package com.example.springbootspringsecurity.config;
import com.example.springbootspringsecurity.authentication.LoginUserDetailsService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true) // 开启方法级安全验证,加了此prePostEnabled = true就可以在方法上使用@PreAuthorize注解进行角色访问方法权限控制
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private LoginUserDetailsService loginUserDetailsService;
/**
* 设置加密方式
* @return
*/
@Bean
public PasswordEncoder passwordEncoder(){
// 使用BCrypt加密密码
return new BCryptPasswordEncoder();
}
/**
* 自定义身份认证接口
* @param auth
* @throws Exception
*/
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(loginUserDetailsService)// 设置自定义的userDetailsService
.passwordEncoder(passwordEncoder());
}
// /**
// * SpringSecurity会默认拦截静态资源文件
// * 如果不加,springsecurity会自动屏蔽我们引用的css,js等静态资源,导致页面不能加载出该有的样式功能。
// * 应在继承了WebSecurityConfigurerAdapter类中的configure方法中添加允许加载的配置
// * @param web
// * @throws Exception
// */
// @Override
// public void configure(WebSecurity web) throws Exception {
// web.ignoring().antMatchers("/css/**","/fonts/**","/images/**","/js/**");
// }
// @Override
// protected void configure(HttpSecurity http) throws Exception {
// http.headers().frameOptions().disable();//开启运行iframe嵌套页面
// http//1、配置权限认证
// .authorizeRequests()
// //配置不拦截路由
// .antMatchers("/500").permitAll()
// .antMatchers("/403").permitAll()
// .antMatchers("/404").permitAll()
// .antMatchers("/goLogin.do").permitAll()
// .antMatchers("/login.do").permitAll()
// .anyRequest() //任何其它请求
// .authenticated() //都需要身份认证
// .and()
// //2、登录配置表单认证方式
// .formLogin()
// .loginPage("/goLogin.do")//自定义登录页面的url
// .usernameParameter("username")//设置登录账号参数,与表单参数一致
// .passwordParameter("password")//设置登录密码参数,与表单参数一致
// // 告诉Spring Security在发送指定路径时处理提交的凭证,默认情况下,将用户重定向回用户来自的页面。登录表单form中action的地址,也就是处理认证请求的路径,
// // 只要保持表单中action和HttpSecurity里配置的loginProcessingUrl一致就可以了,也不用自己去处理,它不会将请求传递给Spring MVC和您的控制器,所以我们就不需要自己再去写一个/user/login的控制器接口了
// .loginProcessingUrl("/login.do")//配置默认登录入口
// .defaultSuccessUrl("/goIndex.do")//登录成功后默认的跳转页面路径
// .failureUrl("/goLogin.do?error=true")
// .and()
// //3、注销
// .logout()
// .logoutUrl("/logout.do")
// .permitAll()
// .and()
// //4、session管理
// .sessionManagement()
// .invalidSessionUrl("/login.html") //失效后跳转到登陆页面
// //单用户登录,如果有一个登录了,同一个用户在其他地方登录将前一个剔除下线
// //.maximumSessions(1).expiredSessionStrategy(expiredSessionStrategy())
// //单用户登录,如果有一个登录了,同一个用户在其他地方不能登录
// //.maximumSessions(1).maxSessionsPreventsLogin(true) ;
// .and()
// //5、禁用跨站csrf攻击防御
// .csrf()
// .disable();
// }
}
没有合适的资源?快使用搜索试试~ 我知道了~
springBoot整合springSecurity
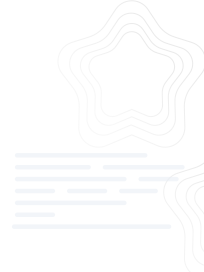
共43个文件
xml:11个
class:10个
java:10个

1 下载量 103 浏览量
2024-01-10
17:40:43
上传
评论
收藏 53KB ZIP 举报
温馨提示
springBoot整合springSecurity完整代码
资源推荐
资源详情
资源评论
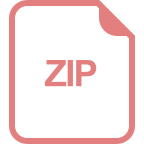
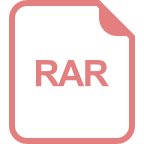
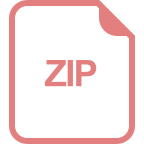
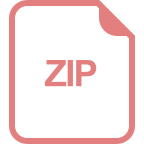
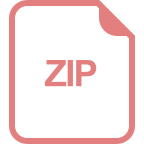
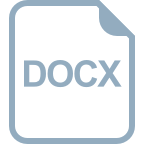
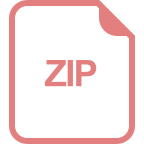
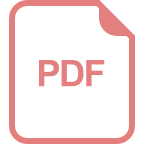
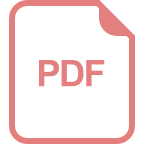
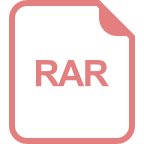
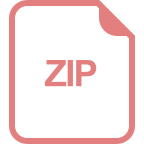
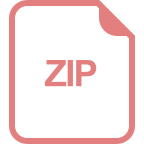
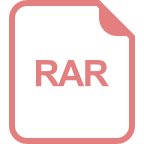
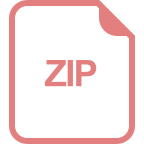
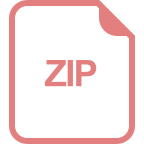
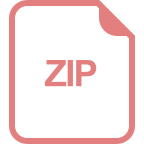
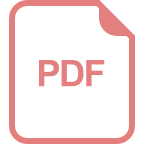
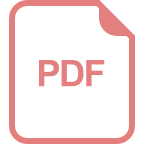
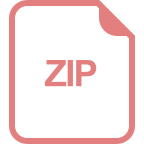
收起资源包目录



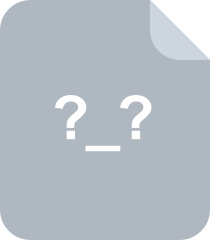

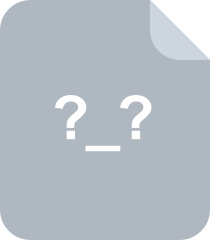
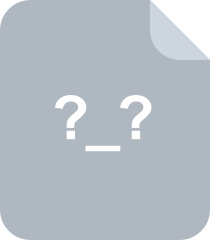






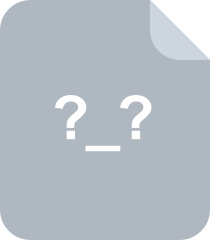



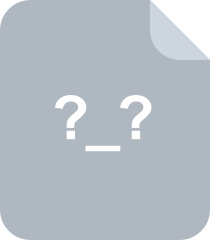

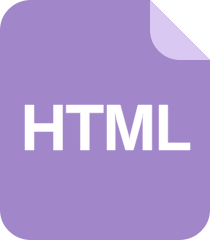
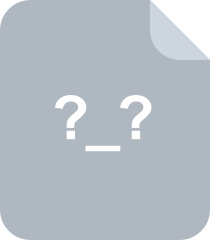





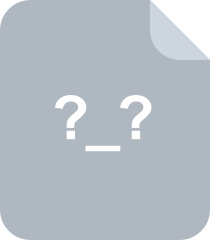

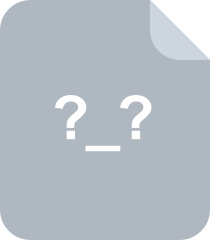
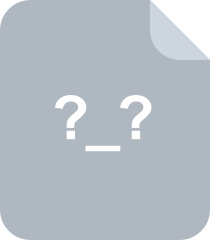

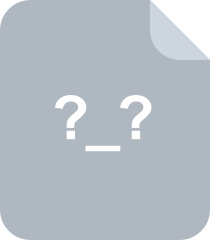

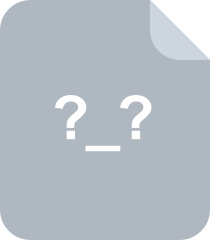

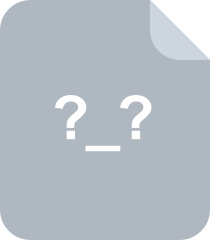

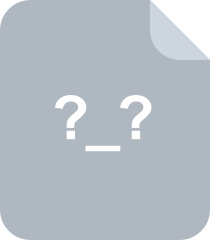

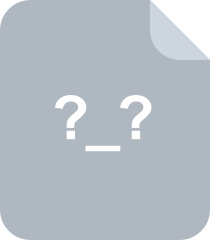

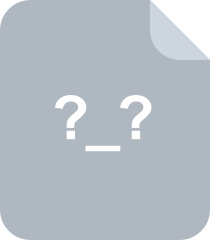

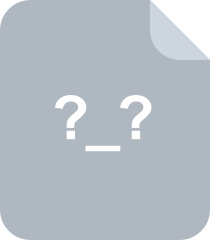





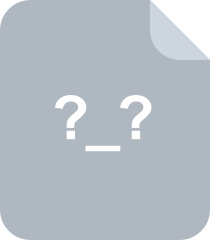
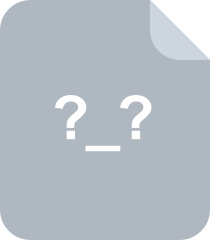
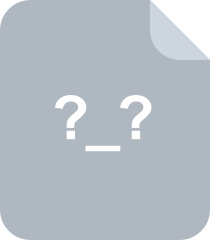
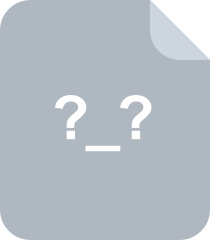
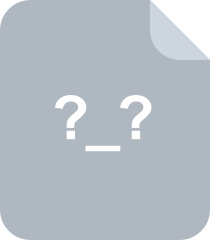
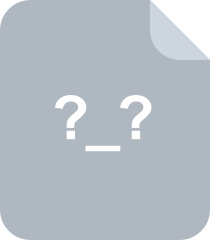
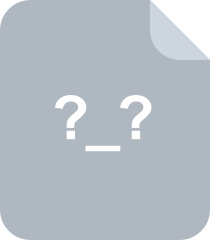
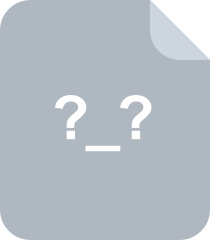
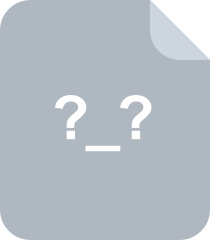
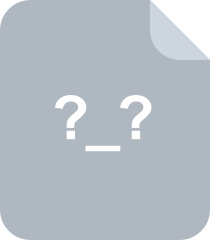
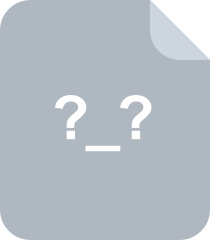
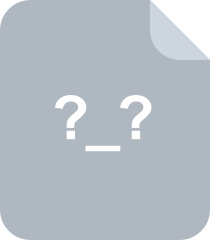



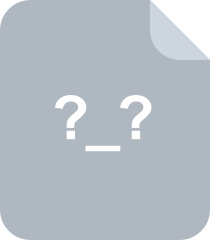

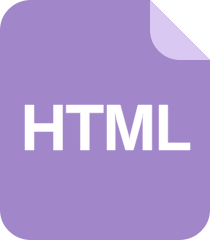
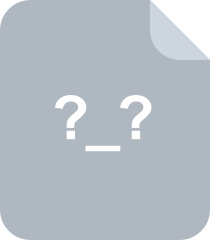




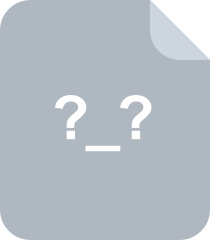

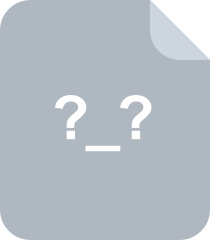

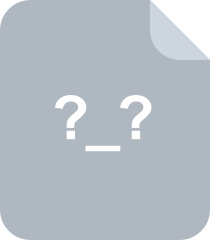

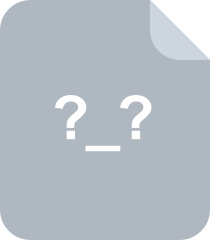
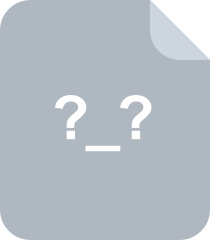

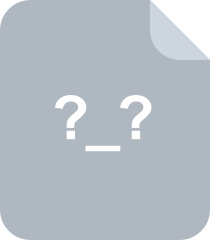

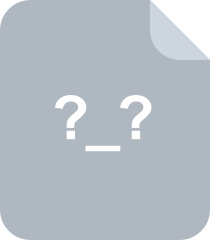

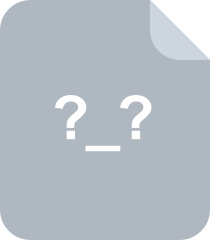

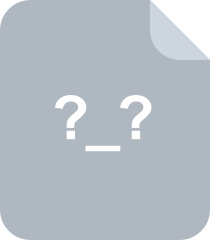




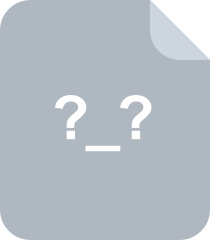




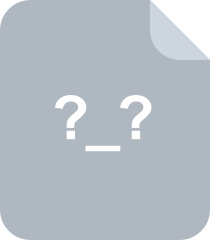
共 43 条
- 1
资源评论
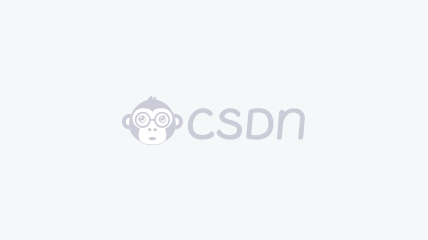

子笙—望舒
- 粉丝: 80
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

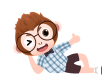
安全验证
文档复制为VIP权益,开通VIP直接复制
