/* The following code was generated by JFlex 1.4.1 on 12/29/16 11:15 PM */
/*
* 01/28/2009
*
* PHPTokenMaker.java - Generates tokens for PHP syntax highlighting.
*
* This library is distributed under a modified BSD license. See the included
* RSyntaxTextArea.License.txt file for details.
*/
package org.fife.ui.rsyntaxtextarea.modes;
import java.io.IOException;
import java.io.Reader;
import java.util.Stack;
import javax.swing.text.Segment;
import org.fife.ui.rsyntaxtextarea.HtmlOccurrenceMarker;
import org.fife.ui.rsyntaxtextarea.OccurrenceMarker;
import org.fife.ui.rsyntaxtextarea.RSyntaxUtilities;
import org.fife.ui.rsyntaxtextarea.Token;
import org.fife.ui.rsyntaxtextarea.TokenImpl;
/**
* Scanner for PHP files.
*
* This implementation was created using
* <a href="http://www.jflex.de/">JFlex</a> 1.4.1; however, the generated file
* was modified for performance. Memory allocation needs to be almost
* completely removed to be competitive with the handwritten lexers (subclasses
* of <code>AbstractTokenMaker</code>, so this class has been modified so that
* Strings are never allocated (via yytext()), and the scanner never has to
* worry about refilling its buffer (needlessly copying chars around).
* We can achieve this because RText always scans exactly 1 line of tokens at a
* time, and hands the scanner this line as an array of characters (a Segment
* really). Since tokens contain pointers to char arrays instead of Strings
* holding their contents, there is no need for allocating new memory for
* Strings.<p>
*
* The actual algorithm generated for scanning has, of course, not been
* modified.<p>
*
* If you wish to regenerate this file yourself, keep in mind the following:
* <ul>
* <li>The generated <code>PHPTokenMaker.java</code> file will contain two
* definitions of both <code>zzRefill</code> and <code>yyreset</code>.
* You should hand-delete the second of each definition (the ones
* generated by the lexer), as these generated methods modify the input
* buffer, which we'll never have to do.</li>
* <li>You should also change the declaration/definition of zzBuffer to NOT
* be initialized. This is a needless memory allocation for us since we
* will be pointing the array somewhere else anyway.</li>
* <li>You should NOT call <code>yylex()</code> on the generated scanner
* directly; rather, you should use <code>getTokenList</code> as you would
* with any other <code>TokenMaker</code> instance.</li>
* </ul>
*
* @author Robert Futrell
* @version 0.9
*/
public class PHPTokenMaker extends AbstractMarkupTokenMaker {
/** This character denotes the end of file */
public static final int YYEOF = -1;
/** lexical states */
public static final int PHP = 19;
public static final int INATTR_SINGLE_SCRIPT = 9;
public static final int JS_CHAR = 14;
public static final int CSS_STRING = 26;
public static final int JS_DOCCOMMENT = 17;
public static final int JS_MLC = 16;
public static final int CSS_CHAR_LITERAL = 27;
public static final int INTAG_SCRIPT = 7;
public static final int JS_TEMPLATE_LITERAL_EXPR = 30;
public static final int CSS_PROPERTY = 24;
public static final int CSS_C_STYLE_COMMENT = 28;
public static final int PHP_MLC = 20;
public static final int CSS = 23;
public static final int CSS_VALUE = 25;
public static final int COMMENT = 1;
public static final int INATTR_DOUBLE_SCRIPT = 8;
public static final int PHP_STRING = 21;
public static final int JAVASCRIPT = 13;
public static final int INTAG = 3;
public static final int INTAG_CHECK_TAG_NAME = 4;
public static final int INATTR_SINGLE_STYLE = 12;
public static final int DTD = 2;
public static final int PHP_CHAR = 22;
public static final int JS_EOL_COMMENT = 18;
public static final int INATTR_DOUBLE_STYLE = 11;
public static final int INATTR_SINGLE = 6;
public static final int JS_TEMPLATE_LITERAL = 29;
public static final int YYINITIAL = 0;
public static final int INATTR_DOUBLE = 5;
public static final int JS_STRING = 15;
public static final int INTAG_STYLE = 10;
/**
* Translates characters to character classes
*/
private static final String ZZ_CMAP_PACKED =
"\11\0\1\4\1\2\1\0\1\1\1\33\22\0\1\4\1\51\1\7"+
"\1\34\1\36\1\50\1\5\1\116\1\114\1\113\1\37\1\42\1\45"+
"\1\31\1\43\1\10\1\25\1\137\1\132\1\136\1\134\1\126\1\133"+
"\1\27\1\140\1\24\1\53\1\6\1\3\1\46\1\17\1\52\1\112"+
"\1\110\1\26\1\12\1\41\1\22\1\107\1\121\1\125\1\14\1\127"+
"\1\122\1\21\1\120\1\111\1\117\1\15\1\123\1\13\1\11\1\16"+
"\1\106\1\124\1\23\1\40\1\20\1\23\1\115\1\35\1\115\1\47"+
"\1\30\1\131\1\64\1\67\1\70\1\77\1\62\1\63\1\71\1\73"+
"\1\56\1\135\1\76\1\65\1\57\1\72\1\74\1\100\1\104\1\61"+
"\1\66\1\60\1\32\1\102\1\75\1\103\1\101\1\130\1\105\1\55"+
"\1\44\1\54\uff81\0";
/**
* Translates characters to character classes
*/
private static final char [] ZZ_CMAP = zzUnpackCMap(ZZ_CMAP_PACKED);
/**
* Translates DFA states to action switch labels.
*/
private static final int [] ZZ_ACTION = zzUnpackAction();
private static final String ZZ_ACTION_PACKED_0 =
"\5\0\2\1\1\0\2\1\1\0\2\1\14\0\1\2"+
"\5\0\2\2\1\3\1\4\1\5\1\6\1\1\1\7"+
"\5\1\1\10\1\11\3\12\1\13\1\14\1\15\1\16"+
"\1\17\1\20\1\21\1\22\2\20\2\22\3\20\2\22"+
"\3\20\1\22\5\20\1\22\1\1\1\23\1\24\1\1"+
"\1\25\1\14\1\26\1\27\1\30\1\31\1\32\1\33"+
"\1\34\1\35\1\36\2\16\1\2\1\37\1\16\2\2"+
"\1\16\2\40\1\16\1\2\1\35\2\16\1\2\1\41"+
"\1\35\21\2\1\42\1\43\1\1\1\44\1\23\1\45"+
"\1\46\1\1\1\47\1\23\1\50\1\51\1\1\1\52"+
"\6\1\1\53\10\1\1\54\4\1\1\55\1\16\1\56"+
"\1\16\3\2\1\57\1\2\1\16\32\2\1\35\1\60"+
"\2\2\1\1\1\61\2\1\1\62\1\63\1\64\2\1"+
"\1\65\1\66\1\67\1\70\1\71\1\70\1\72\1\70"+
"\1\73\1\70\1\74\1\75\1\70\1\76\1\77\1\100"+
"\2\77\1\101\1\77\1\102\1\103\1\104\1\105\1\104"+
"\1\106\2\2\1\40\1\2\2\104\1\107\1\110\1\111"+
"\1\112\1\113\1\114\1\115\2\1\1\116\1\117\1\120"+
"\1\121\1\1\1\122\1\1\1\123\1\4\2\124\1\125"+
"\1\126\1\6\5\0\1\127\32\20\2\22\2\20\1\22"+
"\44\20\1\130\1\131\2\0\1\127\1\0\1\132\1\0"+
"\1\133\1\16\1\35\1\2\1\16\1\134\1\40\1\134"+
"\2\135\1\134\1\136\1\134\2\2\1\101\1\2\1\101"+
"\45\2\1\101\5\2\1\137\1\140\1\141\1\142\1\143"+
"\1\0\1\144\6\0\1\145\1\146\33\0\1\147\11\2"+
"\1\150\1\151\105\2\1\101\102\2\1\101\23\2\1\152"+
"\4\2\1\101\1\2\1\152\30\2\1\153\12\2\1\154"+
"\1\64\1\155\1\66\1\0\1\156\1\150\15\0\1\157"+
"\1\40\5\0\1\40\1\0\1\160\1\161\1\162\1\163"+
"\2\124\2\0\1\164\4\0\1\12\14\20\1\22\63\20"+
"\3\0\1\165\1\166\1\35\1\2\1\135\1\0\2\136"+
"\4\2\1\73\23\2\1\167\34\2\71\0\14\2\1\101"+
"\53\2\1\152\22\2\1\152\13\2\1\152\44\2\1\152"+
"\3\2\1\152\27\2\1\101\16\2\1\152\2\2\1\152"+
"\13\2\1\152\16\2\1\152\65\2\1\152\5\2\1\152"+
"\11\2\1\152\61\2\1\152\1\2\1\152\44\2\1\152"+
"\6\2\1\101\15\2\1\35\13\2\25\0\2\124\1\170"+
"\3\0\1\171\1\12\21\20\1\22\17\20\1\22\1\20"+
"\3\0\1\172\1\35\7\2\1\173\4\2\1\174\5\2"+
"\1\73\17\2\2\0\1\1\4\0\1\175\2\0\1\176"+
"\41\0\1\177\24\0\1\200\15\2\1\152\21\2\1\152"+
"\77\2\1\152\3\2\1\152\16\2\1\152\22\2\1\152"+
"\1\2\1\101\11\2\1\201\3\2\1\152\6\2\1\152"+
"\3\2\1\152\15\2\1\152\16\2\1\152\4\2\1\152"+
"\11\2\2\152\12\2\1\152\156\2\1\152\11\2\2\152"+
"\122\2\2\152\24\2\1\152\63\2\1\35\12\2\17\0"+
"\1\101\4\0\2\124\1\126\2\0\1\127\17\20\1\22"+
"\4\20\1\130\1\0\1\127\1\35\5\2\1\101\3\2"+
"\1\101\12\2\1\137\1\143\17\0\1\177\23\0\1\177"+
"\12\0\56\2\1\152\53\2\1\152\44\2\1\152\41\2"+
"\1\152\2\2\3\152\6\2\1\101\135\2\1\152\35\2"+
"\1\152\13\2\1\152\13\2\1\101\1\152\1\101\6\2"+
"\1\152\1\101\251\2\1\152\17\2\1\152\23\2\1\101"+
"\102\2\1\152\13\2\1\152\2\2\1\35\14\2\16\0"+
"\1\124\1\202\12\20\1\0\1\35\13\2\34\0\14\2"+
"\1\152\41\2\1\152\2\2\1\152\31\2\1\152\106\2"+
"\1\152\7\2\1\152\1\2\2\15
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Linux远程命令行工具(Remote Command Line Tools)是Linux操作系统中一种特别实用的工具,可以帮助用户很容易地进行远程控制。由于它具有易用性和广泛适用性,它已经成为Linux系统管理和开发的必备工具。本文将介绍Linux远程命令行工具的快速入门方法,便于初学者可以快速开始使用这些工具。 1. 安装 SSH(Secure Shell)客户端 Linux远程命令行工具的基础是SSH。在使用远程命令行工具之前,首先需要确保SSH客户端已正确安装在本地主机上。SSH客户端 solutions 如 OpenSSH、Putty 等根据自己系统情况自行选择安装。 2. 连接远程主机 使用 SSH 客户端来连接远程主机,只需输入一个命令 : SSH [user]@hostnmae 其中user表示用户名,hostname表示主机名/IP地址。登录远程主机后,使用者可以在远程主机上执行任意命令。 3. 配置SSH 要正确配置SSH,首先应在远程主机上运行以下命令:
资源推荐
资源详情
资源评论
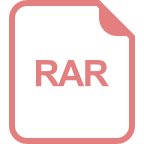
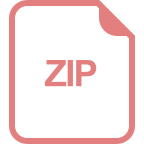
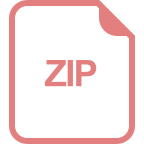
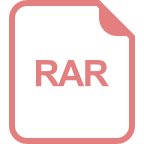
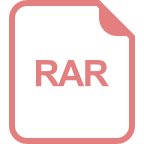
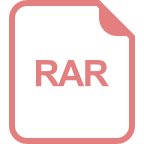
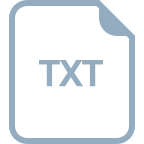
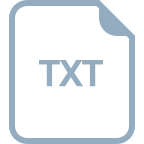
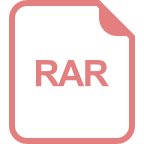
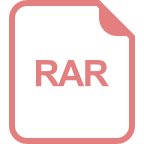
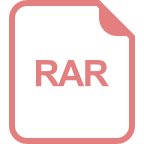
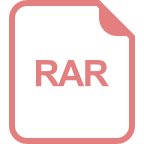
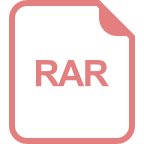
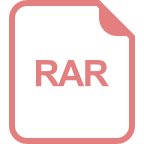
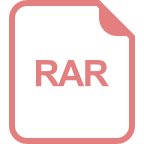
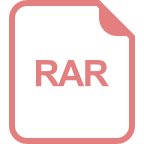
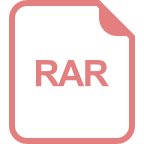
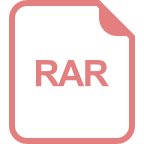
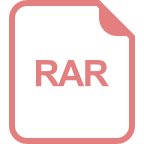
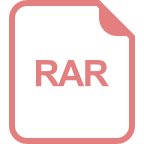
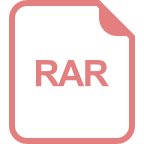
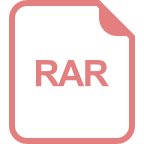
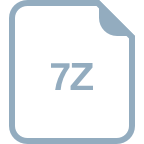
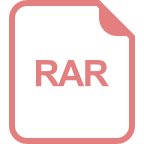
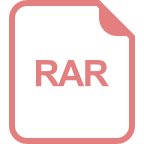
收起资源包目录

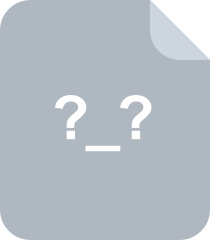
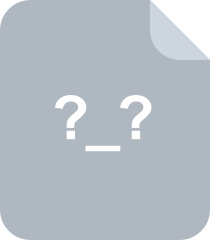
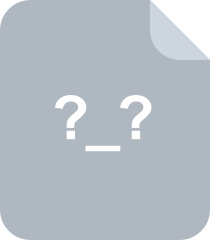
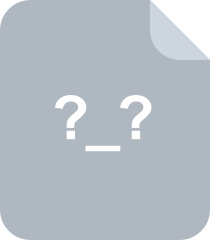
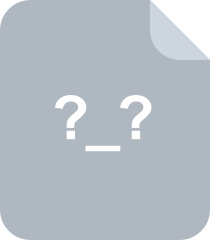
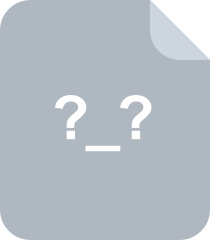
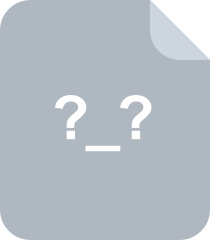
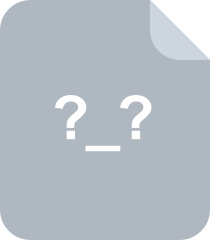
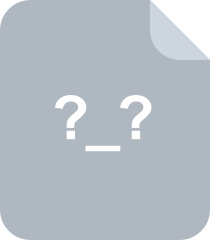
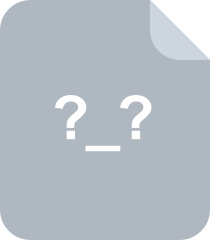
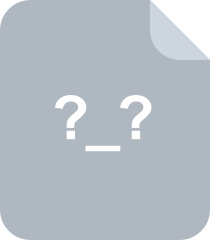
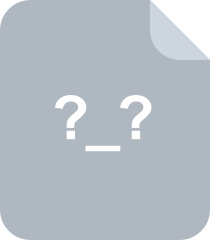
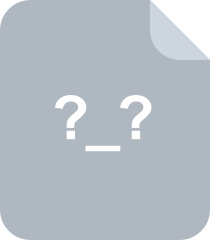
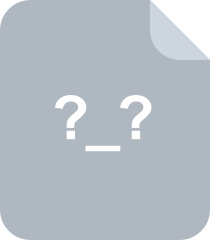
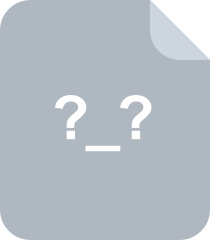
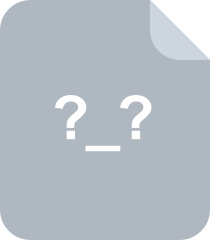
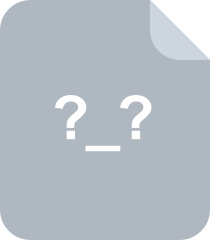
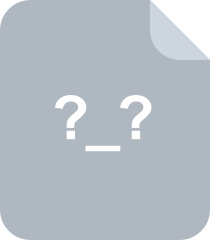
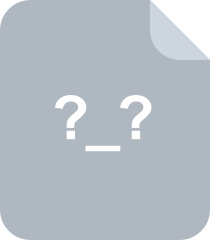
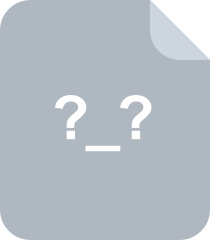
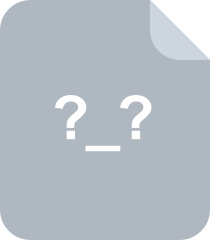
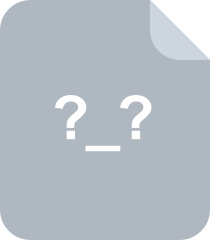
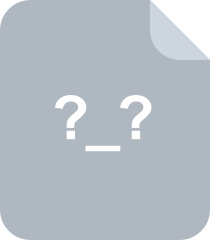
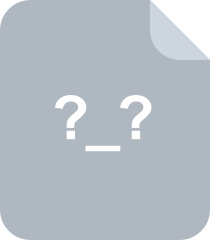
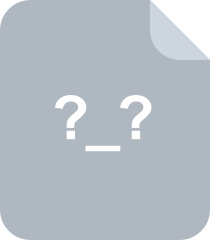
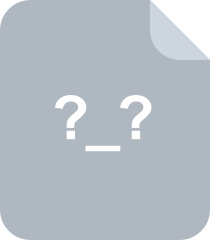
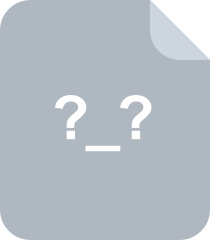
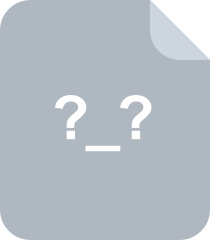
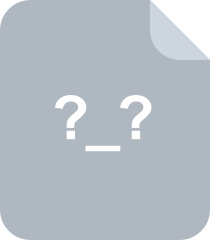
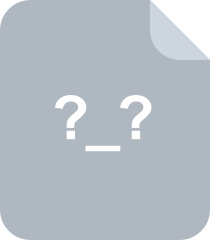
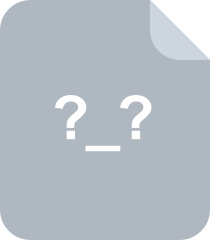
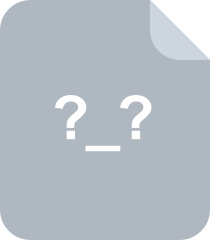
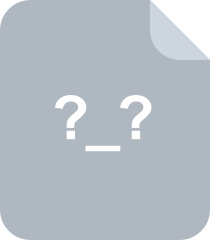
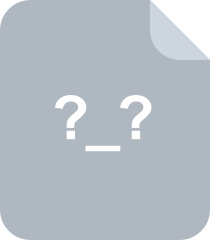
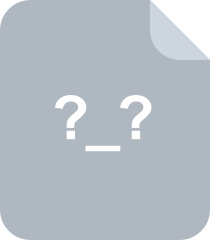
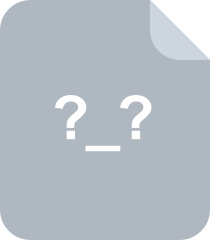
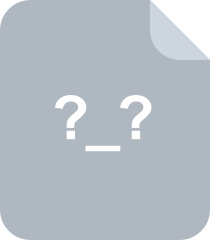
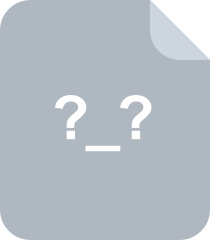
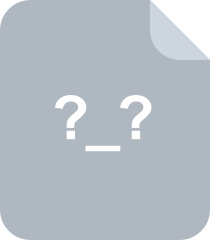
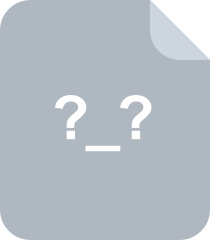
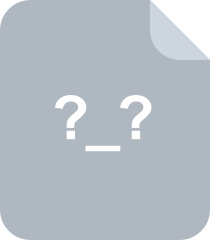
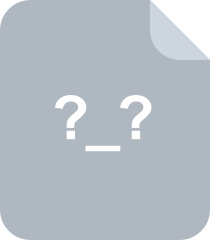
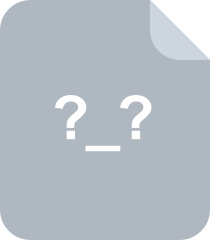
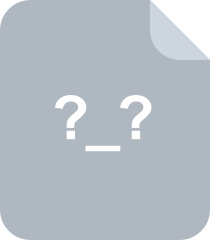
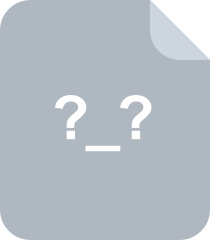
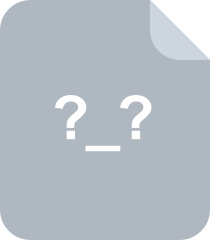
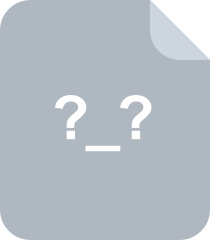
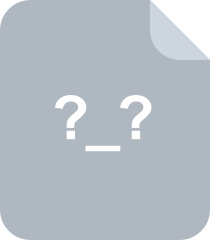
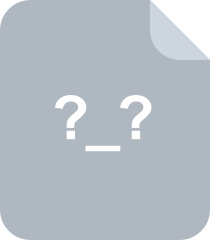
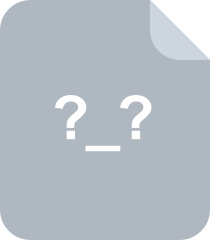
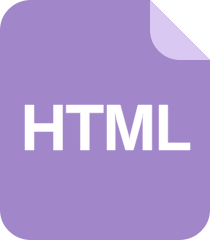
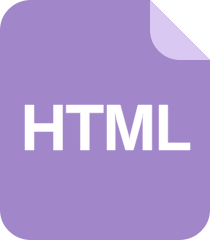
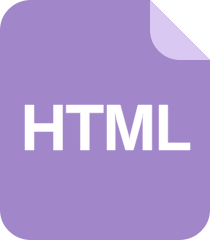
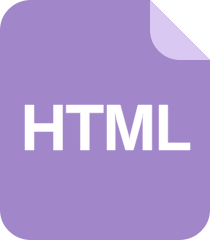
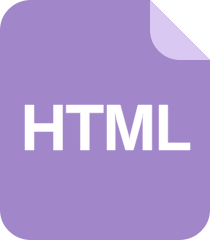
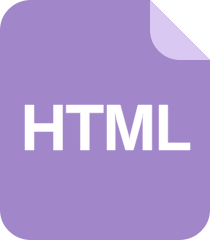
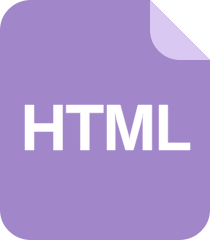
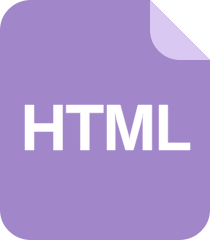
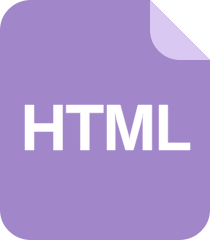
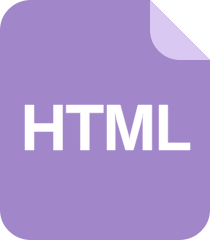
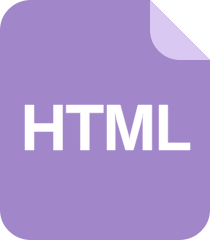
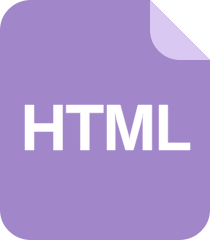
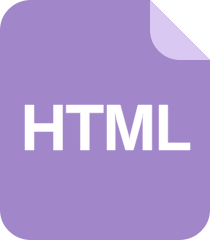
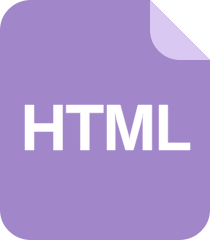
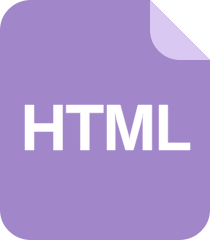
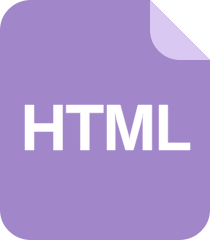
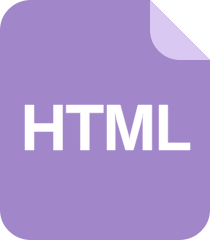
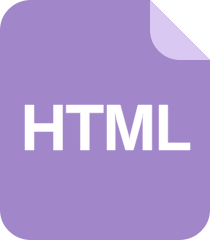
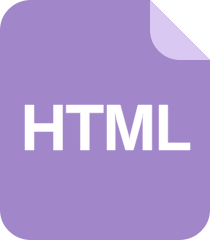
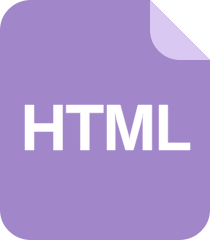
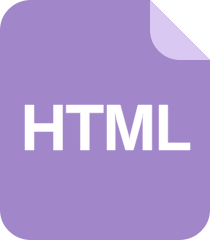
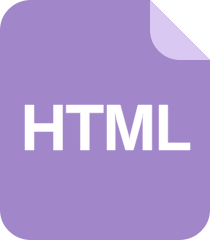
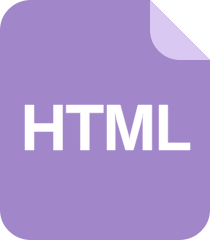
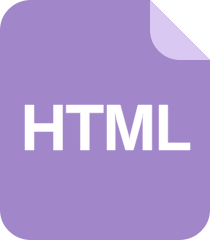
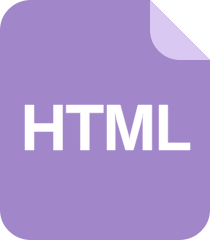
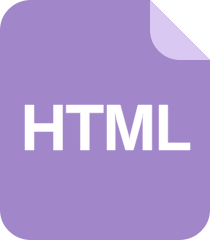
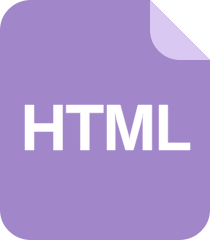
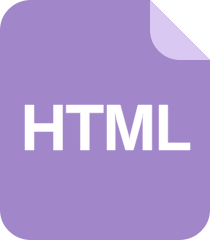
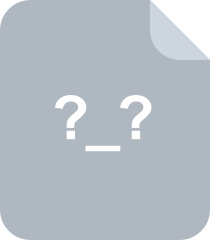
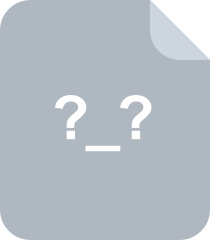
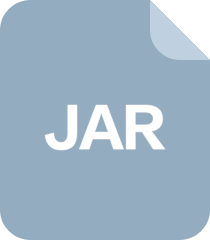
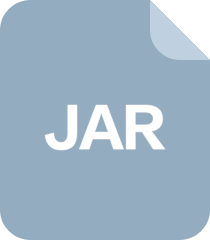
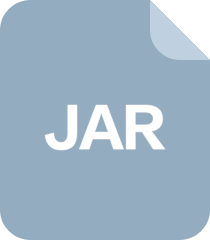
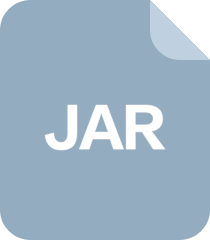
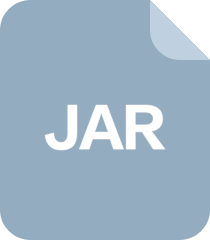
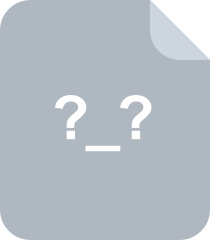
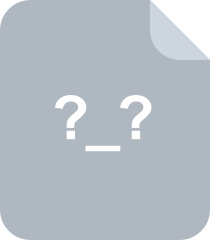
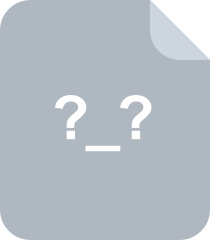
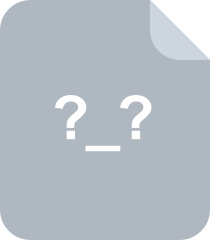
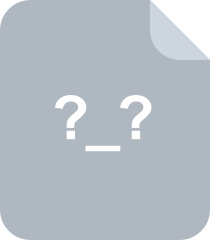
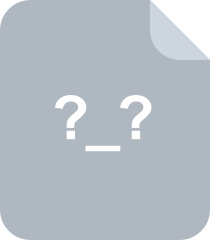
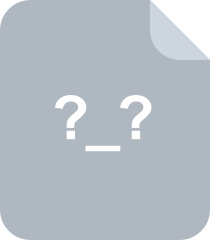
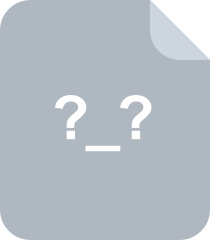
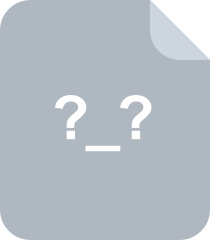
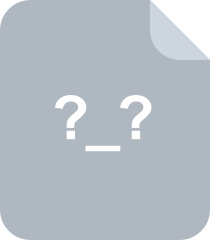
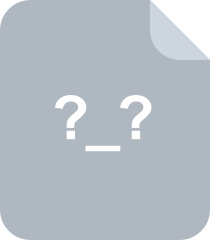
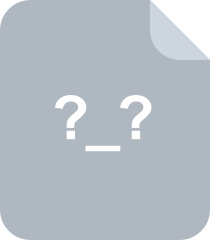
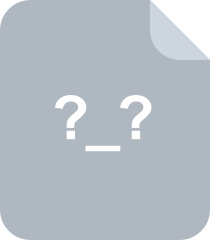
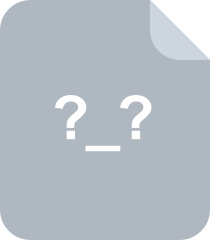
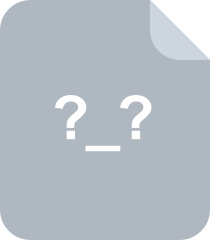
共 1196 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
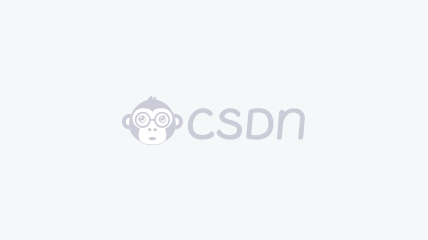
- df5954204692023-07-24这个文件提供了一种方便快捷的方法来管理远程服务器和命令行工具,对于经常处理远程工作的人来说真的很有用。
- 普通网友2023-07-24这个文件具有很好的稳定性,让我在长时间运行的过程中没有遇到任何问题,给我带来了很大的信心和便利。
- 西西里的小裁缝2023-07-24这个Linux远程工具的文件,使用起来非常简单,界面清晰明了,让我在工作中能够快速上手,不再被繁琐的命令行操作所困扰。
- 鸣泣的海猫2023-07-24它不仅可以保存常用命令,还可以保存远程SSH连接,让我在不同服务器间切换变得简单而高效。
- 苗苗小姐2023-07-24它帮助我提高了工作效率,减少了操作失误的可能性,尤其是对于需要频繁切换服务器的工作,简直是一大福音。
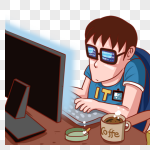
野生的狒狒
- 粉丝: 1497
- 资源: 1530
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

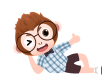
安全验证
文档复制为VIP权益,开通VIP直接复制
