tm_sec Seconds after minute (0 – 59)
tm_min Minutes after hour (0 – 59)
tm_hour Hours after midnight (0 – 23)
tm_mday Day of month (1 – 31)
tm_mon Month (0 – 11; January = 0)
tm_year Year (current year minus 1900)
tm_wday Day of week (0 – 6; Sunday = 0)
tm_yday Day of year (0 – 365; January 1 = 0)
int tm_sec; /* seconds after the minute - [0,59] */
int tm_min; /* minutes after the hour - [0,59] */
int tm_hour; /* hours since midnight - [0,23] */
int tm_mday; /* day of the month - [1,31] */
int tm_mon; /* months since January - [0,11] */
int tm_year; /* years since 1900 */
int tm_wday; /* days since Sunday - [0,6] */
int tm_yday; /* days since January 1 - [0,365] */
int tm_isdst; /* daylight savings time flag */
//---------------------------------------------------------------
#include <time.h>
#include <stdio.h>
struct tm *newtime;
time_t aclock;
void main( void )
{
time( &aclock ); /* Get time in seconds */
newtime = localtime( &aclock ); /* Convert time to struct */
/* tm form */
/* Print local time as a string */
printf( "The current date and time are: %s", asctime( newtime ) );
}
//-----------------------------------------------------------------
#include <time.h>
#include <stdio.h>
void main( void )
{
struct tm when;
time_t now, result;
int days;
time( &now );
when = *localtime( &now );
printf( "Current time is %s\n", asctime( &when ) );
printf( "How many days to look ahead: " );
scanf( "%d", &days );
when.tm_mday = when.tm_mday + days;
if( (result = mktime( &when )) != (time_t)-1 )
printf( "In %d days the time will be %s\n",
days, asctime( &when ) );
else
perror( "mktime failed" );
}
// hl_view.cpp : implementation file
//
//显示文本有三种方法.
#include "stdafx.h"
#include "a1234.h"
#include "hl_view.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////
// hldata m_hldata;
hl_view::hl_view(CWnd* pParent /*=NULL*/)
: CDialog(hl_view::IDD, pParent)
{
EnableAutomation();
//{{AFX_DATA_INIT(hl_view)
m_edit1_send_txt = _T("");
m_check1_run = FALSE;
m_2_time_picke = COleDateTime::GetCurrentTime();
m_1_time_picke = 0;
m_STATIC_1 = _T("");
//}}AFX_DATA_INIT
//static -----
//需要初始化的变量放在这里.
m_static1_txt="初始化显示";
m_send =0;
m_receive=0;
m_check2_doing =true;
m_check1_doing =false;
}
void hl_view::OnFinalRelease()
{
// When the last reference for an automation object is released
// OnFinalRelease is called. The base class will automatically
// deletes the object. Add additional cleanup required for your
// object before calling the base class.
CDialog::OnFinalRelease();
}
void hl_view::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(hl_view)
DDX_Control(pDX, IDC_CHECK1, m_CHECK1_TEXT);
DDX_Text(pDX, IDC_EDIT1_send, m_edit1_send_txt);
DDX_Check(pDX, IDC_CHECK1, m_check1_run);
DDX_DateTimeCtrl(pDX, IDC_DATETIMEPICKER2, m_2_time_picke);
DDX_DateTimeCtrl(pDX, IDC_DATETIMEPICKER1, m_1_time_picke);
DDX_Text(pDX, IDC_STATIC1, m_STATIC_1);
//}}AFX_DATA_MAP
DDX_Control(pDX, IDC_STATIC2, STATIC2_static); //需要自己添加.添加1步
DDX_Text(pDX, IDC_EDIT1_send, m_send); //
// DDV_MinMaxDouble(pDX, m_send, 76., 108.);
DDX_Text(pDX, IDC_EDIT2_receive, m_receive);
DDX_Text(pDX, IDC_STATIC1, m_static1_txt);
DDX_Check(pDX, IDC_CHECK1, m_check1_doing); //复选框 加1步
DDX_Check(pDX, IDC_CHECK2, m_check2_doing);
}
BEGIN_MESSAGE_MAP(hl_view, CDialog)
//{{AFX_MSG_MAP(hl_view)
ON_BN_CLICKED(IDC_key_ok, Onkeyok)
ON_BN_CLICKED(IDC_CHECK2, OnCheck2)
ON_BN_CLICKED(IDC_RADIO2, OnRadio2)
ON_BN_CLICKED(IDC_RADIO1, OnRadio1)
ON_BN_CLICKED(IDC_CHECK1, OnCheck1)
ON_BN_CLICKED(IDC_STATIC2, OnStatic2)
ON_BN_CLICKED(IDC_STATIC1, OnStatic1)
ON_EN_CHANGE(IDC_EDIT1_send, OnChangeEDIT1send)
ON_EN_CHANGE(IDC_EDIT2_receive, OnChangeEDIT2receive)
ON_BN_CLICKED(IDC_dan_secelt, Ondansecelt)
ON_BN_CLICKED(IDC_duo_select, Onduoselect)
ON_NOTIFY(DTN_CLOSEUP, IDC_DATETIMEPICKER1, OnCloseupDatetimepicker1)
ON_NOTIFY(DTN_FORMAT, IDC_DATETIMEPICKER2, OnFormatDatetimepicker2)
ON_BN_CLICKED(IDC_data_time, Ondatatime)
ON_WM_TIMER()
ON_WM_PAINT()
ON_WM_CANCELMODE()
ON_BN_CLICKED(IDC_RADIO3, OnRadio3)
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
BEGIN_DISPATCH_MAP(hl_view, CDialog)
//{{AFX_DISPATCH_MAP(hl_view)
// NOTE - the ClassWizard will add and remove mapping macros here.
//}}AFX_DISPATCH_MAP
END_DISPATCH_MAP()
// Note: we add support for IID_Ihl_view to support typesafe binding
// from VBA. This IID must match the GUID that is attached to the
// dispinterface in the .ODL file.
// {8EB35B8C-AADC-41C3-8031-76B235F9E6BE}
static const IID IID_Ihl_view =
{ 0x8eb35b8c, 0xaadc, 0x41c3, { 0x80, 0x31, 0x76, 0xb2, 0x35, 0xf9, 0xe6, 0xbe } };
BEGIN_INTERFACE_MAP(hl_view, CDialog)
INTERFACE_PART(hl_view, IID_Ihl_view, Dispatch)
END_INTERFACE_MAP()
/////////////////////////////////////////////////////////////////////////////
// hl_view message handlers
void hl_view::Onkeyok()
{
UpdateData();
//----------------------------
// using namespace std;
time_t tU = 9011111;
struct tm* mytime;
mytime = localtime( &tU );
switch(mytime->tm_wday)
{
case 0:
cout << "Sunday" << endl;
break;
case 1:
cout << "Monday" << endl;
break;
case 2:
cout << "Tuesday" << endl;
break;
case 3:
cout << "Wednesday" << endl;
break;
case 4:
cout << "Thursday" << endl;
break;
case 5:
cout << "Friday" << endl;
break;
case 6:
cout << "Saterday" << endl;
break;
};
//----------------------------
tm date_tm;
tm t;
time_t t_of_day;
t.tm_year=2007-1900; // 初始值为//1900-1-1 00 :00:00
t.tm_mon=0;
t.tm_mday=3; //注意如果多了,会往前注入.1-31 结果为3-3 因为2月只有28天.
t.tm_wday=0;
t.tm_isdst=0;
t.tm_hour=1; //01:00:01
t.tm_min=0;
t.tm_sec=1;
t_of_day=mktime(&t);
//m_2_time_picke = t_of_day;
// m_1_time_picke = t_of_day; ;//t_of_day ;
//---------------------------比较两个时间
time_t start, finish;
double result, elapsed_time;
// time( &start );
time( &finish );
elapsed_time = difftime( finish, t_of_day ); //如果两个相等,比较结果为0
//--------------------------
//==================
/*
CString mTime;
mTime="2008-5-25 12:25:32"; //自设时间
//在界面上有两DataTimePicker控件
CTime m_1_time_picke;//短日期:IDC_DATETIMEPICKER1
CTime m_2_time_picke;//时间: IDC_DATETIMEPICKER2 请问如何将字符串赋值到此二控件上
//答://如果不想拆分字符串,那么可以通过COleDateTime来转换成CTime
COleDateTime dt;
dt.ParseDateTime(mTime); //将mTime的时间值存到dt中
SYSTEMTIME st; //定义一个系统时间类型的变量
dt.GetAsSystemTime(st); //将dt中的时间按系统时间格式化
CTime tm(st); //定义CTime对象并将st赋值给它
m_1_time_picke = tm; //控件会自动获取其需要的时间部分
m_2_time_picke = tm;
//-----------------
CString strm = "2000-1-1 23:01:22" ;
COleDateTime tmm;
tmm.ParseDateTime(strm);
SYSTEMTIME stt;
tmm.GetAsSystemTime(stt);
CTime ct(stt);
没有合适的资源?快使用搜索试试~ 我知道了~
收起资源包目录


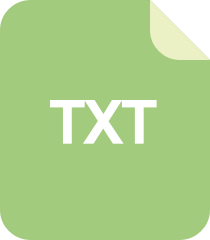
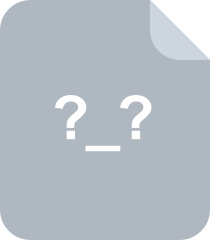
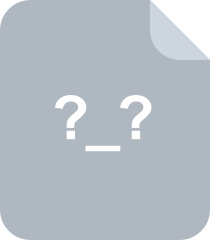

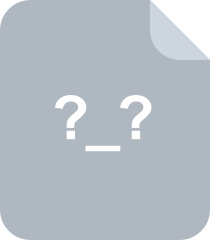
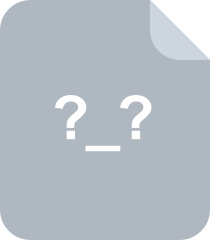
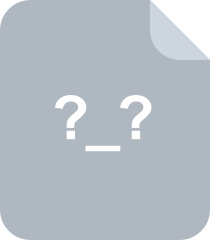
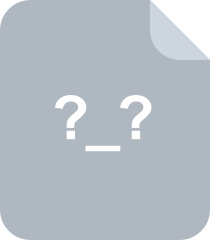
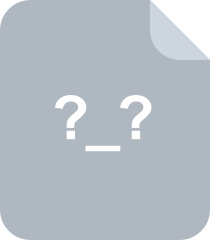
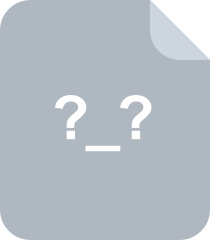
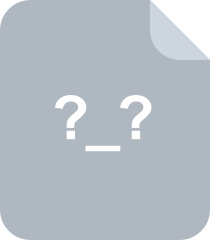
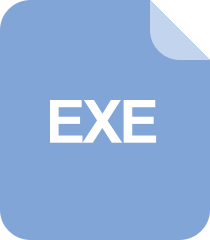
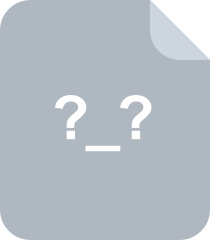

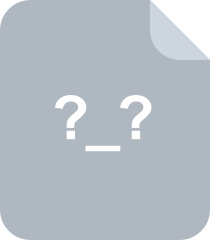
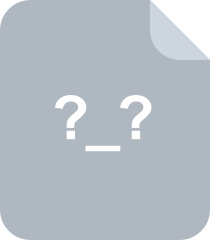
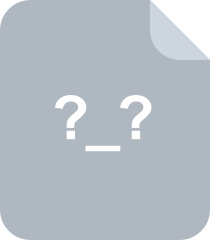
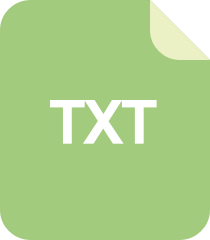

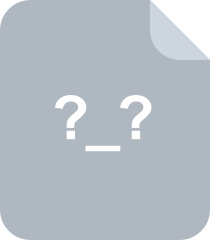
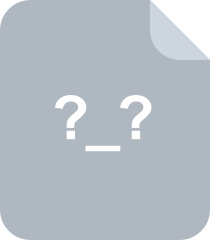
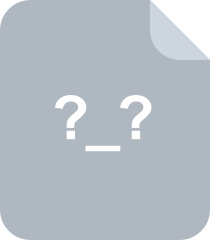
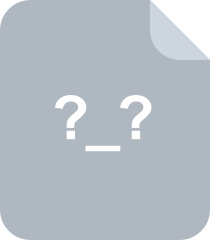
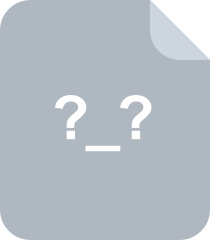
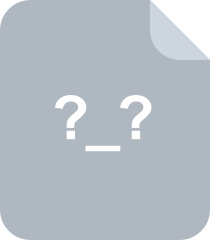
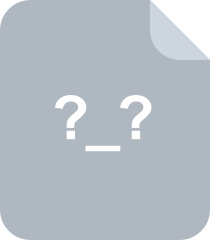
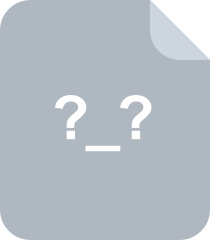
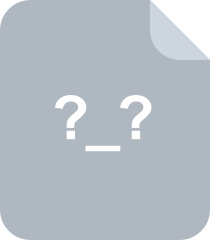
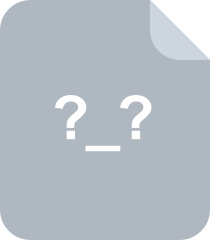
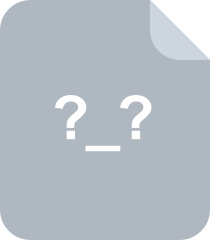
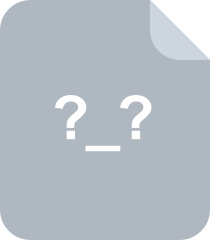
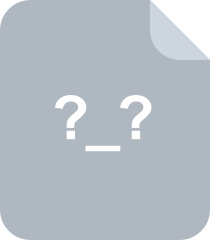
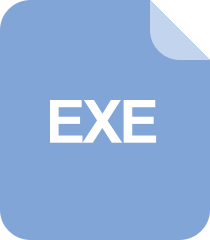
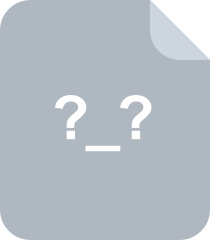
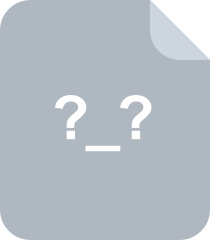
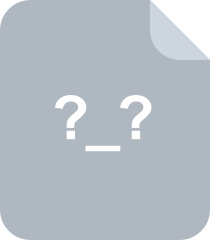
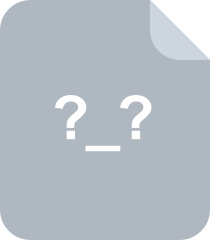
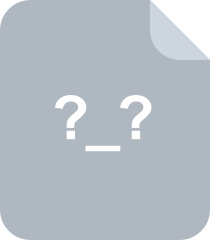
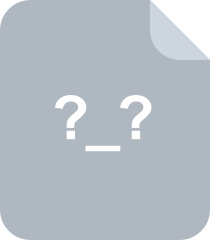
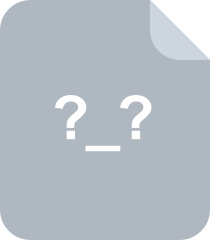
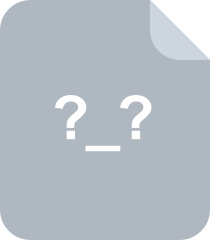
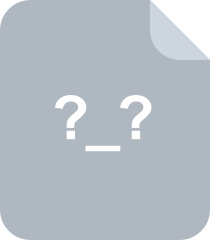
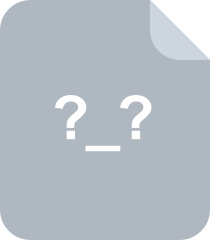
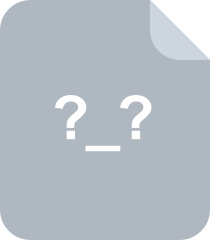
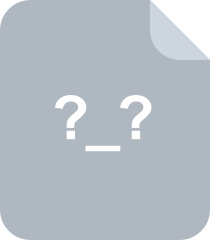
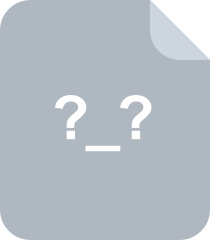
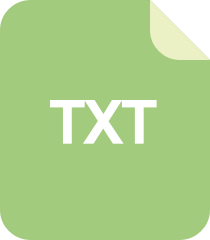
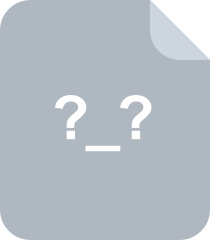
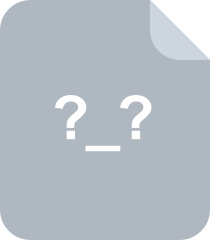
共 46 条
- 1
资源推荐
资源预览
资源评论
158 浏览量
145 浏览量

117 浏览量
2019-05-26 上传
2009-09-28 上传
172 浏览量
150 浏览量
165 浏览量
2023-10-24 上传
187 浏览量
2008-09-13 上传
167 浏览量
2011-12-17 上传
193 浏览量
154 浏览量
136 浏览量
2023-09-21 上传
111 浏览量
101 浏览量
148 浏览量
2007-11-15 上传
2021-11-23 上传
2011-04-19 上传
2007-07-26 上传
157 浏览量
2024-06-04 上传

145 浏览量
资源评论
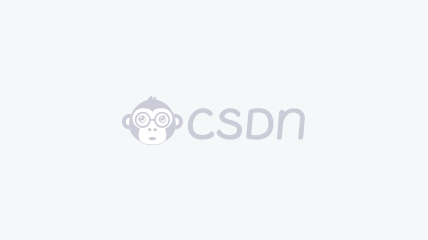

HLIANG8899
- 粉丝: 2
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

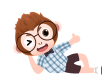
安全验证
文档复制为VIP权益,开通VIP直接复制
