# google-java-format
`google-java-format` is a program that reformats Java source code to comply with
[Google Java Style][].
[Google Java Style]: https://google.github.io/styleguide/javaguide.html
## Using the formatter
### from the command-line
[Download the formatter](https://github.com/google/google-java-format/releases)
and run it with:
```
java -jar /path/to/google-java-format-${GJF_VERSION?}-all-deps.jar <options> [files...]
```
The formatter can act on whole files, on limited lines (`--lines`), on specific
offsets (`--offset`), passing through to standard-out (default) or altered
in-place (`--replace`).
To reformat changed lines in a specific patch, use
[`google-java-format-diff.py`](https://github.com/google/google-java-format/blob/master/scripts/google-java-format-diff.py).
***Note:*** *There is no configurability as to the formatter's algorithm for
formatting. This is a deliberate design decision to unify our code formatting on
a single format.*
### IntelliJ, Android Studio, and other JetBrains IDEs
A
[google-java-format IntelliJ plugin](https://plugins.jetbrains.com/plugin/8527)
is available from the plugin repository. To install it, go to your IDE's
settings and select the `Plugins` category. Click the `Marketplace` tab, search
for the `google-java-format` plugin, and click the `Install` button.
The plugin will be disabled by default. To enable it in the current project, go
to `File→Settings...→google-java-format Settings` (or `IntelliJ
IDEA→Preferences...→Other Settings→google-java-format Settings` on macOS) and
check the `Enable google-java-format` checkbox. (A notification will be
presented when you first open a project offering to do this for you.)
To enable it by default in new projects, use `File→Other Settings→Default
Settings...`.
When enabled, it will replace the normal `Reformat Code` and `Optimize Imports`
actions.
#### IntelliJ JRE Config
The google-java-format plugin uses some internal classes that aren't available
without extra configuration. To use the plugin, go to `Help→Edit Custom VM
Options...` and paste in these lines:
```
--add-exports=jdk.compiler/com.sun.tools.javac.api=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.code=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.file=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.parser=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.tree=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.util=ALL-UNNAMED
```
Once you've done that, restart the IDE.
### Eclipse
The latest version of the `google-java-format` Eclipse plugin can be downloaded
from the [releases page](https://github.com/google/google-java-format/releases).
Drop it into the Eclipse
[drop-ins folder](http://help.eclipse.org/neon/index.jsp?topic=%2Forg.eclipse.platform.doc.isv%2Freference%2Fmisc%2Fp2_dropins_format.html)
to activate the plugin.
The plugin adds a `google-java-format` formatter implementation that can be
configured in `Window > Preferences > Java > Code Style > Formatter > Formatter
Implementation`.
### Third-party integrations
* Gradle plugins
* [spotless](https://github.com/diffplug/spotless/tree/main/plugin-gradle#google-java-format)
* [sherter/google-java-format-gradle-plugin](https://github.com/sherter/google-java-format-gradle-plugin)
* Apache Maven plugins
* [spotless](https://github.com/diffplug/spotless/tree/main/plugin-maven#google-java-format)
* [spotify/fmt-maven-plugin](https://github.com/spotify/fmt-maven-plugin)
* [talios/googleformatter-maven-plugin](https://github.com/talios/googleformatter-maven-plugin)
* [Cosium/maven-git-code-format](https://github.com/Cosium/maven-git-code-format):
A maven plugin that automatically deploys google-java-format as a
pre-commit git hook.
* SBT plugins
* [sbt/sbt-java-formatter](https://github.com/sbt/sbt-java-formatter)
* [Github Actions](https://github.com/features/actions)
* [googlejavaformat-action](https://github.com/axel-op/googlejavaformat-action):
Automatically format your Java files when you push on github
### as a library
The formatter can be used in software which generates java to output more
legible java code. Just include the library in your maven/gradle/etc.
configuration.
`google-java-format` uses internal javac APIs for parsing Java source. The
following JVM flags are required when running on JDK 16 and newer, due to
[JEP 396: Strongly Encapsulate JDK Internals by Default](https://openjdk.java.net/jeps/396):
```
--add-exports=jdk.compiler/com.sun.tools.javac.api=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.code=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.file=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.parser=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.tree=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.util=ALL-UNNAMED
```
#### Maven
```xml
<dependency>
<groupId>com.google.googlejavaformat</groupId>
<artifactId>google-java-format</artifactId>
<version>${google-java-format.version}</version>
</dependency>
```
#### Gradle
```groovy
dependencies {
implementation 'com.google.googlejavaformat:google-java-format:$googleJavaFormatVersion'
}
```
You can then use the formatter through the `formatSource` methods. E.g.
```java
String formattedSource = new Formatter().formatSource(sourceString);
```
or
```java
CharSource source = ...
CharSink output = ...
new Formatter().formatSource(source, output);
```
Your starting point should be the instance methods of
`com.google.googlejavaformat.java.Formatter`.
## Building from source
```
mvn install
```
## Contributing
Please see [the contributors guide](CONTRIBUTING.md) for details.
## License
```text
Copyright 2015 Google Inc.
Licensed under the Apache License, Version 2.0 (the "License"); you may not
use this file except in compliance with the License. You may obtain a copy of
the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
License for the specific language governing permissions and limitations under
the License.
```
没有合适的资源?快使用搜索试试~ 我知道了~
谷歌java格式-重新格式化 Java 源代码以符合 Google Java 风格
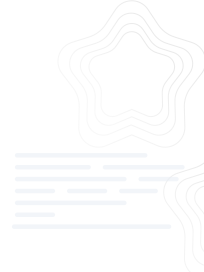
共488个文件
input:191个
output:190个
java:78个

需积分: 5 1 下载量 83 浏览量
2023-10-25
18:32:51
上传
评论
收藏 431KB ZIP 举报
温馨提示
谷歌java格式 google-java-format是一个重新格式化 Java 源代码以符合 Google Java Style的程序。 使用格式化程序 从命令行 下载格式化程序 并运行它: java -jar /path/to/google-java-format-${GJF_VERSION?}-all-deps.jar <options> [files...] 格式化程序可以作用于整个文件、有限行 ( --lines)、特定偏移量 ( --offset)、传递到标准输出(默认)或就地更改 ( --replace)。 要重新格式化特定补丁中更改的行,请使用 google-java-format-diff.py. 注意: 格式化程序的格式化算法不可配置。这是一个经过深思熟虑的设计决定,旨在将我们的代码格式统一为单一格式。
资源推荐
资源详情
资源评论
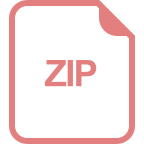
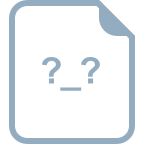
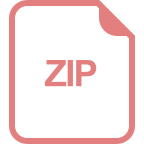
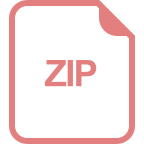
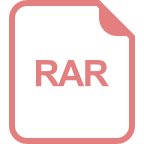
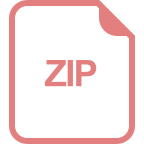
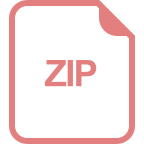
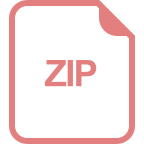
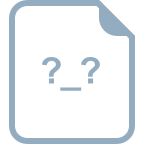
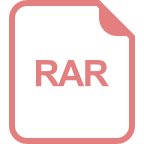
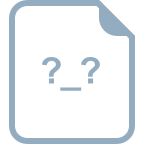
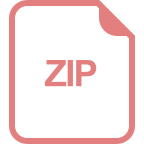
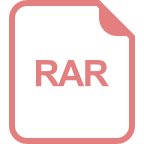
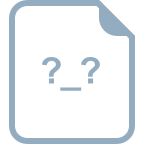
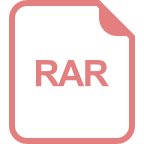
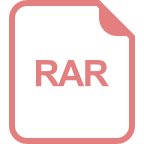
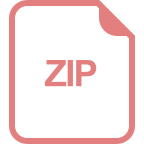
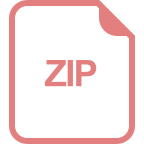
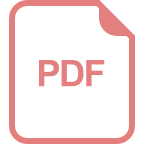
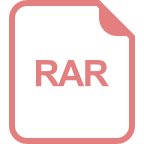
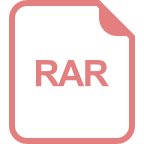
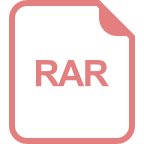
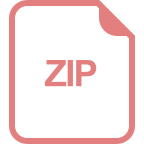
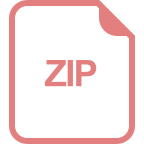
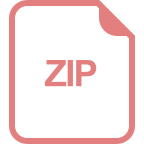
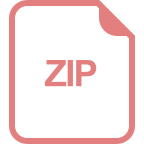
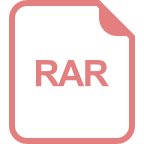
收起资源包目录

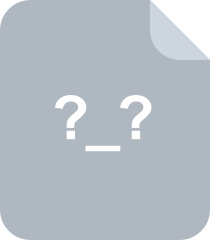
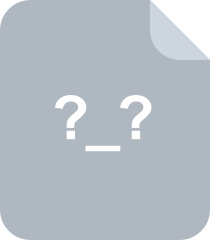
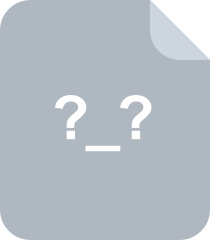
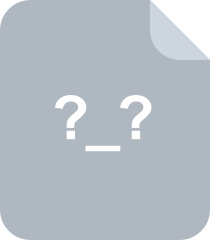
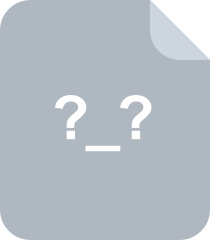
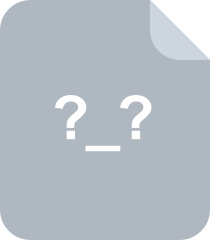
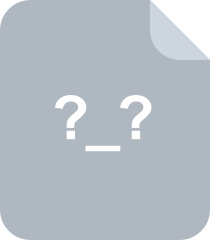
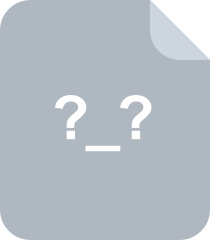
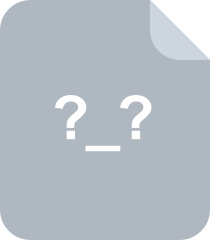
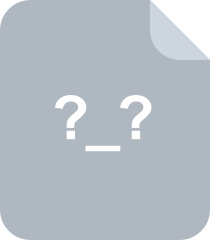
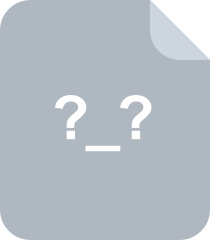
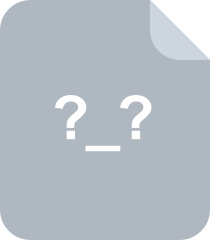
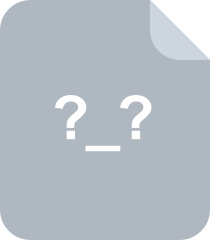
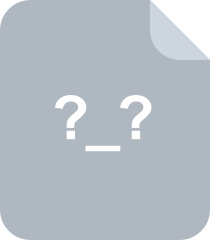
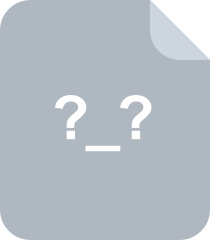
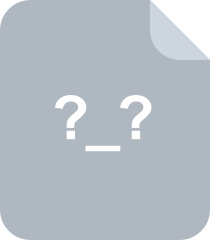
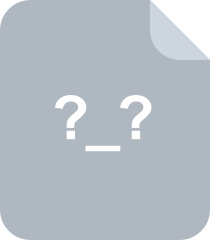
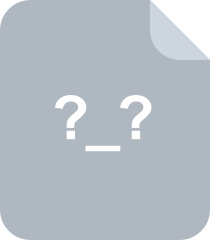
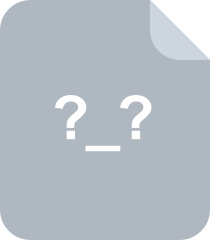
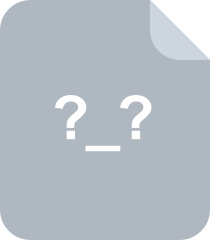
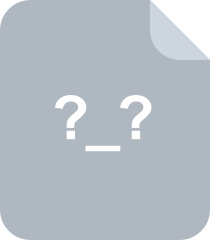
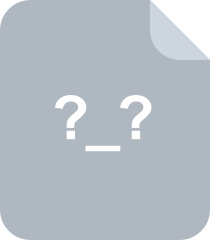
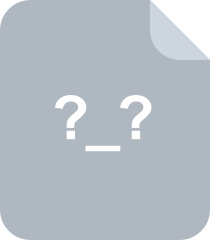
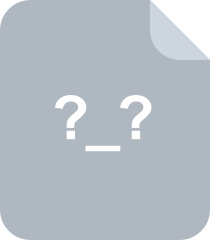
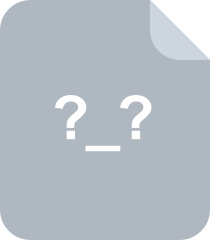
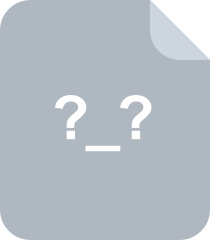
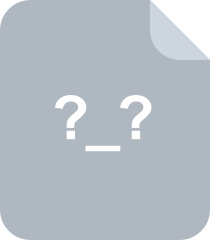
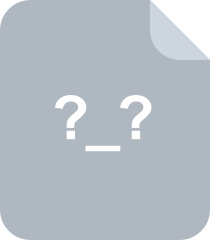
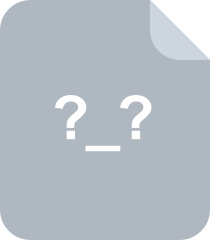
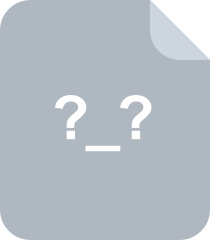
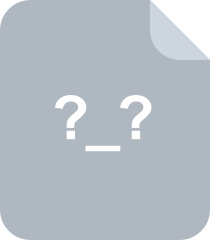
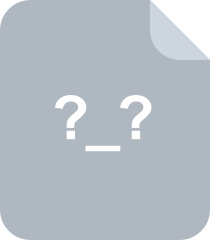
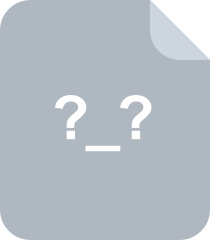
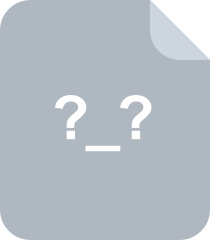
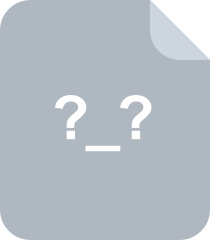
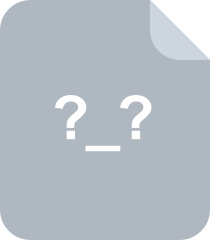
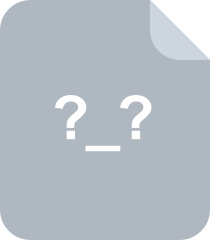
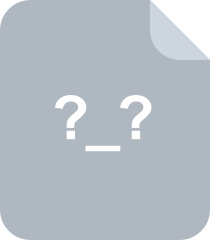
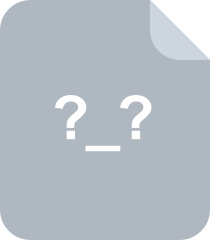
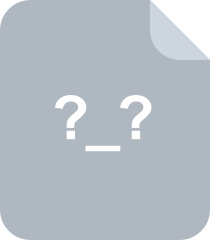
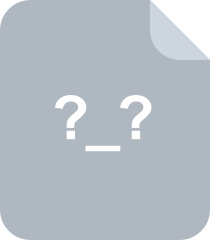
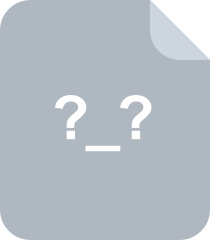
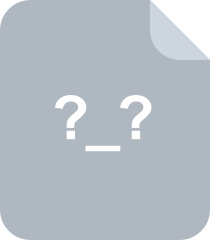
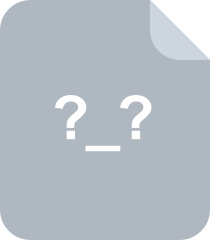
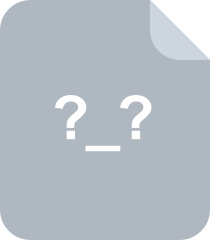
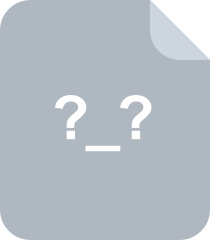
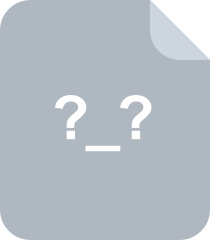
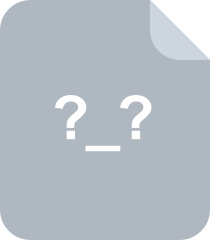
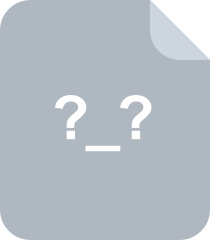
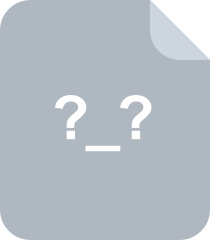
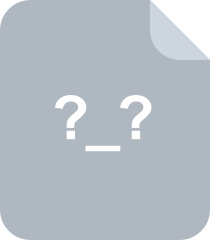
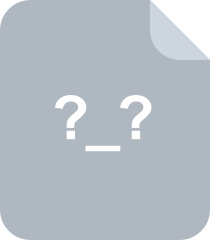
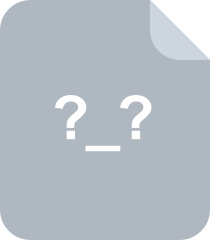
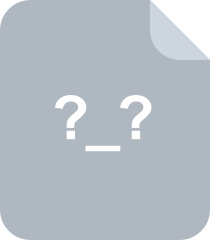
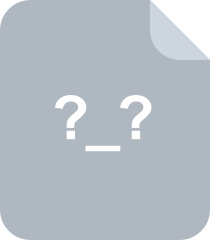
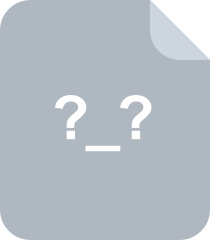
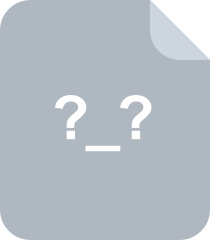
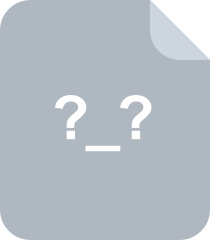
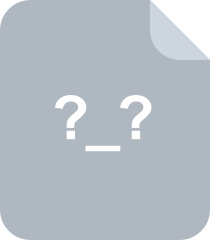
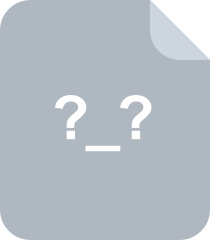
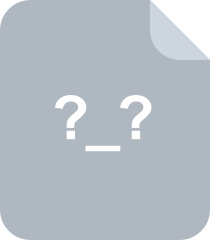
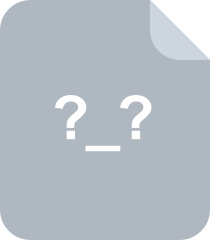
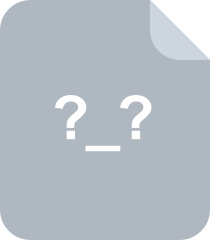
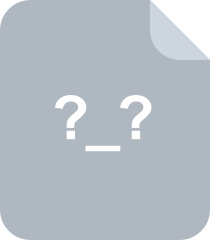
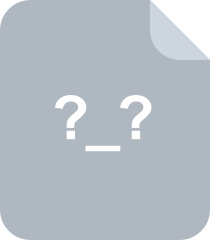
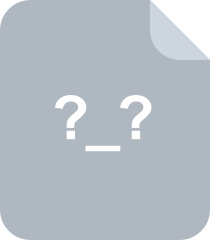
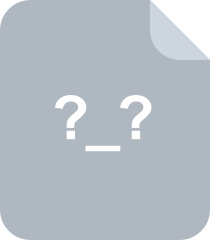
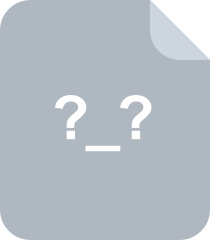
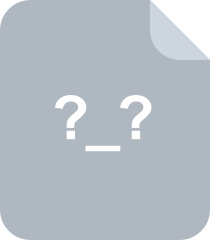
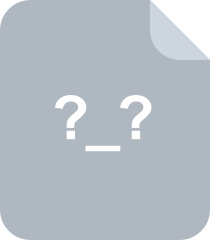
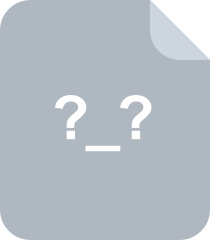
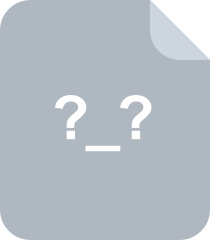
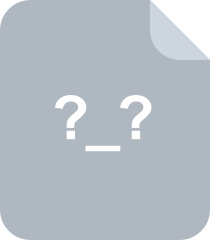
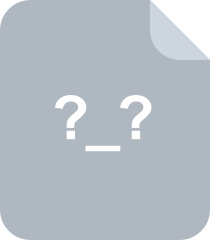
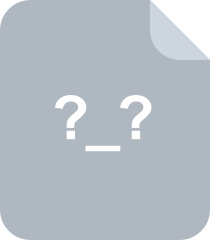
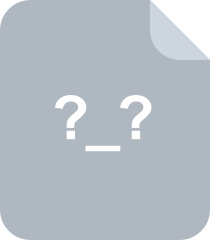
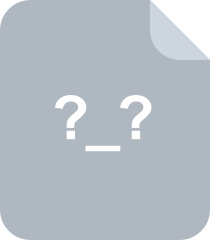
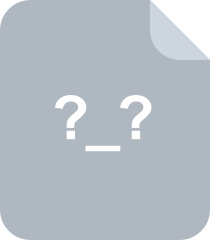
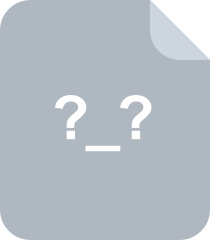
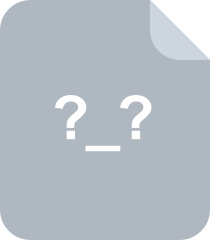
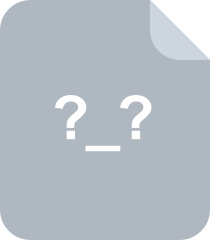
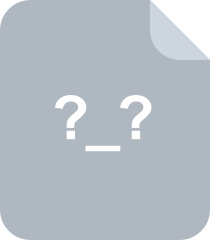
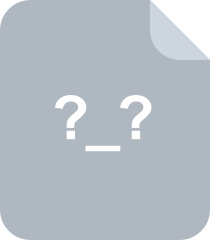
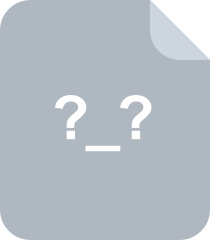
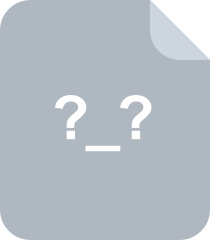
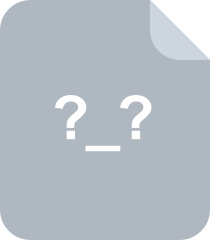
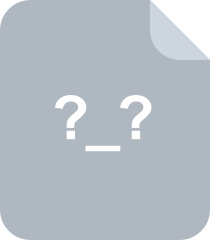
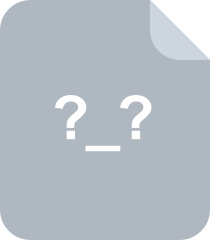
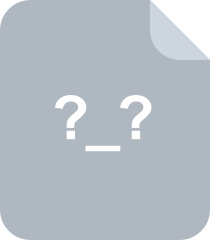
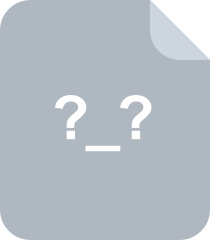
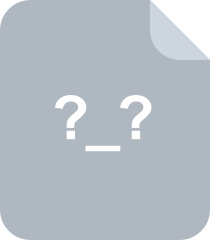
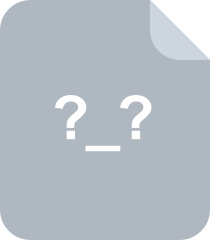
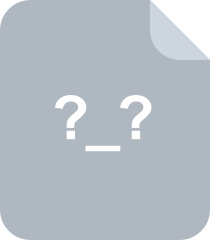
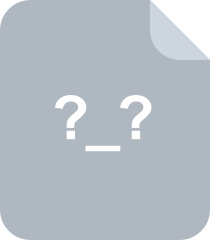
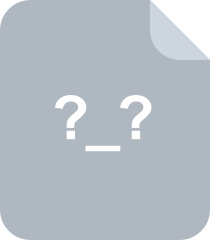
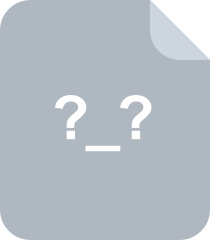
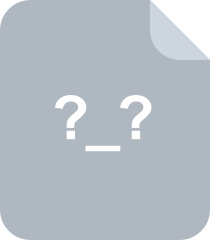
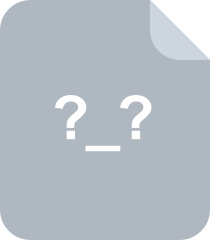
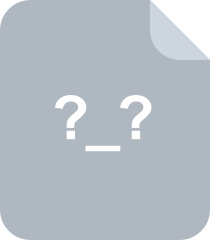
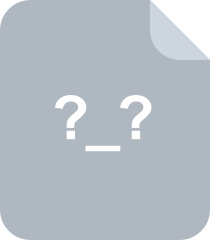
共 488 条
- 1
- 2
- 3
- 4
- 5
资源评论
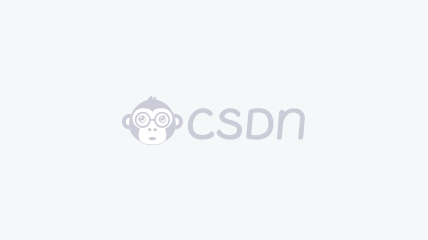

Web面试那些事儿
- 粉丝: 5813
- 资源: 101
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

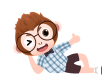
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


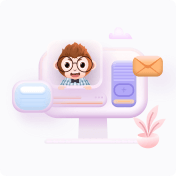
安全验证
文档复制为VIP权益,开通VIP直接复制
