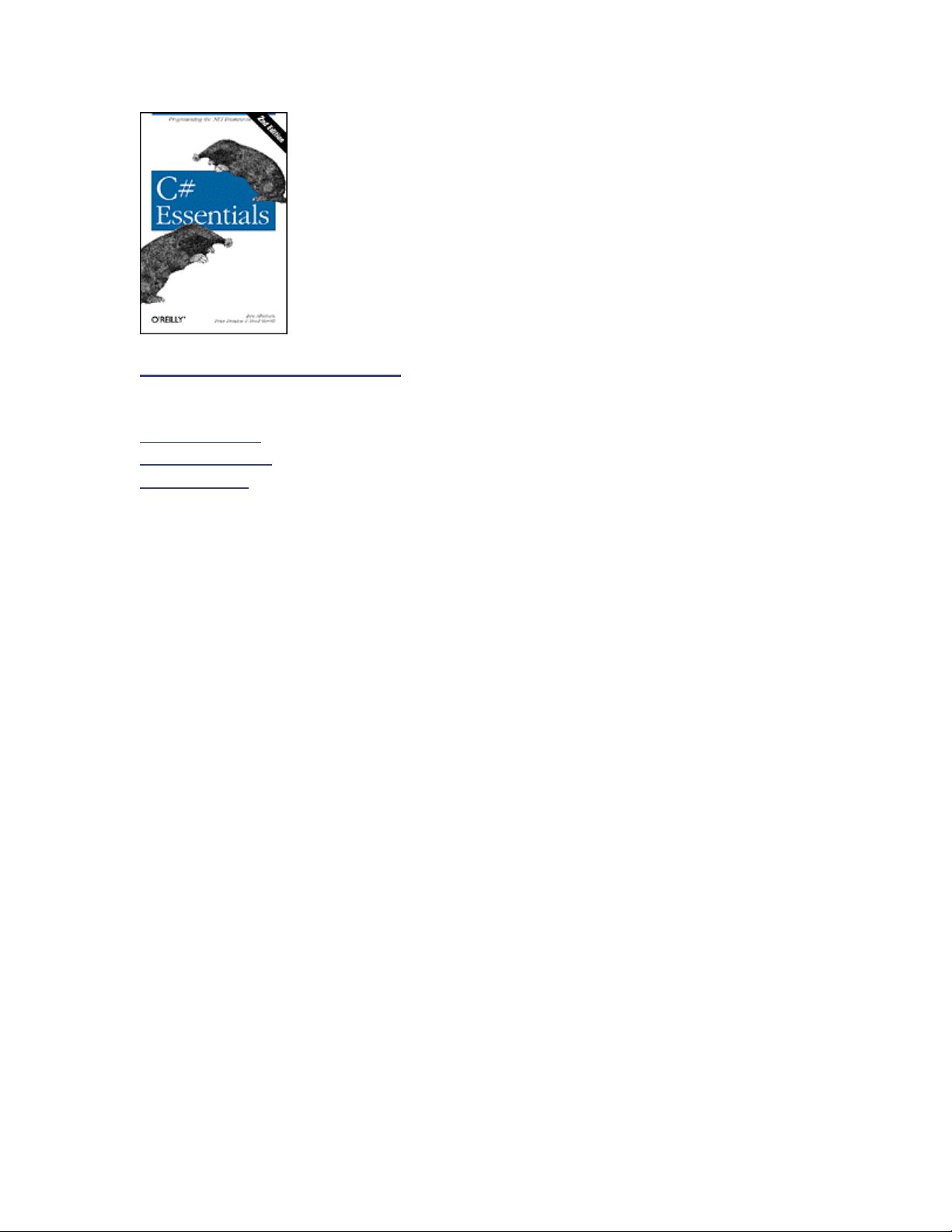
C# Essentials, 2nd Edition
Ben Albahari
Peter Drayton
Brad Merrill
Publisher: O'Reilly
Second Edition February 2001
ISBN: 0-596-00315-3, 216 pages
Concise but thorough, this second edition of C# Essentials introduces the Microsoft C#
programming language, including the Microsoft .NET Common Language Runtime (CLR) and
.NET Framework Class Libraries (FCL) that support it. This book's compact format and terse
presentation of key concepts serve as a roadmap to the online documentation included with the
Microsoft .NET Framework SDK; the many examples provide much-needed context.
My Release J 2002
For OR Forum
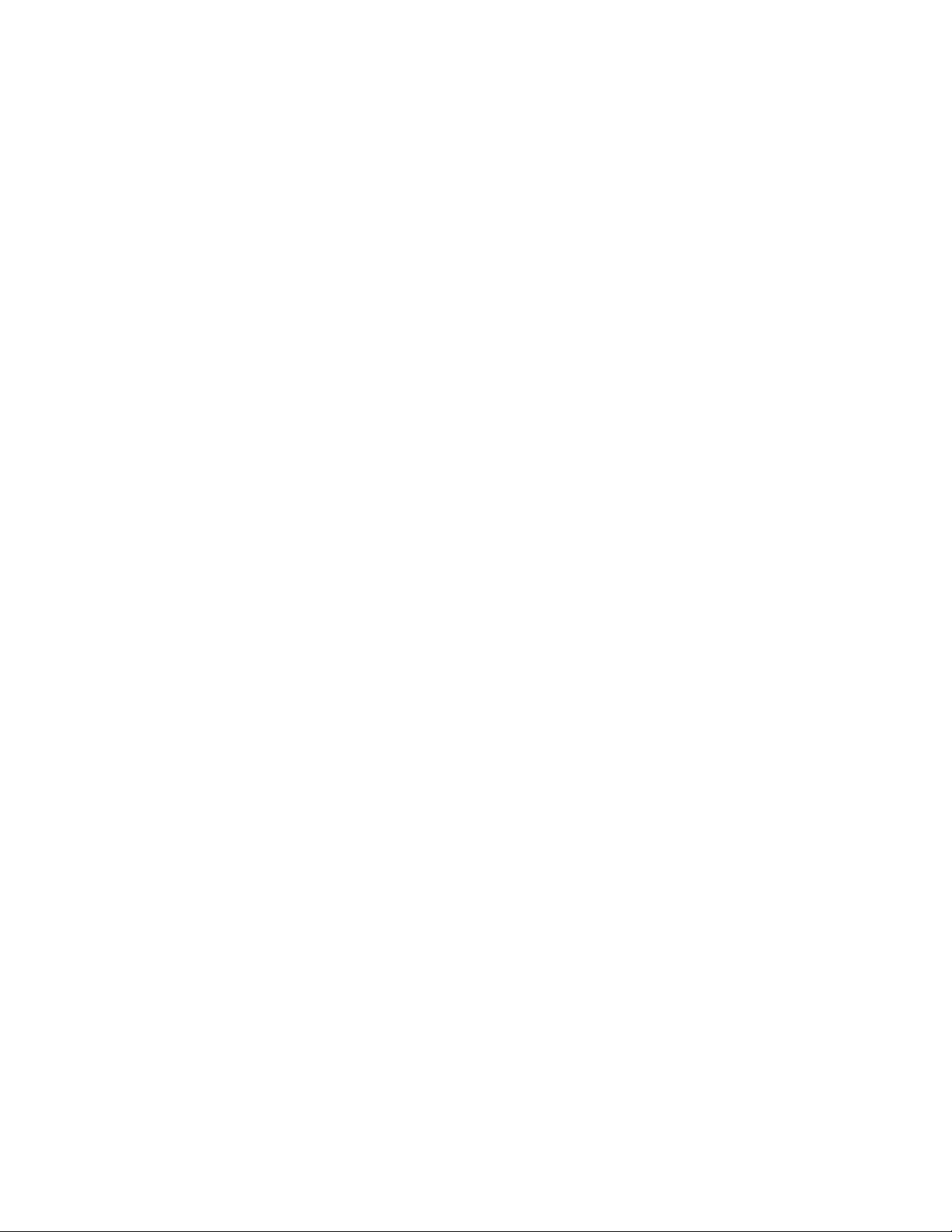
2
Preface........................................................................................................................... 4
Audience.................................................................................................................... 4
About This Book ....................................................................................................... 4
C# Online ................................................................................................................... 4
Conventions Used in This Book............................................................................. 5
How to Contact Us ................................................................................................... 7
Acknowledgments .................................................................................................... 7
Chapter 1. Introduction................................................................................................ 9
1.1 C# Language...................................................................................................... 9
1.2 Common Language Runtime......................................................................... 10
1.3 Framework Class Library............................................................................... 11
1.4 A First C# Program.......................................................................................... 11
Chapter 2. C# Language Reference ....................................................................... 13
2.1 Identifiers........................................................................................................... 13
2.2 Types ................................................................................................................. 13
2.3 Variables ........................................................................................................... 23
2.4 Expressions and Operators ........................................................................... 24
2.5 Statements........................................................................................................ 26
2.6 Organizing Types............................................................................................. 33
2.7 Inheritance ........................................................................................................ 35
2.8 Access Modifiers.............................................................................................. 39
2.9 Classes and Structs ........................................................................................ 41
2.10 Interfaces ........................................................................................................ 56
2.11 Arrays .............................................................................................................. 59
2.12 Enums ............................................................................................................. 61
2.13 Delegates........................................................................................................ 62
2.14 Events ............................................................................................................. 65
2.15 try Statements and Exceptions ................................................................... 67
2.16 Attributes......................................................................................................... 71
2.17 Unsafe Code and Pointers........................................................................... 73
2.18 Preprocessor Directives ............................................................................... 75
2.19 XML Documentation..................................................................................... 76
Chapter 3. Programming the.NET Framework...................................................... 82
3.1 Common Types................................................................................................ 82
3.2 Math................................................................................................................... 87
3.3 Strings ............................................................................................................... 88
3.4 Collections ........................................................................................................ 91
3.5 Regular Expressions ....................................................................................... 97
3.6 Input/Output...................................................................................................... 99
3.7 Networking ...................................................................................................... 102
3.8 Threading ........................................................................................................ 106
3.9 Assemblies ..................................................................................................... 109
3.10 Reflection...................................................................................................... 112
3.11 Custom Attributes........................................................................................ 118
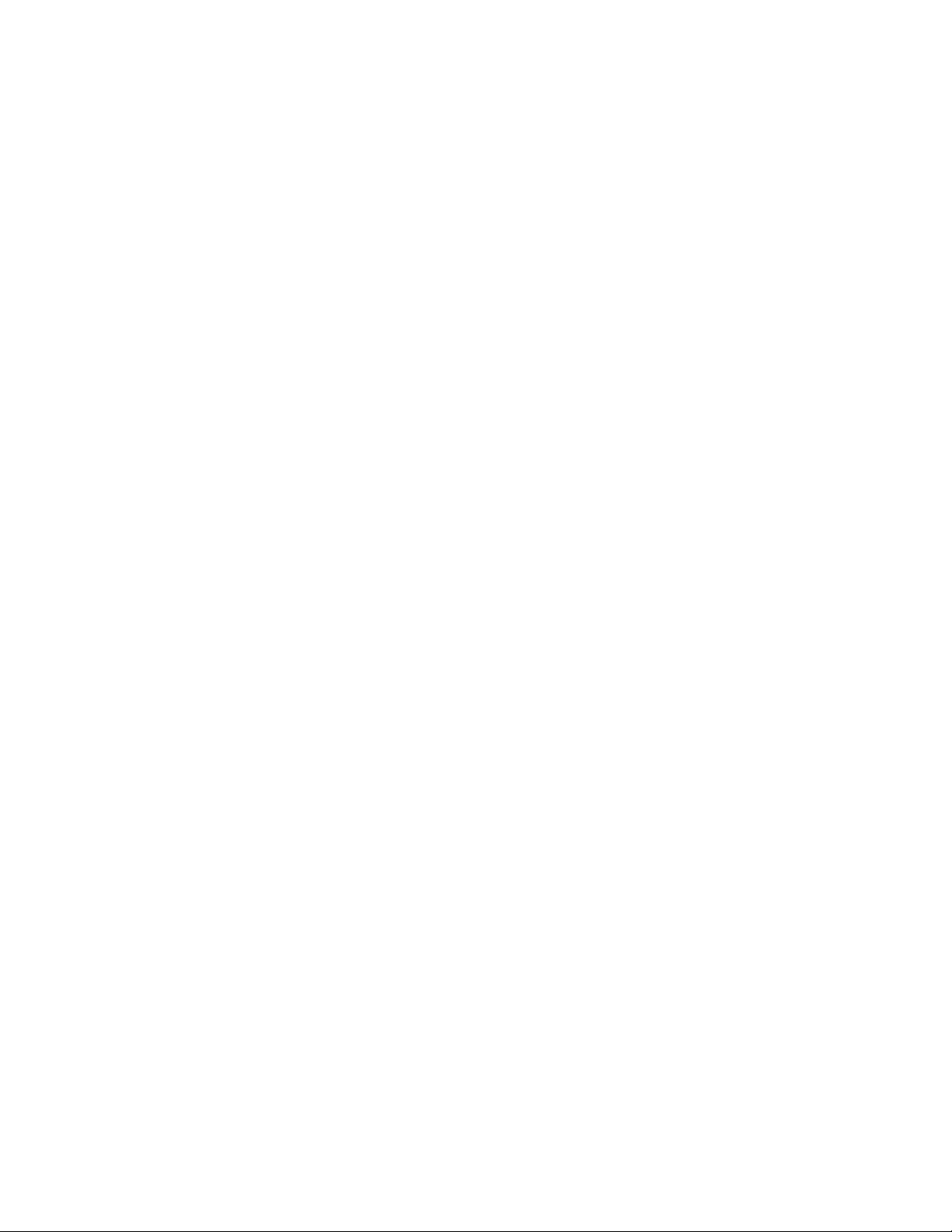
3
3.12 Automatic Memory Management.............................................................. 124
3.13 Interop with Native DLLs............................................................................ 127
3.14 Interop with COM......................................................................................... 133
Chapter 4. Framework Class Library Overview................................................... 137
4.1 Core Types ..................................................................................................... 137
4.2 Text .................................................................................................................. 137
4.3 Collections ...................................................................................................... 138
4.4 Streams and I/O............................................................................................. 138
4.5 Networking ...................................................................................................... 138
4.6 Threading ........................................................................................................ 138
4.7 Security........................................................................................................... 139
4.8 Reflection and Metadata............................................................................... 139
4.9 Assemblies ..................................................................................................... 139
4.10 Serialization.................................................................................................. 140
4.11 Remoting....................................................................................................... 140
4.12 Web Services............................................................................................... 140
4.13 Data Access ................................................................................................. 141
4.14 XML ............................................................................................................... 141
4.15 Graphics........................................................................................................ 141
4.16 Rich Client Applications.............................................................................. 142
4.17 Web-Based Applications ............................................................................ 142
4.18 Globalization................................................................................................. 142
4.19 Configuration................................................................................................ 143
4.20 Advanced Component Services................................................................ 143
4.21 Diagnostics and Debugging ....................................................................... 143
4.22 Interoperating with Unmanaged Code ..................................................... 144
4.23 Compiler and Tool Support........................................................................ 144
4.24 Runtime Facilities........................................................................................ 144
4.25 Native OS Facilities..................................................................................... 144
4.26 Undocumented Types................................................................................. 145
Chapter 5. Essential .NET Tools ........................................................................... 147
Appendix A. C# Keywords ...................................................................................... 149
Appendix B. Regular Expressions ......................................................................... 153
Appendix C. Format Specifiers .............................................................................. 156
C.1 Picture Format Specifiers ............................................................................ 157
C.2 DateTime Format Specifiers ....................................................................... 159
Appendix D. Data Marshaling................................................................................. 161
Appendix E. Working with Assemblies ................................................................. 162
E.1 Building Shareable Assemblies .................................................................. 162
E.2 Managing the Global Assembly Cache ..................................................... 163
E.3 Using nmake .................................................................................................. 163
Appendix F. Namespaces and Assemblies ......................................................... 165
Colophon................................................................................................................... 169
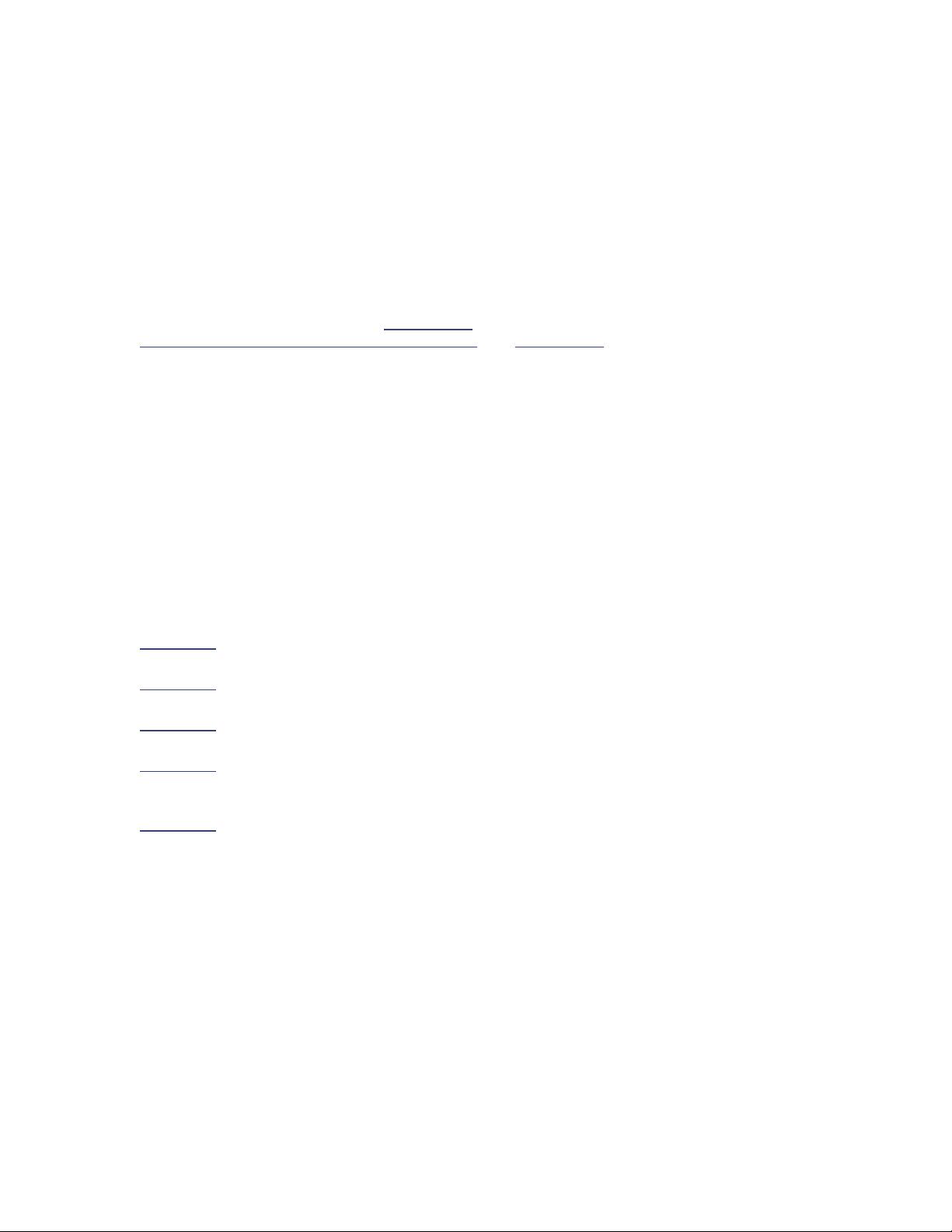
4
Preface
C# Essentials is a highly condensed introduction to the C# language and the .NET Framework.
C# and the .NET initiative were both unveiled in July 2000 at the Microsoft Professional
Developers Conference in Orlando, Florida, and shortly thereafter, the .NET Software
Development Kit (SDK) was released on the Internet.
The information in this book is based on Release Candidate 1 (RC1) of the .NET SDK released
by Microsoft in October 2001. We expect that version to be largely compatible with the final
release, but Microsoft may make minor changes that affect this book. To stay current, be sure to
check the online resources listed in Section P.3 as well as the O'Reilly web page for this book,
http://www.oreilly.com/catalog/csharpess2 (see Section P.5).
Audience
While we have tried to make this book useful to anyone interested in learning about C#, our
primary audience is developers already familiar with an object-oriented language such as C++,
Smalltalk, Java, or Delphi. C# facilitates writing web applications and services, as well as
traditional standalone and client/server-based applications. Experience in any of these areas will
make the advantages of C# and the .NET Framework more immediately apparent but isn't
required.
About This Book
This book is divided into five chapters and six appendixes:
Chapter 1 orients you to C# and the .NET Framework.
Chapter 2 introduces the C# language and serves as a language reference.
Chapter 3 explains how to use C# and the .NET Framework.
Chapter 4 provides an overview of the key libraries in .NET—organized by function—and
documents the most essential namespaces and types of each.
Chapter 5 is an overview of essential .NET tools that ship with the .NET Framework SDK,
including the C# compiler and utilities for importing COM objects and exporting .NET objects.
The six appendixes provide additional information of interest to working programmers, including
an alphabetical C# keyword reference, codes for regular expressions and string formats, and a
cross reference of assembly and namespace mappings
This book assumes that you have access to the .NET Framework SDK. For additional details on
language features and class libraries covered here, we recommend the Microsoft online .NET
documentation.
C# Online
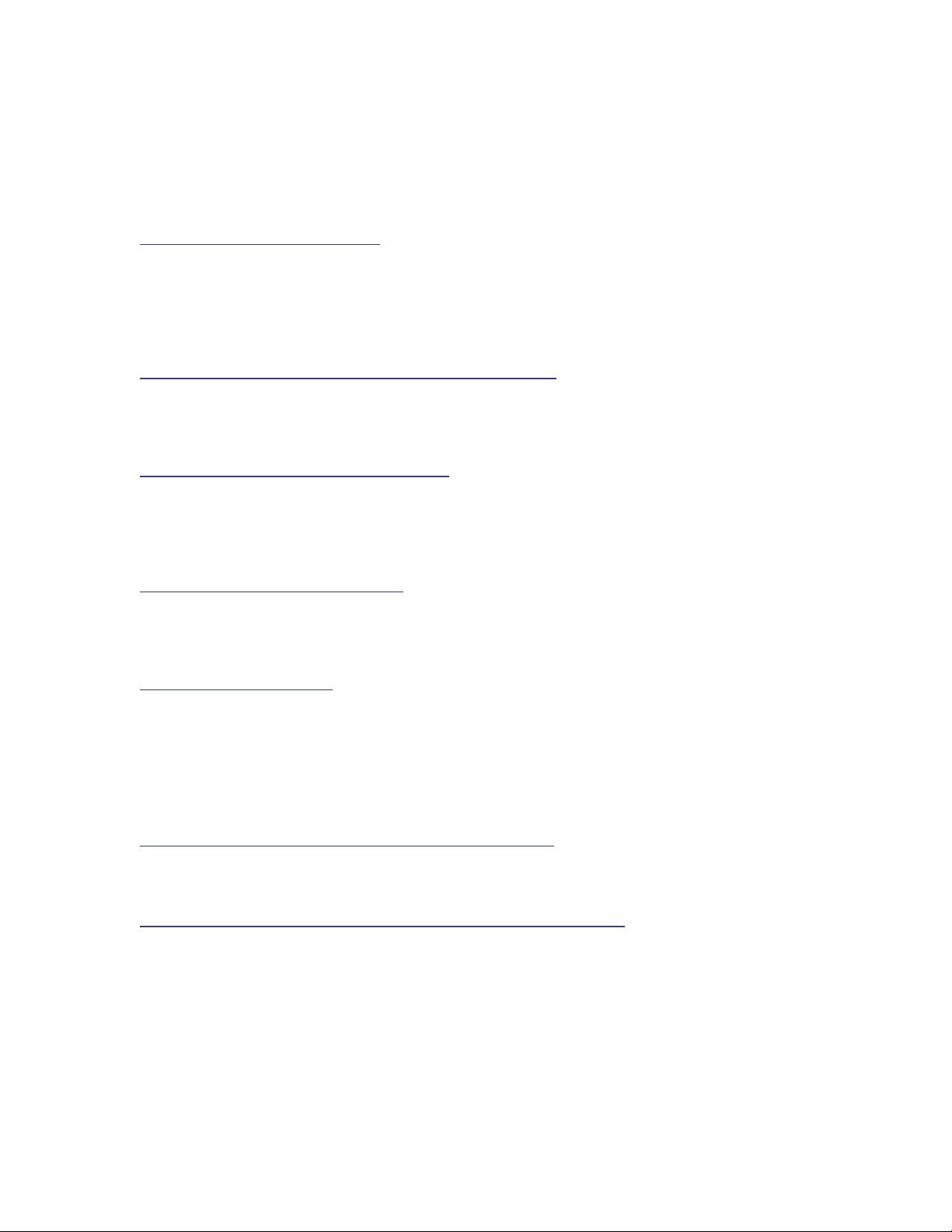
5
Since this book is a condensed introduction to C#, it cannot answer every question you might
have about the language. There are many online resources that can help you get the most out of
C#.
We recommend the following sites:
http://msdn.microsoft.com/net
The Microsoft .NET Developer Center is the official site for all things .NET, including the
latest version of the .NET Framework SDK, which includes the C# compiler, as well as
documentation, technical articles, sample code, pointers to discussion groups, and third-
party resources.
http://msdn.microsoft.com/net/thirdparty/default.asp
A complete list of third-party resources of interest to C# and .NET Framework
developers.
http://discuss.develop.com/dotnet.html
The DevelopMentor DOTNET discussion list. Possibly the best site for freewheeling
independent discussion of the .NET languages and framework; participants often include
key Microsoft engineers.
http://www.oreillynet.com/dotnet
The O'Reilly Network .NET DevCenter, which features original articles, news, and
weblogs of interest to .NET programmers.
http://dotnet.oreilly.com
The O'Reilly .NET Center. Visit this page frequently for information on current and
upcoming .NET books from O'Reilly. You'll find sample chapters, articles, and other
resources.
Two articles of interest include:
http://windows.oreilly.com/news/hejlsberg_0800.html
An interview with chief C# architect Anders Hejlsberg, by O'Reilly editor John Osborn.
http://www.genamics.com/developer/csharp_comparative.htm
A comparison of C# to C++ and Java, by coauthor Ben Albahari.
You can find Usenet discussions about .NET in the microsoft.public.dotnet.* family of
newsgroups. In addition, the newsgroup microsoft.public.dotnet.languages.csharp specifically
addresses C#. If your news server does not carry these groups, you can find them at
news://msnews.microsoft.com.
Conventions Used in This Book