clc;
clear;
close all
warning off;
data = xlsread('data.xlsx', 'Sheet1', 'A3:M1250');
x=data(:,1:12);
y=data(:,13);
% 求因子维度
[r,c]=size(x);
% 求因子均值
aver=mean(x);
% 求因子标准差
stdcov=std(x);
% 求目标均值
y_aver=mean(y);
% 求目标方差
y_stdcov=std(y);
% 数据标准化
stdarr=(x-aver(ones(r,1),:))./stdcov(ones(r,1),:);
std_y=(y-y_aver(ones(r,1)))./y_stdcov(ones(r,1));
% 计算相关系数矩阵
covArr=cov(stdarr);
%% 相关系数矩阵进行主成分分析
[pc1,latent,explain]=pcacov(covArr);
d=repmat(sign(sum(pc1)),size(pc1,1),1);
pc=pc1.*d;
sumcontr=0;
i=1;
% 选取贡献率大于90%的主成分
er=90;
while sumcontr<er
sumcontr=sumcontr+explain(i);
i=i+1;
end
pcNum=i-1;
% 计算各个主成分
F=stdarr*pc(:,1:pcNum);
FF=[ones(r,1),F];
Fcoeff=regress(std_y,FF);
Recoeff=pc(:,1:pcNum)*Fcoeff(2:pcNum+1,:);
result(1)=Fcoeff(1);
result(2:c+1)=Recoeff;
y_p=Fcoeff(1)+stdarr*Recoeff;
y_p=y_p.*y_stdcov(ones(r,1))+y_aver(ones(r,1));
%% BiLSTM
%%
% 输入数据
input =F';
output=y';
nwhole =size(data,1);
temp=randperm(nwhole);
train_ratio=0.9;
ntrain=round(nwhole*train_ratio);
ntest =nwhole-ntrain;
% 准备输入和输出训练数据
input_train =input(:,temp(1:ntrain));
output_train=output(:,temp(1:ntrain));
% 准备测试数据
input_test =input(:, temp(ntrain+1:ntrain+ntest));
output_test=output(:,temp(ntrain+1:ntrain+ntest));
%% 归一化(全部特征 均归一化)
[inputn_train,inputps] =mapminmax(input_train);
[outputn_train,outputps]=mapminmax(output_train);
inputn_test=mapminmax('apply',input_test,inputps);
%% BiLSTM层设置,参数设置
inputSize = size(inputn_train,1); %数据输入x的特征维度
outputSize = size(outputn_train,1); %数据输出y的维度
numhidden_units=240;
%% BiLSTM
%输入层设、学习层、全连接层
layers = [ ...
sequenceInputLayer(inputSize)
fullyConnectedLayer(2*inputSize)
lstmLayer(numhidden_units)
dropoutLayer(0.2)
fullyConnectedLayer(outputSize)
regressionLayer];
%% trainoption(BiLSTM)
%优化算法、训练次数、梯度阈值、运行环境、学习率、学习计划
opts = trainingOptions('adam', ...
'MaxEpochs',800, ...
'MiniBatchSize',48,...
'GradientThreshold',1,...
'ExecutionEnvironment','gpu',...
'InitialLearnRate',0.005, ...
'LearnRateSchedule','piecewise', ...
'LearnRateDropPeriod',100, ...
'LearnRateDropFactor',0.8, ...
'Verbose',0, ...
'Plots','training-progress'...
);
%% BiLSTM网络训练
tic
BiLSTMnet = trainNetwork(inputn_train,outputn_train,layers,opts);
toc;
[BiLSTMnet,BiLSTMoutputr_train]= predictAndUpdateState(BiLSTMnet,inputn_train);
BiLSTMoutput_train = mapminmax('reverse',BiLSTMoutputr_train,outputps);
%网络测试输出
[BiLSTMnet,BiLSTMoutputr_test] = predictAndUpdateState(BiLSTMnet,inputn_test);
%网络输出反归一化
BiLSTMoutput_test= mapminmax('reverse',BiLSTMoutputr_test,outputps);
%% BiLSTM数据输出
BiLSTMerror_test=BiLSTMoutput_test'-output_test';
BiLSTMpererror_test=BiLSTMerror_test./output_test';
RMSEtest = sqrt(sumsqr(output_test - BiLSTMoutput_test)/length(output_test));
MAPEtest = mean(abs(BiLSTMpererror_test));
%--------------------------------------------------------------------------
disp('PCA-BiLSTM神经网络测试平均绝对误差百分比MAPE');
disp(MAPEtest)
disp('PCA-BiLSTM神经网络测试均方根误差RMSE')
disp(RMSEtest)
%--------------------------------------------------------------------------
%% PCA可视化
%%
% 主成分贡献率分析
figure
percent_explained = 100*explain / sum(explain);
[H,ax] =pareto(percent_explained);
set(ax,'linewidth',2,'FontName','Times New Roman')
set(H(1),'FaceColor',[180 60 0]./255)
set(H(2),'linestyle','--','Marker','s','Color',[255 0 0]./255,'linewidth',2,'Markersize',6,'MarkerFaceColor',[255 0 0]./255)
xlabel('主成分')
ylabel('贡献率(%)')
title('主成分贡献率')
h = gca;
h.FontName = '黑体';
h.FontSize = 12;
%--------------------------------------------------------------------------
figure
plot(1:r,y,'k-','linewidth',0.8);
hold on
plot(1:r,y_p,'r-.','linewidth',1);
title('结果比较');
legend('原始数据','协方差主成分回归预测值','Location','Best');
legend box off
h = gca;
h.FontName = '黑体';
h.FontSize = 10;
axis tight
%-------------------------------------------------------------------------------------
%% PCA-BiLSTM可视化
%-------------------------------------------------------------------------------------
figure
plot(BiLSTMoutput_test,'-.','Color',[0 0 255]./255,'linewidth',1,'Markersize',3,'MarkerFaceColor',[0 0 255]./255)
hold on
plot(output_test,'-.','Color',[0 0 0]./255,'linewidth',0.8,'Markersize',3,'MarkerFaceColor',[0 0 0]./255)
legend( '测试数据','实际数据','Location','NorthWest','FontName','黑体');
title('PCA-BiLSTM网络模型测试结果及真实值','fontsize',12,'FontName','黑体')
xlabel('时间','fontsize',12,'FontName','黑体');
ylabel('数值','fontsize',12,'FontName','黑体');
axis tight
%-------------------------------------------------------------------------------------
figure
plot(BiLSTMpererror_test,'-.','Color',[128 0 255]./255,'linewidth',1)
legend('PCA-BiLSTM网络测试相对误差','Location','NorthEast','FontName','黑体')
title('PCA-BiLSTM网络模型测试相对误差','fontsize',12,'FontName','黑体')
ylabel('误差','fontsize',12,'FontName','黑体')
xlabel('样本','fontsize',12,'FontName','黑体')
axis tight
没有合适的资源?快使用搜索试试~ 我知道了~
【LSTM回归预测】基于matlab主成分分析结合LSTM数据回归预测【含Matlab源码 9884期】.zip
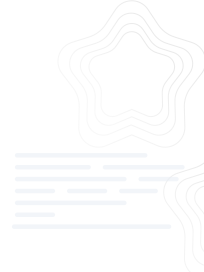
共6个文件
png:4个
xlsx:1个
m:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 109 浏览量
2024-12-18
15:47:56
上传
评论
收藏 462KB ZIP 举报
温馨提示
CSDN海神之光上传的全部代码均可运行,亲测可用,直接替换数据即可,适合小白; 1、代码压缩包内容 主函数:Main .m; 调用函数:其他m文件;无需运行 运行结果效果图; 2、代码运行版本 Matlab 2019b;若运行有误,根据提示修改;若不会,可私信博主; 3、运行操作步骤 步骤一:将所有文件放到Matlab的当前文件夹中; 步骤二:双击打开除Main.m的其他m文件; 步骤三:点击运行,等程序运行完得到结果; 4、仿真咨询 如需其他服务,可私信博主或扫描博主博客文章底部QQ名片; 4.1 CSDN博客或资源的完整代码提供 4.2 期刊或参考文献复现 4.3 Matlab程序定制 4.4 科研合作
资源推荐
资源详情
资源评论
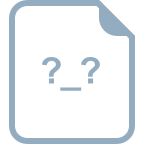
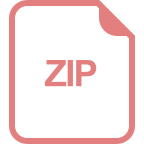
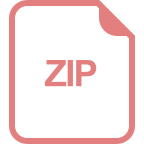
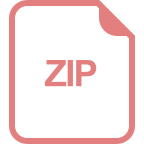
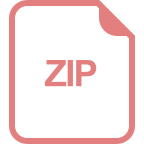
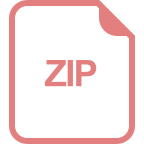
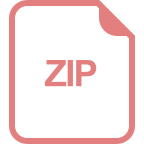
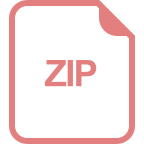
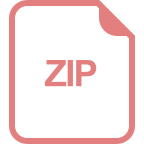
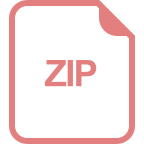
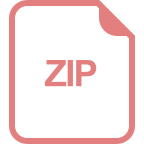
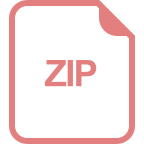
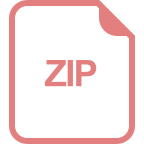
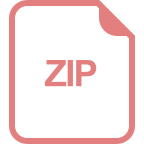
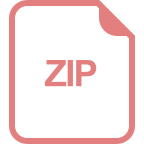
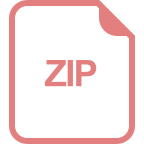
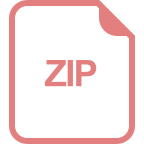
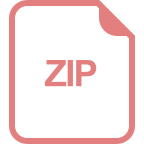
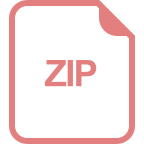
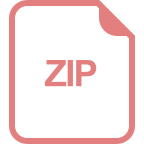
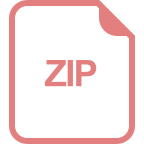
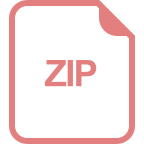
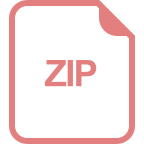
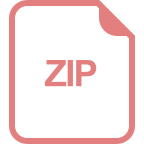
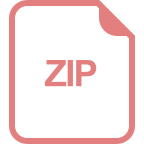
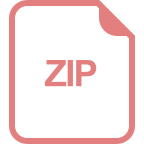
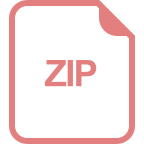
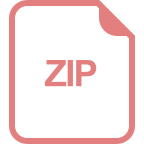
收起资源包目录


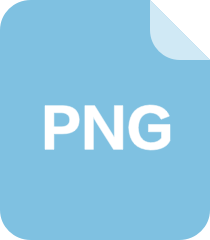
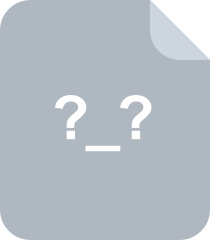
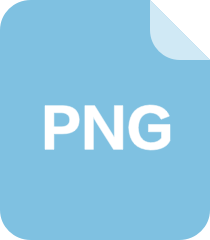
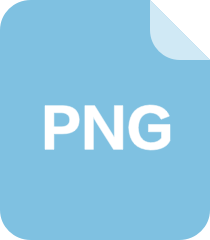
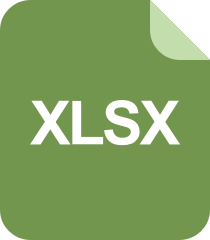
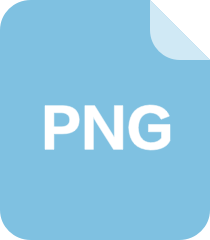
共 6 条
- 1
资源评论
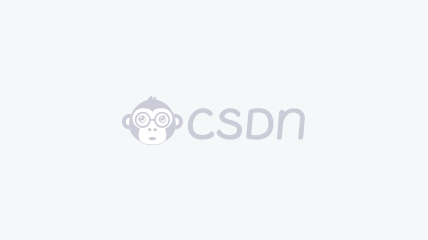


海神之光
- 粉丝: 5w+
- 资源: 6477
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

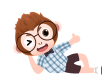
最新资源
- 企业级NoSql数据库REDIS集群
- 倍增发求LCA(最近公共祖先)
- 【2024年最新】基于jsp+mysql远程餐厅预约系统-毕业设计.7z
- 非常好看的二次元BT宝塔面板美化透明版主题包
- 一个 photoshop脚本 功能: 将photoshop的分层图片导入到spine
- 钢铁行业供需分析:淡季库存矛盾有限,价格预计震荡运行
- MCBOK - Strategy Implementation - 1st Edition-final Copyright.pdf
- Strategy Consultant’s Guide to Implementing Strategy
- 迪哲医药-U:专注小分子原始创新,差异化管线厚积薄发
- 图表作文模板@考研经验超市.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


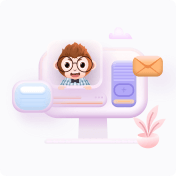
安全验证
文档复制为VIP权益,开通VIP直接复制
