package com.hb.cad;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.RenderingHints;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.event.MouseMotionAdapter;
import java.awt.image.BufferedImage;
import java.io.File;
import java.util.ArrayList;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPopupMenu;
import javax.swing.WindowConstants;
import javax.swing.filechooser.FileFilter;
import com.hb.cad.baseShapeInterface.BaseShapeInterface;
import com.hb.cad.canvas.CanvasJPanel;
/**
* This code was edited or generated using CloudGarden's Jigloo
* SWT/Swing GUI Builder, which is free for non-commercial
* use. If Jigloo is being used commercially (ie, by a corporation,
* company or business for any purpose whatever) then you
* should purchase a license for each developer using Jigloo.
* Please visit www.cloudgarden.com for details.
* Use of Jigloo implies acceptance of these licensing terms.
* A COMMERCIAL LICENSE HAS NOT BEEN PURCHASED FOR
* THIS MACHINE, SO JIGLOO OR THIS CODE CANNOT BE USED
* LEGALLY FOR ANY CORPORATE OR COMMERCIAL PURPOSE.
*/
public class CADJFrame extends javax.swing.JFrame implements MouseListener{
private JMenuBar jMenuBar;
private JMenuItem newMenuItem;
private JLabel ellipseLabel;
private JLabel greenLabel;
private JLabel redLabel;
private JLabel yellowLabel;
private JLabel turnLabel;
private JLabel selectLabel;
private JLabel undoLabel;
private JLabel doLabel;
private JLabel pentacleLabel;
private JPopupMenu jPopupMenu1;
private JButton moreColorButton;
private JLabel orangeLabel;
private JLabel lightgrayLabel;
private JLabel magentaLabel;
private JLabel darkgrayLabel;
private JLabel grayLabel;
private JLabel cyanLabel;
private JLabel purpleLabel;
private JLabel blueLabel;
private JLabel blackLabel;
private JLabel colorLabel;
private JLabel pentagonLabel;
private JLabel triangleLabel;
private JLabel roundLabel;
private JLabel rectLabel;
private JLabel lineLabel;
private JPanel toolsPanel;
private CanvasJPanel drawPanel;
private JLabel positionLabel;
private JMenuItem editcolorMenuItem;
private JMenuItem openMenuItem;
private JMenuItem deleteMenuItem;
private JMenuItem undoMenuItem;
private JMenu pictureMenu;
private JMenuItem exitMenuItem;
private JMenuItem saveMenuItem;
private JMenu fileMenu;
private boolean isSame = false; // 校验上次点击和当前鼠标进入是否同一个JLabel
private boolean isClick = false; // 校验是否点击了JLabel
private int flag = -1; // 标记点击的对象是否相同,0代表两次点击对象相同,1代表两次点击对象不同
private String conName = null; // 记录上一次点击对象的名字
/**
* Auto-generated main method to display this JFrame
*/
public static void main(String[] args) {
CADJFrame inst = new CADJFrame();
inst.setLocationRelativeTo(null);
inst.setVisible(true);
}
public CADJFrame() {
super("画图");
initGUI();
}
private void initGUI() {
try {
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
{
{
positionLabel = new JLabel();
getContentPane().add(positionLabel, BorderLayout.SOUTH);
positionLabel.setText(" ");
positionLabel.setPreferredSize(new java.awt.Dimension(392, 26));
}
{
drawPanel = new CanvasJPanel();
getContentPane().add(drawPanel, BorderLayout.CENTER);
drawPanel.setBackground(Color.white);
drawPanel.setLayout(null);
drawPanel.addMouseMotionListener(new MouseMotionAdapter() {
public void mouseDragged(MouseEvent evt) {
drawPanelMouseMoved(evt);
}
public void mouseMoved(MouseEvent evt) {
drawPanelMouseMoved(evt);
}
});
drawPanel.setManage(CanvasJPanel.DRAW_LINE);
}
{
toolsPanel = new JPanel();
getContentPane().add(toolsPanel, BorderLayout.WEST);
toolsPanel.setPreferredSize(new java.awt.Dimension(126, 518));
toolsPanel.setLayout(null);
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\line.jpg");
image = new javax.swing.ImageIcon(getScaledImage(image.getImage(), 25, 25));
lineLabel = new JLabel(image);
toolsPanel.add(lineLabel);
lineLabel.setBounds(17, 14, 29, 29);
lineLabel.setBackground(new Color(171,215,250));
lineLabel.setOpaque(true);
lineLabel.setName("line");
conName = lineLabel.getName().toString(); // 初始化为直线
lineLabel.addMouseListener(this);
{
jPopupMenu1 = new JPopupMenu();
jPopupMenu1.add("直线");
lineLabel.setComponentPopupMenu(jPopupMenu1);
}
}
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\rect.jpg");
image = new javax.swing.ImageIcon(getScaledImage(image.getImage(), 25, 25));
rectLabel = new JLabel(image);
toolsPanel.add(rectLabel);
rectLabel.setBounds(46, 14, 29, 29);
rectLabel.setOpaque(true);
rectLabel.setName("rect");
rectLabel.addMouseListener(this);
{
jPopupMenu1 = new JPopupMenu();
jPopupMenu1.add("矩形");
rectLabel.setComponentPopupMenu(jPopupMenu1);
}
}
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\round.jpg");
image = new javax.swing.ImageIcon(getScaledImage(image.getImage(), 25, 25));
roundLabel = new JLabel(image);
toolsPanel.add(roundLabel);
roundLabel.setBounds(75, 14, 29, 29);
roundLabel.setOpaque(true);
roundLabel.setName("round");
roundLabel.addMouseListener(this);
{
jPopupMenu1 = new JPopupMenu();
jPopupMenu1.add("圆");
roundLabel.setComponentPopupMenu(jPopupMenu1);
}
}
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\ellipse.jpg");
image = new javax.swing.ImageIcon(getScaledImage(image.getImage(), 25, 25));
ellipseLabel = new JLabel(image);
toolsPanel.add(ellipseLabel);
ellipseLabel.setBounds(17, 43, 29, 29);
ellipseLabel.setOpaque(true);
ellipseLabel.setName("ellipse");
ellipseLabel.addMouseListener(this);
{
jPopupMenu1 = new JPopupMenu();
jPopupMenu1.add("椭圆");
ellipseLabel.setComponentPopupMenu(jPopupMenu1);
}
}
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\triangle.jpg");
image = new javax.swing.ImageIcon(getScaledImage(image.getImage(), 25, 25));
triangleLabel = new JLabel(image);
toolsPanel.add(triangleLabel);
triangleLabel.setBounds(46, 43, 29, 29);
triangleLabel.setOpaque(true);
triangleLabel.setName("triangle");
triangleLabel.addMouseListener(this);
{
jPopupMenu1 = new JPopupMenu();
jPopupMenu1.add("正三角形");
triangleLabel.setComponentPopupMenu(jPopupMenu1);
}
}
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\pentagon.jpg");
image = new javax.swing.ImageIcon(getScaledImage(image.getImage(), 25, 25));
pentagonLabel = new JLabel(image);
toolsPanel.add(pentagonLabel);
pentagonLabel.setBounds(75, 43, 29, 29);
pentagonLabel.setOpaque(true);
pentagonLabel.setName("pentagon");
pentagonLabel.addMouseListener(this);
{
jPopupMenu1 = new JPopupMenu();
jPopupMenu1.add("正五边形");
pentagonLabel.setComponentPopupMenu(jPopupMenu1);
}
}
{
javax.swing.ImageIcon image = new javax.swing.ImageIcon("icon\\pentacle.jpg");
image = n

NE_cogo
- 粉丝: 1
- 资源: 1
最新资源
- TouchGFX 实现图片资源存储在外部flash中(2)
- nginx-1.28.1 arm64架构 docker 镜像包
- fir滤波器实现,包含vivado视频和代码
- 用Python编写一个学生成绩管理系统
- Typora 适配MacBook M系列
- 非凸优化算法的测试函数Goldstein-Price函数(Goldstein-Price function)的Python代码,实现3D效果
- Android Studio4.0版本 适配MacBook M系列芯片
- mysql8.4.3 arm64架构 docker 镜像包
- DH-Live部署与训练的相关代码
- 非凸优化算法的测试函数Eggholder函数(Eggholder function)的Python代码,实现3D效果
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


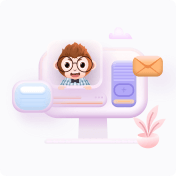