/*
* Copyright (c) Meta Platforms, Inc. and affiliates.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
#import <XCTest/XCTest.h>
#import "OCMock/OCMock.h"
#import <React/RCTNativeAnimatedNodesManager.h>
#import <React/RCTUIManager.h>
#import <React/RCTValueAnimatedNode.h>
static const NSTimeInterval FRAME_LENGTH = 1.0 / 60.0;
@interface RCTFakeDisplayLink : CADisplayLink
@end
@implementation RCTFakeDisplayLink {
NSTimeInterval _timestamp;
}
- (instancetype)init
{
self = [super init];
if (self) {
_timestamp = 1124.1234143251; // Random
}
return self;
}
- (NSTimeInterval)timestamp
{
_timestamp += FRAME_LENGTH;
return _timestamp;
}
@end
@interface RCTFakeValueObserver : NSObject <RCTValueAnimatedNodeObserver>
@property (nonatomic, strong) NSMutableArray<NSNumber *> *calls;
@end
@implementation RCTFakeValueObserver
- (instancetype)init
{
self = [super init];
if (self) {
_calls = [NSMutableArray new];
}
return self;
}
- (void)animatedNode:(__unused RCTValueAnimatedNode *)node didUpdateValue:(CGFloat)value
{
[_calls addObject:@(value)];
}
@end
@interface RCTFakeEvent : NSObject <RCTEvent>
@end
@implementation RCTFakeEvent {
NSArray *_arguments;
}
@synthesize eventName = _eventName;
@synthesize viewTag = _viewTag;
@synthesize coalescingKey = _coalescingKey;
- (instancetype)initWithName:(NSString *)name viewTag:(NSNumber *)viewTag arguments:(NSArray *)arguments
{
self = [super init];
if (self) {
_eventName = name;
_viewTag = viewTag;
_arguments = arguments;
}
return self;
}
- (NSArray *)arguments
{
return _arguments;
}
RCT_NOT_IMPLEMENTED(+(NSString *)moduleDotMethod);
RCT_NOT_IMPLEMENTED(-(BOOL)canCoalesce);
RCT_NOT_IMPLEMENTED(-(id<RCTEvent>)coalesceWithEvent : (id<RCTEvent>)newEvent);
@end
static id RCTPropChecker(NSString *prop, NSNumber *value)
{
return [OCMArg checkWithBlock:^BOOL(NSDictionary<NSString *, NSNumber *> *props) {
BOOL match = fabs(props[prop].doubleValue - value.doubleValue) < FLT_EPSILON;
if (!match) {
NSLog(@"Props `%@` with value `%@` is not close to `%@`", prop, props[prop], value);
}
return match;
}];
}
@interface RCTNativeAnimatedNodesManagerTests : XCTestCase
@end
@implementation RCTNativeAnimatedNodesManagerTests {
id _uiManager;
RCTNativeAnimatedNodesManager *_nodesManager;
RCTFakeDisplayLink *_displayLink;
}
- (void)setUp
{
[super setUp];
RCTBridge *bridge = [OCMockObject niceMockForClass:[RCTBridge class]];
_uiManager = [OCMockObject niceMockForClass:[RCTUIManager class]];
OCMStub([bridge uiManager]).andReturn(_uiManager);
_nodesManager = [[RCTNativeAnimatedNodesManager alloc] initWithBridge:bridge
surfacePresenter:bridge.surfacePresenter];
_displayLink = [RCTFakeDisplayLink new];
}
/**
* Generates a simple animated nodes graph and attaches the props node to a given viewTag
* Parameter opacity is used as a initial value for the "opacity" attribute.
*
* Nodes are connected as follows (nodes IDs in parens):
* ValueNode(1) -> StyleNode(3) -> PropNode(5)
*/
- (void)createSimpleAnimatedView:(NSNumber *)viewTag withOpacity:(CGFloat)opacity
{
[_nodesManager createAnimatedNode:@101 config:@{@"type" : @"value", @"value" : @(opacity), @"offset" : @0}];
[_nodesManager createAnimatedNode:@201 config:@{@"type" : @"style", @"style" : @{@"opacity" : @101}}];
[_nodesManager createAnimatedNode:@301 config:@{@"type" : @"props", @"props" : @{@"style" : @201}}];
[_nodesManager connectAnimatedNodes:@101 childTag:@201];
[_nodesManager connectAnimatedNodes:@201 childTag:@301];
[_nodesManager connectAnimatedNodeToView:@301 viewTag:viewTag viewName:@"UIView"];
}
- (void)testFramesAnimation
{
[self createSimpleAnimatedView:@1001 withOpacity:0];
NSArray<NSNumber *> *frames = @[ @0, @0.2, @0.4, @0.6, @0.8, @1 ];
[_nodesManager startAnimatingNode:@1
nodeTag:@101
config:@{@"type" : @"frames", @"frames" : frames, @"toValue" : @1}
endCallback:nil];
for (NSNumber *frame in frames) {
[[_uiManager expect] synchronouslyUpdateViewOnUIThread:@1001
viewName:@"UIView"
props:RCTPropChecker(@"opacity", frame)];
[_nodesManager stepAnimations:_displayLink];
[_uiManager verify];
}
[[_uiManager expect] synchronouslyUpdateViewOnUIThread:@1001 viewName:@"UIView" props:RCTPropChecker(@"opacity", @1)];
[_nodesManager stepAnimations:_displayLink];
[_uiManager verify];
[[_uiManager reject] synchronouslyUpdateViewOnUIThread:OCMOCK_ANY viewName:OCMOCK_ANY props:OCMOCK_ANY];
[_nodesManager stepAnimations:_displayLink];
[_uiManager verify];
}
- (void)testFramesAnimationLoop
{
[self createSimpleAnimatedView:@1001 withOpacity:0];
NSArray<NSNumber *> *frames = @[ @0, @0.2, @0.4, @0.6, @0.8, @1 ];
[_nodesManager startAnimatingNode:@1
nodeTag:@101
config:@{@"type" : @"frames", @"frames" : frames, @"toValue" : @1, @"iterations" : @5}
endCallback:nil];
for (NSUInteger it = 0; it < 5; it++) {
for (NSNumber *frame in frames) {
[[_uiManager expect] synchronouslyUpdateViewOnUIThread:@1001
viewName:@"UIView"
props:RCTPropChecker(@"opacity", frame)];
[_nodesManager stepAnimations:_displayLink];
[_uiManager verify];
}
}
[[_uiManager expect] synchronouslyUpdateViewOnUIThread:@1001 viewName:@"UIView" props:RCTPropChecker(@"opacity", @1)];
[_nodesManager stepAnimations:_displayLink];
[_uiManager verify];
[[_uiManager reject] synchronouslyUpdateViewOnUIThread:OCMOCK_ANY viewName:OCMOCK_ANY props:OCMOCK_ANY];
[_nodesManager stepAnimations:_displayLink];
[_uiManager verify];
}
- (void)testNodeValueListenerIfNotListening
{
NSNumber *nodeId = @101;
[self createSimpleAnimatedView:@1001 withOpacity:0];
NSArray<NSNumber *> *frames = @[ @0, @0.2, @0.4, @0.6, @0.8, @1 ];
RCTFakeValueObserver *observer = [RCTFakeValueObserver new];
[_nodesManager startListeningToAnimatedNodeValue:nodeId valueObserver:observer];
[_nodesManager startAnimatingNode:@1
nodeTag:nodeId
config:@{@"type" : @"frames", @"frames" : frames, @"toValue" : @1}
endCallback:nil];
[_nodesManager stepAnimations:_displayLink];
XCTAssertEqual(observer.calls.count, 1UL);
XCTAssertEqualObjects(observer.calls[0], @0);
[_nodesManager stopListeningToAnimatedNodeValue:nodeId];
[_nodesManager stepAnimations:_displayLink];
XCTAssertEqual(observer.calls.count, 1UL);
}
- (void)testNodeValueListenerIfListening
{
NSNumber *nodeId = @101;
[self createSimpleAnimatedView:@1001 withOpacity:0];
NSArray<NSNumber *> *frames = @[ @0, @0.2, @0.4, @0.6, @0.8, @1 ];
RCTFakeValueObserver *observer = [RCTFakeValueObserver new];
[_nodesManager startListeningToAnimatedNodeValue:nodeId valueObserver:observer];
[_nodesManager startAnimatingNode:@1
nodeTag:nodeId
config:@{@"type" : @"frames", @"frames" : frames, @"toValue" : @1}
endCallback:nil];
for (NSUInteger i = 0; i < frames.count; i++) {
[_nodesManager stepAnimations:_displayLink];
XCTAssertEqual(observer.calls.count, i + 1);
XCTAssertEqualWithAccuracy(observer.calls[i].doubleValue, frames[i].doubleValue, FLT_EPSILON);
}
[_nodesManager stepAnimations:_displayLink];
XCTAssertEqual(observer.calls.count, 7UL);
XCTAssertEqualObjects(observer.calls[6], @1);
[_nodesManager stepAnimations:_displayLink];
XCTAssertEqual(observer.calls.count, 7UL);
}
- (voi
没有合适的资源?快使用搜索试试~ 我知道了~
react-native-0.75.1.zip
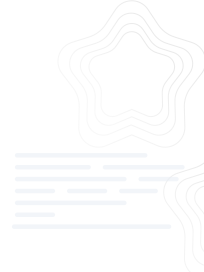
共2000个文件
js:1599个
md:170个
h:84个

需积分: 0 1 下载量 169 浏览量
2024-08-30
10:45:05
上传
评论
收藏 15.44MB ZIP 举报
温馨提示
一个使用React 构建 app 应用程序的框架 A framework for building native applications using React
资源推荐
资源详情
资源评论
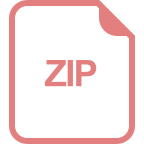
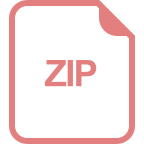
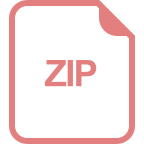
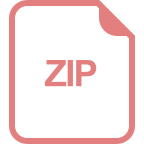
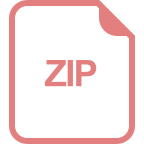
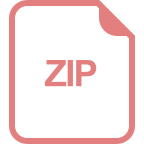
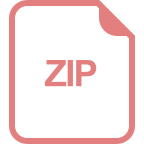
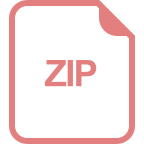
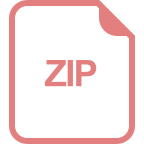
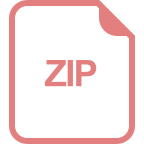
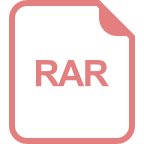
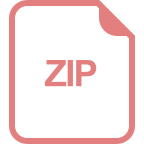
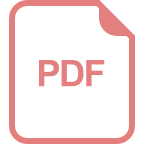
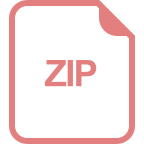
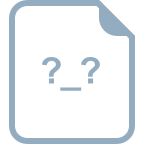
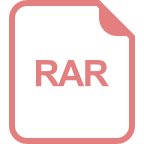
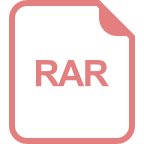
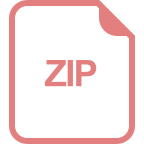
收起资源包目录

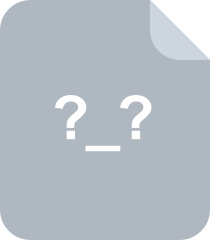
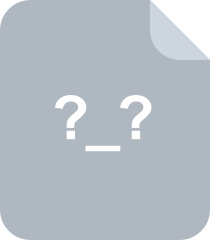
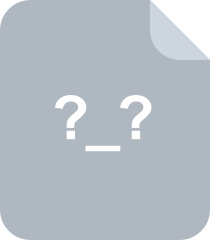
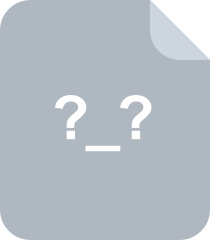
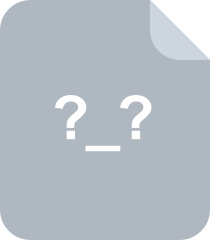
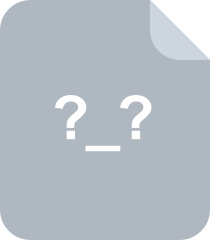
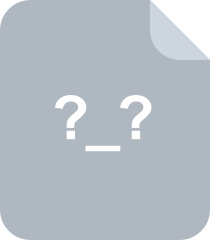
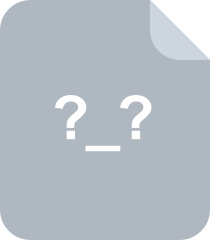
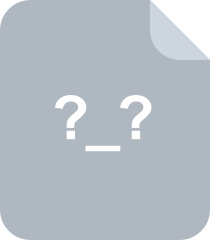
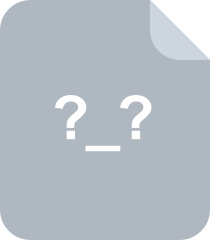
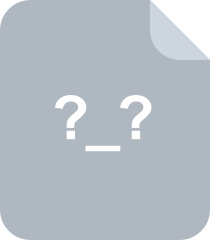
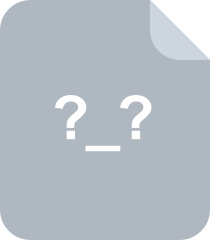
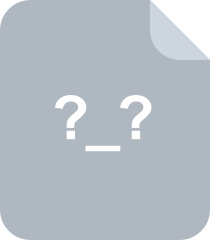
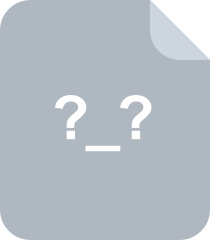
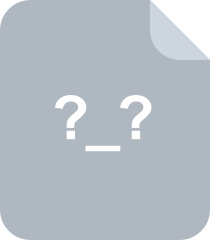
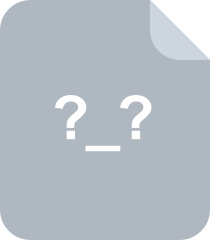
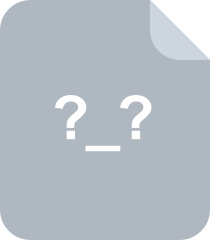
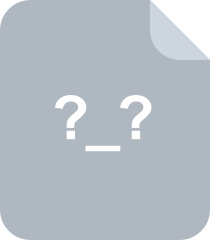
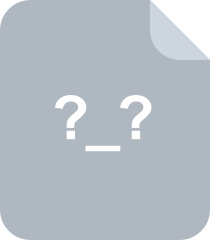
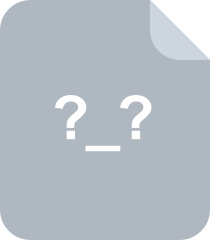
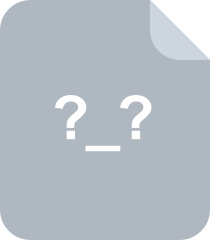
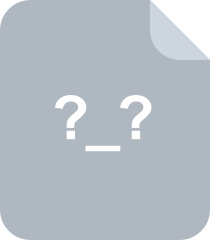
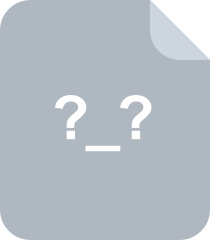
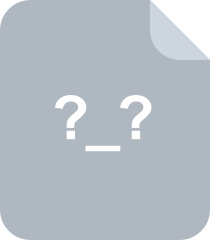
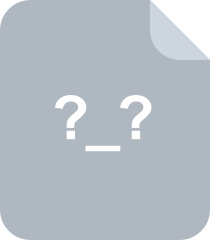
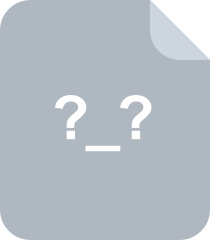
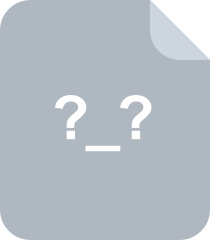
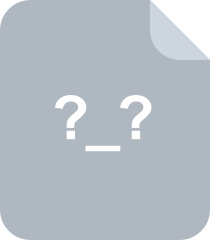
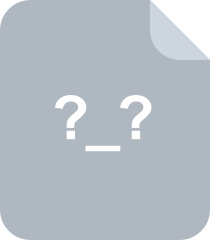
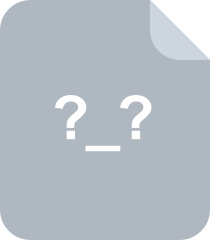
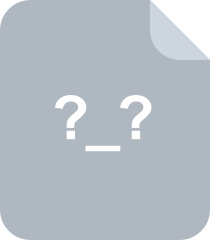
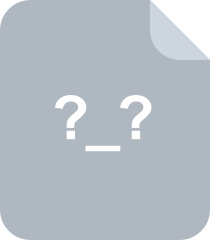
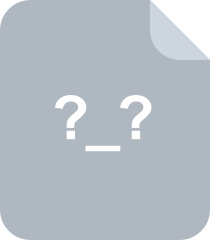
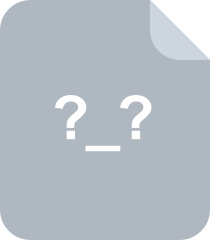
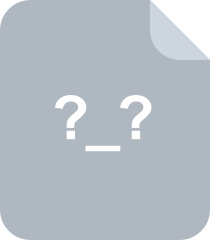
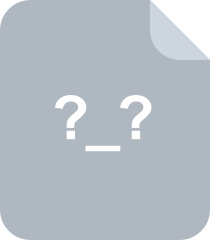
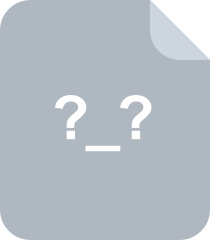
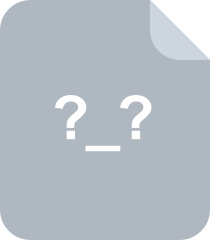
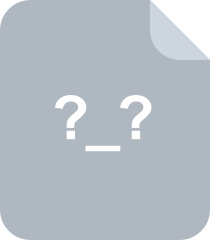
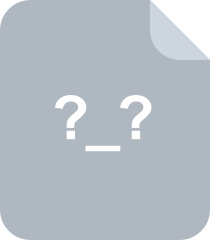
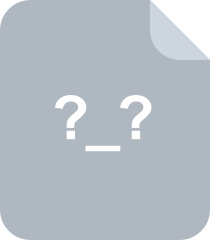
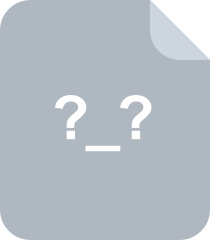
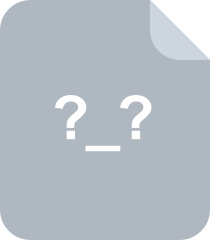
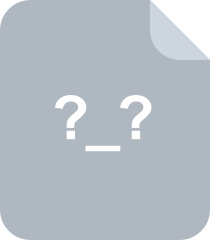
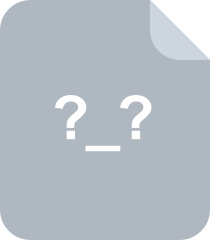
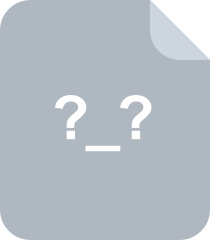
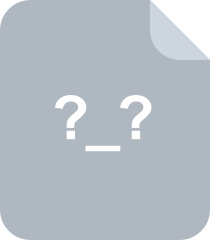
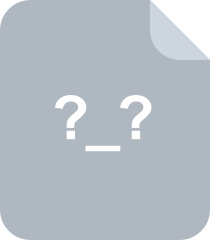
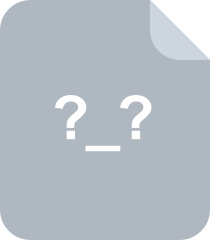
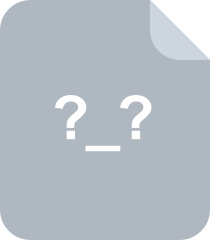
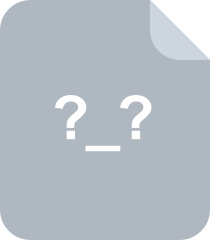
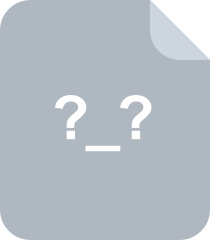
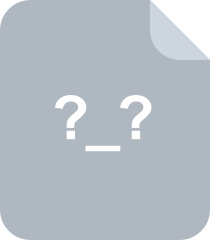
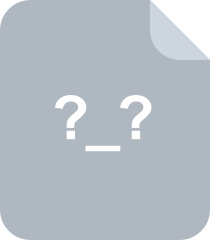
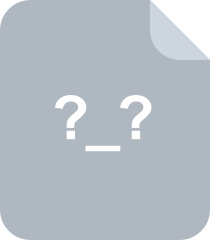
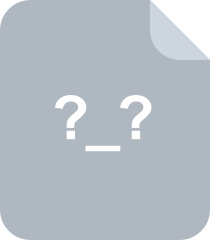
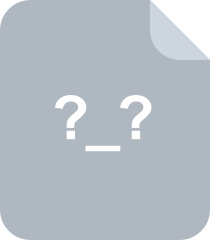
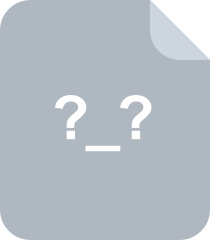
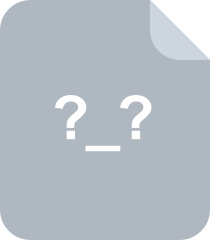
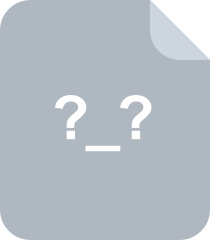
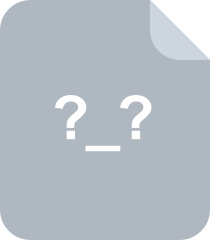
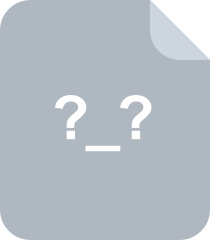
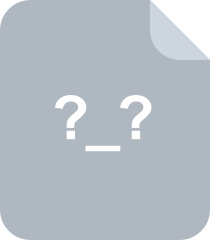
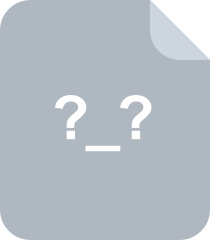
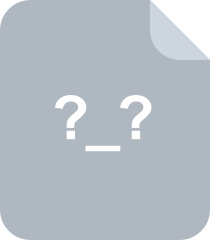
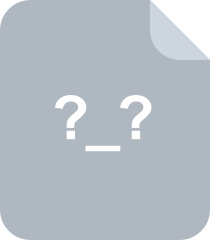
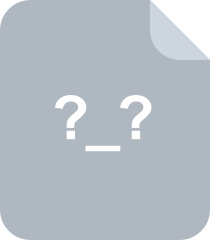
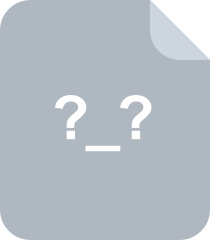
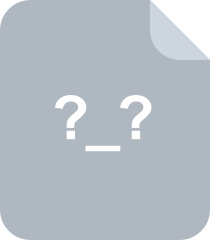
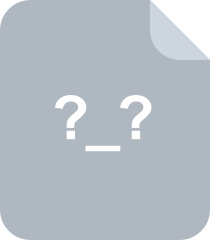
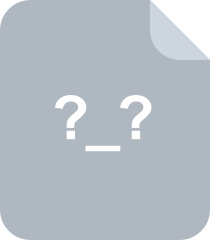
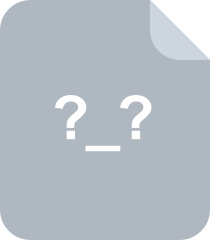
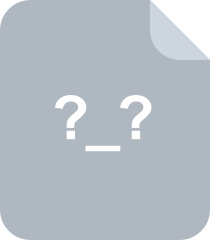
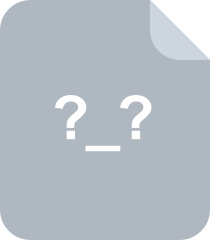
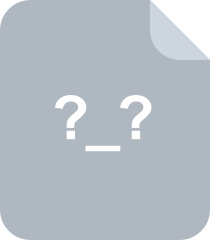
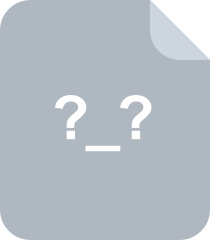
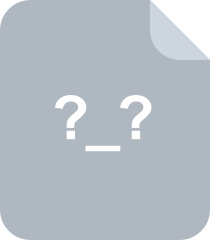
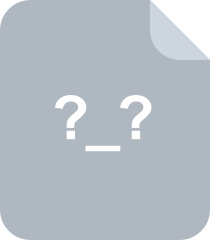
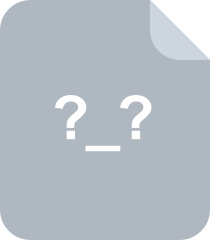
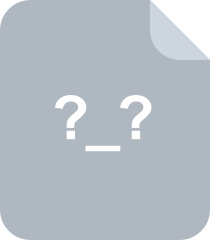
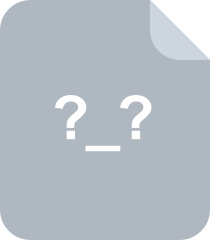
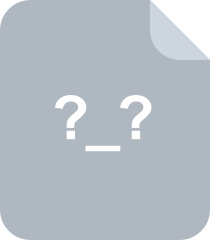
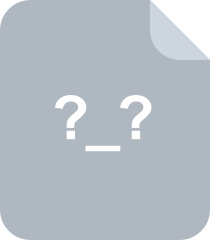
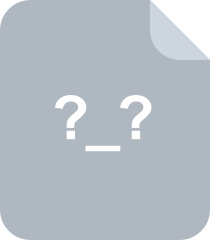
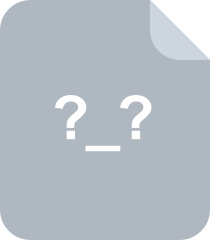
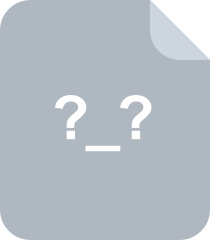
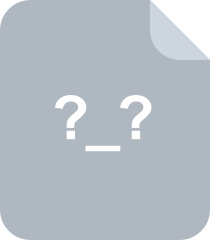
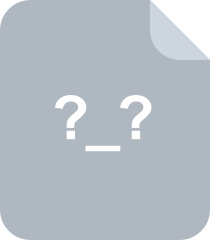
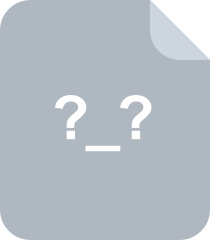
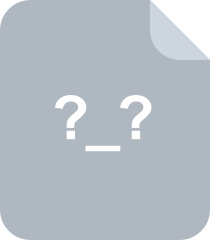
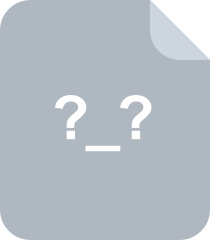
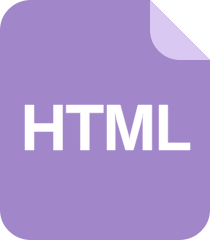
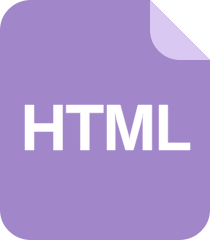
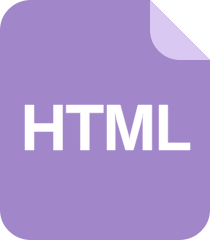
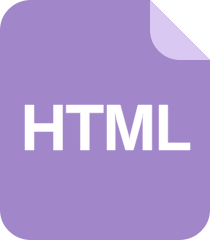
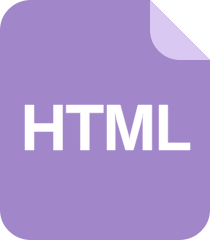
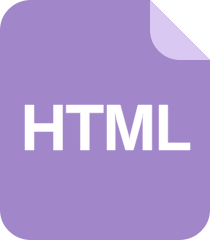
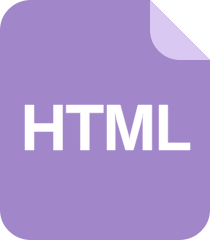
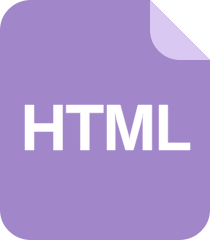
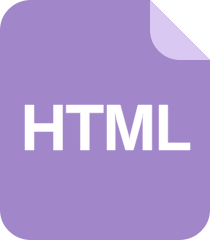
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
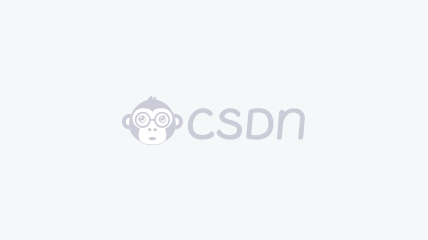

a3737337
- 粉丝: 0
- 资源: 2869
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

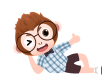
最新资源
- 基于阻抗的微电网下垂控制 在微电网中,由于线路阻抗的不同,造成无功功率无法均分,通过添加阻抗是应用最为广泛的一种方法 仿真以两个DG为例,仿真的波形有有功功率、无功功率、频率、电流、电压这些波形,通
- 冰桶大战-打地鼠游戏JS源码,小游戏源码.zip
- CNN和Transformer.7z
- 基于Web的校内二手商品交易系统的设计与实现.doc
- Python Django 数据采集系统的基本框架与实例
- (22016244)多目标粒子群算法分享 - CSDN博主dkjkls
- Carsim Simulink联合仿真-基于LQR 模糊PID 滑模控制的横摆稳定性控制系统 综合跟随理想横摆角速度的方法和抑制汽车质心侧偏角的汽车稳定性控制方法,以线性二自由度车辆操纵特性模型为控制
- 基于安卓的智能化家庭理财管理app论文.doc
- (25103842)基于STM32的智能万年历课程设计
- 3_新建 DOCX 文档 (2).docx
- 农产品管理与销售小程序的设计与实现论文
- .Net通用运动控制系统 雷赛运动控制卡控制系统 像高川控制卡、高川控制器、或者固高运动控制卡以及正运动控制器、正运动控制卡可以用这个框架,自己替一下库文件等代码就可以 功能丰富,注释多,非常适
- (25778258)典型的多目标优化算法matlab代码-PlatEMO(你所需要多目标优化代码都有)
- Java+Swing+Mysql实现学生成绩管理系统源码+PDF报告(高分项目)
- (2793848)软件工程课件PPT和复习试题
- (33272006)6到9届蓝桥杯国赛 软件类.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


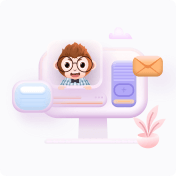
安全验证
文档复制为VIP权益,开通VIP直接复制
