/*
UploadiFive 1.2.2
Copyright (c) 2012 Reactive Apps, Ronnie Garcia
Released under the UploadiFive Standard License <http://www.uploadify.com/uploadifive-standard-license>
*/
;(function($) {
var methods = {
init : function(options) {
return this.each(function() {
// Create a reference to the jQuery DOM object
var $this = $(this);
$this.data('uploadifive', {
inputs : {}, // The object that contains all the file inputs
inputCount : 0, // The total number of file inputs created
fileID : 0,
queue : {
count : 0, // Total number of files in the queue
selected : 0, // Number of files selected in the last select operation
replaced : 0, // Number of files replaced in the last select operation
errors : 0, // Number of files that returned an error in the last select operation
queued : 0, // Number of files added to the queue in the last select operation
cancelled : 0 // Total number of files that have been cancelled or removed from the queue
},
uploads : {
current : 0, // Number of files currently being uploaded
attempts : 0, // Number of file uploads attempted in the last upload operation
successful : 0, // Number of files successfully uploaded in the last upload operation
errors : 0, // Number of files returning errors in the last upload operation
count : 0 // Total number of files uploaded successfully
}
});
var $data = $this.data('uploadifive');
// Set the default options
var settings = $data.settings = $.extend({
'auto' : true, // Automatically upload a file when it's added to the queue
'buttonClass' : false, // A class to add to the UploadiFive button
'buttonText' : 'Select Files', // The text that appears on the UploadiFive button
'checkScript' : false, // Path to the script that checks for existing file names
'dnd' : true, // Allow drag and drop into the queue
'dropTarget' : false, // Selector for the drop target
'fileObjName' : 'Filedata', // The name of the file object to use in your server-side script
'fileSizeLimit' : 0, // Maximum allowed size of files to upload
'fileType' : false, // Type of files allowed (image, etc), separate with a pipe character |
'formData' : {}, // Additional data to send to the upload script
'height' : 30, // The height of the button
'itemTemplate' : false, // The HTML markup for the item in the queue
'method' : 'post', // The method to use when submitting the upload
'multi' : true, // Set to true to allow multiple file selections
'overrideEvents' : [], // An array of events to override
'queueID' : false, // The ID of the file queue
'queueSizeLimit' : 0, // The maximum number of files that can be in the queue
'removeCompleted' : false, // Set to true to remove files that have completed uploading
'simUploadLimit' : 0, // The maximum number of files to upload at once
'truncateLength' : 0, // The length to truncate the file names to
'uploadLimit' : 0, // The maximum number of files you can upload
'uploadScript' : 'uploadifive.php', // The path to the upload script
'width' : 100 // The width of the button
/*
// Events
'onAddQueueItem' : function(file) {}, // Triggered for each file that is added to the queue
'onCancel' : function(file) {}, // Triggered when a file is cancelled or removed from the queue
'onCheck' : function(file, exists) {}, // Triggered when the server is checked for an existing file
'onClearQueue' : function(queue) {}, // Triggered during the clearQueue function
'onDestroy' : function() {} // Triggered during the destroy function
'onDrop' : function(files, numberOfFilesDropped) {}, // Triggered when files are dropped into the file queue
'onError' : function(file, fileType, data) {}, // Triggered when an error occurs
'onFallback' : function() {}, // Triggered if the HTML5 File API is not supported by the browser
'onInit' : function() {}, // Triggered when UploadiFive if initialized
'onQueueComplete' : function() {}, // Triggered once when an upload queue is done
'onProgress' : function(file, event) {}, // Triggered during each progress update of an upload
'onSelect' : function() {}, // Triggered once when files are selected from a dialog box
'onUpload' : function(file) {}, // Triggered when an upload queue is started
'onUploadComplete' : function(file, data) {}, // Triggered when a file is successfully uploaded
'onUploadFile' : function(file) {}, // Triggered for each file being uploaded
*/
}, options);
// Calculate the file size limit
if (isNaN(settings.fileSizeLimit)) {
var fileSizeLimitBytes = parseInt(settings.fileSizeLimit) * 1.024
if (settings.fileSizeLimit.indexOf('KB') > -1) {
settings.fileSizeLimit = fileSizeLimitBytes * 1000;
} else if (settings.fileSizeLimit.indexOf('MB') > -1) {
settings.fileSizeLimit = fileSizeLimitBytes * 1000000;
} else if (settings.fileSizeLimit.indexOf('GB') > -1) {
settings.fileSizeLimit = fileSizeLimitBytes * 1000000000;
}
} else {
settings.fileSizeLimit = settings.fileSizeLimit * 1024;
}
// Create a template for a file input
$data.inputTemplate = $('<input type="file">')
.css({
'font-size' : settings.height + 'px',
'opacity' : 0,
'position' : 'absolute',
'right' : '-3px',
'top' : '-3px',
uploadifive.js 源码.zip
版权申诉
58 浏览量
2023-01-12
10:30:02
上传
评论
收藏 12KB ZIP 举报

GZM888888
- 粉丝: 121
- 资源: 2902
最新资源
- XILINXFPGA源码Xilinxspratan3xcs100E(VGAPS2)
- XILINXFPGA源码XilinxSPARTAN-3E入门开发板实例
- XILINXFPGA源码XilinxSdramVerilog和VHDL版本文档
- 物联网智能家居方案-基于Nucleo-STM32L073&机智云(大赛作品,文档齐全,可直接运行)(文档加Matlab源码)
- XILINXFPGA源码XilinxISE9.xFPGACPLD设计源码
- 成都市地图含高新区(高新南区,高新西区),天府新区,东部新区虚拟行政区划
- XILINXFPGA源码XilinxEDK设计试验
- XILINXFPGA源码XilinxEDKMicroBlaze内置USB固件程序
- 基于 django 的视频点播后台管理系统源代码+数据库
- 基于Java的网上医院预约挂号系统的设计与实现(部署视频)-kaic.mp4
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


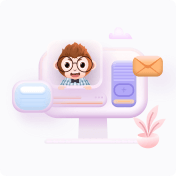