/*++
Module Name:
intpnp.c
Abstract:
Plug and Play module.
This file contains routines to handle pnp requests.
These routines are not USB specific but is required
for every driver which conforms to the WDM model.
Environment:
Kernel mode
Notes:
--*/
#include "rtcapi.h"
#include "intusb.h"
#include "intpnp.h"
#include "intpwr.h"
#include "intdev.h"
#include "intrwr.h"
#include "intwmi.h"
#include "intusr.h"
#ifdef WIN98DRIVER
#include "intwdm98.h"
#endif
NTSTATUS
IntUsb_DispatchPnP(
IN PDEVICE_OBJECT DeviceObject,
IN PIRP Irp
)
/*++
Routine Description:
The plug and play dispatch routines.
Most of these requests the driver will completely ignore.
In all cases it must pass on the IRP to the lower driver.
Arguments:
DeviceObject - pointer to a device object.
Irp - pointer to an I/O Request Packet.
Return Value:
NT status value
--*/
{
PIO_STACK_LOCATION irpStack;
PDEVICE_EXTENSION deviceExtension;
KEVENT startDeviceEvent;
NTSTATUS ntStatus;
//
// initialize variables
//
irpStack = IoGetCurrentIrpStackLocation(Irp);
deviceExtension = DeviceObject->DeviceExtension;
//
// since the device is removed, fail the Irp.
//
if(Removed == deviceExtension->DeviceState) {
ntStatus = STATUS_DELETE_PENDING;
Irp->IoStatus.Status = ntStatus;
Irp->IoStatus.Information = 0;
IoCompleteRequest(Irp, IO_NO_INCREMENT);
return ntStatus;
}
KdPrint( ("///////////////////////////////////////////\n"));
KdPrint( ("IntUsb_DispatchPnP::"));
IntUsb_IoIncrement(deviceExtension);
if(irpStack->MinorFunction == IRP_MN_START_DEVICE) {
ASSERT(deviceExtension->IdleReqPend == 0);
}
else {
if(deviceExtension->SSEnable) {
CancelSelectSuspend(deviceExtension);
}
}
KdPrint( (PnPMinorFunctionString(irpStack->MinorFunction)));
switch(irpStack->MinorFunction) {
case IRP_MN_START_DEVICE:
ntStatus = HandleStartDevice(DeviceObject, Irp);
break;
case IRP_MN_QUERY_STOP_DEVICE:
//
// if we cannot stop the device, we fail the query stop irp
//
ntStatus = CanStopDevice(DeviceObject, Irp);
if(NT_SUCCESS(ntStatus)) {
ntStatus = HandleQueryStopDevice(DeviceObject, Irp);
return ntStatus;
}
break;
case IRP_MN_CANCEL_STOP_DEVICE:
ntStatus = HandleCancelStopDevice(DeviceObject, Irp);
break;
case IRP_MN_STOP_DEVICE:
ntStatus = HandleStopDevice(DeviceObject, Irp);
KdPrint( ("IntUsb_DispatchPnP::IRP_MN_STOP_DEVICE::"));
IntUsb_IoDecrement(deviceExtension);
return ntStatus;
case IRP_MN_QUERY_REMOVE_DEVICE:
//
// if we cannot remove the device, we fail the query remove irp
//
ntStatus = HandleQueryRemoveDevice(DeviceObject, Irp);
return ntStatus;
case IRP_MN_CANCEL_REMOVE_DEVICE:
ntStatus = HandleCancelRemoveDevice(DeviceObject, Irp);
break;
case IRP_MN_SURPRISE_REMOVAL:
ntStatus = HandleSurpriseRemoval(DeviceObject, Irp);
KdPrint( ("IntUsb_DispatchPnP::IRP_MN_SURPRISE_REMOVAL::"));
IntUsb_IoDecrement(deviceExtension);
return ntStatus;
case IRP_MN_REMOVE_DEVICE:
ntStatus = HandleRemoveDevice(DeviceObject, Irp);
return ntStatus;
case IRP_MN_QUERY_CAPABILITIES:
ntStatus = HandleQueryCapabilities(DeviceObject, Irp);
break;
default:
IoSkipCurrentIrpStackLocation(Irp);
ntStatus = IoCallDriver(deviceExtension->TopOfStackDeviceObject, Irp);
KdPrint( ("IntUsb_DispatchPnP::default::"));
IntUsb_IoDecrement(deviceExtension);
return ntStatus;
} // switch
//
// complete request
//
Irp->IoStatus.Status = ntStatus;
Irp->IoStatus.Information = 0;
IoCompleteRequest(Irp, IO_NO_INCREMENT);
//
// decrement count
//
KdPrint( ("IntUsb_DispatchPnP::"));
IntUsb_IoDecrement(deviceExtension);
return ntStatus;
}
NTSTATUS
HandleStartDevice(
IN PDEVICE_OBJECT DeviceObject,
IN PIRP Irp
)
/*++
Routine Description:
This is the dispatch routine for IRP_MN_START_DEVICE
Arguments:
DeviceObject - pointer to a device object.
Irp - I/O request packet
Return Value:
NT status value
--*/
{
KIRQL oldIrql;
KEVENT startDeviceEvent;
NTSTATUS ntStatus;
PDEVICE_EXTENSION deviceExtension;
LARGE_INTEGER dueTime;
KdPrint( ("HandleStartDevice - begins\n"));
//
// initialize variables
//
deviceExtension = (PDEVICE_EXTENSION) DeviceObject->DeviceExtension;
deviceExtension->UsbConfigurationDescriptor = NULL;
deviceExtension->UsbInterface = NULL;
deviceExtension->PipeContext = NULL;
//
// We cannot touch the device (send it any non pnp irps) until a
// start device has been passed down to the lower drivers.
// first pass the Irp down
//
KeInitializeEvent(&startDeviceEvent, NotificationEvent, FALSE);
IoCopyCurrentIrpStackLocationToNext(Irp);
IoSetCompletionRoutine(Irp,
(PIO_COMPLETION_ROUTINE)IrpCompletionRoutine,
(PVOID)&startDeviceEvent,
TRUE,
TRUE,
TRUE);
ntStatus = IoCallDriver(deviceExtension->TopOfStackDeviceObject, Irp);
if(ntStatus == STATUS_PENDING) {
KeWaitForSingleObject(&startDeviceEvent,
Executive,
KernelMode,
FALSE,
NULL);
ntStatus = Irp->IoStatus.Status;
}
if(!NT_SUCCESS(ntStatus)) {
KdPrint( ("Lower drivers failed this Irp\n"));
return ntStatus;
}
//
// Read the device descriptor, configuration descriptor
// and select the interface descriptors
//
ntStatus = ReadandSelectDescriptors(DeviceObject);
if(!NT_SUCCESS(ntStatus)) {
KdPrint( ("ReadandSelectDescriptors failed\n"));
return ntStatus;
}
//
// enable the symbolic links for system components to open
// handles to the device
//
ntStatus = IoSetDeviceInterfaceState(&deviceExtension->InterfaceName,
TRUE);
if(!NT_SUCCESS(ntStatus)) {
KdPrint( ("IoSetDeviceInterfaceState:enable:failed\n"));
return ntStatus;
}
KeAcquireSpinLock(&deviceExtension->DevStateLock, &oldIrql);
SET_NEW_PNP_STATE(deviceExtension, Working);
deviceExtension->QueueState = AllowRequests;
KeReleaseSpinLock(&deviceExtension->DevStateLock, oldIrql);
//
// initialize wait wake outstanding flag to false.
// and issue a wait wake.
deviceExtension->FlagWWOutstanding = 0;
deviceExtension->FlagWWCancel = 0;
deviceExtension->WaitWakeIrp = NULL;
if(deviceExtension->WaitWakeEnable) {
IssueWaitWake(deviceExtension);
}
ProcessQueuedRequests(deviceExtension);
if(WinXpOrBetter == deviceExtension->WdmVersion) {
deviceExtension->SSEnable = deviceExtension->SSRegistryEnable;
//
// set timer.for selective suspend requests
//
if(deviceExtension->SSEnable) {
dueTime.QuadPart = -10000 * IDLE_INTERVAL; // 5000 ms
KeSetTimerEx(&deviceExtension->Timer,
due
没有合适的资源?快使用搜索试试~ 我知道了~
C8051F单片机全系列(24款型号)参考程序例程源代码.rar
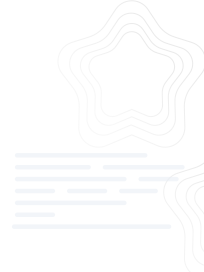
共1860个文件
c:1038个
h:341个
wsp:68个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
C8051F单片机全系列(24款型号)参考程序例程源代码,可供学习及设计参考。 C8051F00x_01x C8051F02x C8051F04x C8051F06x C8051F12x_13x C8051F2xx C8051F30x C8051F31x C8051F320_1 C8051F326_7 C8051F330_5 C8051F336_9 C8051F34x C8051F35x C8051F36x C8051F41x C8051F50x_51x C8051F52xA_53xA C8051F58x_59x C8051F70x_71x C8051F93x_92x C8051T60x C8051T61x C8051T63x
资源推荐
资源详情
资源评论
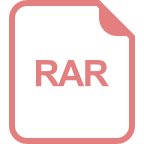
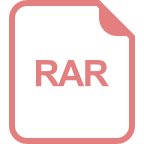
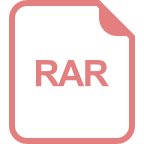
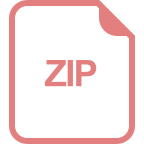
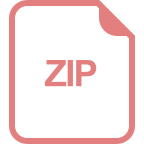
收起资源包目录

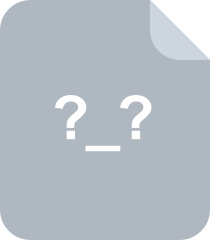
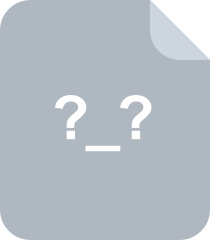
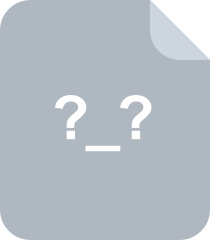
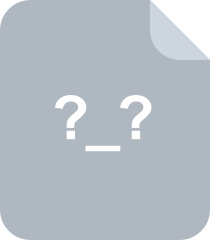
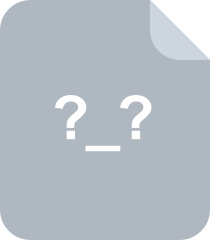
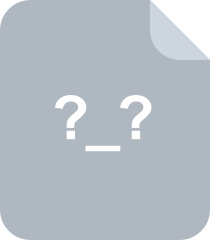
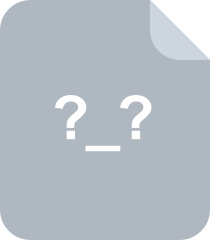
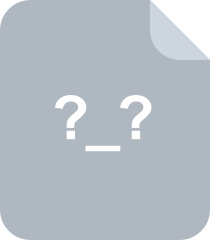
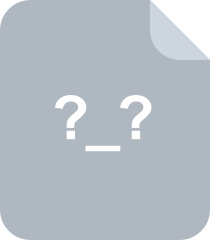
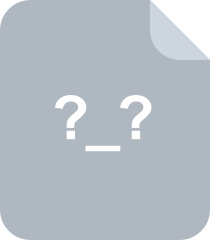
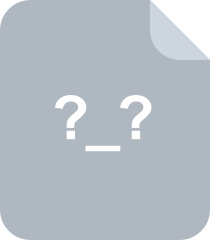
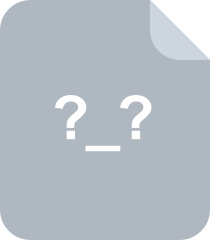
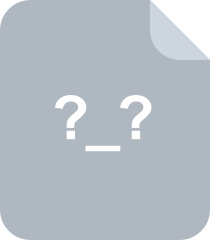
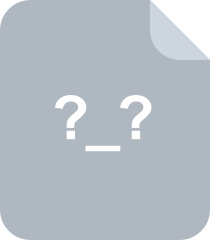
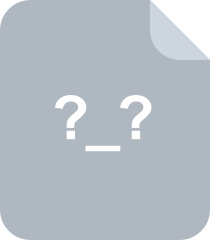
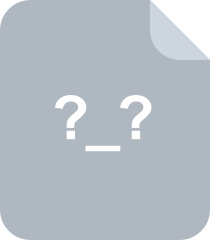
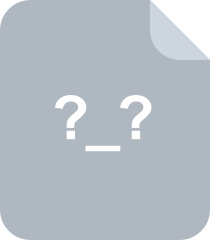
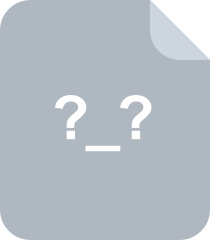
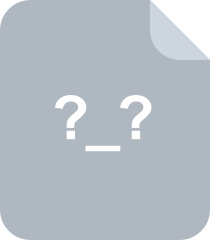
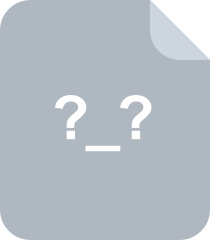
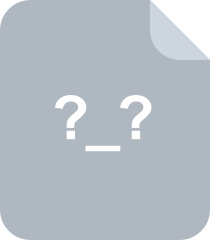
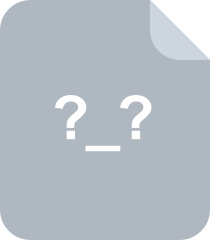
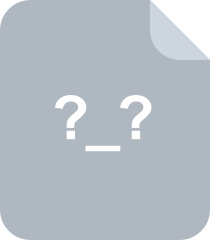
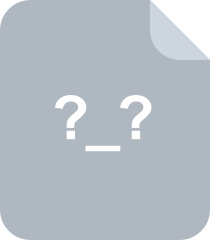
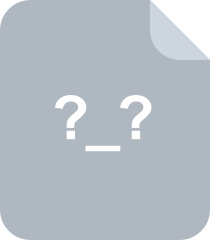
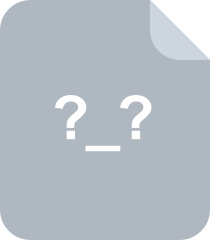
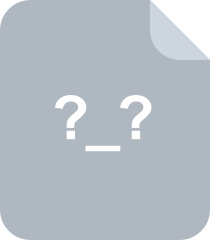
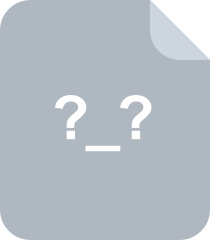
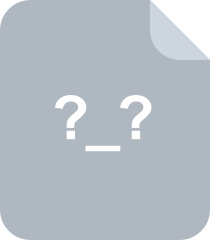
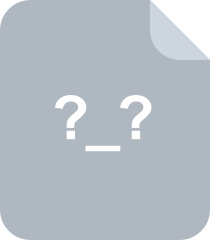
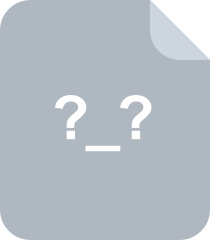
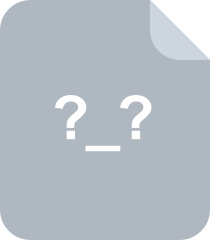
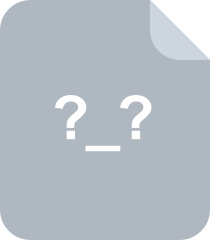
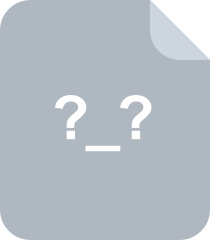
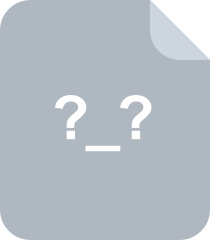
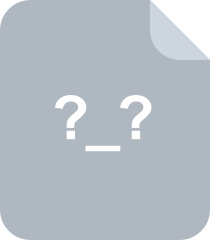
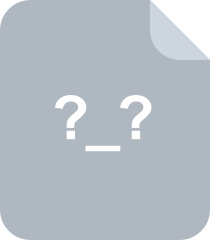
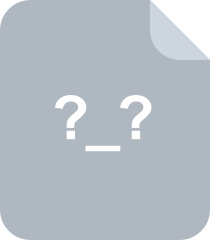
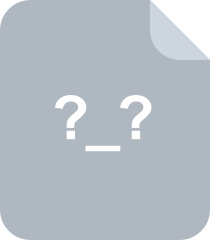
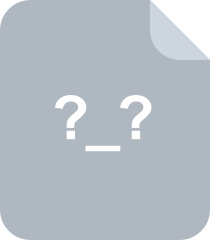
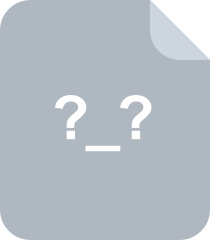
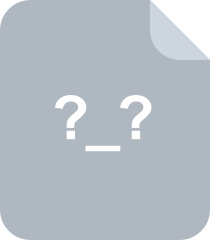
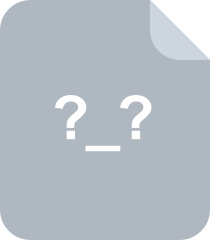
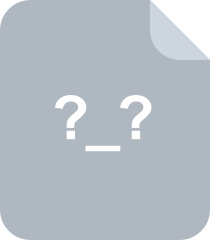
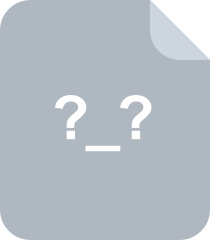
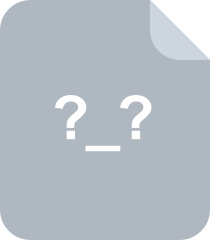
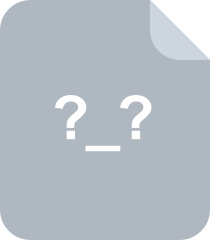
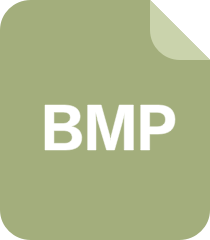
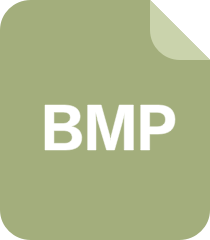
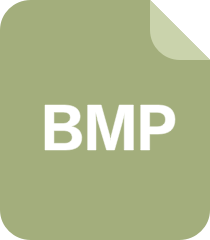
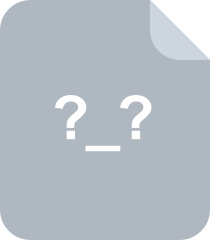
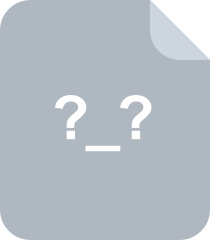
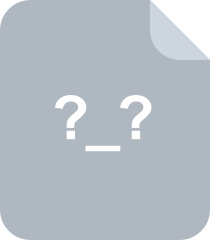
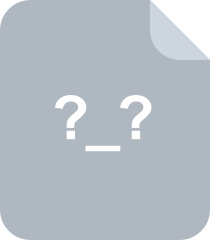
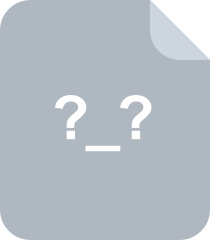
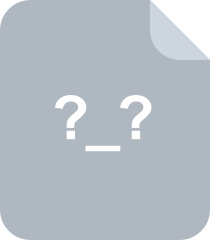
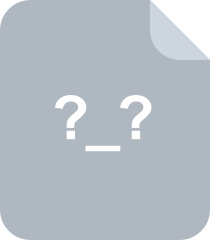
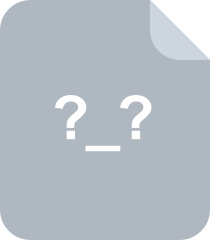
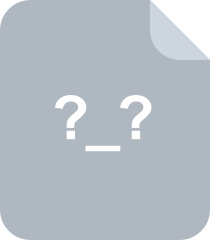
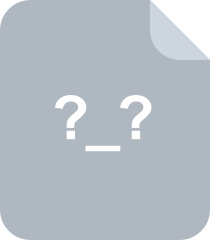
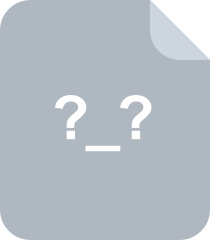
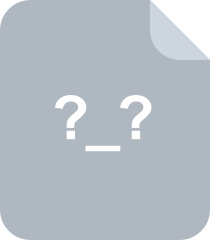
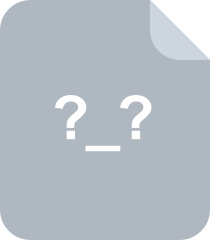
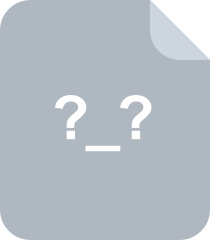
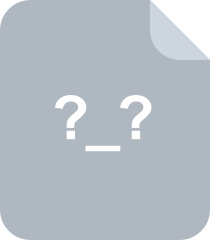
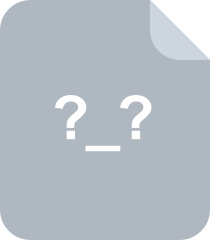
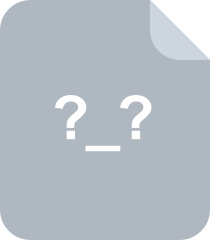
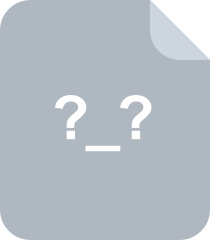
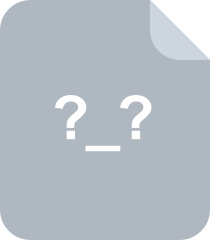
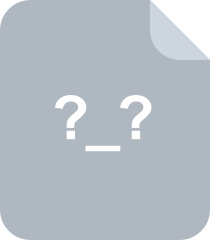
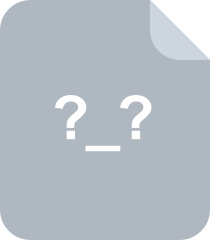
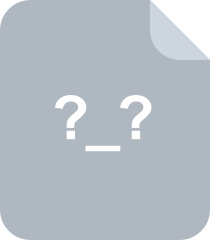
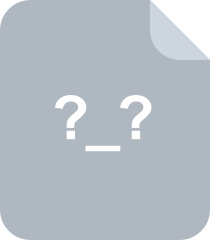
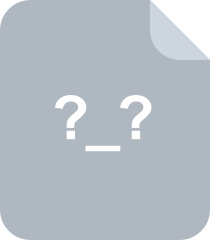
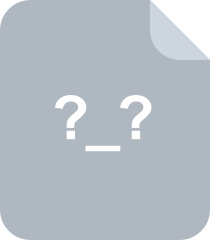
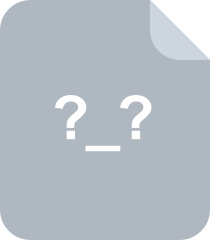
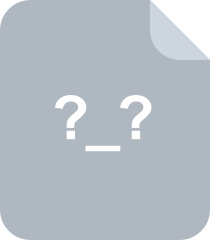
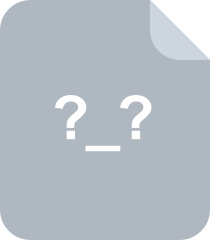
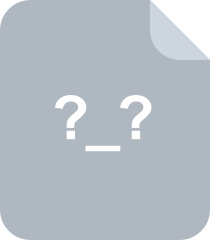
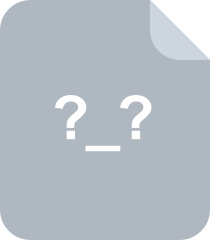
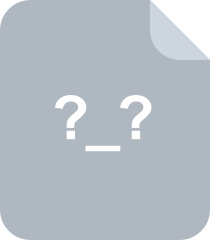
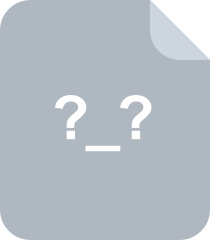
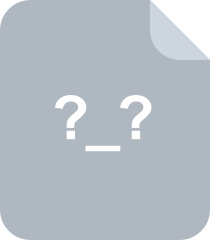
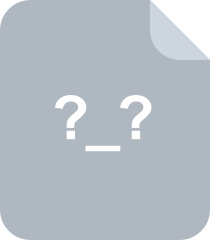
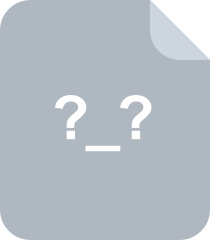
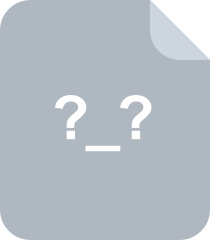
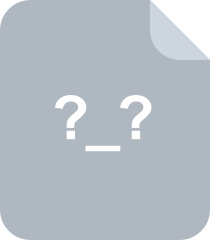
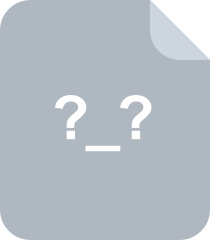
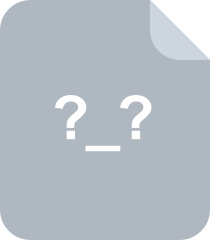
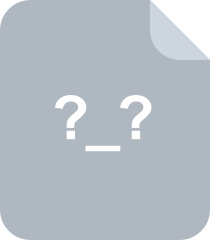
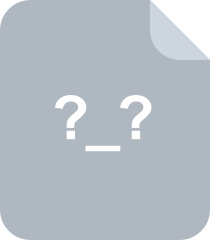
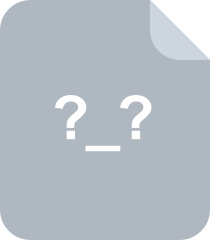
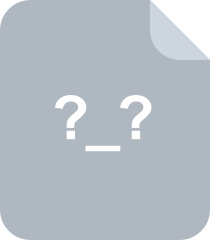
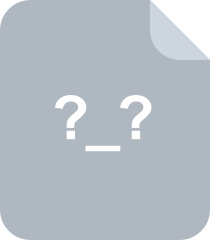
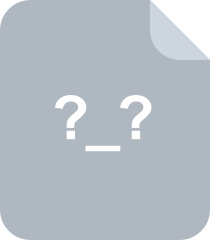
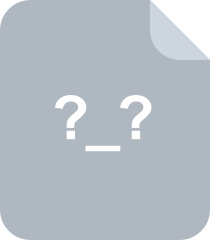
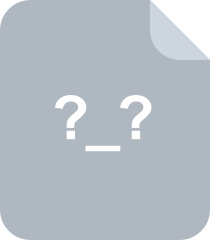
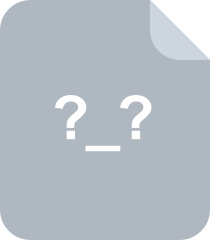
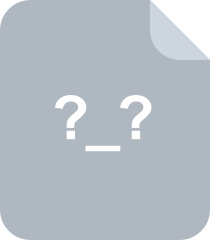
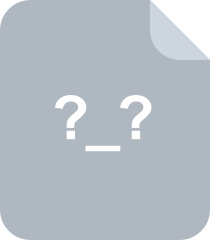
共 1860 条
- 1
- 2
- 3
- 4
- 5
- 6
- 19
资源评论
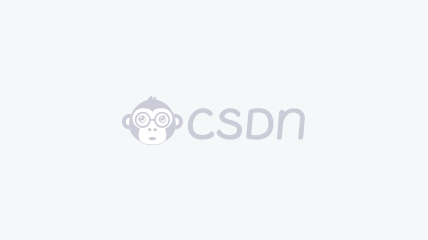
- wushiwang0002024-01-15资源内容详实,描述详尽,解决了我的问题,受益匪浅,学到了。
- weixin_506511132023-11-13资源内容详细,总结地很全面,与描述的内容一致,对我启发很大,学习了。
- weixin_392885402024-02-15感谢大佬分享的资源给了我灵感,果断支持!感谢分享~
- lixiyuan198808072023-11-09资源很实用,内容详细,值得借鉴的内容很多,感谢分享。

GJZGRB
- 粉丝: 1008
- 资源: 5700

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

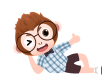
安全验证
文档复制为VIP权益,开通VIP直接复制
