# Form
### Intro
Used for data entry and verification, and supports input boxes, radio buttons, check boxes, file uploads and other types. Should be used with [Field](#/en-US/field) component.
### Install
Register component globally via `app.use`, refer to [Component Registration](#/en-US/advanced-usage#zu-jian-zhu-ce) for more registration ways.
```js
import { createApp } from 'vue';
import { Form, Field, CellGroup } from 'vant';
const app = createApp();
app.use(Form);
app.use(Field);
app.use(CellGroup);
```
## Usage
### Basic Usage
```html
<van-form @submit="onSubmit">
<van-cell-group inset>
<van-field
v-model="username"
name="Username"
label="Username"
placeholder="Username"
:rules="[{ required: true, message: 'Username is required' }]"
/>
<van-field
v-model="password"
type="password"
name="Password"
label="Password"
placeholder="Password"
:rules="[{ required: true, message: 'Password is required' }]"
/>
</van-cell-group>
<div style="margin: 16px;">
<van-button round block type="primary" native-type="submit">
Submit
</van-button>
</div>
</van-form>
```
```js
import { ref } from 'vue';
export default {
setup() {
const username = ref('');
const password = ref('');
const onSubmit = (values) => {
console.log('submit', values);
};
return {
username,
password,
onSubmit,
};
},
};
```
### Validate Rules
```html
<van-form @failed="onFailed">
<van-cell-group inset>
<van-field
v-model="value1"
name="pattern"
placeholder="Use pattern"
:rules="[{ pattern, message: 'Error message' }]"
/>
<van-field
v-model="value2"
name="validator"
placeholder="Use validator"
:rules="[{ validator, message: 'Error message' }]"
/>
<van-field
v-model="value3"
name="validatorMessage"
placeholder="Use validator to return message"
:rules="[{ validator: validatorMessage }]"
/>
<van-field
v-model="value4"
name="asyncValidator"
placeholder="Use async validator"
:rules="[{ validator: asyncValidator, message: 'Error message' }]"
/>
</van-cell-group>
<div style="margin: 16px;">
<van-button round block type="primary" native-type="submit">
Submit
</van-button>
</div>
</van-form>
```
```js
import { ref } from 'vue';
import { closeToast, showLoadingToast } from 'vant';
export default {
setup() {
const value1 = ref('');
const value2 = ref('');
const value3 = ref('abc');
const value4 = ref('');
const pattern = /\d{6}/;
const validator = (val) => /1\d{10}/.test(val);
const validatorMessage = (val) => `${val} is invalid`;
const asyncValidator = (val) =>
new Promise((resolve) => {
showLoadingToast('Validating...');
setTimeout(() => {
closeToast();
resolve(val === '1234');
}, 1000);
});
const onFailed = (errorInfo) => {
console.log('failed', errorInfo);
};
return {
value1,
value2,
value3,
value4,
pattern,
onFailed,
validator,
asyncValidator,
validatorMessage,
};
},
};
```
### Field Type - Switch
```html
<van-field name="switch" label="Switch">
<template #input>
<van-switch v-model="checked" />
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const checked = ref(false);
return { checked };
},
};
```
### Field Type - Checkbox
```html
<van-field name="checkbox" label="Checkbox">
<template #input>
<van-checkbox v-model="checked" shape="square" />
</template>
</van-field>
<van-field name="checkboxGroup" label="CheckboxGroup">
<template #input>
<van-checkbox-group v-model="groupChecked" direction="horizontal">
<van-checkbox name="1" shape="square">Checkbox 1</van-checkbox>
<van-checkbox name="2" shape="square">Checkbox 2</van-checkbox>
</van-checkbox-group>
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const checked = ref(false);
const groupChecked = ref([]);
return {
checked,
groupChecked,
};
},
};
```
### Field Type - Radio
```html
<van-field name="radio" label="Radio">
<template #input>
<van-radio-group v-model="checked" direction="horizontal">
<van-radio name="1">Radio 1</van-radio>
<van-radio name="2">Radio 2</van-radio>
</van-radio-group>
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const checked = ref('1');
return { checked };
},
};
```
### Field Type - Stepper
```html
<van-field name="stepper" label="Stepper">
<template #input>
<van-stepper v-model="value" />
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const value = ref(1);
return { value };
},
};
```
### Field Type - Rate
```html
<van-field name="rate" label="Rate">
<template #input>
<van-rate v-model="value" />
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const value = ref(3);
return { value };
},
};
```
### Field Type - Slider
```html
<van-field name="slider" label="Slider">
<template #input>
<van-slider v-model="value" />
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const value = ref(50);
return { value };
},
};
```
### Field Type - Uploader
```html
<van-field name="uploader" label="Uploader">
<template #input>
<van-uploader v-model="value" />
</template>
</van-field>
```
```js
import { ref } from 'vue';
export default {
setup() {
const value = ref([
{ url: 'https://fastly.jsdelivr.net/npm/@vant/assets/leaf.jpeg' },
]);
return { value };
},
};
```
### Field Type - Picker
```html
<van-field
v-model="result"
is-link
readonly
name="picker"
label="Picker"
placeholder="Select city"
@click="showPicker = true"
/>
<van-popup v-model:show="showPicker" position="bottom">
<van-picker
:columns="columns"
@confirm="onConfirm"
@cancel="showPicker = false"
/>
</van-popup>
```
```js
import { ref } from 'vue';
export default {
setup() {
const result = ref('');
const showPicker = ref(false);
const columns = [
{ text: 'Delaware', value: 'Delaware' },
{ text: 'Florida', value: 'Florida' },
{ text: 'Georqia', value: 'Georqia' },
{ text: 'Indiana', value: 'Indiana' },
{ text: 'Maine', value: 'Maine' },
];
const onConfirm = ({ selectedOptions }) => {
result.value = selectedOptions[0]?.text;
showPicker.value = false;
};
return {
result,
columns,
onConfirm,
showPicker,
};
},
};
```
### Field Type - DatePicker
```html
<van-field
v-model="result"
is-link
readonly
name="datePicker"
label="Date Picker"
placeholder="Select date"
@click="showPicker = true"
/>
<van-popup v-model:show="showPicker" position="bottom">
<van-date-picker @confirm="onConfirm" @cancel="showPicker = false" />
</van-popup>
```
```js
import { ref } from 'vue';
export default {
setup() {
const result = ref('');
const showPicker = ref(false);
const onConfirm = ({ selectedValues }) => {
result.value = selectedValues.join('/');
showPicker.value = false;
};
return {
result,
onConfirm,
showPicker,
};
},
};
```
### Field Type - Area
```html
<van-field
v-model="result"
is-link
readonly
name="area"
label="Area Picker"
placeholder="Select area"
@click="showArea = true"
/>
<van-popup v-model:show="showArea" position="bottom">
<van-area
:area-list="areaList"
@confirm="onConfirm"
@cancel="showArea = false"
/>
</van-popup>
```
```js
import { ref } from 'vue';
import { areaList } from '@vant/area-
没有合适的资源?快使用搜索试试~ 我知道了~
Vant移动端组件库.rar
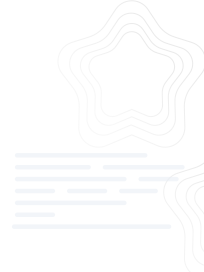
共1519个文件
ts:544个
md:234个
snap:228个

需积分: 5 2 下载量 6 浏览量
2023-07-11
22:38:04
上传
评论
收藏 21.41MB RAR 举报
温馨提示
1.0 vant组件库-介绍 vant是一个轻量、可靠的移动端 Vue 组件库, 开箱即用 vant官网 特点: 提供 60 多个高质量组件,覆盖移动端各类场景 性能极佳,组件平均体积不到 1kb 完善的中英文文档和示例 支持 Vue 2 & Vue 3 支持按需引入和主题定制 1.1 全部引入 1.全部引入, 快速开始: https://vant-contrib.gitee.io/vant/#/zh-CN/quickstart 2.下载Vant组件库到当前项目中 3.在main.js中全局导入所有组件,
资源推荐
资源详情
资源评论
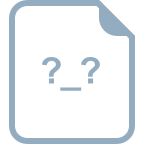
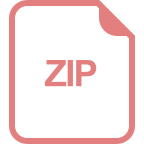
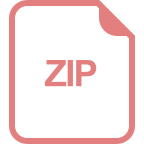
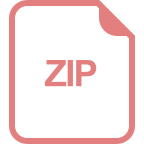
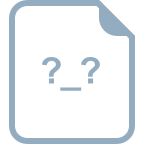
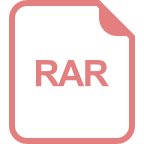
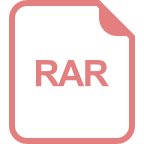
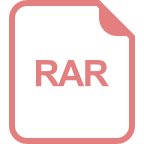
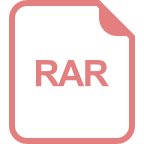
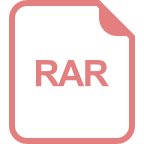
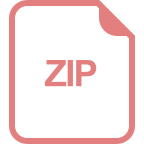
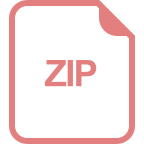
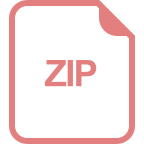
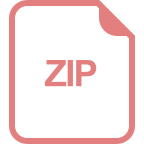
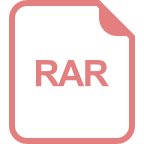
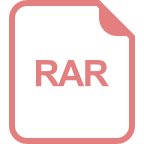
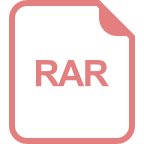
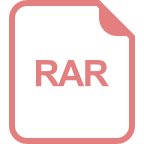
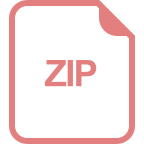
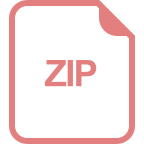
收起资源包目录

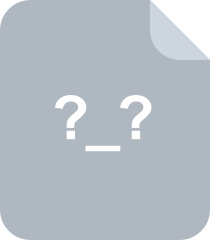
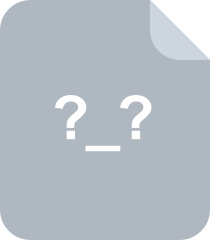
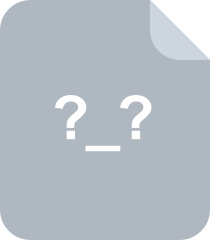
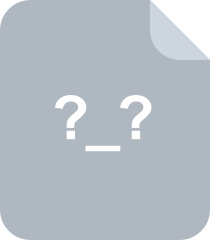
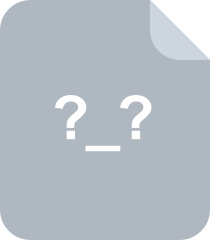
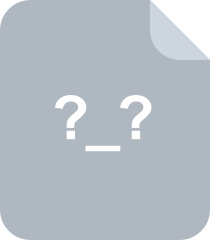
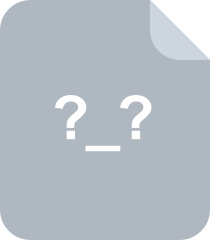
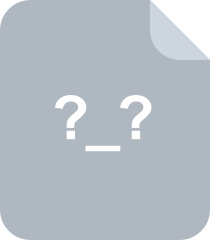
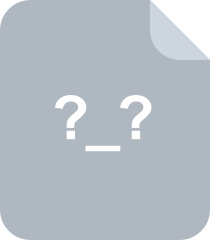
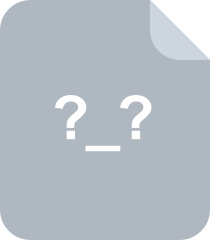
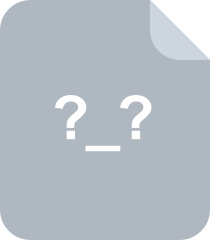
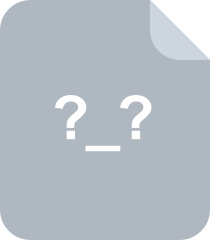
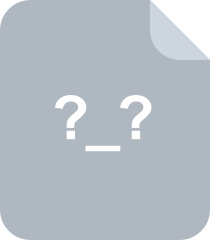
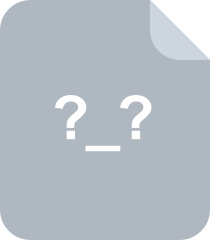
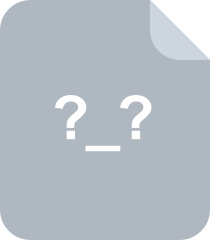
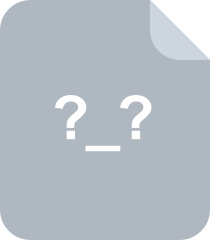
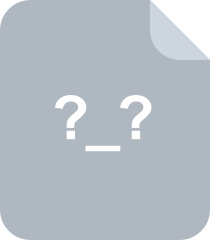
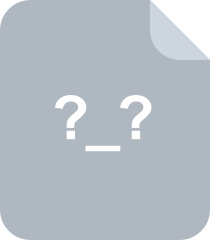
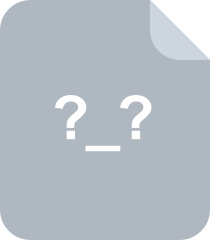
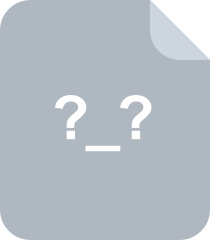
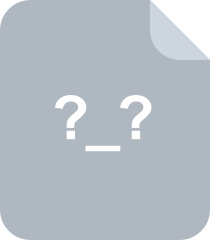
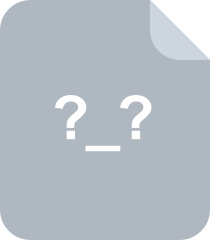
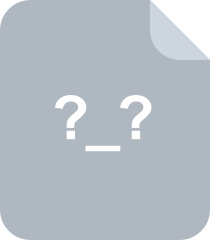
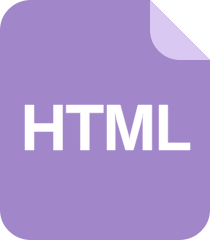
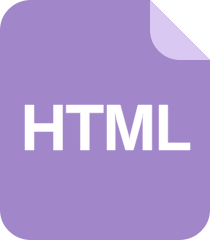
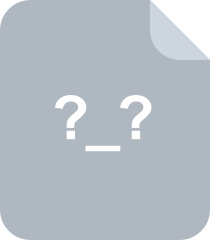
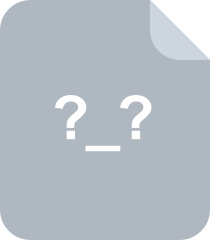
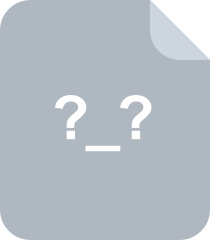
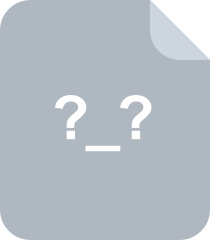
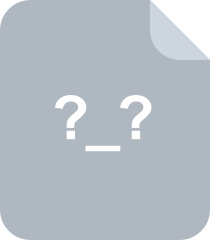
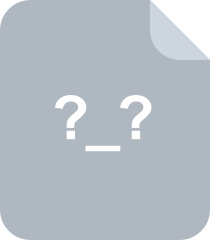
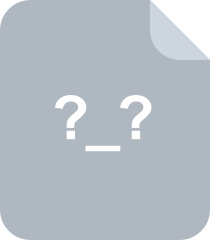
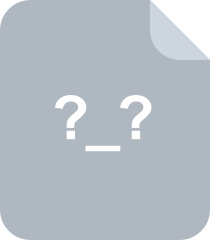
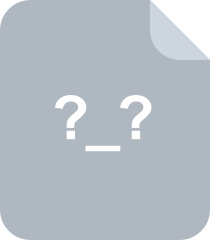
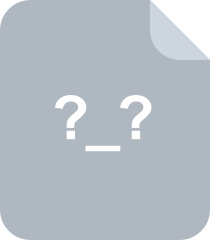
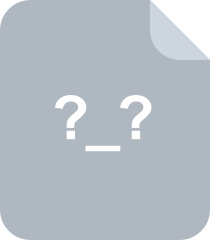
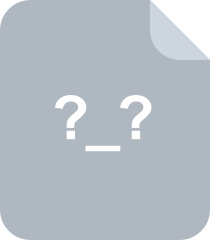
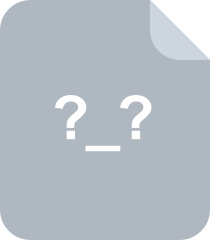
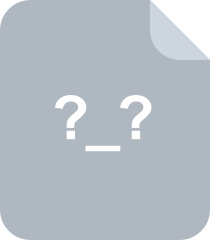
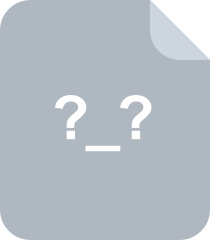
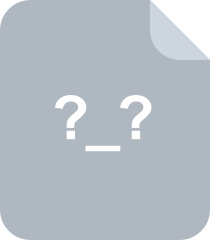
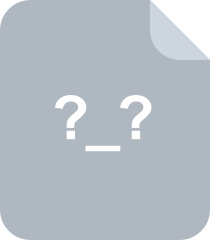
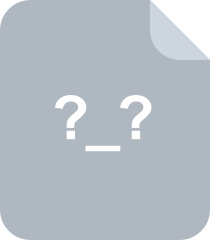
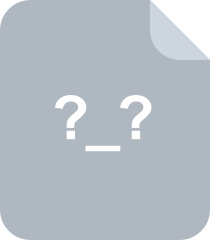
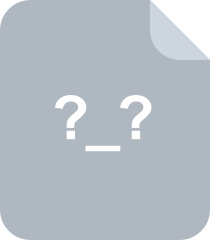
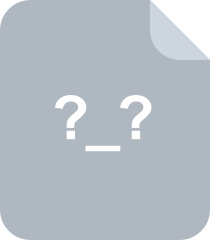
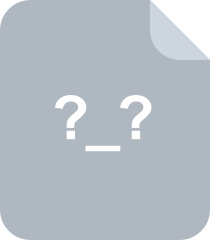
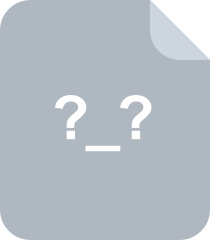
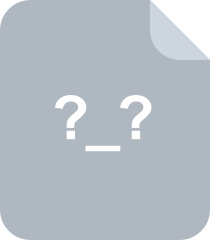
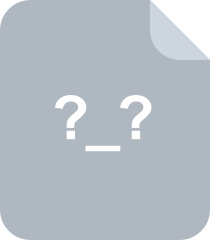
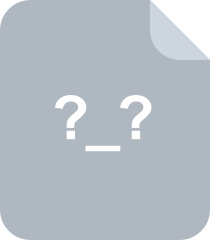
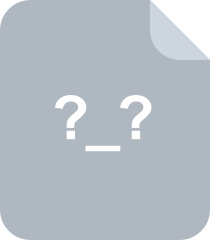
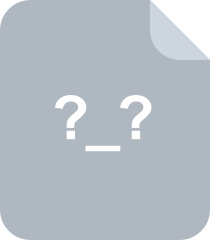
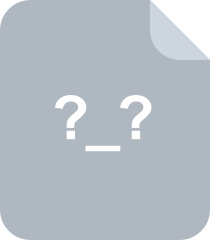
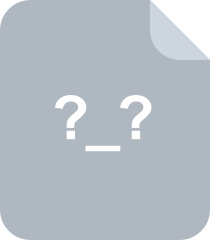
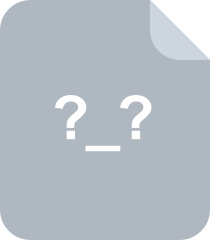
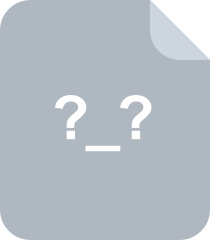
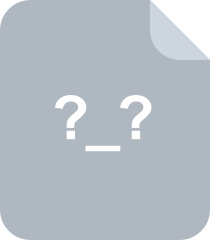
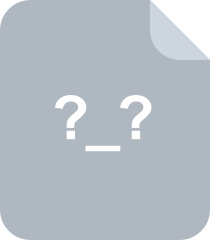
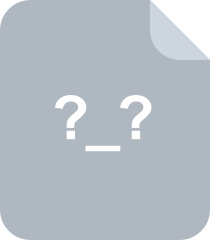
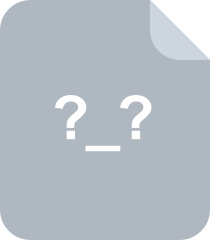
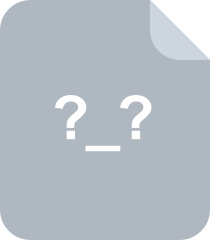
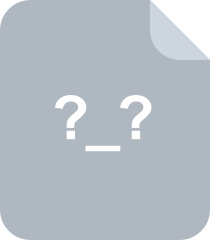
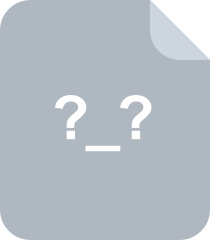
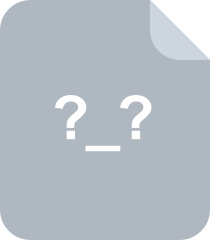
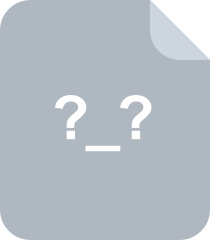
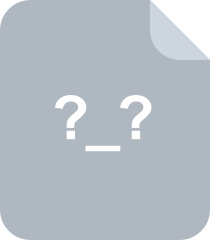
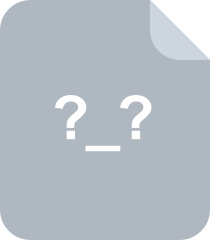
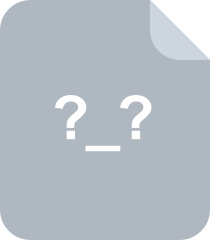
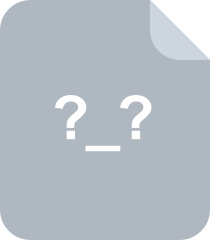
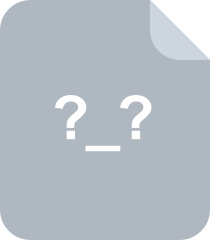
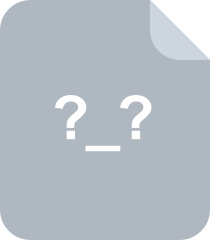
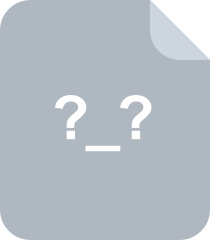
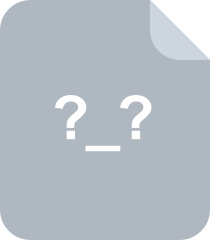
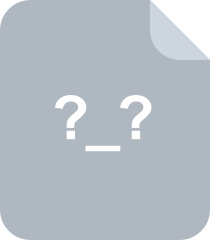
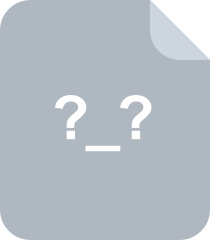
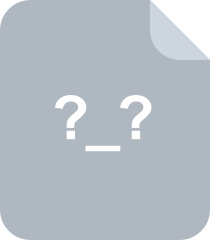
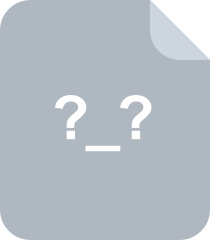
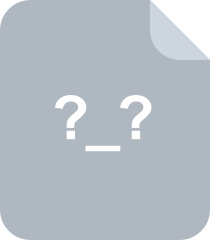
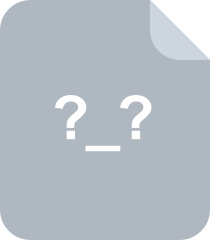
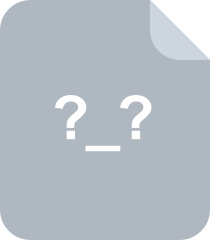
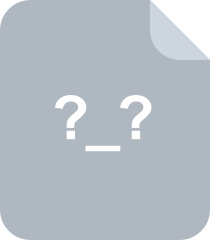
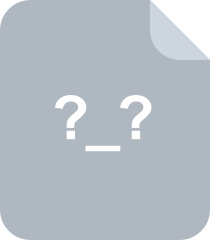
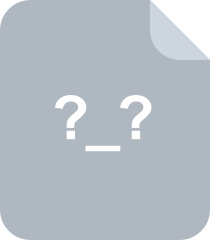
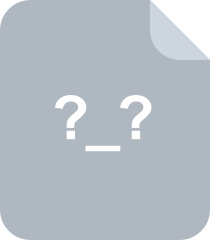
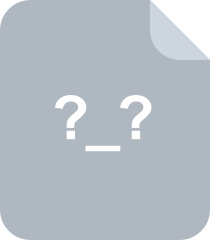
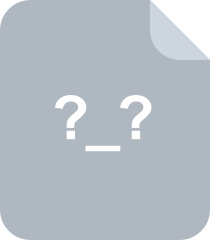
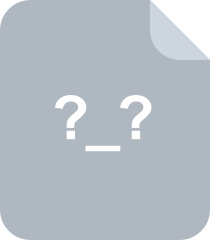
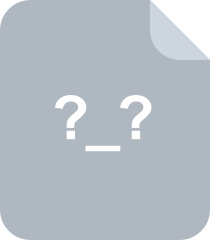
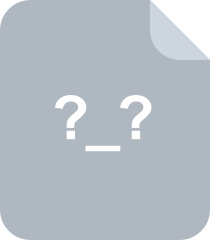
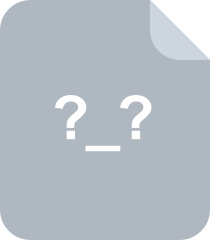
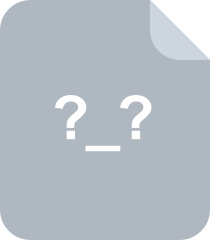
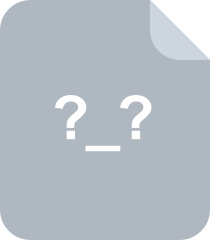
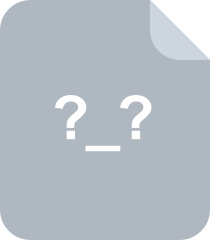
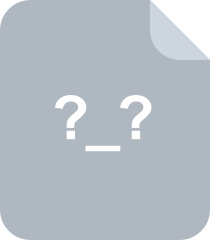
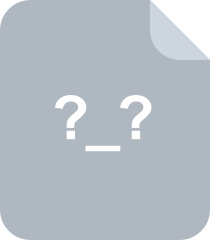
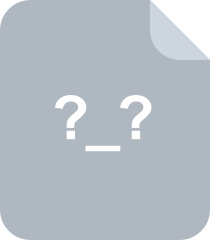
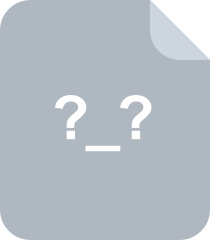
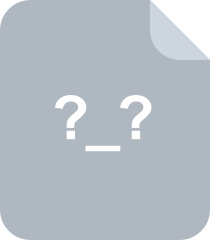
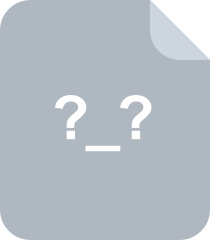
共 1519 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
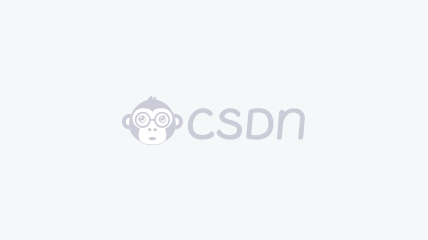
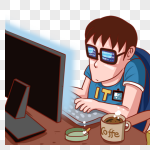
野生的狒狒
- 粉丝: 3393
- 资源: 2436
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

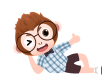
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


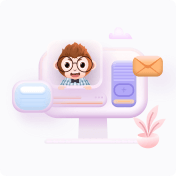
安全验证
文档复制为VIP权益,开通VIP直接复制
