/*!
\file gd32f10x_enet.c
\brief ENET driver
\version 2014-12-26, V1.0.0, firmware for GD32F10x
\version 2017-06-20, V2.0.0, firmware for GD32F10x
\version 2018-07-31, V2.1.0, firmware for GD32F10x
*/
/*
Copyright (c) 2018, GigaDevice Semiconductor Inc.
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
3. Neither the name of the copyright holder nor the names of its contributors
may be used to endorse or promote products derived from this software without
specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY
OF SUCH DAMAGE.
*/
#include "gd32f10x_enet.h"
#ifdef GD32F10X_CL
#if defined (__CC_ARM) /*!< ARM compiler */
__align(4)
enet_descriptors_struct rxdesc_tab[ENET_RXBUF_NUM]; /*!< ENET RxDMA descriptor */
__align(4)
enet_descriptors_struct txdesc_tab[ENET_TXBUF_NUM]; /*!< ENET TxDMA descriptor */
__align(4)
uint8_t rx_buff[ENET_RXBUF_NUM][ENET_RXBUF_SIZE]; /*!< ENET receive buffer */
__align(4)
uint8_t tx_buff[ENET_TXBUF_NUM][ENET_TXBUF_SIZE]; /*!< ENET transmit buffer */
#elif defined ( __ICCARM__ ) /*!< IAR compiler */
#pragma data_alignment=4
enet_descriptors_struct rxdesc_tab[ENET_RXBUF_NUM]; /*!< ENET RxDMA descriptor */
#pragma data_alignment=4
enet_descriptors_struct txdesc_tab[ENET_TXBUF_NUM]; /*!< ENET TxDMA descriptor */
#pragma data_alignment=4
uint8_t rx_buff[ENET_RXBUF_NUM][ENET_RXBUF_SIZE]; /*!< ENET receive buffer */
#pragma data_alignment=4
uint8_t tx_buff[ENET_TXBUF_NUM][ENET_TXBUF_SIZE]; /*!< ENET transmit buffer */
#elif defined (__GNUC__) /* GNU Compiler */
enet_descriptors_struct rxdesc_tab[ENET_RXBUF_NUM] __attribute__ ((aligned (4))); /*!< ENET RxDMA descriptor */
enet_descriptors_struct txdesc_tab[ENET_TXBUF_NUM] __attribute__ ((aligned (4))); /*!< ENET TxDMA descriptor */
uint8_t rx_buff[ENET_RXBUF_NUM][ENET_RXBUF_SIZE] __attribute__ ((aligned (4))); /*!< ENET receive buffer */
uint8_t tx_buff[ENET_TXBUF_NUM][ENET_TXBUF_SIZE] __attribute__ ((aligned (4))); /*!< ENET transmit buffer */
#endif /* __CC_ARM */
/* global transmit and receive descriptors pointers */
enet_descriptors_struct *dma_current_txdesc;
enet_descriptors_struct *dma_current_rxdesc;
/* structure pointer of ptp descriptor for normal mode */
enet_descriptors_struct *dma_current_ptp_txdesc = NULL;
enet_descriptors_struct *dma_current_ptp_rxdesc = NULL;
/* init structure parameters for ENET initialization */
static enet_initpara_struct enet_initpara ={0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
static uint32_t enet_unknow_err = 0U;
/* array of register offset for debug information get */
static const uint16_t enet_reg_tab[] = {
0x0000, 0x0004, 0x0008, 0x000C, 0x0010, 0x0014, 0x0018, 0x1080, 0x001C, 0x0028, 0x002C,
0x0038, 0x003C, 0x0040, 0x0044, 0x0048, 0x004C, 0x0050, 0x0054, 0x0058, 0x005C,
0x0100, 0x0104, 0x0108, 0x010C, 0x0110, 0x014C, 0x0150, 0x0168, 0x0194, 0x0198, 0x01C4,
0x0700, 0x0704,0x0708, 0x070C, 0x0710, 0x0714, 0x0718, 0x071C, 0x0720,
0x1000, 0x1004, 0x1008, 0x100C, 0x1010, 0x1014, 0x1018, 0x101C, 0x1020, 0x1048, 0x104C,
0x1050, 0x1054};
/*!
\brief deinitialize the ENET, and reset structure parameters for ENET initialization
\param[in] none
\param[out] none
\retval none
*/
void enet_deinit(void)
{
rcu_periph_reset_enable(RCU_ENETRST);
rcu_periph_reset_disable(RCU_ENETRST);
enet_initpara_reset();
}
/*!
\brief configure the parameters which are usually less cared for initialization
note -- this function must be called before enet_init(), otherwise
configuration will be no effect
\param[in] option: different function option, which is related to several parameters,
only one parameter can be selected which is shown as below, refer to enet_option_enum
\arg FORWARD_OPTION: choose to configure the frame forward related parameters
\arg DMABUS_OPTION: choose to configure the DMA bus mode related parameters
\arg DMA_MAXBURST_OPTION: choose to configure the DMA max burst related parameters
\arg DMA_ARBITRATION_OPTION: choose to configure the DMA arbitration related parameters
\arg STORE_OPTION: choose to configure the store forward mode related parameters
\arg DMA_OPTION: choose to configure the DMA descriptor related parameters
\arg VLAN_OPTION: choose to configure vlan related parameters
\arg FLOWCTL_OPTION: choose to configure flow control related parameters
\arg HASHH_OPTION: choose to configure hash high
\arg HASHL_OPTION: choose to configure hash low
\arg FILTER_OPTION: choose to configure frame filter related parameters
\arg HALFDUPLEX_OPTION: choose to configure halfduplex mode related parameters
\arg TIMER_OPTION: choose to configure time counter related parameters
\arg INTERFRAMEGAP_OPTION: choose to configure the inter frame gap related parameters
\param[in] para: the related parameters according to the option
all the related parameters should be configured which are shown as below
FORWARD_OPTION related parameters:
- ENET_AUTO_PADCRC_DROP_ENABLE/ ENET_AUTO_PADCRC_DROP_DISABLE ;
- ENET_FORWARD_ERRFRAMES_ENABLE/ ENET_FORWARD_ERRFRAMES_DISABLE ;
- ENET_FORWARD_UNDERSZ_GOODFRAMES_ENABLE/ ENET_FORWARD_UNDERSZ_GOODFRAMES_DISABLE .
DMABUS_OPTION related parameters:
- ENET_ADDRESS_ALIGN_ENABLE/ ENET_ADDRESS_ALIGN_DISABLE ;
- ENET_FIXED_BURST_ENABLE/ ENET_FIXED_BURST_DISABLE ;
DMA_MAXBURST_OPTION related parameters:
- ENET_RXDP_1BEAT/ ENET_RXDP_2BEAT/ ENET_RXDP_4BEAT/
ENET_RXDP_8BEAT/ ENET_RXDP_16BEAT/ ENET_RXDP_32BEAT/
ENET_RXDP_4xPGBL_4BEAT/ ENET_RXDP_4xPGBL_8BEAT/
ENET_RXDP_4xPGBL_16BEAT/ ENET_RXDP_4xPGBL_32BEAT/
ENET_RXDP_4xPGBL_64BEAT/ ENET_RXDP_4xPGBL_128BEAT ;
- ENET_PGBL_1BEAT/ ENET_PGBL_2BEAT/ ENET_PGBL_4BEAT/
ENET_PGBL_8BEAT/ ENET_PGBL_16BEAT/ ENET_PGBL_32BEAT/
ENET_PGBL_4xPGBL_4BEAT/ ENET_PGBL_4xPGBL_8BEAT/
ENET_PGBL_4xPGBL_16BEAT/ ENET_PGBL_4xP
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
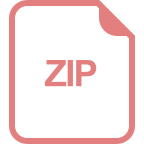
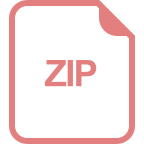
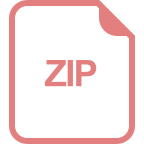
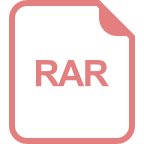
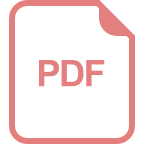
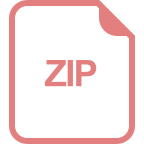
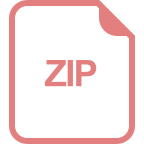
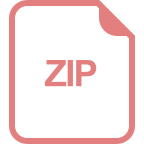
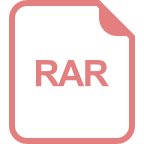
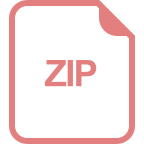
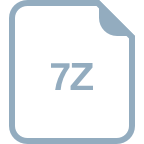
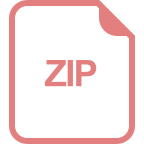
收起资源包目录

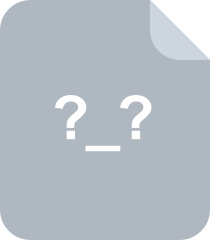
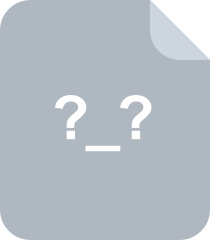
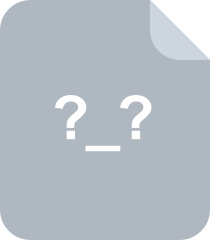
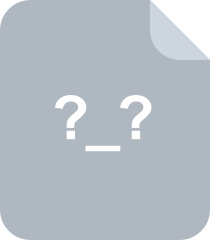
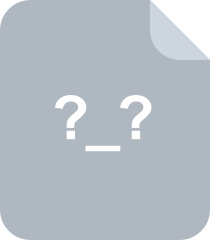
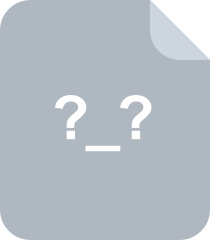
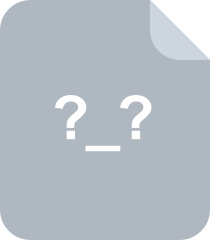
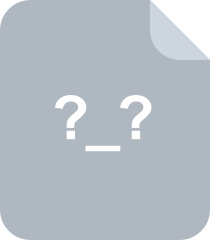
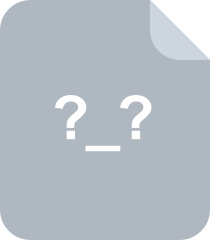
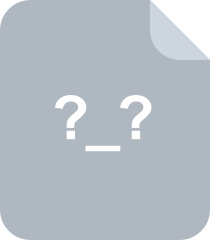
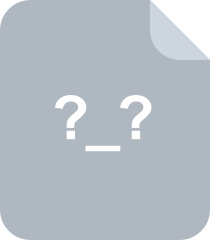
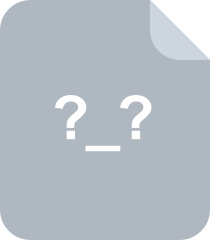
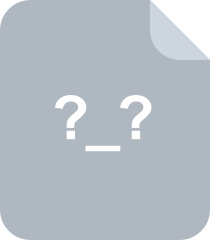
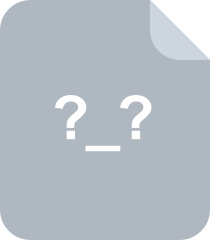
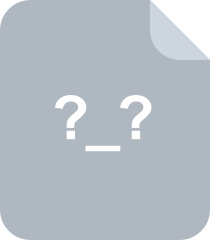
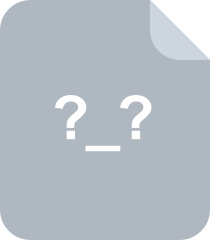
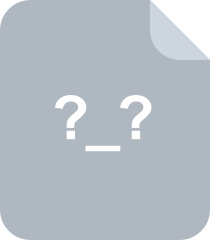
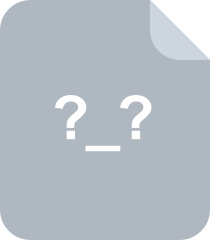
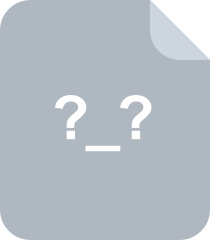
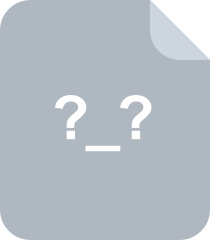
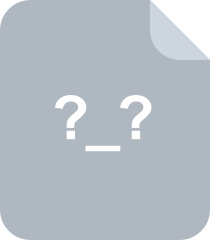
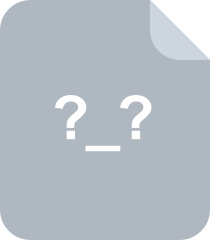
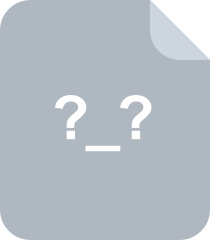
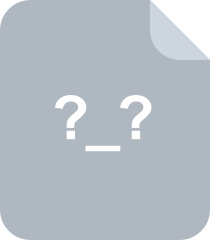
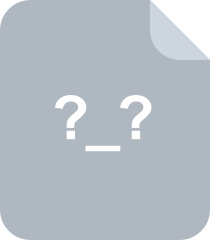
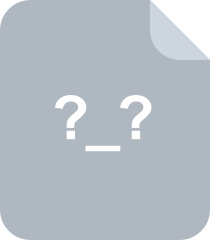
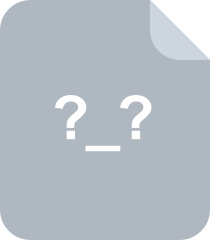
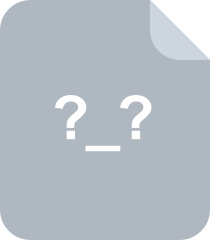
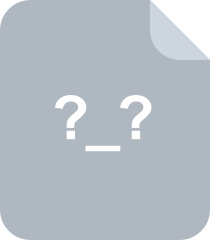
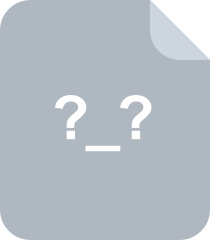
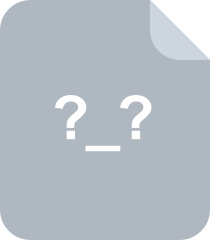
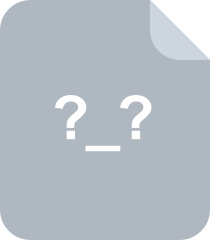
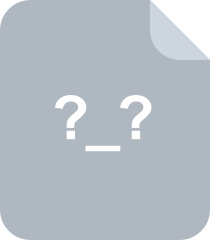
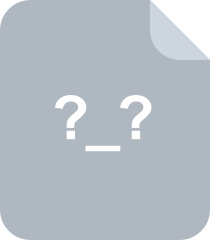
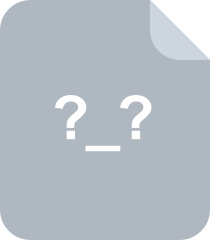
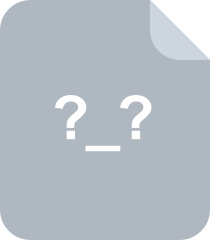
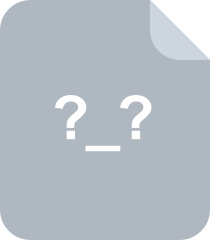
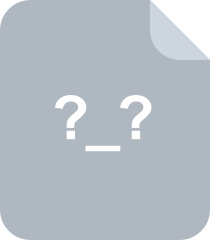
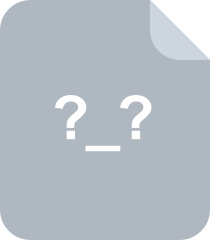
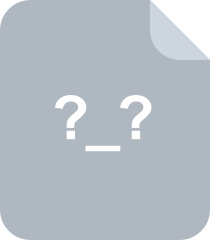
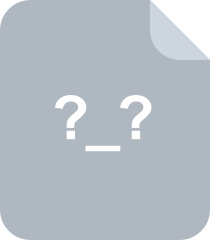
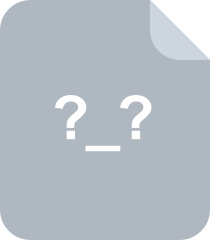
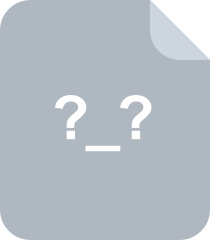
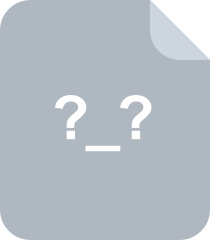
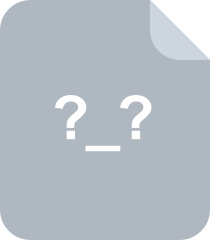
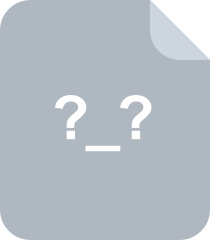
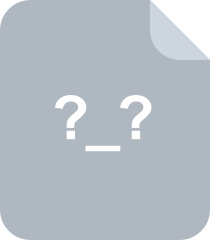
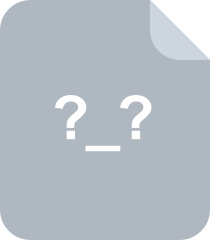
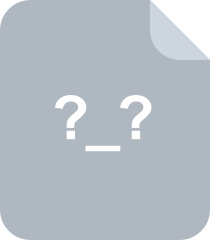
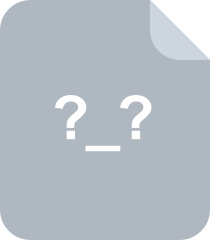
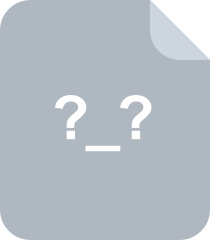
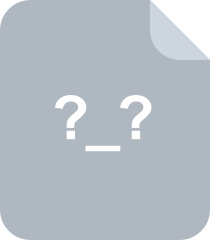
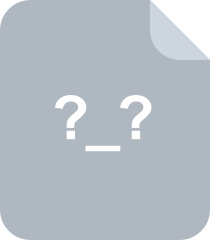
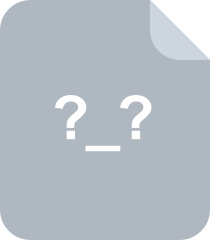
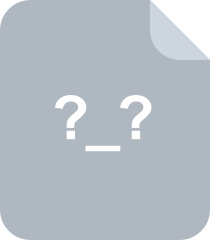
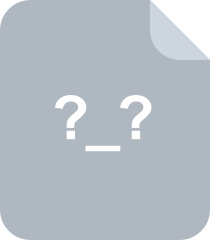
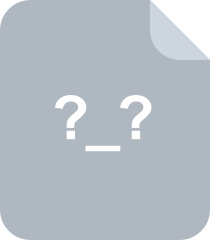
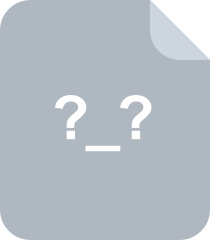
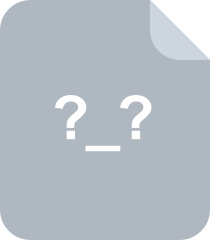
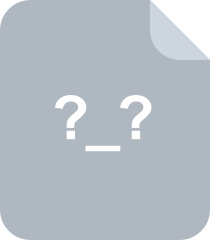
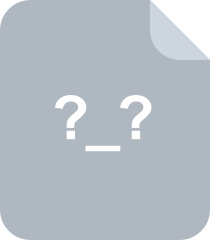
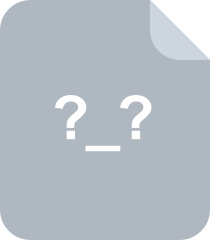
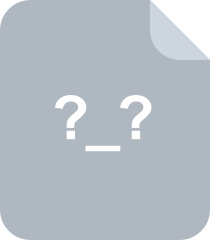
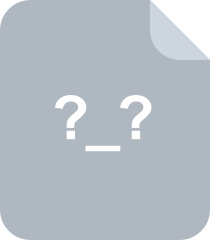
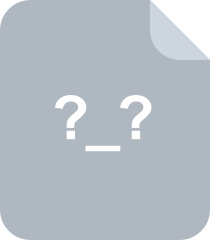
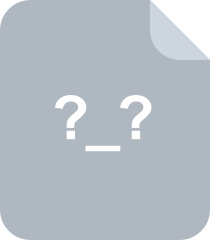
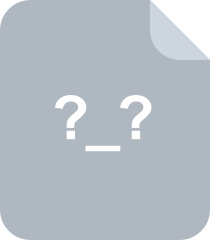
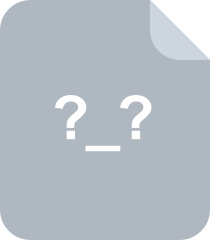
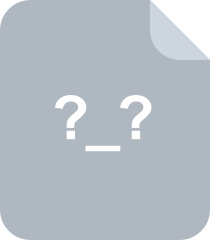
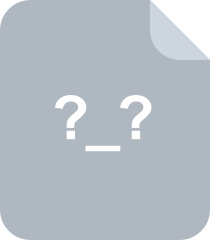
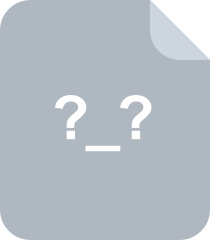
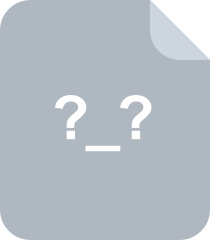
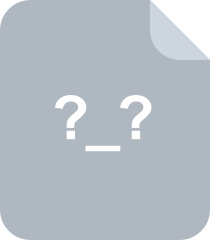
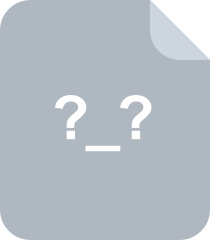
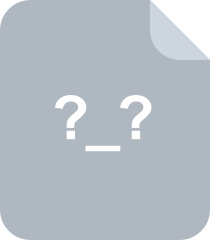
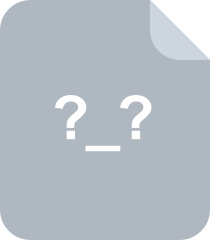
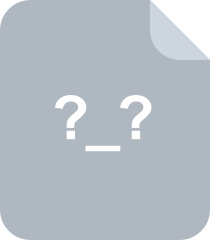
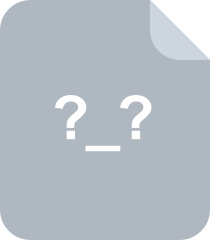
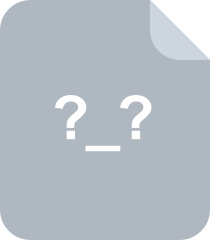
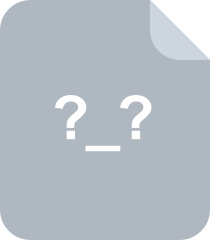
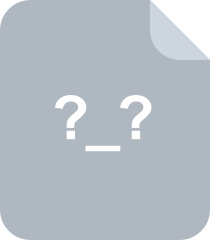
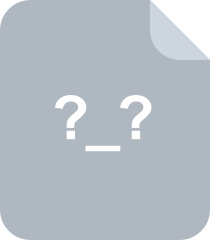
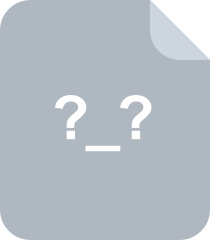
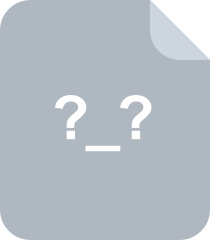
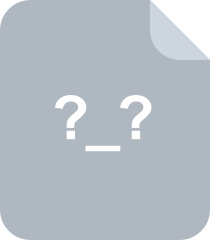
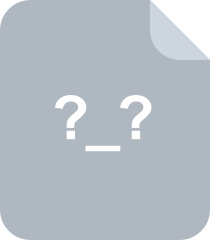
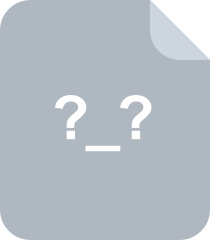
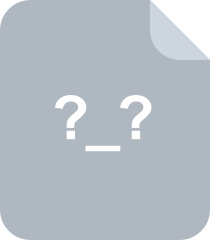
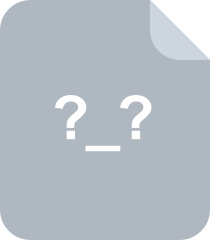
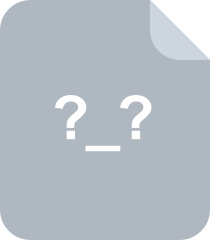
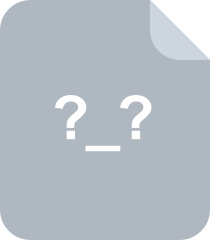
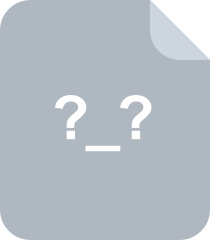
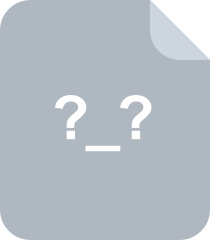
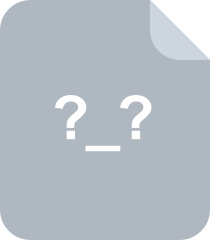
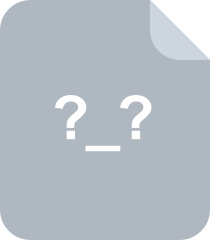
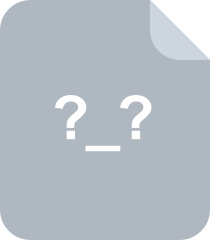
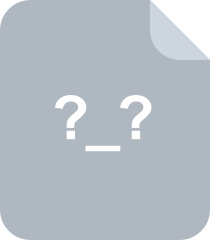
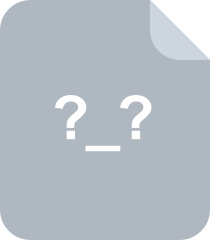
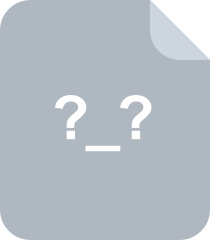
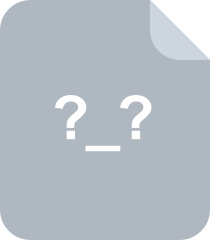
共 423 条
- 1
- 2
- 3
- 4
- 5
资源评论
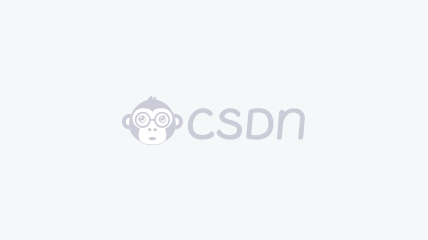

时光の尘
- 粉丝: 1w+
- 资源: 230
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

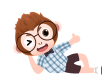
最新资源
- 基于小程序的社区志愿者服务平台源代码(java+小程序+mysql).zip
- 机器学习(预测模型):数字金融对城市经济韧性的影响数据集
- UG NX二次开发源代码:空间点沿着指定矢量IJK移动指定距离求新点坐标
- 基于小程序的短文写作竞赛管理系统源代码(java+小程序+mysql+LW).zip
- 基于小程序的电影院票务系统源代码(java+小程序+mysql).zip
- 基于python flask+mysql的多端点餐系统
- 基于小程序的电影交流平台源代码(java+小程序+mysql+LW).zip
- 基于小程序的游泳馆管理系统源代码(java+小程序+mysql+LW).zip
- 基于小程序的校园商铺系统源代码(java+小程序+mysql+LW).zip
- 基于小程序的校医务室健康服务系统源代码(java+小程序+mysql).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


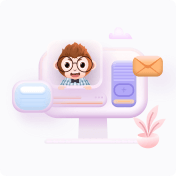
安全验证
文档复制为VIP权益,开通VIP直接复制
