/******************************************************
* EasyX Library for C++ (Ver:20240601)
* https://easyx.cn
*
* EasyX.h
* Provides the latest API.
******************************************************/
#pragma once
#ifndef WINVER
#define WINVER 0x0400 // Specifies that the minimum required platform is Windows 95 and Windows NT 4.0.
#endif
#ifndef _WIN32_WINNT
#define _WIN32_WINNT 0x0500 // Specifies that the minimum required platform is Windows 2000
#endif
#ifndef _WIN32_WINDOWS
#define _WIN32_WINDOWS 0x0410 // Specifies that the minimum required platform is Windows 98
#endif
#ifdef UNICODE
#pragma comment(lib,"EasyXw.lib")
#else
#pragma comment(lib,"EasyXa.lib")
#endif
#ifndef __cplusplus
#error EasyX is only for C++
#endif
#include <windows.h>
#include <tchar.h>
// EasyX Window Properties
#define EX_SHOWCONSOLE 1 // Maintain the console window when creating a graphics window
#define EX_NOCLOSE 2 // Disable the close button
#define EX_NOMINIMIZE 4 // Disable the minimize button
#define EX_DBLCLKS 8 // Support double-click events
// Color constant
#define BLACK 0
#define BLUE 0xAA0000
#define GREEN 0x00AA00
#define CYAN 0xAAAA00
#define RED 0x0000AA
#define MAGENTA 0xAA00AA
#define BROWN 0x0055AA
#define LIGHTGRAY 0xAAAAAA
#define DARKGRAY 0x555555
#define LIGHTBLUE 0xFF5555
#define LIGHTGREEN 0x55FF55
#define LIGHTCYAN 0xFFFF55
#define LIGHTRED 0x5555FF
#define LIGHTMAGENTA 0xFF55FF
#define YELLOW 0x55FFFF
#define WHITE 0xFFFFFF
// Color conversion macro
#define BGR(color) ( (((color) & 0xFF) << 16) | ((color) & 0xFF00FF00) | (((color) & 0xFF0000) >> 16) )
class IMAGE;
// Line style class
class LINESTYLE
{
public:
LINESTYLE();
LINESTYLE(const LINESTYLE &style);
LINESTYLE& operator = (const LINESTYLE &style);
virtual ~LINESTYLE();
DWORD style;
DWORD thickness;
DWORD *puserstyle;
DWORD userstylecount;
};
// Fill style class
class FILLSTYLE
{
public:
FILLSTYLE();
FILLSTYLE(const FILLSTYLE &style);
FILLSTYLE& operator = (const FILLSTYLE &style);
virtual ~FILLSTYLE();
int style; // Fill style
long hatch; // Hatch pattern
IMAGE* ppattern; // Fill image
};
// Image class
class IMAGE
{
public:
int getwidth() const; // Get the width of the image
int getheight() const; // Get the height of the image
private:
int width, height; // Width and height of the image
HBITMAP m_hBmp;
HDC m_hMemDC;
float m_data[6];
COLORREF m_LineColor; // Current line color
COLORREF m_FillColor; // Current fill color
COLORREF m_TextColor; // Current text color
COLORREF m_BkColor; // Current background color
DWORD* m_pBuffer; // Memory buffer of the image
LINESTYLE m_LineStyle; // Current line style
FILLSTYLE m_FillStyle; // Current fill style
virtual void SetDefault(); // Set the graphics environment as default
public:
IMAGE(int _width = 0, int _height = 0);
IMAGE(const IMAGE &img);
IMAGE& operator = (const IMAGE &img);
virtual ~IMAGE();
virtual void Resize(int _width, int _height); // Resize image
};
// Graphics window related functions
HWND initgraph(int width, int height, int flag = 0); // Create graphics window
void closegraph(); // Close graphics window
// Graphics environment related functions
void cleardevice(); // Clear device
void setcliprgn(HRGN hrgn); // Set clip region
void clearcliprgn(); // Clear clip region
void getlinestyle(LINESTYLE* pstyle); // Get line style
void setlinestyle(const LINESTYLE* pstyle); // Set line style
void setlinestyle(int style, int thickness = 1, const DWORD *puserstyle = NULL, DWORD userstylecount = 0); // Set line style
void getfillstyle(FILLSTYLE* pstyle); // Get fill style
void setfillstyle(const FILLSTYLE* pstyle); // Set fill style
void setfillstyle(int style, long hatch = NULL, IMAGE* ppattern = NULL); // Set fill style
void setfillstyle(BYTE* ppattern8x8); // Set fill style
void setorigin(int x, int y); // Set coordinate origin
void getaspectratio(float *pxasp, float *pyasp); // Get aspect ratio
void setaspectratio(float xasp, float yasp); // Set aspect ratio
int getrop2(); // Get binary raster operation mode
void setrop2(int mode); // Set binary raster operation mode
int getpolyfillmode(); // Get polygon fill mode
void setpolyfillmode(int mode); // Set polygon fill mode
void graphdefaults(); // Reset the graphics environment as default
COLORREF getlinecolor(); // Get line color
void setlinecolor(COLORREF color); // Set line color
COLORREF gettextcolor(); // Get text color
void settextcolor(COLORREF color); // Set text color
COLORREF getfillcolor(); // Get fill color
void setfillcolor(COLORREF color); // Set fill color
COLORREF getbkcolor(); // Get background color
void setbkcolor(COLORREF color); // Set background color
int getbkmode(); // Get background mode
void setbkmode(int mode); // Set background mode
// Color model transformation related functions
COLORREF RGBtoGRAY(COLORREF rgb);
void RGBtoHSL(COLORREF rgb, float *H, float *S, float *L);
void RGBtoHSV(COLORREF rgb, float *H, float *S, float *V);
COLORREF HSLtoRGB(float H, float S, float L);
COLORREF HSVtoRGB(float H, float S, float V);
// Drawing related functions
COLORREF getpixel(int x, int y); // Get pixel color
void putpixel(int x, int y, COLORREF color); // Set pixel color
void line(int x1, int y1, int x2, int y2); // Draw a line
void rectangle (int left, int top, int right, int bottom); // Draw a rectangle without filling
void fillrectangle (int left, int top, int right, int bottom); // Draw a filled rectangle with a border
void solidrectangle(int left, int top, int right, int bottom); // Draw a filled rectangle without a border
void clearrectangle(int left, int top, int right, int bottom); // Clear a rectangular region
void circle (int x, int y, int radius); // Draw a circle without filling
void fillcircle (int x, int y, int radius); // Draw a filled circle with a border
void solidcircle(int x, int y, int radius); // Draw a filled circle without a border
void clearcircle(int x, int y, int radius); // Clear a circular region
void ellipse (int left, int top, int right, int bottom); // Draw an ellipse without filling
void fillellipse (int left, int top, int right, int bottom); // Draw a filled ellipse with a border
void solidellipse(int left, int top, int right, int bottom); // Draw a filled ellipse without a border
void clearellipse(int left, int top, int right, int bottom); // Clear an elliptical region
void roundrect (int left, int top, int right, int bottom, int ellipsewidth, int ellipseheight); // Draw a rounded rectangle without filling
void fillroundrect (int left, int top, int right, int bottom, int ellipsewidth, int ellipseheight); // Draw a filled rounded rectangle with a border
void solidroundrect(int left, int top, int right, int bottom, int ellipsewidth, int ellipseheight); // Draw a filled rounded rectangle without a border
void clearroundrect(int left, int top, int right, int bottom, int ellipsewidth, int ellipseheight); // Clear a rounded rectangular region
void arc (int left, int top, int right, int bottom, double stangle, double endangle); // Draw an arc
void pie (int left, int top, int right, int bottom, double stangle, double endangle); // Draw a sector without filling
void fillpie (int left, int top, int right, int bottom, double stangle, double endangle); // Draw a filled sector with a border
void solidpie(int left, int top, int right, int bottom, double stangle, double endangle); // Draw a filled sector without a border
void clearpie(int left, int top, int right, int bottom, double stangle, double endangle); // Clear a rounded rectangular region
void

a789156
- 粉丝: 8
- 资源: 1
最新资源
- Quantitative Research learning plan part1
- 声学振动分析:三倍频程与三分之一倍频程Matlab代码及Z振级衰减关系一键操作全数据保存方案,环境振动分析工具:一键操作批量输出1/3倍频程与最大Z振级数据并保存至指定文件夹,1 3倍频程代码 三分之
- 《DeepSeek:从入门到精通》
- 校园管理系统源码.zip
- fastlio2+gps+loopclosure
- 北京市1175个应急避难场所-所属区县+街道+名称类别+地址+邮编+联系方式
- 光伏MPPT仿真中灰狼算法与扰动观察法相结合的技术优化与应用分析,光伏MPPT仿真:灰狼算法与扰动观察法的结合及其在阴影变化下的应用策略,光伏MPPT仿真-灰狼算法结合扰动观察法,变阴影 ,光伏MPP
- 基于51单片机的直流电机PID调速系统设计与实现:Protues与Keil仿真测试,独立按键控制及数码管速度显示,基于Protues和Keil仿真的直流电机PID调速系统设计与实现:功能包括目标速度设
- 基于交通流数据优化预测算法的Matlab例程及其详细说明文档,交通流量预测代码与说明文档:Matlab例程的实践与应用,交通流量预测代码,含说明文档,Matlab例程 ,交通流量预测; 代码; 说明文
- (源码)基于Python的加密通信综合系统.zip
- 深入探讨COOFDM技术的Matlab仿真:文档代码解析与理论解释的融合研究,CO-OFDM技术的Matlab仿真实践:从文档代码到理论解释的全面解析,COOFDM的Matlab仿真包括文档代码解释和
- 小游戏中心,附加计算器 (免费)
- (源码)基于Chrome插件的Todo神奇助手.zip
- 工具变量-数据资产信息披露水平数据集(2000-2023年).xlsx
- IMG_7290.PNG
- 利用Simulink技术进行光伏电源与控制系统仿真:国家创新设计大赛项目三电平NPC整流器设计与实现附详细文档程序,Simulink在光伏电源仿真及控制中的应用:MPPT控制、光伏逆变控制与三电平NP
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


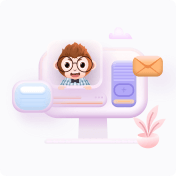