jquery.serializeJSON
====================
Adds the method `.serializeJSON()` to [jQuery](http://jquery.com/) (or [Zepto](http://zeptojs.com/)) that serializes a form into a JavaScript Object, using the same format as the default Ruby on Rails request params.
Install
-------
Install with [bower](http://bower.io/) `bower install jquery.serializeJSON`, or [npm](https://www.npmjs.com/) `npm install jquery-serializejson`, or just download the [jquery.serializejson.js](https://raw.githubusercontent.com/marioizquierdo/jquery.serializeJSON/master/jquery.serializejson.js) script.
And make sure it is included after jQuery, for example:
```html
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="jquery.serializejson.js"></script>
```
Usage Example
-------------
HTML form:
```html
<form>
<input type="text" name="title" value="Finding Loot"/>
<input type="text" name="author[name]" value="John Smith"/>
<input type="text" name="author[job]" value="Legendary Pirate"/>
</form>
```
JavaScript:
```javascript
$('form').serializeJSON();
// returns =>
{
title: "Finding Loot",
author: {
name: "John Smith",
job: "Legendary Pirate"
}
}
```
Form input, textarea and select tags are supported. Nested attributes and arrays can be specified by using the `attr[nested][nested]` syntax.
HTML form:
```html
<form id="my-profile">
<!-- simple attribute -->
<input type="text" name="fullName" value="Mario Izquierdo" />
<!-- nested attributes -->
<input type="text" name="address[city]" value="San Francisco" />
<input type="text" name="address[state][name]" value="California" />
<input type="text" name="address[state][abbr]" value="CA" />
<!-- array -->
<input type="text" name="jobbies[]" value="code" />
<input type="text" name="jobbies[]" value="climbing" />
<!-- nested arrays, textareas, checkboxes ... -->
<textarea name="projects[0][name]">serializeJSON</textarea>
<textarea name="projects[0][language]">javascript</textarea>
<input type="hidden" name="projects[0][popular]" value="0" />
<input type="checkbox" name="projects[0][popular]" value="1" checked />
<textarea name="projects[1][name]">tinytest.js</textarea>
<textarea name="projects[1][language]">javascript</textarea>
<input type="hidden" name="projects[1][popular]" value="0" />
<input type="checkbox" name="projects[1][popular]" value="1"/>
<!-- select -->
<select name="selectOne">
<option value="paper">Paper</option>
<option value="rock" selected>Rock</option>
<option value="scissors">Scissors</option>
</select>
<!-- select multiple options, just name it as an array[] -->
<select multiple name="selectMultiple[]">
<option value="red" selected>Red</option>
<option value="blue" selected>Blue</option>
<option value="yellow">Yellow</option>
</select>
</form>
```
JavaScript:
```javascript
$('#my-profile').serializeJSON();
// returns =>
{
fullName: "Mario Izquierdo",
address: {
city: "San Francisco",
state: {
name: "California",
abbr: "CA"
}
},
jobbies: ["code", "climbing"],
projects: {
'0': { name: "serializeJSON", language: "javascript", popular: "1" },
'1': { name: "tinytest.js", language: "javascript", popular: "0" }
},
selectOne: "rock",
selectMultiple: ["red", "blue"]
}
```
The `serializeJSON` function returns a JavaScript object, not a JSON String. The plugin should probably have been called `serializeObject` or similar, but those plugins already existed.
To convert into a JSON String, use the `JSON.stringify` method, that is available on all major [new browsers](http://caniuse.com/json).
If you need to support very old browsers, just include the [json2.js](https://github.com/douglascrockford/JSON-js) polyfill (as described on [stackoverfow](http://stackoverflow.com/questions/191881/serializing-to-json-in-jquery)).
```javascript
var obj = $('form').serializeJSON();
var jsonString = JSON.stringify(obj);
```
The plugin implememtation relies on jQuery's [.serializeArray()](https://api.jquery.com/serializeArray/) method.
This means that it only serializes the inputs supported by `.serializeArray()`, which follows the standard W3C rules for [successful controls](http://www.w3.org/TR/html401/interact/forms.html#h-17.13.2). In particular, the included elements **cannot be disabled** and must contain a **name attribute**. No submit button value is serialized since the form was not submitted using a button. And data from file select elements is not serialized.
Parse values with :types
------------------------
All attribute values are **strings** by default. But you can force values to be parsed with specific types by appending the type with a colon.
```html
<form>
<input type="text" name="strbydefault" value=":string is the default (implicit) type"/>
<input type="text" name="text:string" value=":string type can still be used to overrid other parsing options"/>
<input type="text" name="excluded:skip" value="Use :skip to not include this field in the result"/>
<input type="text" name="numbers[1]:number" value="1"/>
<input type="text" name="numbers[1.1]:number" value="1.1"/>
<input type="text" name="numbers[other stuff]:number" value="other stuff"/>
<input type="text" name="bools[true]:boolean" value="true"/>
<input type="text" name="bools[false]:boolean" value="false"/>
<input type="text" name="bools[0]:boolean" value="0"/>
<input type="text" name="nulls[null]:null" value="null"/>
<input type="text" name="nulls[other stuff]:null" value="other stuff"/>
<input type="text" name="autos[string]:auto" value="text with stuff"/>
<input type="text" name="autos[0]:auto" value="0"/>
<input type="text" name="autos[1]:auto" value="1"/>
<input type="text" name="autos[true]:auto" value="true"/>
<input type="text" name="autos[false]:auto" value="false"/>
<input type="text" name="autos[null]:auto" value="null"/>
<input type="text" name="autos[list]:auto" value="[1, 2, 3]"/>
<input type="text" name="arrays[empty]:array" value="[]"/>
<input type="text" name="arrays[list]:array" value="[1, 2, 3]"/>
<input type="text" name="objects[empty]:object" value="{}"/>
<input type="text" name="objects[dict]:object" value='{"my": "stuff"}'/>
</form>
```
```javascript
$('form').serializeJSON();
// returns =>
{
"strbydefault": ":string is the default (implicit) type",
"text": ":string type can still be used to overrid other parsing options",
// excluded:skip is not included in the output
"numbers": {
"1": 1,
"1.1": 1.1,
"other stuff": NaN, // <-- Not a Number
},
"bools": {
"true": true,
"false": false,
"0": false, // <-- "false", "null", "undefined", "", "0" parse as false
},
"nulls": {
"null": null, // <-- "false", "null", "undefined", "", "0" parse as null
"other stuff": "other stuff"
},
"autos": { // <-- works like the parseAll option
"string": "text with stuff",
"0": 0, // <-- parsed as number
"1": 1, // <-- parsed as number
"true": true, // <-- parsed as boolean
"false": false, // <-- parsed as boolean
"null": null, // <-- parsed as null
"list": "[1, 2, 3]" // <-- array and object types are not auto-parsed
},
"arrays": { // <-- uses JSON.parse
"empty": [],
"not empty": [1,2,3]
},
"objects": { // <-- uses JSON.parse
"empty": {},
"not empty": {"my": "stuff"}
}
}
```
Types can also be specified with the attribute `data-value-type`, instead of having to add the ":type" suffix:
```html
<form>
<input type="text" name="anumb" data-value-type="number" value="1"/>
<input type="text" name="abool" data-value-type="
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
## 功能实现: 管理员操作包含以下功能:管理员登录,客户管理,添加客户,订单管理,添加快递单,处理快递单,审核财务单,货物入库,管理员管理,角色管理,修改权限,基础数据菜单管理等功能。 用了技术框架: HTML+CSS+JavaScript+jsp+mysql+mybatis+Spring 管理员账号/密码:admin/123456 ## 运行环境:jdk1.8/jdk1.9 ## IDE环境: Eclipse,Myeclipse,IDEA都可以 ## tomcat环境: Tomcat8.x/9.x
资源推荐
资源详情
资源评论
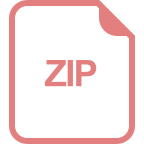
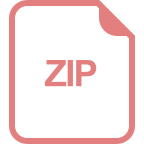
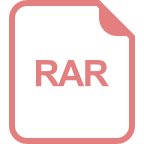
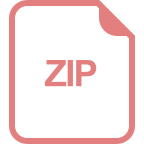
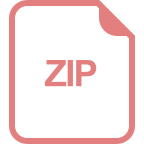
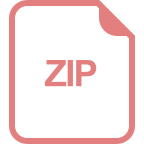
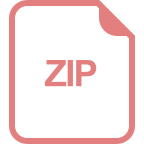
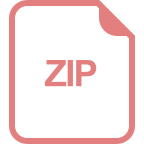
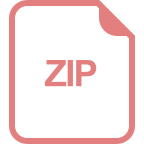
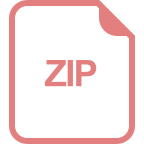
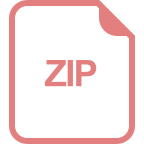
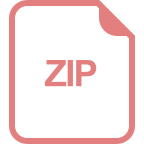
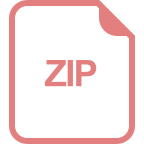
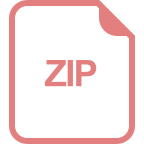
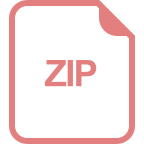
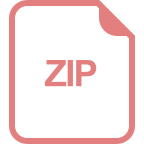
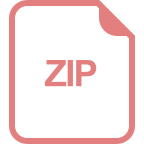
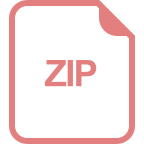
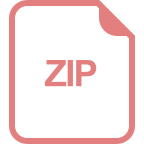
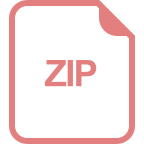
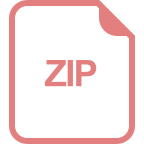
收起资源包目录

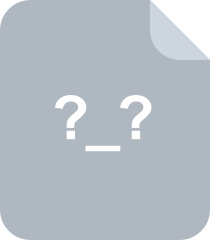
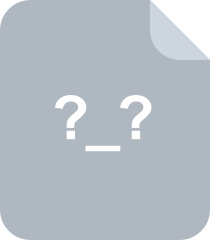
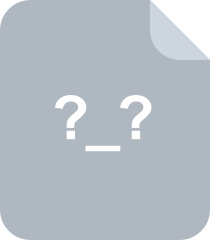
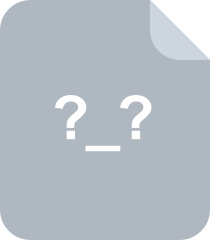
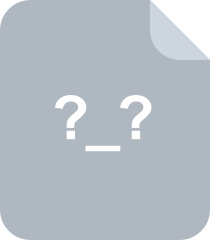
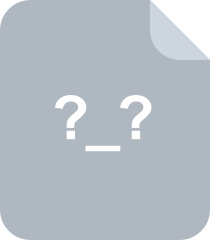
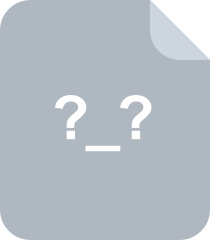
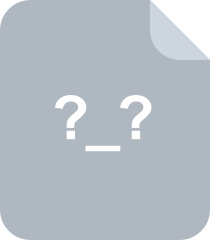
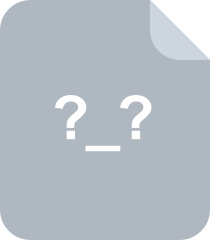
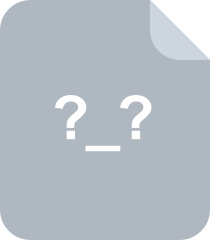
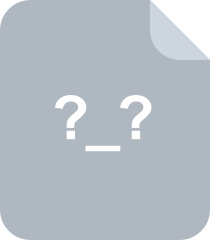
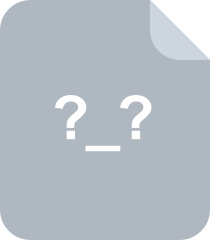
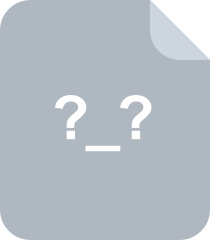
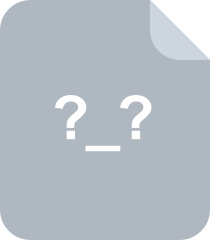
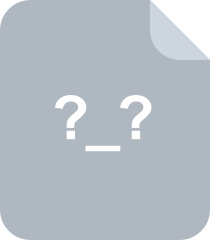
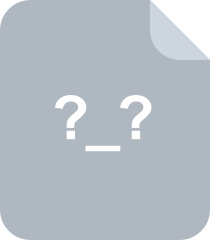
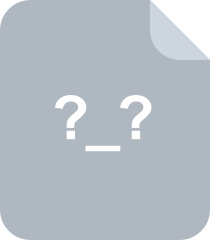
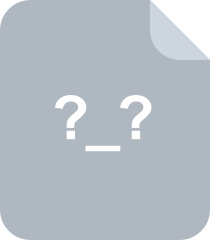
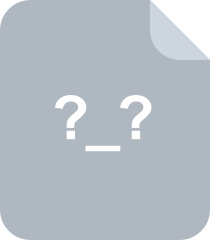
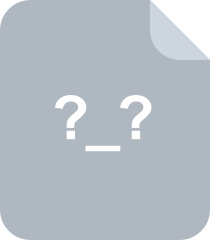
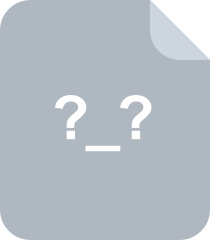
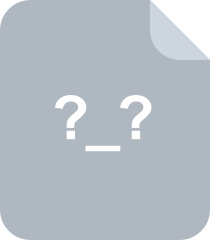
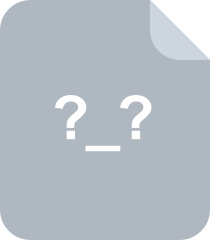
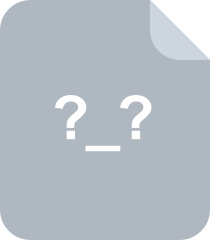
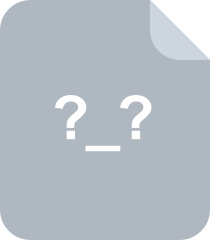
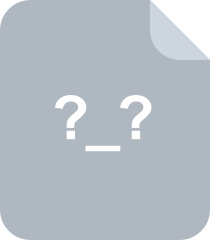
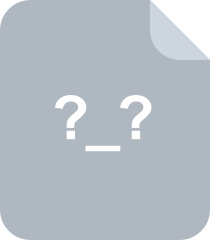
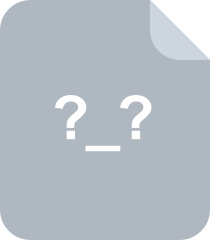
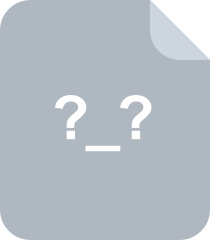
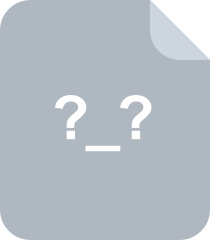
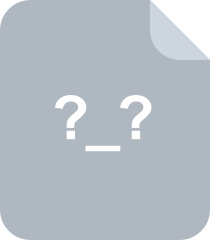
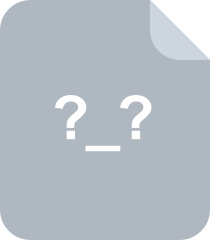
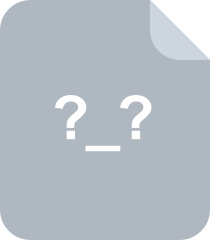
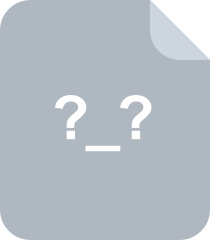
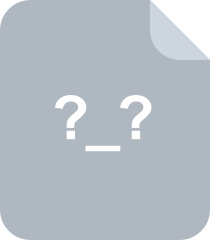
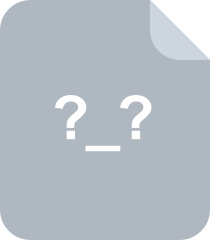
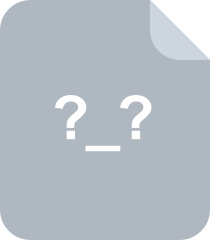
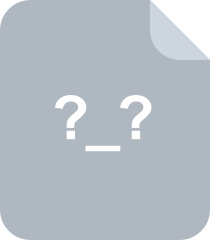
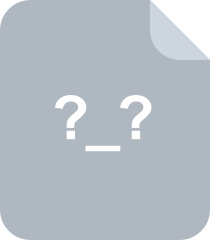
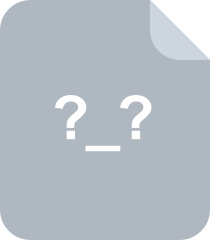
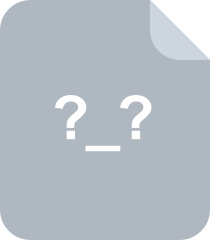
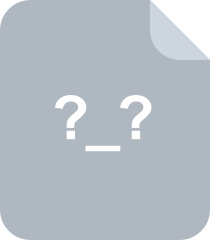
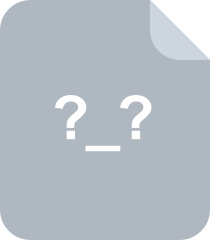
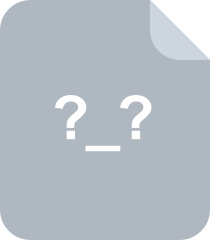
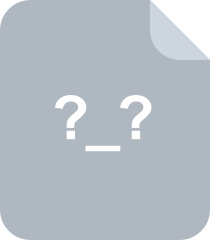
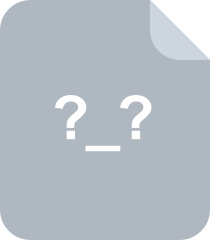
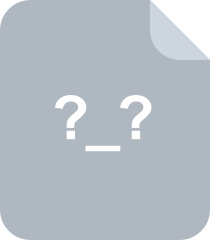
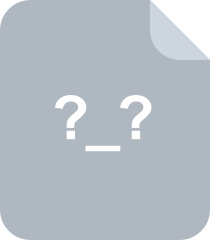
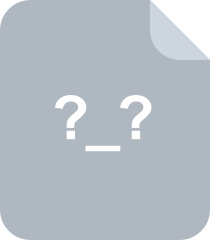
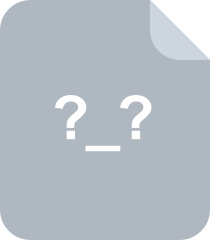
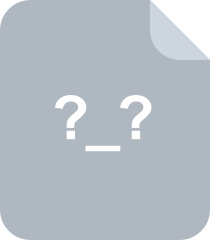
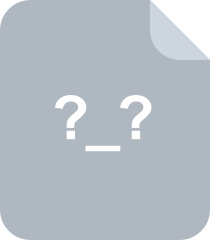
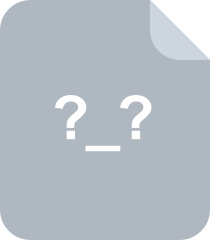
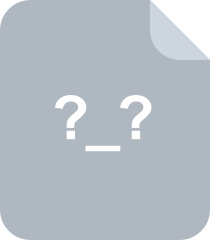
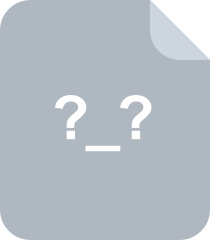
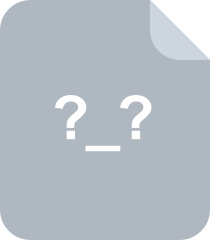
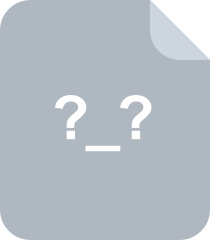
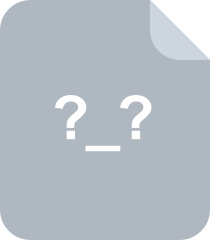
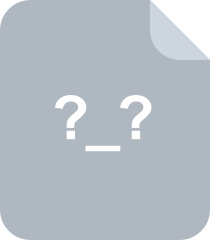
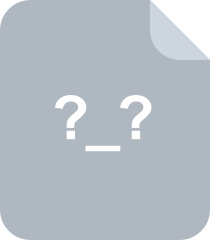
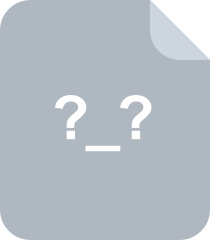
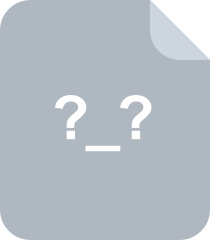
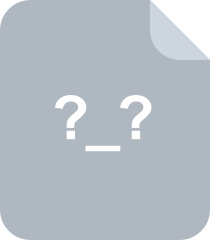
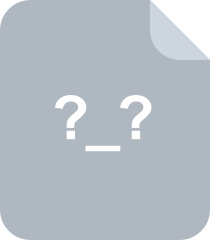
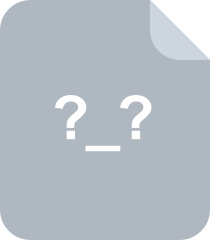
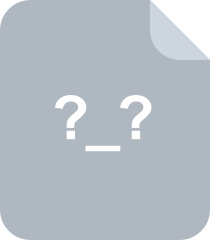
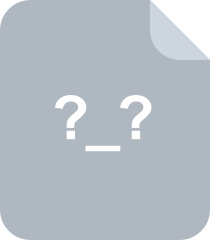
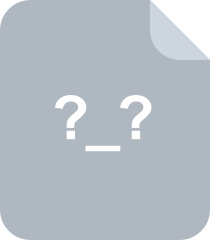
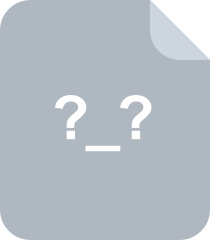
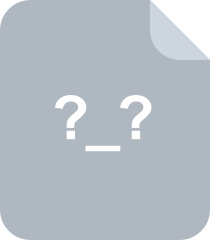
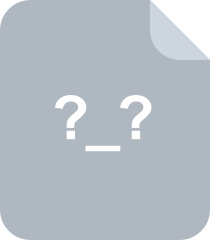
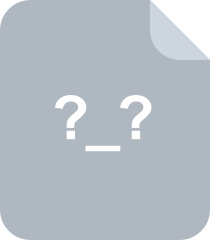
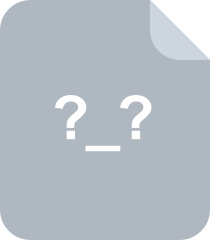
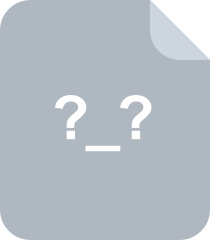
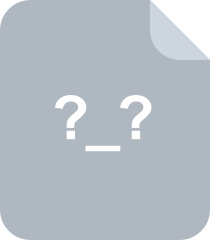
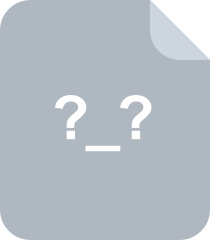
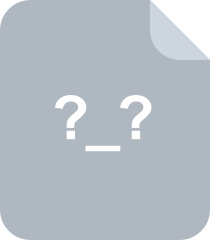
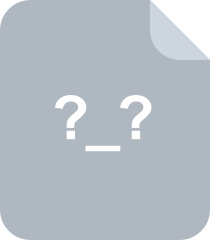
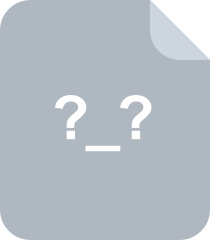
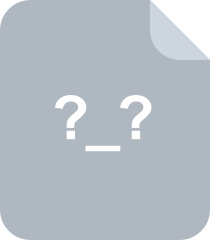
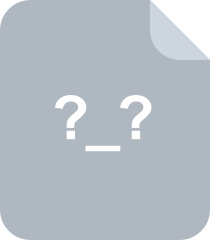
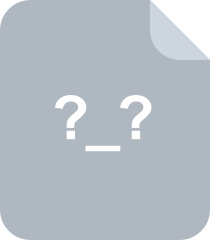
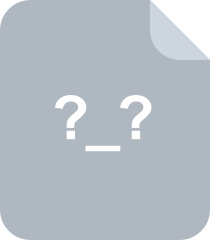
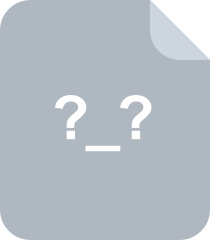
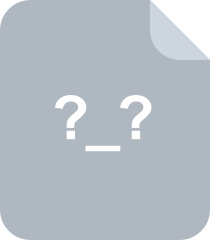
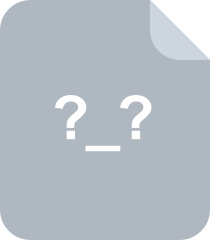
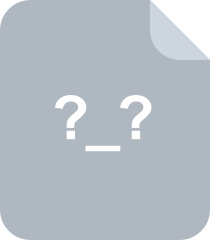
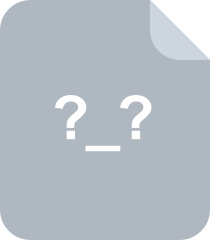
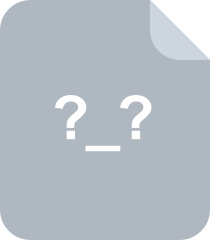
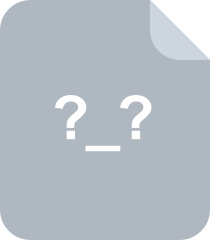
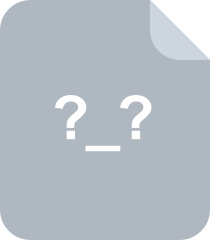
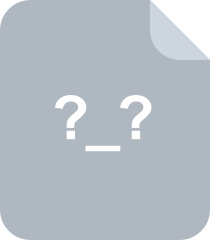
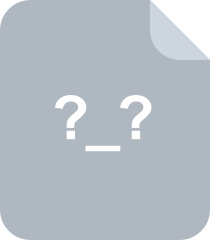
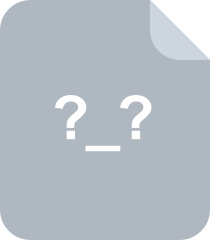
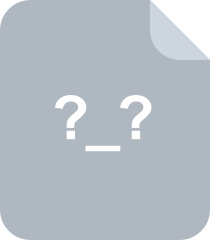
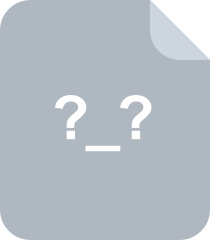
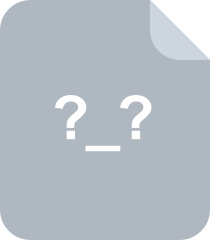
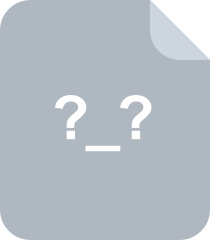
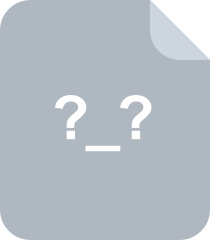
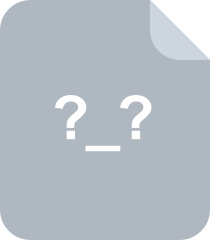
共 1607 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
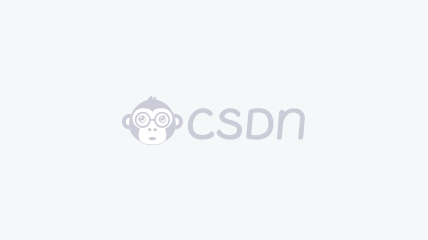

程序源码工
- 粉丝: 47
- 资源: 469
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

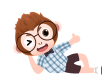
最新资源
- 基于语音识别的智能垃圾分类系统源代码(完整前后端+mysql+说明文档+LW).zip
- 基于网易新闻+评论的舆情热点分析平台源代码(完整前后端+mysql+说明文档+LW).zip
- MATLAB实现BiLSTM(双向长短期记忆神经网络)数据异常检测(含完整的程序,GUI设计和代码详解)
- 653152225001783外卖管理系统.apk
- CodeBlocks_播放音乐.pdf
- 差分放大电路在电流采样中的应用
- 定制-红米7国际版解锁固件fast线刷
- STM32基础入门开发:设计按键点灯程序.pdf
- 基于B站用户行为分析系统源代码(完整前后端+mysql+说明文档+LW).zip
- STM32基础入门开发:串口数据发送与接收.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


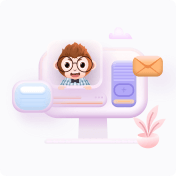
安全验证
文档复制为VIP权益,开通VIP直接复制
