function [out,OKflag] = ISM_AbsCoeff(rttype,rt,room,weight,method,varargin)
%ISM_AbsCoeff Calculates absorption coefficients for a given reverberation time
%
% [ALPHA,OKFLAG] = ISM_AbsCoeff(RT_TYPE,RT_VAL,ROOM,ABS_WEIGHT,METHOD)
% [ALPHA,OKFLAG] = ISM_AbsCoeff( ... ,'c',SOUND_SPEED_VAL)
%
% Returns the six absorption coefficients in the vector ALPHA for a given
% vector of room dimensions ROOM and a given value RT_VAL of reverberation
% time, with RT_TYPE corresponding to the desired measure of reverberation
% time, i.e., either 'T60' or 'T20'. Calling this function with RT_VAL=0
% simply returns ALPHA=[1 1 1 1 1 1] (anechoic case), regardless of the
% settings of the other input parameters.
%
% The parameter ABS_WEIGHTS is a 6 element vector of absorption
% coefficients weights which adjust the relative amplitude ratios between
% the six absorption coefficients in the resulting ALPHA vector. This
% allows the simulation of materials with different absorption levels on
% the room boundaries. Leave empty or set ABS_WEIGHTS=ones(1,6) to obtain
% uniform absorption coefficients for all room boundaries.
%
% If the desired reverberation time could not be reached with the desired
% environmental setup (i.e., practically impossible reverberation time
% value given ROOM and ABS_WEIGHTS), the function will issue a warning on
% screen accordingly. If the function is used with two output arguments,
% the on-screen warnings are disabled and the function sets the flag OKFLAG
% to 0 instead (OKFLAG is set to 1 if the computations are successful).
%
% The returned coefficients are calculated using one of the following
% methods, defined by the METHOD parameter:
%
% * Lehmann and Johansson (METHOD='LehmannJohansson')
% * Sabine (METHOD='Sabine')
% * Norris and Eyring (METHOD='NorrisEyring')
% * Millington-Sette (METHOD='MillingtonSette')
% * Fitzroy (METHOD='Fitzroy')
% * Arau (METHOD='Arau')
% * Neubauer and Kostek (METHOD='NeubauerKostek')
%
% In case the first computation method is selected (i.e., if METHOD is set
% to 'LehmannJohansson'), this function also accepts an additional
% (optional) argument 'c', which will set the value of the sound wave
% propagation speed to SOUND_SPEED_VAL. If omitted, 'c' will default to 343
% m/s. This parameter has no influence on the other six computation
% methods.
%
% Lehmann & Johansson's method relies on a numerical estimation of the
% energy decay in the considered environment, which leads to accurate RT
% prediction results. For more detail, see: "Prediction of energy decay in
% room impulse responses simulated with an image-source model", J. Acoust.
% Soc. Am., vol. 124(1), pp. 269-277, July 2008. The definition of T20 used
% with the 'LehmannJohansson' method corresponds to the time required by
% the energy--time curve to decay from -5 to -25dB, whereas the definition
% of T60 corresponds to the time required by the energy--time curve to
% decay by 60dB from the time lag of the direct path in the transfer
% function.
%
% On the other hand, the last six calculation methods are based on various
% established equations that attempt to predict the physical reverberation
% time T60 resulting from given environmental factors. These methods are
% known to provide relatively inaccurate results. If RT_TYPE='T20', the
% value of T20 for these methods then simply corresponds to T60/3 (linear
% energy decay assumption). For more information, see: "Measurement of
% Absorption Coefficients: Sabine and Random Incidence Absorption
% Coefficients" in the online room acoustics teaching material "AEOF3/AEOF4
% Acoustics of Enclosed Spaces" by Y.W. Lam, The University of Salford,
% 1995, as well as the paper: "Prediction of the Reverberation Time in
% Rectangular Rooms with Non-Uniformly Distributed Sound Absorption" by R.
% Neubauer and B. Kostek, Archives of Acoustics, vol. 26(3), pp. 183�202,
% 2001.
% Release date: November 2009
% Author: Eric A. Lehmann, Perth, Australia (www.eric-lehmann.com)
%
% Copyright (C) 2009 Eric A. Lehmann
%
% This program is free software: you can redistribute it and/or modify
% it under the terms of the GNU General Public License as published by
% the Free Software Foundation, either version 3 of the License, or
% (at your option) any later version.
%
% This program is distributed in the hope that it will be useful,
% but WITHOUT ANY WARRANTY; without even the implied warranty of
% MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
% GNU General Public License for more details.
%
% You should have received a copy of the GNU General Public License
% along with this program. If not, see <http://www.gnu.org/licenses/>.
VarList = {'c' 343}; % default sound propagation speed
eval(SetUserVars(VarList,varargin));
if ~strcmpi(rttype,'t60') && ~strcmpi(rttype,'t20'),
error('Unrecognised ''RT_TYPE'' parameter (must be either ''T60'' or ''T20'').');
end
if rt==0,
out = ones(size(weight));
OKflag = 1;
return
end
if isempty(weight),
weight = ones(1,6);
else
weight = weight./max(weight);
end
if strcmpi(method,'sabine'),
if strcmpi(rttype,'t20'), rt = 3*rt; end % linear energy decay assumption
out = fminbnd(@sabine, 0.0001, 0.9999, [], rt, room, weight);
elseif strcmpi(method,'norriseyring'),
if strcmpi(rttype,'t20'), rt = 3*rt; end % linear energy decay assumption
out = fminbnd(@norris_eyring, 0.0001, 0.9999, [], rt, room, weight);
elseif strcmpi(method,'millingtonsette'),
if strcmpi(rttype,'t20'), rt = 3*rt; end % linear energy decay assumption
out = fminbnd(@millington_sette, 0.0001, 0.9999, [], rt, room, weight);
elseif strcmpi(method,'fitzroy'),
if strcmpi(rttype,'t20'), rt = 3*rt; end % linear energy decay assumption
out = fminbnd(@fitzroy, 0.0001, 0.9999, [], rt, room, weight);
elseif strcmpi(method,'arau'),
if strcmpi(rttype,'t20'), rt = 3*rt; end % linear energy decay assumption
out = fminbnd(@arau, 0.0001, 0.9999, [], rt, room, weight);
elseif strcmpi(method,'neubauerkostek'),
if strcmpi(rttype,'t20'), rt = 3*rt; end % linear energy decay assumption
out = fminbnd(@neubauer_kostek, 0.0001, 0.9999, [], rt, room, weight);
elseif strcmpi(method,'lehmannjohansson'),
if strcmpi(rttype,'t20'),
out = fminbnd(@lehmann_johansson_20, 0.0001, 0.9999, [], rt, room, weight, c);
else
out = fminbnd(@lehmann_johansson_60, 0.0001, 0.9999, [], rt, room, weight, c);
end
else
error('Unrecognised ''METHOD'' parameter (see help for a list of accepted methods).');
end
foo = optimset('fminbnd');
tolx = foo.TolX;
if out<.0001+3*tolx,
if nargout<2,
warning(['Some absorption coefficients are close to the allowable limits (alpha->0). The \n' ...
'resulting reverberation time might end up lower than desired for the given environmental \n' ...
'setup. Try to relax some environmental constraints so that the desired reverberation time \n' ...
'is physically achievable (e.g., by increasing the room volume, increasing the maximum gap \n' ...
'between the absorption weights, or decreasing the desired RT value).%s'],'');
end
OKflag = 0;
elseif out>.9999-3*tolx,
if nargout<2,
warning(['Some absorption coefficients are close to the allowable limits (alpha->1). The \n' ...
'resulting reverberation time might end up higher than desired for the given environmental \n' ...
'setup. Try to relax some environmental constraints so that the desired reverberation time \n' ...
'is physically achievable (e.g., by reducing the room volume, reducing the maximum gap \n' ...
'
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Matlab的声源定位算法源码.zip基于Matlab的声源定位算法源码.zip基于Matlab的声源定位算法源码.zip基于Matlab的声源定位算法源码.zip基于Matlab的声源定位算法源码.zip基于Matlab的声源定位算法源码.zip基于Matlab的声源定位算法源码.zip 【资源说明】 该项目是个人毕设项目,答辩评审分达到95分,代码都经过调试测试,确保可以运行!欢迎下载使用,可用于小白学习、进阶。 该资源主要针对计算机、通信、人工智能、自动化等相关专业的学生、老师或从业者下载使用,亦可作为期末课程设计、课程大作业、毕业设计等。 项目整体具有较高的学习借鉴价值!基础能力强的可以在此基础上修改调整,以实现不同的功能。
资源推荐
资源详情
资源评论
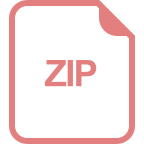
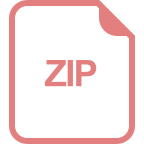
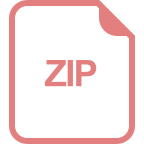
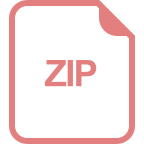
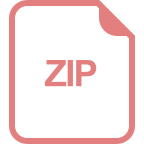
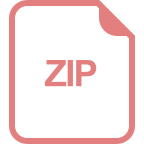
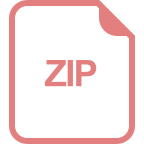
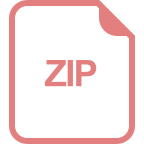
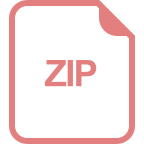
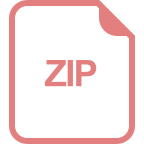
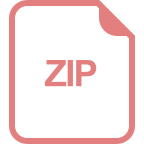
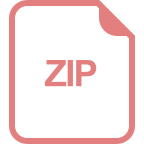
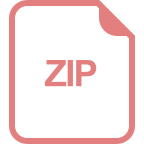
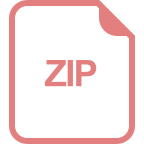
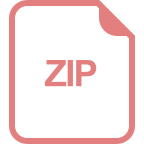
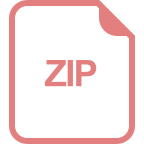
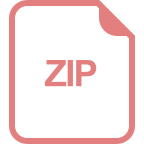
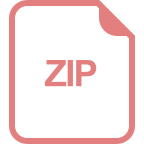
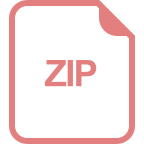
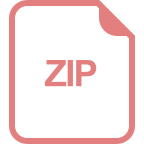
收起资源包目录

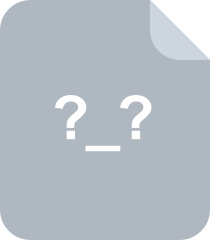
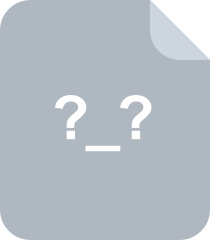
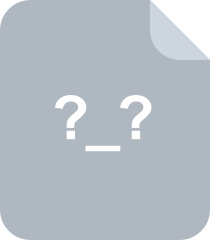
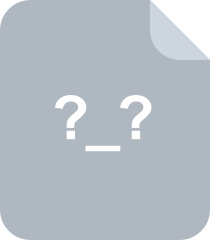
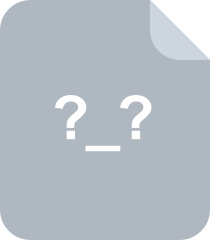
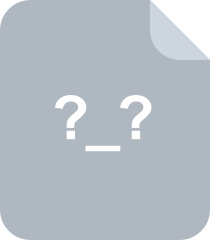
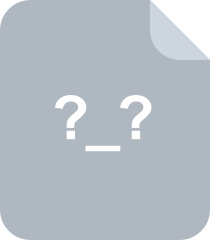
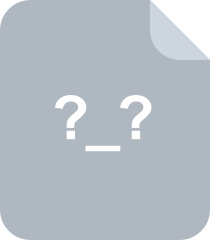
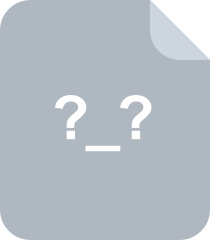
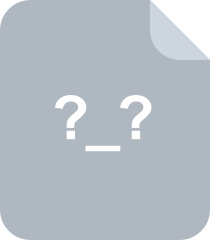
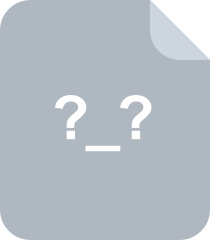
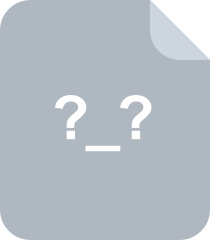
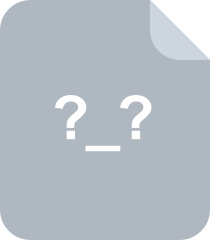
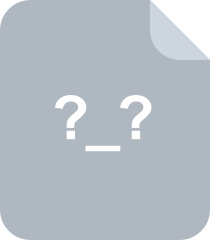
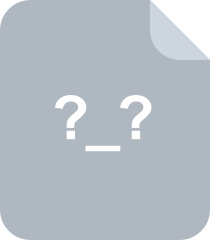
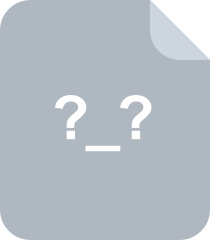
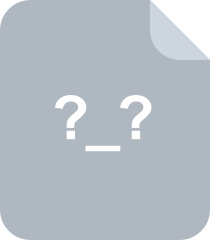
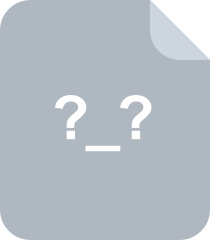
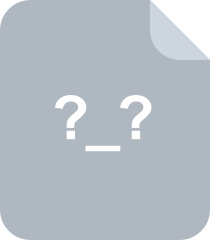
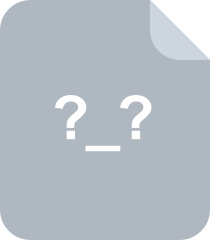
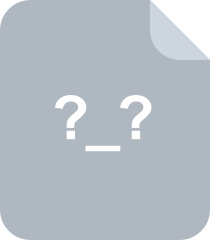
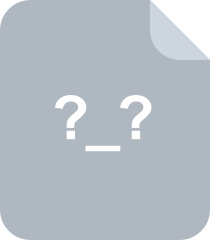
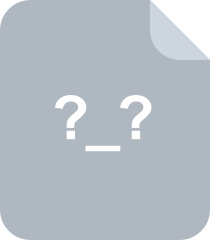
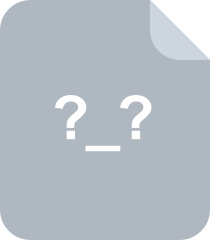
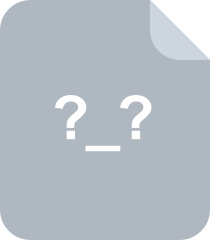
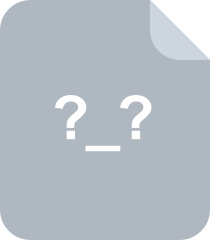
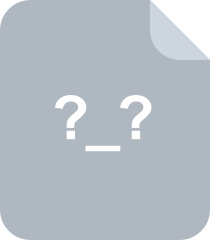
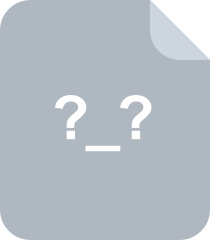
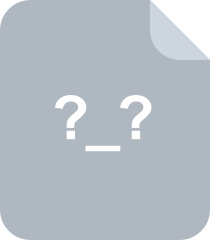
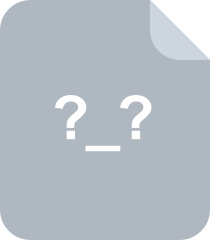
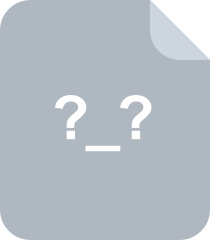
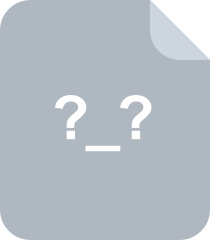
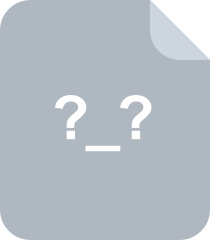
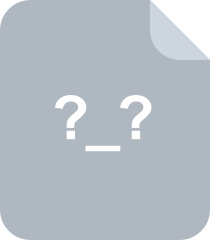
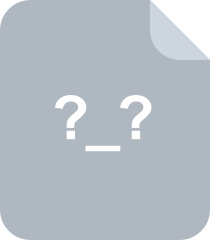
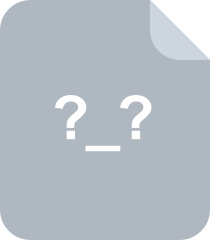
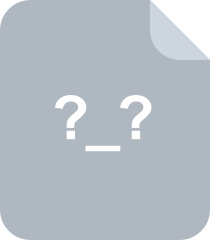
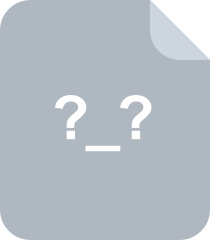
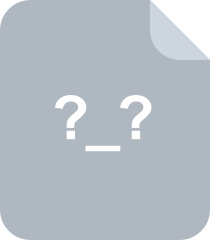
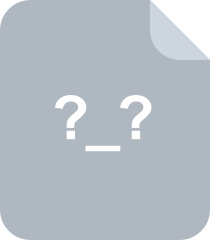
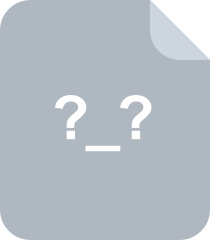
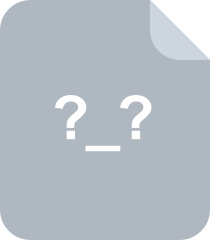
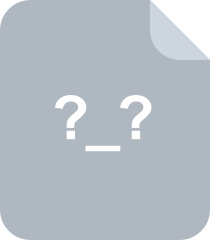
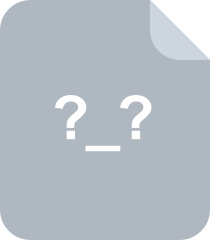
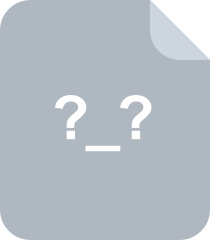
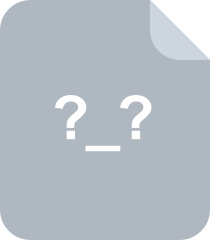
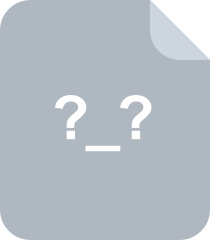
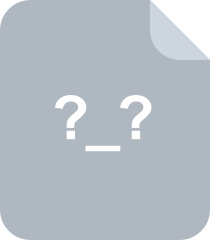
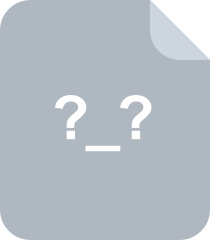
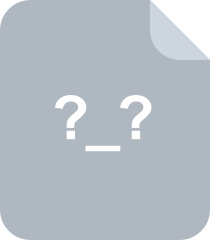
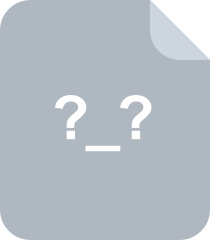
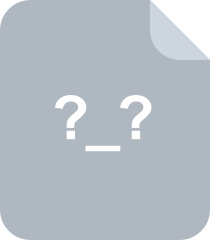
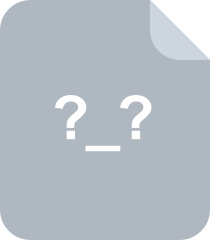
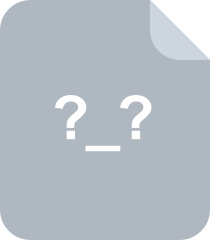
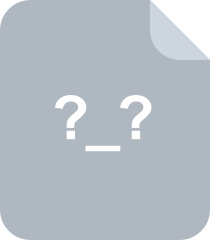
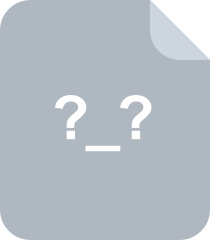
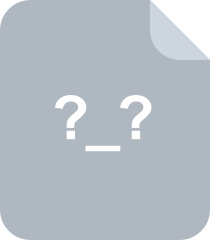
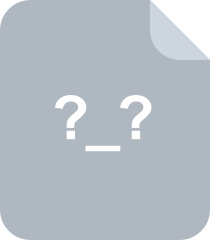
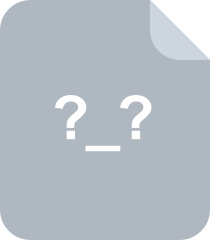
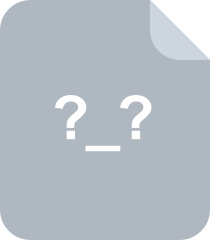
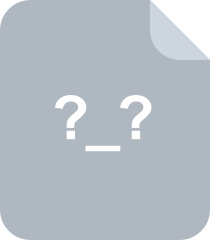
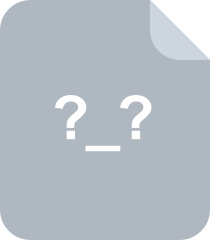
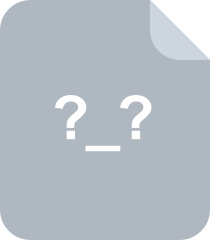
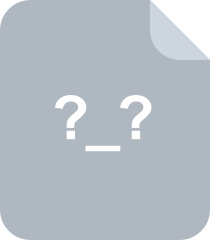
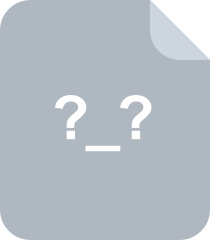
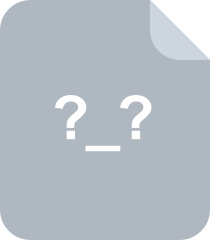
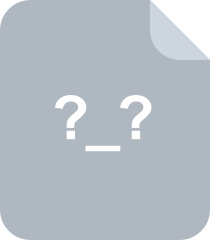
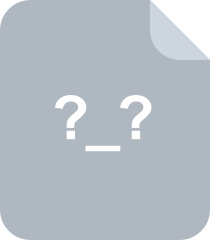
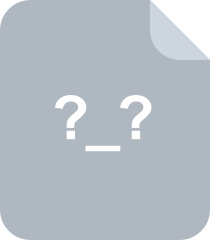
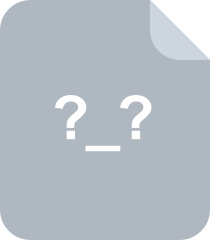
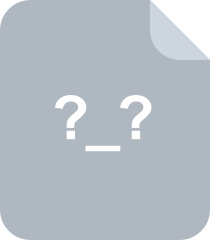
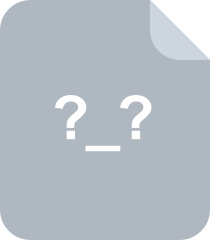
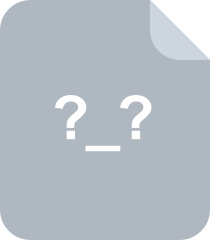
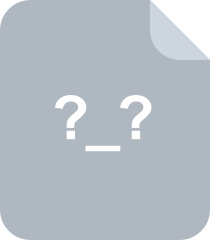
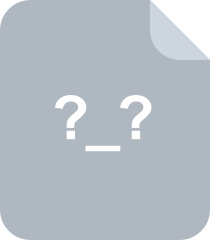
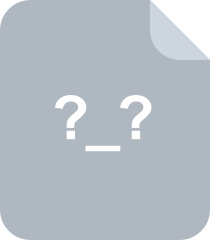
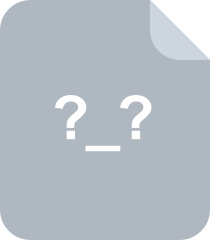
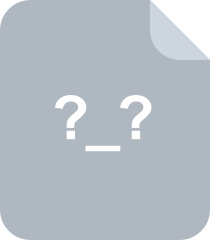
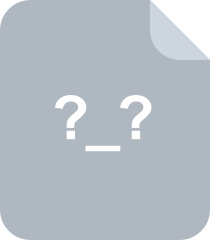
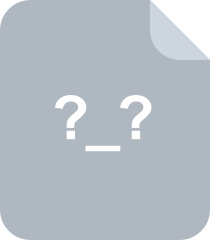
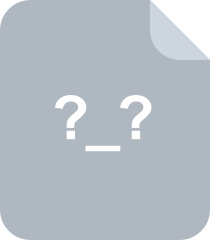
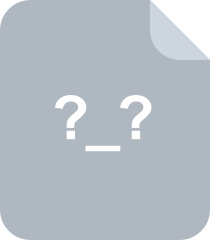
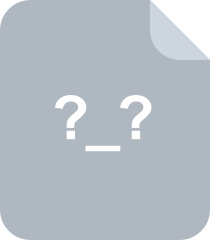
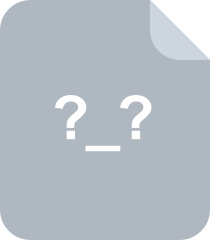
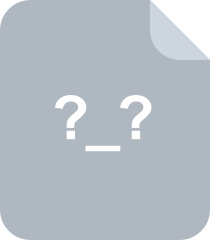
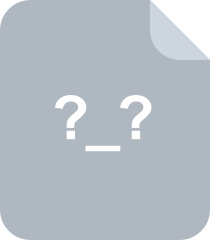
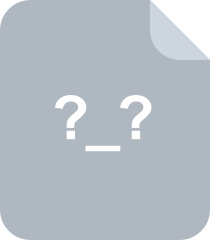
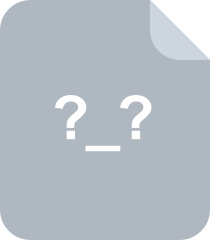
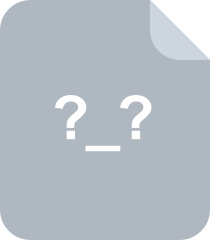
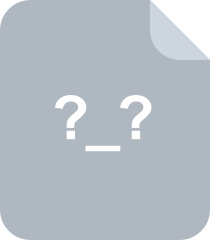
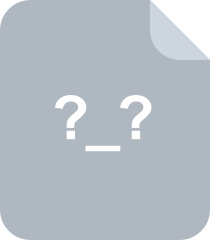
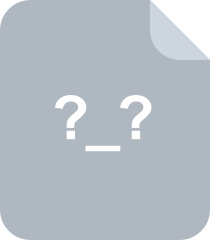
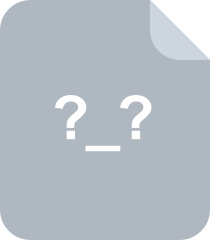
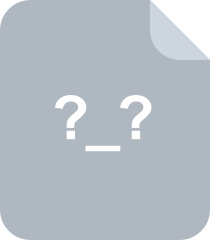
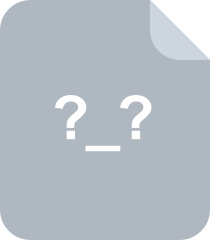
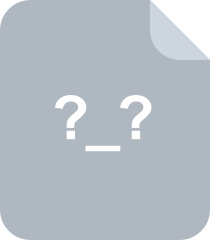
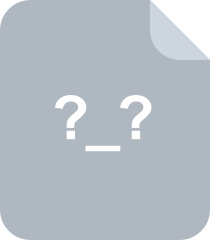
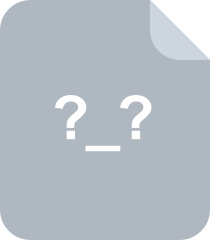
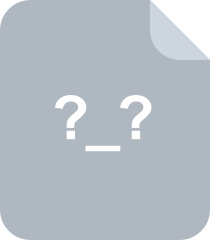
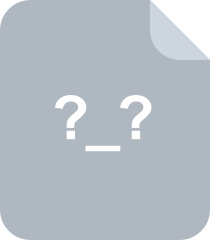
共 126 条
- 1
- 2
资源评论
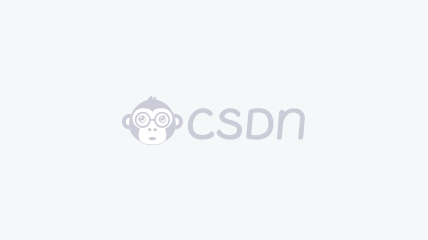
- m0_711840412024-06-04总算找到了自己想要的资源,对自己的启发很大,感谢分享~
- mowosjwzz92024-02-26资源内容总结地很全面,值得借鉴,对我来说很有用,解决了我的燃眉之急。.whl2024-05-28嗯嗯,能解决您的燃眉之急就好
- 2301_799413972024-02-22简直是宝藏资源,实用价值很高,支持!.whl2024-05-28谢谢支持项目,欢迎学习

.whl
- 粉丝: 3823
- 资源: 4648
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

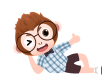
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


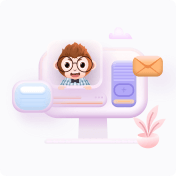
安全验证
文档复制为VIP权益,开通VIP直接复制
