<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>dom-plus</title>
<style>
#all{border: 1px dashed red; width: 600px; height: auto; margin: 0 auto;}
#add{border: 1px dashed red; width: 600px; height: auto; margin: 0 auto;}
#application{display: none;border: 1px dashed red;width: 600px;height: auto;margin: 0 auto;}
</style>
<script>
//增加一个用户的函数
var count=1;//计数的
var index=0;
function addOneData(){
var ul=document.createElement("ul");//创造一个ul标签元素
var li0=document.createElement("li");//游客的li
li0.setAttribute("name","msg");
var jp=document.createTextNode("第"+count+"位游客");
li0.appendChild(jp);
ul.appendChild(li0);
//---------------第一个游客加载完毕---------------------
var li1=document.createElement("li");//用户姓名li
var userNameInfo=document.createTextNode("用户姓名:");
var inputUserName=document.createElement("input");
inputUserName.setAttribute("type","text");
inputUserName.setAttribute("name","username");
//------------------------------
inputUserName.onblur=function(){
var v=this.value.trim();//拿到用户的姓名的取值
if(v==''){
spanUserName.innerHTML='用户名不允许为空';
return false;
}else{
spanUserName.innerHTML='用户名OK';
return true;
}
}
var spanUserName=document.createElement("span");
spanUserName.setAttribute("name","username1");
var msgUserNameInfo=document.createTextNode("请输入用户姓名");
//------------开始组装第二个li--------------
spanUserName.appendChild(msgUserNameInfo);//span标签加载信息提示
li1.appendChild(userNameInfo);
li1.appendChild(inputUserName);
li1.appendChild(spanUserName);
ul.appendChild(li1);
//-----------------编写身份证号相关信息--------------------
var li2=document.createElement("li");//身份证号li
var cardInfo=document.createTextNode("身份证号:");
var inputCardInfo=document.createElement("input");
inputCardInfo.setAttribute("type","text");
inputCardInfo.setAttribute("name","cardNumber");
//--------------设置身份证号的onBlur事件---------------
inputCardInfo.onblur=function(){
var v=this.value.trim();
var reg = /^[1-9]\d{5}(18|19|([23]\d))\d{2}((0[1-9])|(10|11|12))(([0-2][1-9])|10|20|30|31)\d{3}[0-9Xx]$/; //身份证号的正则
if(!reg.test(v)){//== !=
spanCardInfo.innerHTML='身份证号不符合要求';
return false;
}else{
spanCardInfo.innerHTML='身份证号OK';
return true;
}
}
var spanCardInfo=document.createElement("span");
spanCardInfo.setAttribute("name","cardNumber1");
var msgCardInfo=document.createTextNode("请输入身份证号");
// //--------------开始组装第三个身份证的li-------------------
spanCardInfo.appendChild(msgCardInfo);//span标签加载信息提示
li2.appendChild(cardInfo);
li2.appendChild(inputCardInfo);
li2.appendChild(spanCardInfo);
ul.appendChild(li2);
//-----------------编写用户头像关信息--------------------
var li3=document.createElement("li");//用户头像li
var userPhoto=document.createTextNode("用户头像:");
var inputPhotoInfo=document.createElement("input");
inputPhotoInfo.setAttribute("type","file");
inputPhotoInfo.setAttribute("name","file");
inputPhotoInfo.onchange=function(){
var fileName=inputPhotoInfo.files[0].name;
var fileSize=inputPhotoInfo.files[0].size;
var type=inputPhotoInfo.files[0].type;
//console.log(type);
if(type!='image/png'&type!='image/jpeg'){
photoInfo.innerHTML='请上传图片类型';
return false;
}else if(fileSize>1049329){
photoInfo.innerHTML='请控制上传图片的大小在1MB以内';
return false;
}else{
photoInfo.innerHTML='图片OK';
return true;
}
}
var photoInfo=document.createElement("span");
photoInfo.setAttribute("name","file1");
var msgPhotoInfo=document.createTextNode("请上传头像");
// //--------------开始组装第四个头像的li-------------------
photoInfo.appendChild(msgPhotoInfo);//span标签加载信息提示
li3.appendChild(userPhoto);
li3.appendChild(inputPhotoInfo);
li3.appendChild(photoInfo);
ul.appendChild(li3);
//--------------------开始第5个li(性别)的创建----------------------------------
var li4=document.createElement("li");//用户性别li
var userSex=document.createTextNode("用户性别:");
var inputSex1=document.createElement("input");
inputSex1.setAttribute("type","radio");
inputSex1.setAttribute("name","sex"+index);//name的值是每一组为一个用户
inputSex1.setAttribute("value",1);
inputSex1.setAttribute("checked","checked");
var manSex=document.createTextNode("男");
//---------------组装女------------------
var inputSex2=document.createElement("input");
inputSex2.setAttribute("type","radio");
inputSex2.setAttribute("name","sex"+index);//name的值是每一组为一个用户
inputSex2.setAttribute("value",0);
var womanSex=document.createTextNode("女");
//--------------开始组装性别------------
li4.appendChild(userSex);
li4.appendChild(inputSex1);
li4.appendChild(manSex)
li4.appendChild(inputSex2);
li4.appendChild(womanSex)
ul.appendChild(li4);
//-------------接下来开始创建手机的组件了------------------
var li5=document.createElement("li");//手机号li
var phoneInfo=document.createTextNode("手机号:");
var inputPhone=document.createElement("input");
inputPhone.setAttribute("type","text");
inputPhone.setAttribute("name","phone");
var spanPhone=document.createElement("span");
spanPhone.setAttribute("name","phone1");
var msgPhoneInfo=document.createTextNode("请输入手机号");
//------------开始组装第手机的li--------------
spanPhone.appendChild(msgPhoneInfo);//span标签加载信息提示
li5.appendChild(phoneInfo);
li5.appendChild(inputPhone);
li5.appendChild(spanPhone);
ul.appendChild(li5);
//--------------开始创建最后一个li--------------------
var li6=document.createElement("li");
var delBtn=document.createElement("input");
delBtn.setAttribute("type","button");
delBtn.setAttribute("value","删除");
//编写删除按钮的删除事件
delBtn.onclick=function(){
document.getElementById("all").removeChild(ul);//移除ul
count--;//是为了重新排序
var box=document.getElementsByName("msg");
for(var i=0;i<box.length;i++){
box[i].innerHTML="第"+(i+1)+"位游客";
}
//接下来还需要操作是否开启我的报名按钮
var str=document.getElementById("all").innerHTML.trim();
if(str==''){
document.getElementById("application").style.display='none';
}else{
document.getElementById("application").style.display='block';
}
}
li6.appendChild(delBtn);
ul.appendChild(li6);
count++;
index++;
document.getElementById("application").style.display='block';
document.getElementById("all").appendChild(ul);
}
//判定用户名称是否每一个用户都输入了内容
function check(){
//找到所有的用户的姓名的onBlur事件,主动触发
var str1=true;//默认全部通过 用户姓名
var str2=true;//用户的身份证号
var str3=true;//用户的头像
var box=document.getElementsByName("username");
for(var i=0;i<box.length;i++){
var result=box[i].onblur();//得到,
if(result==false){
str1=false;
continue;
}
}
//cardNumber
var box1=do

SuSan1648077
- 粉丝: 0
- 资源: 12
最新资源
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
- (源码)基于计算机系统原理与Arduino技术的学习平台.zip
- (源码)基于SSM框架的大学消息通知系统服务端.zip
- (源码)基于Java Servlet的学生信息管理系统.zip
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip
- (源码)基于Java的DVD管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


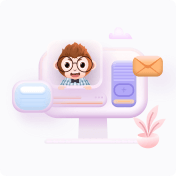