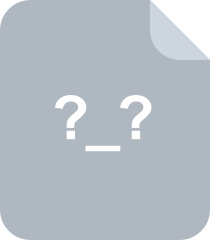
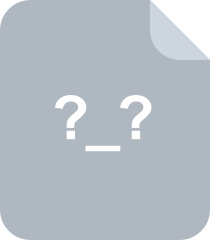
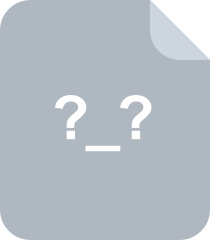
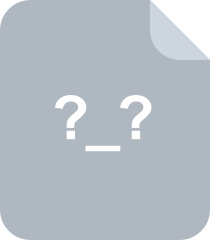
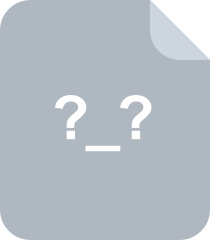
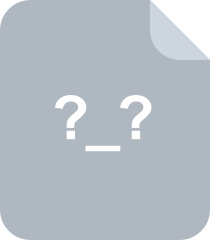
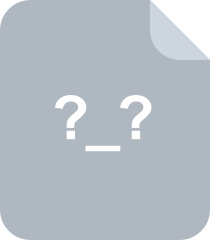
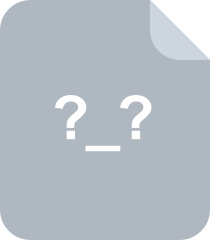
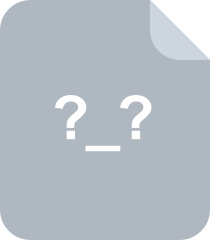
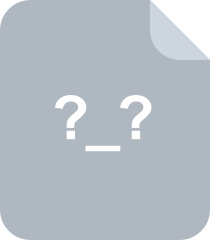
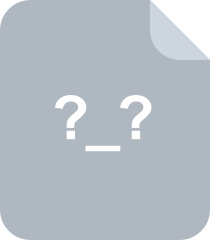
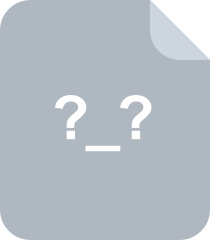
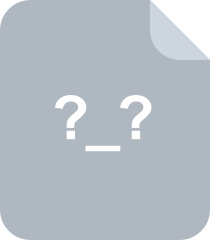
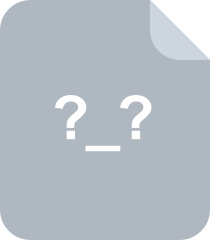
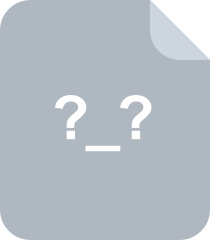
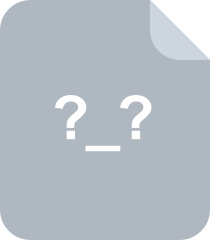
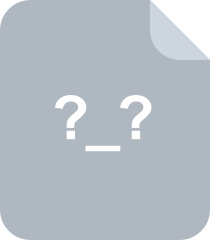
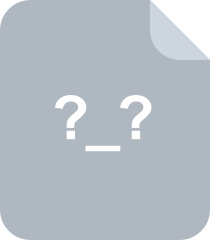
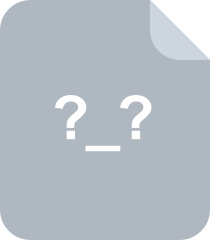
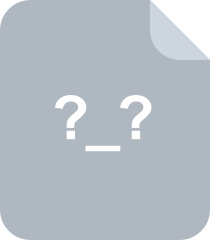
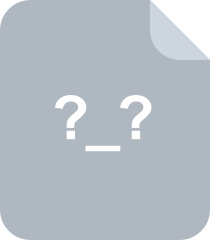
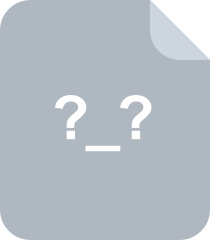
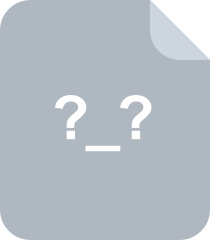
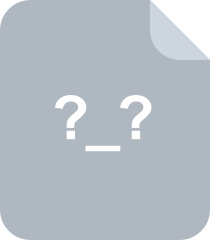
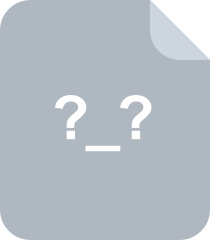
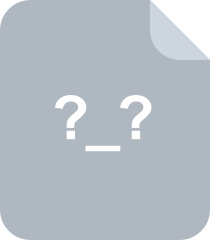
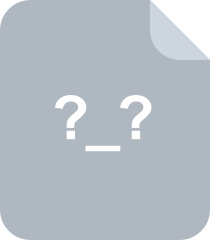
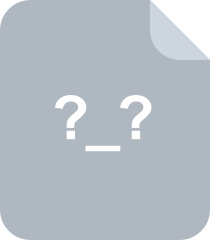
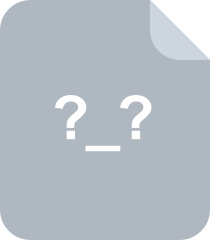
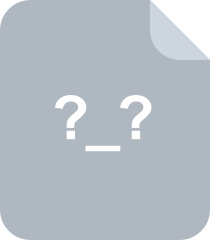
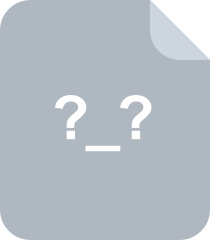
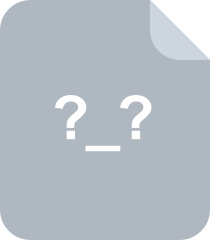
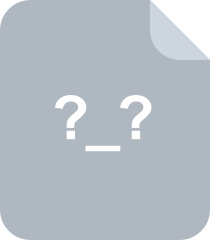
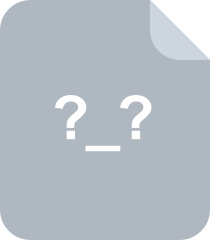
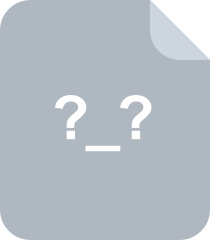
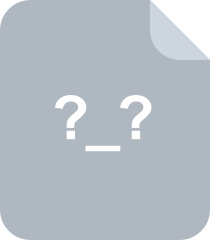
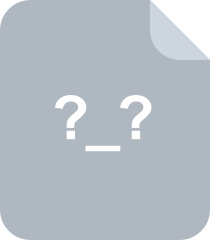
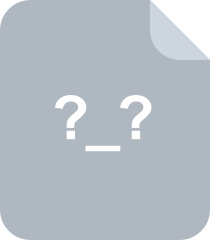
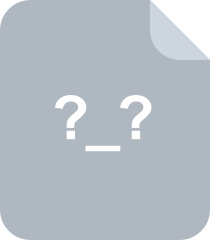
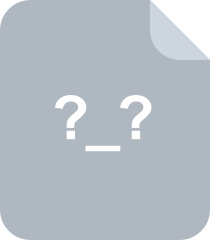
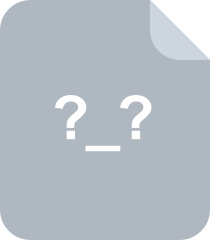
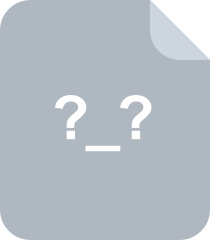
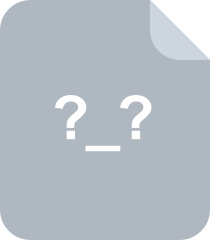
共 545 条
- 1
- 2
- 3
- 4
- 5
- 6

aaawqqq
- 粉丝: 533
- 资源: 21
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

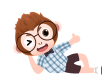
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页