#include "StdAfx.h"
#include "TestCefAPP.h"
#include "include/cef_browser.h"
#include "include/cef_command_line.h"
#include "include/views/cef_browser_view.h"
#include "include/views/cef_window.h"
#include "include/wrapper/cef_helpers.h"
#include "TestCefHandler.h"
TestCefAPP::TestCefAPP(void)
{
}
TestCefAPP::~TestCefAPP(void)
{
}
namespace {
// When using the Views framework this object provides the delegate
// implementation for the CefWindow that hosts the Views-based browser.
class SimpleWindowDelegate : public CefWindowDelegate {
public:
explicit SimpleWindowDelegate(CefRefPtr<CefBrowserView> browser_view)
: browser_view_(browser_view) {
}
void OnWindowCreated(CefRefPtr<CefWindow> window) OVERRIDE {
// Add the browser view and show the window.
window->AddChildView(browser_view_);
window->Show();
// Give keyboard focus to the browser view.
browser_view_->RequestFocus();
}
void OnWindowDestroyed(CefRefPtr<CefWindow> window) OVERRIDE {
browser_view_ = NULL;
}
bool CanClose(CefRefPtr<CefWindow> window) OVERRIDE {
// Allow the window to close if the browser says it's OK.
CefRefPtr<CefBrowser> browser = browser_view_->GetBrowser();
if (browser)
return browser->GetHost()->TryCloseBrowser();
return true;
}
private:
CefRefPtr<CefBrowserView> browser_view_;
IMPLEMENT_REFCOUNTING(SimpleWindowDelegate);
DISALLOW_COPY_AND_ASSIGN(SimpleWindowDelegate);
};
} // namespace
void TestCefAPP::OnContextInitialized() {
CEF_REQUIRE_UI_THREAD();
CefRefPtr<CefCommandLine> command_line =
CefCommandLine::GetGlobalCommandLine();
#if defined(OS_WIN) || defined(OS_LINUX)
// Create the browser using the Views framework if "--use-views" is specified
// via the command-line. Otherwise, create the browser using the native
// platform framework. The Views framework is currently only supported on
// Windows and Linux.
const bool use_views = command_line->HasSwitch("use-views");
#else
const bool use_views = false;
#endif
// SimpleHandler implements browser-level callbacks.
CefRefPtr<TestCefHandler> handler(new TestCefHandler(use_views));
// Specify CEF browser settings here.
CefBrowserSettings browser_settings;
std::string url;
// Check if a "--url=" value was provided via the command-line. If so, use
// that instead of the default URL.
url = command_line->GetSwitchValue("url");
if (url.empty())
{
//url = "file:///C:/Users/Administrator/Desktop/flash/test.html";
url = "www.baidu.com";
}
if (use_views) {
// Create the BrowserView.
CefRefPtr<CefBrowserView> browser_view = CefBrowserView::CreateBrowserView(
handler, url, browser_settings, NULL, NULL, NULL);
// Create the Window. It will show itself after creation.
CefWindow::CreateTopLevelWindow(new SimpleWindowDelegate(browser_view));
}
else {
// Information used when creating the native window.
CefWindowInfo window_info;
#if defined(OS_WIN)
// On Windows we need to specify certain flags that will be passed to
// CreateWindowEx().
window_info.SetAsPopup(NULL, "cefsimple");
#endif
// Create the first browser window.
CefBrowserHost::CreateBrowser(window_info, handler, url, browser_settings,
NULL, NULL);
}
}
void TestCefAPP::OnBeforeCommandLineProcessing(const CefString & process_type, CefRefPtr<CefCommandLine> command_line)
{
//加载flash插件
command_line->AppendSwitchWithValue("--ppapi-flash-path", "pepflashplayer32_32_0_0_207.dll");
//manifest.json中的version
command_line->AppendSwitchWithValue("--ppapi-flash-version", "32.0.0.207");
//使用系统flash
//command_line->AppendSwitchWithValue("enable-system-flash", "true");
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
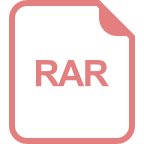
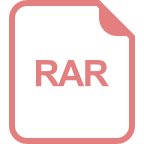
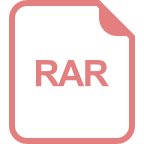
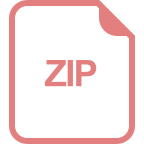
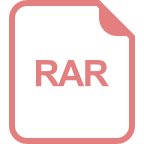
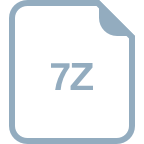
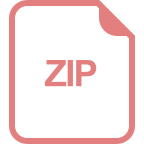
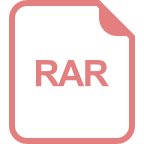
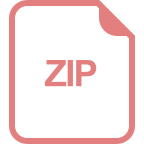
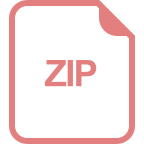
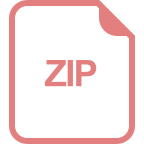
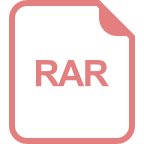
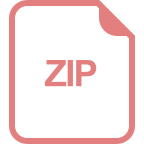
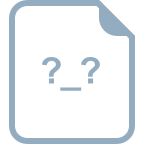
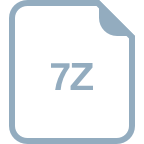
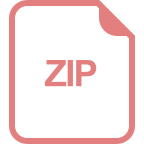
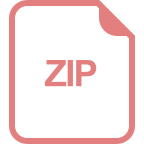
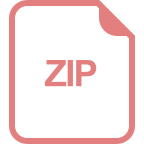
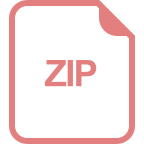
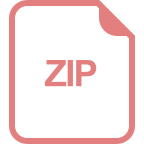
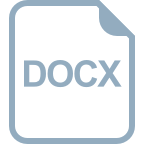
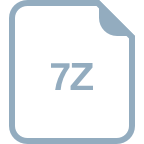
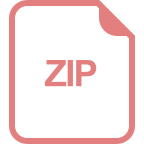
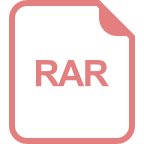
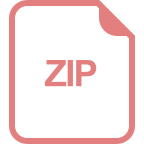
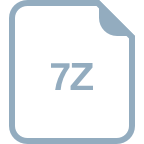
收起资源包目录

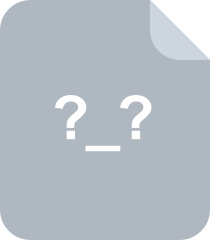
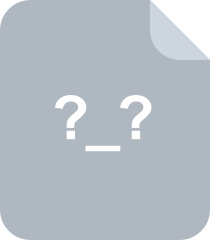
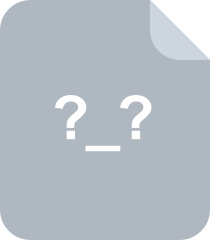
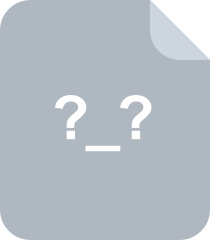
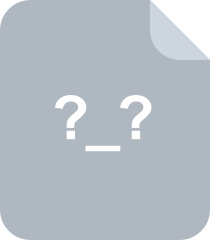
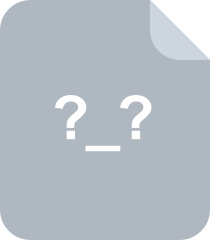
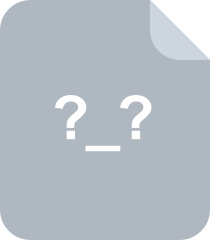
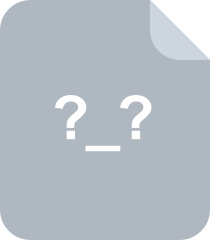
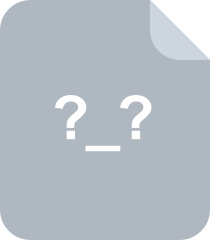
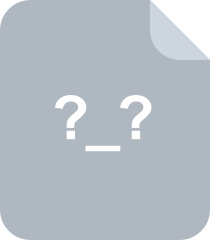
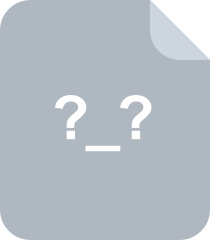
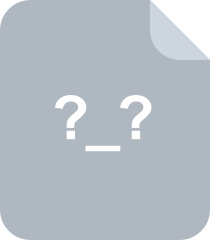
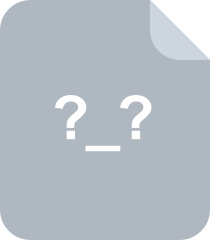
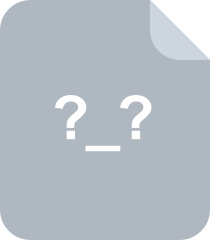
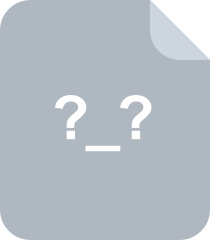
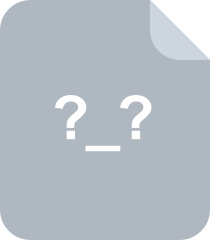
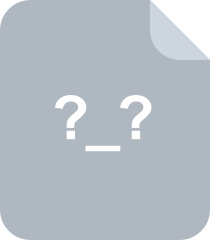
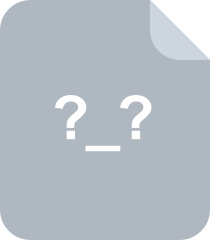
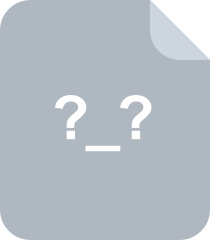
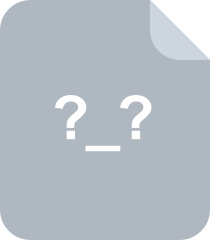
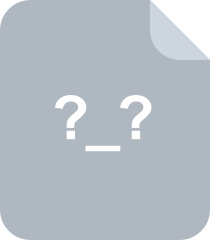
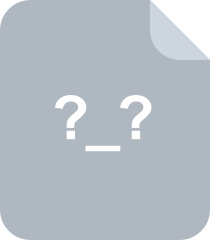
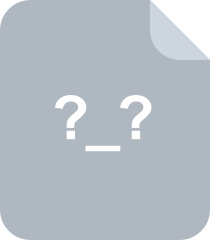
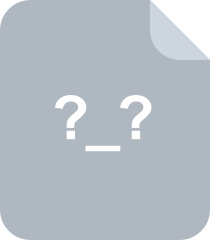
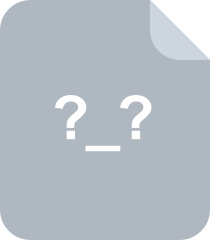
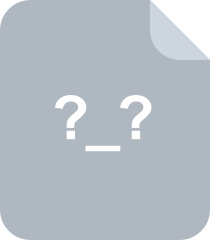
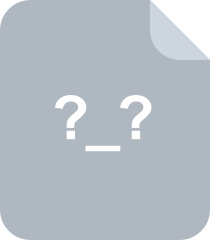
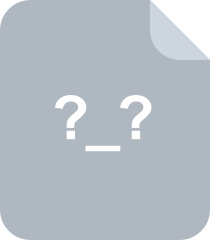
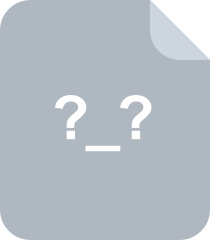
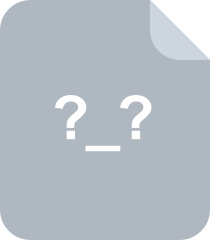
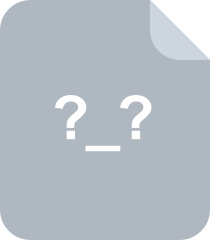
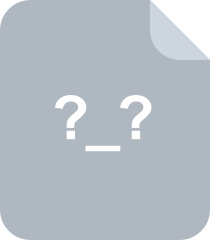
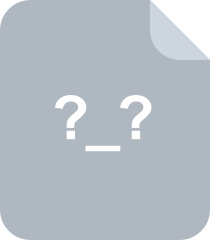
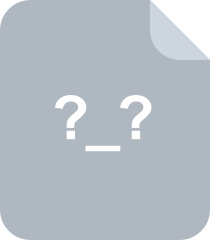
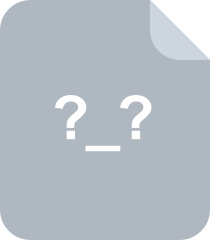
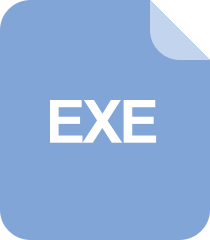
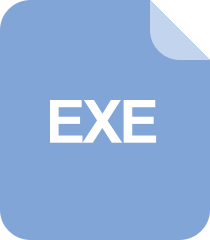
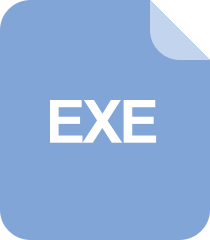
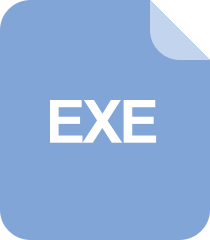
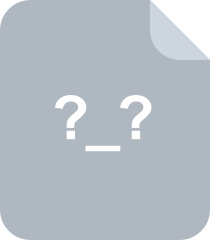
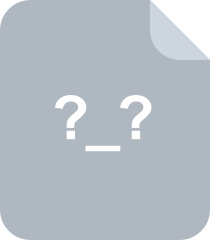
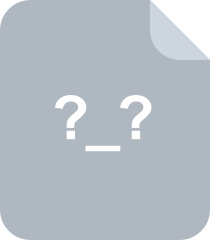
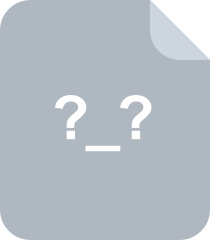
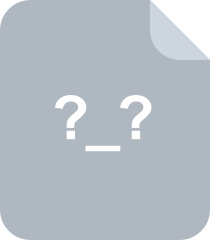
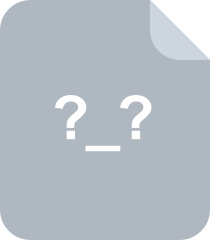
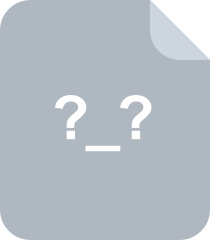
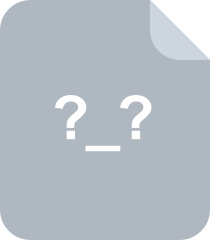
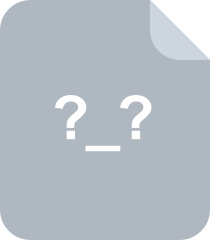
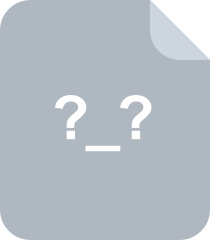
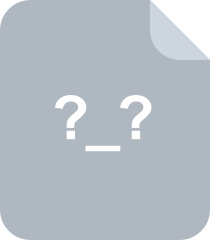
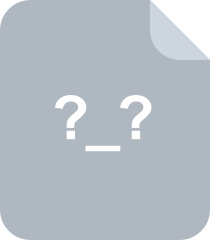
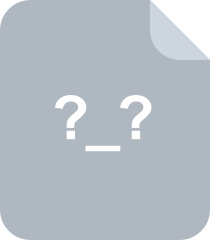
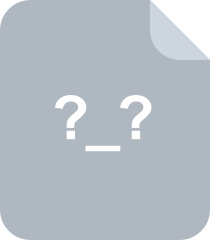
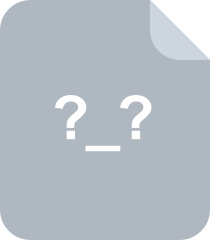
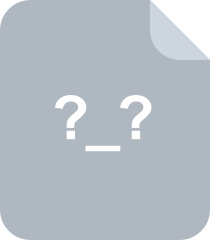
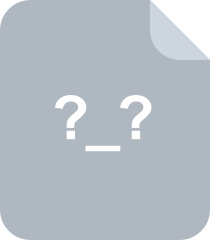
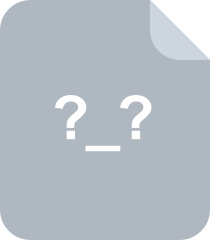
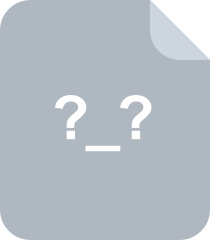
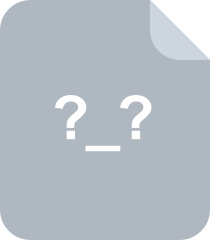
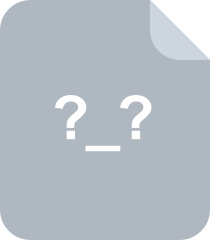
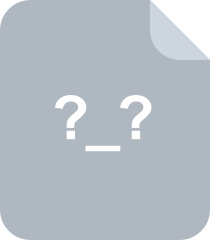
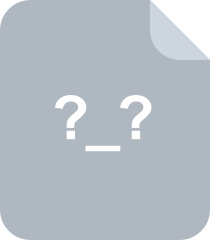
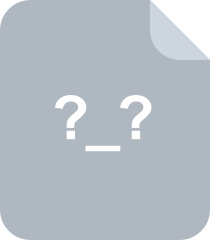
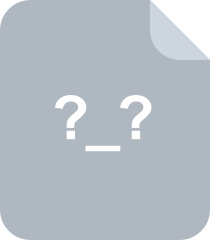
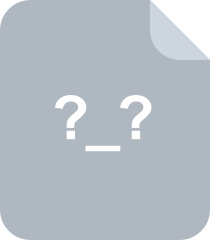
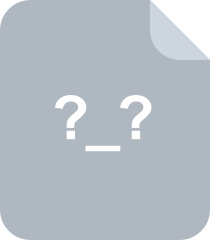
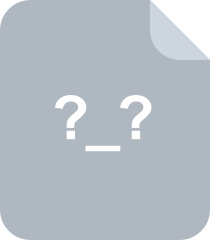
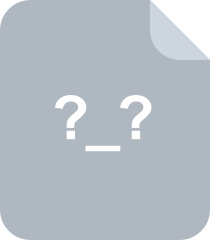
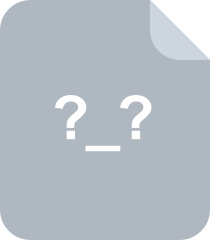
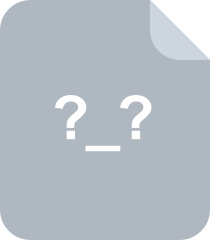
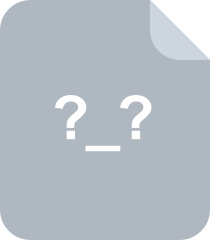
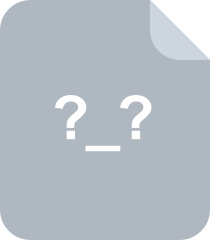
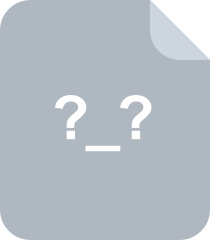
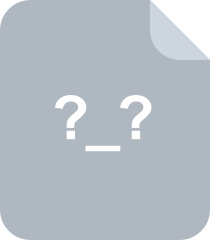
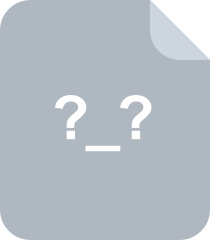
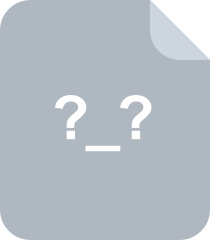
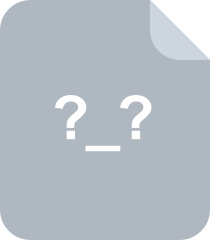
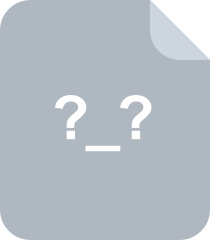
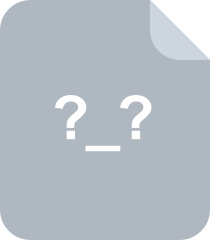
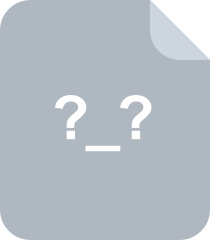
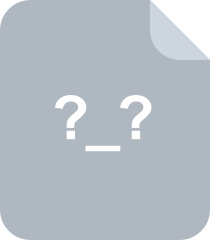
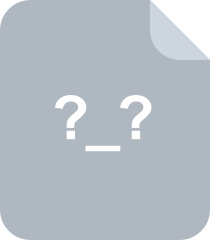
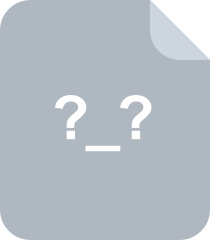
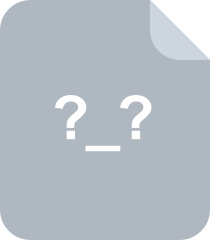
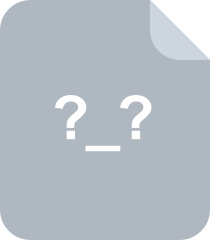
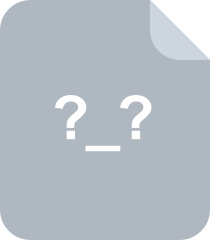
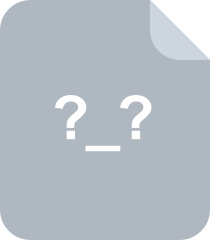
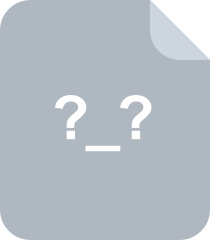
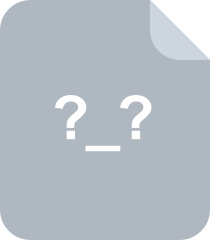
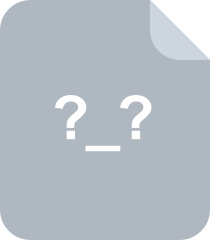
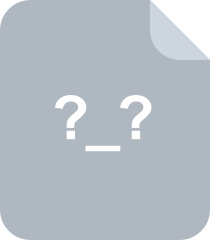
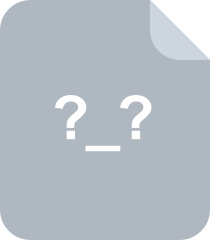
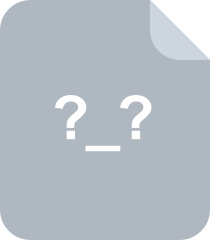
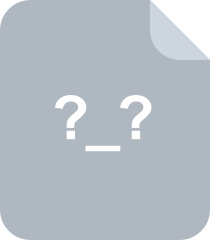
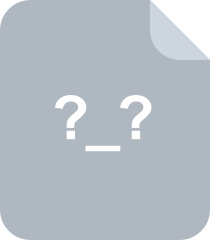
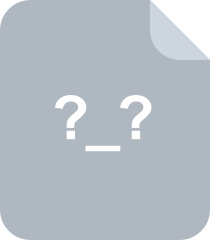
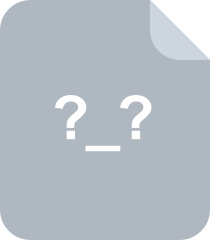
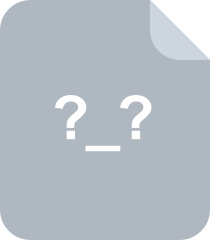
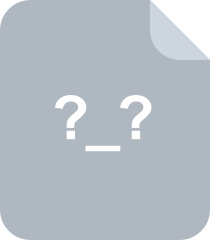
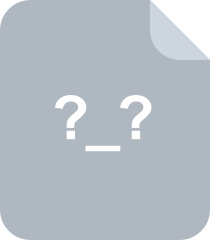
共 456 条
- 1
- 2
- 3
- 4
- 5
资源评论
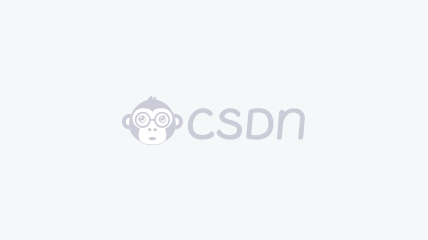

N89757
- 粉丝: 3
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

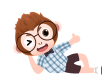
最新资源
- 离线OCR(此软件解压后双击即可运行, 免费)
- 公开整理-上市公司员工学历及工资数据(1999-2023年).xlsx
- 公开整理-上市公司员工学历及工资数据集(1999-2023年).dta
- GDAL-3.4.3-cp38-cp38-win-amd64.whl(GDAL轮子-免编译pip直接装,下载即用)
- 基于Java实现WIFI探针的商业大数据分析技术
- 抖音5.6版本、抖音短视频5.6版、抖音iOS5.6版、抖音ipa包5.6
- 图像处理领域、QT技术、架构,可直接借鉴
- 【源码+数据库】基于Spring Boot+Mybatis+Thymeleaf实现的宠物医院管理系统
- H5漂流瓶交友源码 社交漂流瓶H5源码+对接Z支付+视频教程
- 华为ICT大赛云赛道真题资源库.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


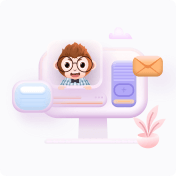
安全验证
文档复制为VIP权益,开通VIP直接复制
