# This is an auto-generated Django model module.
# You'll have to do the following manually to clean this up:
# * Rearrange models' order
# * Make sure each model has one field with primary_key=True
# * Make sure each ForeignKey and OneToOneField has `on_delete` set to the desired behavior
# * Remove `managed = False` lines if you wish to allow Django to create, modify, and delete the table
# Feel free to rename the models, but don't rename db_table values or field names.
from django.db import models
class AuthGroup(models.Model):
name = models.CharField(unique=True, max_length=150)
class Meta:
managed = False
db_table = 'auth_group'
class AuthGroupPermissions(models.Model):
id = models.BigAutoField(primary_key=True)
group = models.ForeignKey(AuthGroup, models.DO_NOTHING)
permission = models.ForeignKey('AuthPermission', models.DO_NOTHING)
class Meta:
managed = False
db_table = 'auth_group_permissions'
unique_together = (('group', 'permission'),)
class AuthPermission(models.Model):
name = models.CharField(max_length=255)
content_type = models.ForeignKey('DjangoContentType', models.DO_NOTHING)
codename = models.CharField(max_length=100)
class Meta:
managed = False
db_table = 'auth_permission'
unique_together = (('content_type', 'codename'),)
class AuthUser(models.Model):
password = models.CharField(max_length=128)
last_login = models.DateTimeField(blank=True, null=True)
is_superuser = models.IntegerField()
username = models.CharField(unique=True, max_length=150)
first_name = models.CharField(max_length=150)
last_name = models.CharField(max_length=150)
email = models.CharField(max_length=254)
is_staff = models.IntegerField()
is_active = models.IntegerField()
date_joined = models.DateTimeField()
class Meta:
managed = False
db_table = 'auth_user'
class AuthUserGroups(models.Model):
id = models.BigAutoField(primary_key=True)
user = models.ForeignKey(AuthUser, models.DO_NOTHING)
group = models.ForeignKey(AuthGroup, models.DO_NOTHING)
class Meta:
managed = False
db_table = 'auth_user_groups'
unique_together = (('user', 'group'),)
class AuthUserUserPermissions(models.Model):
id = models.BigAutoField(primary_key=True)
user = models.ForeignKey(AuthUser, models.DO_NOTHING)
permission = models.ForeignKey(AuthPermission, models.DO_NOTHING)
class Meta:
managed = False
db_table = 'auth_user_user_permissions'
unique_together = (('user', 'permission'),)
class CanteenInfo(models.Model):
canteen_id = models.IntegerField(primary_key=True)
canteen_name = models.CharField(max_length=20, blank=True, null=True)
max_capacity = models.IntegerField(blank=True, null=True)
class Meta:
managed = False
db_table = 'canteen_info'
class CuisineInfo(models.Model):
cuisine_id = models.IntegerField(primary_key=True)
store = models.ForeignKey('StoreInfo', models.DO_NOTHING, blank=True, null=True)
cuisine_name = models.CharField(max_length=20, blank=True, null=True)
cuisine_price = models.FloatField(blank=True, null=True)
cuisine_photo = models.TextField(blank=True, null=True)
cuisine_comment = models.CharField(max_length=100, blank=True, null=True)
cuisine_description = models.CharField(max_length=100, blank=True, null=True)
class Meta:
managed = False
db_table = 'cuisine_info'
class CustomerInfo(models.Model):
customer_id = models.IntegerField(primary_key=True)
customer_name = models.CharField(max_length=20, blank=True, null=True)
address = models.CharField(max_length=50, blank=True, null=True)
phone_number = models.DecimalField(max_digits=12, decimal_places=0, blank=True, null=True)
�

.whl
- 粉丝: 3939
- 资源: 4861
最新资源
- 02358 单片机原理及应用.zip
- Java SpringBoot使用Apache POI导入导出Excel文件(源代码)
- Screenshot_20250103_193853.jpg
- 双行程电极可调电阻焊机sw19全套技术资料100%好用.zip
- 托盘供料机sw18可编辑全套技术资料100%好用.zip
- 二维经验模式分解(BEMD)算法在图像上的应用Matlab实现 代码质量极高,方便学习和修改数据使用
- 收料扎制机sw18可编辑全套技术资料100%好用.zip
- 基于Python的决策树用于员工离职预测(数据+代码)
- stm32F3平台,基于sogi pll锁相环的并网逆变资料,含原理图和代码
- 基于AlexNet的FashionMNIST图像分类项目,包含FashionMNIST数据集,使用pytorch框架
- 连接器插拔力abaqus CAE仿真,提供原仿真 3D模型,已经处理好的CAE文件 此模型整体难度中等,适合初学者和自己有点基础的abaqus学习者
- 橡胶自动化称重切料机step全套技术资料100%好用.zip
- Matlab 永磁同步风力发电机 并网故障 低电压穿越策略 可以设计串电阻Bar策略 也可以增加三相故障
- NetCore开发的文件下载器,国外文件地址可下载
- 直驱风机simulink仿真模型,永磁直驱式风力发电系统 matlab simulink整体仿真,有380V和690V两个仿真,波形如图,现有2018 和 2021 两个版本,可导出2015b-202
- 东芝龙系列复合机服务便携手册e-STUDIO2006/2306/2506/2307/2507
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


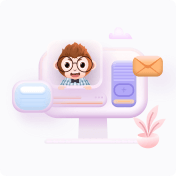