package com.web.controller;
import com.web.controller.utils.*;
import com.web.controller.utils.HbaseUtils;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.CellUtil;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.client.Table;
import org.apache.hadoop.hbase.util.Bytes;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.io.IOException;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Scanner;
import static java.util.Arrays.asList;
@Controller
public class WebController {
String hbaseQuorum = "hadoop10,hadoop11,hadoop12,hadoop13";
String zookeeperPort = "2181";
String tableName = "tmdb";
String tmdb_popularity = "tmdb_popularity";
String tmdb_runtime = "tmdb_runtime";
Configuration hbaseConf = new HbaseUtils().getHbaseConf(hbaseQuorum, zookeeperPort);
public WebController() throws Exception {
}
@RequestMapping(value = {"", "/index"})
public String index(Model model) {
// model.addAttribute("msg", "hello world");
return "index";
}
@RequestMapping(value = {"/demo"})
@ResponseBody
public List<Product> myProject() {
String[] data = {"羊毛衫","雪纺衫","裤子","高跟鞋","袜子"};
long[] nums = {20, 36, 10, 10, 20};
ArrayList<Product> productArr = new ArrayList<Product>();
for (int i=0; i<data.length; i++){
Product p = new Product();
p.setProductName(data[i]);
p.setNums(nums[i]);
productArr.add(p);
}
return productArr;
}
@RequestMapping(value = {"/popularity"})
@ResponseBody
public List<Product> Popularity() throws IOException {
Table table = new HbaseUtils().getTable(hbaseConf, tmdb_popularity);
Scan scan = new Scan();
ResultScanner resultScanner = table.getScanner(scan);
ArrayList<Product> productArr = new ArrayList<Product>();
long[] popularity = new long[10];
String[] original_title = new String[10];
int oindex = 0;
int pindex = 0;
for(Result result : resultScanner){
Cell[] cells = result.rawCells();
for(Cell cell : cells) {
// //得到rowkey
// System.out.println("行键:" + Bytes.toString(CellUtil.cloneRow(cell)));
// //得到列族
// System.out.println("列族" + Bytes.toString(CellUtil.cloneFamily(cell)));
// System.out.println("列:" + Bytes.toString(CellUtil.cloneQualifier(cell)));
// System.out.println("值:" + Bytes.toString(CellUtil.cloneValue(cell)));
String col = Bytes.toString(CellUtil.cloneQualifier(cell));
if (col.equals("original_title")) {
String value = Bytes.toString(CellUtil.cloneValue(cell));
original_title[oindex++] = value.split(":")[0];
} else if (col.equals("popularity")) {
Float value = Bytes.toFloat(CellUtil.cloneValue(cell));
popularity[pindex++] = value.longValue();
}
}
}
System.out.println(Arrays.toString(original_title));
System.out.println(Arrays.toString(popularity));
for (int i=0; i<original_title.length; i++){
Product p = new Product();
p.setProductName(original_title[i]);
p.setNums(popularity[i]);
productArr.add(p);
}
return productArr;
}
@RequestMapping(value = {"/runtime"})
@ResponseBody
public List<Product> Runtime() throws IOException {
Table table = new HbaseUtils().getTable(hbaseConf, tmdb_runtime);
Scan scan = new Scan();
ResultScanner resultScanner = table.getScanner(scan);
ArrayList<Product> productArr = new ArrayList<Product>();
long[] count = new long[16];
String[] runtime = new String[16];
int rindex = 0;
int cindex = 0;
for(Result result : resultScanner){
Cell[] cells = result.rawCells();
for(Cell cell : cells) {
String col = Bytes.toString(CellUtil.cloneQualifier(cell));
if (col.equals("runtime")) {
String value = Bytes.toString(CellUtil.cloneValue(cell));
runtime[rindex++] = value;
} else if (col.equals("count")) {
Long value = Bytes.toLong(CellUtil.cloneValue(cell));
count[cindex++] = value;
}
}
}
for (int i=0; i<runtime.length; i++){
Product p = new Product();
p.setProductName(runtime[i]);
p.setNums(count[i]);
productArr.add(p);
}
return productArr;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip 基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip 基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip 基于spark和hbase的电影数据可视化及推荐系统源码(课程设计).zip 【备注】 1.项目代码均经过功能验证ok,确保稳定可靠运行。欢迎下载使用体验! 2.主要针对各个计算机相关专业,包括计算机科学、信息安全、数据科学与大数据技术、人工智能、通信、物联网等领域的在校学生、专业教师、企业员工。 3.项目具有丰富的拓展空间,不仅可作为入门进阶,也可直接作为毕设、课程设计、大作业、初期项目立项演示等用途。 4.当然也鼓励大家基于此进行二次开发。在使用过程中,如有问题或建议,请及时沟通。 5.期待你能在项目中找到乐趣和灵感,也欢迎你的分享和反馈!
资源推荐
资源详情
资源评论
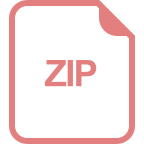
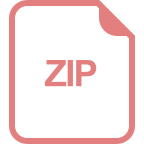
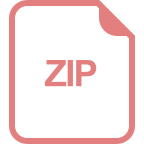
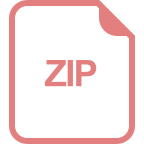
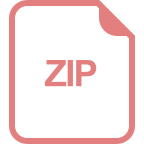
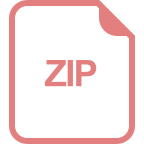
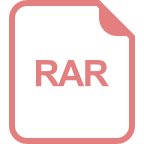
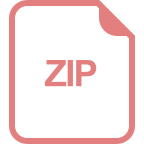
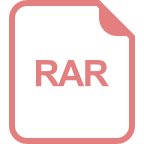
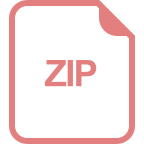
收起资源包目录

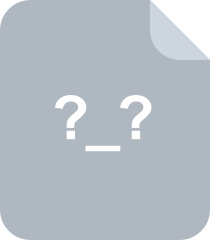
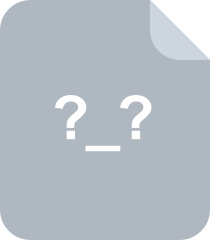
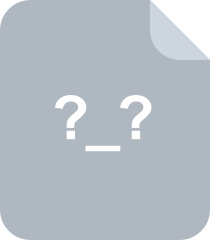

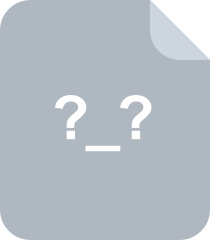
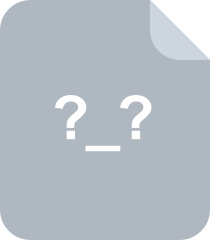
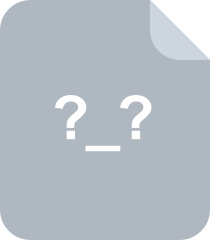





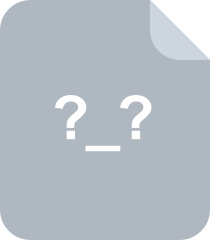



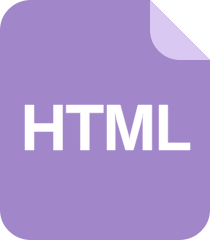


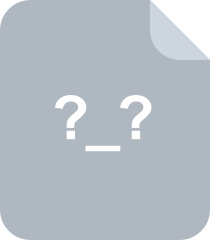
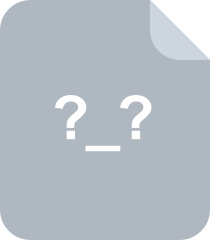
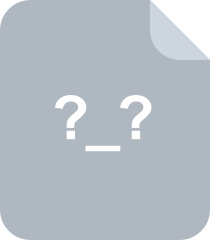
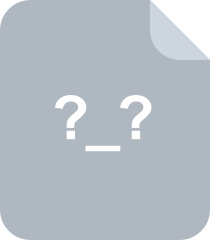



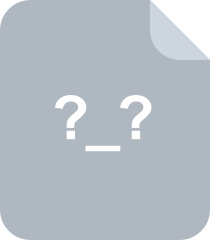


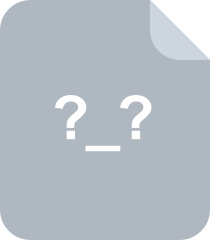
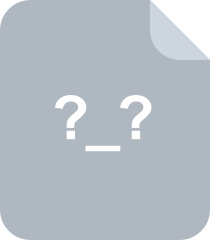
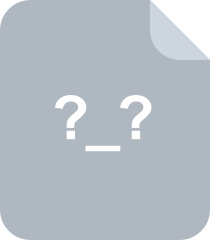



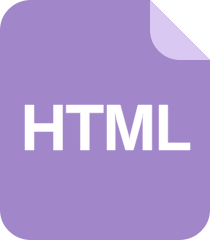


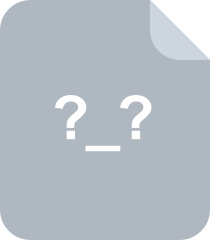
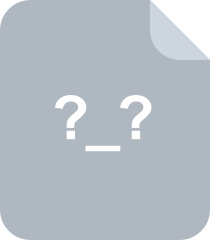
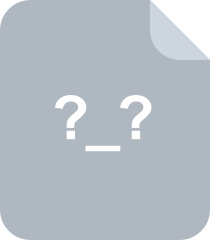
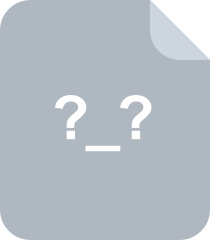


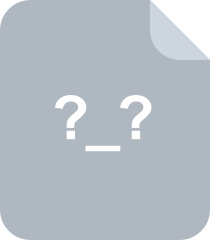


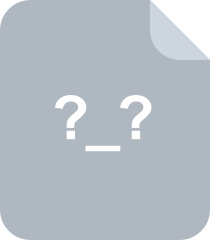
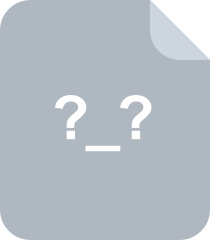
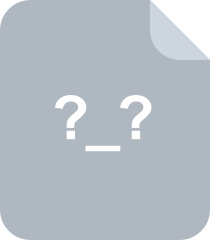



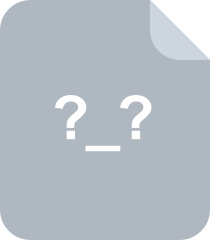
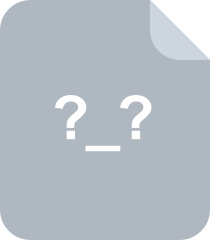
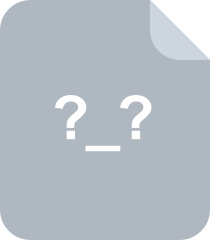
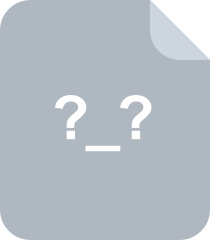
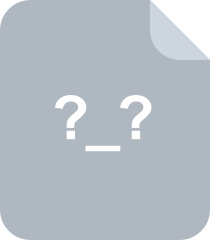

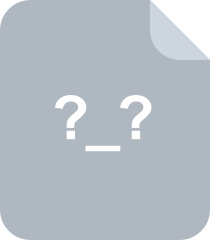
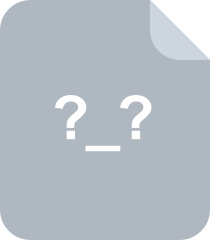



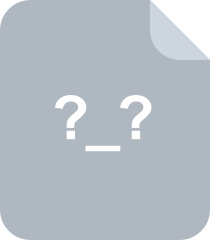
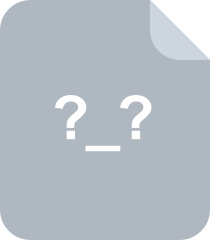
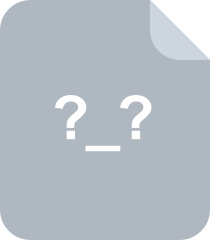




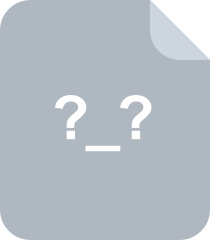

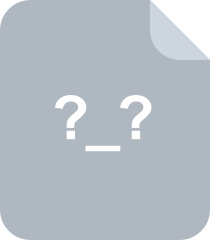

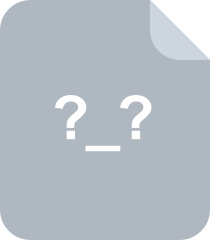

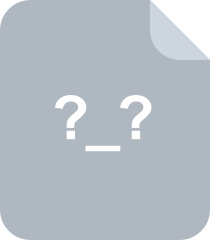


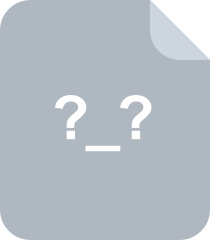
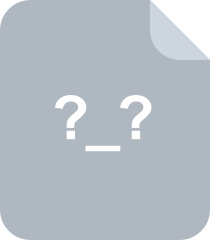
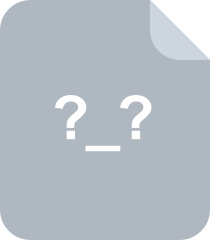



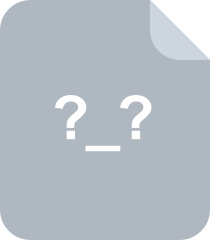
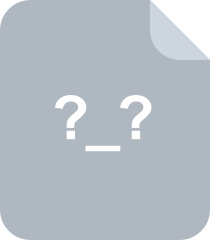
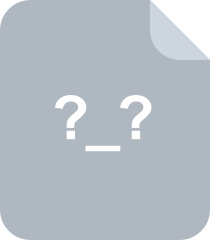
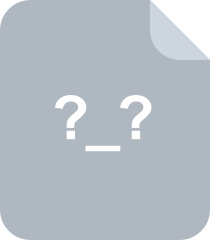
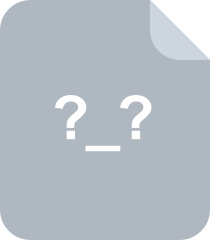
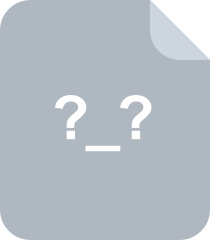
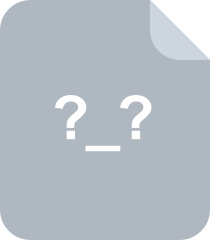
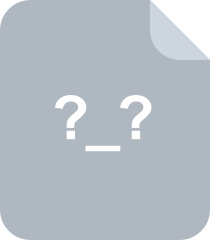

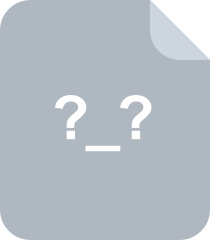
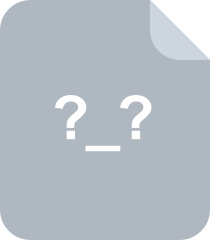
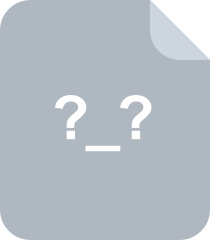
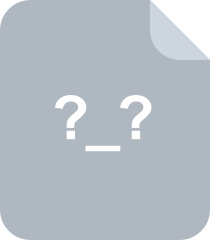
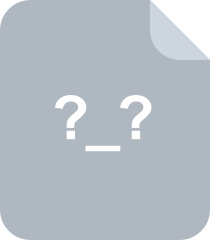

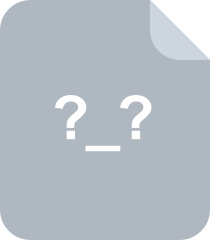
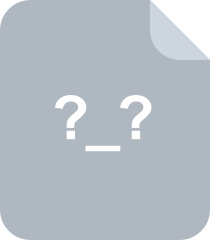
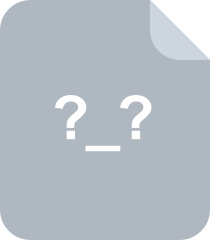
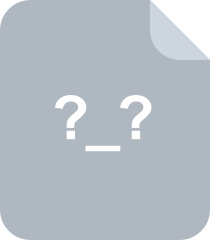

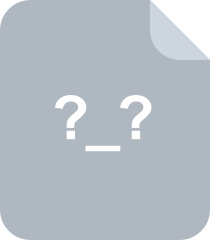
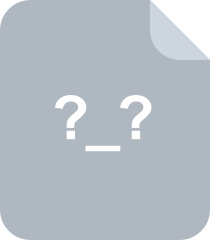
共 61 条
- 1
资源评论
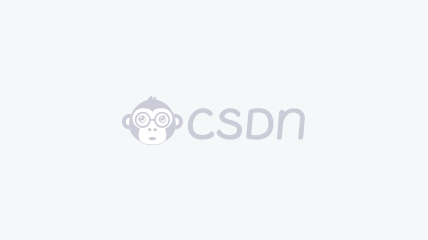

北航程序员小C
- 粉丝: 2222
- 资源: 1823
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

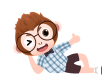
安全验证
文档复制为VIP权益,开通VIP直接复制
