let info;
let currentPage = {};
const app = getApp();
function getSystemInfo() {
if (info) return info;
info = wx.getSystemInfoSync();
return info;
}
function isIos() {
const sys = getSystemInfo();
return /iphone|ios/i.test(sys.platform);
}
/**
* 获取当前页面实例
*/
function getCurrentPage() {
const pages = getCurrentPages();
const last = pages.length - 1;
return pages[last];
}
/**
* new Date 区分平台
* @param {number} year
* @param {number} month
* @param {number} day
*/
function newDate(year, month, day) {
let cur = `${year}-${month}-${day}`;
if (isIos()) {
cur = `${year}/${month}/${day}`;
}
return new Date(cur);
}
/**
* todo 数组去重
* @param {array} array todo 数组
*/
function uniqueTodoLabels(array = []) {
let uniqueObject = {};
let uniqueArray = [];
array.forEach(item => {
uniqueObject[`${item.year}-${item.month}-${item.day}`] = item;
});
for (let i in uniqueObject) {
uniqueArray.push(uniqueObject[i]);
}
return uniqueArray;
}
/**
* 上滑
* @param {object} e 事件对象
* @returns {boolean} 布尔值
*/
export function isUpSlide(e) {
const { startX, startY } = this.data.gesture;
if (this.slideLock) {
const t = e.touches[0];
const deltaX = t.clientX - startX;
const deltaY = t.clientY - startY;
if (deltaY < -60 && deltaX < 20 && deltaX > -20) {
this.slideLock = false;
return true;
} else {
return false;
}
}
}
/**
* 下滑
* @param {object} e 事件对象
* @returns {boolean} 布尔值
*/
export function isDownSlide(e) {
const { startX, startY } = this.data.gesture;
if (this.slideLock) {
const t = e.touches[0];
const deltaX = t.clientX - startX;
const deltaY = t.clientY - startY;
if (deltaY > 60 && deltaX < 20 && deltaX > -20) {
this.slideLock = false;
return true;
} else {
return false;
}
}
}
/**
* 左滑
* @param {object} e 事件对象
* @returns {boolean} 布尔值
*/
export function isLeftSlide(e) {
const { startX, startY } = this.data.gesture;
if (this.slideLock) {
const t = e.touches[0];
const deltaX = t.clientX - startX;
const deltaY = t.clientY - startY;
if (deltaX < -60 && deltaY < 20 && deltaY > -20) {
this.slideLock = false;
return true;
} else {
return false;
}
}
}
/**
* 右滑
* @param {object} e 事件对象
* @returns {boolean} 布尔值
*/
export function isRightSlide(e) {
const { startX, startY } = this.data.gesture;
if (this.slideLock) {
const t = e.touches[0];
const deltaX = t.clientX - startX;
const deltaY = t.clientY - startY;
if (deltaX > 60 && deltaY < 20 && deltaY > -20) {
this.slideLock = false;
return true;
} else {
return false;
}
}
}
// function info(msg) {
// console.log('%cInfo: %c' + msg, 'color:#FF0080;font-weight:bold', 'color: #FF509B');
// }
function warn(msg) {
console.log(
'%cWarn: %c' + msg,
'color:#FF6600;font-weight:bold',
'color: #FF9933'
);
}
function tips(msg) {
console.log(
'%cTips: %c' + msg,
'color:#00B200;font-weight:bold',
'color: #00CC33'
);
}
const conf = {
/**
* 计算指定月份共多少天
* @param {number} year 年份
* @param {number} month 月份
*/
getThisMonthDays(year, month) {
return new Date(year, month, 0).getDate();
},
/**
* 计算指定月份第一天星期几
* @param {number} year 年份
* @param {number} month 月份
*/
getFirstDayOfWeek(year, month) {
return new Date(Date.UTC(year, month - 1, 1)).getDay();
},
/**
* 计算指定日期星期几
* @param {number} year 年份
* @param {number} month 月份
* @param {number} date 日期
*/
getDayOfWeek(year, month, date) {
return new Date(Date.UTC(year, month - 1, date)).getDay();
},
/**
* 渲染日历
* @param {number} curYear
* @param {number} curMonth
* @param {number} curDate
*/
renderCalendar(curYear, curMonth, curDate) {
conf.calculateEmptyGrids.call(this, curYear, curMonth);
conf.calculateDays.call(this, curYear, curMonth, curDate);
const { todoLabels } = this.data.calendar || {};
const { afterCalendarRender } = this.config;
if (todoLabels && todoLabels instanceof Array) {
conf.setTodoLabels.call(this);
}
if (
afterCalendarRender &&
typeof afterCalendarRender === 'function' &&
!this.firstRender
) {
afterCalendarRender();
this.firstRender = true;
}
},
/**
* 计算当前月份前后两月应占的格子
* @param {number} year 年份
* @param {number} month 月份
*/
calculateEmptyGrids(year, month) {
conf.calculatePrevMonthGrids.call(this, year, month);
conf.calculateNextMonthGrids.call(this, year, month);
},
/**
* 计算上月应占的格子
* @param {number} year 年份
* @param {number} month 月份
*/
calculatePrevMonthGrids(year, month) {
let empytGrids = [];
const prevMonthDays = conf.getThisMonthDays(year, month - 1);
const firstDayOfWeek = conf.getFirstDayOfWeek(year, month);
if (firstDayOfWeek > 0) {
const len = prevMonthDays - firstDayOfWeek;
for (let i = prevMonthDays; i > len; i--) {
empytGrids.push(i);
}
this.setData({
'calendar.empytGrids': empytGrids.reverse()
});
} else {
this.setData({
'calendar.empytGrids': null
});
}
},
/**
* 计算下月应占的格子
* @param {number} year 年份
* @param {number} month 月份
*/
calculateNextMonthGrids(year, month) {
let lastEmptyGrids = [];
const thisMonthDays = conf.getThisMonthDays(year, month);
const lastDayWeek = conf.getDayOfWeek(year, month, thisMonthDays);
if (+lastDayWeek !== 6) {
const len = 7 - (lastDayWeek + 1);
for (let i = 1; i <= len; i++) {
lastEmptyGrids.push(i);
}
this.setData({
'calendar.lastEmptyGrids': lastEmptyGrids
});
} else {
this.setData({
'calendar.lastEmptyGrids': null
});
}
},
/**
* 设置日历面板数据
* @param {number} year 年份
* @param {number} month 月份
*/
calculateDays(year, month, curDate) {
let days = [];
const {
todayTimestamp,
disableDays = [],
enableArea = [],
enableDays = [],
enableAreaTimestamp = []
} = this.data.calendar;
const thisMonthDays = conf.getThisMonthDays(year, month);
let expectEnableDaysTimestamp = converEnableDaysToTimestamp(enableDays);
if (enableArea.length) {
expectEnableDaysTimestamp = delRepeatedEnableDay(enableDays, enableArea);
}
let selectedDay = [];
if (this.config.defaultDay !== undefined && !this.config.defaultDay) {
selectedDay = [];
this.config.defaultDay = undefined;
} else {
selectedDay = curDate
? [
{
day: curDate,
choosed: true,
year,
month
}
]
: this.data.calendar.selectedDay;
}
for (let i = 1; i <= thisMonthDays; i++) {
days.push({
day: i,
choosed: false,
year,
month
});
}
const selectedDayCol = selectedDay.map(
d => `${d.year}-${d.month}-${d.day}`
);
const disableDaysCol = disableDays.map(
d => `${d.year}-${d.month}-${d.day}`
);
days.forEach(item => {
const cur = `${item.year}-${item.month}-${item.day}`;
if (selectedDayCol.indexOf(cur) !== -1) item.choosed = true;
if (disableDaysCol.indexOf(cur) !== -1) item.disable = true;
const timestamp = newDate(item.year, item.month, item.day).getTime();
let setDisable = false;
if (enableAreaTimestamp.length) {
if (
(+enableAreaTimestamp[0] > +timestamp ||
+timestamp > +enableAreaTimestamp[1]) &&
!expectEnableDaysTimestamp.includes(+timestamp)

小徐博客
- 粉丝: 1976
- 资源: 5883
最新资源
- springboot项目家乡特色推荐系统.zip
- 电源开关电源200W 12V 24V,0.95效率 集成PFC+LLC方案稳定,电路外围简单,工作稳定,多重保护,低纹波,低成本,超高效率,芯片好买 电源架构PFC+LLC+同步整流,高效率高功率
- springboot项目基于vue的地方美食分享网站.zip
- springboot项目基于web的智慧养老平台.zip
- springboot项目基于Web的社区医院管理服务系统.zip
- springboot项目基于Springboot的漫画网站.zip
- springboot项目基于vue的MOBA类游戏攻略分享平台.zip
- springboot项目基于SpringBoot的冬奥会科普平台.zip
- [Matlab Simulink] 电动汽车制动能量回收 刹车充电仿真 PMSM永磁同步电机转速SVPWM控制 双有源桥DAB移相控制 电动汽车充放电 个人搭建,确保运行
- springboot项目基于Java的超市进销存系统.zip
- springboot项目基于Spring Boot的在线考试系统.zip
- springboot项目基于SpringBoot的CSGO赛事管理系统.zip
- springboot项目广场舞团.zip
- springboot项目高校食堂移动预约点餐系统.zip
- springboot项目会员制医疗预约服务管理信息系统.zip
- springboot项目福聚苑社区团购.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


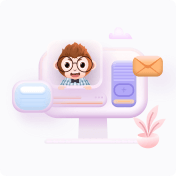