# Go Code Examples
## Topics
- Back-end
- Clean Architecture
- CRUD
- Debugging Mode
- [Delve](https://github.com/go-delve/delve)
- Dependency Injection
- Design Patterns
- Docker Compose
- Dockerfile with multi-stage builds
- DTO
- [Gin Web Framework](https://github.com/gin-gonic/gin)
- Golang 1.21
- [Goose](https://github.com/pressly/goose)
- [GORM](https://github.com/go-gorm/gorm)
- Linux
- [Logrus](https://github.com/sirupsen/logrus)
- Makefile
- MVC
- PostgreSQL 16
- RESTful API
- SOLID
- SQL
- [Viper](https://github.com/spf13/viper)
## Installation
1. Clone this repository:
```
git clone git@github.com:d-alejandro/go-code-examples.git
```
2. Go to `go-code-examples` directory:
```
cd go-code-examples
```
3. Create a new .env file:
```
cp .env.example .env
```
4. Download and install `Go` SDK `1.21.6`.
5. Build and run the application:
```
make run
```
or
```
make
```
6. Start the debugging process in the IDE with `Go Remote` configuration.
```
Host: localhost
Port: 7000
```
7. Apply migrations:
```
make migrate
```
## API Endpoints
### All Orders with pagination
- Request URL: `http://localhost:8080/api/orders?start=0&end=1&sort_column=id&sort_type=asc`
- Method: `GET`
- Path: `/orders`
- Headers: `Accept:application/json, Content-Type:application/json`
- Parameters: `start, end, sort_column, sort_type`
- Status Code: `200`
- Response:
```json
{
"data": [
{
"id": 10000001,
"agency_name": "ОАО ITНефтьРыбОпт",
"status": "waiting",
"is_confirmed": false,
"is_checked": false,
"rental_date": "31-12-2023",
"user_name": "Валерия Фёдоровна Вишнякова",
"transport_count": 3,
"guest_count": 3,
"admin_note": null
}
]
}
```
### Create Order
- Request URL: `http://localhost:8080/api/orders`
- Method: `POST`
- Path: `/orders`
- Headers: `Accept:application/json, Content-Type:application/json`
- Parameters: `agency_name, rental_date, guest_count, transport_count, user_name, email, phone`
- Status Code: `200`
- Response:
```json
{
"data": {
"id": 10000011,
"agency_name": "Test Agency Name",
"status": "waiting",
"is_confirmed": false,
"is_checked": false,
"rental_date": "01-02-2024",
"user_name": "Test User Name",
"transport_count": 1,
"guest_count": 1,
"admin_note": "",
"note": "",
"email": "test@test.ru",
"phone": "71437854547",
"confirmed_at": null,
"created_at": "31-01-2024 22:11:14",
"updated_at": "31-01-2024 22:11:14"
}
}
```
### Order Details
- Request URL: `http://localhost:8080/api/orders/10000011`
- Method: `GET`
- Path: `/orders/{id}`
- Headers: `Accept:application/json, Content-Type:application/json`
- Status Code: `200`
- Response:
```json
{
"data": {
"id": 10000011,
"agency_name": "Test Agency Name",
"status": "waiting",
"is_confirmed": false,
"is_checked": false,
"rental_date": "01-02-2024",
"user_name": "Test User Name",
"transport_count": 1,
"guest_count": 1,
"admin_note": "",
"note": "",
"email": "test@test.ru",
"phone": "71437854547",
"confirmed_at": null,
"created_at": "31-01-2024 22:11:15",
"updated_at": "31-01-2024 22:11:15"
}
}
```
### Update Order
- Request URL: `http://localhost:8080/api/orders/10000011`
- Method: `PUT`
- Path: `/orders/{id}`
- Headers: `Accept:application/json, Content-Type:application/json`
- Parameters: `guest_count, transport_count, user_name, email, phone`
- Status Code: `200`
- Response:
```json
{
"data": {
"id": 10000011,
"agency_name": "Test Agency Name",
"status": "waiting",
"is_confirmed": false,
"is_checked": false,
"rental_date": "01-02-2024",
"user_name": "Test7",
"transport_count": 7,
"guest_count": 7,
"admin_note": "qq",
"note": "ww",
"email": "777@777.test",
"phone": "71111111111",
"confirmed_at": null,
"created_at": "31-01-2024 22:11:15",
"updated_at": "31-01-2024 22:17:47"
}
}
```
### Delete Order
- Request URL: `http://localhost:8080/api/orders/10000011`
- Method: `DELETE`
- Path: `/orders/{id}`
- Headers: `Accept:application/json, Content-Type:application/json`
- Status Code: `200`
- Response:
```json
{
"data": {
"id": 10000011,
"agency_name": "Test Agency Name",
"status": "waiting",
"is_confirmed": false,
"is_checked": false,
"rental_date": "01-02-2024",
"user_name": "Test7",
"transport_count": 7,
"guest_count": 7,
"admin_note": "qq",
"note": "ww",
"email": "777@777.test",
"phone": "71111111111",
"confirmed_at": null,
"created_at": "31-01-2024 22:11:15",
"updated_at": "31-01-2024 22:17:47"
}
}
```
没有合适的资源?快使用搜索试试~ 我知道了~
Go,Clean Architecture,SOLID,Golang 1.21
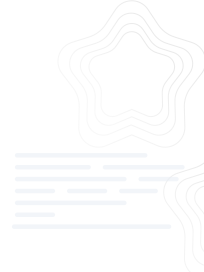
共80个文件
go:65个
gitignore:3个
example:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 115 浏览量
2024-02-02
10:55:57
上传
评论
收藏 70KB ZIP 举报
温馨提示
Go,Clean Architecture,SOLID,Golang 1.21,Gin Web Framework,Delve调试模式,GORM,Goose,Logrus,Viper,依赖注入,RESTful API,PostgreSQL 16,CRUD,DTO,MVC,Docker Compose,SQL,设计模式.zip
资源推荐
资源详情
资源评论
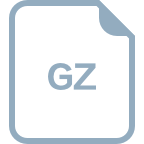
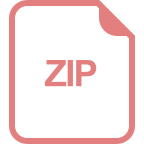
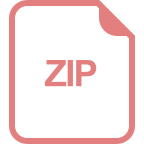
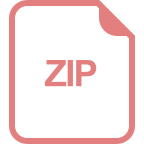
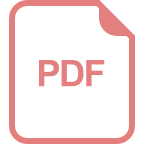
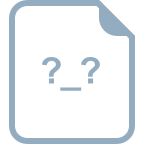
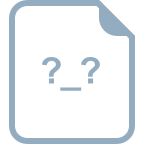
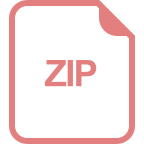
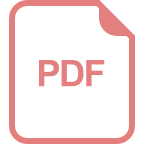
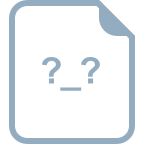
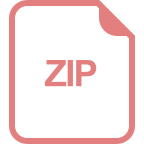
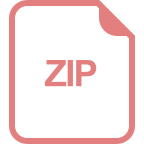
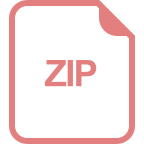
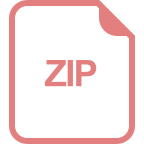
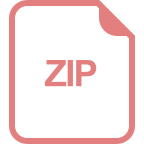
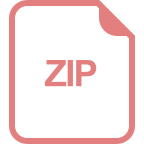
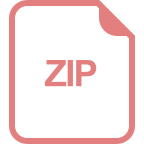
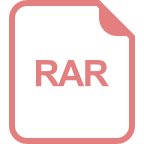
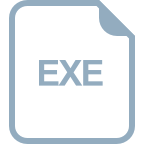
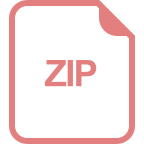
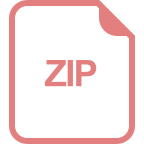
收起资源包目录






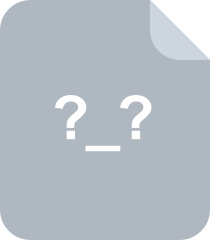
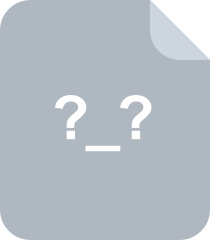
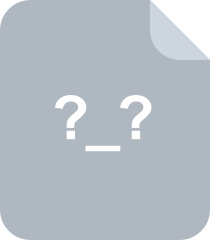
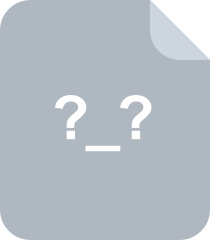
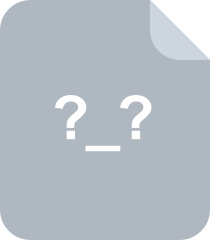
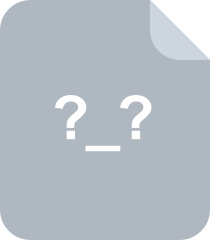

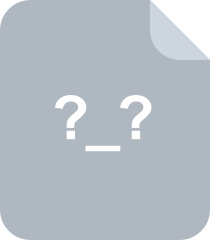
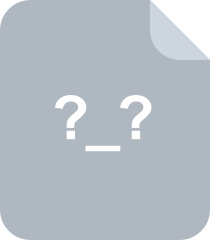
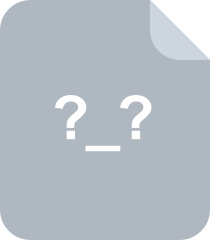



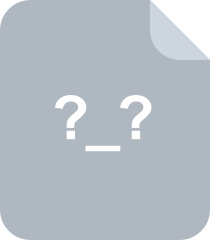

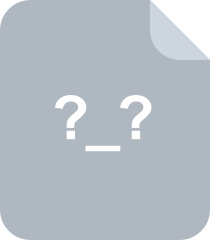

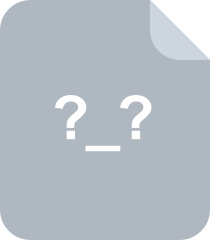
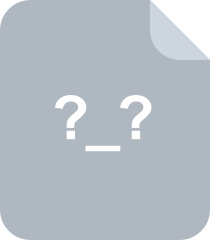



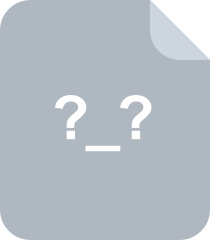
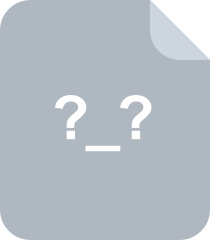
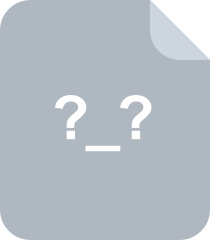

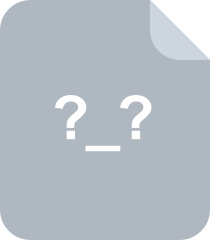
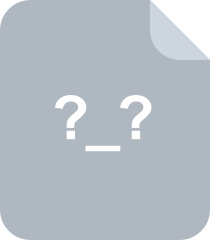
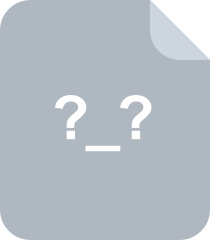


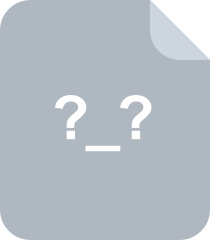
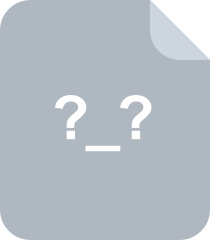
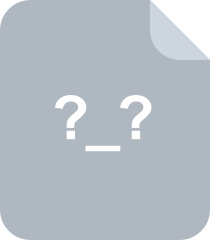


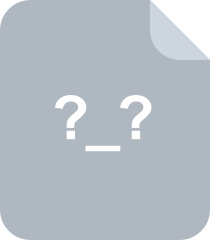
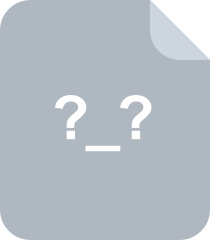
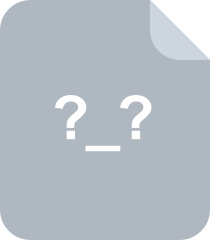
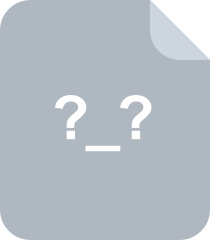
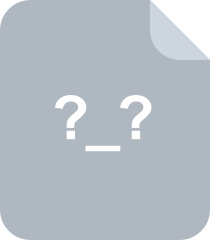
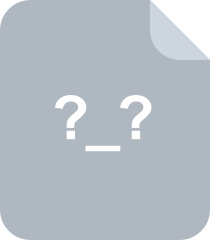
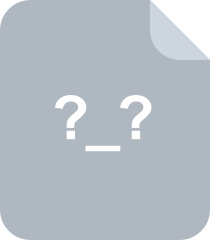
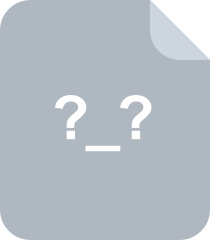
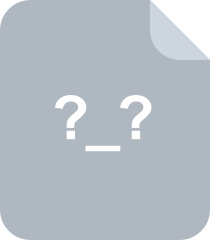
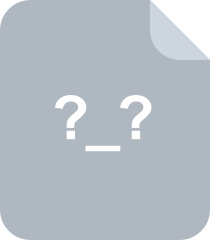

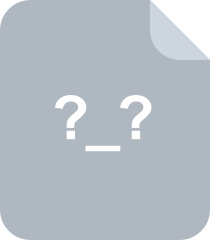
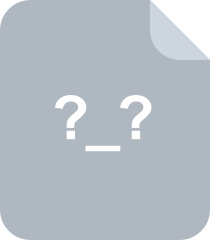
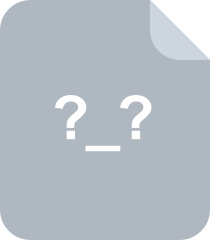

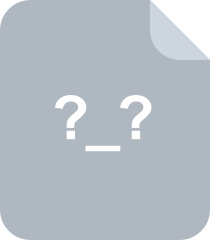
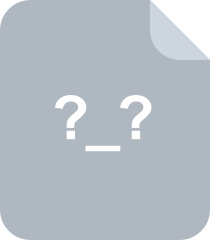
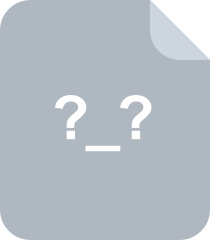
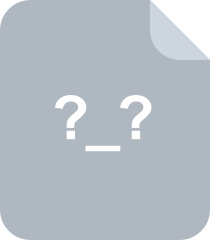
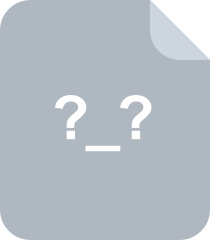

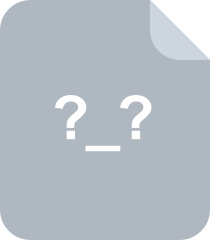
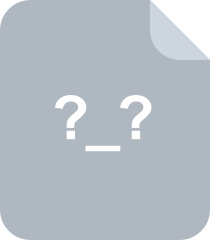
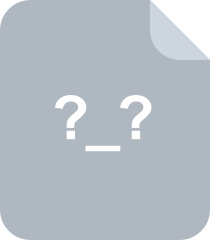
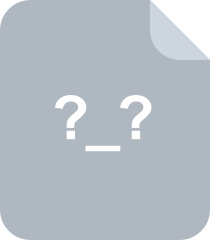

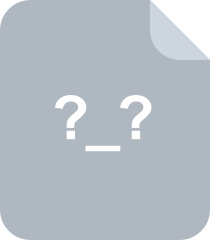

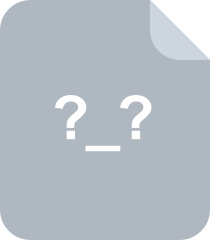
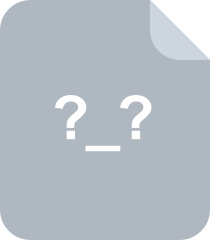

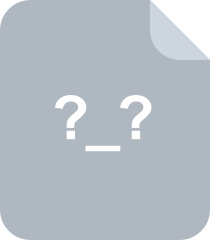

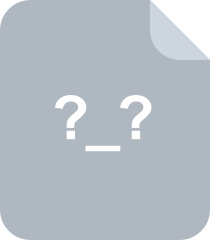
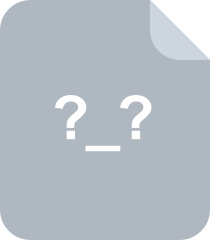
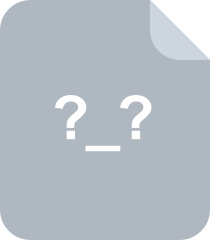
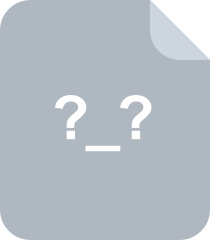
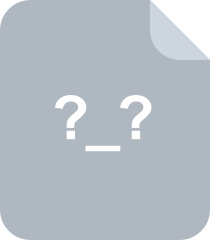
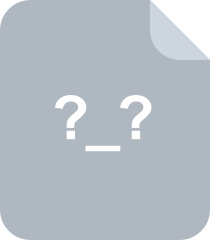
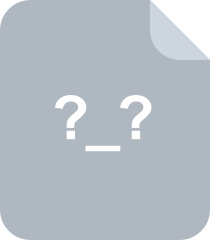
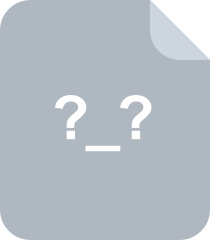

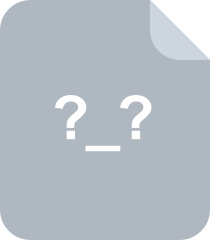
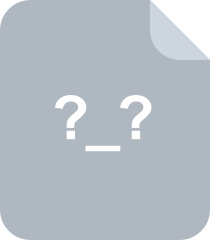
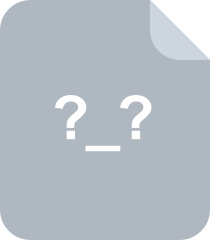
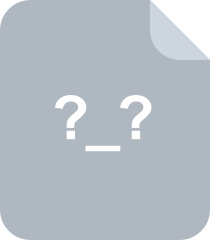
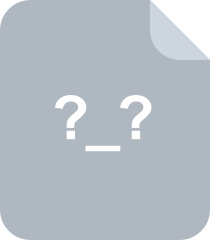
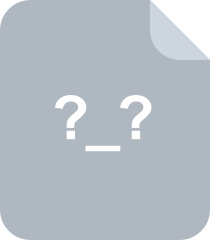
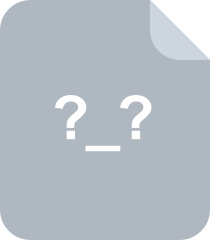
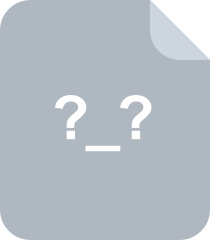
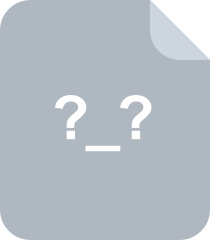
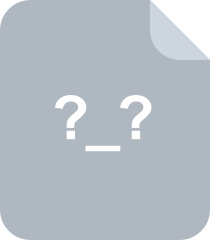

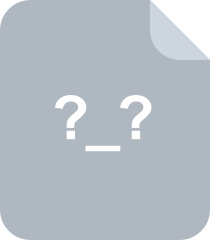


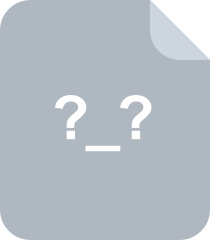
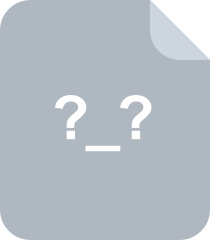
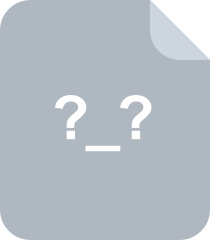

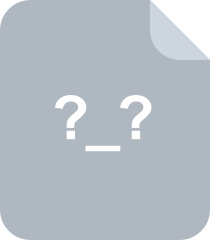
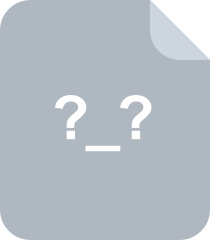

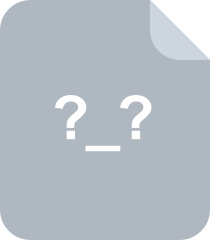
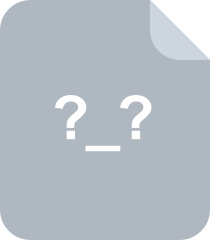
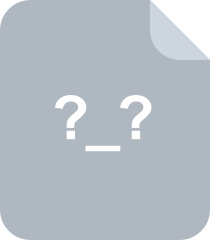


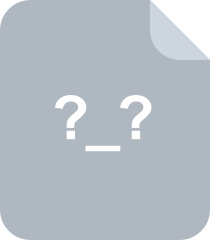

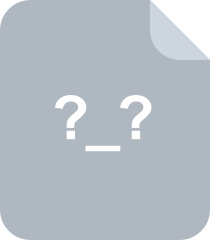

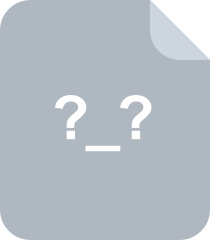
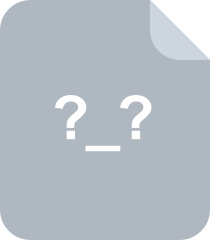
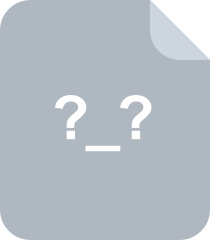
共 80 条
- 1
资源评论
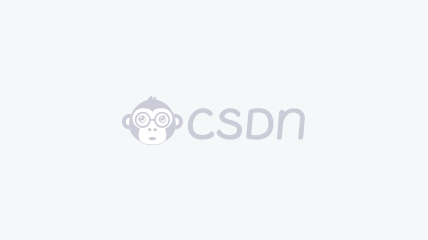

N201871643
- 粉丝: 1387
- 资源: 2713

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

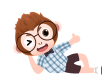
最新资源
- [面板构架]Matlab设计-图像去雾基于Matlab设计-(多方法对比,PSNR,信息熵,GUI界面).zip
- springboot项目智能物流管理系统.zip
- [面板构架]Matlab设计-图像去雾系统(彩色,灰色均可处理,多方法对比,GUI框架).zip
- [面板构架]Matlab设计-图像去雾(多方法,GUI界面).zip
- [面板构架]Matlab设计-雾霾车牌识别GUI设计.zip
- [面板构架]Matlab设计-香烟汉字识别(模板匹配,多过程图,GUI界面).zip
- [面板构架]Matlab设计-危险区域预警(详细解析,GUI).zip
- [面板构架]Matlab设计-芯片字符识别(多过程图,模板匹配).zip
- [面板构架]Matlab设计-信号与系统,数字信号设计(含有GUI).zip
- springboot项目植物健康系统.zip
- springboot项目知识管理系统.zip
- Keil5集成开发环境在嵌入式系统中的应用与详细部署流程详解
- [面板构架]Matlab设计-印刷品缺陷检测(GUI界面,缺陷定位和计数).zip
- [面板构架]Matlab设计-指纹识别(GUI框架).zip
- springboot项目中小企业设备管理系统设计与实现.zip
- springboot项目中小型医院网站.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


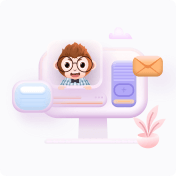
安全验证
文档复制为VIP权益,开通VIP直接复制
