#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <fstream>
#include <string>
#include <math.h>
#include <opencv2/imgproc.hpp>
#include <opencv2/highgui.hpp>
//#include <cuda_provider_factory.h>
#include <onnxruntime_cxx_api.h>
using namespace cv;
using namespace std;
using namespace Ort;
class Informative_Drawings
{
public:
Informative_Drawings(string modelpath);
Mat detect(Mat& cv_image);
private:
vector<float> input_image_;
int inpWidth;
int inpHeight;
int outWidth;
int outHeight;
Env env = Env(ORT_LOGGING_LEVEL_ERROR, "Informative Drawings");
Ort::Session *ort_session = nullptr;
SessionOptions sessionOptions = SessionOptions();
vector<char*> input_names;
vector<char*> output_names;
vector<vector<int64_t>> input_node_dims; // >=1 outputs
vector<vector<int64_t>> output_node_dims; // >=1 outputs
};
Informative_Drawings::Informative_Drawings(string model_path)
{
std::wstring widestr = std::wstring(model_path.begin(), model_path.end());
//OrtStatus* status = OrtSessionOptionsAppendExecutionProvider_CUDA(sessionOptions, 0);
sessionOptions.SetGraphOptimizationLevel(ORT_ENABLE_BASIC);
ort_session = new Session(env, widestr.c_str(), sessionOptions);
size_t numInputNodes = ort_session->GetInputCount();
size_t numOutputNodes = ort_session->GetOutputCount();
AllocatorWithDefaultOptions allocator;
for (int i = 0; i < numInputNodes; i++)
{
input_names.push_back(ort_session->GetInputName(i, allocator));
Ort::TypeInfo input_type_info = ort_session->GetInputTypeInfo(i);
auto input_tensor_info = input_type_info.GetTensorTypeAndShapeInfo();
auto input_dims = input_tensor_info.GetShape();
input_node_dims.push_back(input_dims);
}
for (int i = 0; i < numOutputNodes; i++)
{
output_names.push_back(ort_session->GetOutputName(i, allocator));
Ort::TypeInfo output_type_info = ort_session->GetOutputTypeInfo(i);
auto output_tensor_info = output_type_info.GetTensorTypeAndShapeInfo();
auto output_dims = output_tensor_info.GetShape();
output_node_dims.push_back(output_dims);
}
this->inpHeight = input_node_dims[0][2];
this->inpWidth = input_node_dims[0][3];
this->outHeight = output_node_dims[0][2];
this->outWidth = output_node_dims[0][3];
}
Mat Informative_Drawings::detect(Mat& srcimg)
{
Mat dstimg;
resize(srcimg, dstimg, Size(this->inpWidth, this->inpHeight));
this->input_image_.resize(this->inpWidth * this->inpHeight * dstimg.channels());
for (int c = 0; c < 3; c++)
{
for (int i = 0; i < this->inpHeight; i++)
{
for (int j = 0; j < this->inpWidth; j++)
{
float pix = dstimg.ptr<uchar>(i)[j * 3 + 2 - c];
this->input_image_[c * this->inpHeight * this->inpWidth + i * this->inpWidth + j] = pix;
}
}
}
array<int64_t, 4> input_shape_{ 1, 3, this->inpHeight, this->inpWidth };
auto allocator_info = MemoryInfo::CreateCpu(OrtDeviceAllocator, OrtMemTypeCPU);
Value input_tensor_ = Value::CreateTensor<float>(allocator_info, input_image_.data(), input_image_.size(), input_shape_.data(), input_shape_.size());
// 开始推理
vector<Value> ort_outputs = ort_session->Run(RunOptions{ nullptr }, &input_names[0], &input_tensor_, 1, output_names.data(), output_names.size()); // 开始推理
float* pred = ort_outputs[0].GetTensorMutableData<float>();
Mat result(outHeight, outWidth, CV_32FC1, pred);
result *= 255;
result.convertTo(result, CV_8UC1);
resize(result, result, Size(srcimg.cols, srcimg.rows));
return result;
}
int main()
{
Informative_Drawings mynet("weights/opensketch_style_512x512.onnx"); ///choices=["weights/opensketch_style_512x512.onnx", "weights/anime_style_512x512.onnx", "weights/contour_style_512x512.onnx"]
string imgpath = "images/2.jpg";
Mat srcimg = imread(imgpath);
Mat result = mynet.detect(srcimg);
resize(result, result, Size(srcimg.cols, srcimg.rows));
namedWindow("srcimg", WINDOW_NORMAL);
imshow("srcimg", srcimg);
static const string kWinName = "Deep learning in ONNXRuntime";
namedWindow(kWinName, WINDOW_NORMAL);
imshow(kWinName, result);
waitKey(0);
destroyAllWindows();
}
没有合适的资源?快使用搜索试试~ 我知道了~
基于ONNXRuntime部署Informative-Drawings生成素描画源码(c++版本和python版本).zip
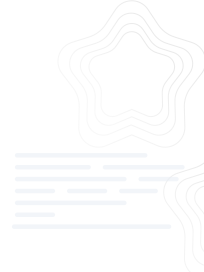
共9个文件
jpg:3个
onnx:3个
py:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 135 浏览量
2023-12-15
14:39:14
上传
评论
收藏 46.17MB ZIP 举报
温馨提示
【项目介绍】 基于ONNXRuntime部署Informative-Drawings生成素描画源码(c++版本和python版本).zip基于ONNXRuntime部署Informative-Drawings生成素描画源码(c++版本和python版本).zip基于ONNXRuntime部署Informative-Drawings生成素描画源码(c++版本和python版本).zip基于ONNXRuntime部署Informative-Drawings生成素描画源码(c++版本和python版本).zip 【备注】 1.项目代码均经过功能验证ok,确保稳定可靠运行。欢迎下载食用体验! 2.主要针对各个计算机相关专业,包括计算机科学、信息安全、数据科学与大数据技术、人工智能、通信、物联网等领域的在校学生、专业教师、企业员工。 3.项目具有丰富的拓展空间,不仅可作为入门进阶,也可直接作为毕设、课程设计、大作业、初期项目立项演示等用途。 4.当然也鼓励大家基于此进行二次开发。在使用过程中,如有问题或建议,请及时沟通。 5.期待你能在项目中找到乐趣和灵感,也欢迎你的分享和反馈!
资源推荐
资源详情
资源评论
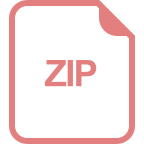
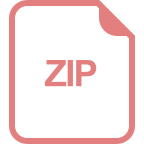
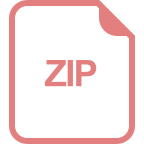
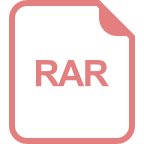
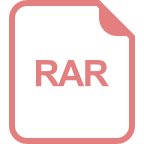
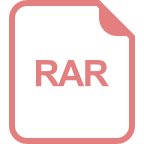
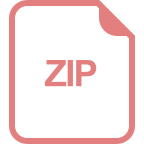
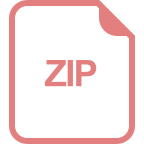
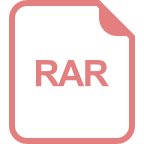
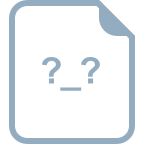
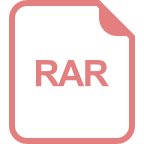
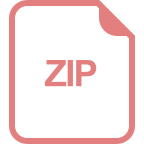
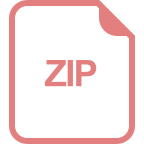
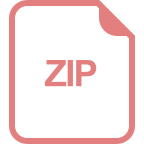
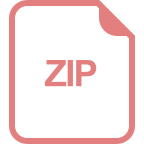
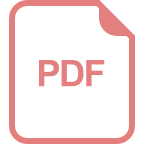
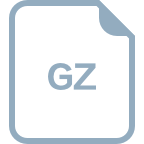
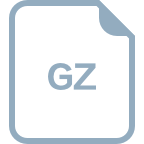
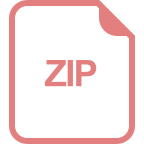
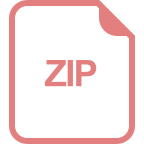
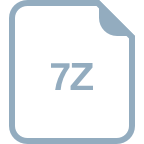
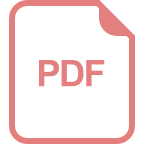
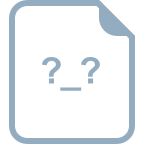
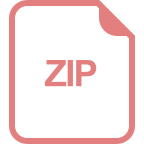
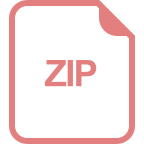
收起资源包目录

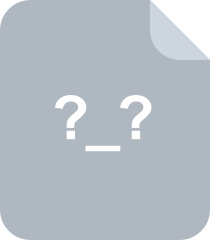

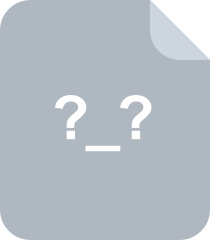
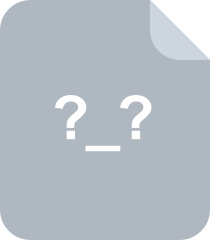
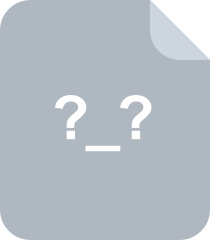
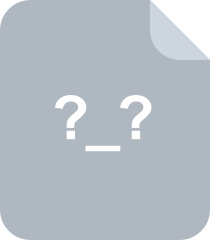
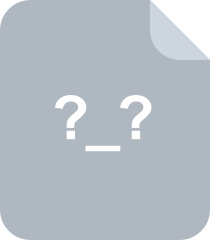

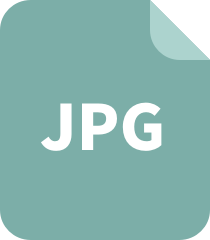
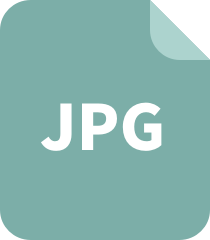
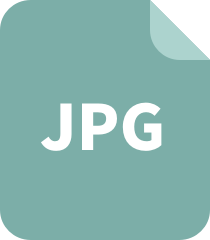
共 9 条
- 1
资源评论
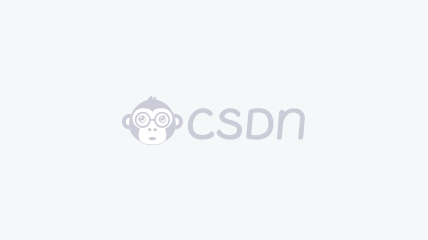

.whl
- 粉丝: 3824
- 资源: 4664
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

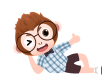
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


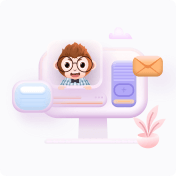
安全验证
文档复制为VIP权益,开通VIP直接复制
