/*
* Copyright 2012 Bill La Forge
*
* This file is part of AgileWiki and is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License (LGPL) as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This code is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
* or navigate to the following url http://www.gnu.org/licenses/lgpl-2.1.txt
*
* Note however that only Scala, Java and JavaScript files are being covered by LGPL.
* All other files are covered by the Common Public License (CPL).
* A copy of this license is also included and can be
* found as well at http://www.opensource.org/licenses/cpl1.0.txt
*/
package org.agilewiki.jfile.transactions.db.inMemory;
import org.agilewiki.jactor.Actor;
import org.agilewiki.jactor.ExceptionHandler;
import org.agilewiki.jactor.MailboxFactory;
import org.agilewiki.jactor.RP;
import org.agilewiki.jactor.factory.JAFactory;
import org.agilewiki.jfile.ForceBeforeWriteRootJid;
import org.agilewiki.jfile.JFile;
import org.agilewiki.jfile.block.Block;
import org.agilewiki.jfile.block.LTA32Block;
import org.agilewiki.jfile.transactions.db.DB;
import org.agilewiki.jid.JidFactories;
import org.agilewiki.jid._Jid;
import org.agilewiki.jid.collection.vlenc.map.StringMapJid;
import org.agilewiki.jid.scalar.flens.bool.BooleanJid;
import org.agilewiki.jid.scalar.flens.dbl.DoubleJid;
import org.agilewiki.jid.scalar.flens.flt.FloatJid;
import org.agilewiki.jid.scalar.flens.integer.IntegerJid;
import org.agilewiki.jid.scalar.flens.lng.LongJid;
import org.agilewiki.jid.scalar.vlens.actor.ActorJid;
import org.agilewiki.jid.scalar.vlens.actor.RootJid;
import org.agilewiki.jid.scalar.vlens.bytes.BytesJid;
import org.agilewiki.jid.scalar.vlens.string.StringJid;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
/**
* In-memory database.
*/
public class IMDB extends DB {
public static final String LOG_POSITION = "$$LOG_POSITION";
public static final String LOG_FILE_NAME = "$$LOG_FILE_NAME";
private JFile dbFile;
private RootJid rootJid;
private StringMapJid stringMapJid;
private boolean pendingWrite;
private boolean isFirstRootJid;
public int maxSize;
private boolean closed;
private boolean online;
public IMDB() {}
public IMDB(MailboxFactory mailboxFactory, Actor parent, Path directoryPath, int maxSize)
throws Exception {
super(mailboxFactory, parent, directoryPath);
this.maxSize = maxSize;
}
@Override
protected boolean generateCheckpoints() {
return online;
}
@Override
public void openDbFile(int logReaderMaxSize, RP rp)
throws Exception {
if (dbFile == null) {
dbFile = new JFile();
dbFile.initialize(getMailboxFactory().createAsyncMailbox());
}
Path dbPath = directoryPath.resolve("imdb.jadb");
dbFile.open(
dbPath,
StandardOpenOption.READ,
StandardOpenOption.WRITE,
StandardOpenOption.CREATE);
initializeDb(logReaderMaxSize);
Block block0 = newDbBlock();
block0.setCurrentPosition(0L);
block0.setFileName(dbPath.toString());
dbFile.readRootJid(block0, maxSize);
RootJid rootJid0 = block0.getRootJid(getMailboxFactory().createMailbox(), getParent());
long timestamp0 = block0.getTimestamp();
Block block1 = newDbBlock();
block1.setCurrentPosition(maxSize);
block1.setFileName(dbPath.toString());
dbFile.readRootJid(block1, maxSize);
RootJid rootJid1 = block1.getRootJid(getMailboxFactory().createMailbox(), getParent());
long timestamp1 = block0.getTimestamp();
if (rootJid0 == null) {
rootJid = rootJid1;
isFirstRootJid = false;
} else if (rootJid1 == null) {
rootJid = rootJid0;
isFirstRootJid = true;
} else if (timestamp0 < timestamp1) {
rootJid = rootJid1;
isFirstRootJid = false;
} else {
rootJid = rootJid0;
isFirstRootJid = true;
}
Long position = getLong(LOG_POSITION);
if (position == null) {
processLogFile(0L, 0, rp);
return;
}
String logFileName = getString(LOG_FILE_NAME);
int fileIndex = 0;
while (fileIndex < logFileNames.length && !logFileNames[fileIndex].equals(logFileName))
fileIndex += 1;
if (fileIndex == logFileNames.length)
throw new IllegalStateException("Missing log file: " + logFileName);
processLogFile(position, fileIndex, rp);
online = true;
}
@Override
public void closeDbFile() {
super.closeDbFile();
closed = true;
dbFile.close();
}
protected Block newDbBlock() {
return new LTA32Block();
}
protected RootJid makeRootJid() throws Exception {
if (rootJid == null) {
JAFactory factory = (JAFactory) getAncestor(JAFactory.class);
rootJid = (RootJid) factory.newActor(
JidFactories.ROOT_JID_TYPE,
getMailboxFactory().createMailbox(),
getParent());
}
return rootJid;
}
public StringMapJid getStringMapJid()
throws Exception {
if (rootJid == null)
return null;
if (stringMapJid == null) {
stringMapJid = (StringMapJid) rootJid.getValue();
}
return stringMapJid;
}
public StringMapJid makeStringMapJid()
throws Exception {
if (stringMapJid == null) {
RootJid rj = makeRootJid();
stringMapJid = (StringMapJid) rj.getValue();
if (stringMapJid == null) {
rj.setValue(JidFactories.STRING_ACTOR_MAP_JID_TYPE);
stringMapJid = (StringMapJid) rj.getValue();
}
}
return stringMapJid;
}
public ActorJid getActorJid(String key)
throws Exception {
StringMapJid smj = getStringMapJid();
if (smj == null)
return null;
return (ActorJid) smj.kGet(key);
}
public ActorJid makeActorJid(String key)
throws Exception {
StringMapJid smj = makeStringMapJid();
ActorJid actorJid = (ActorJid) smj.kGet(key);
if (actorJid == null) {
smj.kMake(key);
actorJid = (ActorJid) smj.kGet(key);
}
return actorJid;
}
public _Jid getJid(String key)
throws Exception {
ActorJid aj = getActorJid(key);
if (aj == null)
return null;
_Jid jid = aj.getValue();
return jid;
}
public _Jid makeJid(String key, String factoryName)
throws Exception {
ActorJid aj = makeActorJid(key);
_Jid jid = aj.getValue();
if (jid == null) {
aj.setValue(factoryName);
jid = aj.getValue();
}
return jid;
}
public BooleanJid getBooleanJid(String key)
throws Exception {
return (BooleanJid) getJid(key);
}
public Boolean getBoolean(String key)
throws Exception {
BooleanJid ij = getBooleanJid(key);
if (ij == null)
return null;
return ij.getValue();
}
public BooleanJid makeBooleanJid(String key)
throws Exception {
return
没有合适的资源?快使用搜索试试~ 我知道了~
小程序 JActor的文件持久化组件 JFile(源码).zip
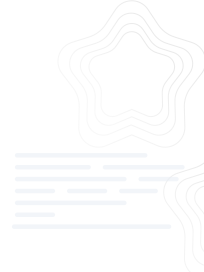
共76个文件
java:72个
xml:2个
readme:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 44 浏览量
2023-02-03
13:24:45
上传
评论
收藏 93KB ZIP 举报
温馨提示
免责声明:资料部分来源于合法的互联网渠道收集和整理,部分自己学习积累成果,供大家学习参考与交流。收取的费用仅用于收集和整理资料耗费时间的酬劳。 本人尊重原创作者或出版方,资料版权归原作者或出版方所有,本人不对所涉及的版权问题或内容负法律责任。如有侵权,请举报或通知本人删除。
资源推荐
资源详情
资源评论
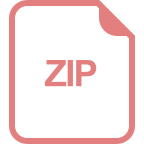
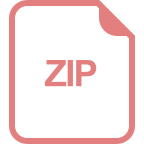
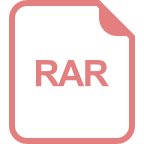
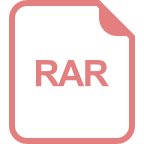
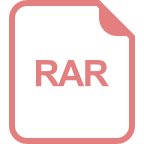
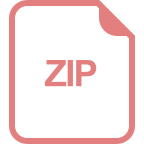
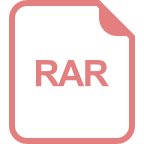
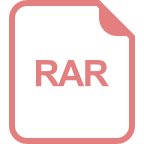
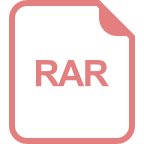
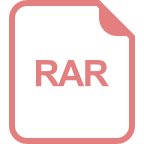
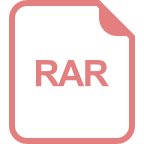
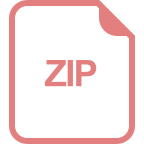
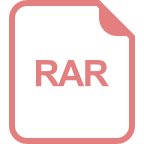
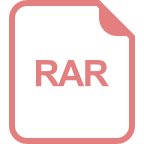
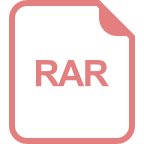
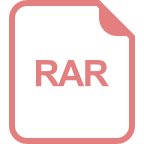
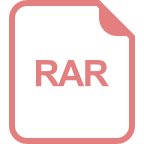
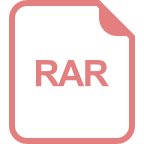
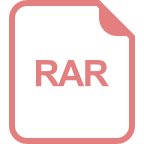
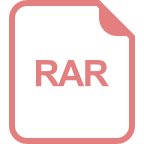
收起资源包目录


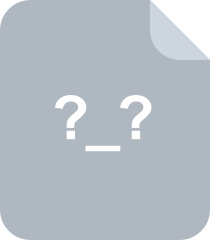
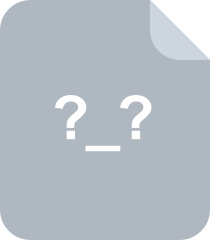






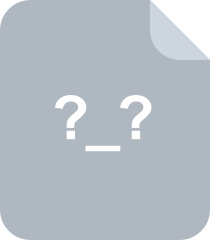

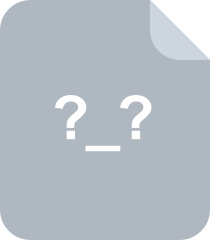
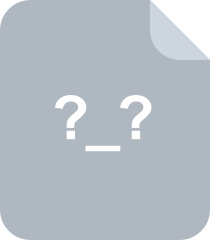
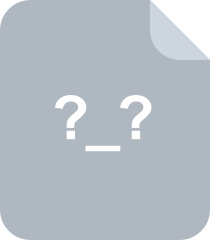
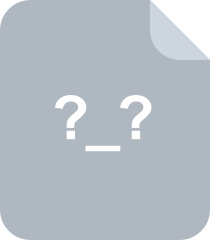
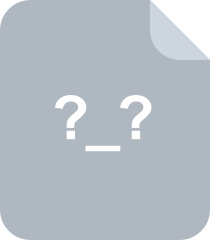
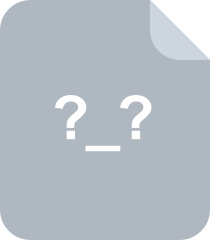

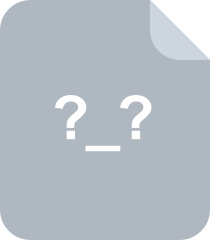
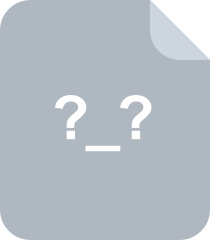


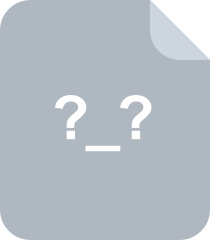
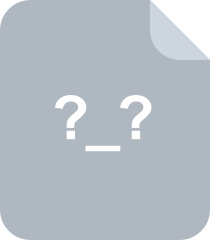
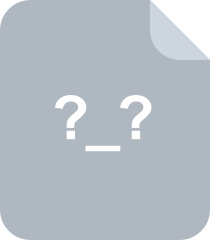
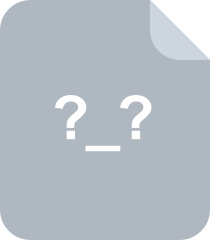
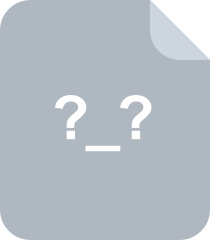
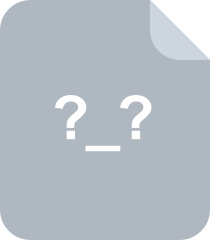
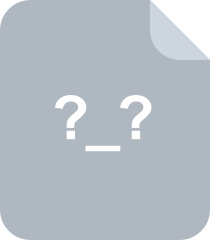
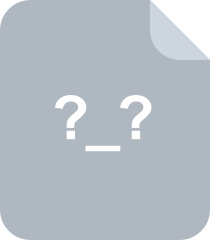

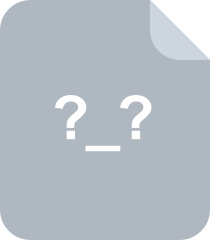
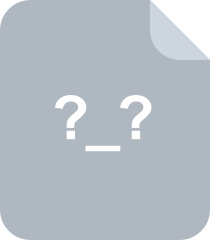
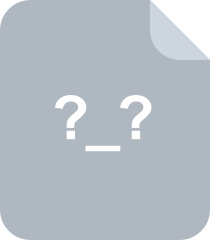
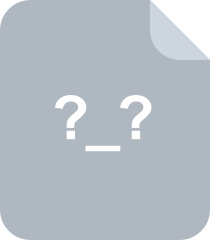

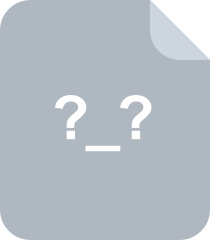





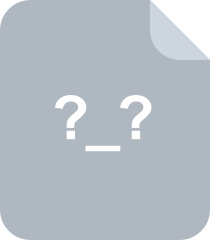
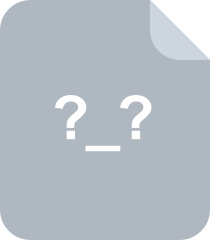
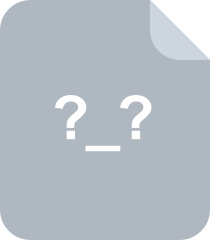

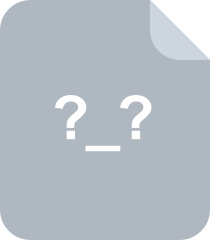
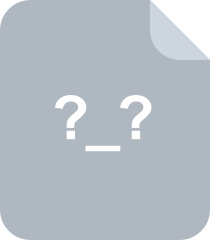
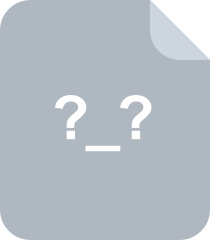
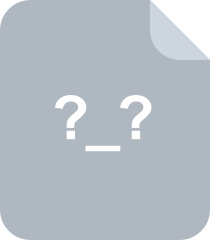
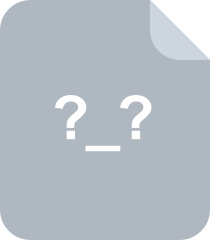
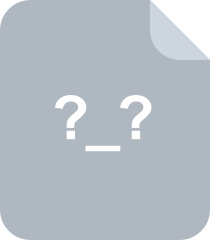

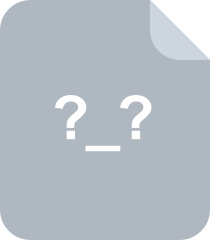
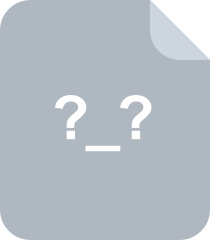
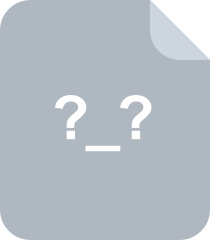
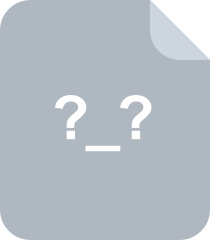
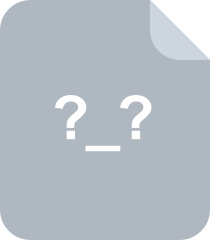
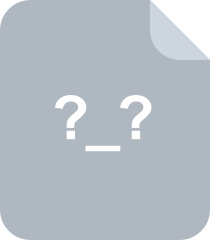
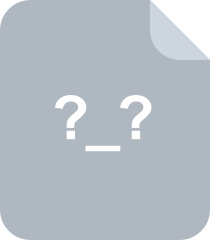
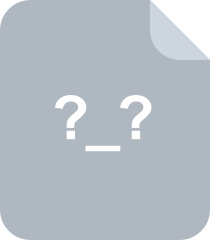
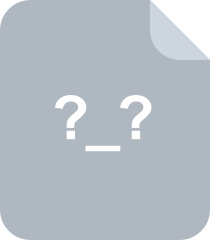
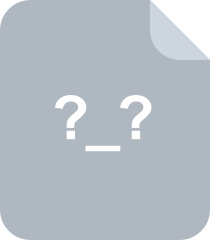
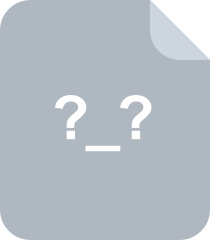
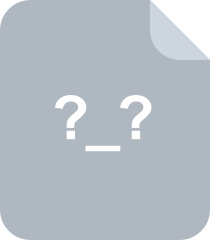
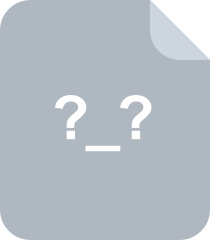
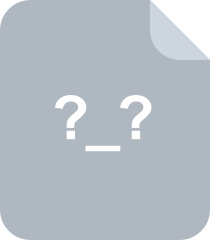
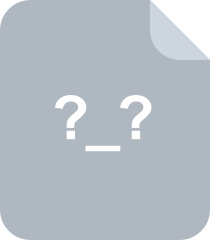
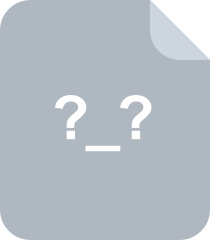

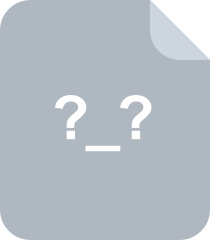
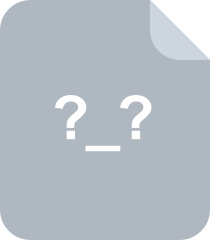
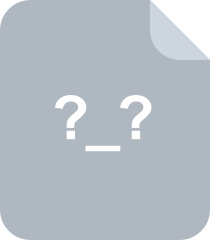
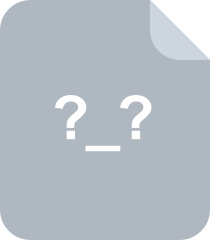

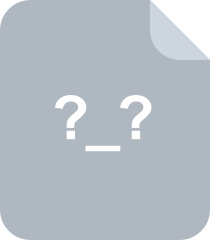
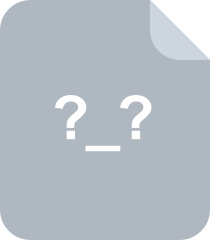

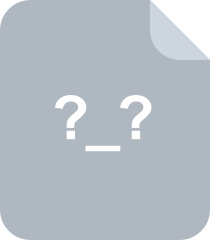
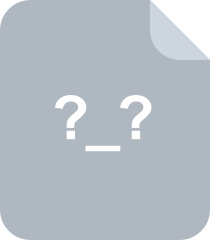
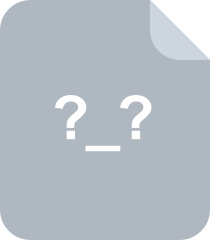
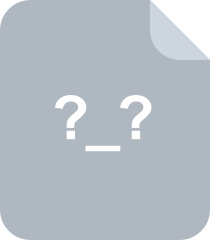
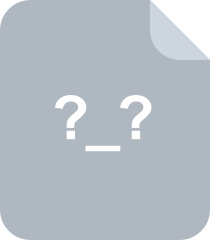
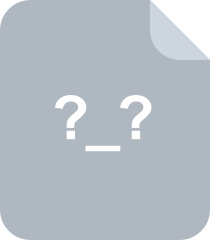
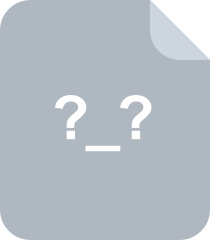
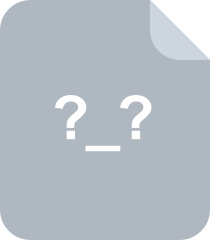
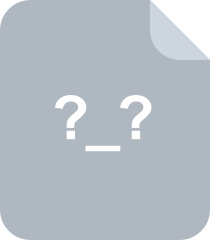
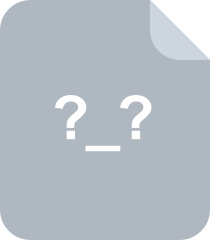

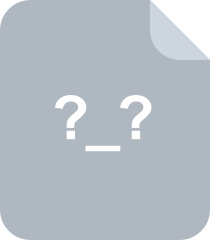
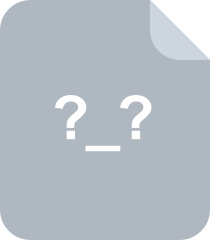
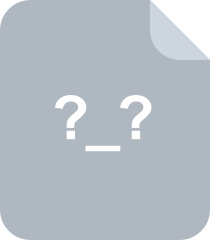
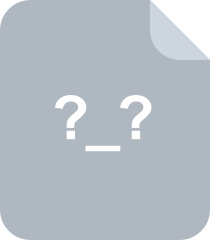
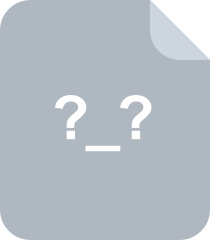
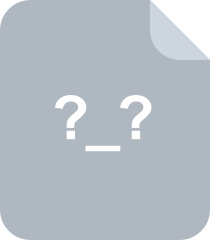
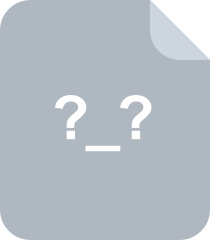
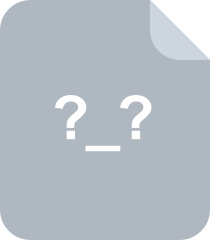
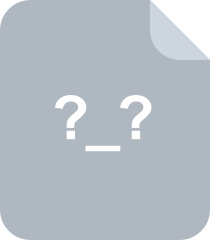
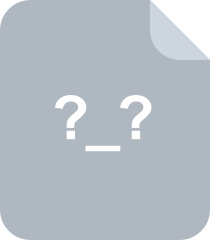
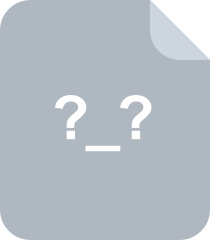
共 76 条
- 1
资源评论
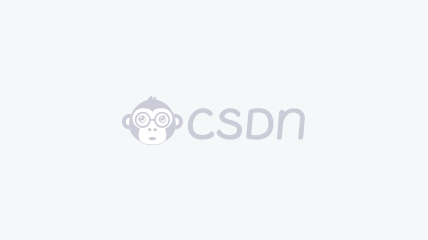

大富大贵7
- 粉丝: 390
- 资源: 8868

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

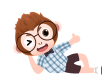
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


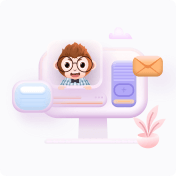
安全验证
文档复制为VIP权益,开通VIP直接复制
