import datetime
import json
import os
from django.core.paginator import Paginator
from django.db.models import Count
from django.http import HttpResponseRedirect, HttpResponse, HttpResponseForbidden, JsonResponse
from django.shortcuts import render
from user.models import User
from .predict import main
from .models import *
workdir = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
dict_city = {
'京': '北京', '津': '天津', '沪': '上海',
'渝': '重庆', '宁': '宁夏', '新': '疆维', '西藏': '藏', '桂': '广西',
'蒙': '内蒙古', '辽': '辽宁', '吉': '吉林', '黑': '黑龙江',
'冀': '河北', '晋': '山西', '皖': '安徽',
'苏': '江苏', '浙': '浙江', '闽': '福建', '赣': '江西',
'鲁': '山东', '湘': '湖南', '粤': '广东',
'豫': '河南', '鄂': '湖北', '云': '云南', '陕': '陕西',
'琼': '海南', '川': '四川', '贵': '贵州', '甘': '甘肃',
'青': '青海'
}
def login(req):
"""
跳转登录
:param req:
:return:
"""
return render(req, 'login.html')
def register(req):
"""
跳转注册
:param req:
:return:
"""
return render(req, 'register.html')
def index(request):
"""
跳转首页
:param req:
:return:
"""
username = request.session.get('username', 'admin')
role = int(request.session.get('role', 3))
user_id = request.session.get('user_id', 1)
total_user = len(User.objects.all())
total_car = len(Car.objects.all())
date = datetime.datetime.today()
month = date.month
year = date.year
return render(request, 'index.html', locals())
def login_out(req):
"""
注销登录
:param req:
:return:
"""
del req.session['username']
return HttpResponseRedirect('/')
def personal(req):
username = req.session['username']
role_id = req.session['role']
user = User.objects.filter(name=username).first()
return render(req, 'personal.html', locals())
def get_data(request):
"""
获取列表信息 | 模糊查询
:param request:
:return:
"""
keyword = request.GET.get('name')
page = request.GET.get("page", '')
limit = request.GET.get("limit", '')
role_id = request.GET.get('position', '')
response_data = {}
response_data['code'] = 0
response_data['msg'] = ''
data = []
if keyword is None:
results_obj = Car.objects.all()
else:
results_obj = Car.objects.filter(name__contains=keyword).all()
paginator = Paginator(results_obj, limit)
results = paginator.page(page)
if results:
for user in results:
record = {
"id": user.id,
"name": user.name,
"license_plate": user.license_plate,
"province": user.province,
"color": user.color,
'create_time': user.create_time.strftime('%Y-%m-%d %H:%m:%S'),
"owner": user.owner,
}
data.append(record)
response_data['count'] = len(results_obj)
response_data['data'] = data
return JsonResponse(response_data)
def data(request):
"""
跳转页面
"""
username = request.session['username']
role = int(request.session['role'])
user_id = request.session['user_id']
return render(request, 'pics.html', locals())
def detection(request):
"""
跳转页面
"""
username = request.session['username']
role = int(request.session['role'])
user_id = request.session['user_id']
results = Car.objects.all()
image_list = []
for i in results:
record= {
'id':i.id,
'name':i.name
}
image_list.append(record)
return render(request, 'detection.html', locals())
def edit_data(request):
"""
修改信息
"""
response_data = {}
user_id = request.POST.get('id')
username = request.POST.get('username')
phone = request.POST.get('phone')
User.objects.filter(id=user_id).update(
name=username,
phone=phone)
response_data['msg'] = 'success'
return JsonResponse(response_data, status=201)
def del_data(request):
"""
删除信息
"""
user_id = request.POST.get('id')
result = Car.objects.filter(id=user_id).first()
try:
if not result:
response_data = {'error': '删除失败!', 'message': '找不到id为%s' % user_id}
return JsonResponse(response_data, status=403)
result.delete()
response_data = {'message': '删除成功!'}
return JsonResponse(response_data, status=201)
except Exception as e:
response_data = {'message': '删除失败!'}
return JsonResponse(response_data, status=403)
# 保存缓存文件
def save_file(file):
if file is not None:
file_name = os.path.join(workdir, 'static', 'uploadImg', file.name)
with open(file_name, 'wb')as f:
# chunks()每次读取数据默认 我64k
for chunk in file.chunks():
f.write(chunk)
f.close()
return file_name
else:
return None
def predict(request):
image = request.FILES.get('image')
file_name = save_file(image)
file_path = os.path.join(workdir, 'static', 'uploadImg', image.name)
# try:
number, score = main(file_path)
shengfen = number[0]
length = len(number)
if length == 7:
color='蓝牌'
else:
color='绿牌'
Car.objects.create(name=image.name,
license_plate=number,
province=dict_city[shengfen],
color=color,
owner=request.session.get('username', 'admin'),
)
return JsonResponse({"number": number, 'score': score, 'error': 0})
# except Exception as e:
# print(e)
# return JsonResponse({"error":403})
def get_result(request,pic_id):
result = Car.objects.filter(id=pic_id).first()
license_plate = result.license_plate
province = result.province
return JsonResponse({'province': province,'license_plate':license_plate})
def map(request):
country = ['台湾', '云南', '香港', '上海', '浙江', '内蒙古', '福建', '甘肃', '广东', '广西', '山东', '陕西', '河南', '北京', '四川', '天津', '辽宁', '宁夏', '澳门', '湖北', '湖南', '江苏', '吉林', '黑龙江', '贵州', '青海', '山西', '河北', '重庆', '海南', '安徽', '西藏', '江西', '新疆']
dict_value = dict.fromkeys(country,0)
results = Car.objects.values('province').annotate(myCount=Count('province')) # 返回查询集合
county_total_list = []
for i in results:
dict_value[i['province']]=i['myCount']
for key,value in dict_value.items():
county_total_list.append({'name':key,'value':value})
username = request.session.get('username', 'admin')
role = int(request.session.get('role', 3))
user_id = request.session.get('user_id', 1)
return render(request, 'map.html', locals())
def analysis(request):
results = Car.objects.values('province').annotate(myCount=Count('province')) # 返回查询集合
country = []
count = []
for i in results:
country.append(i['province'])
count.append(i['myCount'])
result1 = Car.objects.values('color').annotate(myCount=Count('color')) # 返回查询集合
values = []
for i in result1:
values.append({'name':i['color'],'value':i['myCount']})
username = request.session.get('username', 'admin')
role = int(request.session.get('role', 3))
user_id = request.session.get('user_id', 1)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Python 完整项目,Python 毕业设计,包含:项目源码、数据库脚本、软件工具等,前后端代码都在里面。可用于 Python 毕业设计,Python 课程设计,Python 期末大作业。 该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。 项目都经过严格调试,确保可以运行!可以放心下载, 1. 技术组成 前端: html 后台框架:Python 开发环境:pycharm 数据库可视化工具:使用 Navicat 2 部署 用 pycharm 打开,使用 pip 下载相关依赖,然后 run 就 ok 了,如果不会部署的话,可以联系我
资源推荐
资源详情
资源评论
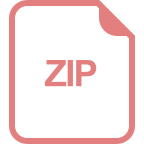
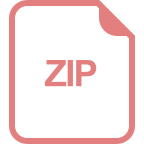
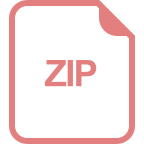
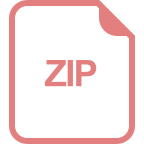
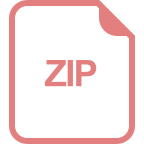
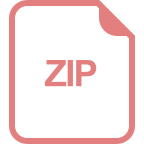
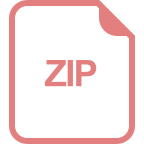
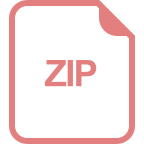
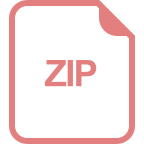
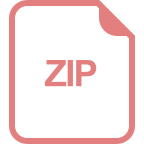
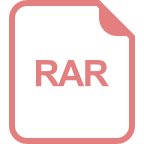
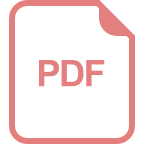
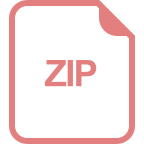
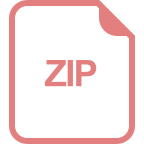
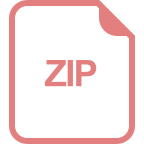
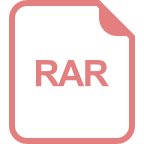
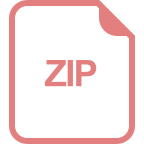
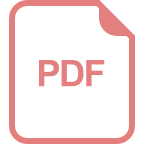
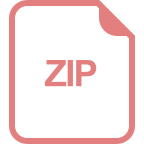
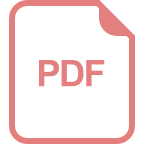
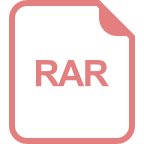
收起资源包目录

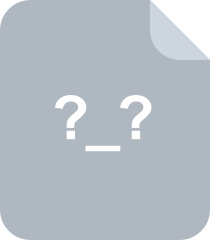
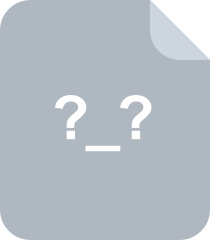
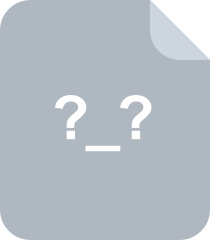
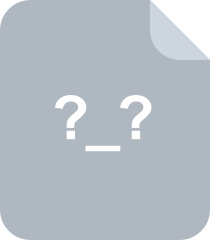
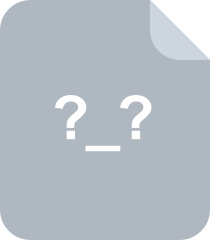
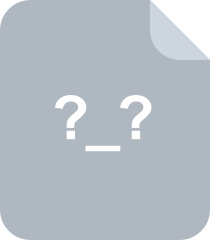
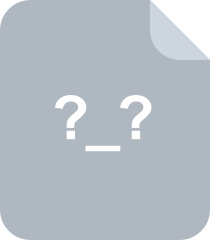
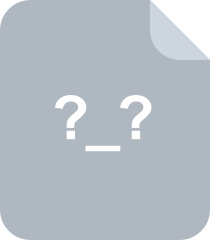
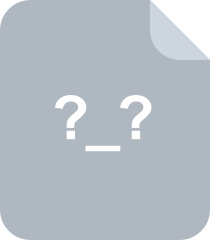
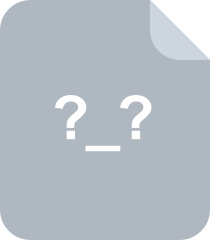
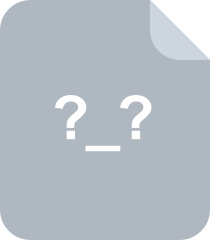
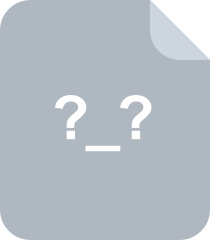
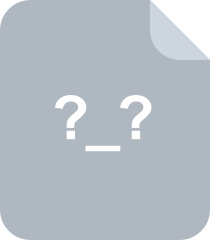
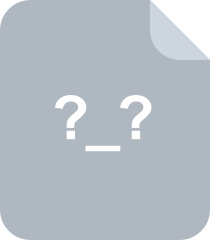
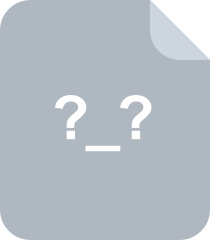
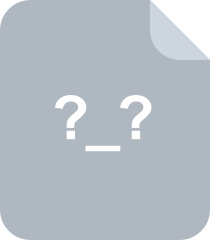
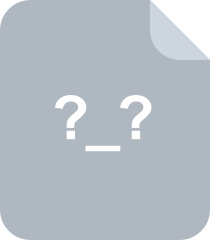
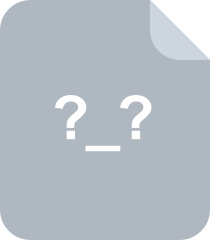
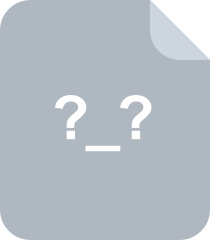
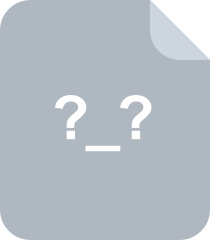
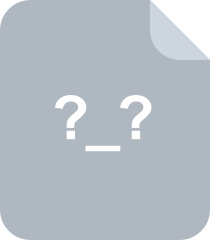
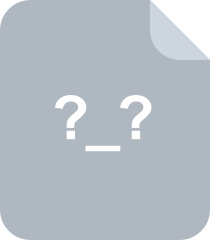
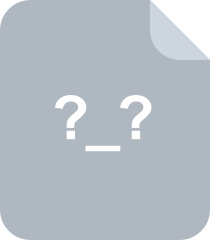
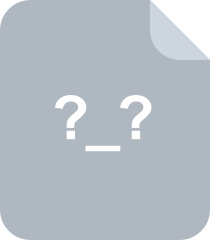
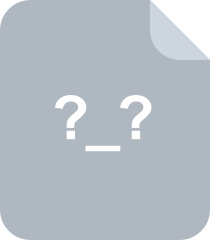
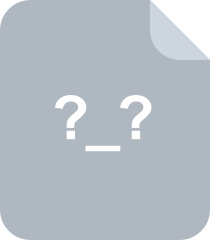
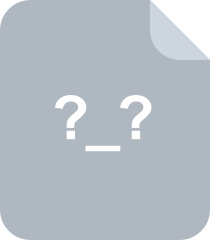
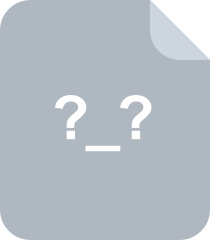
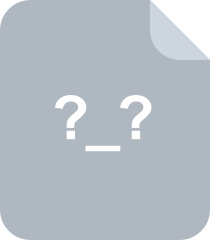
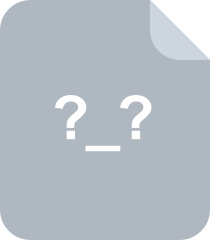
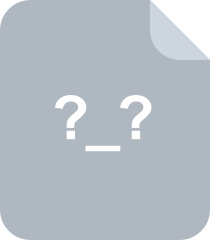
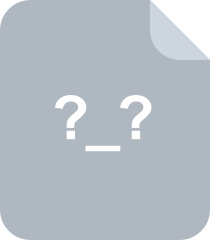
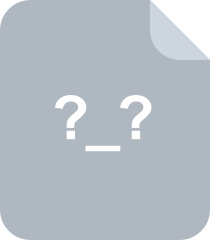
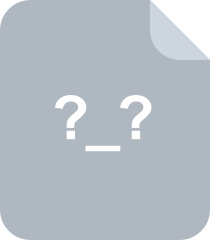
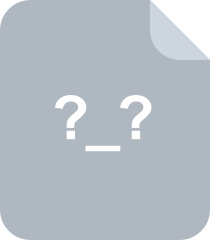
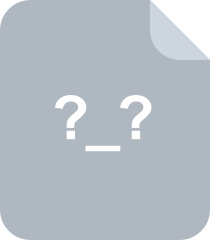
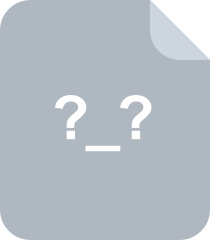
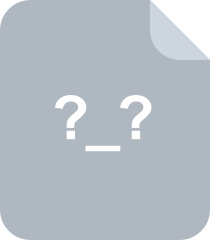
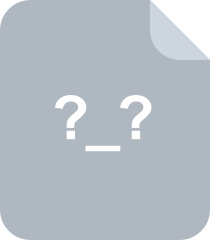
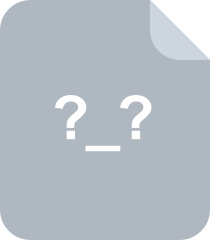
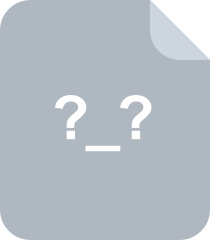
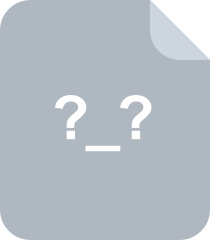
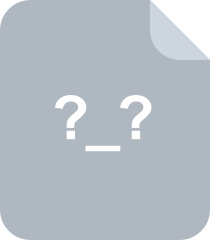
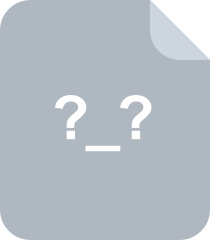
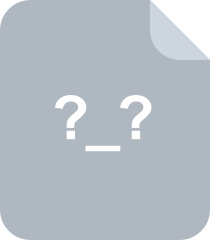
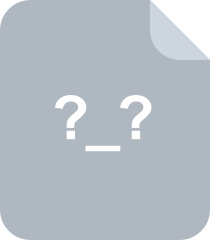
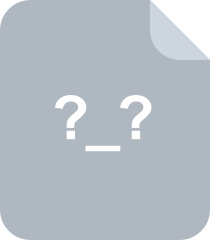
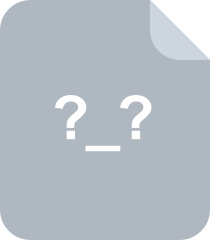
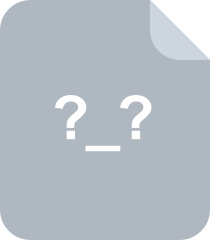
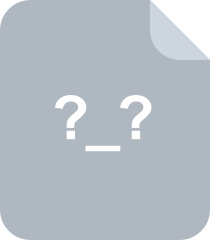
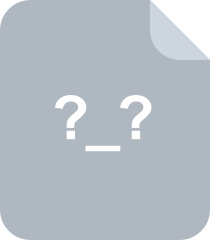
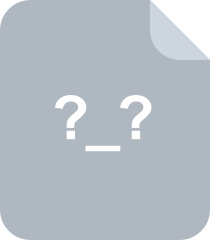
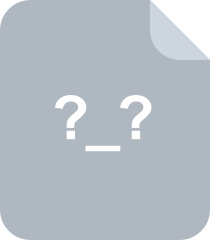
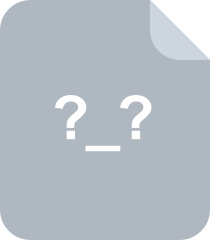
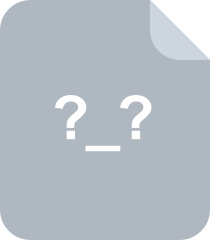
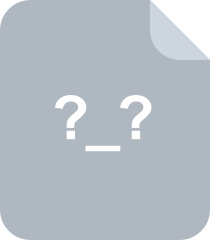
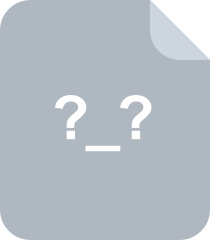
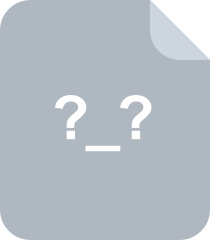
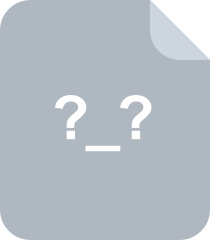
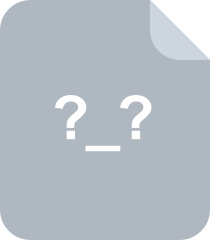
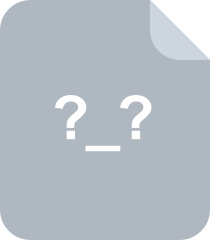
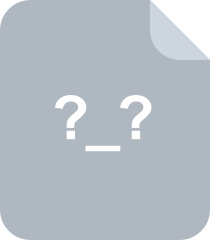
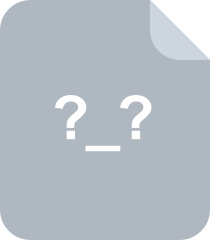
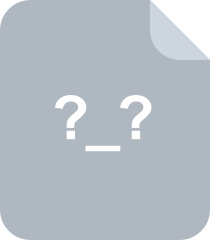
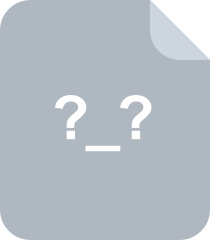
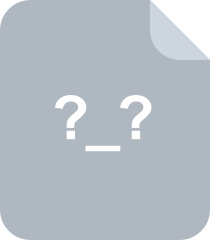
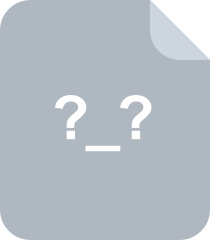
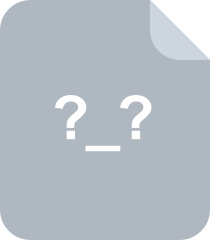
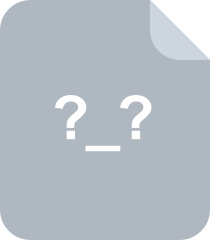
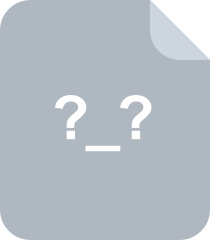
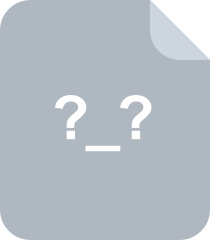
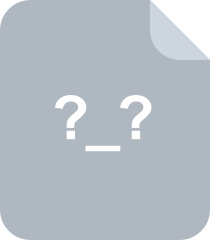
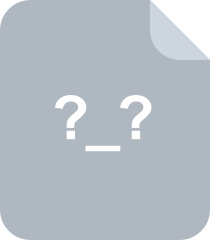
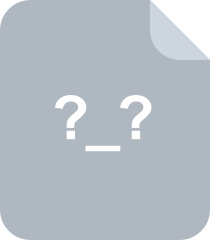
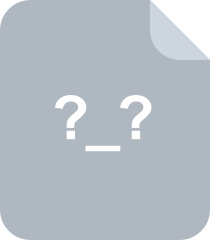
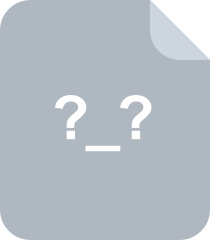
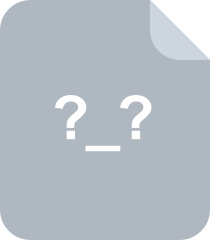
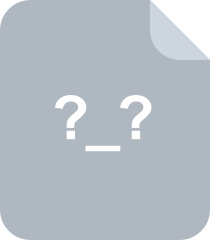
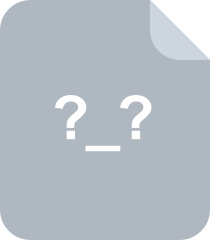
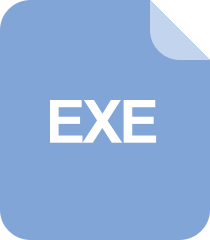
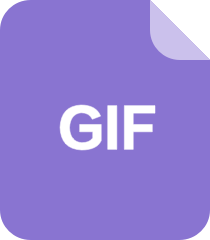
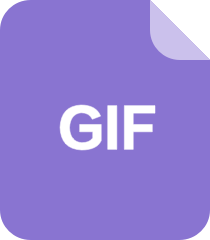
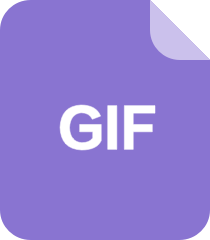
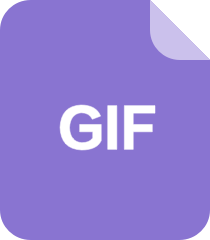
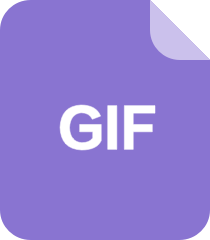
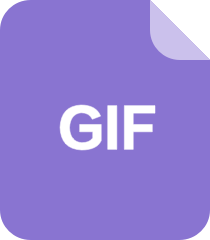
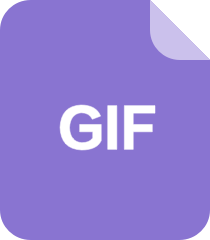
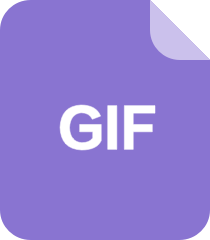
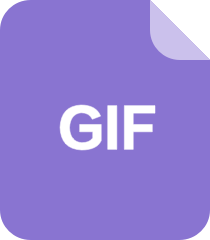
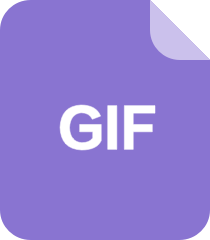
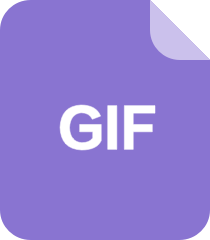
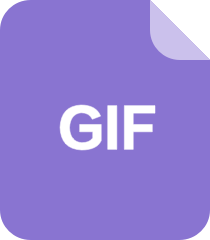
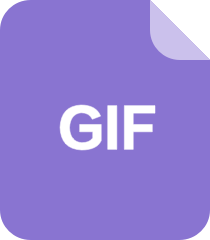
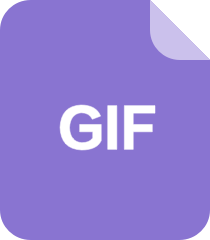
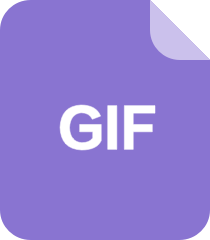
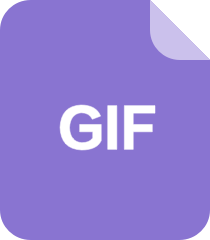
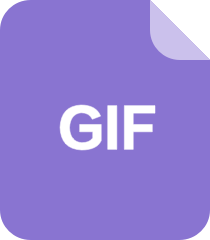
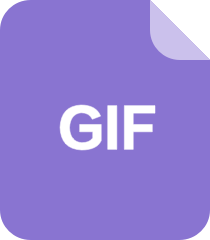
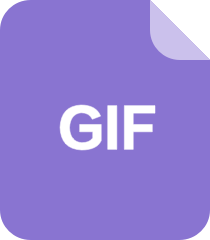
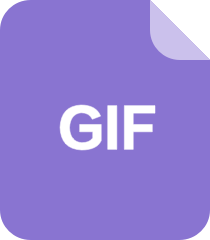
共 858 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
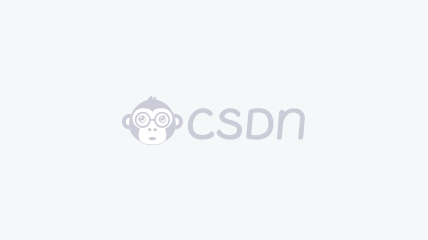

程序员徐师兄
- 粉丝: 1163
- 资源: 2379
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

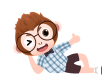
安全验证
文档复制为VIP权益,开通VIP直接复制
