package cc.gzvtc.photographer.controller;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import cc.gzvtc.common.util.OperationFileUtil;
import cc.gzvtc.model.TPhotographer;
import cc.gzvtc.model.TPhotographerLabel;
import cc.gzvtc.model.TPhotographerLevel;
import cc.gzvtc.model.TPhotographerSpots;
import cc.gzvtc.photographer.service.IPhotographerLabelService;
import cc.gzvtc.photographer.service.IPhotographerLevelService;
import cc.gzvtc.photographer.service.IPhotographerService;
import cc.gzvtc.photographer.service.IPhotographerSpotsService;
import cc.gzvtc.vo.PageVO;
import cc.gzvtc.vo.ReturnCodeType;
import cc.gzvtc.vo.ReturnResult;
/**
*
* @author me 2019年9月12日
*
*/
@Controller
@Scope("prototype")
public class PhotographerController {
private static final Logger logger = LoggerFactory.getLogger(PhotographerController.class);
private ReturnResult returnResult = new ReturnResult();
@Resource(name = "photographerService")
private IPhotographerService photographerService;
@Resource(name = "photographerLabelService")
IPhotographerLabelService photographerLabelService;
@Resource(name = "photographerLevelService")
IPhotographerLevelService photographerLevelService;
@Resource(name = "photographerSpotsService")
IPhotographerSpotsService photographerSpotsService;
/**
* 添加摄影师
*
* @param photographer
* @param HttpServletRequest
* @return
*/
@RequestMapping(value = "addPhotographer", method = RequestMethod.POST)
@ResponseBody
public ReturnResult addPhotographer(TPhotographer photographer, HttpServletRequest request, Integer labelId,
Integer levelId, Integer spotsId) {
returnResult.setStatus(ReturnCodeType.FAILURE);
try {
Map<String, String> map = OperationFileUtil.multiFileUpload(request,
request.getServletContext().getRealPath("/") + "uploads/photographer/");
String filePath = "";
for (Map.Entry<String, String> entry : map.entrySet()) {
filePath = entry.getValue();
}
filePath = filePath.replace(request.getServletContext().getRealPath("/"), "/");
photographer.setHead(filePath);
photographer.setCreatetime(new Date());
photographerService.insert(photographer);
int id = photographer.getId();
TPhotographerLabel label = new TPhotographerLabel(labelId, id, new Date(), "0");
photographerLabelService.insert(label);
TPhotographerLevel level = new TPhotographerLevel(levelId, id, new Date(), "0");
photographerLevelService.insert(level);
TPhotographerSpots spots = new TPhotographerSpots(spotsId, id, new Date(), "0");
photographerSpotsService.insert(spots);
returnResult.setStatus(ReturnCodeType.SUCCESS);
} catch (Exception e) {
logger.error("新增photographer失败" + e);
}
return returnResult;
}
/**
* 修改photographer状态
*
* @param photographer
* @return
*/
@RequestMapping(value = "updatePhotographerStatus", method = RequestMethod.GET)
@ResponseBody
public ReturnResult updatePhotographerStatus(TPhotographer photographer) {
returnResult.setStatus(ReturnCodeType.FAILURE);
try {
photographerService.updateBySQL("UPDATE t_photographer SET status=" + photographer.getStatus()
+ " WHERE id=" + photographer.getId());
returnResult.setStatus(ReturnCodeType.SUCCESS);
} catch (Exception e) {
logger.error("更新photographer状态失败" + e);
}
return returnResult;
}
/**
* 修改photographer的name和summary
*
* @param photographer
* @return
*/
@RequestMapping(value = "updatePhotographer", method = RequestMethod.POST)
@ResponseBody
public ReturnResult updatePhotographer(TPhotographer photographer, Integer labelId, Integer levelId,
Integer spotsId) {
returnResult.setStatus(ReturnCodeType.FAILURE);
try {
photographerService.updateBySQL("UPDATE t_photographer SET name='" + photographer.getName() + "',summary='"
+ photographer.getSummary() + "',status=" + photographer.getStatus() + " WHERE id="
+ photographer.getId());
photographerLabelService.updateBySQL("UPDATE t_photographer_label SET labelId=" + labelId
+ " WHERE photographerId=" + photographer.getId());
photographerLevelService.updateBySQL("UPDATE t_photographer_level SET levelId=" + levelId
+ " WHERE photographer=" + photographer.getId());
photographerSpotsService.updateBySQL("UPDATE t_photographer_spots SET spotsId=" + spotsId
+ " WHERE photographerId=" + photographer.getId());
returnResult.setStatus(ReturnCodeType.SUCCESS);
} catch (Exception e) {
logger.error("修改photographer失败" + e);
e.printStackTrace();
}
return returnResult;
}
/**
* 根据id获取Photographer
* admin
* @param Photographer
* @return
*/
@RequestMapping(value = "getPhotographerById", method = RequestMethod.POST)
@ResponseBody
public ReturnResult getPhotographerById(Integer id) {
returnResult.setStatus(ReturnCodeType.FAILURE);
try {
returnResult.setStatus(ReturnCodeType.SUCCESS)
.setData(photographerService.selectBySQL(
"SELECT a.*,b.labelId,c.levelId,d.spotsId FROM t_photographer a,t_photographer_label b,t_photographer_level c,t_photographer_spots d WHERE a.id ="
+ id + " AND b.photographerId=" + id + " AND c.photographer=" + id
+ " AND d.photographerId=" + id + "")
.get(0));
} catch (Exception e) {
logger.error("根据id获取Photographer失败" + e);
}
return returnResult;
}
/**
* 根据id获取Photographer
* user
* @param Photographer
* @return
*/
@RequestMapping(value = "getPhotographer")
@ResponseBody
public ReturnResult getPhotographer(Integer id) {
returnResult.setStatus(ReturnCodeType.FAILURE);
try {
returnResult.setStatus(ReturnCodeType.SUCCESS)
.setData(photographerService.selectBySQL(
"SELECT a.name,a.head,a.summary,d.`name` AS level,e.`name` AS spots FROM t_photographer a,t_photographer_level b,t_photographer_spots c,t_level d,t_spots e WHERE a.id="+id+" AND b.photographer="+id+" AND c.photographerId="+id+" AND d.id=b.levelId AND e.id = c.spotsId AND a.`status`=0")
.get(0));
} catch (Exception e) {
logger.error("根据id获取Photographer失败" + e);
}
return returnResult;
}
/**
* 分页获取photographer
*
* @return
*/
@RequestMapping(value = "getPhotographerListByPage", method = RequestMethod.POST)
@ResponseBody
public ReturnResult getPhotographerListByPage(PageVO page, String labelId, String levelId, String spotsId,
String beforeTime, String afterTime, String status, String name) {
returnResult.setStatus(ReturnCodeType.FAILURE);
try {
Map<String, Object> resultMap = new HashMap<String, Object>();
StringBuffer sql = new StringBuffer(
"SELECT a.*,f.`name` AS label,g.`name` AS level,h.`name` AS spots FROM t_photographer a,t_photographer_label b,t_photographer_level c ,t_photographer_spots d,t_label f,t_level g,t_spots h WHERE a.id=b.photographerId AND a.id = c.photographer AND a.id = d.photographerId AND f.id=b.labelId AND g.id=c.levelId AND h.id= d.spotsId");
if (StringUtils.isNotBlank(labelId)) {
sql.append(" AND b.labelId=" + labelId);
sql.append(" AND f.id=" + labelId);
}
if (StringUtils.isNotBlank(levelId)) {
sql.append(" AND c.levelId=" + levelId);
sql.append(" AND g.id=" + levelId);
}
if (StringUtils.isNotBlank(spotsId)) {
sql.append(" AND d.spotsId=" + spotsId);
sql.append(" AND h.id=" + spotsId);
}
if (StringUtils.isNotBlank(status)) {
sql.append(" AND a.status=" + status);
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
## 功能说明: 管理员角色包含以下功能:管理员登录,订单管理,摄影师管理,级别管理,标签管理,摄影地点管理,客片管理,轮播图管理,资讯管理等功能。 客户角色包含以下功能:客户首页,客片欣赏,预约摄影师,会员登录,填写预约摄影师信息,查看活动,订单查看等功能。 用了技术框架: HTML+CSS+JavaScript+jsp+mysql+Spring+mybatis+Spring boot 管理员账号/密码:admin/admin 用户账号/密码: xiaozhang/123456 ## 运行环境:jdk1.8/jdk1.9 ## IDE环境: Eclipse,Myeclipse,IDEA都可以 ## tomcat环境: Tomcat8.x/9.x
资源推荐
资源详情
资源评论
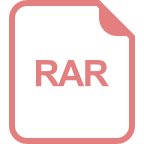
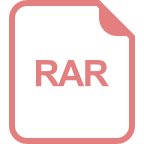
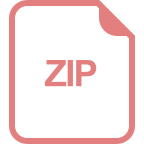
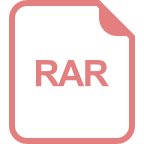
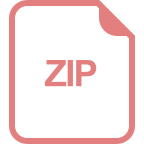
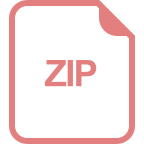
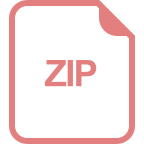
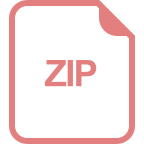
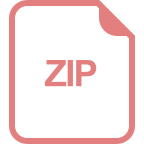
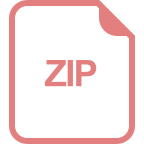
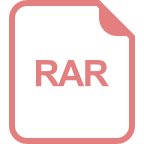
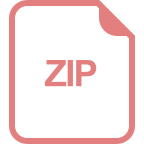
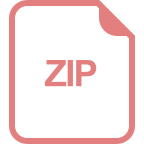
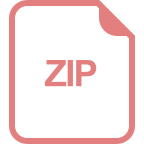
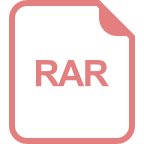
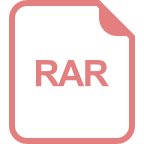
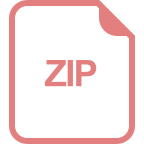
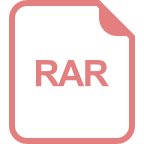
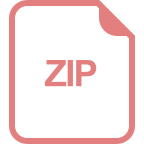
收起资源包目录

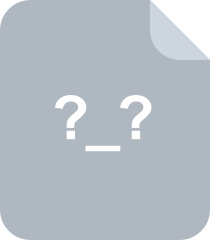
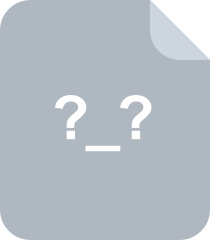
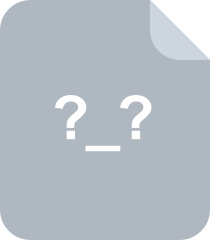
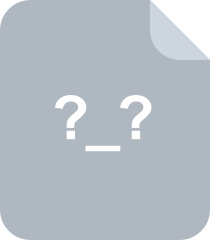
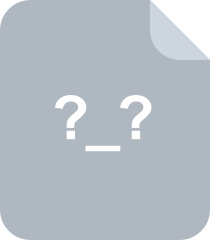
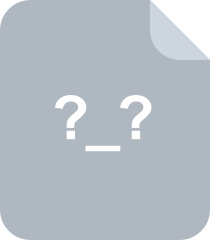
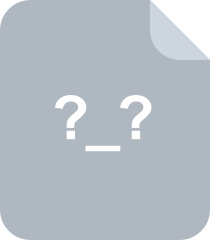
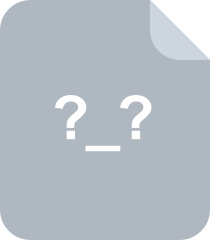
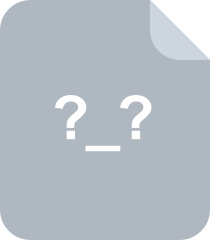
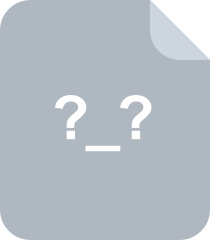
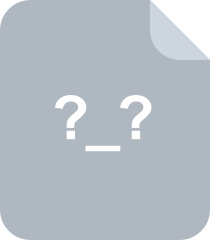
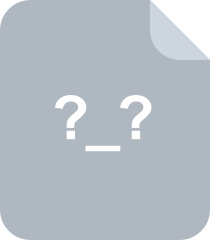
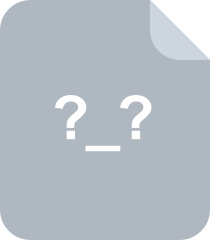
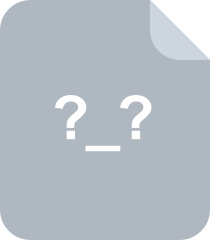
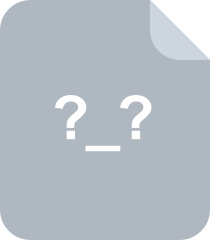
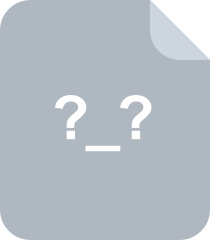
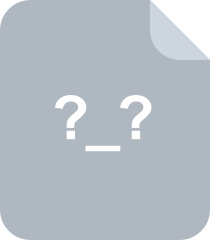
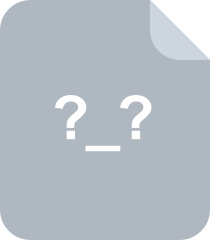
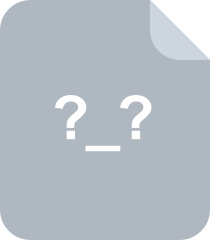
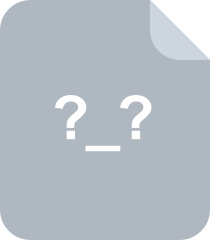
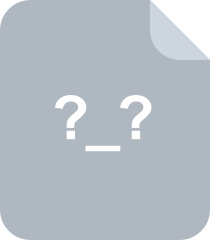
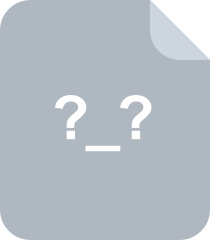
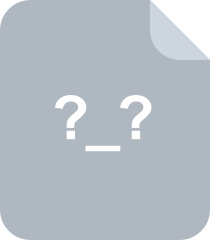
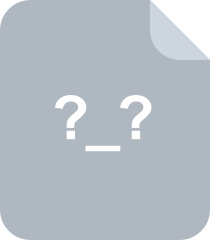
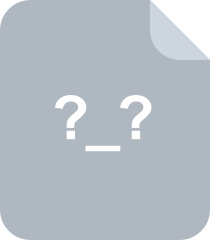
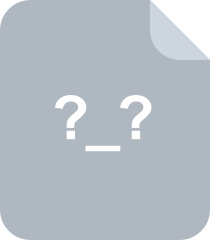
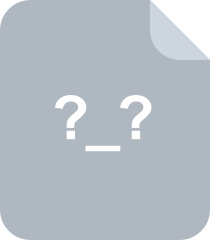
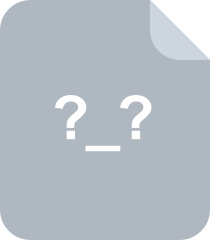
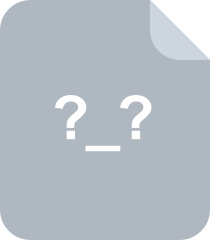
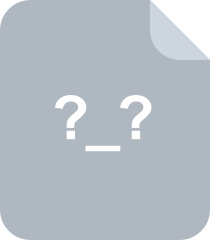
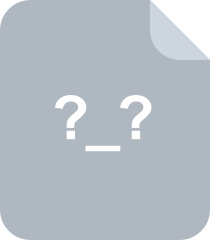
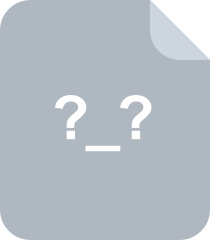
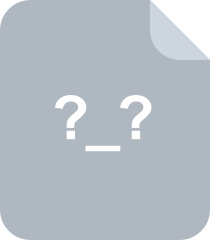
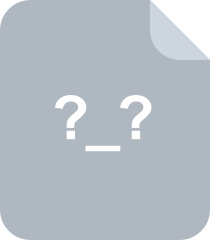
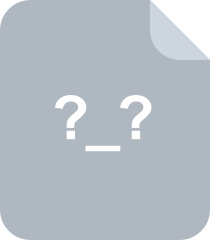
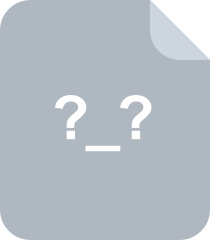
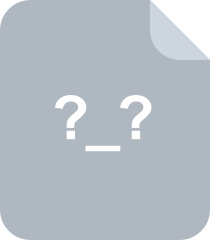
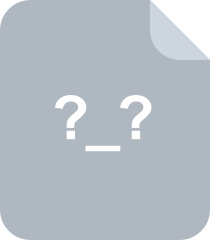
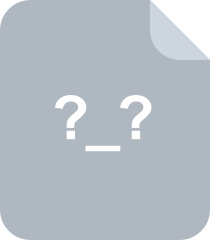
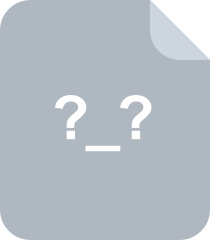
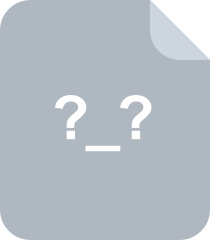
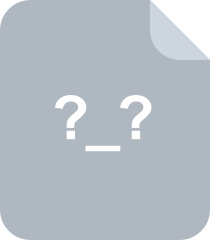
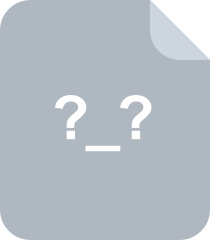
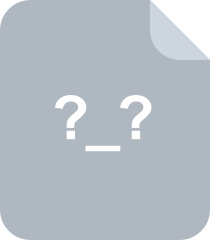
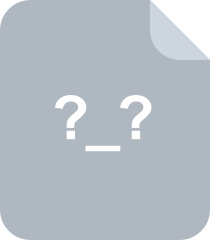
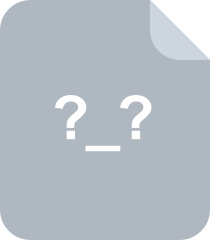
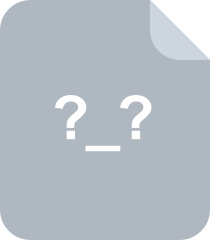
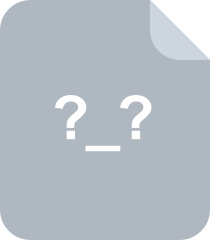
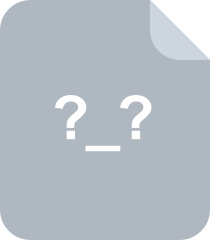
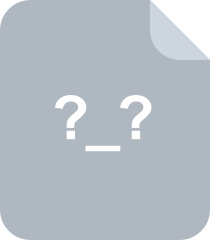
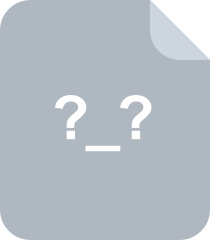
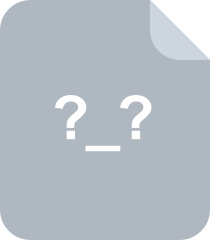
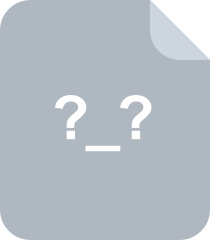
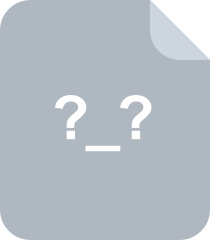
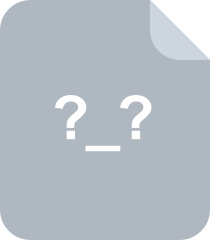
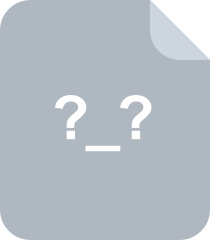
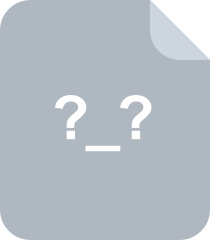
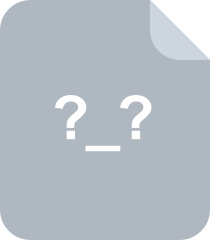
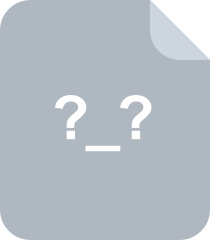
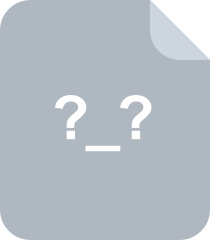
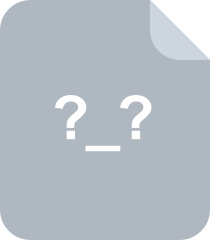
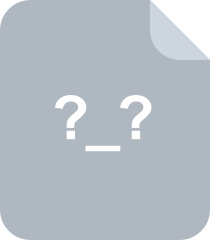
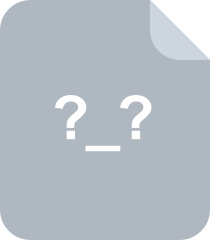
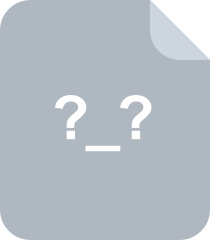
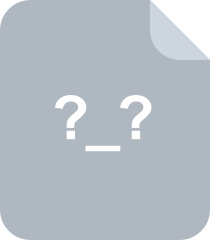
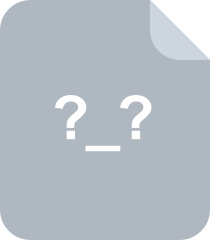
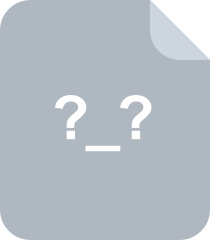
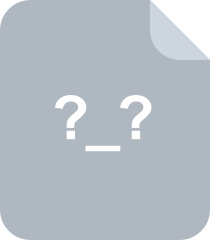
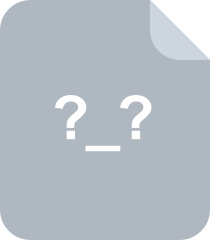
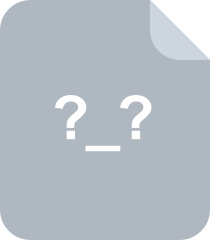
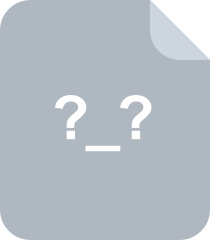
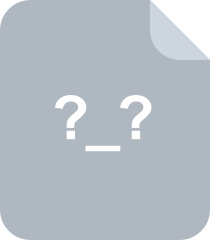
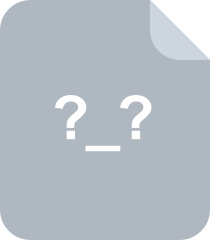
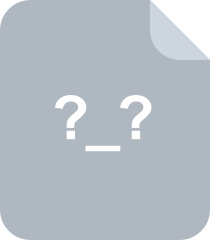
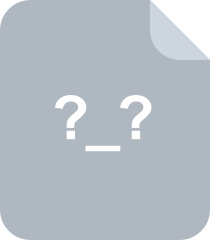
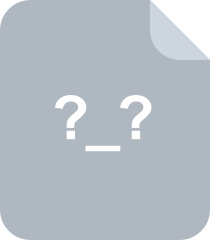
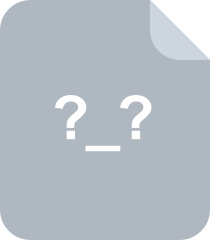
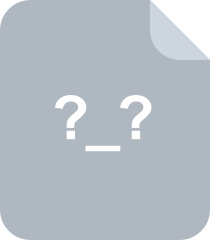
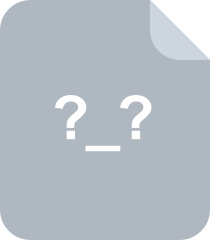
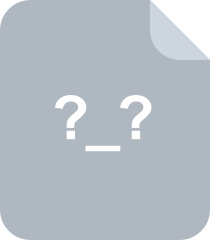
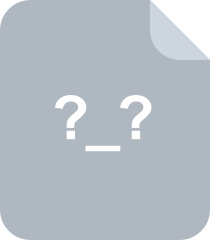
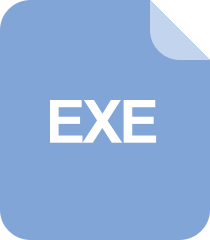
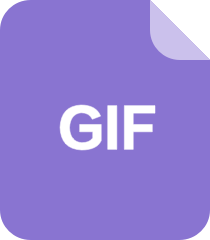
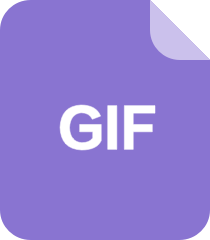
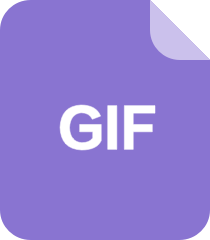
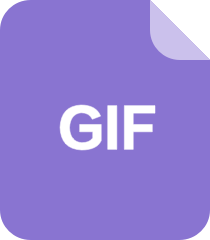
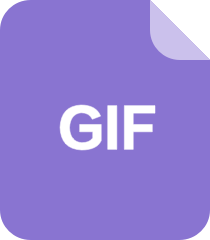
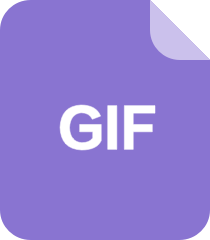
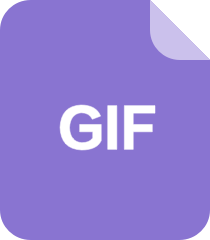
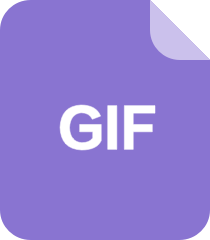
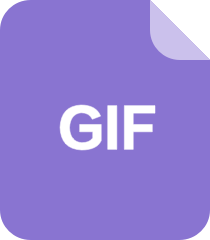
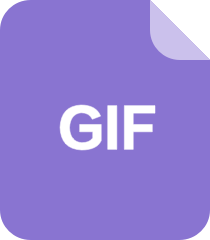
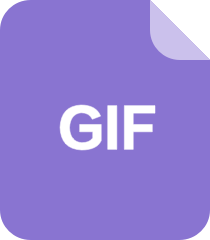
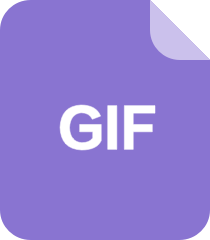
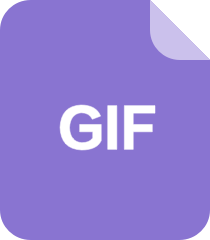
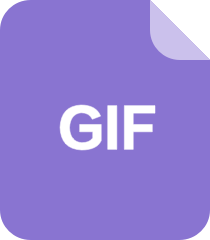
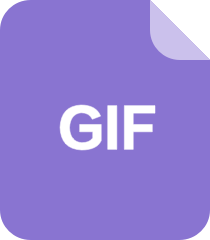
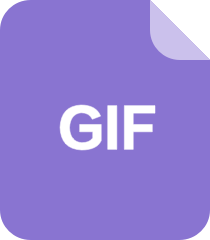
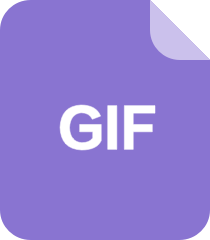
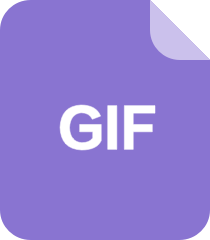
共 1142 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
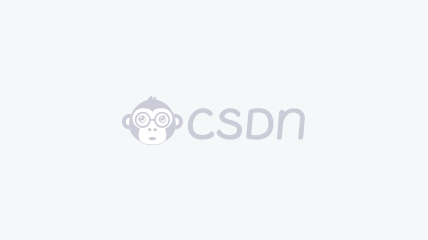

程序源码工
- 粉丝: 47
- 资源: 469
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

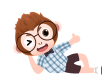
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


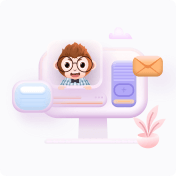
安全验证
文档复制为VIP权益,开通VIP直接复制
