/**
* Copyright (c) 2014-present, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*/
package com.facebook.csslayout;
import com.facebook.infer.annotation.Assertions;
import static com.facebook.csslayout.CSSLayout.DIMENSION_HEIGHT;
import static com.facebook.csslayout.CSSLayout.DIMENSION_WIDTH;
import static com.facebook.csslayout.CSSLayout.POSITION_BOTTOM;
import static com.facebook.csslayout.CSSLayout.POSITION_LEFT;
import static com.facebook.csslayout.CSSLayout.POSITION_RIGHT;
import static com.facebook.csslayout.CSSLayout.POSITION_TOP;
/**
* Calculates layouts based on CSS style. See {@link #layoutNode(CSSNode, float, float)}.
*/
public class LayoutEngine {
private static final int CSS_FLEX_DIRECTION_COLUMN =
CSSFlexDirection.COLUMN.ordinal();
private static final int CSS_FLEX_DIRECTION_COLUMN_REVERSE =
CSSFlexDirection.COLUMN_REVERSE.ordinal();
private static final int CSS_FLEX_DIRECTION_ROW =
CSSFlexDirection.ROW.ordinal();
private static final int CSS_FLEX_DIRECTION_ROW_REVERSE =
CSSFlexDirection.ROW_REVERSE.ordinal();
private static final int CSS_POSITION_RELATIVE = CSSPositionType.RELATIVE.ordinal();
private static final int CSS_POSITION_ABSOLUTE = CSSPositionType.ABSOLUTE.ordinal();
private static final int[] leading = {
POSITION_TOP,
POSITION_BOTTOM,
POSITION_LEFT,
POSITION_RIGHT,
};
private static final int[] trailing = {
POSITION_BOTTOM,
POSITION_TOP,
POSITION_RIGHT,
POSITION_LEFT,
};
private static final int[] pos = {
POSITION_TOP,
POSITION_BOTTOM,
POSITION_LEFT,
POSITION_RIGHT,
};
private static final int[] dim = {
DIMENSION_HEIGHT,
DIMENSION_HEIGHT,
DIMENSION_WIDTH,
DIMENSION_WIDTH,
};
private static final int[] leadingSpacing = {
Spacing.TOP,
Spacing.BOTTOM,
Spacing.START,
Spacing.START
};
private static final int[] trailingSpacing = {
Spacing.BOTTOM,
Spacing.TOP,
Spacing.END,
Spacing.END
};
private static boolean isFlexBasisAuto(CSSNode node) {
return CSSConstants.isUndefined(node.style.flexBasis);
}
private static float getFlexGrowFactor(CSSNode node) {
return node.style.flexGrow;
}
private static float getFlexShrinkFactor(CSSNode node) {
return node.style.flexShrink;
}
private static float boundAxisWithinMinAndMax(CSSNode node, int axis, float value) {
float min = CSSConstants.UNDEFINED;
float max = CSSConstants.UNDEFINED;
if (axis == CSS_FLEX_DIRECTION_COLUMN ||
axis == CSS_FLEX_DIRECTION_COLUMN_REVERSE) {
min = node.style.minHeight;
max = node.style.maxHeight;
} else if (axis == CSS_FLEX_DIRECTION_ROW ||
axis == CSS_FLEX_DIRECTION_ROW_REVERSE) {
min = node.style.minWidth;
max = node.style.maxWidth;
}
float boundValue = value;
if (!Float.isNaN(max) && max >= 0.0 && boundValue > max) {
boundValue = max;
}
if (!Float.isNaN(min) && min >= 0.0 && boundValue < min) {
boundValue = min;
}
return boundValue;
}
private static float boundAxis(CSSNode node, int axis, float value) {
float paddingAndBorderAxis =
node.style.padding.getWithFallback(leadingSpacing[axis], leading[axis]) +
node.style.border.getWithFallback(leadingSpacing[axis], leading[axis]) +
node.style.padding.getWithFallback(trailingSpacing[axis], trailing[axis]) +
node.style.border.getWithFallback(trailingSpacing[axis], trailing[axis]);
return Math.max(boundAxisWithinMinAndMax(node, axis, value), paddingAndBorderAxis);
}
private static float getRelativePosition(CSSNode node, int axis) {
float lead = node.style.position.getWithFallback(leadingSpacing[axis], leading[axis]);
if (!Float.isNaN(lead)) {
return lead;
}
float trailingPos = node.style.position.getWithFallback(trailingSpacing[axis], trailing[axis]);
return Float.isNaN(trailingPos) ? 0 : -trailingPos;
}
private static void setPosition(CSSNode node, CSSDirection direction) {
int mainAxis = resolveAxis(getFlexDirection(node), direction);
int crossAxis = getCrossFlexDirection(mainAxis, direction);
node.layout.position[leading[mainAxis]] = node.style.margin.getWithFallback(leadingSpacing[mainAxis], leading[mainAxis]) +
getRelativePosition(node, mainAxis);
node.layout.position[trailing[mainAxis]] = node.style.margin.getWithFallback(trailingSpacing[mainAxis], trailing[mainAxis]) +
getRelativePosition(node, mainAxis);
node.layout.position[leading[crossAxis]] = node.style.margin.getWithFallback(leadingSpacing[crossAxis], leading[crossAxis]) +
getRelativePosition(node, crossAxis);
node.layout.position[trailing[crossAxis]] = node.style.margin.getWithFallback(trailingSpacing[crossAxis], trailing[crossAxis]) +
getRelativePosition(node, crossAxis);
}
private static int resolveAxis(
int axis,
CSSDirection direction) {
if (direction == CSSDirection.RTL) {
if (axis == CSS_FLEX_DIRECTION_ROW) {
return CSS_FLEX_DIRECTION_ROW_REVERSE;
} else if (axis == CSS_FLEX_DIRECTION_ROW_REVERSE) {
return CSS_FLEX_DIRECTION_ROW;
}
}
return axis;
}
private static CSSDirection resolveDirection(CSSNode node, CSSDirection parentDirection) {
CSSDirection direction = node.style.direction;
if (direction == CSSDirection.INHERIT) {
direction = (parentDirection == null ? CSSDirection.LTR : parentDirection);
}
return direction;
}
private static int getFlexDirection(CSSNode node) {
return node.style.flexDirection.ordinal();
}
private static int getCrossFlexDirection(
int axis,
CSSDirection direction) {
if (axis == CSS_FLEX_DIRECTION_COLUMN ||
axis == CSS_FLEX_DIRECTION_COLUMN_REVERSE) {
return resolveAxis(CSS_FLEX_DIRECTION_ROW, direction);
} else {
return CSS_FLEX_DIRECTION_COLUMN;
}
}
private static CSSAlign getAlignItem(CSSNode node, CSSNode child) {
if (child.style.alignSelf != CSSAlign.AUTO) {
return child.style.alignSelf;
}
return node.style.alignItems;
}
private static boolean isMeasureDefined(CSSNode node) {
return node.isMeasureDefined();
}
/*package*/ static void layoutNode(
CSSLayoutContext layoutContext,
CSSNode node,
float availableWidth,
float availableHeight,
CSSDirection parentDirection) {
// Increment the generation count. This will force the recursive routine to visit
// all dirty nodes at least once. Subsequent visits will be skipped if the input
// parameters don't change.
layoutContext.currentGenerationCount++;
CSSMeasureMode widthMeasureMode = CSSMeasureMode.UNDEFINED;
CSSMeasureMode heightMeasureMode = CSSMeasureMode.UNDEFINED;
if (!Float.isNaN(availableWidth)) {
widthMeasureMode = CSSMeasureMode.EXACTLY;
} else if (node.style.dimensions[DIMENSION_WIDTH] >= 0.0) {
float marginAxisRow = (node.style.margin.getWithFallback(leadingSpacing[CSS_FLEX_DIRECTION_ROW], leading[CSS_FLEX_DIRECTION_ROW]) + node.style.margin.getWithFallback(trailingSpacing[CSS_FLEX_DIRECTION_ROW], trailing[CSS_FLEX_DIRECTION_ROW]));
availableWidth = node.style.dimensions[DIMENSION_WIDTH] + marginAxisRow;
widthMeasureMode = CSSMeasureMode.EXACTLY;
} else if (node.style.maxWidth >= 0.0) {
availableWidth = node.style.maxWidth;
widthMeasureMode = CSSMeasureMode.AT_MOST;
}
if (!Float.isNaN(availableHeight)) {
heightMeasureMode = CSSMeasureMode.EXACTLY;
} else if (node.style.dimensions[DIMENSION_HEIGHT] >= 0.0) {
float marginAxisColumn = (node.style.margin.getWithFallback(lead
没有合适的资源?快使用搜索试试~ 我知道了~
react-native-0.34.0-rc.1.zip
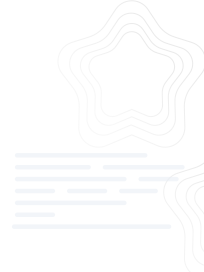
共2000个文件
js:714个
java:561个
h:323个

需积分: 0 0 下载量 35 浏览量
2024-08-29
15:49:57
上传
评论
收藏 19.3MB ZIP 举报
温馨提示
一个使用 React 构建 app 应用程序的框架 A framework for building native applications using React
资源推荐
资源详情
资源评论
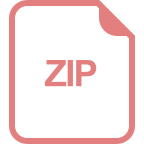
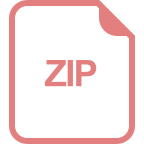
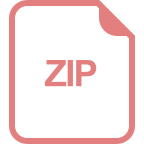
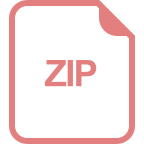
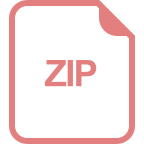
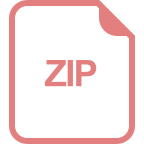
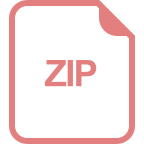
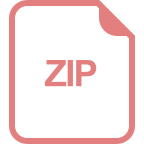
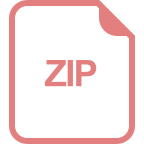
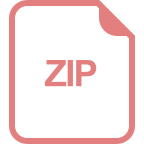
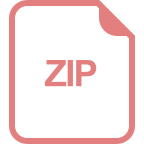
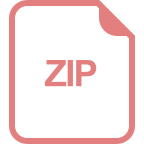
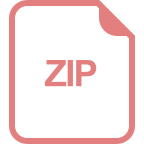
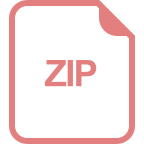
收起资源包目录

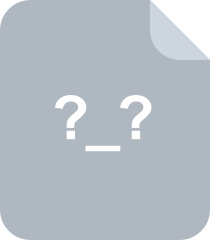
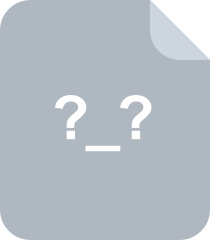
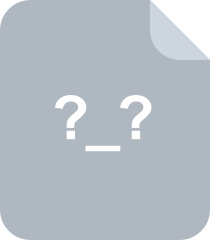
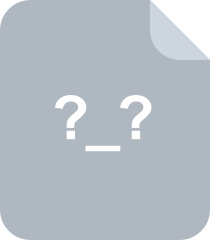
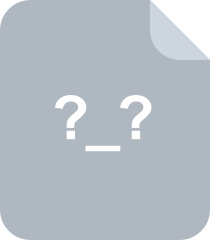
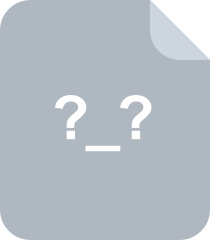
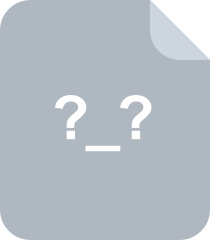
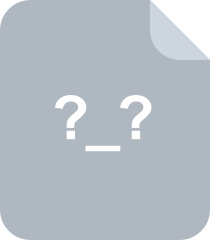
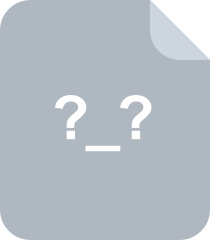
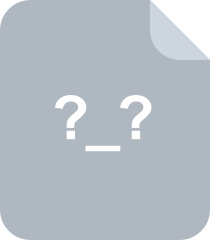
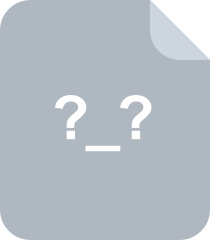
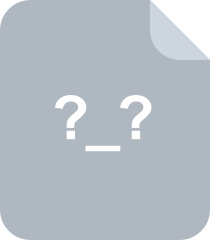
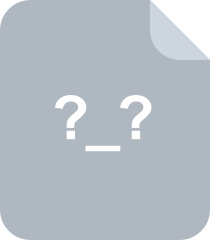
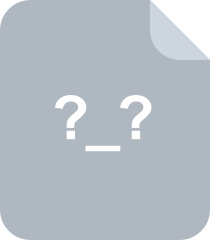
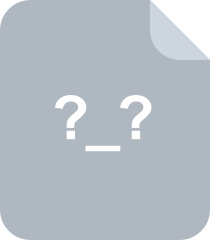
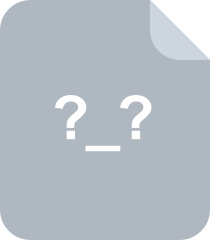
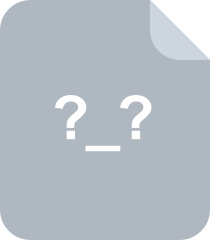
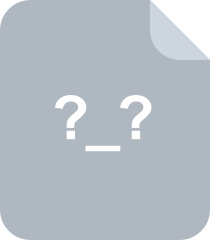
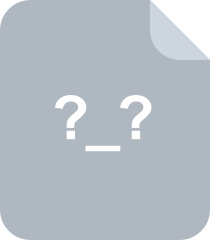
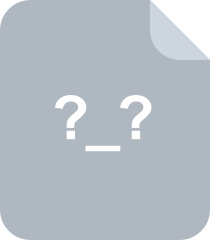
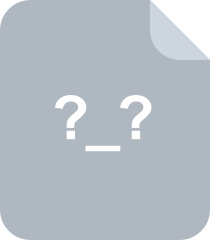
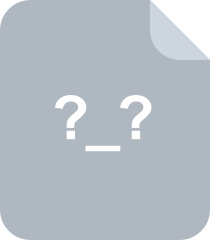
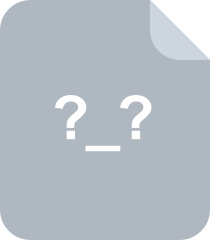
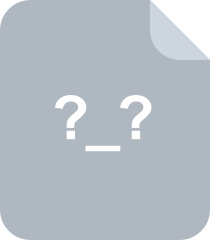
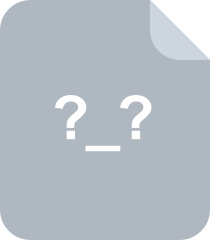
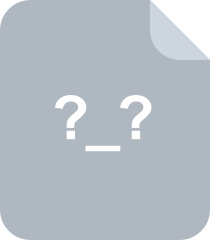
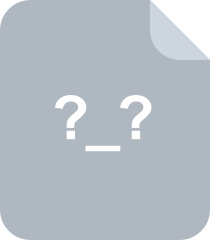
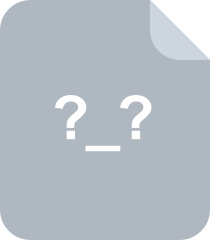
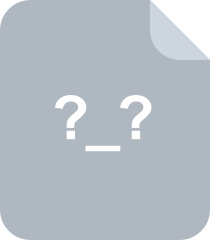
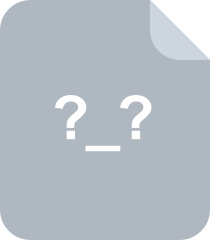
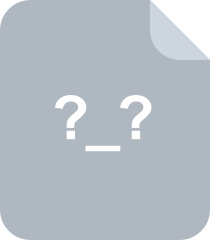
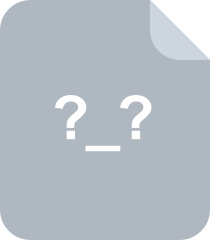
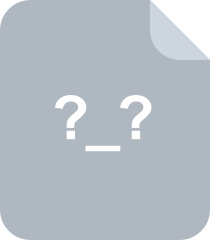
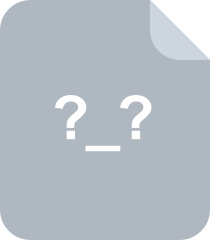
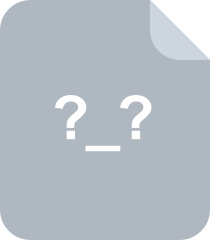
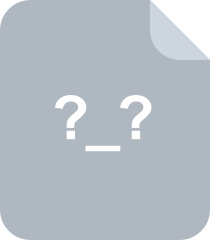
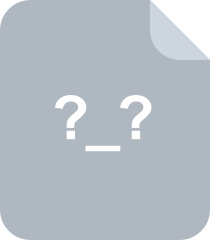
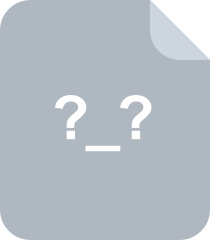
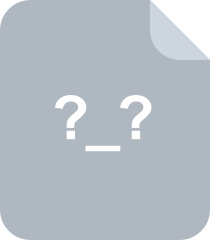
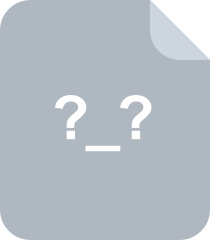
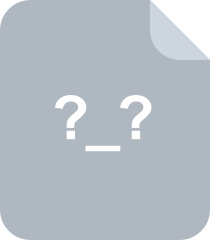
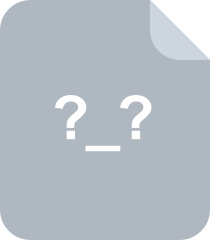
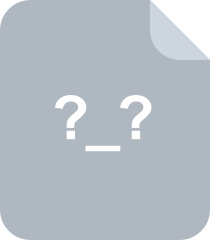
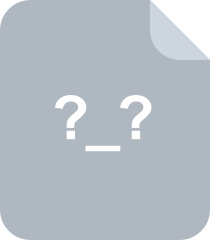
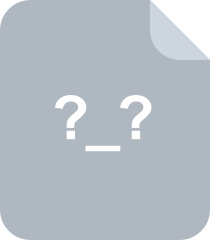
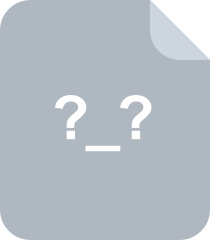
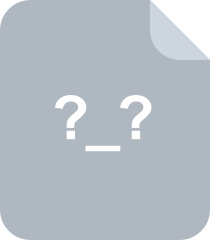
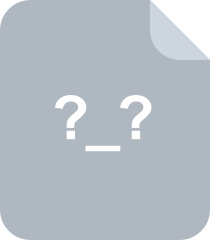
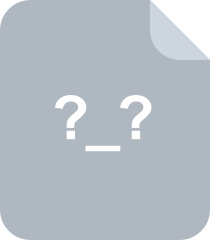
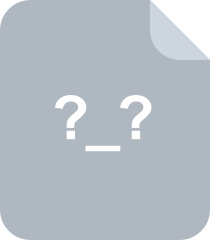
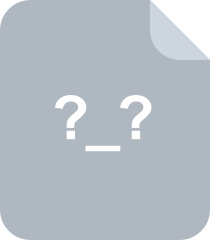
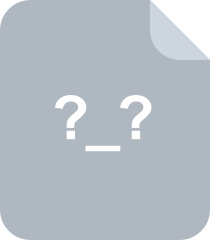
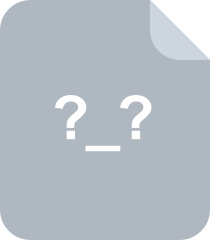
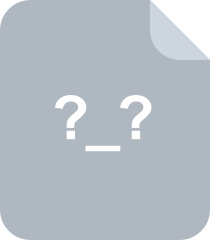
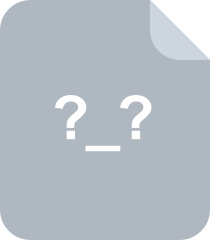
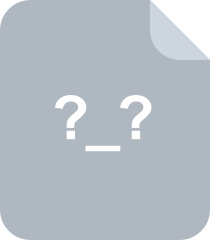
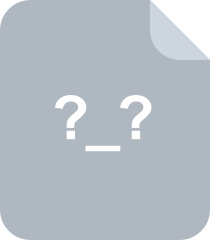
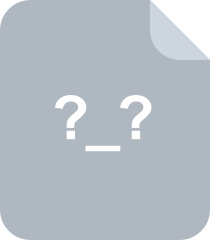
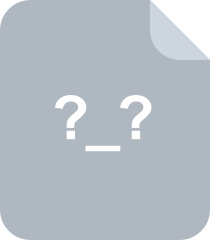
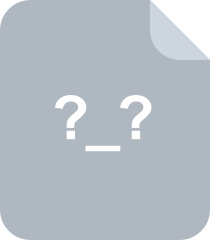
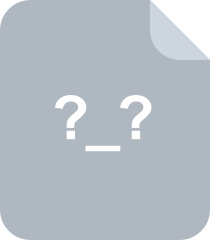
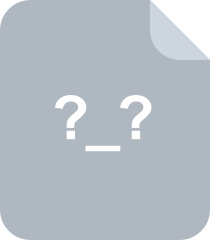
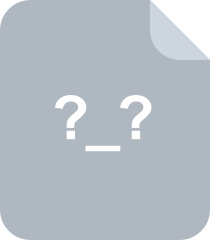
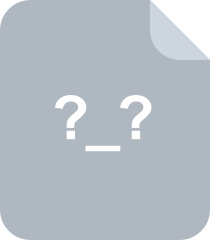
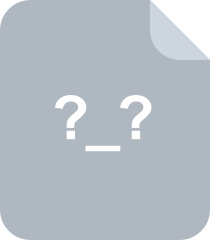
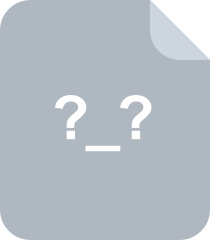
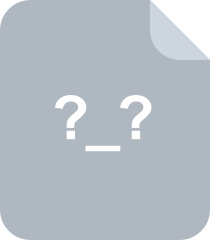
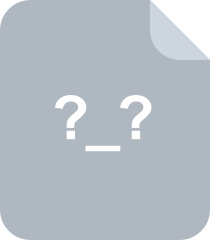
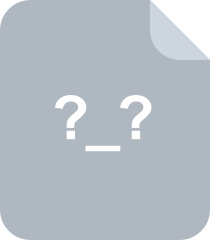
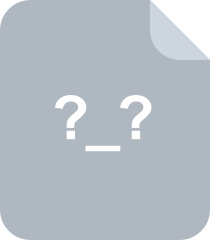
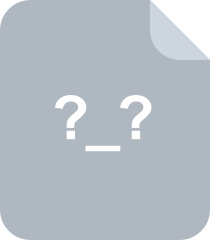
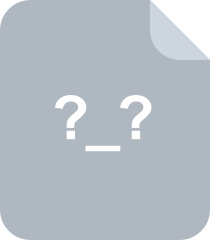
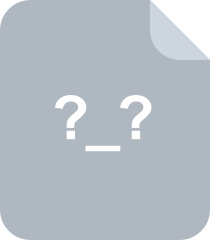
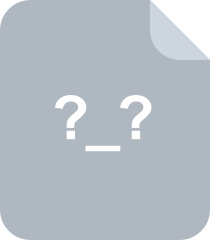
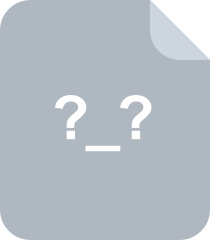
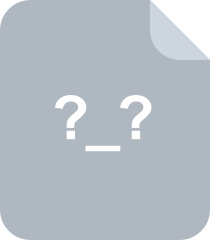
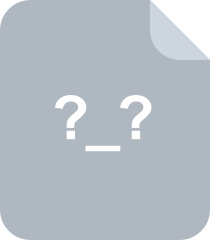
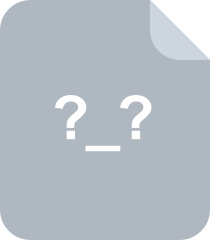
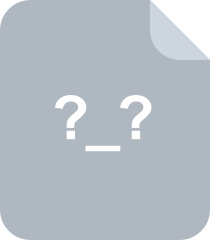
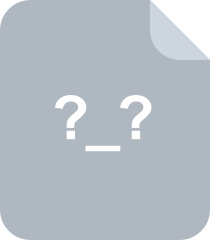
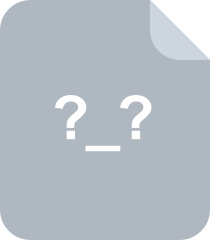
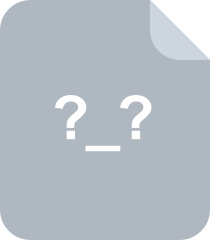
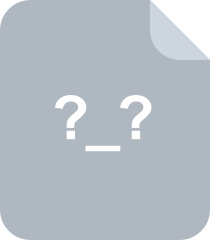
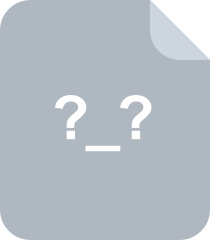
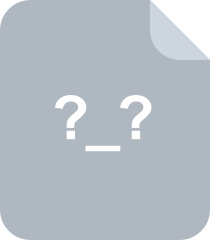
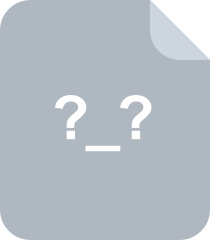
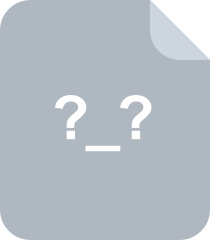
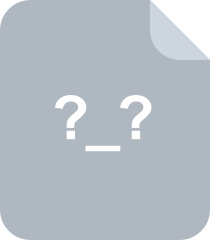
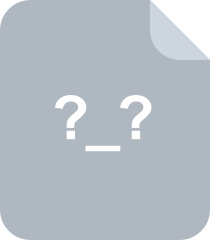
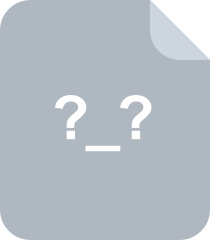
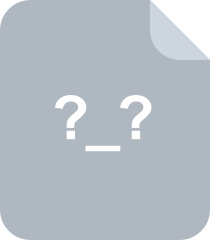
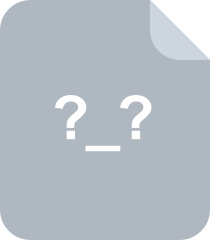
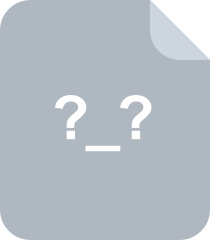
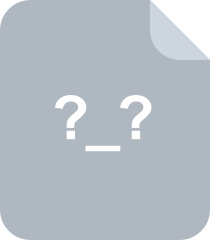
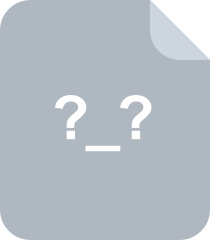
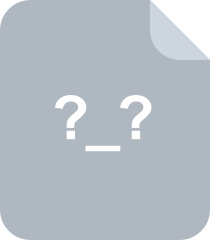
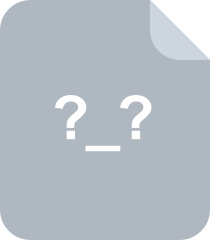
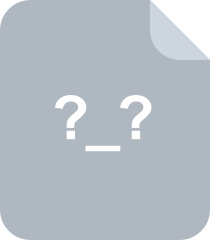
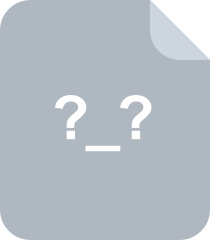
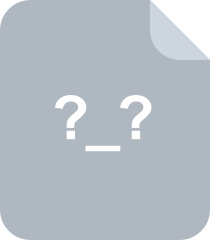
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
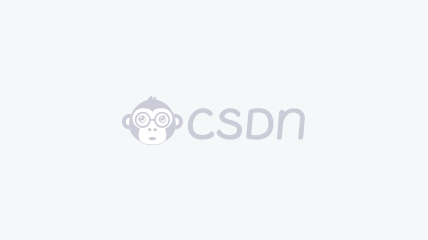

a3737337
- 粉丝: 0
- 资源: 2869
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

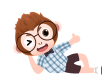
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


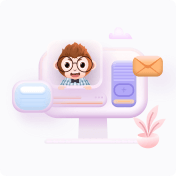
安全验证
文档复制为VIP权益,开通VIP直接复制
