import { config } from '../config.js'
const codeMessage = {
200: '服务器成功返回请求的数据。',
201: '新建或修改数据成功。',
202: '一个请求已经进入后台排队(异步任务)。',
204: '删除数据成功。',
400: '发出的请求有错误,服务器没有进行新建或修改数据的操作。',
401: '用户没有权限(令牌、用户名、密码错误)。',
403: '用户得到授权,但是访问是被禁止的。',
404: '发出的请求针对的是不存在的记录,服务器没有进行操作。',
406: '请求的格式不可得。',
410: '请求的资源被永久删除,且不会再得到的。',
422: '当创建一个对象时,发生一个验证错误。',
500: '服务器发生错误,请检查服务器。',
502: '网关错误。',
503: '服务不可用,服务器暂时过载或维护。',
504: '网关超时。',
}
export default class HTTP {
//*** 微信请求接口 ****//
static request(url,{ data = {}, method = 'GET', dataType = 'json',...otherParams}) {
return new Promise((resolve, reject) => {
wx.request({
url: url,
data: data,
method: method,
dataType: dataType,
...otherParams,
header: {
'ACJSESSIONID': wx.getStorageSync('ACJSESSIONID')|| [],
'content-type': 'application/json;charset=UTF-8',
},
success: (res) => {
//startsWith 头的截取 //endsWith 尾巴的截取
const code = res.statusCode.toString()
if (code.startsWith('2')) {
} else {
this._show_error(code)
}
},
fail: (err) => {
reject(err)
this._show_error(504)
}
})
})
}
//*** 微信下载文件接口 ****//
static downloadFile(params){
return new Promise((resolve,reject)=>{
wx.downloadFile({
url: 'https://example.com/audio/123', //仅为示例,并非真实的资源
success (res) {
// 只要服务器有响应数据,就会把响应内容写入文件并进入 success 回调,业务需要自行判断是否下载到了想要的内容
if (res.statusCode === 200) {
// wx.playVoice({ filePath: res.tempFilePath })
resolve(res)
}
}
})
})
}
//*** 微信上传文件接口 ****//
static uploadFile(params){
return new Promise((resolve,reject)=>{
// wx.chooseImage({
// success (res) {
// const tempFilePaths = res.tempFilePaths
wx.uploadFile({
// url: 'https://example.weixin.qq.com/upload', //仅为示例,非真实的接口地址
// filePath: tempFilePaths[0],
// name: 'file',
// formData: {
// 'user': 'test'
// },
...params,
success (res){
const data = res.data
if(data){
resolve(data)
}
}
})
// }
// })
})
}
_show_error(code) {
wx.showToast({
title: codeMessage ? codeMessage[code] : codeMessage[1],
icon: 'none',
duration: 1500
})
}
};
//todo kim-stamp设置缓存 wx.setStorageSync('ACJSESSIONID', ACJSESSIONID)
//—————————————————————————————— 微信请求接口文档 ———————————————————————————————————————————
// RequestTask wx.request(Object object)
// 发起 HTTPS 网络请求。使用前请注意阅读相关说明。
// 参数
// Object object
// 属性 类型 默认值 必填 说明 最低版本
// url string 是 开发者服务器接口地址
// data string/object/ArrayBuffer 否 请求的参数
// header Object 否 设置请求的 header,header 中不能设置 Referer。
// content-type 默认为 application/json
// method string GET 否 HTTP 请求方法
// dataType string json 否 返回的数据格式
// responseType string text 否 响应的数据类型 1.7.0
// success function 否 接口调用成功的回调函数
// fail function 否 接口调用失败的回调函数
// complete function 否 接口调用结束的回调函数(调用成功、失败都会执行)
// object.method 的合法值
// 值 说明 最低版本
// OPTIONS HTTP 请求 OPTIONS
// GET HTTP 请求 GET
// HEAD HTTP 请求 HEAD
// POST HTTP 请求 POST
// PUT HTTP 请求 PUT
// DELETE HTTP 请求 DELETE
// TRACE HTTP 请求 TRACE
// CONNECT HTTP 请求 CONNECT
// object.dataType 的合法值
// 值 说明 最低版本
// json 返回的数据为 JSON,返回后会对返回的数据进行一次 JSON.parse
// 其他 不对返回的内容进行 JSON.parse
// object.responseType 的合法值
// 值 说明 最低版本
// text 响应的数据为文本
// arraybuffer 响应的数据为 ArrayBuffer
// object.success 回调函数
// 参数 Object res
// 属性 类型 说明 最低版本
// data string/Object/Arraybuffer 开发者服务器返回的数据
// statusCode number 开发者服务器返回的 HTTP 状态码
// header Object 开发者服务器返回的 HTTP Response Header 1.2.0
// ------------------------------------------------------------------------------------
// 通常http的header()
// header()函数的作用是:发送一个原始 HTTP 标头[Http Header]到客户端。
// 标头 (header) 是服务器以 HTTP 协义传 HTML 资料到浏览器前所送出的字串,在标头与 HTML 文件之间尚需空一行分隔。在 PHP 中送回 HTML 资料前,需先传完所有的标头。
// 常用header汇总:
// header('Content-Type: text/html; charset=utf-8'); //网页编码
// header('Content-Type: text/plain'); //纯文本格式
// header('Content-Type: image/jpeg'); //JPG、JPEG
// header('Content-Type: application/zip'); // ZIP文件
// header('Content-Type: application/pdf'); // PDF文件
// header('Content-Type: audio/mpeg'); // 音频文件
// header('Content-type: text/css'); //css文件
// header('Content-type: text/javascript'); //js文件
// header('Content-type: application/json'); //json
// header('Content-type: application/pdf'); //pdf
// header('Content-type: text/xml'); //xml
// header('Content-Type: application/x-shockw**e-flash'); //Flash动画
// ------------------------------------------------------------------------------------
// data 参数说明
// 最终发送给服务器的数据是 String 类型,如果传入的 data 不是 String 类型,会被转换成 String 。转换规则如下:
// 对于 GET 方法的数据,会将数据转换成 query string(encodeURIComponent(k)=encodeURIComponent(v)&encodeURIComponent(k)=encodeURIComponent(v)...)
// 对于 POST 方法且 header['content-type'] 为 application/json 的数据,会对数据进行 JSON 序列化
// 对于 POST 方法且 header['content-type'] 为 application/x-www-form-urlencoded 的数据,会将数据转换成 query string (encodeURIComponent(k)=encodeURIComponent(v)&encodeURIComponent(k)=encodeURIComponent(v)...)
// 返回值
// RequestTask
// 基础库 1.4.0 开始支持,低版本需做兼容处理。
// 请求任务对象
// RequestTask.abort() 中断请求任务
// RequestTask.onHeadersReceived(function callback) 监听 HTTP Response Header 事件。会比请求完成事件更早
// RequestTask.offHeadersReceived(function callback) 取消监听 HTTP Response Header 事件
// 示例代码
// const requestTask = wx.request({
// url: '
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
收起资源包目录

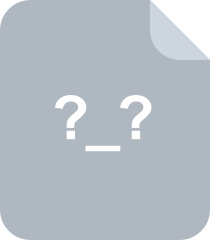
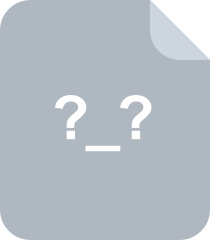
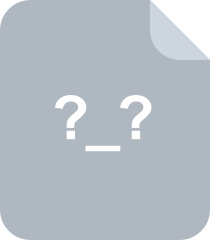
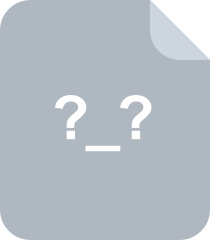
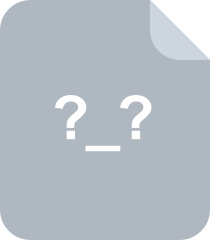
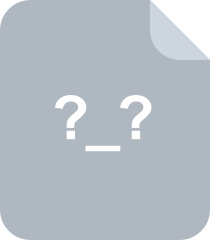
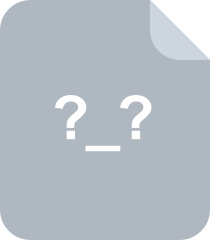
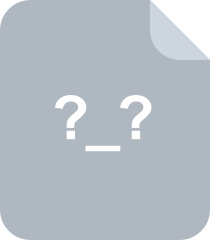
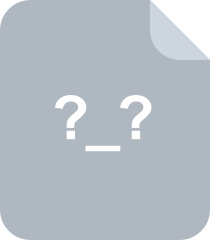
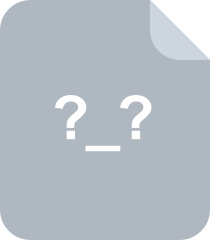
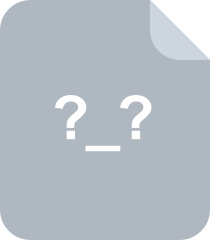
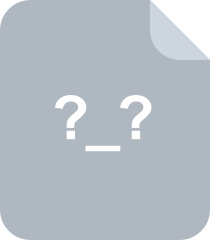
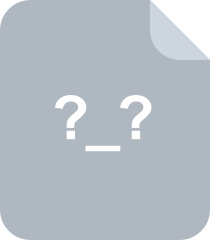
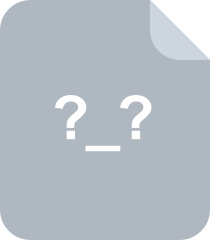
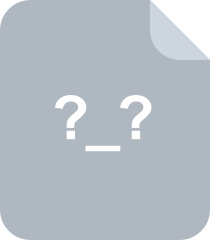
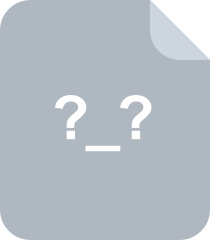
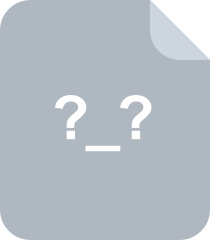
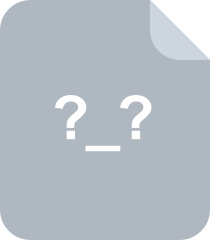
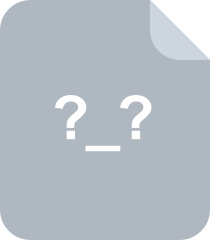
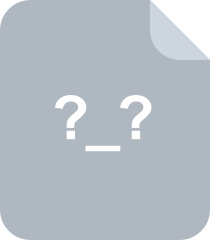
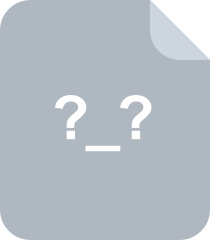
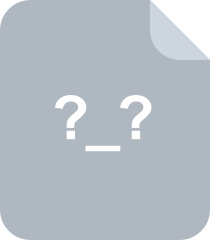
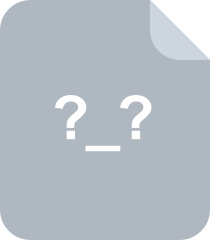
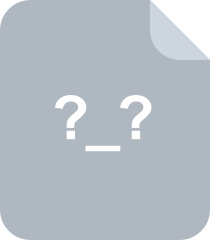
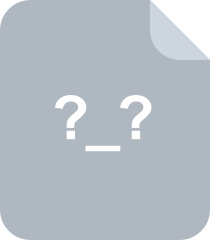
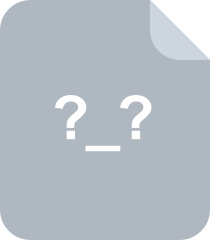
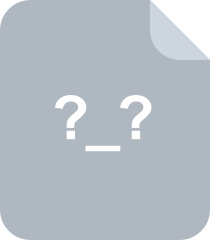
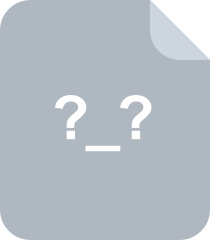
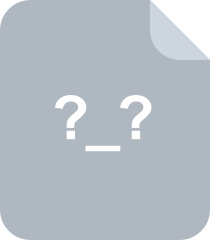
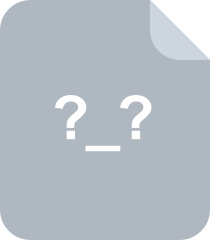
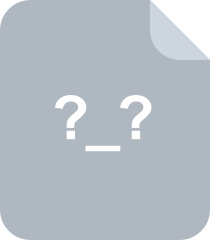
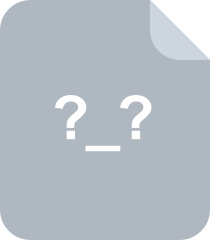
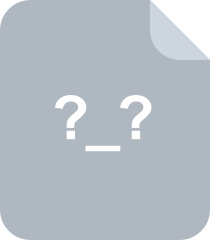
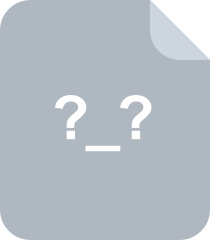
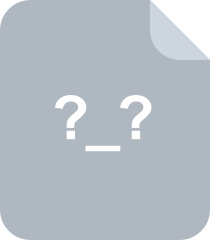
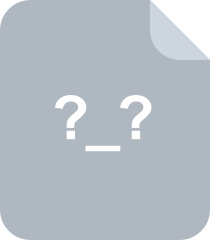
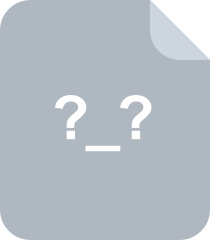
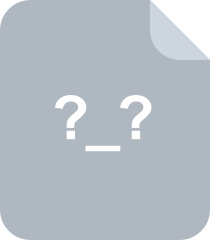
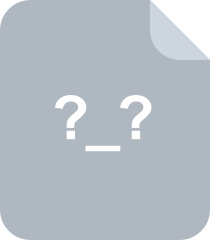
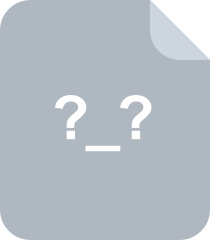
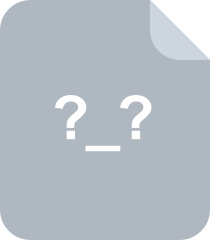
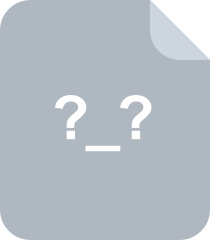
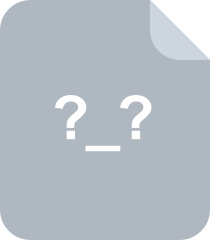
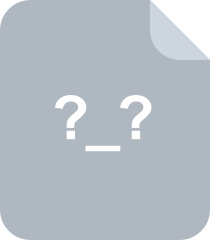
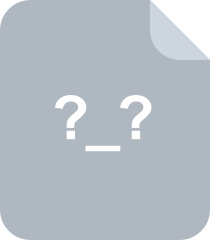
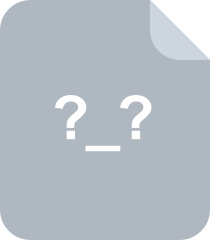
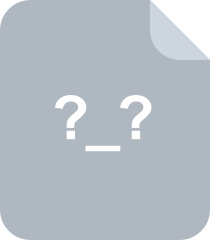
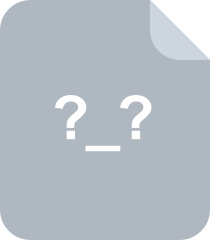
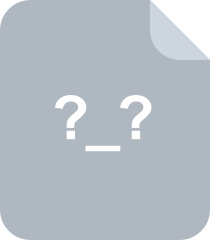
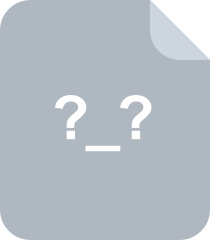
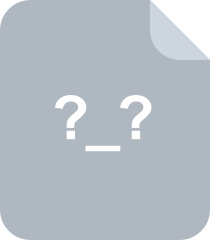
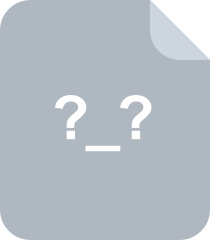
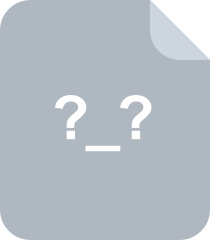
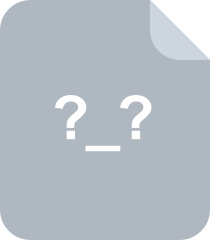
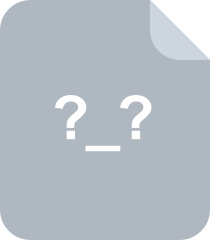
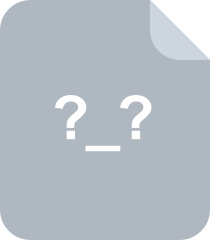
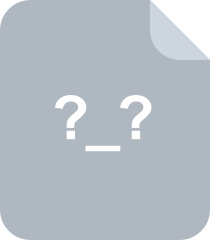
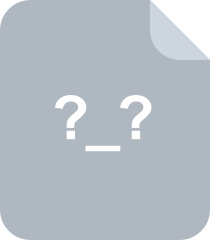
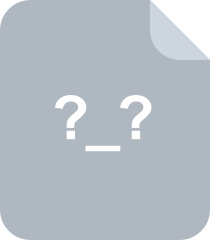
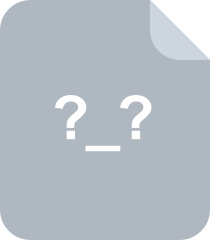
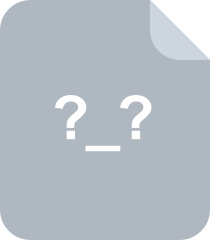
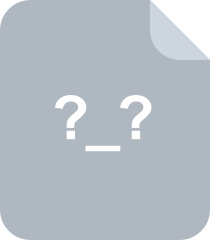
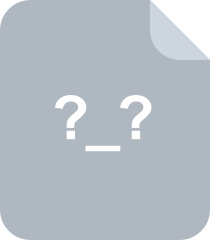
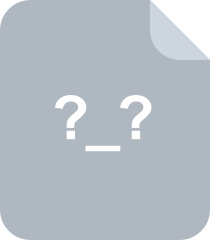
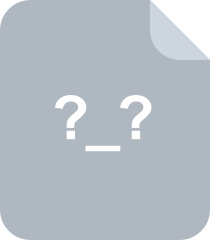
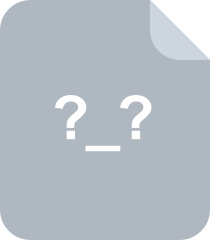
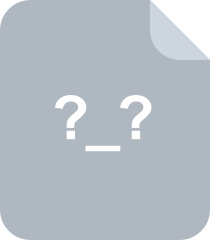
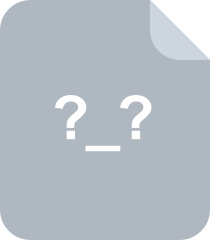
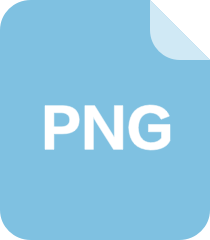
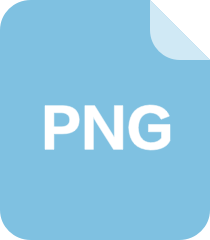
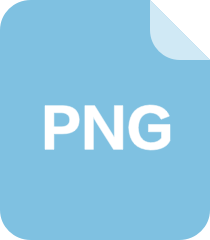
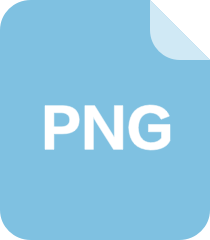
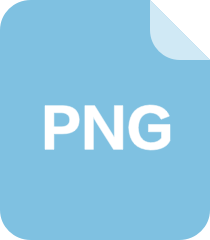
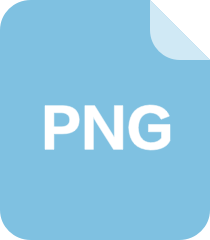
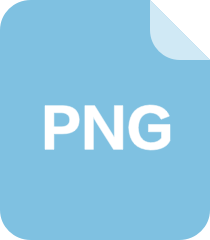
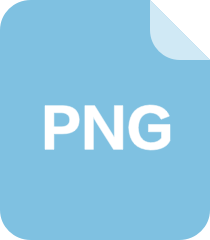
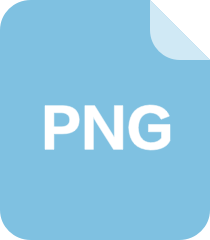
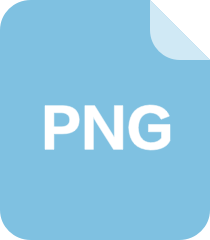
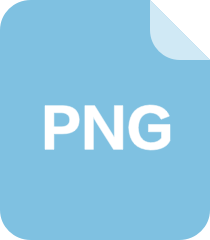
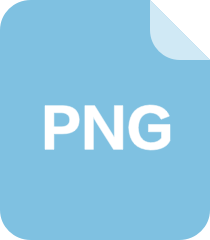
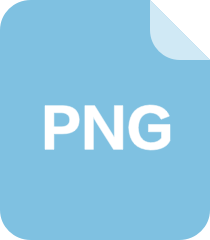
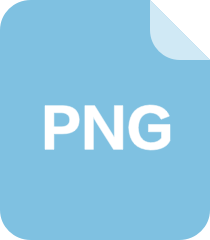
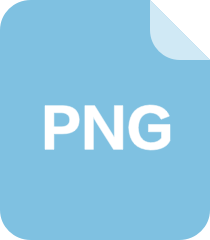
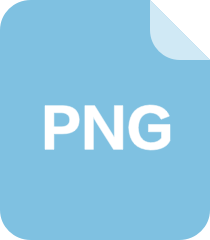
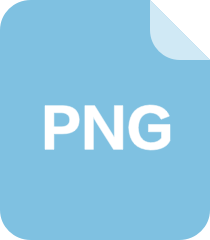
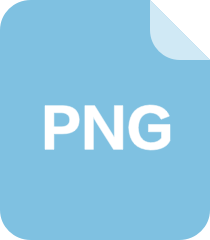
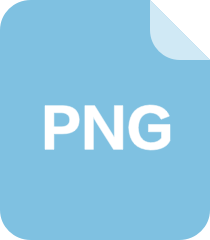
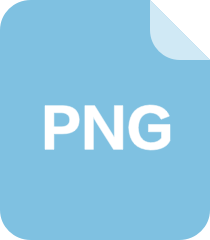
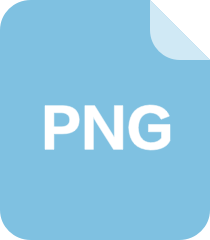
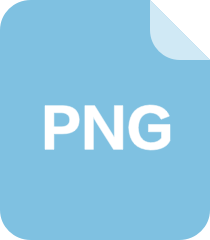
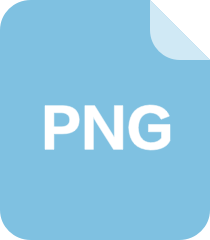
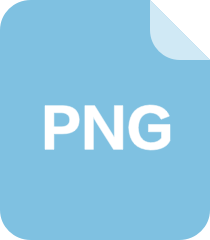
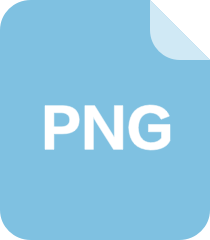
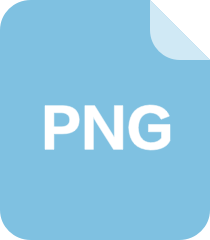
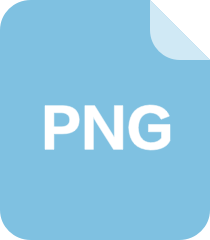
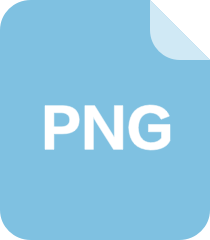
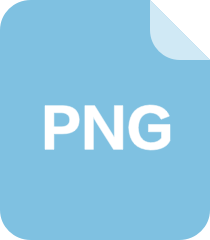
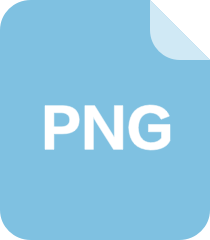
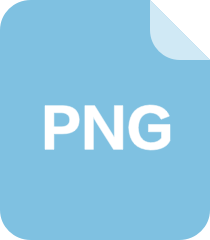
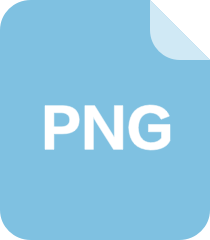
共 194 条
- 1
- 2
资源评论
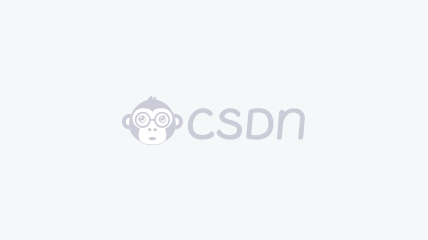

小徐博客
- 粉丝: 1973
- 资源: 2718
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

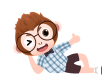
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


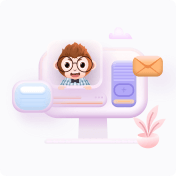
安全验证
文档复制为VIP权益,开通VIP直接复制
