import numpy as np
import math
import random
import heapq
from test_func import *
import matplotlib.pyplot as plt
class wpa():
def __init__(self, pop_size=50, n_dim=2, alpha=4, beta=6, w=100, lb=-1e5, ub=1e5, max_iter=300, func=None):
self.pop = pop_size
self.n_dim = n_dim
self.func = func
self.max_iter = max_iter # max iter
self.alpha = alpha # 探狼比例因子 (取[n/(α+1),n/α]之间的整数)
self.beta = beta # 狼群更新比例因子
self.w = w # 距离判定因子
self.S = 1000 # 步长因子
self.number_T = np.random.randint(self.pop / (self.alpha + 1), self.pop / self.alpha) # 探狼数量
self.h = 10 # 探狼探寻方向总数
self.T_max = 30 # 最大游走次数
self.lb, self.ub = np.array(lb) * np.ones(self.n_dim), np.array(ub) * np.ones(self.n_dim)
assert self.n_dim == len(self.lb) == len(self.ub), 'dim == len(lb) == len(ub) is not True'
assert np.all(self.ub > self.lb), 'upper-bound must be greater than lower-bound'
self.step_a = (self.ub - self.lb) / self.S # 探狼游走步长
self.step_b = 2 * self.step_a # 猛狼奔袭步长
self.step_c = self.step_a / 2 # 围攻步长
self.X = np.random.uniform(low=self.lb, high=self.ub, size=(self.pop, self.n_dim))
self.Y = [self.func(self.X[i]) for i in range(len(self.X))] # y = f(x) for all particles
self.pbest_x = self.X.copy() # personal best location of every particle in history
self.pbest_y = [self.Y[i] for i in range(self.pop)] # best image of every particle in history
self.gbest_x = self.pbest_x.mean(axis=0).reshape(1, -1) # global best location for all particles
self.gbest_y = np.inf # global best y for all particles
self.gbest_y_hist = [] # gbest_y of every iteration
self.update_gbest()
def update_pbest(self):
'''
personal best
:return:
'''
# self.need_update = self.pbest_y > self.Y
# print(self.need_update)
# print(self.pbest_y)
# print(self.Y)
for i in range(len(self.Y)):
if self.pbest_y[i] > self.Y[i]:
self.pbest_x[i] = self.X[i]
self.pbest_y[i] = self.Y[i]
# self.pbest_x = np.where(self.need_update, self.X, self.pbest_x)
# self.pbest_y = np.where(self.need_update, self.Y, self.pbest_y)
# print(self.pbest_x)
def update_gbest(self):
'''
global best
:return:
'''
idx_min = self.pbest_y.index(min(self.pbest_y))
if self.gbest_y > self.pbest_y[idx_min]:
self.gbest_x = self.X[idx_min, :].copy()
self.gbest_y = self.pbest_y[idx_min]
def update(self):
L_Wolf_Y = np.min(self.Y) # 记录头狼的值
L_Wolf_index = np.argmin(self.Y) # 头狼在狼群(wol)f_colony_X)中下标位置
L_Wolf_X = self.X[L_Wolf_index] # 记录头狼的变量信息
L_Wolf = [L_Wolf_Y, L_Wolf_index]
# 初始化探狼
T_Wolf_V = heapq.nsmallest(self.number_T, self.Y.copy())
T_Wolf = self.find_index(T_Wolf_V, self.Y) # 0:探狼在狼群中位置 1:解
# 初始化猛狼
M_Wolf_V = heapq.nlargest(self.pop - self.number_T - 1, self.Y.copy())
M_Wolf = self.find_index(M_Wolf_V, self.Y) # 0:探狼在狼群中位置 1:解
self.detective_wolf(T_Wolf, L_Wolf_Y, L_Wolf_X, L_Wolf)
# 需要修改,参考一种新的群体智能算法 狼群算法> 2013.11 式(3)
# 言w增大会加速算法收敛,但W过大会使得人工狼很难进入围攻行为,缺乏对猎物的精细搜索。
d_near = 0
for d in range(self.n_dim):
d_near += ((1 / (1 * self.w * self.n_dim)) * (self.ub[d] - self.lb[d])) # dnear值,判定距离
self.call_followers(d_near, M_Wolf, L_Wolf_Y, L_Wolf_X, L_Wolf_index)
self.siege(M_Wolf, L_Wolf_X, L_Wolf_Y, L_Wolf_index)
self.live()
def find_index(self, value, wolf_colony):
ret = np.zeros((len(value), 2))
for i in range(0, len(value)):
ret[i][0] = wolf_colony.index(value[i])
ret[i][1] = value[i]
return ret
def detective_wolf(self, T_Wolf, L_Wolf_Y, L_Wolf_X, L_Wolf):
# 探狼开始游走
for i in range(0, T_Wolf.shape[0]):
H = np.random.randint(2, self.h) # 尝试的方向
single_T_Wolf = self.X[int(T_Wolf[i][0])] # 当前探狼
optimum_value = T_Wolf[i][1] # 游走的最优解
optimum_position = single_T_Wolf.copy() # 游走最优位置
find = False
# 探狼游走行为 一旦探狼发现目标 -> 开始召唤
for t in range(0, self.T_max):
# 在初始位置朝H个方向试探,直到找到一个优解
for p in range(1, H + 1):
single_T_Wolf_trial = single_T_Wolf.copy()
# 一种新的群体智能算法 狼群算法> 2013.11 式(1)
# h越大探狼搜寻得越精细但同时速度也相对较慢
# 这个部分有点像果蝇的搜素
single_T_Wolf_trial = single_T_Wolf_trial + math.sin(2 * math.pi * p / H) * self.step_a # 根据原式不需要转化类型为int
# 一种基于领导者策略的狼群搜索算法> 2013.9 式(2)
# single_T_Wolf_trial = single_T_Wolf_trial + np.random.uniform(-1, 1,(single_T_Wolf_trial.shape[0],)) * self.step_a
single_T_Wolf_V = self.func(single_T_Wolf_trial) # [0]
# 探狼转变为头狼
if L_Wolf_Y > single_T_Wolf_V:
find = True
L_Wolf_Y = single_T_Wolf_V # 更新头狼解
L_Wolf_X = single_T_Wolf_trial
L_Wolf_index = int(T_Wolf[i][0]) # 更新头狼下标
self.X[L_Wolf_index] = single_T_Wolf_trial # 更新头狼位置参数
T_Wolf = np.delete(T_Wolf, i, axis=0) # 探狼转为头狼,发起召唤,删除探狼
break
elif optimum_value > single_T_Wolf_V:
optimum_value = single_T_Wolf_V
optimum_position = single_T_Wolf_trial
else:
# print(" > 第%d只探狼完成第%d次游走,未发现猎物" % ((i + 1), (t + 1))) # 记录上次的最优位置
single_T_Wolf = optimum_position
if find is True:
break
if find is True:
print("第%d只探狼发现猎物 %f" % (i, single_T_Wolf_V))
break
else:
# 若游走完成探狼没找到猎物,更新所有游走过程中最优的一次位置
self.X[int(T_Wolf[i][0])] = optimum_position
def call_followers(self, d_near, M_Wolf, L_Wolf_Y, L_Wolf_X, L_Wolf_index):
# 召唤行为
surrounded = False
# 所有猛狼进入围攻范围才能结束
while ~surrounded:
ready = 0
for m in range(0, M_Wolf.shape[0]):
s_m_index = int(M_Wolf[m][0]) # 猛狼在狼群中下标
single_M_Wolf = self.X[s_m_index] # 猛狼变量
d = np.abs(L_Wolf_X - single_M_Wolf)
dd = np.sum(d)
while d_near < dd:
for d in range(self.n_dim):
single_M_Wolf[d] = single_M_Wolf[d] + self.step_b[d] * (L_Wolf_X[d] - single_M_Wolf[d]) / np.abs(L_Wolf_X[d] - single_M_Wolf[d])
single_M_Wolf_V = self.func(single_M_Wolf)
# 更新猛狼位置
self.X[s_m_index] = single_M_Wolf
M_Wolf[m][1] = single_M
没有合适的资源?快使用搜索试试~ 我知道了~
基于python的狼群搜索算法WPA
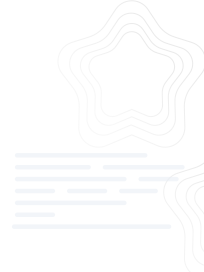
共1个文件
py:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
基于python的狼群搜索算法WPA
资源详情
资源评论
资源推荐
收起资源包目录

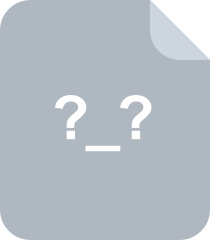
共 1 条
- 1
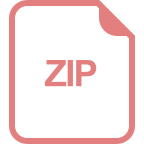
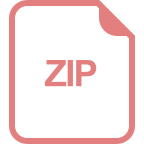
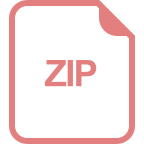
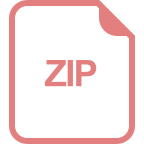
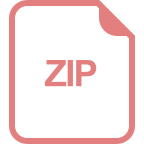
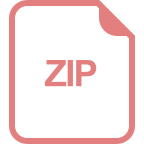
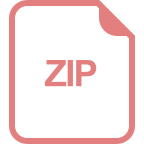
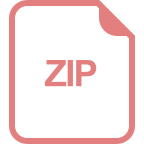
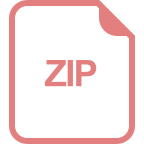
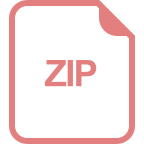
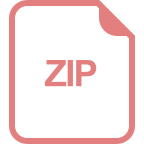
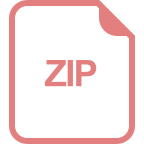
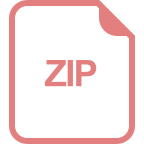
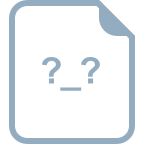
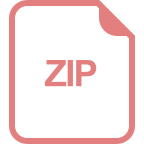

Sherry_shiry
- 粉丝: 2
- 资源: 1097
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

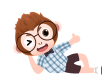
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1