// MainFrm.cpp : implmentation of the CMainFrame class
//
/////////////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "resource.h"
#include "aboutdlg.h"
#include "TaskbarDemoView.h"
#include "MainFrm.h"
BOOL CMainFrame::PreTranslateMessage(MSG* pMsg)
{
if(CFrameWindowImpl<CMainFrame>::PreTranslateMessage(pMsg))
return TRUE;
if(pMsg->message == _WMTaskbarButtonCreated)
{
//ToDo::TaskbarBtn Created
OnTaskbarCreated();
}
return m_view.PreTranslateMessage(pMsg);
}
BOOL CMainFrame::OnIdle()
{
UIUpdateToolBar();
return FALSE;
}
LRESULT CMainFrame::OnCreate(UINT /*uMsg*/, WPARAM /*wParam*/, LPARAM /*lParam*/, BOOL& /*bHandled*/)
{
_WMTaskbarButtonCreated = ::RegisterWindowMessage(L"TaskbarButtonCreated");
::ChangeWindowMessageFilter(_WMTaskbarButtonCreated, MSGFLT_ADD);
m_bProgress = FALSE;
m_nProgress = 0;
// create command bar window
HWND hWndCmdBar = m_CmdBar.Create(m_hWnd, rcDefault, NULL, ATL_SIMPLE_CMDBAR_PANE_STYLE);
// attach menu
m_CmdBar.AttachMenu(GetMenu());
// load command bar images
m_CmdBar.LoadImages(IDR_MAINFRAME);
// remove old menu
SetMenu(NULL);
HWND hWndToolBar = CreateSimpleToolBarCtrl(m_hWnd, IDR_MAINFRAME, FALSE, ATL_SIMPLE_TOOLBAR_PANE_STYLE);
CreateSimpleReBar(ATL_SIMPLE_REBAR_NOBORDER_STYLE);
AddSimpleReBarBand(hWndCmdBar);
AddSimpleReBarBand(hWndToolBar, NULL, TRUE);
CreateSimpleStatusBar();
m_hWndClient = m_view.Create(m_hWnd, rcDefault, NULL, WS_CHILD | WS_VISIBLE | WS_CLIPSIBLINGS | WS_CLIPCHILDREN, WS_EX_CLIENTEDGE);
UIAddToolBar(hWndToolBar);
UISetCheck(ID_VIEW_TOOLBAR, 1);
UISetCheck(ID_VIEW_STATUS_BAR, 1);
// register object for message filtering and idle updates
CMessageLoop* pLoop = _Module.GetMessageLoop();
ATLASSERT(pLoop != NULL);
pLoop->AddMessageFilter(this);
pLoop->AddIdleHandler(this);
CMenuHandle menuMain = m_CmdBar.GetMenu();
m_view.SetWindowMenu(menuMain.GetSubMenu(WINDOW_MENU_POSITION));
return 0;
}
LRESULT CMainFrame::OnDestroy(UINT /*uMsg*/, WPARAM /*wParam*/, LPARAM /*lParam*/, BOOL& bHandled)
{
// unregister message filtering and idle updates
CMessageLoop* pLoop = _Module.GetMessageLoop();
ATLASSERT(pLoop != NULL);
pLoop->RemoveMessageFilter(this);
pLoop->RemoveIdleHandler(this);
bHandled = FALSE;
return 1;
}
LRESULT CMainFrame::OnFileExit(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
PostMessage(WM_CLOSE);
return 0;
}
LRESULT CMainFrame::OnFileNew(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
CTaskbarDemoView* pView = new CTaskbarDemoView;
pView->Create(m_view, rcDefault, NULL, WS_CHILD | WS_VISIBLE | WS_CLIPSIBLINGS | WS_CLIPCHILDREN, 0);
m_view.AddPage(pView->m_hWnd, _T("Document"));
// TODO: add code to initialize document
return 0;
}
LRESULT CMainFrame::OnViewToolBar(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
static BOOL bVisible = TRUE; // initially visible
bVisible = !bVisible;
CReBarCtrl rebar = m_hWndToolBar;
int nBandIndex = rebar.IdToIndex(ATL_IDW_BAND_FIRST + 1); // toolbar is 2nd added band
rebar.ShowBand(nBandIndex, bVisible);
UISetCheck(ID_VIEW_TOOLBAR, bVisible);
UpdateLayout();
return 0;
}
LRESULT CMainFrame::OnViewStatusBar(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
BOOL bVisible = !::IsWindowVisible(m_hWndStatusBar);
::ShowWindow(m_hWndStatusBar, bVisible ? SW_SHOWNOACTIVATE : SW_HIDE);
UISetCheck(ID_VIEW_STATUS_BAR, bVisible);
UpdateLayout();
return 0;
}
LRESULT CMainFrame::OnAppAbout(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
CAboutDlg dlg;
dlg.DoModal();
return 0;
}
LRESULT CMainFrame::OnWindowClose(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
int nActivePage = m_view.GetActivePage();
if(nActivePage != -1)
m_view.RemovePage(nActivePage);
else
::MessageBeep((UINT)-1);
return 0;
}
LRESULT CMainFrame::OnWindowCloseAll(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
m_view.RemoveAllPages();
return 0;
}
LRESULT CMainFrame::OnWindowActivate(WORD /*wNotifyCode*/, WORD wID, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
int nPage = wID - ID_WINDOW_TABFIRST;
m_view.SetActivePage(nPage);
return 0;
}
LRESULT CMainFrame::OnTaskbarCreated()
{
::CoCreateInstance(CLSID_TaskbarList, NULL, CLSCTX_INPROC_SERVER, IID_PPV_ARGS(&_pTaskbar));
_pTaskbar->HrInit();
//TabWindow::EnableCustomPreviews(_hwnd);
return S_OK;
}
LRESULT CMainFrame::OnTaskbaropShowthumbnail(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
// TODO: Add your command handler code here
HICON hicon = ::LoadIcon(NULL, MAKEINTRESOURCE(IDI_SHIELD));
HRESULT hr = _pTaskbar->SetOverlayIcon(m_hWnd, hicon, L"Spelling error");
hr = _pTaskbar->SetProgressState(m_hWnd, TBPF_INDETERMINATE);
return 0;
}
LRESULT CMainFrame::OnTaskbaropShowprogress(WORD /*wNotifyCode*/, WORD /*wID*/, HWND /*hWndCtl*/, BOOL& /*bHandled*/)
{
// TODO: Add your command handler code here
m_bProgress = !m_bProgress;
if(m_bProgress)
{
SetTimer(1, 1000);
}
else
{
KillTimer(1);
_pTaskbar->SetProgressValue(m_hWnd, 0, 10);
}
return 0;
}
LRESULT CMainFrame::OnTimer(UINT /*uMsg*/, WPARAM /*wParam*/, LPARAM /*lParam*/, BOOL& /*bHandled*/)
{
// TODO: Add your message handler code here and/or call default
if(m_bProgress)
{
_pTaskbar->SetProgressValue(m_hWnd, m_nProgress++, 10);
}
return 0;
}
没有合适的资源?快使用搜索试试~ 我知道了~
Windows7 Taskbar特效实例
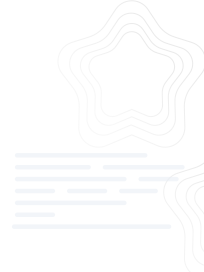
共19个文件
h:6个
cpp:5个
mht:1个

需积分: 9 10 下载量 120 浏览量
2009-12-15
18:59:09
上传
评论
收藏 1.46MB RAR 举报
温馨提示
Windows7 Taskbar特效实例 Windows7 Taskbar特效实例 Windows7 Taskbar特效实例 Windows7 Taskbar特效实例 Windows7 Taskbar特效实例 Windows7 Taskbar特效实例
资源推荐
资源详情
资源评论
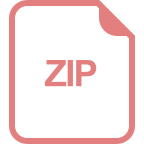
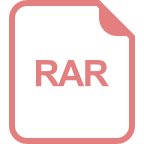
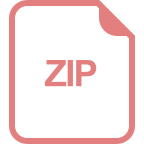
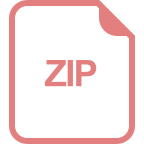
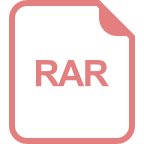
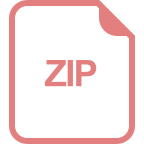
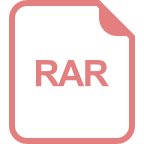
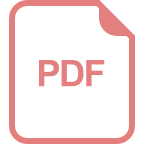
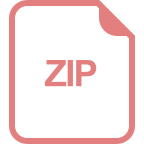
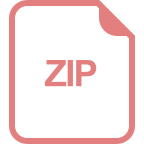
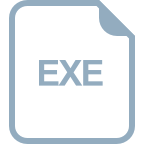
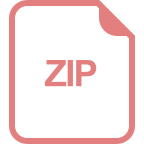
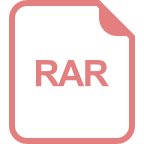
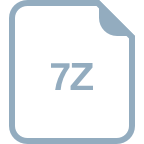
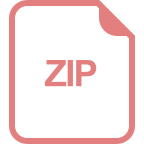
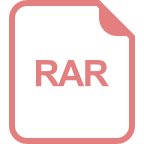
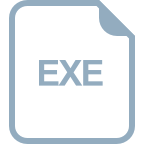
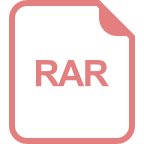
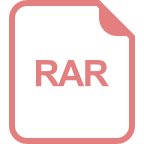
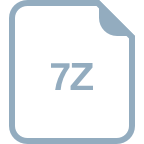
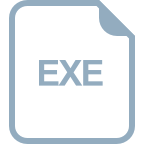
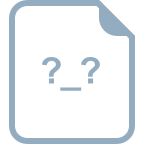
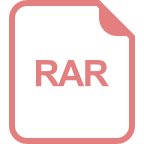
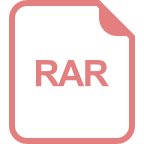
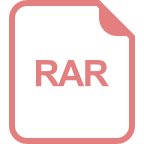
收起资源包目录



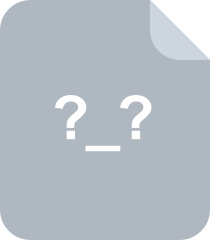
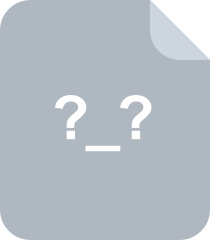
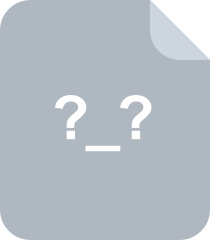
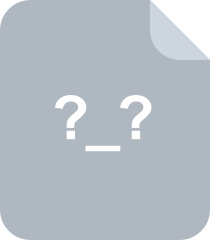
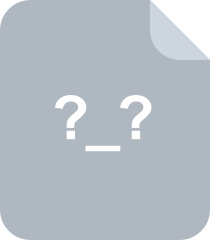
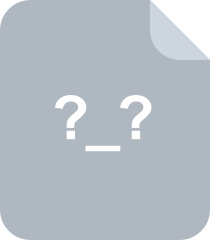
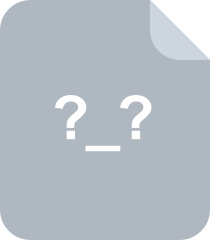
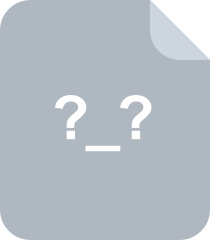
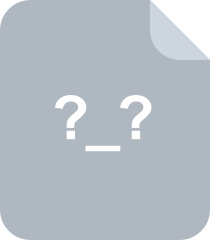
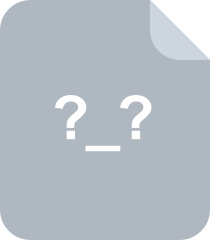
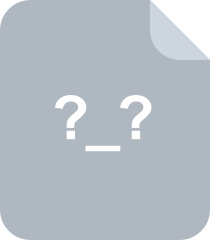
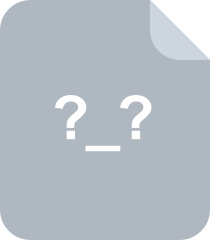
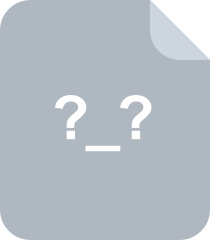
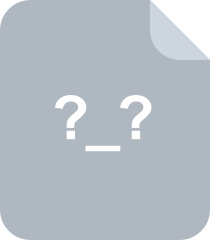
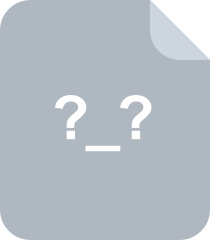

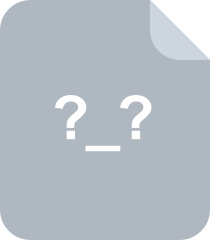
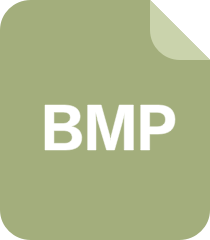
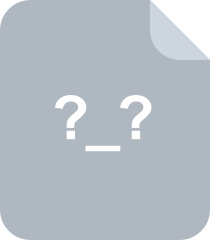
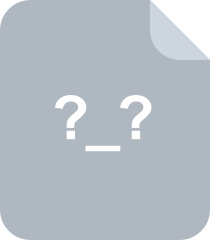
共 19 条
- 1
资源评论
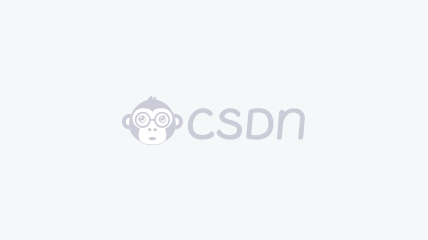

RandomName
- 粉丝: 3
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

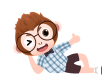
最新资源
- 计算机毕业设计:python+爬虫+cnki网站爬
- nyakumi-lewd-snack-3-4k_720p.7z.002
- 现在微信小程序能用的mqtt.min.js
- 基于MPC的非线性摆锤系统轨迹跟踪控制matlab仿真,包括程序中文注释,仿真操作步骤
- 基于MATLAB的ITS信道模型数值模拟仿真,包括程序中文注释,仿真操作步骤
- 基于Java、JavaScript、CSS的电子产品商城设计与实现源码
- 基于Vue 2的zjc项目设计源码,适用于赶项目需求
- 基于跨语言统一的C++头文件设计源码开发方案
- 基于MindSpore 1.3的T-GCNTemporal Graph Convolutional Network设计源码
- 基于Java的贝塞尔曲线绘制酷炫轮廓背景设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


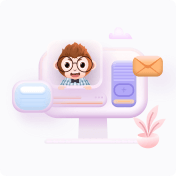
安全验证
文档复制为VIP权益,开通VIP直接复制
