#include "char_img.h"
#include <stdio.h>
#include <windows.h>
#include <malloc.h>
#include <WinUser.h> //为了可以使用GetAsyncKeyState函数
#pragma comment(lib,"User32.lib")
#define cTRUE 1
#define cFALSE 0
//用户交互函数 按键输入
char ui_key_is_pressed(char Key)
{
if(GetAsyncKeyState(Key)&0x8000)
return cTRUE;//按键按下
else
return cFALSE;//没按
}
//用户交互函数 屏幕输出
void ui_putchar(char a)
{
putchar(a);
}
/*
0------------>x,w,i
|
|
|
|
|
v
y,h,j
*/
static HANDLE handle;
void ui_init()
{
CONSOLE_CURSOR_INFO cinfo;
handle = GetStdHandle(STD_OUTPUT_HANDLE);
cinfo.bVisible = 0;
cinfo.dwSize = 1;
SetConsoleCursorInfo(handle,&cinfo);
}
void ui_set_xy(short x, short y)
{
COORD coord = { x,y };
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE),coord);
}
//------------------------------------------------------------------------
#define IMG_GET_PIXEL(img,i,j) (*(img->buffer + (j)*(img->w) +(i)))
#define IMG_CHAR_NONE (' ')
//图形
void img_foreach(struct st_img *img,void (*every_pixel)(struct st_img *img,int i,int j,void *arg),void *arg)
{
int i,j;
for(j=0;j<img->h;j++)
for(i=0;i<img->w;i++)
{
every_pixel(img,i,j,arg);
}
}
static void pixel_fill(struct st_img *img,int i,int j,void *arg)
{
IMG_GET_PIXEL(img,i,j) = *(char *)arg;
}
void img_init(struct st_img *img,char *buffer,int h,int w)
{
img->buffer = buffer;
img->h = h;
img->w = w;
}
void img_fill(struct st_img *img,char fill_char)
{
img_foreach(img,pixel_fill,&fill_char);
}
static void pixel_put(struct st_img *img,int i,int j,void *arg)
{
ui_set_xy(i,j);
ui_putchar(IMG_GET_PIXEL(img,i,j));
}
void img_print(struct st_img *img)
{
img_foreach(img,pixel_put,NULL);
}
struct st_point img_check(struct st_img *img_back,struct st_img *img_icon,int img_icon_x,int img_icon_y)
{
int i,j;
struct st_point point_overlap = {-1,-1};
for(j=0;j<img_icon->h;j++)
for(i=0;i<img_icon->w;i++)
{
if(IMG_GET_PIXEL(img_icon,i,j) != IMG_CHAR_NONE)
{
if(IMG_GET_PIXEL(img_back,i+img_icon_x,j+img_icon_y)!= IMG_CHAR_NONE)
{
point_overlap.x = i+img_icon_x;
point_overlap.y = j+img_icon_y;
return point_overlap;
}
}
}
return point_overlap;
}
int img_copy(struct st_img *img_dst,struct st_img *img_src)
{
int i,j;
if((img_dst->h != img_src->h)
|| (img_dst->w != img_src->w))
{
return cFALSE;
}
for(j=0;j<img_src->h;j++)
for(i=0;i<img_src->w;i++)
{
IMG_GET_PIXEL(img_dst,i,j) = IMG_GET_PIXEL(img_src,i,j);
}
return cTRUE;
}
void img_write(struct st_img *img_back,struct st_img *img_icon,int x,int y)
{
int i,j;
for(j=0;j<img_icon->h;j++)
for(i=0;i<img_icon->w;i++)
{
if( (i + x < img_back->w)
&& (j + y < img_back->h)
&& (i + x >= 0)
&& (j + y >= 0)
)
{
char pixel = IMG_GET_PIXEL(img_icon,i ,j );
if(pixel != IMG_CHAR_NONE)
{
IMG_GET_PIXEL(img_back, i + x, j + y) =pixel;
}
}
}
}
void img_erase(struct st_img *img_back,struct st_img *img_icon,int x,int y)
{
int i,j;
for(j=0;j<img_icon->h;j++)
for(i=0;i<img_icon->w;i++)
{
if( (i + x < img_back->w)
&& (j + y < img_back->h)
&& (i + x >= 0)
&& (j + y >= 0)
)
{
char pixel = IMG_GET_PIXEL(img_icon,i ,j );
if(pixel != IMG_CHAR_NONE)
{
IMG_GET_PIXEL(img_back, i+ x, j+ y) = IMG_CHAR_NONE;
}
}
}
}

大富大贵7
- 粉丝: 389
- 资源: 8868
最新资源
- (源码)基于C语言的操作系统实验项目.zip
- (源码)基于C++的分布式设备配置文件管理系统.zip
- (源码)基于ESP8266和Arduino的HomeMatic水表读数系统.zip
- (源码)基于Django和OpenCV的智能车视频处理系统.zip
- (源码)基于ESP8266的WebDAV服务器与3D打印机管理系统.zip
- (源码)基于Nio实现的Mycat 2.0数据库代理系统.zip
- (源码)基于Java的高校学生就业管理系统.zip
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


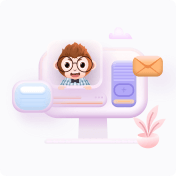
- 1
- 2
- 3
前往页