/*
* GL Space Scene...
* Copyright (c) 2000, Jeff Molofee.
* All Rights Reserved.
*/
#include <windows.h> // Header File For Windows
#include <math.h> //
#include <stdio.h> // Header File For Standard Input/Output
#include <gl\gl.h> // Header File For The OpenGL32 Library
#include <gl\glu.h> // Header File For The GLu32 Library
#include <gl\glaux.h> // Header File For The Glaux Library
HDC hDC=NULL; // Private GDI Device Context
HGLRC hRC=NULL; // Permanent Rendering Context
HWND hWnd=NULL; // Holds Our Window Handle
HINSTANCE hInstance; // Holds The Instance Of The Application
bool keys[256]; // Array Used For The Keyboard Routine
bool active=TRUE; // Window Active Flag Set To TRUE By Default
bool fullscreen=TRUE; // Fullscreen Flag Set To Fullscreen Mode By Default
bool bump=TRUE,bp; // Bump Mapping TRUE / FALSE
GLuint texture[6]; // Textures
GLuint loop; // Loop Variable
GLuint ship; // Ship Display List
GLuint planet; // Planet Display List
GLuint stage; // Animation Stage
GLfloat count; // Counter
GLfloat fly=80.0f; // Ship Distance
GLfloat pan; // Pan The Planet
GLfloat rot; // Rotation
GLfloat rotstar; // Star Rotation
GLfloat zoom=60.0f; // Zoom In
GLfloat speed; // Speed Governor
GLUquadricObj* quad=gluNewQuadric();
struct // Create A Structure For The Timer Information
{
__int64 frequency; // Timer Frequency
float resolution; // Timer Resolution
unsigned long mm_timer_start; // Multimedia Timer Start Value
unsigned long mm_timer_elapsed; // Multimedia Timer Elapsed Time
bool performance_timer; // Using The Performance Timer?
__int64 performance_timer_start; // Performance Timer Start Value
__int64 performance_timer_elapsed; // Performance Timer Elapsed Time
} timer; // Structure Is Named timer
static GLfloat axes[]={1.0f,0.0f,0.0f,0.0f};
static GLfloat vdata[982][3] = {
{-0.03468f, 0.05543f, -0.12471f}, {-0.09011f, 0.04157f, -0.19123f}, {-0.17325f, 0.00000f, -0.29100f}, {-0.09011f, -0.04157f, -0.19123f},
{-0.03468f, -0.05543f, -0.12471f}, {-0.03468f, -0.13857f, -0.12471f}, {-0.11782f, -0.18014f, -0.22448f}, {-0.17325f, -0.13857f, -0.29100f},
{-0.17325f, 0.13857f, -0.29100f}, {-0.11782f, 0.18014f, -0.22448f}, {-0.03468f, 0.13857f, -0.12471f}, {-0.03468f, -0.09700f, -0.12471f},
{-0.03468f, 0.09700f, -0.12471f}, {-0.03468f, -0.13857f, -0.06929f}, {-0.03468f, -0.05543f, -0.06928f}, {-0.03468f, -0.09700f, -0.06929f},
{-0.03468f, 0.13857f, -0.06928f}, {-0.03468f, 0.05543f, -0.06928f}, {-0.03468f, 0.09700f, -0.06928f}, {0.02075f, -0.11086f, -0.42957f},
{0.02075f, -0.11086f, -0.06928f}, {-0.11782f, -0.18014f, -0.42957f}, {0.02075f, -0.11086f, -0.24943f}, {-0.11782f, -0.18014f, -0.32702f},
{-0.17325f, -0.13857f, -0.42957f}, {-0.17325f, 0.13857f, -0.42957f}, {-0.17325f, 0.00000f, -0.42957f}, {-0.11782f, 0.18014f, -0.42957f},
{-0.11782f, 0.18014f, -0.32702f}, {0.02075f, 0.11086f, -0.42957f}, {0.02075f, 0.11086f, -0.06928f}, {0.02075f, 0.11086f, -0.24943f},
{0.02075f, 0.00000f, -0.06928f}, {-0.03468f, -0.13857f, 0.01386f}, {-0.03468f, 0.13857f, 0.01386f}, {-0.03468f, 0.05543f, 0.01386f},
{-0.03468f, -0.05543f, 0.01386f}, {-0.03468f, -0.09700f, 0.01386f}, {-0.00702f, -0.12474f, -0.02763f}, {-0.00702f, 0.12474f, -0.02763f},
{-0.03468f, 0.09700f, 0.01386f}, {-0.03468f, -0.13857f, -0.02771f}, {-0.03468f, -0.05543f, -0.02771f}, {-0.03468f, 0.05543f, -0.02771f},
{-0.03468f, 0.13857f, -0.02771f}, {-0.09011f, 0.04157f, 0.20785f}, {-0.03468f, 0.05543f, 0.20785f}, {-0.03468f, 0.05543f, 0.11086f},
{-0.09011f, 0.04157f, 0.07483f}, {-0.09011f, 0.04157f, -0.05820f}, {-0.03468f, -0.05543f, 0.20785f}, {-0.03468f, -0.00000f, 0.20785f},
{-0.09011f, -0.04157f, 0.20785f}, {-0.03468f, -0.05543f, 0.11086f}, {-0.09011f, -0.04157f, -0.05820f}, {-0.09011f, -0.04157f, 0.07483f},
{-0.16478f, -0.07433f, 0.15781f}, {-0.16478f, -0.07433f, 0.49479f}, {-0.16478f, -0.00000f, 0.49479f}, {-0.16478f, -0.07433f, 0.32630f},
{-0.13168f, -0.10393f, 0.12471f}, {-0.13168f, -0.10393f, 0.59585f}, {-0.13168f, -0.10393f, 0.28176f}, {-0.01390f, -0.05946f, 0.26359f},
{-0.10397f, -0.09347f, 0.09700f}, {-0.01390f, -0.05946f, 0.20773f}, {-0.16478f, 0.07433f, 0.49479f}, {-0.16478f, 0.07433f, 0.15781f},
{-0.13168f, 0.10393f, 0.12471f}, {-0.13168f, 0.10393f, 0.59585f}, {-0.13168f, 0.10393f, 0.28176f}, {-0.01390f, 0.05946f, 0.26359f},
{-0.10397f, 0.09346f, 0.09700f}, {-0.01390f, 0.05946f, 0.20773f}, {-0.04854f, -0.20747f, -0.51289f}, {-0.10283f, -0.17538f, -0.51289f},
{-0.10168f, -0.11361f, -0.51289f}, {-0.04854f, -0.08353f, -0.51289f}, {0.00575f, -0.11562f, -0.51289f}, {0.00460f, -0.17738f, -0.51289f},
{-0.06545f, -0.12858f, -0.11086f}, {-0.06545f, -0.14528f, -0.11086f}, {-0.06545f, -0.16237f, -0.11086f}, {-0.04875f, -0.12858f, -0.11086f},
{-0.04875f, -0.14528f, -0.11086f}, {-0.04875f, -0.16237f, -0.11086f}, {-0.03165f, -0.12858f, -0.11086f}, {-0.03165f, -0.14528f, -0.11086f},
{-0.03165f, -0.16237f, -0.11086f}, {-0.04854f, -0.17975f, -0.11086f}, {-0.07787f, -0.16317f, -0.11086f}, {-0.07813f, -0.12826f, -0.11086f},
{-0.04854f, -0.11122f, -0.11086f}, {-0.01920f, -0.12783f, -0.11086f}, {-0.01895f, -0.16274f, -0.11086f}, {-0.10283f, -0.17538f, -0.32573f},
{-0.04854f, -0.20747f, -0.32573f}, {-0.07906f, -0.19943f, -0.32573f}, {-0.10168f, -0.11361f, -0.32573f}, {-0.11050f, -0.14449f, -0.32573f},
{-0.04854f, -0.08353f, -0.32573f}, {-0.07805f, -0.09100f, -0.32573f}, {0.00460f, -0.17738f, -0.32573f}, {0.00575f, -0.11562f, -0.32573f},
{0.01342f, -0.14650f, -0.32573f}, {-0.01903f, -0.19999f, -0.32573f}, {-0.04854f, -0.20747f, -0.13857f}, {-0.02483f, -0.20274f, -0.13857f},
{-0.00469f, -0.18934f, -0.13857f}, {0.00868f, -0.16919f, -0.13857f}, {0.01347f, -0.14550f, -0.13857f}, {0.00869f, -0.12180f, -0.13857f},
{-0.00469f, -0.10165f, -0.13857f}, {-0.02484f, -0.08828f, -0.13857f}, {-0.04854f, -0.08349f, -0.13857f}, {-0.07224f, -0.08828f, -0.13857f},
{-0.09238f, -0.10165f, -0.13857f}, {-0.10576f, -0.12180f, -0.13857f}, {-0.11055f, -0.14550f, -0.13857f}, {-0.10576f, -0.16919f, -0.13857f},
{-0.09238f, -0.18934f, -0.13857f}, {-0.07225f, -0.20274f, -0.13857f}, {-0.04854f, -0.20747f, -0.23215f}, {-0.01903f, -0.19999f, -0.23215f},
{-0.03383f, -0.20570f, -0.32573f}, {-0.03383f, -0.20570f, -0.23215f}, {0.00460f, -0.17738f, -0.23215f}, {-0.00571f, -0.19029f, -0.32573f},
{-0.00571f, -0.19029f, -0.23215f}, {0.01342f, -0.14650f, -0.23215f}, {0.01120f, -0.16199f, -0.32573f}, {0.01120f, -0.16199f, -0.23215f},
{0.00575f, -0.11562f, -0.23215f}, {0.01174f, -0.13113f, -0.32573f}, {0.01174f, -0.13113f, -0.23215f}, {-0.01802f, -0.09156f, -0.32573f},
{-0.01802f, -0.09156f, -0.23215f}, {-0.00446f, -0.10194f, -0.32573f}, {-0.00446f, -0.10194f, -0.23215f}, {-0.04854f, -0.08353f, -0.23215f},
{-0.03330f, -0.08543f, -0.32573f}, {-0.03330f, -0.08543f, -0.23215f}, {-0.07805f, -0.09100f, -0.23215f}, {-0.06324f, -0.08530f, -0.32573f},
{-0.06324f, -0.08530f, -0.23215f}, {-0.10168f, -0.11361f, -0.23215f}, {-0.09136f, -0.10071f, -0.32573f}, {-0.09136f, -0.10071f, -0.23215f},
{-0.11050f, -0.14449f, -0.23215f}, {-0.10827f, -0.12901f, -0.32573f}, {-0.10827f, -0.12901f, -0.23215f}, {-0.10283f, -0.17538f, -0.23215f},
{-0.10882f, -0.15987f, -0.32573f}, {-0.10882f, -0.15987f, -0.23215f}, {-0.07906f, -0.19943f, -0.23215f}, {-0.09262f, -0.18905f, -0.32573f},
{-0.09262f, -0.18905f, -0.23215f}, {-0.06377f, -0.20557f, -0.32573f}, {-0.06377f, -0.20557f, -0.23215f}, {-0.04854f, -0.19935f, -0.11897f},
{-0.00004f, -0.14550f, -0.59585f}, {-0.00697f, -0.14550f, -0.59585f}, {-0.04854f, -0.09700f, -0.59585f}, {-0.04854f, -0.10393f, -0.59585f},
{-0.09704f, -0.14550f, -0.59585f}, {-0.09011f, -0.14550f, -0.59585f}, {-0.00344f, -0.12764f, -0.59585f}, {-0.01424f, -0.11120f, -0.59585f},
{-0.03068f, -0.10040f, -0.59585f}, {-0.0
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
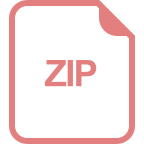
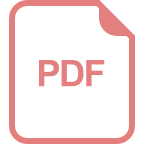
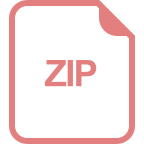
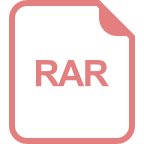
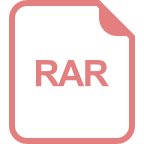
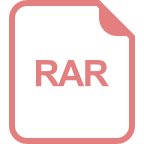
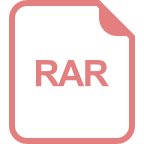
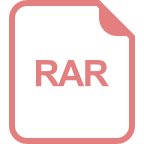
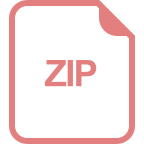
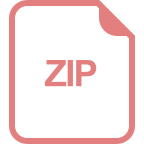
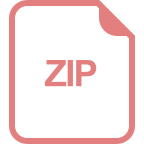
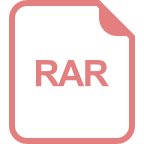
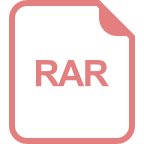
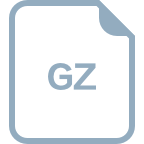
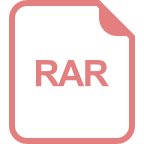
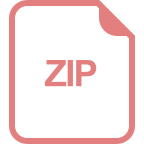
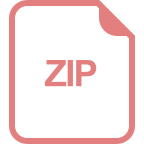
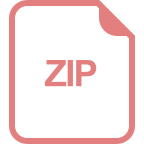
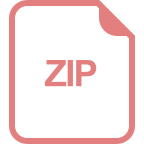
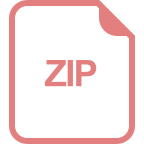
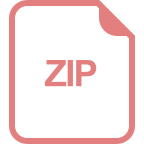
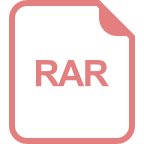
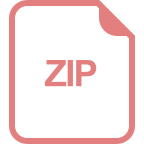
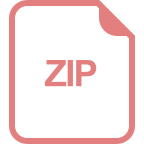
收起资源包目录


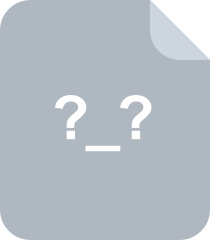

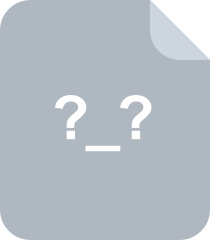
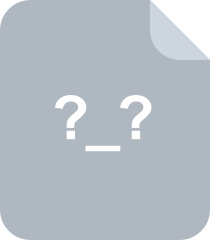
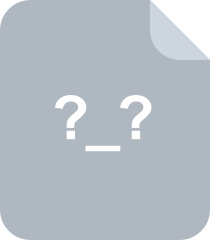
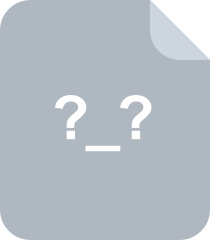
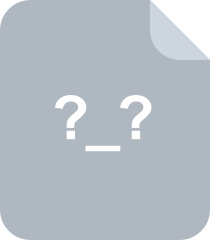
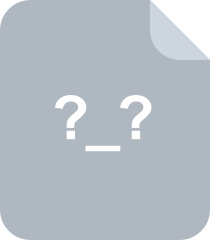
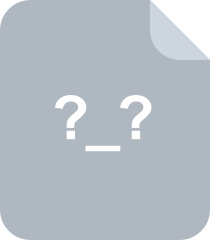
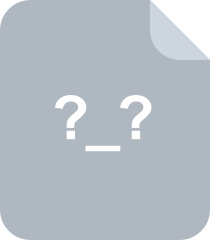
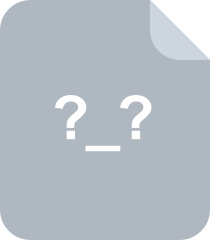
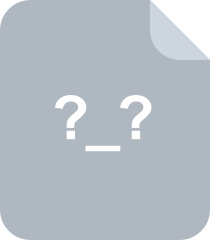
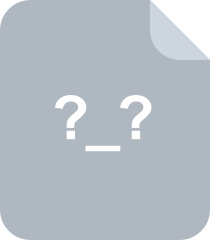
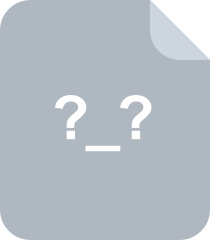
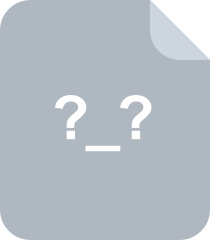

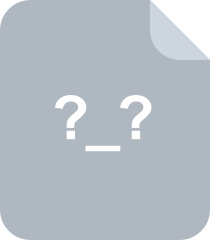
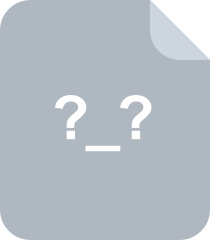
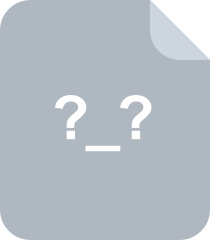
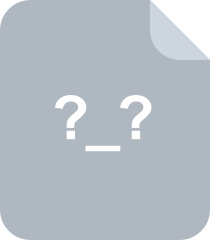
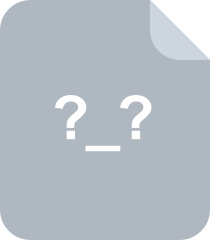
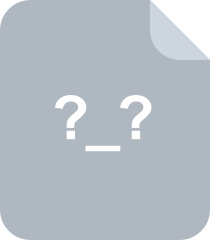
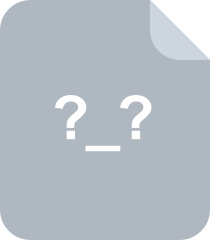
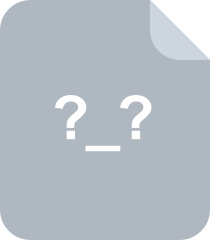
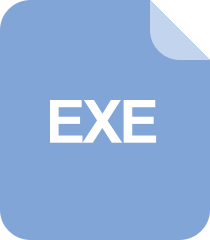
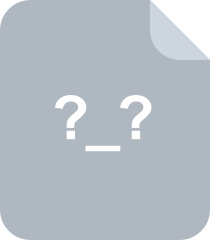
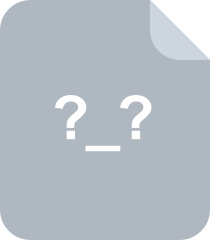
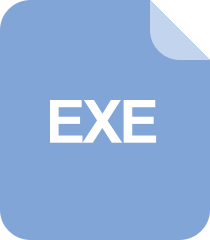
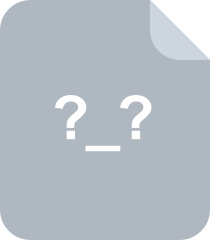
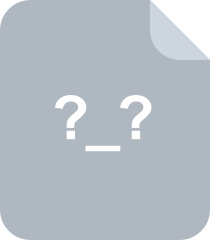
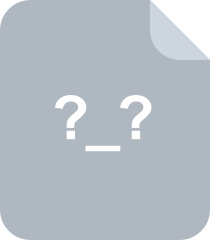
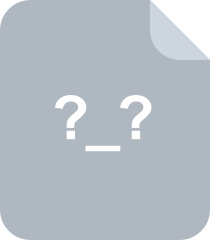
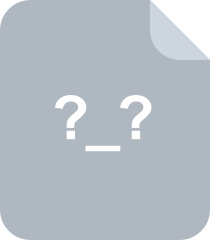
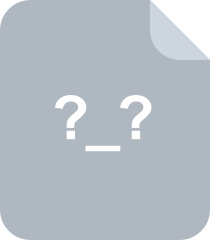

共 32 条
- 1
资源评论
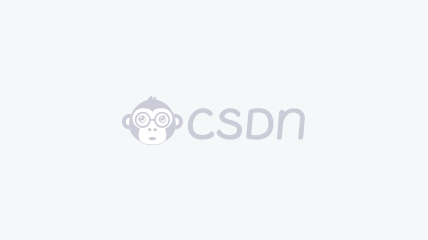
- Noonell2022-07-05资源很好用,有较大的参考价值,资源不错,支持一下。

N201871643
- 粉丝: 1217
- 资源: 2671

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

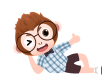
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


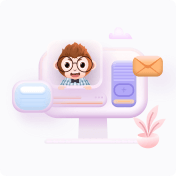
安全验证
文档复制为VIP权益,开通VIP直接复制
