// DO NOT EDIT THIS FILE - it is automatically generated, edit file under project/java dir
// This string is autogenerated by ChangeAppSettings.sh, do not change spaces amount
package com.xianle.traffic_sh;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.zip.CRC32;
import java.util.zip.CheckedInputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import android.content.res.Resources;
import android.widget.TextView;
class CountingInputStream extends BufferedInputStream {
private long bytesReadMark = 0;
private long bytesRead = 0;
public CountingInputStream(InputStream in, int size) {
super(in, size);
}
public CountingInputStream(InputStream in) {
super(in);
}
public long getBytesRead() {
return bytesRead;
}
public synchronized int read() throws IOException {
int read = super.read();
if (read >= 0) {
bytesRead++;
}
return read;
}
public synchronized int read(byte[] b, int off, int len) throws IOException {
int read = super.read(b, off, len);
if (read >= 0) {
bytesRead += read;
}
return read;
}
public synchronized long skip(long n) throws IOException {
long skipped = super.skip(n);
if (skipped >= 0) {
bytesRead += skipped;
}
return skipped;
}
public synchronized void mark(int readlimit) {
super.mark(readlimit);
bytesReadMark = bytesRead;
}
public synchronized void reset() throws IOException {
super.reset();
bytesRead = bytesReadMark;
}
}
/**
* @author Administrator
*
*/
class DataDownloader extends Thread
{
class StatusWriter
{
private TextView Status;
private MainActivity Parent;
private String oldText = "";
public StatusWriter( TextView _Status, MainActivity _Parent )
{
Status = _Status;
Parent = _Parent;
}
public void setParent( TextView _Status, MainActivity _Parent )
{
synchronized(DataDownloader.this) {
Status = _Status;
Parent = _Parent;
setText( oldText );
}
}
public void setText(final String str)
{
class Callback implements Runnable
{
public TextView Status;
public String text;
public void run()
{
Status.setText(text);
}
}
synchronized(DataDownloader.this) {
Callback cb = new Callback();
oldText = new String(str);
cb.text = new String(str);
cb.Status = Status;
if( Parent != null && Status != null )
Parent.runOnUiThread(cb);
}
}
}
public DataDownloader( MainActivity _Parent, TextView _Status )
{
Parent = _Parent;
Status = new StatusWriter( _Status, _Parent );
//Status.setText( "Connecting to " + Globals.DataDownloadUrl );
outFilesDir = Globals.DataDir;
DownloadComplete = false;
this.start();
}
public void setStatusField(TextView _Status)
{
synchronized(this) {
Status.setParent( _Status, Parent );
}
}
@Override
public void run()
{
String [] downloadFiles = Globals.DataDownloadUrl.split("\\^");
for( int i = 0; i < downloadFiles.length; i++ )
{
if( downloadFiles[i].length() > 0 /*&& Globals.OptionalDataDownload.length > i && Globals.OptionalDataDownload[i] */)
if( ! DownloadDataFile(downloadFiles[i], "DownloadFinished-" + String.valueOf(i) + ".flag") )
{
DownloadFailed = true;
return;
}
}
DownloadComplete = true;
initParent();
}
public boolean DownloadDataFile(final String DataDownloadUrl, final String DownloadFlagFileName)
{
String [] downloadUrls = DataDownloadUrl.split("[|]");
if( downloadUrls.length < 2 )
return false;
Resources res = Parent.getResources();
String path = getOutFilePath(DownloadFlagFileName);
InputStream checkFile = null;
try {
checkFile = new FileInputStream( path );
} catch( FileNotFoundException e ) {
} catch( SecurityException e ) { };
if( checkFile != null )
{
try {
byte b[] = new byte[ Globals.DataDownloadUrl.getBytes("UTF-8").length + 1 ];
int readed = checkFile.read(b);
String compare = new String( b, 0, readed, "UTF-8" );
boolean matched = false;
//System.out.println("Read URL: '" + compare + "'");
for( int i = 1; i < downloadUrls.length; i++ )
{
//System.out.println("Comparing: '" + downloadUrls[i] + "'");
if( compare.compareTo(downloadUrls[i]) == 0 )
matched = true;
}
//System.out.println("Matched: " + String.valueOf(matched));
if( ! matched )
throw new IOException();
Status.setText( res.getString(R.string.download_unneeded) );
return true;
} catch ( IOException e ) {};
}
checkFile = null;
// Create output directory (not necessary for phone storage)
// System.out.println("Downloading data to: '" + outFilesDir + "'");
try {
(new File( outFilesDir )).mkdirs();
OutputStream out = new FileOutputStream( getOutFilePath(".nomedia") );
out.flush();
out.close();
}
catch( SecurityException e ) {}
catch( FileNotFoundException e ) {}
catch( IOException e ) {};
HttpResponse response = null;
HttpGet request;
long totalLen = 0;
CountingInputStream stream;
byte[] buf = new byte[16384];
boolean DoNotUnzip = false;
boolean FileInAssets = false;
String url = "";
int downloadUrlIndex = 1;
while( downloadUrlIndex < downloadUrls.length )
{
System.out.println("Processing download " + downloadUrls[downloadUrlIndex]);
url = new String(downloadUrls[downloadUrlIndex]);
DoNotUnzip = false;
if(url.indexOf(":") == 0)
{
url = url.substring( url.indexOf(":", 1) + 1 );
DoNotUnzip = true;
}
Status.setText( res.getString(R.string.connecting_to, url) );
if( url.indexOf("http://") == -1 && url.indexOf("https://") == -1 ) // File inside assets
{
System.out.println("Fetching file from assets: " + url);
FileInAssets = true;
break;
}
else
{
System.out.println("Connecting to: " + url);
request = new HttpGet(url);
request.addHeader("Accept", "*/*");
try {
DefaultHttpClient client = HttpWithDisabledSslCertCheck();
client.getParams().setBooleanParameter("http.protocol.handle-redirects", true);
response = client.execute(request);
} catch (IOException e) {
System.out.println("Failed to connect to " + url);
downloadUrlIndex++;
};
if( response != null )
{
if( response.getStatusLine().getStatusCode() != 200 )
{
response = null;
System.out.println("Failed to connect to " + url);
downloadUrlIndex++;
}
else
break;
}
}
}
if( FileInAssets )
{
System.out.println("Unpacking from assets: '" + url + "'");
try {
// System.out.println("Unpacking from assets: '" + url + "' 1");
stream = new CountingInputStream(Parent.getAssets().open(url), 8192);
// System.out.println("Unpacking from assets: '" + url + "' 2");
while( stream.skip(65536) > 0 ) { };
// System.out.println("Unpacking from assets: '" + url + "' 3");
totalLen = stream.getBytesRead();
// System.out.println("Unpacking from assets: '" + url + "' 4 totalLen = " + String.valueOf(totalLen));
stream.close();
// System.out.println("Unpacking from assets: '" + url + "' 5");
stream = new CountingInputStream(Parent.getAssets().open(url), 8192);
// System.out.println("Unpacking from assets: '" + url + "' 6");
} catch( IOException e ) {
System.out.println("Unpacking from assets '" + url + "' - error: " + e.toString());
没有合适的资源?快使用搜索试试~ 我知道了~
小程序 公交查询.rar
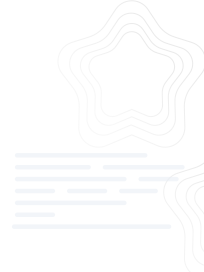
共56个文件
java:34个
xml:8个
png:3个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 38 浏览量
2023-02-03
11:36:03
上传
评论
收藏 805KB RAR 举报
温馨提示
免责声明:资料部分来源于合法的互联网渠道收集和整理,部分自己学习积累成果,供大家学习参考与交流。收取的费用仅用于收集和整理资料耗费时间的酬劳。 本人尊重原创作者或出版方,资料版权归原作者或出版方所有,本人不对所涉及的版权问题或内容负法律责任。如有侵权,请举报或通知本人删除。
资源推荐
资源详情
资源评论
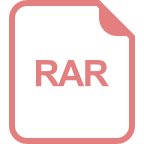
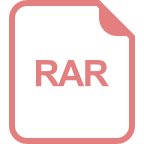
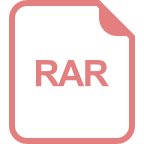
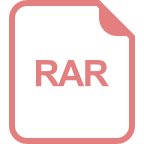
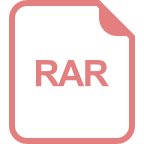
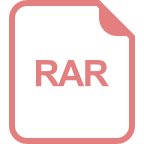
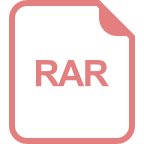
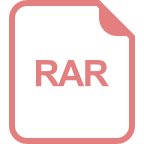
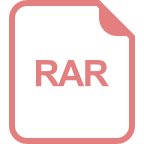
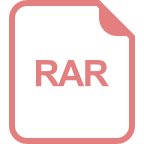
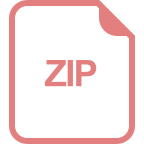
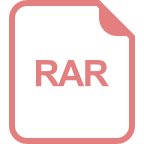
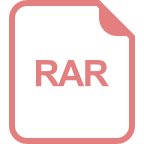
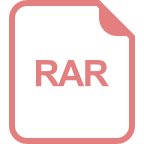
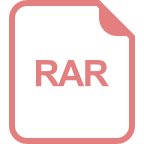
收起资源包目录



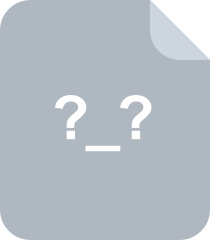
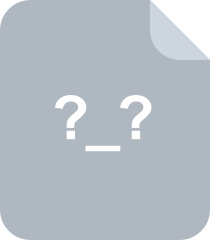
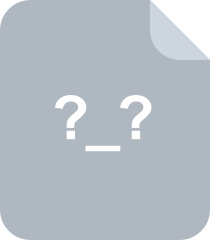

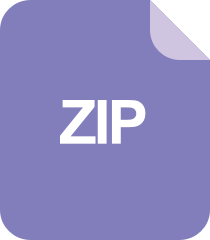





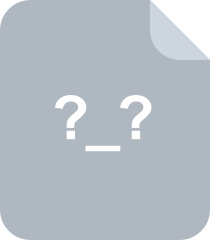
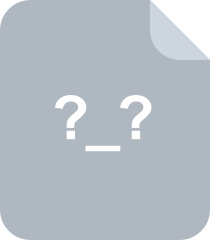
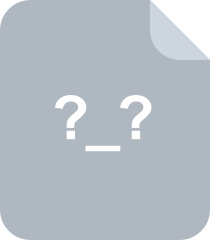
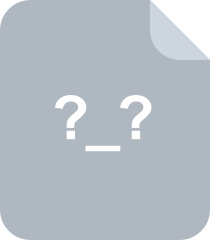
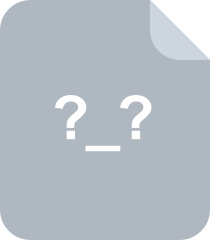
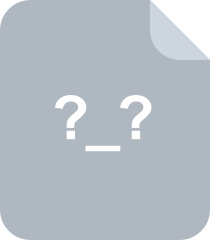
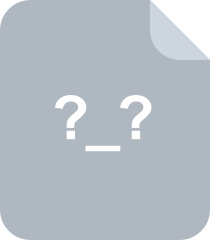
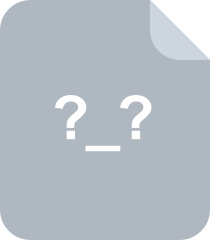
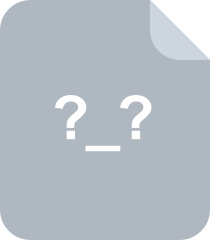
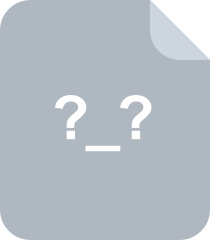
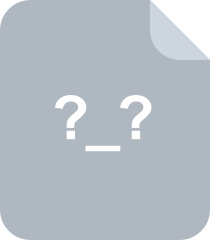
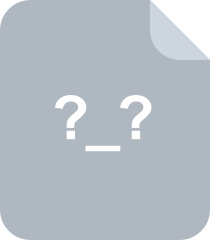
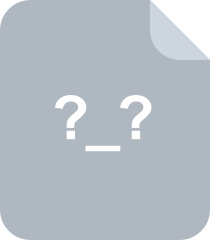
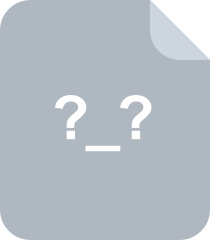
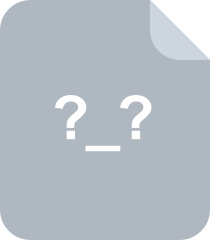
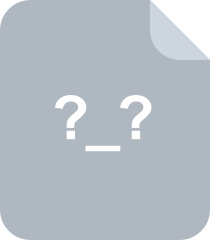
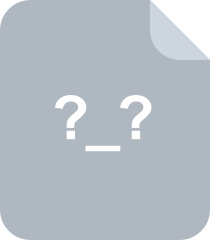
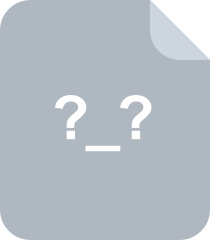
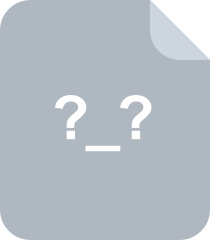
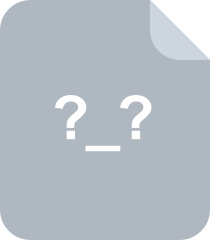
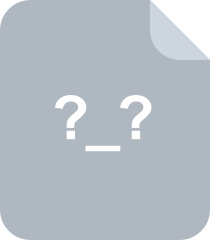
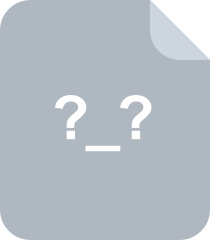
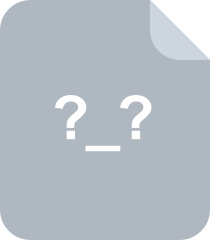
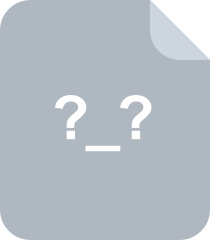
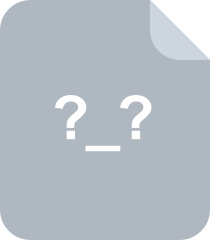
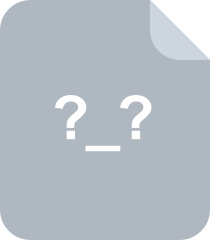
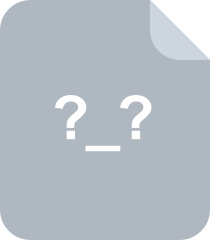
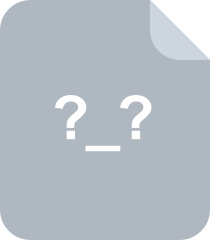



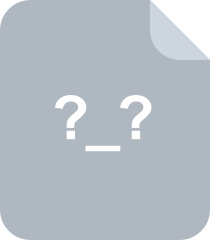
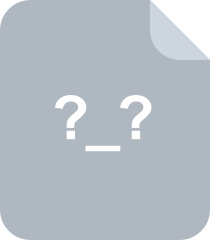
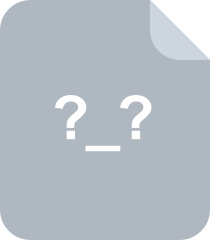
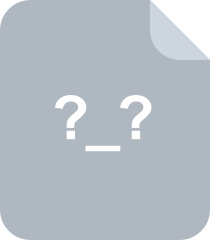
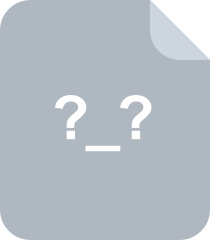
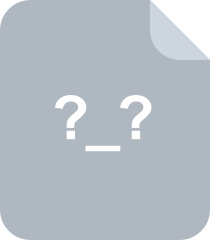
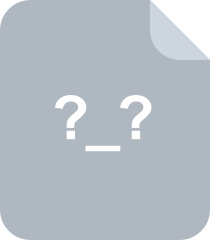

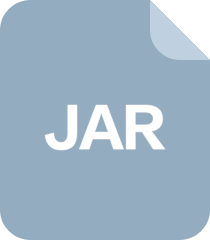
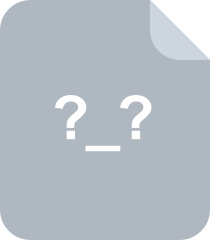



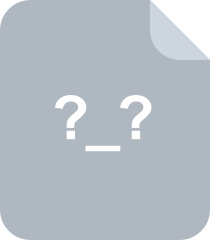
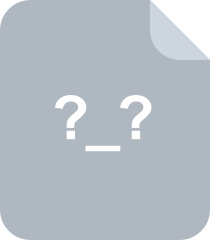
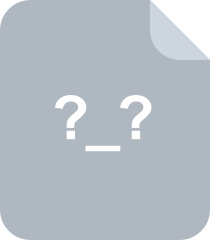

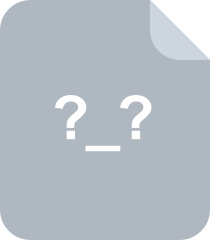
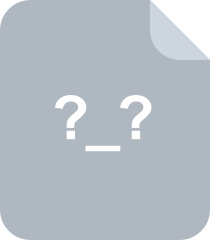
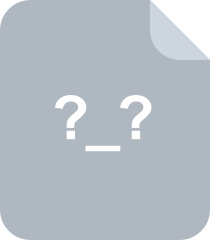

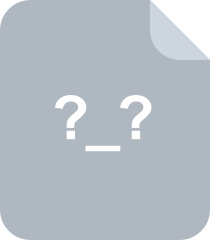
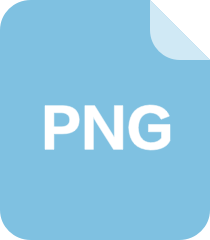
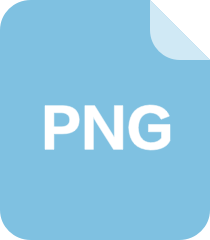
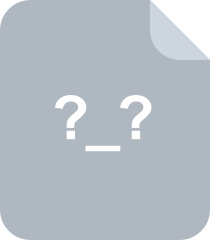

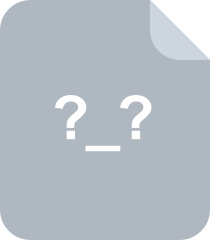
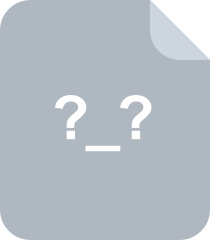
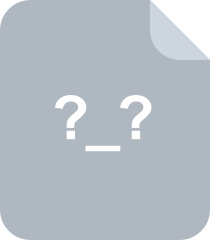
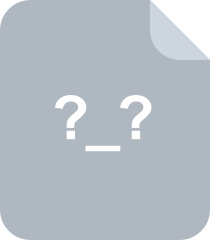
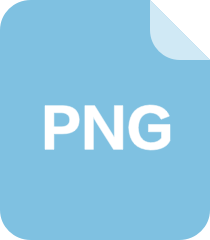
共 56 条
- 1
资源评论
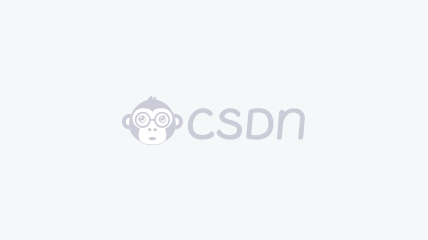

大富大贵7
- 粉丝: 389
- 资源: 8868

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

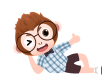
最新资源
- C183579-123578-c1235789.jpg
- Qt5.14 绘画板 Qt Creator C++项目
- python实现Excel表格合并
- Java实现读取Excel批量发送邮件.zip
- 【java毕业设计】商城后台管理系统源码(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】开发停车位管理系统(调用百度地图API)源码(springboot+vue+mysql+说明文档).zip
- 星耀软件库(升级版).apk.1
- 基于Django后端和Vue前端的多语言购物车项目设计源码
- 基于Python与Vue的浮光在线教育平台源码设计
- 31129647070291Eclipson MXS R.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


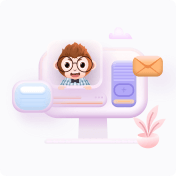
安全验证
文档复制为VIP权益,开通VIP直接复制
