C#银行家算法(考虑安全问题)

C#银行家算法(考虑安全问题) private int m; private int n; private int[] Available; private int[][] Max; private int[][] Allocation; private int[][] Need; private readonly char[] sprit ={ ' ', '\t', '\n' }; 根据提供的文件信息,我们可以深入探讨C#中的银行家算法实现及其在资源分配和死锁避免中的应用。银行家算法是一种著名的算法,用于预防系统中出现死锁情况,它由计算机科学先驱Edsger Dijkstra提出。下面我们将详细介绍该算法的基本原理、数据结构以及具体的实现细节。 ### 银行家算法概述 银行家算法是一种动态资源分配策略,旨在通过事先检查资源分配的安全性来避免死锁。该算法基于这样的假设:每个进程在执行前会声明其所需的最大资源数量,系统根据这些信息决定是否将资源分配给请求它们的进程。 ### 数据结构 银行家算法涉及到以下几种关键的数据结构: - **Available**:一个长度为m的一维数组,表示每种资源的可用数量。 - **Max**:一个n×m的二维数组,定义了每个进程的最大需求。 - **Allocation**:一个n×m的二维数组,定义了当前分配给每个进程的资源数量。 - **Need**:一个n×m的二维数组,表示每个进程还需要多少资源才能完成任务(即Max - Allocation)。 ### 算法步骤 1. **初始化**:用户输入进程数量(n)、资源类型数量(m),以及每种资源的可用数量、每个进程的最大需求和当前已分配的资源数量。 2. **计算Need矩阵**:根据公式 `Need[i][j] = Max[i][j] - Allocation[i][j]` 计算每个进程还需要多少资源。 3. **安全性检查**:进行安全性检查,确定是否存在一种序列,使得所有进程都能获得所需资源并正常完成任务。这通常通过模拟资源分配过程来进行。 4. **资源分配**:如果存在安全序列,则按照安全序列分配资源;否则拒绝进程的资源请求。 ### C#实现细节 #### 类定义与初始化 定义了一个`Program`类,其中包含了上述提到的所有数据结构。初始化方法`Initialize()`负责读取用户输入并设置各个数组的值。 ```csharp public void Initialize() { Console.Write("请输入进程数量(n):"); n = Int32.Parse(Console.ReadLine()); Console.Write("请输入每种资源的数量(m):"); m = Int32.Parse(Console.ReadLine()); Available = new int[m]; Console.WriteLine("请输入每种资源的数量。(Available)"); ReadOneDimension(Available); Max = new int[n][]; for (int i = 0; i < n; i++) Max[i] = new int[m]; Allocation = new int[n][]; for (int i = 0; i < n; i++) Allocation[i] = new int[m]; Need = new int[n][]; for (int i = 0; i < n; i++) Need[i] = new int[m]; Console.WriteLine("请输入每个进程的最大需求矩阵。(Max)"); ReadMaxtrix(Max); Console.WriteLine("请输入每个进程当前已分配资源的矩阵。(Allocation)"); ReadMaxtrix(Allocation); // 因为 Need[i,j] = Max[i,j] - Allocation[i,j] for (int i = 0; i < n; i++) for (int j = 0; j < m; j++) Need[i][j] = Max[i][j] - Allocation[i][j]; } ``` #### 输入处理 提供了两个辅助方法`ReadOneDimension`和`ReadMaxtrix`来处理一维和二维数组的输入。 ```csharp public void ReadOneDimension(int[] oneDimension) { int i = 0; string temp; string[] array = new string[m]; while (i < m) { temp = Console.ReadLine(); array = temp.Split(new char[] { ' ', '\t', '\n' }, StringSplitOptions.RemoveEmptyEntries); foreach (string item in array) { oneDimension[i++] = Int32.Parse(item); if (i == m) break; } } } public void ReadMaxtrix(int[][] maxtrix) { int i = 0, j = 0; string temp; string[] array = new string[n * m]; while (i < n && j < m) { temp = Console.ReadLine(); array = temp.Split(new char[] { ' ', '\t', '\n' }, StringSplitOptions.RemoveEmptyEntries); foreach (string item in array) { maxtrix[i][j++] = Int32.Parse(item); if (j == m) { i++; j = 0; } } } } ``` 以上是基于给定代码片段对C#银行家算法的详细解释和实现。通过这种方式,可以有效地避免死锁,并确保系统的稳定运行。
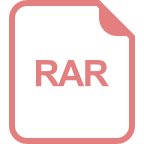
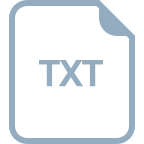
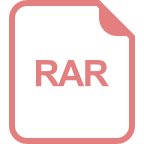
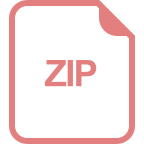
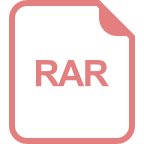
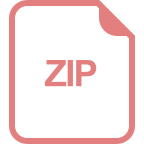
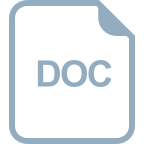
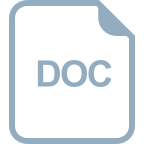
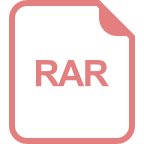
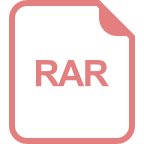
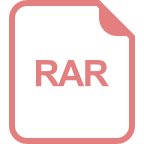
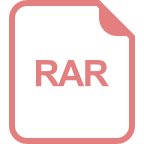
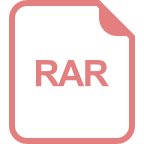
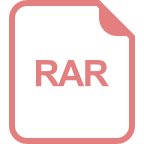
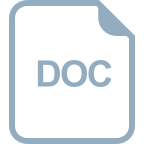
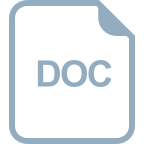
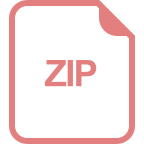
using System.Collections.Generic;
using System.Text;
namespace BankerAlgorithm
{
class Program
{
/*@ n: the number of processes
*@ m: the number of available resources of each type
*@ Available: A vector of length m indicates the numnber of available resources of each type
*@ Max: An n * m matrix defines the maximum demand of each process
*@ Allocation: An n * m matrix defines the number of resources of each type currently allocated to each process.
*@ Need: An n * m matrix indicates the remaining resource need of each process
*/
private int m;
private int n;
private int[] Available;
private int[][] Max;
private int[][] Allocation;
private int[][] Need;
private readonly char[] sprit ={ ' ', '\t', '\n' };
Program()
{
Initialize();
}
//get data from standard input
{
Console.Write("Please enter the number of processes (n) : ");
n=Int32.Parse(Console.ReadLine());
Console.Write("Please enter the number of available resources of each type (m) : ");
m = Int32.Parse(Console.ReadLine());
Available=new int[m];
Console.WriteLine("Enter the available resources of each type.(Available)");
ReadOneDimension(Available);
Max=new int[n][];
for (int i = 0; i < n; i++)
Max[i] = new int[m];
Allocation = new int[n][];
for (int i = 0; i < n; i++)
Allocation[i] = new int[m];
Need = new int[n][];
for (int i = 0; i < n; i++)
Need[i] = new int[m];
Console.WriteLine("Enter the matrix which indicates the maximum demand of each process.(Max)");
ReadMaxtrix(Max);
Console.WriteLine("Enter the matrix which indicates the number of resources of each type currently allocated to each process.(Allocation)");
ReadMaxtrix(Allocation);
//because Need[i,j] = Max[i,j] - Allocation[i,j]
剩余6页未读,继续阅读
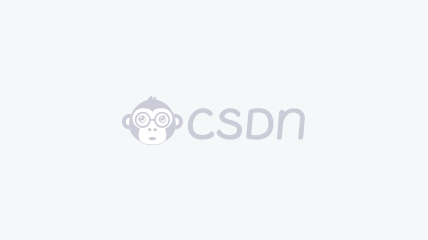
- 肥羊爱看书2012-11-26这个算法多我这个初学者来说很有用

- 粉丝: 0
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

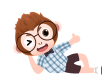
最新资源
- 基于Java的共享客栈管理系统+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于Java的菜匣子优选系统设计与实现+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于springboot+Vue的电影院购票系统2(Java毕业设计,附源码,部署教程).zip
- 基于springboot+Vue的电影院购票系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+Vue的电商应用系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于springboot+Vue的在线考试系统(Java毕业设计,附源码,部署教程).zip
- 基于SpringBoot+Vue的在线远程考试系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于JavaWeb的家居商城系统的设计与实现+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于SpringBoot+Vue的的游戏交易系统2(Java毕业设计,附源码,部署教程).zip
- 本地h264视频推流 Rtsp
- 基于SpringBoot+Vue的的中山社区医疗综合服务平台2(Java毕业设计,附源码,部署教程).zip
- 基于SpringBoot+Vue的的中山社区医疗综合服务平台(Java毕业设计,附源码,部署教程).zip
- 基于SpringBoot+Vue的在线课程管理系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于Java的汽车客运站管理系统的设计与实现+jsp(Java毕业设计,附源码,数据库,教程).zip
- PySpark数据处理技术大全
- 基于java的少儿编程网上报名系统+vue(Java毕业设计,附源码,数据库,教程).zip

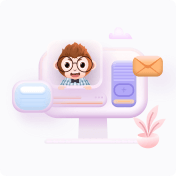
