C_pointer_chtp4_07.pdf
### C Pointers: A Comprehensive Guide #### Introduction (7.1) Pointers are one of the most powerful features of the C programming language, enabling programmers to simulate call-by-reference and create dynamic data structures that can grow and shrink during program execution. Examples of such structures include linked lists, queues, stacks, and trees. #### Pointer Variable Definitions and Initialization (7.2) A pointer is a variable whose value is a memory address. Unlike regular variables that directly contain specific values, pointers contain the address of another variable that contains a specific value. This indirect reference to a value through a pointer is called indirection. Before using a pointer, it must be defined. The definition: ```c int *countPtr, count; ``` specifies that `countPtr` is of type `int*`, which means it is a pointer to an integer. It is read as "countPtr is a pointer to int" or "countPtr points to an object of type int." Additionally, the variable `count` is defined to be an `int`, not a pointer to an int. #### Understanding Pointers and Pointer Operators To effectively use pointers in C, you need to understand two key concepts: pointers themselves and pointer operators. There are two primary pointer operators: 1. **The Address-of Operator (&):** This operator returns the memory address of its operand. For example: ```c int x = 10; int *ptr = &x; // ptr now holds the address of x ``` 2. **The Indirection Operator (*):** Also known as the dereference operator, this operator retrieves the value stored at the memory address specified by its operand. For example: ```c int x = 10; int *ptr = &x; int y = *ptr; // y is now 10, the value stored at the address held by ptr ``` #### Using Pointers to Pass Arguments to Functions by Reference One of the most significant uses of pointers in C is to pass arguments to functions by reference. This technique allows functions to modify the actual parameters passed to them. For example: ```c void swap(int *a, int *b) { int temp = *a; *a = *b; *b = temp; } int main() { int x = 10, y = 20; swap(&x, &y); printf("x: %d, y: %d\n", x, y); // Output: x: 20, y: 10 } ``` In this example, the `swap` function takes two pointers to integers as arguments and swaps the values they point to. By passing the addresses of `x` and `y` to `swap`, we can change their values without returning anything from the function. #### Pointers, Arrays, and Strings Pointers have a close relationship with arrays and strings. In fact, many operations on arrays and strings can be performed more efficiently using pointers. Here are some key points: 1. **Arrays and Pointers:** An array name, when used without an index, decays into a pointer to its first element. For example: ```c int arr[5] = {1, 2, 3, 4, 5}; int *ptr = arr; // ptr now points to the first element of arr ``` 2. **Strings and Pointers:** A string in C is essentially an array of characters terminated by a null character (`\0`). Pointers can be used to iterate over strings and manipulate them. For instance: ```c char str[] = "Hello"; char *p = str; // p points to the first character of the string while (*p != '\0') { printf("%c", *p); p++; } ``` #### Pointers to Functions Pointers can also point to functions. This concept is particularly useful for implementing callbacks and higher-order functions. To declare a pointer to a function, specify the return type, followed by asterisks (`*`), and then the parameter types in parentheses. For example: ```c int add(int a, int b) { return a + b; } int (*funcPtr)(int, int) = add; // funcPtr points to a function that takes two ints and returns an int int main() { int result = funcPtr(5, 10); printf("Result: %d\n", result); // Output: Result: 15 } ``` In this example, `funcPtr` is a pointer to a function that takes two integers and returns an integer. It is initialized to point to the `add` function. #### Arrays of Strings Finally, consider how to define and use arrays of strings. This is often done when dealing with multiple strings in a structured way. For example: ```c char *names[] = {"Alice", "Bob", "Charlie"}; for (int i = 0; i < 3; i++) { printf("%s\n", names[i]); } ``` Here, `names` is an array of pointers, each pointing to a different string. You can iterate over the array and print each string using a loop. #### Conclusion Understanding and mastering pointers is essential for effective C programming. They provide a powerful toolset for managing memory, passing data by reference, manipulating strings, and creating dynamic data structures. As you continue to explore the world of C programming, make sure to practice these concepts thoroughly to become proficient in using pointers.
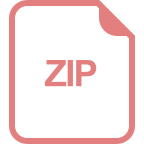
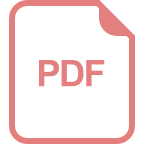
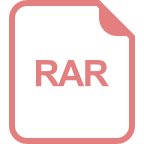
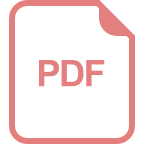
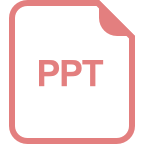
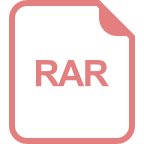
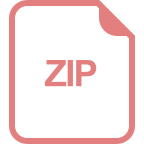
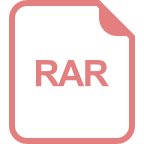
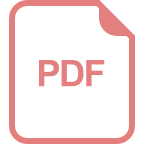
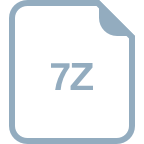
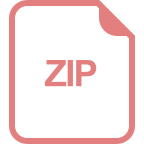
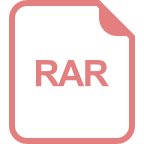
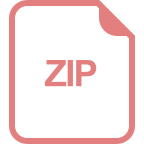
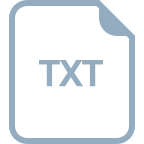
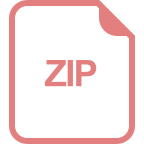
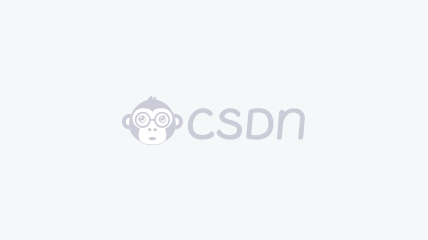

- 粉丝: 4
- 资源: 14
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

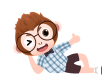
最新资源
- AI生成PPT(免费+收费)总汇
- CCD卷绕检测机sw18可编辑全套技术资料100%好用.zip
- 基于FPGA的自适应滤波器FIR IIR滤波器LMS NLMS RLS算法 FxLMS 分数阶 本设计是在FPGA开发板上实现一个自适应滤波器,只需要输入于扰信号和期望信号(混合信号)即可得到滤波输
- 单机版RS485集中抄表软件,集中抄读645-2007协议的智能电表,645-1997的没有测试过,不清楚能不能抄,本地485有线集中抄表,配合485转网络可实现远程抄表
- 数据分析-62-亿欧企业榜单探索
- AR贴膜主机(sw12可编辑+工程图)全套技术资料100%好用.zip
- 数据分析-63-基于逻辑回归模型的医疗数据分析(拟合度差)
- 半龙门伺服双点电阻焊机sw19全套技术资料100%好用.zip
- 板材定位送料机sw18全套技术资料100%好用.zip
- 黑猫消费者投诉数据集,数据量大概43000条
- 板料翻面滚筒输送机sw19可编辑全套技术资料100%好用.zip
- 2023胡润百富榜-品牌榜数据集
- 半自动螺钉拧紧机step全套技术资料100%好用.zip
- 永磁同步电机磁链、损耗计算模型,基于有限元仿真数据
- 玻璃清洗机器人sw18全套技术资料100%好用.zip
- labview编写的数据回放软件,支持多曲线回放,支持曲线缩放,支持曲线打标签,支持曲线勾选可见不可见,支持点击曲线加粗显示,支持点击曲线显示当前曲线Y标尺,支持曲线配置,支持红蓝标尺,支持曲线时间轴

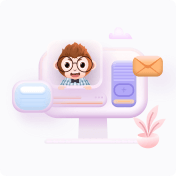
