package com.nicolas.test;
import java.io.Reader;
import java.io.IOException;
import java.util.ArrayList;
import com.ibatis.common.resources.Resources;
import com.ibatis.sqlmap.client.SqlMapClient;
import com.ibatis.sqlmap.client.SqlMapClientBuilder;
import com.nicolas.dao.impl.StudentDaoImpl;
import com.nicolas.pojo.StudentDto;
public class MainTest {
public StudentDaoImpl impl = new StudentDaoImpl();
public StudentDto info = new StudentDto();
public static SqlMapClient sqlmapclient = null;
static {
try {
// 读取xml文件
Reader reader = Resources
.getResourceAsReader("com/nicolas/config/sqlmapconfig.xml");
sqlmapclient = SqlMapClientBuilder.buildSqlMapClient(reader);
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
MainTest stu = new MainTest();
System.out
.println("------------------------------- start ------------------------------");
// 以下为各种方法测试
// 添加student表的数据
// stu.addStudent_test();
// 删除student表的数据
// stu.delStudent_test();
// 删除student表的指定ID数据
// stu.delStudentByID_test();
// 更新student表的数据
// stu.updataStudent_test();
// 查询student表的所有数据
stu.selectStudent_test();
// 查询student表的所有数据
// stu.selectStudentByID_test();
System.out
.println("------------------------------- end ------------------------------");
}
// 添加student表的数据
public void addStudent_test() {
// 把要插入的数据填入info对象中
info.setId(5);
info.setName("zh2208");
info.setSex("男");
info.setAge(24);
info.setAddress("上海");
impl.addStudent(sqlmapclient, info);
}// 删除student表的数据
public void delStudent_test() {
impl.delStudent(sqlmapclient);
}
// 删除student表的指定ID数据
public void delStudentByID_test() {
// 指定ID
info.setId(1);
impl.delStudentByID(sqlmapclient, info);
}
// 更新student表的数据
public void updataStudent_test() {
// 把要更新的数据填入info对象中
info.setId(6);
info.setName("zh2208up");
info.setSex("男");
info.setAge(20);
info.setAddress("上海up");
impl.updataStudent(sqlmapclient, info);
}
// 查询student表的所有数据
public void selectStudent_test() {
StudentDto stu_dto = new StudentDto();
// 检索结果保存到list中
ArrayList resultList = impl.selectStudent(sqlmapclient);
for (int i = 0; i < resultList.size(); i++) {
stu_dto = (StudentDto) resultList.get(i);
// 打印对象中的信息
show(stu_dto);
}
}
// 查询student表的指定ID数据
public void selectStudentByID_test() {
StudentDto stu_dto = new StudentDto();
info.setId(1);
stu_dto = impl.selectStudentByID(sqlmapclient, info);
if (stu_dto != null) {
show(stu_dto);
} else {
System.out.println("no data!!!!");
}
}
// 打印查询结果
public void show(StudentDto stu_dto) {
System.out.print("学生ID :" + stu_dto.getId() + " ; ");
System.out.print("学生姓名 :" + stu_dto.getName() + " ; ");
System.out.print("学生性别 :" + stu_dto.getSex() + " ; ");
System.out.print("学生年龄 :" + stu_dto.getAge() + " ; ");
System.out.print("学生地址 :" + stu_dto.getAddress());
System.out.println();
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
核心提示:实例实现了6个基本功能: 1.向数据库student表插入一条数据 2.删除student表的所有数据 3.删除student表指定ID的数据 4.更新student表指定ID的数据 5.查询student表的所有数据 6.查询student表的指定ID数据 数据库为SQL Server 2005 此资源是根据前辈们写的文档做的。http://blog.csdn.net/jimmy292/article/details/5698618
资源推荐
资源详情
资源评论
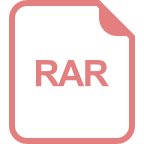
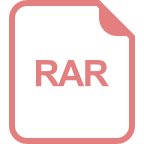
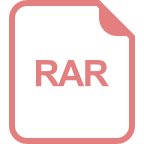
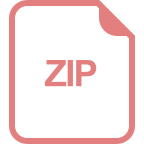
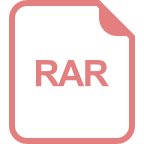
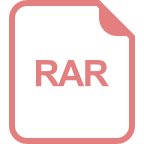
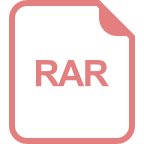
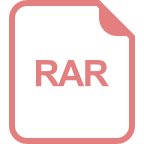
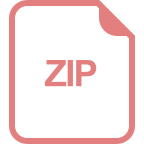
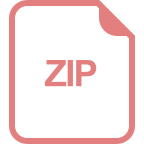
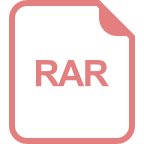
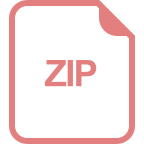
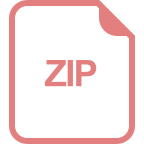
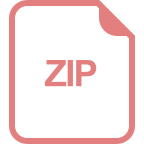
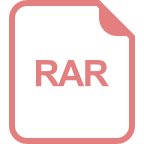
收起资源包目录


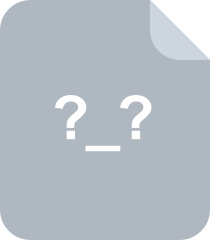

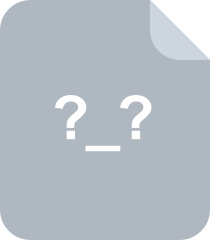
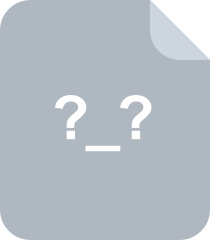


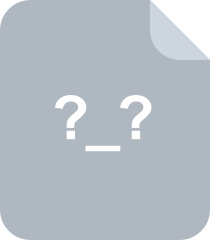

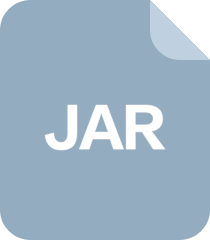
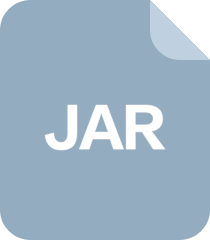




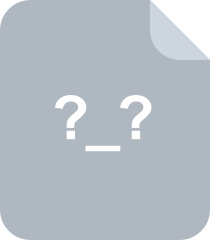

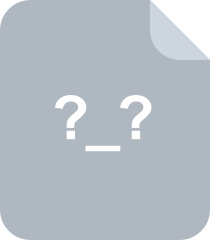

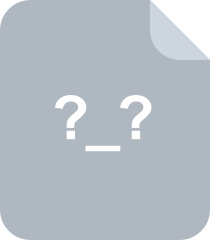

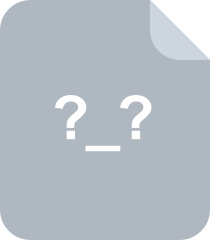

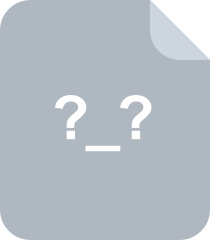
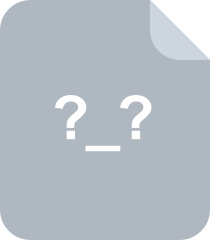
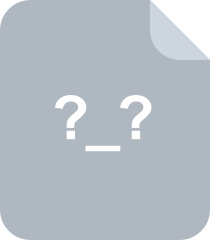

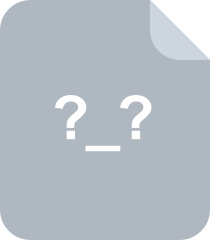
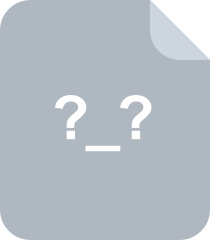

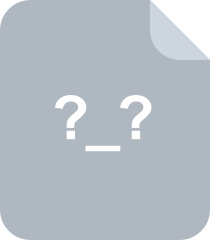






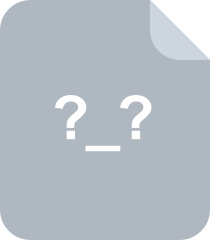
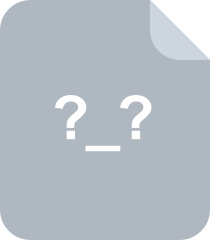

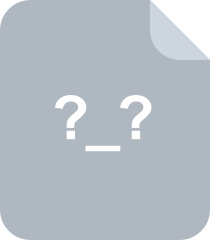

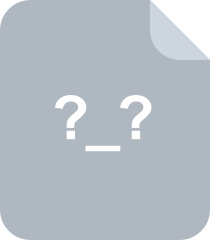

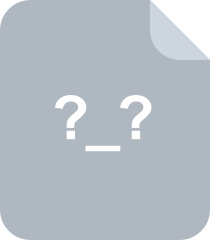
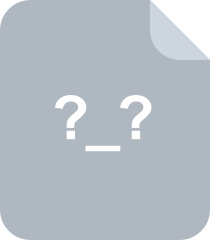
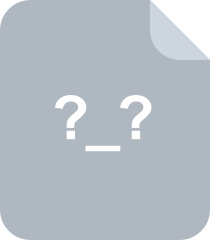

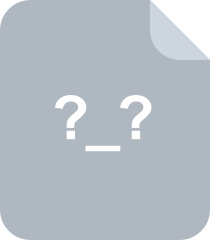
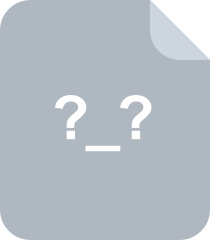
共 25 条
- 1
资源评论
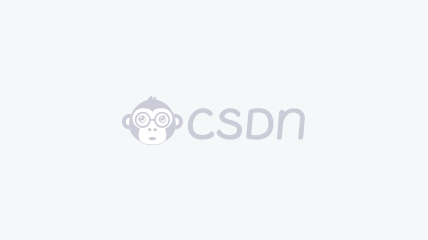
- dinghao3252013-10-19很实用哦!
- sodalee_2012-05-28不能运行,基本的包得自己加才可以
- Fane2013-09-04下载下来 修改一下 就可以了 还不错 对于初学者很受用

木木子兄弟
- 粉丝: 0
- 资源: 38
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

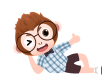
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


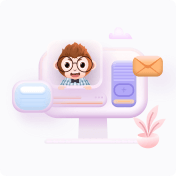
安全验证
文档复制为VIP权益,开通VIP直接复制
