/*++
Copyright (c) 1995 - 1997 Microsoft Corporation
Module Name:
rtm.h
Abstract:
Interface for Routing Table Manager DLL
--*/
#ifndef __ROUTING_RTM_H__
#define __ROUTING_RTM_H__
#ifdef __cplusplus
extern "C" {
#endif
// Currently supported protocol families
#define RTM_PROTOCOL_FAMILY_IPX 0
#define RTM_PROTOCOL_FAMILY_IP 1
// Error messages specific to Routing Table Manager
#define ERROR_MORE_MESSAGES ERROR_MORE_DATA
#define ERROR_CLIENT_ALREADY_EXISTS ERROR_ALREADY_EXISTS
#define ERROR_NO_MESSAGES ERROR_NO_MORE_ITEMS
#define ERROR_NO_MORE_ROUTES ERROR_NO_MORE_ITEMS
#define ERROR_NO_ROUTES ERROR_NO_MORE_ITEMS
#define ERROR_NO_SUCH_ROUTE ERROR_NO_MORE_ITEMS
// Protocol family dependent network number structures
typedef struct _IPX_NETWORK {
DWORD N_NetNumber;
} IPX_NETWORK, *PIPX_NETWORK;
typedef struct _IP_NETWORK {
DWORD N_NetNumber;
DWORD N_NetMask;
} IP_NETWORK, *PIP_NETWORK;
// Protocol family dependent next hop router address structures
typedef struct _IPX_NEXT_HOP_ADDRESS {
BYTE NHA_Mac[6];
} IPX_NEXT_HOP_ADDRESS, *PIPX_NEXT_HOP_ADDRESS;
typedef IP_NETWORK IP_NEXT_HOP_ADDRESS, *PIP_NEXT_HOP_ADDRESS;
// Protocol family dependent specific data structures
typedef struct _IPX_SPECIFIC_DATA {
DWORD FSD_Flags; // should be set to 0 if no flags are defined
USHORT FSD_TickCount; // ticks to destination network
USHORT FSD_HopCount; // hops to destination network
} IPX_SPECIFIC_DATA, *PIPX_SPECIFIC_DATA;
// The following flags are defined for the IPX family dependent Flags field
#define IPX_GLOBAL_CLIENT_WAN_ROUTE 0x00000001
typedef struct _IP_SPECIFIC_DATA
{
DWORD FSD_Type; // One of : other,invalid,local,remote (1-4)
DWORD FSD_Policy; // See RFC 1354
DWORD FSD_NextHopAS; // Autonomous System Number of Next Hop
DWORD FSD_Priority; // For comparing Inter Protocol Metrics
DWORD FSD_Metric; // For comparing Intra Protocol Metrics
DWORD FSD_Metric1; // Protocol specific metrics for MIB II
DWORD FSD_Metric2; // conformance. MIB II agents will not
DWORD FSD_Metric3; // display FSD_Metric, they will only
DWORD FSD_Metric4; // display Metrics1-5, so if you want your
DWORD FSD_Metric5; // metric displayed, put it in one of these
// fields ALSO (It has to be in the
// FSD_Metric field always). It is up to the
// implementor to keep the fields consistent
DWORD FSD_Flags; // Flags defined below
} IP_SPECIFIC_DATA, *PIP_SPECIFIC_DATA;
//
// All routing protocols MUST clear out the FSD_Flags field.
// If RTM notifies a protocol of a route and that route is marked
// invalid, the protocols MUST disregard this route. A protocol
// may mark a route as invalid, if it does not want the route to
// be indicated to other protocols or set to the stack. e.g. RIPv2
// marks summarized routes as INVALID and uses the RTM purely as
// a store for such routes. These routes are not considered when
// comparing metrics etc to decide the "best" route
//
#define IP_VALID_ROUTE 0x00000001
#define ClearRouteFlags(pRoute) \
((pRoute)->RR_FamilySpecificData.FSD_Flags = 0x00000000)
#define IsRouteValid(pRoute) \
((pRoute)->RR_FamilySpecificData.FSD_Flags & IP_VALID_ROUTE)
#define SetRouteValid(pRoute) \
((pRoute)->RR_FamilySpecificData.FSD_Flags |= IP_VALID_ROUTE)
#define ClearRouteValid(pRoute) \
((pRoute)->RR_FamilySpecificData.FSD_Flags &= ~IP_VALID_ROUTE)
#define IsRouteNonUnicast(pRoute) \
(((DWORD)((pRoute)->RR_Network.N_NetNumber & 0x000000FF)) >= ((DWORD)0x000000E0))
#define IsRouteLoopback(pRoute) \
((((pRoute)->RR_Network.N_NetNumber & 0x000000FF) == 0x0000007F) || \
((pRoute)->RR_NextHopAddress.N_NetNumber == 0x0100007F))
// Protocol dependent specific data structure
typedef struct _PROTOCOL_SPECIFIC_DATA {
DWORD PSD_Data[4];
} PROTOCOL_SPECIFIC_DATA, *PPROTOCOL_SPECIFIC_DATA;
#define DWORD_ALIGN(type,field) \
union { \
type field; \
DWORD field##Align; \
}
// Standard header associated with all types of routes
#define ROUTE_HEADER \
DWORD_ALIGN ( \
FILETIME, RR_TimeStamp); \
DWORD RR_RoutingProtocol; \
DWORD RR_InterfaceID; \
DWORD_ALIGN ( \
PROTOCOL_SPECIFIC_DATA, RR_ProtocolSpecificData)
// Ensure dword aligment of all fields
// Protocol family dependent route entries
// (intended for use by protocol handlers to pass paramerters
// to and from RTM routines)
typedef struct _RTM_IPX_ROUTE {
ROUTE_HEADER;
DWORD_ALIGN (
IPX_NETWORK, RR_Network);
DWORD_ALIGN (
IPX_NEXT_HOP_ADDRESS, RR_NextHopAddress);
DWORD_ALIGN (
IPX_SPECIFIC_DATA, RR_FamilySpecificData);
} RTM_IPX_ROUTE, *PRTM_IPX_ROUTE;
typedef struct _RTM_IP_ROUTE {
ROUTE_HEADER;
DWORD_ALIGN (
IP_NETWORK, RR_Network);
DWORD_ALIGN (
IP_NEXT_HOP_ADDRESS, RR_NextHopAddress);
DWORD_ALIGN (
IP_SPECIFIC_DATA, RR_FamilySpecificData);
} RTM_IP_ROUTE, *PRTM_IP_ROUTE;
// RTM route change message flags
// Flags to test the presence of route change information
// in the buffers filled by RTM routines
#define RTM_CURRENT_BEST_ROUTE 0x00000001
#define RTM_PREVIOUS_BEST_ROUTE 0x00000002
// Flags that convey what acutally happened
#define RTM_NO_CHANGE 0
#define RTM_ROUTE_ADDED RTM_CURRENT_BEST_ROUTE
#define RTM_ROUTE_DELETED RTM_PREVIOUS_BEST_ROUTE
#define RTM_ROUTE_CHANGED (RTM_CURRENT_BEST_ROUTE|RTM_PREVIOUS_BEST_ROUTE)
// Enumeration flags limit that enumerations of routes in the table
// to only entries with the specified field(s)
#define RTM_ONLY_THIS_NETWORK 0x00000001
#define RTM_ONLY_THIS_INTERFACE 0x00000002
#define RTM_ONLY_THIS_PROTOCOL 0x00000004
#define RTM_ONLY_BEST_ROUTES 0x00000008
#define RTM_PROTOCOL_SINGLE_ROUTE 0x00000001
/*++
*******************************************************************
R t m R e g i s t e r C l i e n t
Routine Description:
Registers client as a handler of specified protocol.
Establishes route change notification mechanism for the client
Arguments:
ProtocolFamily - protocol family of the routing protocol to register
RoutingProtocol - which routing protocol is handled by the client
ChangeEvent - this event will be signalled when best route to any
network in the table changes
Flags - flags that enable special features applied to routes maintained
by the protocol:
RTM_PROTOCOL_SINGLE_ROUTE - RTM will only keep
one route per destination network for the protocol
Return Value:
Handle to be used to identify the client in calls made to Rtm
NULL - operation failed, call GetLastError() to get reason of
failure:
ERROR_INVALID_PARAMETER - specified protocol family is not supported
ERROR_CLIENT_ALREADY_EXISTS - another client already registered
to handle specified protocol
ERROR_NO_SYSTEM_RESOURCES - not enough resources to perform the opertaion,
try again later
ERROR_NOT_ENOUGH_MEMORY - not enough memory to allocate client
control block
*******************************************************************
--*/
HANDLE WINAPI
RtmRegisterClient (
IN DWORD ProtocolFamily,
IN DWORD RoutingProtocol,
IN HANDLE ChangeEvent OPTIONAL,
IN DWORD Flags
);
/*++
*******************************************************************
R t m D e r e g i s t e r C l i e n t
Routine Description:
Deregister client and frees associated resources (including all
routes added by the client).
Argum

大富大贵7
- 粉丝: 393
- 资源: 8868
最新资源
- 数独游戏app,for安卓
- 我的编程作品:《声音、光和运动》
- SQlServer2005编程入门经典-触发器和存储过程教程pdf最新版本
- 车辆树木检测21-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- SQL经典语句大全及技巧汇集chm版最新版本
- SQLServer入门到精通HTML版最新版本
- 医疗领域数据相关的标准清单.xlsx
- xilinx FPGA利用can IP实现can总线通信verilog源码,直接可用,注释清晰 vivado实现,代码7系列以上都兼容
- SQL2005教程PPT讲义(初级入门基础)最新版本
- CC2530无线点对点传输协议zigbee BasicRF代码实现一发一收无线控制LED灯亮灭.zip
- CC2530无线点对点传输协议zigbee BasicRF代码实现一发一收无线通讯质量检测(误包率、RSSI 值和接收数据包个数等).zip
- comsol仿真,磁屏蔽 铁氧体做磁屏蔽和没有屏蔽时的接受端磁密大小,及屏蔽上的磁密分布
- 四足机器人设计原理与应用探索
- 车辆检测1-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 食品数据相关标准清单.xlsx
- SQLServer入门基础15天掌握最新版本
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


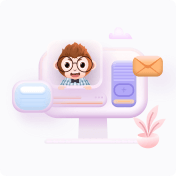