<?php
class PMXI_Import_Record extends PMXI_Model_Record {
public static $cdata = array();
protected $errors;
/**
* Some pre-processing logic, such as removing control characters from xml to prevent parsing errors
* @param string $xml
*/
public static function preprocessXml( &$xml ) {
if ( empty(PMXI_Plugin::$session->is_csv) and empty(PMXI_Plugin::$is_csv)){
self::$cdata = array();
$is_preprocess_enabled = apply_filters('is_xml_preprocess_enabled', true);
if ($is_preprocess_enabled) {
$xml = preg_replace_callback('/<!\[CDATA\[.*?\]\]>/s', 'wp_all_import_cdata_filter', $xml );
$xml = preg_replace_callback('/&(.{2,5};)?/i', 'wp_all_import_amp_filter', $xml );
if ( ! empty(self::$cdata) ){
foreach (self::$cdata as $key => $val) {
$xml = str_replace('{{CPLACE_' . ($key + 1) . '}}', $val, $xml);
}
}
}
}
}
/**
* Validate XML to be valid for import
* @param string $xml
* @param WP_Error[optional] $errors
* @return bool Validation status
*/
public static function validateXml( & $xml, $errors = NULL) {
if (FALSE === $xml or '' == $xml) {
$errors and $errors->add('form-validation', __('WP All Import can\'t read your file.<br/><br/>Probably, you are trying to import an invalid XML feed. Try opening the XML feed in a web browser (Google Chrome is recommended for opening XML files) to see if there is an error message.<br/>Alternatively, run the feed through a validator: http://validator.w3.org/<br/>99% of the time, the reason for this error is because your XML feed isn\'t valid.<br/>If you are 100% sure you are importing a valid XML feed, please contact WP All Import support.', 'wp_all_import_plugin'));
} else {
PMXI_Import_Record::preprocessXml($xml);
if ( function_exists('simplexml_load_string')){
libxml_use_internal_errors(true);
libxml_clear_errors();
$_x = @simplexml_load_string($xml);
$xml_errors = libxml_get_errors();
libxml_clear_errors();
if ($xml_errors) {
$error_msg = '<strong>' . __('Invalid XML', 'wp_all_import_plugin') . '</strong><ul>';
foreach($xml_errors as $error) {
$error_msg .= '<li>';
$error_msg .= __('Line', 'wp_all_import_plugin') . ' ' . $error->line . ', ';
$error_msg .= __('Column', 'wp_all_import_plugin') . ' ' . $error->column . ', ';
$error_msg .= __('Code', 'wp_all_import_plugin') . ' ' . $error->code . ': ';
$error_msg .= '<em>' . trim(esc_html($error->message)) . '</em>';
$error_msg .= '</li>';
}
$error_msg .= '</ul>';
$errors and $errors->add('form-validation', $error_msg);
} else {
return true;
}
}
else{
$errors and $errors->add('form-validation', __('Required PHP components are missing.', 'wp_all_import_plugin'));
$errors and $errors->add('form-validation', __('WP All Import requires the SimpleXML PHP module to be installed. This is a standard feature of PHP, and is necessary for WP All Import to read the files you are trying to import.<br/>Please contact your web hosting provider and ask them to install and activate the SimpleXML PHP module.', 'wp_all_import_plugin'));
}
}
return false;
}
/**
* Initialize model instance
* @param array[optional] $data Array of record data to initialize object with
*/
public function __construct($data = array()) {
parent::__construct($data);
$this->setTable(PMXI_Plugin::getInstance()->getTablePrefix() . 'imports');
$this->errors = new WP_Error();
}
/**
* Import all files matched by path
*
* @param callback[optional] $logger Method where progress messages are submmitted
*
* @return array|PMXI_Import_Record
* @chainable
*/
public function execute($logger = NULL, $cron = true, $history_log_id = false) {
$uploads = wp_upload_dir();
if ($this->path) {
$files = array($this->path);
foreach ($files as $ind => $path) {
$filePath = '';
if ( $this->queue_chunk_number == 0 and $this->processing == 0 ) {
$this->set(array('processing' => 1))->update(); // lock cron requests
$upload_result = FALSE;
if ($this->type == 'ftp'){
try {
$files = PMXI_FTPFetcher::fetch($this->options);
$uploader = new PMXI_Upload($files[0], $this->errors, rtrim(str_replace(basename($files[0]), '', $files[0]), '/'));
$upload_result = $uploader->upload();
} catch (Exception $e) {
$this->errors->add('form-validation', $e->getMessage());
}
} elseif ($this->type == 'url') {
$filesXML = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\r\n<data><node></node></data>";
$filePaths = XmlImportParser::factory($filesXML, '/data/node', $this->path, $file)->parse(); $tmp_files[] = $file;
foreach ($tmp_files as $tmp_file) { // remove all temporary files created
@unlink($tmp_file);
}
$file_to_import = $this->path;
if ( ! empty($filePaths) and is_array($filePaths) ) {
$file_to_import = array_shift($filePaths);
}
$uploader = new PMXI_Upload(trim($file_to_import), $this->errors);
$upload_result = $uploader->url($this->feed_type, $this->path);
} elseif ( $this->type == 'file') {
$uploader = new PMXI_Upload(trim($this->path), $this->errors);
$upload_result = $uploader->file();
} else { // retrieve already uploaded file
$uploader = new PMXI_Upload(trim($this->path), $this->errors, rtrim(str_replace(wp_all_import_basename($this->path), '', $this->path), '/'));
$upload_result = $uploader->upload();
}
if (!count($this->errors->errors)) {
$filePath = $upload_result['filePath'];
}
if ( ! $this->errors->get_error_codes() and "" != $filePath ) {
$this->set(array('queue_chunk_number' => 1))->update();
} elseif ( $this->errors->get_error_codes() ){
$msgs = $this->errors->get_error_messages();
if ( ! is_array($msgs)) {
$msgs = array($msgs);
}
$this->set(array('processing' => 0))->update();
return array(
'status' => 500,
'message' => $msgs
);
}
$this->set(array('processing' => 0))->update(); // unlock cron requests
}
// if empty file path, than it's mean feed in cron process. Take feed path from history.
if (empty($filePath)){
$history = new PMXI_File_List();
$history->setColumns('id', 'name', 'registered_on', 'path')->getBy(array('import_id' => $this->id), 'id DESC');
if ($history->count()){
$history_file = new PMXI_File_Record();
$history_file->getBy('id', $history[0]['id']);
$filePath = wp_all_import_get_absolute_path($history_file->path);
}
}
// If processing is being forced then we must ensure a file exists even if it's empty.
if( apply_filters('wp_all_import_force_cron_processing_on_empty_feed', false, $this->id) ){
if(!empty($filePath) && !file_exists($filePath)){
file_put_contents($filePath, '');
}
}
// if feed path found
if ( ! empty($filePath) and @file_exists($filePath) ) {
$functions = $uploads['basedir'] . DIRECTORY_SEPARATOR . WP_ALL_IMPORT_UPLOADS_BASE_DIRECTORY . DIRECTORY_SEPARATOR . 'functions.php';
$functions = apply_filters( 'import_functions_file_path', $functions );
if ( @file_exists($functions) && PMXI_Plugin::$is_php_allowed)
require_once $functions;
if ( $this->queue_chunk_number === 1 and $this->processing == 0 ){ // import first cron request
$this->set(array('processing' => 1))->update(); // lock cron requests
if (empty($this->options['encoding'])){
$currentOptions = $this->options;
$currentOptions['encoding'] = 'UTF-8';
没有合适的资源?快使用搜索试试~ 我知道了~
WordPress导入导出插件–WP All Import Pro v4.8.7-beta-1.1
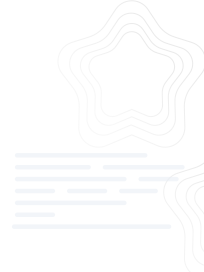
共1108个文件
php:897个
png:75个
js:21个

0 下载量 168 浏览量
2024-03-26
08:59:45
上传
评论
收藏 2.7MB ZIP 举报
温馨提示
WP All Import Pro是WordPress上的一款导入导出插件。可以导入xml、CSV、excel文件。 导入到任何主题或插件字段。 上传任何文件类型、大小和结构。 使用新数据更新现有内容。 导入 WooCommerce、ACF、用户、列表等。 从任何主题或插件导出。 只需几分钟即可完成自定义电子表格。
资源推荐
资源详情
资源评论
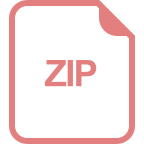
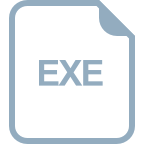
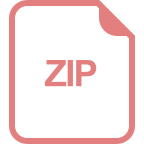
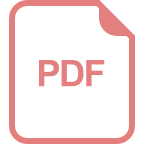
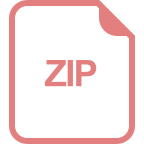
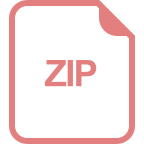
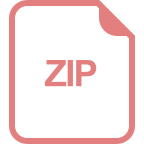
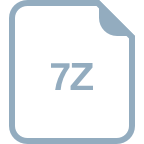
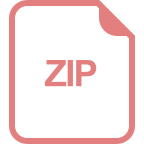
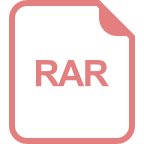
收起资源包目录

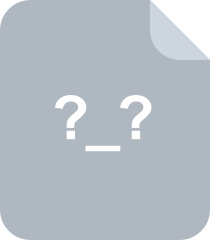
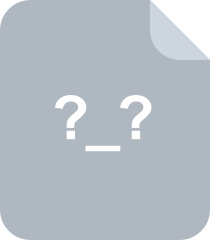
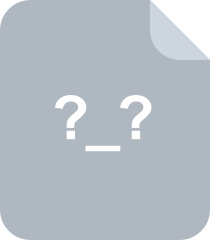
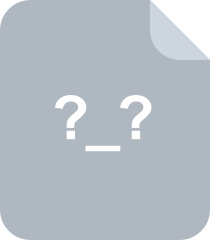
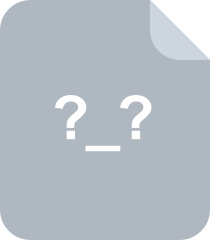
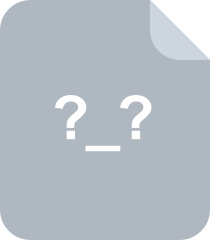
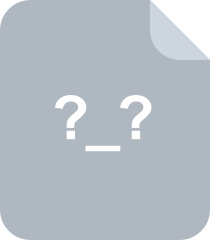
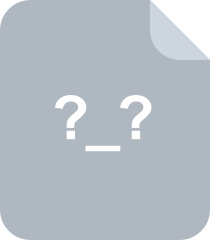
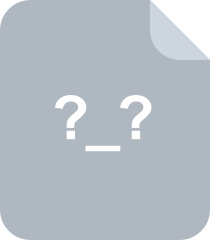
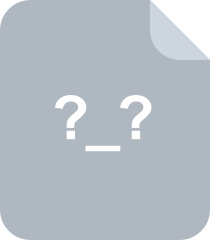
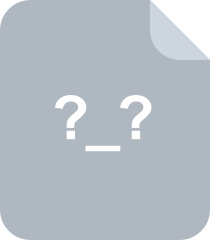
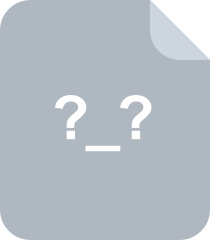
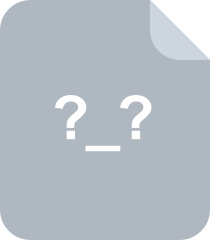
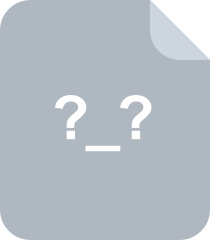
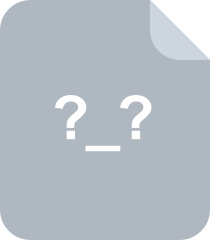
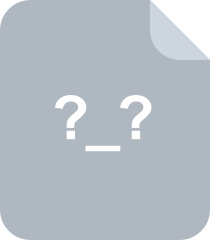
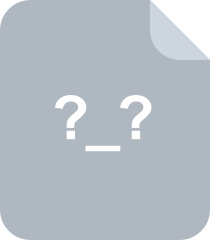
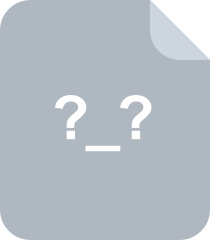
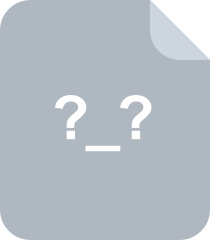
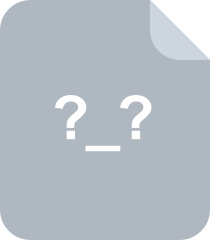
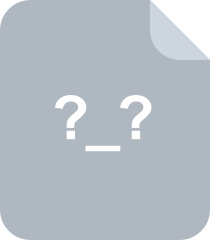
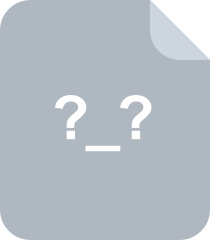
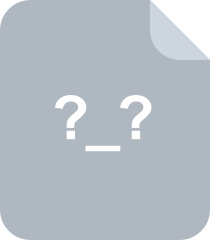
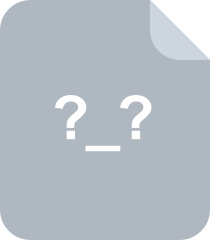
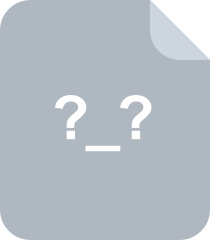
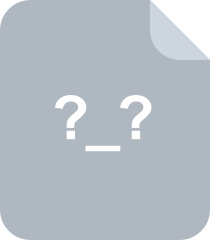
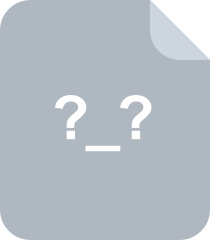
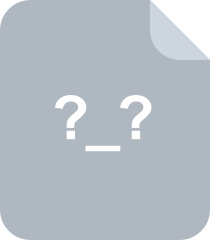
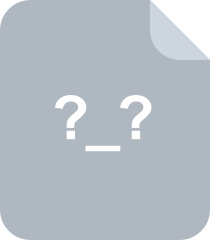
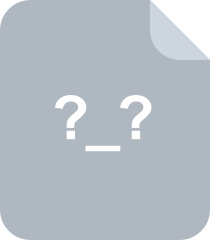
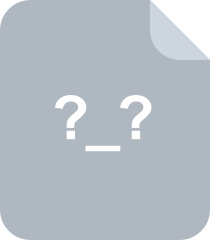
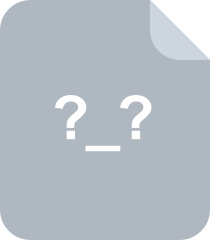
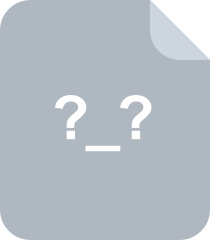
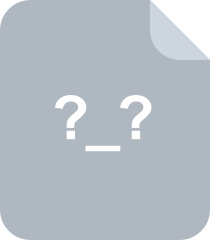
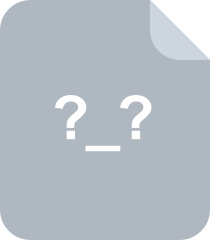
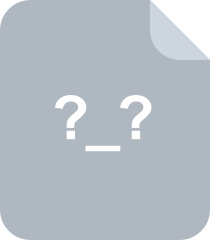
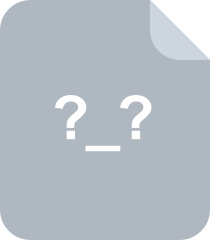
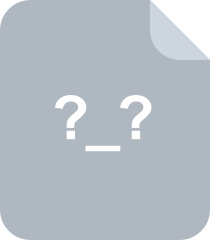
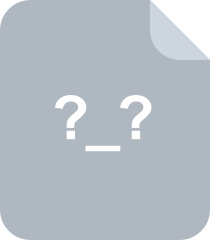
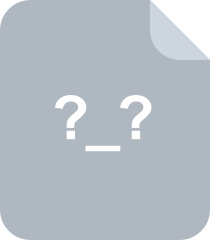
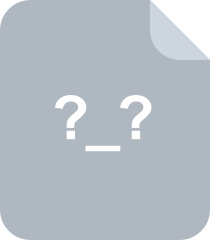
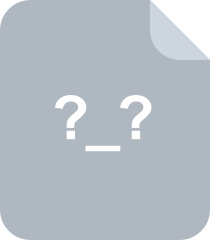
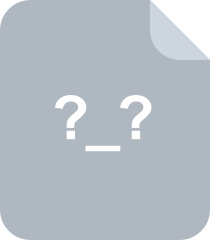
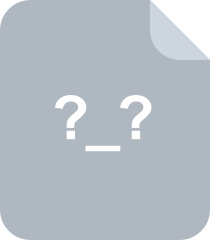
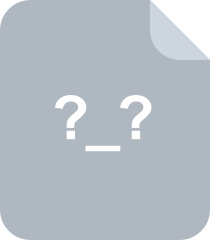
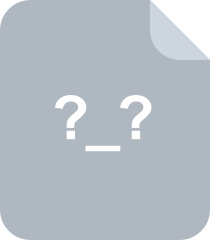
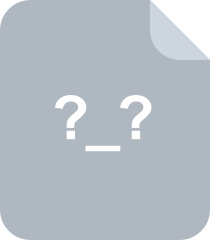
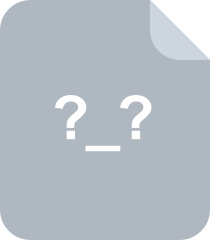
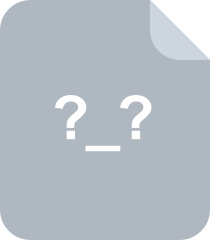
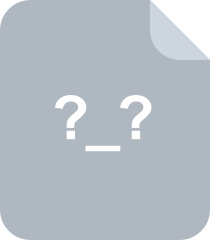
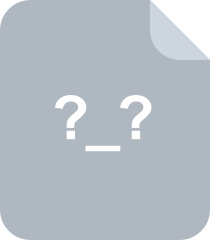
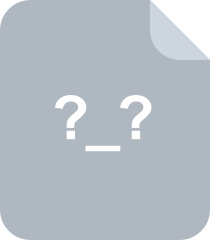
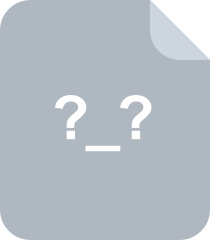
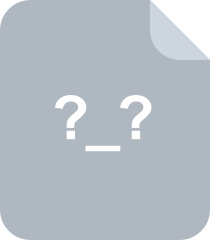
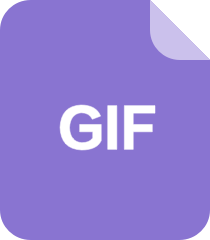
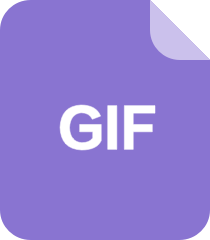
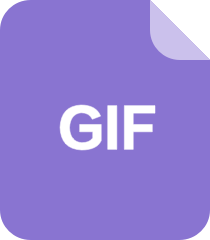
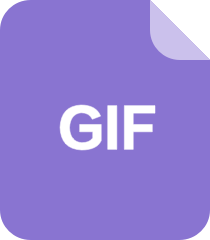
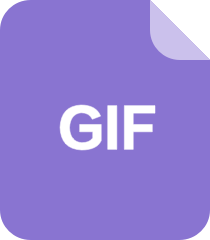
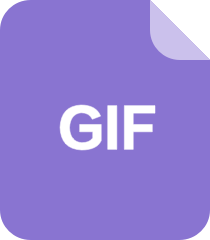
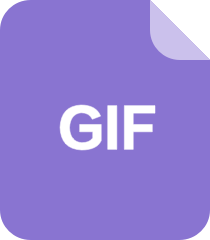
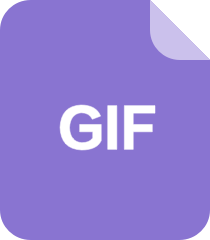
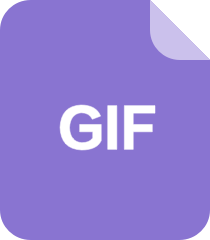
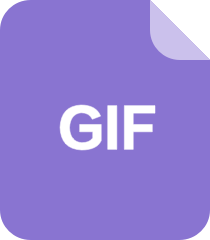
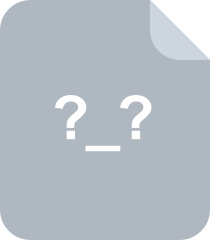
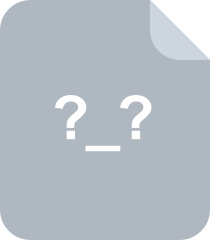
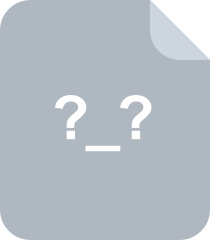
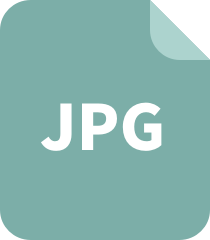
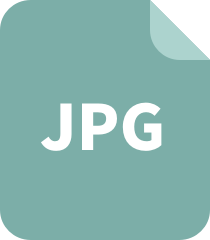
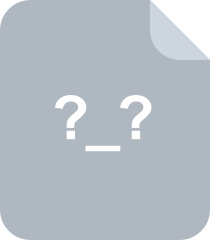
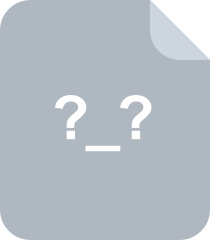
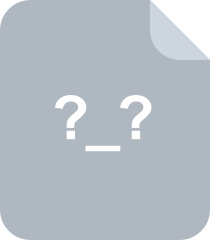
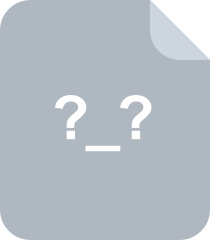
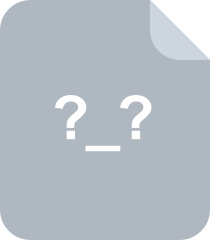
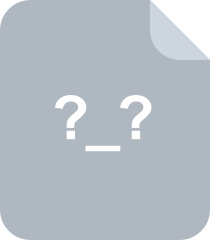
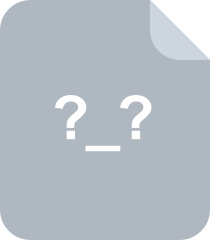
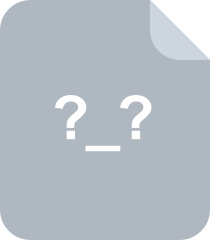
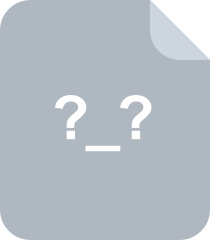
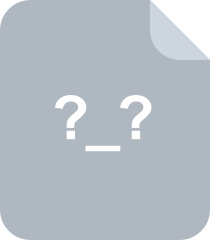
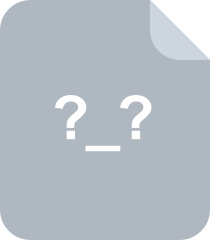
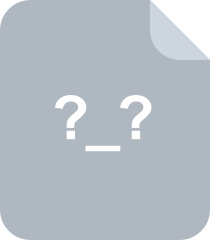
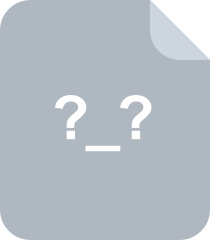
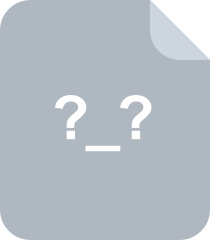
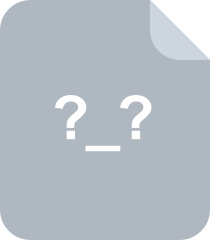
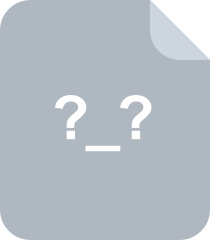
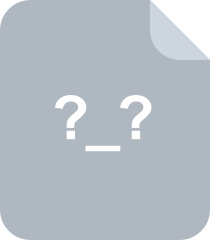
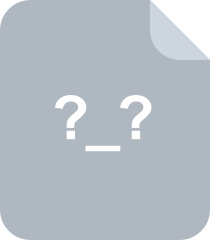
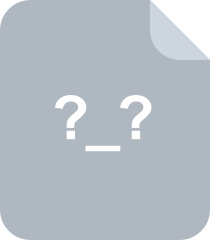
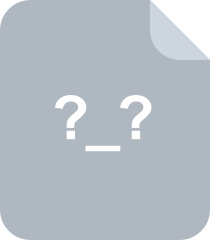
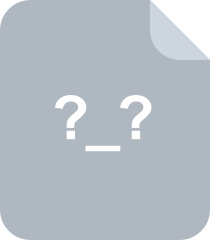
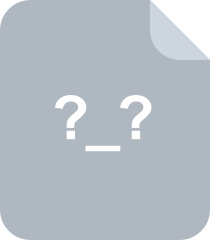
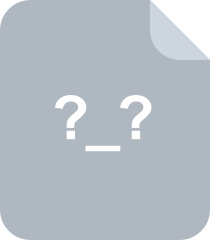
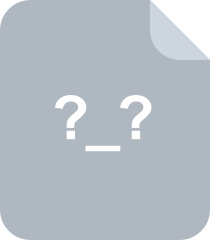
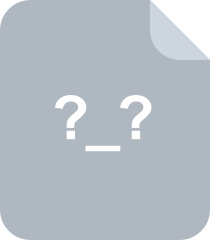
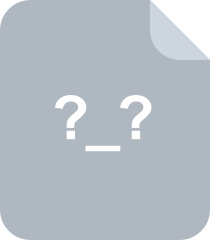
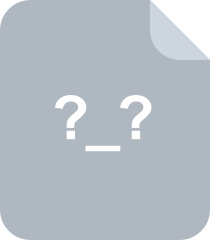
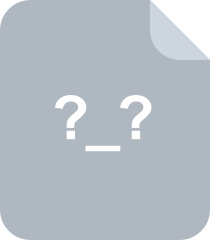
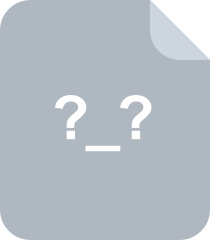
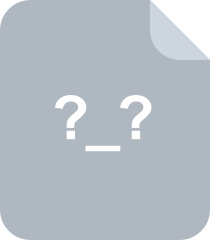
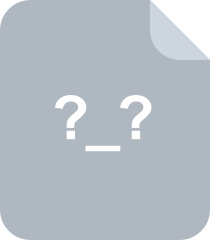
共 1108 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
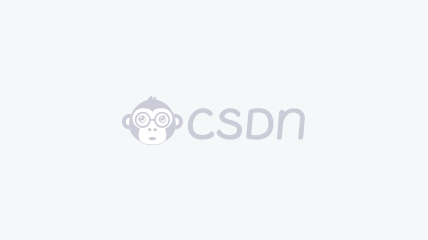


平底斜
- 粉丝: 435
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

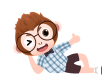
安全验证
文档复制为VIP权益,开通VIP直接复制
