#include <dos.h>
#include <stdio.h>
#include "gformat.h"
#include "genfunc.h"
long cylinder_count;
int head_count,sector_count;
long disk_size,part_size,filespc_size;
unsigned bytes_per_sector;
int sectors_per_cluster;
unsigned number_of_reserved_sectors;
int number_of_FATs;
unsigned maximum_number_of_root_entries;
unsigned total_number_of_sectors;
char media_descriptor;
unsigned sectors_per_FAT;
unsigned sectors_per_track;
unsigned number_of_heads;
long number_of_hidden_sectors;
long volume_id=655627769;
char volume_label[11];
void main(int argc,char * argv[]) /*for example: gformat 2 p 1*/
{
union REGS inregs,outregs;
struct SREGS segs;
BootSector priboot;
DOSBootSector dosboot;
DOSBootSector fpriboot;
FAT16 fat16;
DirEntry * direntry;
int bpl; /* p , P , l , L */
int drive_no; /* 1,2,a*/
int vpno,rpno,ttno; /* Virtual part_no,Real part_no */
int fs;
int speed_idt;
char * sspeed_idt;
char * iddata;
int bfs;
int i,j,k,m;
int bcylinder,bhead,bsector;
int ttcylinder,tthead,ttsector,ttcylinder1,tthead1,ttsector1,ttcylinder2,tthead2,ttsector2;
char tt[2];
FILE * fp;
long ttlogsector;
(void)argc;
bpl=argv[2][0];
drive_no=atoi(argv[1]);
vpno=atoi(argv[3]);
if(drive_no==1)
{
Textout(23,2,"Not test on normal disk");
return;
}
if(bpl==80 || bpl==112)
{
inregs.h.ah=0x8;
inregs.h.dl=0x80+drive_no-1;
int86(0x13,&inregs,&outregs);
head_count=outregs.h.dh+1;
cylinder_count=outregs.h.ch+1+(outregs.h.cl>>6)*256;
sector_count=outregs.h.cl & 63;
disk_size=cylinder_count*sector_count*head_count;
inregs.h.ah=0x2;
inregs.h.al=1;
inregs.h.ch=0;
inregs.h.cl=1;
inregs.h.dh=0;
inregs.h.dl=0x80+drive_no-1;
inregs.x.bx=(unsigned)&priboot;
segread(&segs);
int86x(0x13,&inregs,&outregs,&segs);
rpno=-1;
ttno=0;
for(i=0;i<4;i++)
{
if(priboot.part[i].file_system!=0x0)
{
ttno++;
if(ttno==vpno)
{
rpno=i;
break;
}
}
}
if(rpno==-1)
{
Textout(23,2,"Error part number");
return;
}
part_size=priboot.part[rpno].sector_count;
bytes_per_sector=512;
sectors_per_cluster=8;
number_of_reserved_sectors=1;
number_of_FATs=2;
maximum_number_of_root_entries=512;
total_number_of_sectors=part_size;
media_descriptor=0xf8;
sectors_per_track=sector_count;
number_of_heads=head_count;
number_of_hidden_sectors=priboot.part[rpno].first_sector<=sector_count?
sector_count:0;
sectors_per_FAT=2+((part_size-
number_of_reserved_sectors-
maximum_number_of_root_entries*32/bytes_per_sector)/
sectors_per_cluster*
2)/bytes_per_sector;
filespc_size=part_size-
number_of_reserved_sectors-
maximum_number_of_root_entries*32/bytes_per_sector-
sectors_per_FAT*number_of_FATs;
/*----------------Check all cylinders ------------------------------*/
sspeed_idt=(char *)malloc(5);
bcylinder=priboot.part[rpno].beginning_cylinder;
bhead=priboot.part[rpno].beginning_head;
bsector=1;
ttcylinder=bcylinder;
tthead=bhead;
ttsector=bsector;
/*********check DOSBoot and FAT and Root Entry sectors ************/
for(i=0;i<number_of_reserved_sectors+
sectors_per_FAT*number_of_FATs+
maximum_number_of_root_entries*32/bytes_per_sector;
i++)
{
inregs.h.ah=0x4;
inregs.h.al=1;
inregs.h.ch=ttcylinder;
inregs.h.cl=ttsector;
inregs.h.dh=tthead;
inregs.h.dl=0x80+drive_no-1;
int86x(0x13,&inregs,&outregs,&segs);
if(outregs.h.ah!=0x0)
{
if(i<number_of_reserved_sectors)
Textout(23,2,"Boot Sector destroyed! Can't format!");
else if(i<sectors_per_FAT*number_of_FATs)
Textout(23,2,"FAT Sectors destroyed! Can't format!");
else
Textout(23,2,"Root Entry Sectors destroyed! Can't format!");
return;
}
ttsector++;
if(ttsector>sector_count)
{
ttsector=1;
tthead++;
if(tthead>head_count-1)
{
tthead=0;
ttcylinder++;
}
}
}
/************check file section sector***********/
ttcylinder1=bcylinder;
tthead1=bhead;
ttsector1=bsector+1;
ttcylinder2=bcylinder+(bsector+1+sectors_per_FAT-1)/sector_count/head_count;
tthead2=(bhead+(bsector+1+sectors_per_FAT-1)/sector_count)%head_count;
ttsector2=(bsector+1+sectors_per_FAT-1)%sector_count+1;
for(i=0;i<bytes_per_sector/2;i++)
fat16.item[i]=0xfff0;
for(i=0;i<filespc_size/sectors_per_cluster;i++)
{
inregs.h.ah=0x4;
inregs.h.al=sectors_per_cluster;
inregs.h.ch=ttcylinder;
inregs.h.cl=ttsector;
inregs.h.dh=tthead;
inregs.h.dl=0x80+drive_no-1;
int86x(0x13,&inregs,&outregs,&segs);
if(i==0)
{
fat16.item[0]=0xff+media_descriptor<<8;
fat16.item[1]=0xffff;
}
if(outregs.h.ah!=0x0)
{
fat16.item[(i+2)%(bytes_per_sector/2)]=0xfff7;
}
else
{
fat16.item[(i+2)%(bytes_per_sector/2)]=0x0;
}
if((i>0 && (i+1)*2%bytes_per_sector==0) || (i>=filespc_size/sectors_per_cluster-1))
{
inregs.h.ah=0x3;
inregs.h.al=1;
inregs.h.ch=ttcylinder1;
inregs.h.cl=ttsector1;
inregs.h.dh=tthead1;
inregs.h.dl=0x80+drive_no-1;
inregs.x.bx=(unsigned)&fat16;
segread(&segs);
int86x(0x13,&inregs,&outregs,&segs);
inregs.h.ah=0x3;
inregs.h.al=1;
inregs.h.ch=ttcylinder2;
inregs.h.cl=ttsector2;
inregs.h.dh=tthead2;
inregs.h.dl=0x80+drive_no-1;
inregs.x.bx=(unsigned)&fat16;
segread(&segs);
int86x(0x13,&inregs,&outregs,&segs);
ttsector1++;
if(ttsector1>sector_count)
{
ttsector1=1;
tthead1++;
if(tthead1>head_count-1)
{
tthead1=0;
ttcylinder1++;
}
}
ttsector2++;
if(ttsector2>sector_count)
{
ttsector2=1;
tthead2++;
if(tthead2>head_count-1)
{
tthead2=0;
ttcylinder2++;
}
}
for(k=0;k<256;k++)
fat16.item[k]=0xfff0;
}
ttsector+=sectors_per_cluster;
if(ttsector>sector_count)
{
ttsector=1;
tthead++;
if(tthead>head_count-1)
{
tthead=0;
ttcylinder++;
}
}
speed_idt=(i+1)/(filespc_size/sectors_per_cluster/100);
speed_idt=speed_idt<=100?speed_idt:100;
itoa(speed_idt,sspeed_idt,10);
strcat(sspeed_idt,"%");
inregs.h.ah=0x2;
inregs.h.bh=0;
inregs.h.dh=23;
inregs.h.dl=0;
int86(0x10,&inregs,&outregs);
while(*sspeed_idt)
{
inregs.h.ah=0xE;
inregs.h.bh=0;
inregs.h.bl=16;
inregs.h.al=*sspeed_idt++;
int86(0x10,&inregs,&outregs);
}
}
/*---------------- DOS Boot Sector ------------------------------*/
dosboot.jmp_instruction[0]=-21;
dosboot.jmp_instruction[1]=60;
dosboot.jmp_instruction[2]=-112;
strcpy(dosboot.oem_name,"XMG 1.0");
dosboot.bpb.bytes_per_sector=bytes_per_sector;
dosboot.bpb.sectors_per_cluster=sectors_per_cluster;
dosboot.bpb.number_of_reserved_sectors=number_of_reserved_sectors;
dosboot.bpb.number_of_FATs=number_of_FATs;
dosboot.bpb.maximum_number_of_root_entries=maximum_number_of_root_entries;
if(part_size<=32*(1048576/512))
{
dosboot.bpb.total_number_of_sectors=total_number_of_sectors;
dosboot.huge_sectors=0;
}
else
{
dosboot.bpb.total_number_of_sectors=0;
dosboot.huge_sectors=total_number_of_sectors;
}
dosboot.bpb.media_descriptor=media_descriptor;
dosboot.bpb.sectors_per_FAT=sectors_per_FAT;
dosboot.bpb.sectors_per_track=sectors_per_track;
dosboot.bpb.number_of_heads=number_of_heads;
dosboot.bpb.number_of_hidden_sectors=number_of_hidden_sectors;
dosboot.drive_no=0x80+drive_no-1;
dosboot.boot_signature=0x29;
dosboot.volume_id=volume_id;
strcpy(dosboot.volume_label,"");
strcpy(dosboot.file_system_type,"FAT16");
fp=fopen("\\os\\format\\load.bin","rb");
if(fp==
没有合适的资源?快使用搜索试试~ 我知道了~
用C实现的硬盘格式化程序
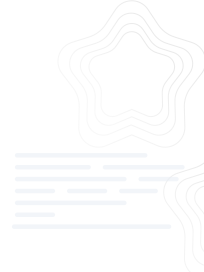
共13个文件
c:2个
h:2个
obj:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 76 浏览量
2022-06-22
10:19:08
上传
评论
收藏 27KB RAR 举报
温馨提示
用C实现的硬盘格式化程序-C formatted drives to achieve procedures
资源推荐
资源详情
资源评论
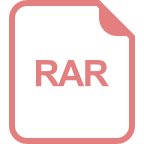
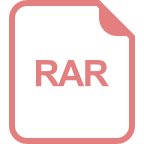
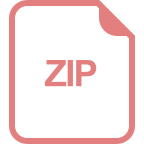
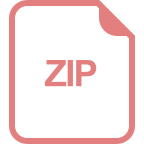
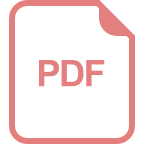
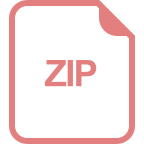
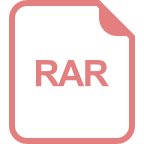
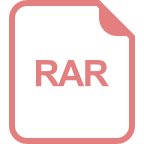
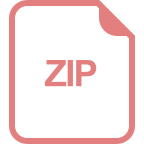
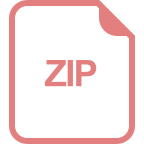
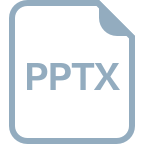
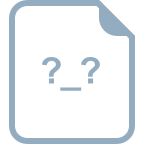
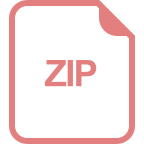
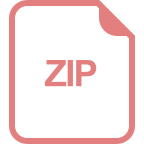
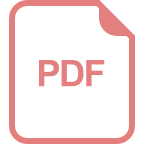
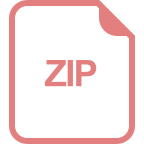
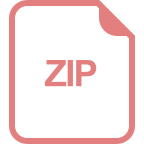
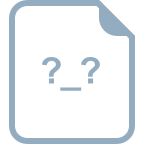
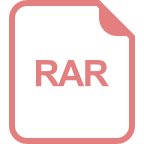
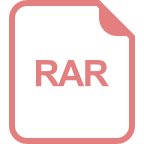
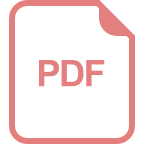
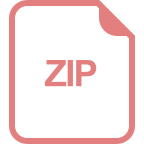
收起资源包目录


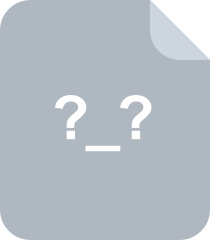
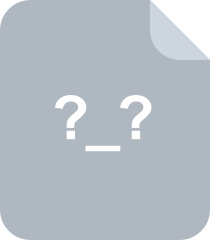
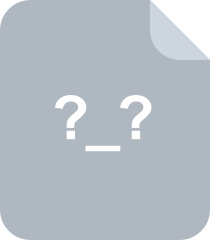
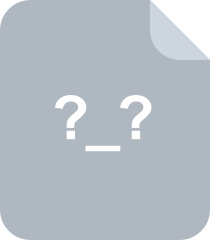
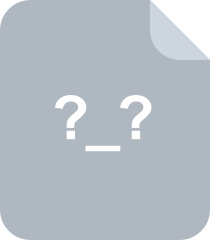
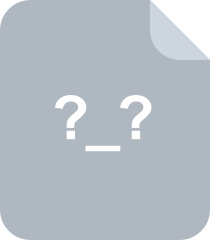
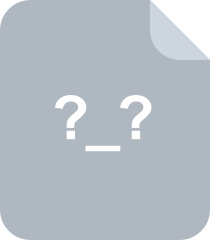
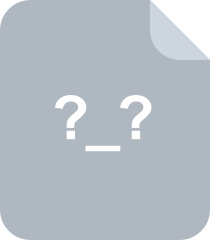
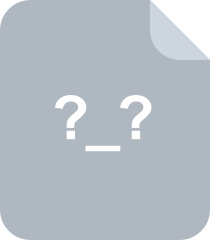
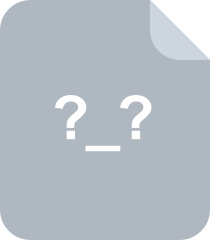
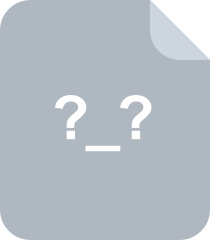
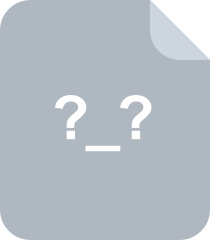
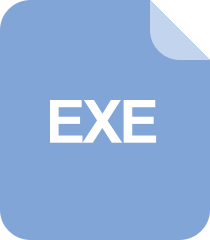
共 13 条
- 1
资源评论
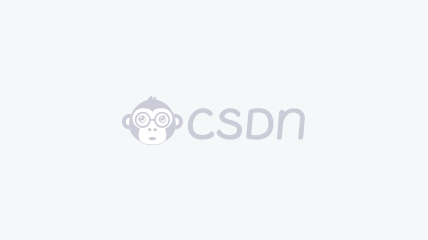

GZM888888
- 粉丝: 144
- 资源: 2912
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

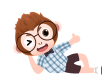
安全验证
文档复制为VIP权益,开通VIP直接复制
