/*********************************************************************
* A C++ class framework for developing NT services
* 一个用于开发NT服务的C++类框架
* IDE: Visual Studio 2022
/*********************************************************************
Classes includes:
class CNTServiceCommandLineInfo:
The CNTServiceCommandLineInfo class aids in parsing the command line at application start-up. It is based almost exactly upon the way that the CCommandLineInfo class in MFC works.
CNT服务命令行信息, 类有助于在应用程序启动时解析命令行。它几乎完全基于MFC中CCommandLineInfo类的工作方式。
class CNTEventLogSource:
CNTEventLogSource provides a wrapper class for writing events to the NT event log. You could consider this as the server side to the Event log APIs.
提供了一个包装类,用于将事件写入NT事件日志。您可以将其视为事件日志API的服务器端。
class CNTService:
CNTService is the class which provides a C++ framework upon which you can develop your own C++ based NT services. The class makes heavy use of virtual functions which your service class should override.
是一个提供C++框架的类,您可以在此框架上开发自己的基于C++的NT服务。该类大量使用服务类应该覆盖的虚拟函数。
class CNTScmService:
CNTScmService is a class which encapsulates a service as returned from the Service Control Manager, in effect a SC_HANDLE. An instance of a CNTScmService is usually acquired by a call to CNTServiceControlManager::Open. Once retrieved the class allows you to control the service, change its configuration and query a service's state.
它封装从服务控制管理器返回的服务,实际上是一个SC_HANDLE。CNTScmService的实例通常通过调用CNTServiceControlManager::Open来获取。一旦检索到该类,就可以控制服务、更改其配置和查询服务的状态。
class CNTServiceControlManager:
CNTServiceControlManager is a class which encapsulates a connection to a Service Control Manager (SCM) on some machine. Functionality provided includes enumeration, database locking and service access.
它封装到某台计算机上的服务控制管理器(SCM)的连接。提供的功能包括枚举、数据库锁定和服务访问。
class CEventLogRecord:
CEventLogRecord is a C++ wrapper class for the EVENTLOGRECORD structure as provided in the SDK. For anyone who has had to use this class using raw SDK calls, you will appreciate the easier access which the class provides.
是SDK中提供的EVENTLOGRECORD结构的C++包装类。对于那些必须使用原始SDK调用来使用此类的人来说,您将欣赏该类提供的更容易访问的功能。
class CNTEventLog:
CNTEventLog is a C++ wrapper class for accessing the NT Event Logs. You can consider this as the client side to the Event Log APIs.
是一个C++包装类,用于访问NT事件日志。您可以将其视为事件日志API的客户端。
Features-特点:
Simple and clean C++ interface using virtual functions.
All the code is Unicode enabled and build configurations are provided.
All code compiles cleanly at the highest warning level of 4. This is the case with all of my other code on my web site as well.
Built in persistence functions which provide support similar to the build in MFC registry / ini functions.
A simple test service has been provided to help you get started developing your own NT services.
Comprehensive documentation is provided for all the classes.
使用虚拟函数的简单干净的C++接口。
所有代码都启用了Unicode,并提供了构建配置。
所有代码都在最高警告级别4下干净地编译。
内置持久性函数,提供类似于内置MFC注册表/ini函数的支持。
提供了一个简单的测试服务来帮助您开始开发自己的NT服务。
为所有类别提供了全面的文档。
附带的zip文件包含类源代码和一个示例NT服务,它会发出声音!
Copyright-版权声明:
You are allowed to include the source code in any product (commercial, shareware, freeware or otherwise) when your product is released in binary form.
You are allowed to modify the source code in any way you want except you cannot modify the copyright details at the top of each module.
If you want to distribute source code with your application, then you are only allowed to distribute versions released by the author. This is to maintain a single distribution point for the source code.
当您的产品以二进制形式发布时,您可以在任何产品(商业、共享软件、免费软件或其他)中包含源代码。
您可以以任何方式修改源代码,但不能修改每个模块顶部的版权详细信息。
如果要随应用程序一起分发源代码,则只允许分发作者发布的版本。这是为了维护源代码的单个分发点。
Updates:
v2.09 (28 March 2024)
Updated copyright details.
Fixed a number of compiler issues reported by Visual Studio 2022.
v2.08 (23 September 2023)
Updated SAL across the code.
v2.07 (10 May 2023)
Updated copyright details
Updated modules to indicate that it needs to be compiled using /std:c++17. Thanks to Martin Richter for reporting this issue.
v2.06 (5 February 2022)
Updated the code to use C++ uniform initialization for all variable declarations
Reworked CNTService::ReportStatus and CNTService::_ReportStatus methods to use std::optional for some of its parameters.
v2.05 (15 January 2022)
Removed defunct _ServiceCtrlHandler method.
Reworked CNTService::RegisterCtrlHandler and CNTService::_ServiceCtrlHandlerEx methods to use third parameter to RegisterServiceCtrlHandlerEx instead of need to use sm_pService static member variable.
Updated the sample service included with the download to log the current system time to the registry value HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Services\PJSERVICE\Parameters\General\CurrentSystemTime.
v2.04 (9 January 2022)
Updated copyright details
Fixed more Clang-Tidy static code analysis warnings in VC 2022 in the code.
30 July 2021
Updated copyright details.
Updated compiler settings to more modern defaults.
v2.03 (26 July 2020)
Fixed more Clang-Tidy static code analysis warnings in the code.
Fixed a compile problem in ntservEventLogRecord.cpp in VC 2019 related to missing iterator header file.
Updated the codebase to support GetSharedServiceRegistryStateKey and GetSharedServiceDirectory APIs.
Fixed up the logic and return values for the CNTService::GetRegistryStateKey and CNTService::GetServiceDirectory methods now that there is proper documentation available for these APIs.
v2.02 (12 April 2020)
Fixed more Clang-Tidy static code analysis warnings in the code.
v2.01 (5 January 2020)
Fixed more Clang-Tidy static code analysis warnings in the code.
v2.0 (28 December 2019)
Fixed various Clang-Tidy static code analysis warnings in the code.
v1.99 (6 November 2019)
Removed unnecessary return value from CNTServiceControlManager::Attach method.
Removed unnecessary return value from CNTScmService::Attach method.
Removed unnecessary return value from CNTEventLogSource::Attach method.
Removed unnecessary return value from CNTEventLog::Attach method.
Replaced BOOL with bool in a number of places throughout the framework.
v1.98 (3 November 2019)
Updated initialization of various structs to use C++ 11 list initialization
v1.97 (26 October 2019)
Updated CNTServiceCommandLineInfo::ParseParam to handle text arguments which may include spaces. This means that for example using the command line form /switch:"Text with Blank
没有合适的资源?快使用搜索试试~ 我知道了~
一个用于开发NT服务的C++类框架
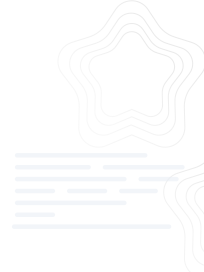
共35个文件
h:13个
cpp:11个
vcxproj:2个

0 下载量 157 浏览量
2024-06-29
07:02:36
上传
评论
收藏 99KB ZIP 举报
温馨提示
IDE: Visual Studio 2022 Classes includes: class CNTServiceCommandLineInfo: CNT服务命令行信息, 类有助于在应用程序启动时解析命令行。它几乎完全基于MFC中CCommandLineInfo类的工作方式。 class CNTEventLogSource: 一个包装类,用于将事件写入NT事件日志。您可以将其视为事件日志API的服务器端。 class CNTService: 一个提供C++框架的类,您可以在此框架上开发自己的基于C++的NT服务。该类大量使用服务类应该覆盖的虚拟函数。 篇幅所限,没办法一一介绍。 在使用本框架前,请仔细阅读项目路径下的README.md
资源推荐
资源详情
资源评论
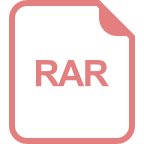
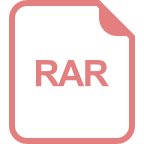
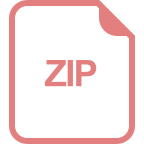
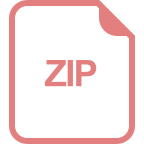
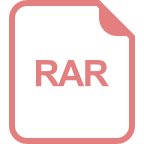
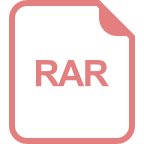
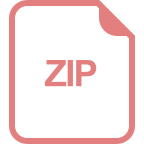
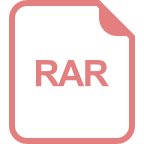
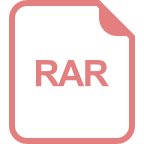
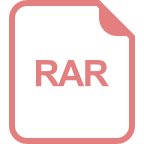
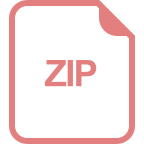
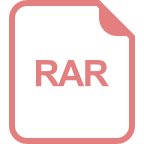
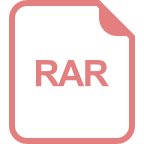
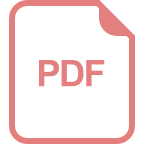
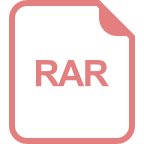
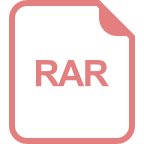
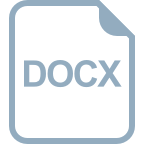
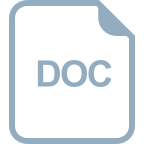
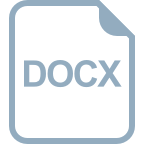
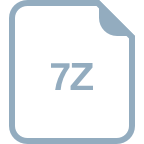
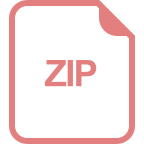
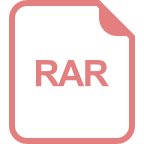
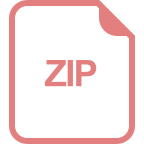
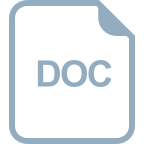
收起资源包目录


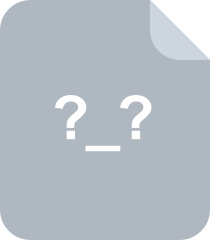
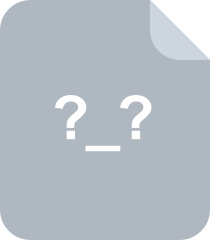
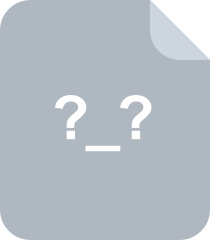
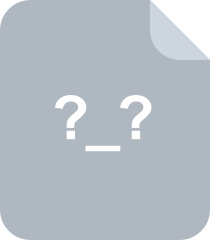
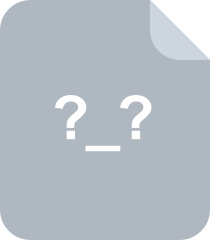
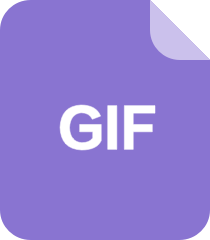
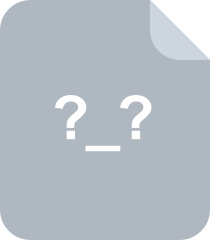
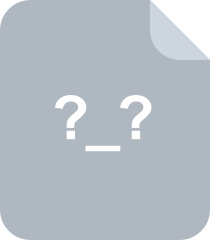
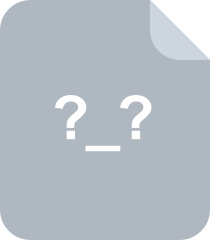
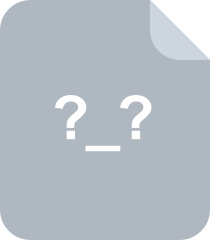
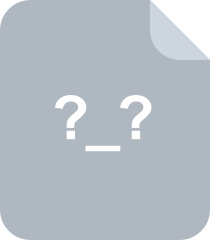
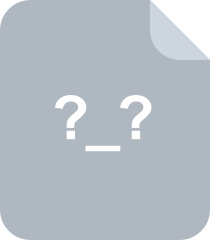
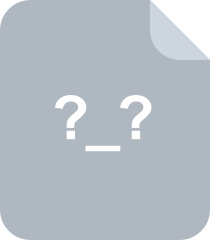
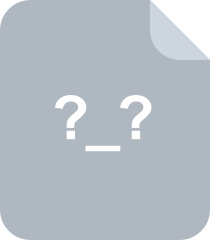
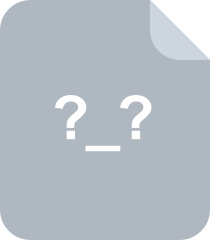

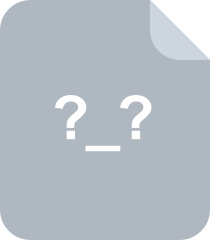
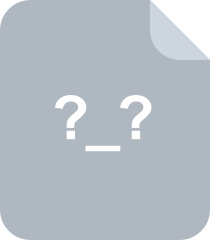
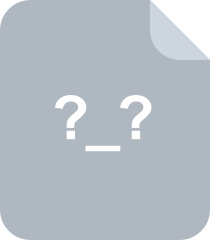
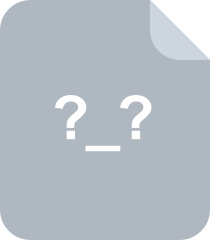
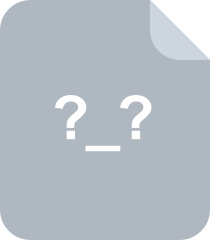
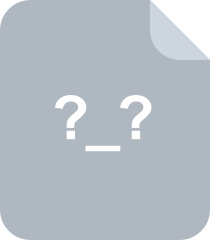
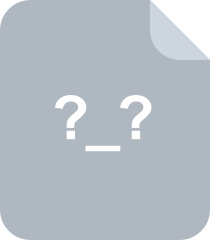
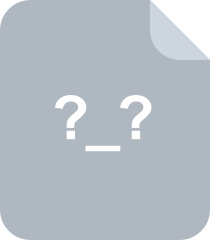
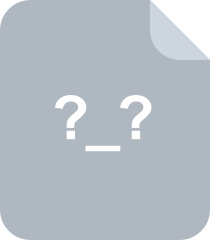
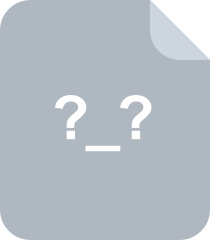
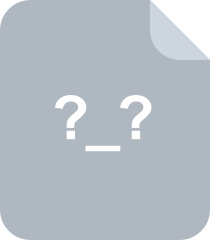
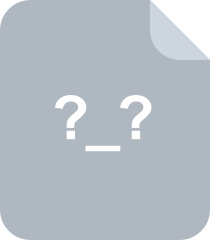
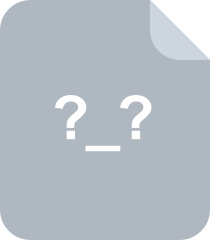
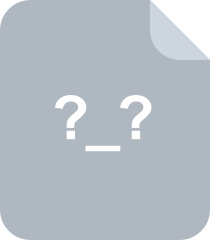
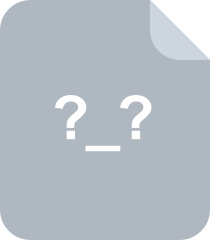
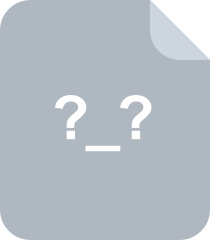
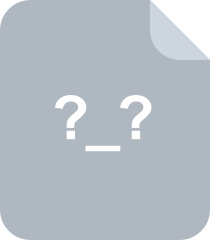
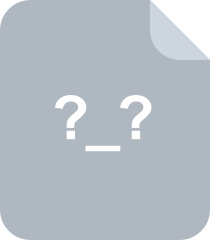
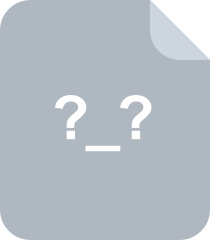
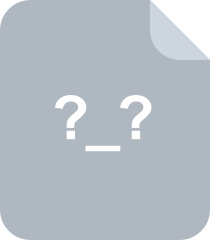
共 35 条
- 1
资源评论
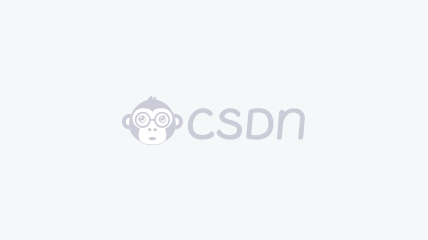

AncleLeen
- 粉丝: 648
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

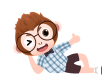
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


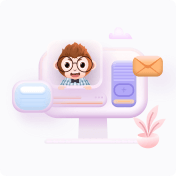
安全验证
文档复制为VIP权益,开通VIP直接复制
