// XMLSettings.cpp : implementation file
//
#include "stdafx.h"
#include "BM.h"
#include "XMLFun.h"
#include <algorithm>
/////////////////////////////////////////////////////////////////////////////
// CXMLFun
CXMLFun::CXMLFun()
{
XmlDocPtr = NULL;
xml_file_name = "config.xml";
}
CXMLFun::~CXMLFun()
{
if(XmlDocPtr!=NULL)
XmlDocPtr.Detach();
}
CXMLFun::CXMLFun(CONST CHAR* filename)
{
::CoInitialize(NULL);
if(XmlDocPtr!=NULL)
XmlDocPtr.Detach();
XmlDocPtr = NULL;
xml_file_name=filename;
load(filename);
}
void CXMLFun::clear()
{
if(XmlDocPtr!=NULL)
XmlDocPtr.Detach();
DeleteFile(xml_file_name.c_str());
load(xml_file_name.c_str());
}
// get a LONG value
LONG CXMLFun::GetLong(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, LONG lDefaultValue)
{
/*
Since XML is text based and we have no schema, just convert to a string and
call the GetString method.
*/
LONG lRetVal = lDefaultValue;
CHAR chs[256];
sprintf(chs,"%d", lRetVal);
lRetVal = atol(GetString(cstrBaseKeyName, cstrValueName, chs).c_str() );
return lRetVal;
}
std::string CXMLFun::GetAttribute(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, CONST CHAR* cstrAttributeName, CONST CHAR* cstrDefaultAttributeValue)
{
std::string strAttributeValue;
std::string strDummy;
GetNodeValue(cstrBaseKeyName, cstrValueName, NULL, strDummy, cstrAttributeName,
cstrDefaultAttributeValue, strAttributeValue);
return strAttributeValue;
}
// get a string value
std::string CXMLFun::GetString(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, CONST CHAR* cstrDefaultValue)
{
std::string strValue;
std::string strDummy;
GetNodeValue(cstrBaseKeyName, cstrValueName, cstrDefaultValue, strValue, NULL, NULL, strDummy);
return strValue;
}
// set a LONG value
LONG CXMLFun::SetLong(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, LONG lValue)
{
LONG lRetVal = 0;
CHAR chsVal[256];
sprintf(chsVal,"%d", lValue);
lRetVal = SetString(cstrBaseKeyName, cstrValueName, chsVal);
return lRetVal;
}
// set a string value
LONG CXMLFun::SetString(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, CONST CHAR* cstrValue)
{
return SetNodeValue(cstrBaseKeyName, cstrValueName,cstrValue);
}
// set a string Attribute
LONG CXMLFun::SetAttribute(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, CONST CHAR* cstrAttributeName, CONST CHAR* cstrAttributeValue)
{
return SetNodeValue(cstrBaseKeyName, cstrValueName, NULL, cstrAttributeName, cstrAttributeValue);
}
LONG CXMLFun::GetNodeValue(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, CONST CHAR* cstrDefaultValue, std::string& strValue, CONST CHAR* cstrAttributeName, CONST CHAR* cstrDefaultAttributeValue,std::string& strAttributeValue)
{
INT iNumKeys = 0;
std::string cstrValue = cstrDefaultValue;
std::string* pCStrKeys = NULL;
std::string strBaseKeyName("//");
strBaseKeyName +=cstrBaseKeyName;
if( strBaseKeyName.at(strBaseKeyName.length() -1) !='/' )
strBaseKeyName += "/";
strBaseKeyName += cstrValueName;
MSXML2::IXMLDOMElementPtr rootElem = NULL;
MSXML2::IXMLDOMNodePtr foundNode = NULL;
foundNode=XmlDocPtr->selectSingleNode( _com_util::ConvertStringToBSTR(strBaseKeyName.c_str()) );
if (foundNode)
{
// get the text of the node (will be the value we requested)
BSTR bstr = NULL;
HRESULT hr = foundNode->get_text(&bstr);
strValue =_com_util::ConvertBSTRToString(bstr);
if(cstrAttributeName!=NULL)
{
MSXML2::IXMLDOMElementPtr elptr=foundNode;
strAttributeValue=_com_util::ConvertBSTRToString(_bstr_t( elptr->getAttribute(_bstr_t(cstrAttributeName))));
}
if (bstr)
{
SysFreeString(bstr);
bstr = NULL;
}
return 0;
}
else
return -1;
}
LONG CXMLFun::SetNodeValue(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName, CONST CHAR* cstrValue, CONST CHAR* cstrAttributeName, CONST CHAR* cstrAttributeValue)
{
/* RETURN VALUES:
0 = SUCCESS -1 = LOAD FAILED -2 = NODE NOT FOUND
-3 = PUT TEXT FAILED -4 = SAVE FAILED
*/
LONG lRetVal = 0;
INT iNumKeys = 0;
std::string* pCStrKeys = NULL;
// Add the value to the base key separated by a '\'
std::string strBaseKeyName(cstrBaseKeyName);
if( strBaseKeyName.at(strBaseKeyName.length() -1) !='/' )
strBaseKeyName += "/";
strBaseKeyName += cstrValueName;
// Parse all keys from the base key name (keys separated by a '\')
pCStrKeys = ParseKeys(strBaseKeyName.c_str(), iNumKeys);
// Traverse the xml using the keys parsed from the base key name to find the correct node
if (pCStrKeys)
{
if (XmlDocPtr == NULL)
return -2;
MSXML2::IXMLDOMElementPtr rootElem = NULL;
MSXML2::IXMLDOMNodePtr foundNode = NULL;
XmlDocPtr->get_documentElement(&rootElem); // root node
if (rootElem)
{
// returns the last node in the chain
foundNode = FindNode(rootElem, pCStrKeys, iNumKeys, true);
if (foundNode)
{
HRESULT hr=NULL;
// set the text of the node (will be the value we sent)
if(cstrValue!=NULL)
hr = foundNode->put_text(_bstr_t(cstrValue));
if(cstrAttributeName!=NULL )
{
MSXML2::IXMLDOMElementPtr elptr=foundNode;
elptr->setAttribute(_bstr_t(cstrAttributeName),
_bstr_t(cstrAttributeValue) );
}
if (!SUCCEEDED(hr))
lRetVal = -3;
foundNode = NULL;
}
else
lRetVal = -2;
rootElem = NULL;
}
delete [] pCStrKeys;
}
return lRetVal;
}
// XMLFun.DeleteSetting("Settings/who","");删除该键及其所有子键
// delete a key or chain of keys
LONG CXMLFun::DeleteSetting(CONST CHAR* cstrBaseKeyName, CONST CHAR* cstrValueName)
{
LONG bRetVal = -1;
INT iNumKeys = 0;
std::string* pCStrKeys = NULL;
std::string strBaseKeyName(cstrBaseKeyName);
if ( strBaseKeyName!="" )
{
if( strBaseKeyName.at(strBaseKeyName.length() -1) !='/' )
strBaseKeyName += "/";
strBaseKeyName += std::string(cstrValueName);
}
// Parse all keys from the base key name (keys separated by a '\')
pCStrKeys = ParseKeys(strBaseKeyName.c_str(), iNumKeys);
// Traverse the xml using the keys parsed from the base key name to find the correct node.
if (pCStrKeys)
{
if (XmlDocPtr == NULL)
return bRetVal;
MSXML2::IXMLDOMElementPtr rootElem = NULL;
MSXML2::IXMLDOMNodePtr foundNode = NULL;
XmlDocPtr->get_documentElement(&rootElem); // root node
if (rootElem)
{
// returns the last node in the chain
foundNode = FindNode(rootElem, pCStrKeys, iNumKeys);
if (foundNode)
{
// get the parent of the found node and use removeChild to delete the found node
MSXML2::IXMLDOMNodePtr parentNode = NULL;
foundNode->get_parentNode(&parentNode);
if (parentNode)
{
HRESULT hr = parentNode->removeChild(foundNode);
parentNode = NULL;
}
foundNode = NULL;
}
rootElem = NULL;
}
delete [] pCStrKeys;
}
return bRetVal;
}
// get a basekey's all children's value
LONG CXMLFun::GetKeysValue(CONST CHAR* cstrBaseKeyName, std::map<std::string, std::string>& keys_val)
{
INT iNumKeys = 0;
std::string* pCStrKeys = NULL;
std::string strValue;
pCStrKeys = ParseKeys(cstrBaseKeyName, iNumKeys);
if (pCStrKeys)
{
if (XmlDocPtr == NULL) // load the xml document
return -1;
MSXML2::IXMLDOMElementPtr rootElem = NULL;
MSXML2::IXMLDOMNodePtr foundNode = NULL;
MSXML2::IXMLDOMNodeListPtr nodelst= NULL;
MSXML2::IXMLDOMNodePtr pNode= NULL;
XmlDocPtr->get_documentElement(&rootElem); // root node
if (rootElem)
{
foundNode = FindNode(rootElem, pCStrKeys, iNumKeys);
if (foundNode)
nodelst=foundNode->GetchildNodes();
if(nodelst!=NULL)
{
for (INT i=0; i<nodelst->length; i++)
{
pNode = nodelst->item[i];
keys_val[(CONST CHAR*)(pNode->nodeName)]=pNode->text;//(CONST CHAR*)pNode->xml;
}
foundNode = NULL;
}
pNode=N

My_lolo
- 粉丝: 11
- 资源: 9
最新资源
- springboot项目基于html的网上团购系统设计与实现.zip
- 220v3300w半桥电磁炉源代码 合泰半桥电磁炉ht45f0074 半桥电磁炉,半桥电磁炉程序电磁炉源程序,电磁炉程序代码,电磁炉开发整套资料,合泰HT45F0074综合资料含有单片机源码,主板PC
- springboot项目基于html的民谣网站的设计与实现.zip
- springboot项目基于Java web的药店管理系统的设计与实现.zip
- springboot项目基于javaEE的校园二手书交易平台的设计与实现.zip
- springboot项目基于HTML语言的环保网站的设计与实现.zip
- springboot项目基于JavaWeb的宠物商城网站设计与实现.zip
- springboot项目基于javaweb的学生用品采购系统.zip
- 电网杆塔训练资源voc格式,已划分好train、val、test
- springboot项目基于JavaWeb的鲜牛奶订购系统的设计与实现.zip
- springboot项目基于javaweb的影院订票系统的设计与实现.zip
- springboot项目基于java的火车票订票系统的设计与实现.zip
- springboot项目基于JAVA的房地产销售管理系统的设计与实现.zip
- OpenCV答题卡识别系统C++实现源码+作业报告.zip
- springboot项目基于Java的家政服务平台的设计与实现.zip
- springboot项目基于Java的民宿管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


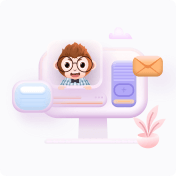
- 1
- 2
- 3
- 4
- 5
前往页