/*
* Copyright (C) 2009 Pierre H�bert <pierrox@pierrox.net>
* http://www.pierrox.net/mcompass/
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.pierrox.mcompass;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.nio.IntBuffer;
import javax.microedition.khronos.opengles.GL10;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.LinearGradient;
import android.graphics.Paint;
import android.graphics.Path;
import android.graphics.RadialGradient;
import android.graphics.Shader;
public class Turntable {
private static final int DETAIL_X[]={ 15, 25, 30 };
private static final int DETAIL_Y[]={ 3, 6, 6 };
private static final int RING_HEIGHT[]={ 2, 3, 3};
private static final int TEXTURE_RING=0;
private static final int TEXTURE_DIAL=1;
private static final String[] CARDINAL_POINTS={ "N", "W", "S", "E" };
// preference values
private int mDetailsLevel;
private boolean mReversedRing;
private int[] mTextures;
private IntBuffer mRingVertexBuffer;
private IntBuffer mRingNormalBuffer;
private IntBuffer mRingTexCoordBuffer;
private ByteBuffer mRingIndexBuffer;
private IntBuffer mDialVertexBuffer;
private IntBuffer mDialNormalBuffer;
private IntBuffer mDialTexCoordBuffer;
private ByteBuffer mDialIndexBuffer;
private IntBuffer mCapVertexBuffer;
private ByteBuffer mCapIndexBuffer;
private boolean mNeedObjectsUpdate;
private boolean mNeedTextureUpdate;
public Turntable() {
mDetailsLevel=0;
mReversedRing=false;
// initially both objects and textures need to be built
mNeedObjectsUpdate=true;
mNeedTextureUpdate=true;
}
private void buildObjects() {
buildRingObject();
buildCapObject();
buildDialObject();
mNeedObjectsUpdate=false;
}
void buildRingObject() {
// build vertices
int dx=DETAIL_X[mDetailsLevel];
int dy=DETAIL_Y[mDetailsLevel];
int rh=RING_HEIGHT[mDetailsLevel];
int vertices[]=new int[((dx+1)*(rh+1))*3];
int normals[]=new int[((dx+1)*(rh+1))*3];
int n=0;
for(int i=0; i<=dx; i++) {
for(int j=0; j<=rh; j++) {
double a = i*(Math.PI*2)/dx;
double b = j*Math.PI/(dy*2);
double x = Math.sin(a)*Math.cos(b);
double y = -Math.sin(b);
double z = Math.cos(a)*Math.cos(b);
vertices[n] = (int) (x*65536);
vertices[n+1] = (int) (y*65536);
vertices[n+2] = (int) (z*65536);
normals[n] = vertices[n];
normals[n+1] = vertices[n+1];
normals[n+2] = vertices[n+2];
n+=3;
}
}
// build textures coordinates
int texCoords[]=new int[(dx+1)*(rh+1)*2];
n=0;
for(int i=0; i<=dx; i++) {
for(int j=0; j<=rh; j++) {
texCoords[n++] = (i<<16)/dx;
texCoords[n++] = (j<<16)/rh;
}
}
// build indices
byte indices[]=new byte[dx*rh*3*2];
n=0;
for(int i=0; i<dx; i++) {
for(int j=0; j<rh; j++) {
byte p0=(byte) ((rh+1)*i+j);
indices[n++]=p0;
indices[n++]=(byte) (p0+rh+1);
indices[n++]=(byte) (p0+1);
indices[n++]=(byte) (p0+rh+1);
indices[n++]=(byte) (p0+rh+2);
indices[n++]=(byte) (p0+1);
}
}
ByteBuffer vbb = ByteBuffer.allocateDirect(vertices.length*4);
vbb.order(ByteOrder.nativeOrder());
mRingVertexBuffer = vbb.asIntBuffer();
mRingVertexBuffer.put(vertices);
mRingVertexBuffer.position(0);
ByteBuffer nbb = ByteBuffer.allocateDirect(normals.length*4);
nbb.order(ByteOrder.nativeOrder());
mRingNormalBuffer = nbb.asIntBuffer();
mRingNormalBuffer.put(normals);
mRingNormalBuffer.position(0);
mRingIndexBuffer = ByteBuffer.allocateDirect(indices.length);
mRingIndexBuffer.put(indices);
mRingIndexBuffer.position(0);
ByteBuffer tbb = ByteBuffer.allocateDirect(texCoords.length*4);
tbb.order(ByteOrder.nativeOrder());
mRingTexCoordBuffer = tbb.asIntBuffer();
mRingTexCoordBuffer.put(texCoords);
mRingTexCoordBuffer.position(0);
}
void buildCapObject() {
int dx=DETAIL_X[mDetailsLevel];
int dy=DETAIL_Y[mDetailsLevel];
int rh=RING_HEIGHT[mDetailsLevel];
int h=dy-rh;
// build vertices
int vertices[]=new int[((dx+1)*(h+1))*3];
int n=0;
for(int i=0; i<=dx; i++) {
for(int j=rh; j<=dy; j++) {
double a = i*(Math.PI*2)/dx;
double b = j*Math.PI/(dy*2);
double x = Math.sin(a)*Math.cos(b);
double y = -Math.sin(b);
double z = Math.cos(a)*Math.cos(b);
vertices[n++] = (int) (x*65536);
vertices[n++] = (int) (y*65536);
vertices[n++] = (int) (z*65536);
}
}
// build indices
byte indices[]=new byte[dx*h*3*2];
n=0;
for(int i=0; i<dx; i++) {
for(int j=0; j<h; j++) {
byte p0=(byte) ((h+1)*i+j);
indices[n++]=p0;
indices[n++]=(byte) (p0+h+1);
indices[n++]=(byte) (p0+1);
indices[n++]=(byte) (p0+h+1);
indices[n++]=(byte) (p0+h+2);
indices[n++]=(byte) (p0+1);
}
}
ByteBuffer vbb = ByteBuffer.allocateDirect(vertices.length*4);
vbb.order(ByteOrder.nativeOrder());
mCapVertexBuffer = vbb.asIntBuffer();
mCapVertexBuffer.put(vertices);
mCapVertexBuffer.position(0);
mCapIndexBuffer = ByteBuffer.allocateDirect(indices.length);
mCapIndexBuffer.put(indices);
mCapIndexBuffer.position(0);
}
void buildDialObject() {
// build vertices
int dx=DETAIL_X[mDetailsLevel];
int vertices[]=new int[(dx+2)*3];
int normals[]=new int[(dx+2)*3];
int n=0;
// center of the dial
vertices[n] = 0;
vertices[n+1] = 0;
vertices[n+2] = 0;
normals[n] = 0;
normals[n+1] = 1<<16;
normals[n+2] = 0;
n+=3;
for(int i=0; i<=dx; i++) {
double a = i*(Math.PI*2)/dx;
double x = Math.sin(a);
double z = Math.cos(a);
vertices[n] = (int) (x*65536);
vertices[n+1] = 0;
vertices[n+2] = (int) (z*65536);
normals[n] = 0;
normals[n+1] = 1<<16;
normals[n+2] = 0;
n+=3;
}
// build textures coordinates
int texCoords[]=new int[(dx+2)*2];
n=0;
texCoords[n++] = (int)(0.5*65536);
texCoords[n++] = (int)(0.5*65536);
for(int i=0; i<=dx; i++) {
double a = i*(Math.PI*2)/dx;
double x = (Math.sin(a)+1)/2;
double z = (Math.cos(a)+1)/2;
texCoords[n++] = (int)(x*65536);
texCoords[n++] = (int)(z*65536);
}
// build indices
byte indices[]=new byte[dx+2];
n=0;
for(int i=0; i<=(dx+1); i++) {
indices[n++]=(byte)i;
}
ByteBuffer vbb = ByteBuffer.allocateDirect(vertices.length*4);
vbb.order(ByteOrder.nativeOrder());
mDialVertexBuffer = vbb.asIntBuffer();
mDialVertexBuffer.put(vertices);
mDialVertexBuffer.position(0);
ByteBuffer nbb = ByteBuffer.allocateDirect(normals.le
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
网上有一个3D的罗盘(英文名叫marine compass),利用orientation sensor做出来的,down下来,改进了一下,让它也可以不使用orientation sensor,而是由gsensor和msensor算出来.可以通过它的设置在这两种方式之间进行选择。
资源推荐
资源详情
资源评论
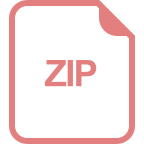
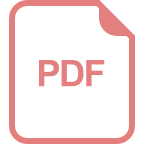
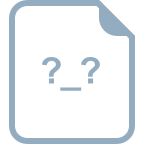
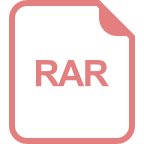
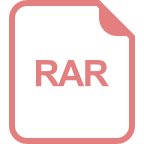
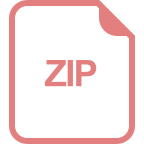
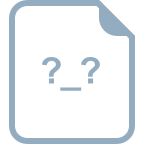
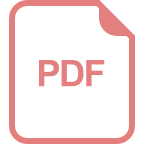
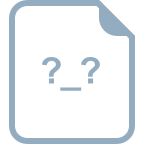
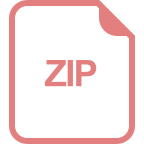
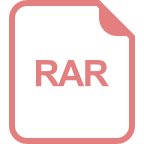
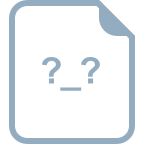
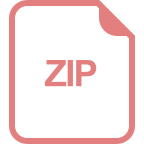
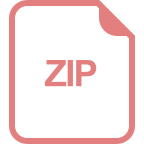
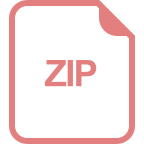
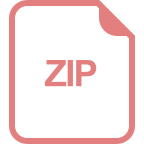
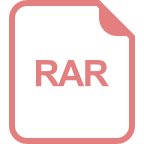
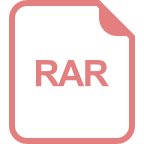
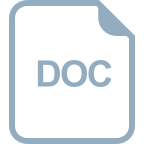
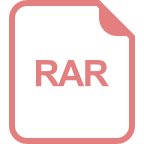
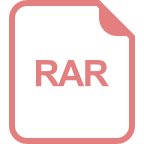
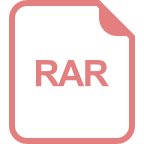
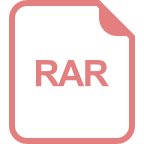
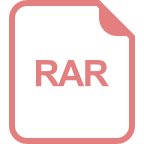
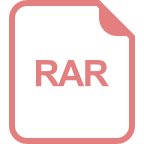
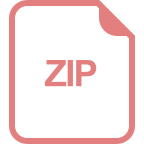
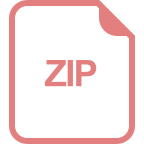
收起资源包目录

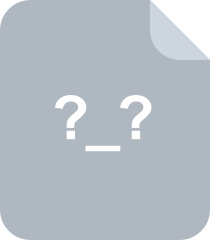




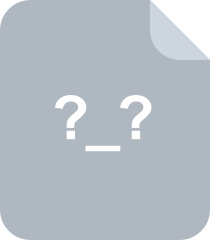
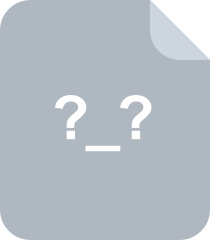





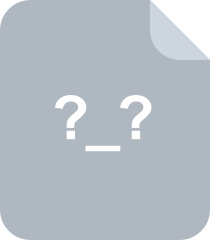
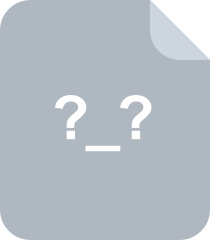



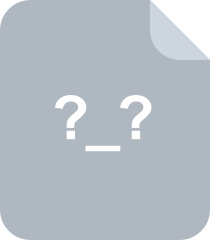
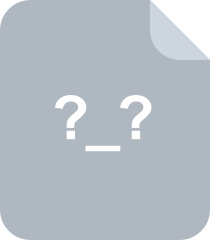
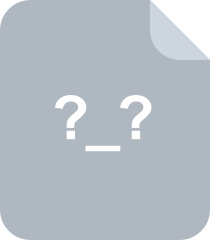
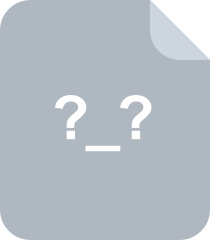


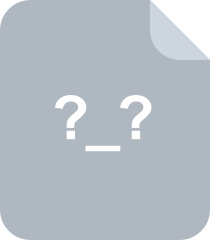
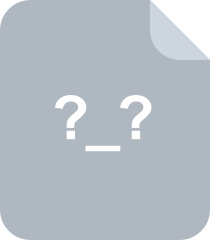

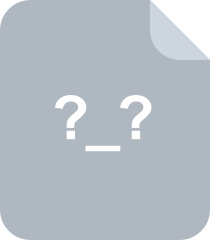
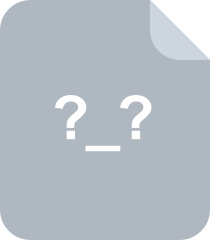
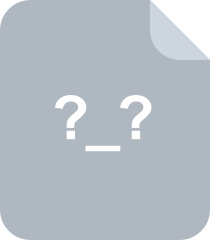
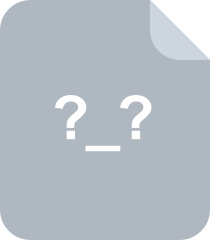


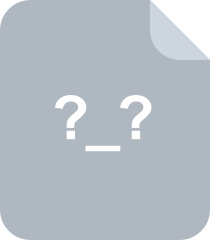
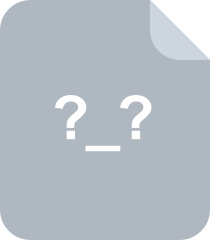
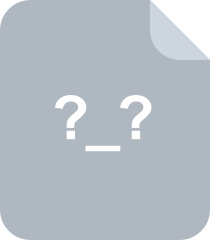
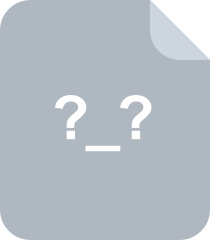
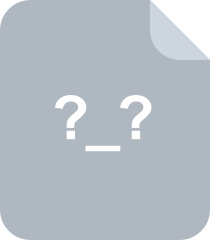
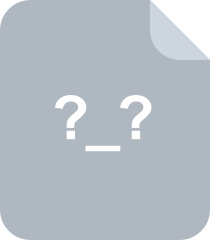


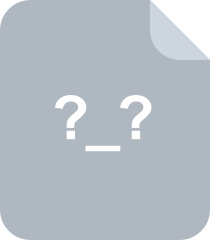
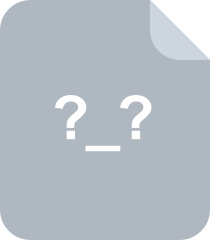


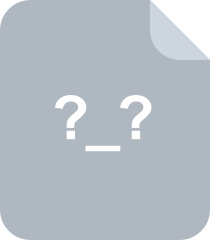
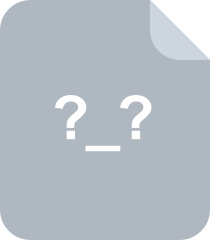
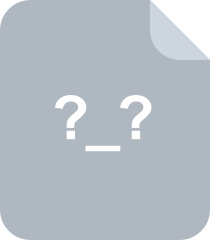
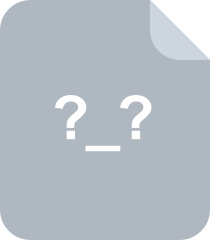



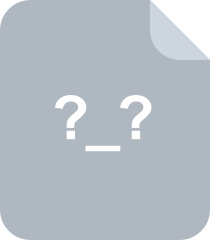
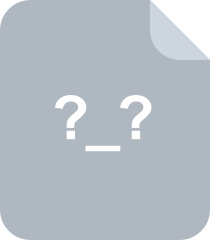

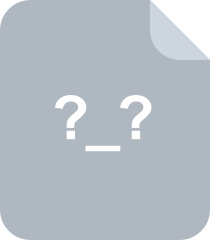
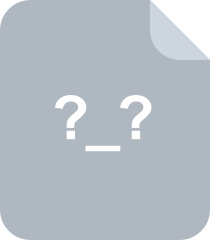





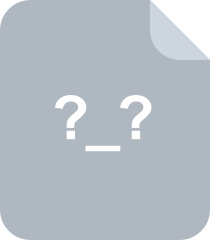
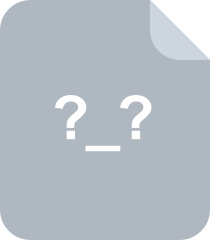
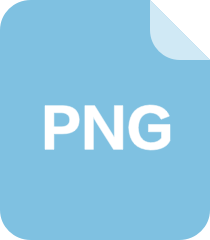
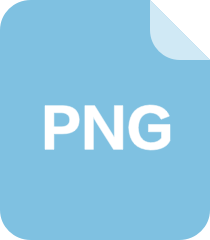



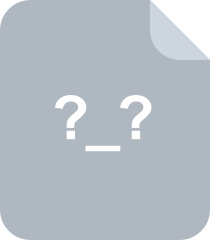
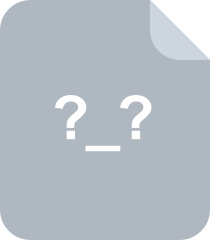

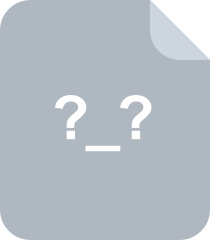





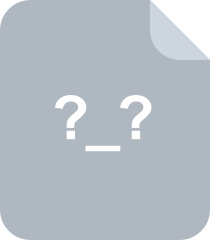
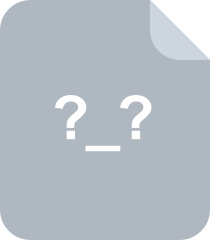




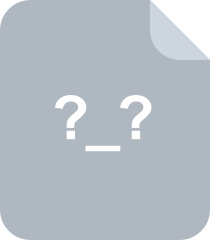
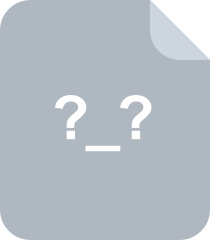









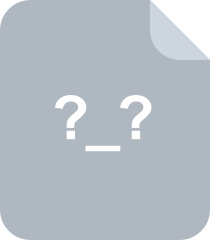
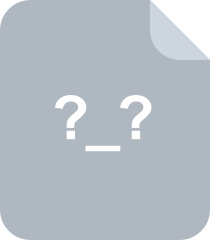







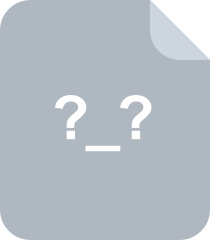
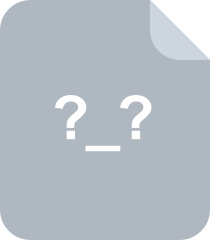


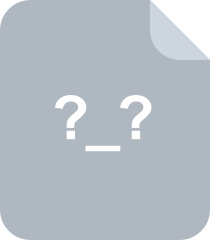
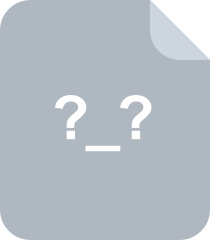

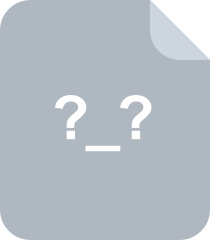
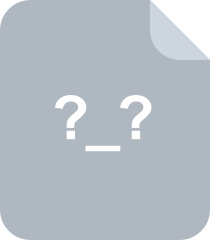
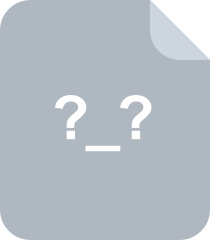
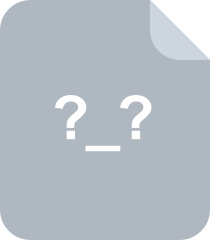





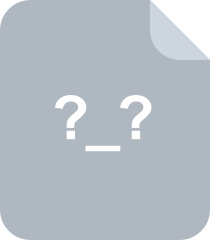
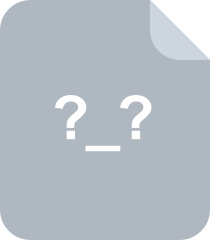


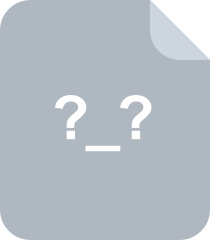
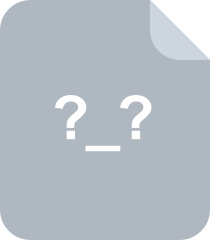










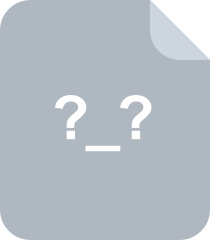
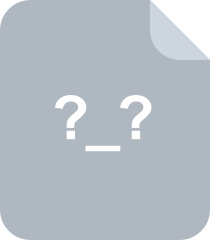

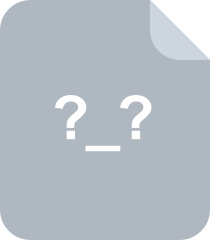





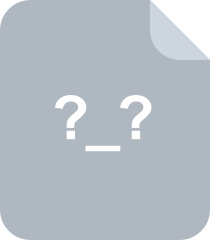



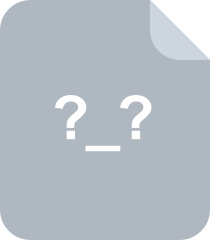
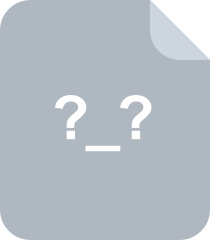









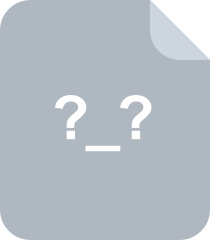
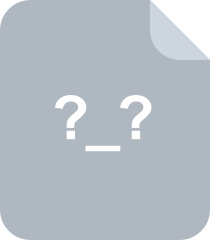

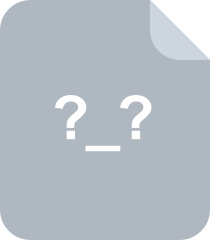





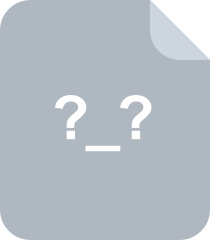



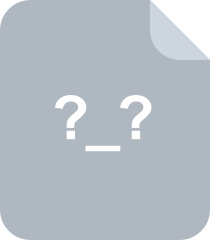
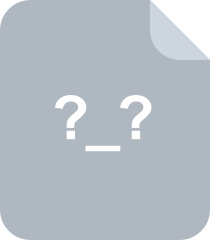

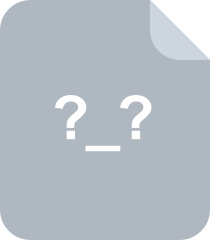





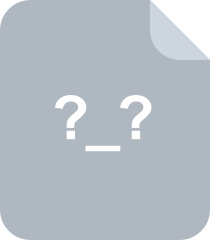



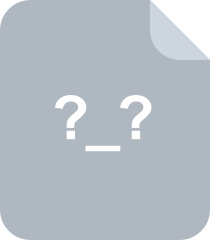
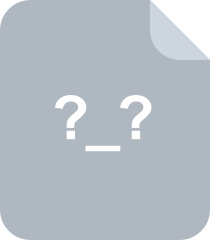

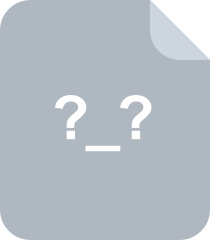





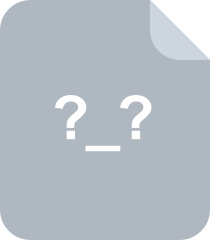


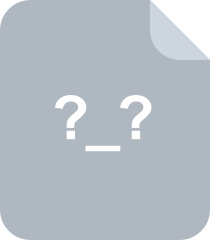
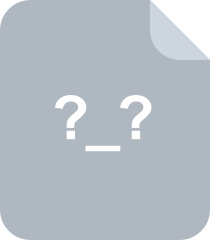









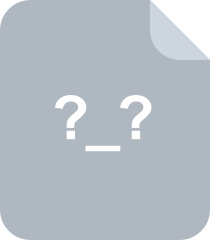
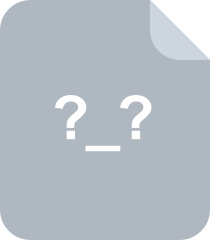

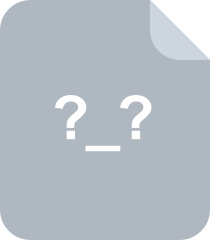





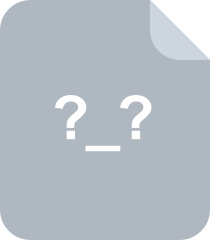



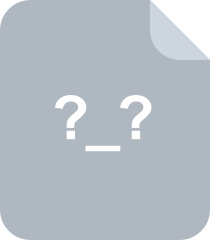
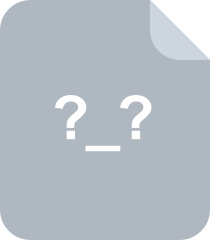

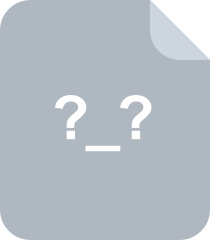





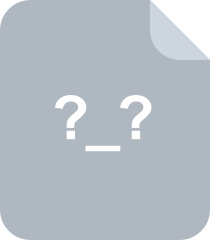
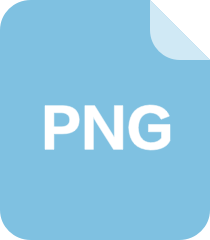



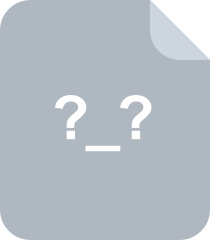
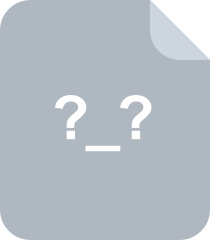

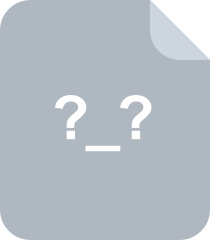





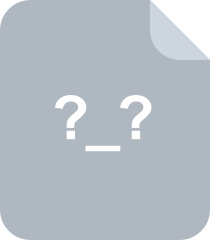




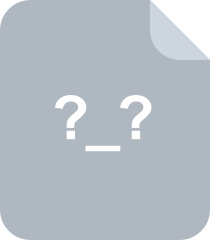



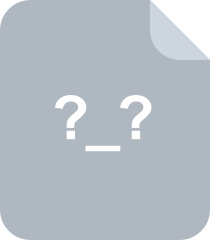
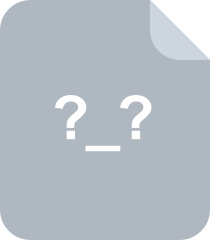






共 92 条
- 1

a345017062
- 粉丝: 1533
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

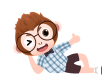
最新资源
- 小伊工具箱小程序源码/趣味工具微信小程序源码
- 网络安全领域中关于防范钓鱼邮件导致的病毒入侵与应对措施探讨
- Build a Large Language Model - 2025
- 郑州升达大学2024-2025第一学期计算机视觉课程期末试卷,
- ztsc_109339.apk
- boost电路电压闭环仿真 有pi控制和零极点补偿器两种 仿真误差0.00705,仿真波形如图二所示 所搭建的模型输入电压5V,输出电压24伏
- COMSOL模拟动水条件联系裂隙注浆扩散,考虑粘度时变
- 学生信息管理系统,该程序用于管理学生的基本信息,包括姓名、年龄、性别和成绩 用户可以添加、删除、修改和查询学生信息
- XC7V2000T+TMS320C6678设计文件,包含原理图,PCB等文件,已验证,可直接生产
- 简易图书管理系统,该程序用于管理图书的基本信息,包括书名、作者、出版年份和库存数量 用户可以添加、删除、修改和查询图书信息
- 简易日程提醒系统, 该程序用于管理用户的日程提醒,包括事件名称、日期、时间和描述 用户可以添加、删除、修改和查询日程提醒
- 无线充电仿真 simulink 磁耦合谐振 无线电能传输 MCR WPT lcc ss llc拓扑补偿 基于matlab 一共四套模型: 1.llc谐振器实现12 24V恒压输出 带调频闭环控制 附
- 直流无刷电机,直径38mm,径向长23.8mm,转速25000rpm,功率200W,可用于磨头加工
- 47191 Python语言程序设计(第2版)(含视频教学)-课后习题答案.zip
- 信息系统管理师试题分享
- FreeRTOS学习之系统移植
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


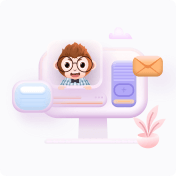
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页