/*
* qrencode - QR Code encoder
*
* Input data chunk class
* Copyright (C) 2006, 2007, 2008, 2009 Kentaro Fukuchi <fukuchi@megaui.net>
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
//#include "config.h"
#include "qrencode.h"
#include "qrspec.h"
#include "bitstream.h"
#include "qrinput.h"
/******************************************************************************
* Entry of input data
*****************************************************************************/
static QRinput_List *QRinput_List_newEntry(QRencodeMode mode, int size, const unsigned char *data)
{
QRinput_List *entry;
if(QRinput_check(mode, size, data)) {
errno = EINVAL;
return NULL;
}
entry = (QRinput_List *)malloc(sizeof(QRinput_List));
if(entry == NULL) return NULL;
entry->mode = mode;
entry->size = size;
entry->data = (unsigned char *)malloc(size);
if(entry->data == NULL) {
free(entry);
return NULL;
}
memcpy(entry->data, data, size);
entry->bstream = NULL;
entry->next = NULL;
return entry;
}
static void QRinput_List_freeEntry(QRinput_List *entry)
{
if(entry != NULL) {
free(entry->data);
BitStream_free(entry->bstream);
free(entry);
}
}
static QRinput_List *QRinput_List_dup(QRinput_List *entry)
{
QRinput_List *n;
n = (QRinput_List *)malloc(sizeof(QRinput_List));
if(n == NULL) return NULL;
n->mode = entry->mode;
n->size = entry->size;
n->data = (unsigned char *)malloc(n->size);
if(n->data == NULL) {
free(n);
return NULL;
}
memcpy(n->data, entry->data, entry->size);
n->bstream = NULL;
n->next = NULL;
return n;
}
/******************************************************************************
* Input Data
*****************************************************************************/
QRinput *QRinput_new(void)
{
return QRinput_new2(0, QR_ECLEVEL_L);
}
QRinput *QRinput_new2(int version, QRecLevel level)
{
QRinput *input;
if(version < 0 || version > QRSPEC_VERSION_MAX || level > QR_ECLEVEL_H) {
errno = EINVAL;
return NULL;
}
input = (QRinput *)malloc(sizeof(QRinput));
if(input == NULL) return NULL;
input->head = NULL;
input->tail = NULL;
input->version = version;
input->level = level;
return input;
}
int QRinput_getVersion(QRinput *input)
{
return input->version;
}
int QRinput_setVersion(QRinput *input, int version)
{
if(version < 0 || version > QRSPEC_VERSION_MAX) {
errno = EINVAL;
return -1;
}
input->version = version;
return 0;
}
QRecLevel QRinput_getErrorCorrectionLevel(QRinput *input)
{
return input->level;
}
int QRinput_setErrorCorrectionLevel(QRinput *input, QRecLevel level)
{
if(level > QR_ECLEVEL_H) {
errno = EINVAL;
return -1;
}
input->level = level;
return 0;
}
static void QRinput_appendEntry(QRinput *input, QRinput_List *entry)
{
if(input->tail == NULL) {
input->head = entry;
input->tail = entry;
} else {
input->tail->next = entry;
input->tail = entry;
}
entry->next = NULL;
}
int QRinput_append(QRinput *input, QRencodeMode mode, int size, const unsigned char *data)
{
QRinput_List *entry;
entry = QRinput_List_newEntry(mode, size, data);
if(entry == NULL) {
return -1;
}
QRinput_appendEntry(input, entry);
return 0;
}
/**
* Insert a structured-append header to the head of the input data.
* @param input input data.
* @param size number of structured symbols.
* @param index index number of the symbol. (1 <= index <= size)
* @param parity parity among input data. (NOTE: each symbol of a set of structured symbols has the same parity data)
* @retval 0 success.
* @retval -1 error occurred and errno is set to indeicate the error. See Execptions for the details.
* @throw EINVAL invalid parameter.
* @throw ENOMEM unable to allocate memory.
*/
//__STATIC
static int QRinput_insertStructuredAppendHeader(QRinput *input, int size, int index, unsigned char parity)
{
QRinput_List *entry;
unsigned char buf[3];
if(size > MAX_STRUCTURED_SYMBOLS) {
errno = EINVAL;
return -1;
}
if(index <= 0 || index > MAX_STRUCTURED_SYMBOLS) {
errno = EINVAL;
return -1;
}
buf[0] = (unsigned char)size;
buf[1] = (unsigned char)index;
buf[2] = parity;
entry = QRinput_List_newEntry(QR_MODE_STRUCTURE, 3, buf);
if(entry == NULL) {
return -1;
}
entry->next = input->head;
input->head = entry;
return 0;
}
void QRinput_free(QRinput *input)
{
QRinput_List *list, *next;
if(input != NULL) {
list = input->head;
while(list != NULL) {
next = list->next;
QRinput_List_freeEntry(list);
list = next;
}
free(input);
}
}
static unsigned char QRinput_calcParity(QRinput *input)
{
unsigned char parity = 0;
QRinput_List *list;
int i;
list = input->head;
while(list != NULL) {
if(list->mode != QR_MODE_STRUCTURE) {
for(i=list->size-1; i>=0; i--) {
parity ^= list->data[i];
}
}
list = list->next;
}
return parity;
}
QRinput *QRinput_dup(QRinput *input)
{
QRinput *n;
QRinput_List *list, *e;
n = QRinput_new2(input->version, input->level);
if(n == NULL) return NULL;
list = input->head;
while(list != NULL) {
e = QRinput_List_dup(list);
if(e == NULL) {
QRinput_free(n);
return NULL;
}
QRinput_appendEntry(n, e);
list = list->next;
}
return n;
}
/******************************************************************************
* Numeric data
*****************************************************************************/
/**
* Check the input data.
* @param size
* @param data
* @return result
*/
static int QRinput_checkModeNum(int size, const char *data)
{
int i;
for(i=0; i<size; i++) {
if(data[i] < '0' || data[i] > '9')
return -1;
}
return 0;
}
/**
* Estimates the length of the encoded bit stream of numeric data.
* @param size
* @return number of bits
*/
int QRinput_estimateBitsModeNum(int size)
{
int w;
int bits;
w = size / 3;
bits = w * 10;
switch(size - w * 3) {
case 1:
bits += 4;
break;
case 2:
bits += 7;
break;
default:
break;
}
return bits;
}
/**
* Convert the number data to a bit stream.
* @param entry
* @retval 0 success
* @retval -1 an error occurred and errno is set to indeicate the error.
* See Execptions for the details.
* @throw ENOMEM unable to allocate memory.
*/
static int QRinput_encodeModeNum(QRinput_List *entry, int version)
{
int words, i, ret;
unsigned int val;
words = entry->size / 3;
entry->bstream = BitStream_new();
if(entry->bstream == NULL) return -1;
val = 0x1;
ret = BitStream_appendNum(entry->bstream, 4, val);
if(ret < 0) goto ABORT;
val = entry->size;
ret = BitStream_appendNum(entry->bstream, QRspec_lengthIndicator(QR_MODE_NUM, version), val);
if(ret < 0) goto ABORT;
for(i=0; i<words; i++) {
val = (entry->data[i*3 ] - '0') * 100;
val += (entry->data[i*3+1] - '0') * 10;
val += (entry->data[i*3+2] - '0');
ret = BitStream_appendNum(entry->bstream, 10, val);
if(ret < 0) goto ABORT;
}
if(entry->size - words * 3 == 1) {
val = entry->data[words*3] - '0';
ret = BitStream_appendNum(entry->bstream, 4, val);
if(ret < 0) goto ABORT;
} else if(entry->size - words * 3 == 2) {
val = (entry->data[words*3 ] - '0') * 10;
val += (entry->data[words*3+1] - '0');
BitStream_appendNum(entry->bstream, 7, val);
if(ret < 0) goto ABORT;
}
ret
没有合适的资源?快使用搜索试试~ 我知道了~
毕业设计: 天气预报、星座运势、老黄历
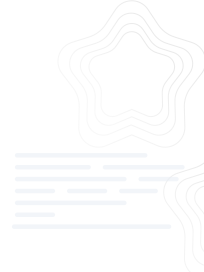
共1063个文件
png:390个
json:298个
h:145个

4 下载量 134 浏览量
2023-02-07
19:50:09
上传
评论 2
收藏 13.09MB ZIP 举报
温馨提示
毕业设计: 天气预报、星座运势、老黄历 一个集天气预报、星座运势、老黄历为一身的超级无敌可直接拿去做毕业设计的项目 1.日历黄历 2.星座运势 3.天气预报 4.主题切换 日历黄历 星座运势 天气预报 主题切换
资源推荐
资源详情
资源评论
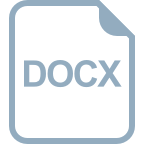
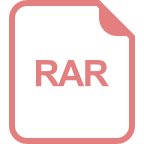
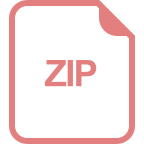
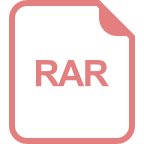
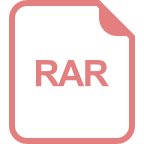
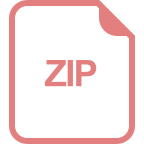
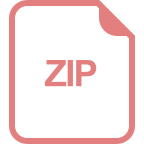
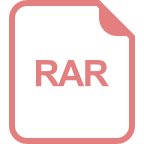
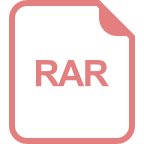
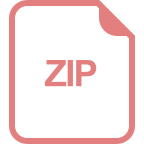
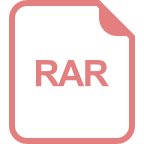
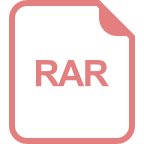
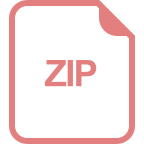
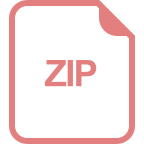
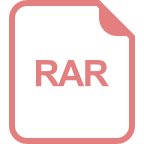
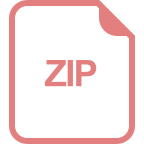
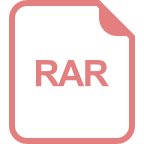
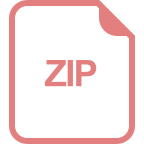
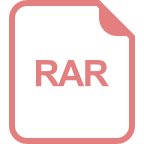
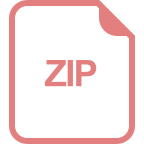
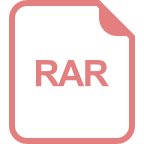
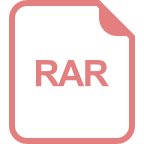
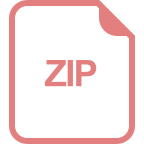
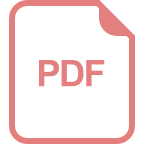
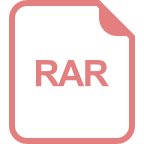
收起资源包目录

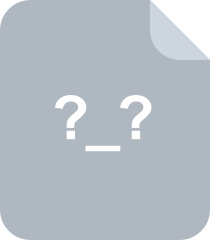
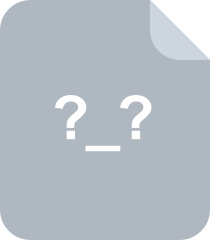
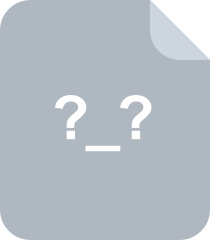
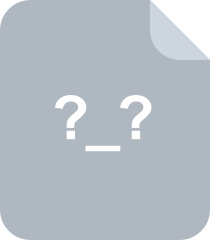
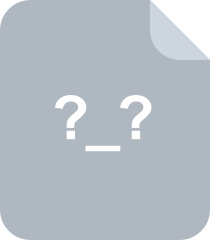
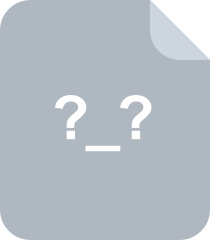
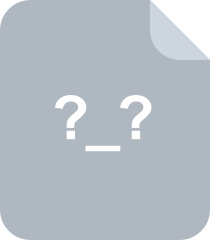
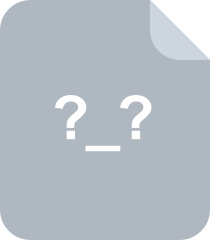
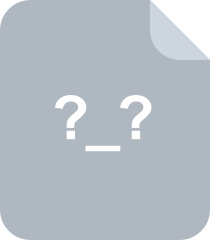
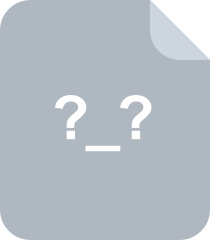
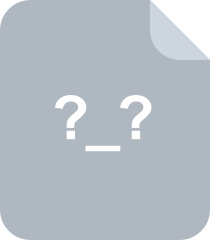
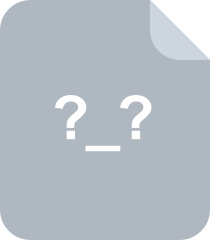
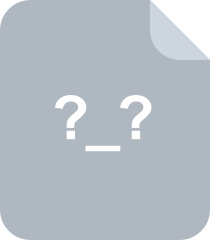
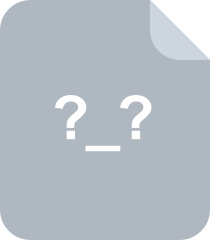
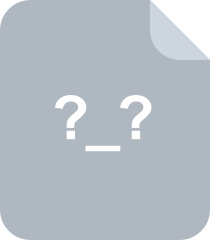
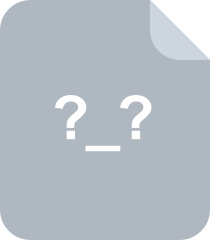
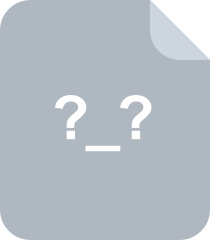
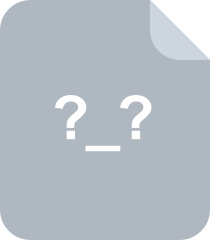
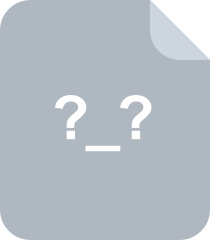
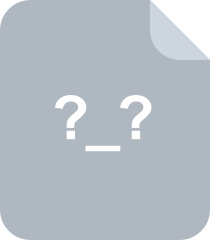
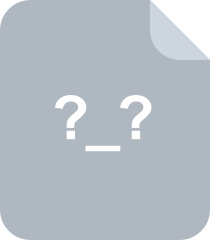
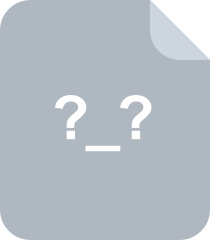
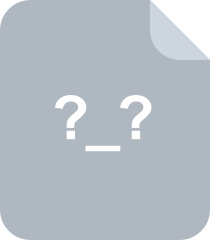
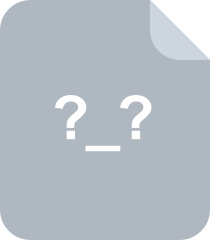
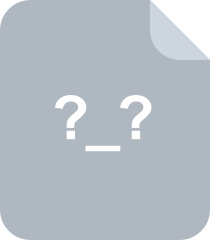
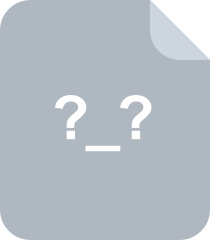
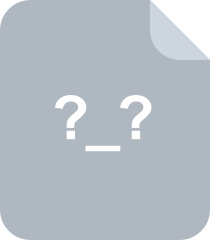
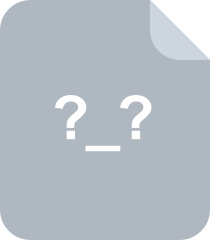
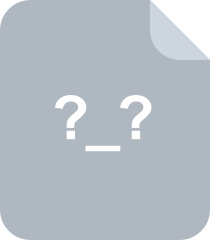
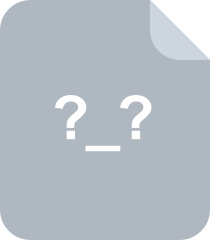
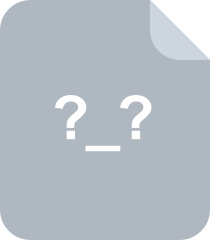
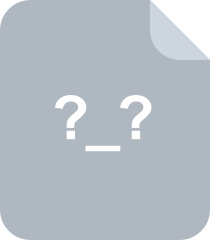
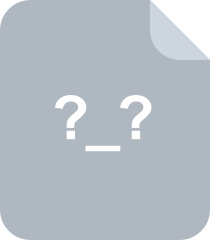
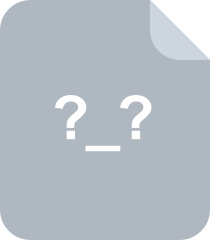
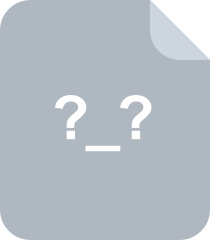
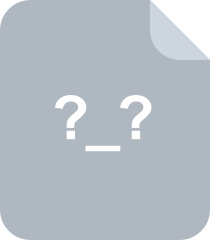
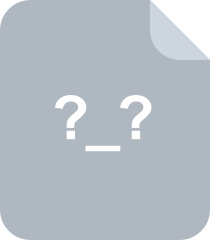
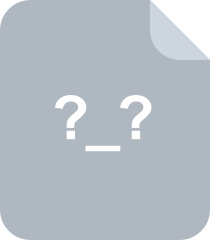
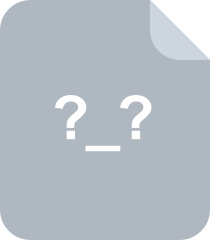
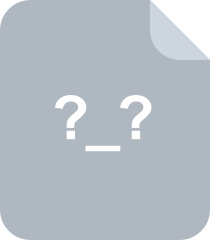
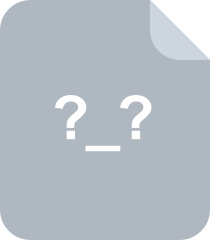
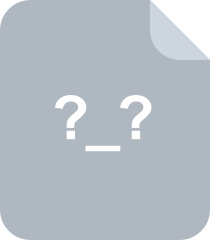
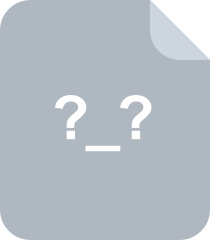
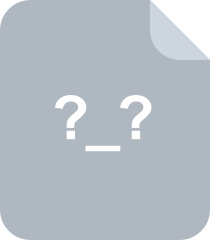
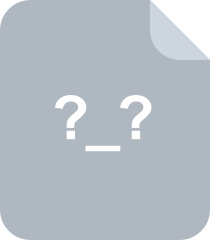
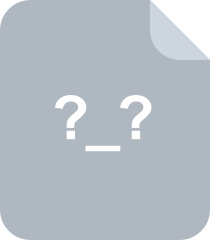
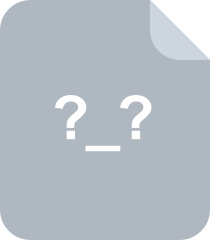
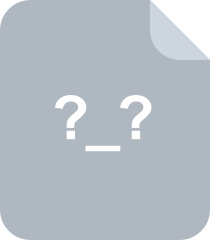
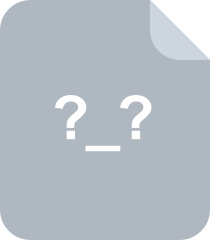
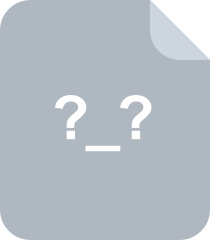
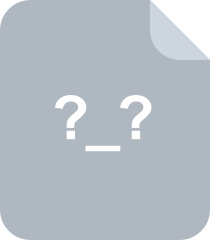
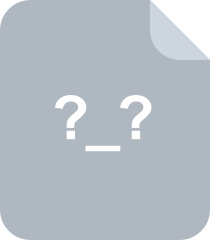
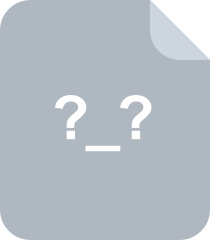
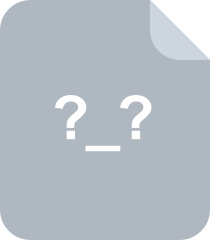
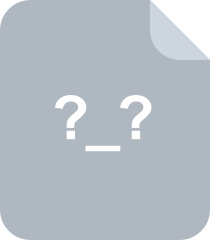
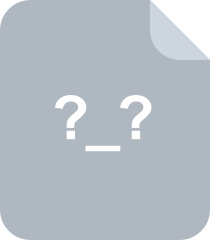
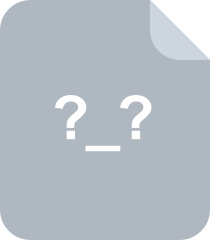
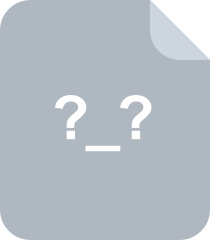
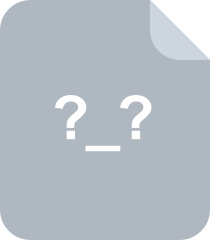
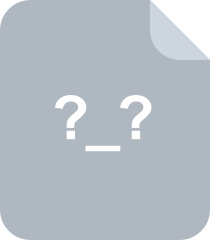
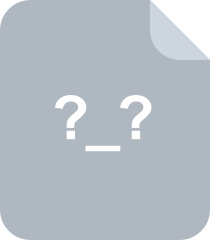
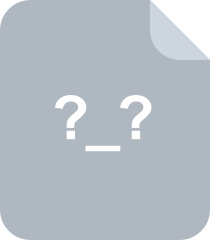
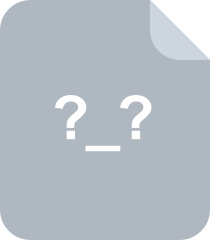
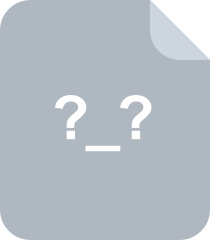
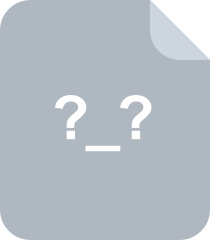
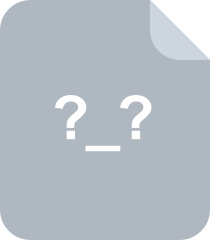
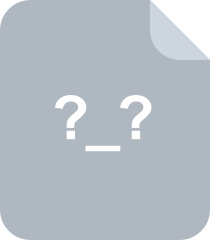
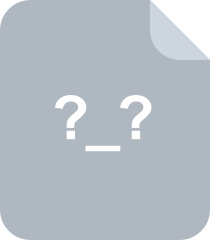
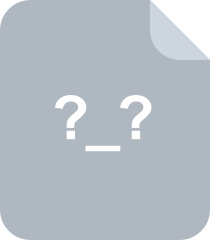
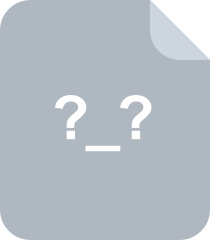
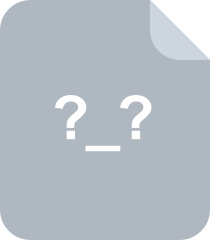
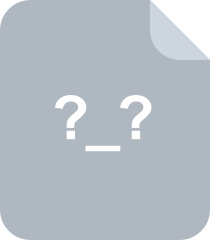
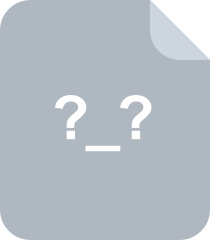
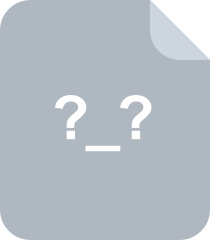
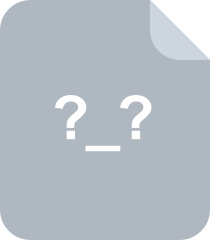
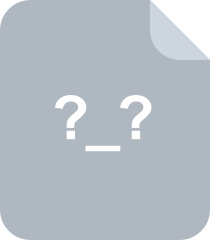
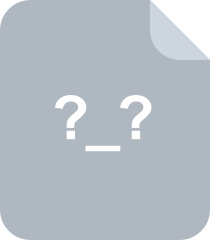
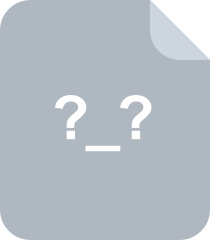
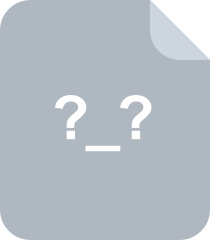
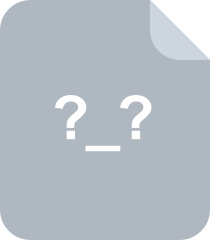
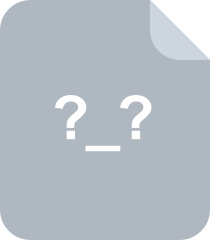
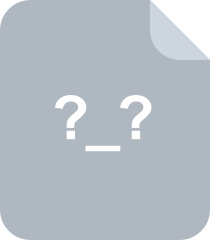
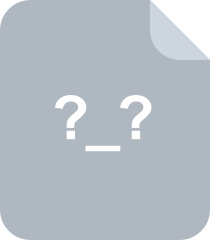
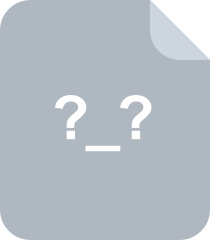
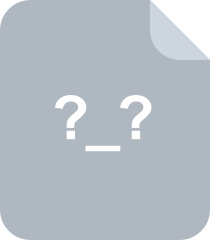
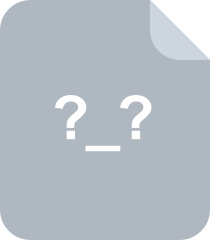
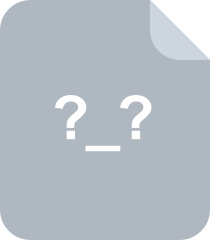
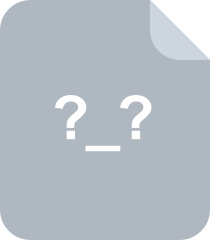
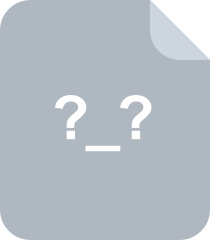
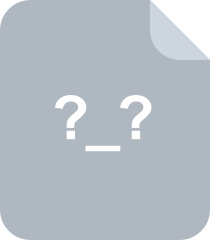
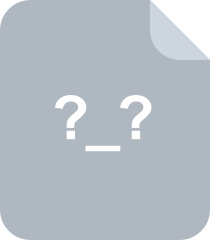
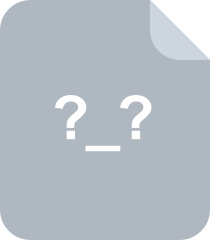
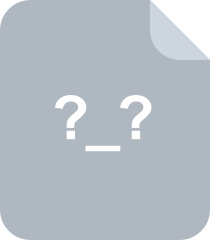
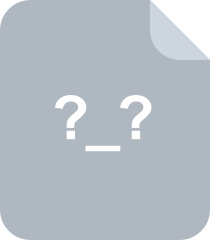
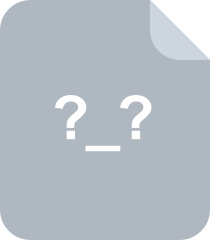
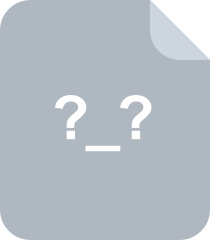
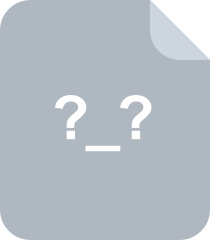
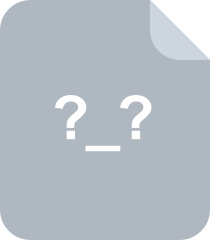
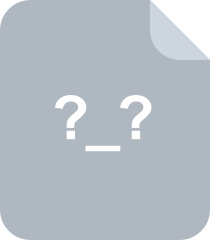
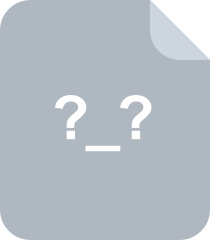
共 1063 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
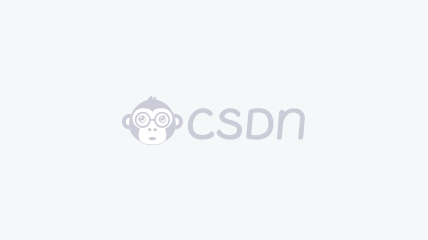

三季人G
- 粉丝: 134
- 资源: 2369
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

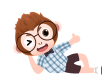
最新资源
- 统一平台 mes 管理系统 vue
- 开心消消乐【python实战小游戏】学习开发路上的最好实战教程.zip
- 利用Gurobi求解工厂生产规划问题代码
- 华为HCIE考试文档.zip
- 2010-2023英语二小作文真题范文.pdf
- bpm 流程管理系统 vue2
- C#ASP.NET视频会议OA源码+手机版OA源码带二次开发文档数据库 SQL2008源码类型 WebForm
- django旅游服务系统程序源码88939
- 【安卓毕业设计】图书管理系统安卓修改源码(完整前后端+mysql+说明文档).zip
- 【安卓毕业设计】基于安卓平台学生课堂质量采集分析查询系统源码(完整前后端+mysql+说明文档).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


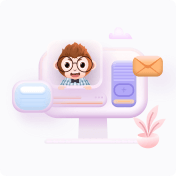
安全验证
文档复制为VIP权益,开通VIP直接复制
